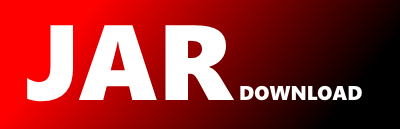
software.amazon.awssdk.services.redshift.model.RestoreStatus Maven / Gradle / Ivy
Show all versions of redshift Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.redshift.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Describes the status of a cluster restore action. Returns null if the cluster was not created by restoring a
* snapshot.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class RestoreStatus implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Status")
.getter(getter(RestoreStatus::status)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Status").build()).build();
private static final SdkField CURRENT_RESTORE_RATE_IN_MEGA_BYTES_PER_SECOND_FIELD = SdkField
. builder(MarshallingType.DOUBLE)
.memberName("CurrentRestoreRateInMegaBytesPerSecond")
.getter(getter(RestoreStatus::currentRestoreRateInMegaBytesPerSecond))
.setter(setter(Builder::currentRestoreRateInMegaBytesPerSecond))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("CurrentRestoreRateInMegaBytesPerSecond").build()).build();
private static final SdkField SNAPSHOT_SIZE_IN_MEGA_BYTES_FIELD = SdkField. builder(MarshallingType.LONG)
.memberName("SnapshotSizeInMegaBytes").getter(getter(RestoreStatus::snapshotSizeInMegaBytes))
.setter(setter(Builder::snapshotSizeInMegaBytes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SnapshotSizeInMegaBytes").build())
.build();
private static final SdkField PROGRESS_IN_MEGA_BYTES_FIELD = SdkField. builder(MarshallingType.LONG)
.memberName("ProgressInMegaBytes").getter(getter(RestoreStatus::progressInMegaBytes))
.setter(setter(Builder::progressInMegaBytes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ProgressInMegaBytes").build())
.build();
private static final SdkField ELAPSED_TIME_IN_SECONDS_FIELD = SdkField. builder(MarshallingType.LONG)
.memberName("ElapsedTimeInSeconds").getter(getter(RestoreStatus::elapsedTimeInSeconds))
.setter(setter(Builder::elapsedTimeInSeconds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ElapsedTimeInSeconds").build())
.build();
private static final SdkField ESTIMATED_TIME_TO_COMPLETION_IN_SECONDS_FIELD = SdkField
. builder(MarshallingType.LONG)
.memberName("EstimatedTimeToCompletionInSeconds")
.getter(getter(RestoreStatus::estimatedTimeToCompletionInSeconds))
.setter(setter(Builder::estimatedTimeToCompletionInSeconds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EstimatedTimeToCompletionInSeconds")
.build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(STATUS_FIELD,
CURRENT_RESTORE_RATE_IN_MEGA_BYTES_PER_SECOND_FIELD, SNAPSHOT_SIZE_IN_MEGA_BYTES_FIELD, PROGRESS_IN_MEGA_BYTES_FIELD,
ELAPSED_TIME_IN_SECONDS_FIELD, ESTIMATED_TIME_TO_COMPLETION_IN_SECONDS_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("Status", STATUS_FIELD);
put("CurrentRestoreRateInMegaBytesPerSecond", CURRENT_RESTORE_RATE_IN_MEGA_BYTES_PER_SECOND_FIELD);
put("SnapshotSizeInMegaBytes", SNAPSHOT_SIZE_IN_MEGA_BYTES_FIELD);
put("ProgressInMegaBytes", PROGRESS_IN_MEGA_BYTES_FIELD);
put("ElapsedTimeInSeconds", ELAPSED_TIME_IN_SECONDS_FIELD);
put("EstimatedTimeToCompletionInSeconds", ESTIMATED_TIME_TO_COMPLETION_IN_SECONDS_FIELD);
}
});
private static final long serialVersionUID = 1L;
private final String status;
private final Double currentRestoreRateInMegaBytesPerSecond;
private final Long snapshotSizeInMegaBytes;
private final Long progressInMegaBytes;
private final Long elapsedTimeInSeconds;
private final Long estimatedTimeToCompletionInSeconds;
private RestoreStatus(BuilderImpl builder) {
this.status = builder.status;
this.currentRestoreRateInMegaBytesPerSecond = builder.currentRestoreRateInMegaBytesPerSecond;
this.snapshotSizeInMegaBytes = builder.snapshotSizeInMegaBytes;
this.progressInMegaBytes = builder.progressInMegaBytes;
this.elapsedTimeInSeconds = builder.elapsedTimeInSeconds;
this.estimatedTimeToCompletionInSeconds = builder.estimatedTimeToCompletionInSeconds;
}
/**
*
* The status of the restore action. Returns starting, restoring, completed, or failed.
*
*
* @return The status of the restore action. Returns starting, restoring, completed, or failed.
*/
public final String status() {
return status;
}
/**
*
* The number of megabytes per second being transferred from the backup storage. Returns the average rate for a
* completed backup. This field is only updated when you restore to DC2 node types.
*
*
* @return The number of megabytes per second being transferred from the backup storage. Returns the average rate
* for a completed backup. This field is only updated when you restore to DC2 node types.
*/
public final Double currentRestoreRateInMegaBytesPerSecond() {
return currentRestoreRateInMegaBytesPerSecond;
}
/**
*
* The size of the set of snapshot data used to restore the cluster. This field is only updated when you restore to
* DC2 node types.
*
*
* @return The size of the set of snapshot data used to restore the cluster. This field is only updated when you
* restore to DC2 node types.
*/
public final Long snapshotSizeInMegaBytes() {
return snapshotSizeInMegaBytes;
}
/**
*
* The number of megabytes that have been transferred from snapshot storage. This field is only updated when you
* restore to DC2 node types.
*
*
* @return The number of megabytes that have been transferred from snapshot storage. This field is only updated when
* you restore to DC2 node types.
*/
public final Long progressInMegaBytes() {
return progressInMegaBytes;
}
/**
*
* The amount of time an in-progress restore has been running, or the amount of time it took a completed restore to
* finish. This field is only updated when you restore to DC2 node types.
*
*
* @return The amount of time an in-progress restore has been running, or the amount of time it took a completed
* restore to finish. This field is only updated when you restore to DC2 node types.
*/
public final Long elapsedTimeInSeconds() {
return elapsedTimeInSeconds;
}
/**
*
* The estimate of the time remaining before the restore will complete. Returns 0 for a completed restore. This
* field is only updated when you restore to DC2 node types.
*
*
* @return The estimate of the time remaining before the restore will complete. Returns 0 for a completed restore.
* This field is only updated when you restore to DC2 node types.
*/
public final Long estimatedTimeToCompletionInSeconds() {
return estimatedTimeToCompletionInSeconds;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(status());
hashCode = 31 * hashCode + Objects.hashCode(currentRestoreRateInMegaBytesPerSecond());
hashCode = 31 * hashCode + Objects.hashCode(snapshotSizeInMegaBytes());
hashCode = 31 * hashCode + Objects.hashCode(progressInMegaBytes());
hashCode = 31 * hashCode + Objects.hashCode(elapsedTimeInSeconds());
hashCode = 31 * hashCode + Objects.hashCode(estimatedTimeToCompletionInSeconds());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof RestoreStatus)) {
return false;
}
RestoreStatus other = (RestoreStatus) obj;
return Objects.equals(status(), other.status())
&& Objects.equals(currentRestoreRateInMegaBytesPerSecond(), other.currentRestoreRateInMegaBytesPerSecond())
&& Objects.equals(snapshotSizeInMegaBytes(), other.snapshotSizeInMegaBytes())
&& Objects.equals(progressInMegaBytes(), other.progressInMegaBytes())
&& Objects.equals(elapsedTimeInSeconds(), other.elapsedTimeInSeconds())
&& Objects.equals(estimatedTimeToCompletionInSeconds(), other.estimatedTimeToCompletionInSeconds());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("RestoreStatus").add("Status", status())
.add("CurrentRestoreRateInMegaBytesPerSecond", currentRestoreRateInMegaBytesPerSecond())
.add("SnapshotSizeInMegaBytes", snapshotSizeInMegaBytes()).add("ProgressInMegaBytes", progressInMegaBytes())
.add("ElapsedTimeInSeconds", elapsedTimeInSeconds())
.add("EstimatedTimeToCompletionInSeconds", estimatedTimeToCompletionInSeconds()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Status":
return Optional.ofNullable(clazz.cast(status()));
case "CurrentRestoreRateInMegaBytesPerSecond":
return Optional.ofNullable(clazz.cast(currentRestoreRateInMegaBytesPerSecond()));
case "SnapshotSizeInMegaBytes":
return Optional.ofNullable(clazz.cast(snapshotSizeInMegaBytes()));
case "ProgressInMegaBytes":
return Optional.ofNullable(clazz.cast(progressInMegaBytes()));
case "ElapsedTimeInSeconds":
return Optional.ofNullable(clazz.cast(elapsedTimeInSeconds()));
case "EstimatedTimeToCompletionInSeconds":
return Optional.ofNullable(clazz.cast(estimatedTimeToCompletionInSeconds()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function