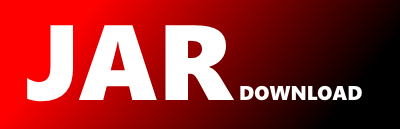
software.amazon.awssdk.services.redshiftdata.model.ColumnMetadata Maven / Gradle / Ivy
Show all versions of redshiftdata Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.redshiftdata.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The properties (metadata) of a column.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ColumnMetadata implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField COLUMN_DEFAULT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("columnDefault").getter(getter(ColumnMetadata::columnDefault)).setter(setter(Builder::columnDefault))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("columnDefault").build()).build();
private static final SdkField IS_CASE_SENSITIVE_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("isCaseSensitive").getter(getter(ColumnMetadata::isCaseSensitive))
.setter(setter(Builder::isCaseSensitive))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("isCaseSensitive").build()).build();
private static final SdkField IS_CURRENCY_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("isCurrency").getter(getter(ColumnMetadata::isCurrency)).setter(setter(Builder::isCurrency))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("isCurrency").build()).build();
private static final SdkField IS_SIGNED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("isSigned").getter(getter(ColumnMetadata::isSigned)).setter(setter(Builder::isSigned))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("isSigned").build()).build();
private static final SdkField LABEL_FIELD = SdkField. builder(MarshallingType.STRING).memberName("label")
.getter(getter(ColumnMetadata::label)).setter(setter(Builder::label))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("label").build()).build();
private static final SdkField LENGTH_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("length").getter(getter(ColumnMetadata::length)).setter(setter(Builder::length))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("length").build()).build();
private static final SdkField NAME_FIELD = SdkField. builder(MarshallingType.STRING).memberName("name")
.getter(getter(ColumnMetadata::name)).setter(setter(Builder::name))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("name").build()).build();
private static final SdkField NULLABLE_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("nullable").getter(getter(ColumnMetadata::nullable)).setter(setter(Builder::nullable))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("nullable").build()).build();
private static final SdkField PRECISION_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("precision").getter(getter(ColumnMetadata::precision)).setter(setter(Builder::precision))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("precision").build()).build();
private static final SdkField SCALE_FIELD = SdkField. builder(MarshallingType.INTEGER).memberName("scale")
.getter(getter(ColumnMetadata::scale)).setter(setter(Builder::scale))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("scale").build()).build();
private static final SdkField SCHEMA_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("schemaName").getter(getter(ColumnMetadata::schemaName)).setter(setter(Builder::schemaName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("schemaName").build()).build();
private static final SdkField TABLE_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("tableName").getter(getter(ColumnMetadata::tableName)).setter(setter(Builder::tableName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("tableName").build()).build();
private static final SdkField TYPE_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("typeName").getter(getter(ColumnMetadata::typeName)).setter(setter(Builder::typeName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("typeName").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(COLUMN_DEFAULT_FIELD,
IS_CASE_SENSITIVE_FIELD, IS_CURRENCY_FIELD, IS_SIGNED_FIELD, LABEL_FIELD, LENGTH_FIELD, NAME_FIELD, NULLABLE_FIELD,
PRECISION_FIELD, SCALE_FIELD, SCHEMA_NAME_FIELD, TABLE_NAME_FIELD, TYPE_NAME_FIELD));
private static final long serialVersionUID = 1L;
private final String columnDefault;
private final Boolean isCaseSensitive;
private final Boolean isCurrency;
private final Boolean isSigned;
private final String label;
private final Integer length;
private final String name;
private final Integer nullable;
private final Integer precision;
private final Integer scale;
private final String schemaName;
private final String tableName;
private final String typeName;
private ColumnMetadata(BuilderImpl builder) {
this.columnDefault = builder.columnDefault;
this.isCaseSensitive = builder.isCaseSensitive;
this.isCurrency = builder.isCurrency;
this.isSigned = builder.isSigned;
this.label = builder.label;
this.length = builder.length;
this.name = builder.name;
this.nullable = builder.nullable;
this.precision = builder.precision;
this.scale = builder.scale;
this.schemaName = builder.schemaName;
this.tableName = builder.tableName;
this.typeName = builder.typeName;
}
/**
*
* The default value of the column.
*
*
* @return The default value of the column.
*/
public final String columnDefault() {
return columnDefault;
}
/**
*
* A value that indicates whether the column is case-sensitive.
*
*
* @return A value that indicates whether the column is case-sensitive.
*/
public final Boolean isCaseSensitive() {
return isCaseSensitive;
}
/**
*
* A value that indicates whether the column contains currency values.
*
*
* @return A value that indicates whether the column contains currency values.
*/
public final Boolean isCurrency() {
return isCurrency;
}
/**
*
* A value that indicates whether an integer column is signed.
*
*
* @return A value that indicates whether an integer column is signed.
*/
public final Boolean isSigned() {
return isSigned;
}
/**
*
* The label for the column.
*
*
* @return The label for the column.
*/
public final String label() {
return label;
}
/**
*
* The length of the column.
*
*
* @return The length of the column.
*/
public final Integer length() {
return length;
}
/**
*
* The name of the column.
*
*
* @return The name of the column.
*/
public final String name() {
return name;
}
/**
*
* A value that indicates whether the column is nullable.
*
*
* @return A value that indicates whether the column is nullable.
*/
public final Integer nullable() {
return nullable;
}
/**
*
* The precision value of a decimal number column.
*
*
* @return The precision value of a decimal number column.
*/
public final Integer precision() {
return precision;
}
/**
*
* The scale value of a decimal number column.
*
*
* @return The scale value of a decimal number column.
*/
public final Integer scale() {
return scale;
}
/**
*
* The name of the schema that contains the table that includes the column.
*
*
* @return The name of the schema that contains the table that includes the column.
*/
public final String schemaName() {
return schemaName;
}
/**
*
* The name of the table that includes the column.
*
*
* @return The name of the table that includes the column.
*/
public final String tableName() {
return tableName;
}
/**
*
* The database-specific data type of the column.
*
*
* @return The database-specific data type of the column.
*/
public final String typeName() {
return typeName;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(columnDefault());
hashCode = 31 * hashCode + Objects.hashCode(isCaseSensitive());
hashCode = 31 * hashCode + Objects.hashCode(isCurrency());
hashCode = 31 * hashCode + Objects.hashCode(isSigned());
hashCode = 31 * hashCode + Objects.hashCode(label());
hashCode = 31 * hashCode + Objects.hashCode(length());
hashCode = 31 * hashCode + Objects.hashCode(name());
hashCode = 31 * hashCode + Objects.hashCode(nullable());
hashCode = 31 * hashCode + Objects.hashCode(precision());
hashCode = 31 * hashCode + Objects.hashCode(scale());
hashCode = 31 * hashCode + Objects.hashCode(schemaName());
hashCode = 31 * hashCode + Objects.hashCode(tableName());
hashCode = 31 * hashCode + Objects.hashCode(typeName());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ColumnMetadata)) {
return false;
}
ColumnMetadata other = (ColumnMetadata) obj;
return Objects.equals(columnDefault(), other.columnDefault())
&& Objects.equals(isCaseSensitive(), other.isCaseSensitive()) && Objects.equals(isCurrency(), other.isCurrency())
&& Objects.equals(isSigned(), other.isSigned()) && Objects.equals(label(), other.label())
&& Objects.equals(length(), other.length()) && Objects.equals(name(), other.name())
&& Objects.equals(nullable(), other.nullable()) && Objects.equals(precision(), other.precision())
&& Objects.equals(scale(), other.scale()) && Objects.equals(schemaName(), other.schemaName())
&& Objects.equals(tableName(), other.tableName()) && Objects.equals(typeName(), other.typeName());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ColumnMetadata").add("ColumnDefault", columnDefault()).add("IsCaseSensitive", isCaseSensitive())
.add("IsCurrency", isCurrency()).add("IsSigned", isSigned()).add("Label", label()).add("Length", length())
.add("Name", name()).add("Nullable", nullable()).add("Precision", precision()).add("Scale", scale())
.add("SchemaName", schemaName()).add("TableName", tableName()).add("TypeName", typeName()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "columnDefault":
return Optional.ofNullable(clazz.cast(columnDefault()));
case "isCaseSensitive":
return Optional.ofNullable(clazz.cast(isCaseSensitive()));
case "isCurrency":
return Optional.ofNullable(clazz.cast(isCurrency()));
case "isSigned":
return Optional.ofNullable(clazz.cast(isSigned()));
case "label":
return Optional.ofNullable(clazz.cast(label()));
case "length":
return Optional.ofNullable(clazz.cast(length()));
case "name":
return Optional.ofNullable(clazz.cast(name()));
case "nullable":
return Optional.ofNullable(clazz.cast(nullable()));
case "precision":
return Optional.ofNullable(clazz.cast(precision()));
case "scale":
return Optional.ofNullable(clazz.cast(scale()));
case "schemaName":
return Optional.ofNullable(clazz.cast(schemaName()));
case "tableName":
return Optional.ofNullable(clazz.cast(tableName()));
case "typeName":
return Optional.ofNullable(clazz.cast(typeName()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function