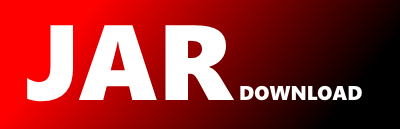
software.amazon.awssdk.services.redshiftdata.model.SubStatementData Maven / Gradle / Ivy
Show all versions of redshiftdata Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.redshiftdata.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Information about an SQL statement.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class SubStatementData implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField CREATED_AT_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("CreatedAt").getter(getter(SubStatementData::createdAt)).setter(setter(Builder::createdAt))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CreatedAt").build()).build();
private static final SdkField DURATION_FIELD = SdkField. builder(MarshallingType.LONG).memberName("Duration")
.getter(getter(SubStatementData::duration)).setter(setter(Builder::duration))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Duration").build()).build();
private static final SdkField ERROR_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Error")
.getter(getter(SubStatementData::error)).setter(setter(Builder::error))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Error").build()).build();
private static final SdkField HAS_RESULT_SET_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("HasResultSet").getter(getter(SubStatementData::hasResultSet)).setter(setter(Builder::hasResultSet))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HasResultSet").build()).build();
private static final SdkField ID_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Id")
.getter(getter(SubStatementData::id)).setter(setter(Builder::id))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Id").build()).build();
private static final SdkField QUERY_STRING_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("QueryString").getter(getter(SubStatementData::queryString)).setter(setter(Builder::queryString))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("QueryString").build()).build();
private static final SdkField REDSHIFT_QUERY_ID_FIELD = SdkField. builder(MarshallingType.LONG)
.memberName("RedshiftQueryId").getter(getter(SubStatementData::redshiftQueryId))
.setter(setter(Builder::redshiftQueryId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RedshiftQueryId").build()).build();
private static final SdkField RESULT_ROWS_FIELD = SdkField. builder(MarshallingType.LONG)
.memberName("ResultRows").getter(getter(SubStatementData::resultRows)).setter(setter(Builder::resultRows))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ResultRows").build()).build();
private static final SdkField RESULT_SIZE_FIELD = SdkField. builder(MarshallingType.LONG)
.memberName("ResultSize").getter(getter(SubStatementData::resultSize)).setter(setter(Builder::resultSize))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ResultSize").build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Status")
.getter(getter(SubStatementData::statusAsString)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Status").build()).build();
private static final SdkField UPDATED_AT_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("UpdatedAt").getter(getter(SubStatementData::updatedAt)).setter(setter(Builder::updatedAt))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("UpdatedAt").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(CREATED_AT_FIELD,
DURATION_FIELD, ERROR_FIELD, HAS_RESULT_SET_FIELD, ID_FIELD, QUERY_STRING_FIELD, REDSHIFT_QUERY_ID_FIELD,
RESULT_ROWS_FIELD, RESULT_SIZE_FIELD, STATUS_FIELD, UPDATED_AT_FIELD));
private static final long serialVersionUID = 1L;
private final Instant createdAt;
private final Long duration;
private final String error;
private final Boolean hasResultSet;
private final String id;
private final String queryString;
private final Long redshiftQueryId;
private final Long resultRows;
private final Long resultSize;
private final String status;
private final Instant updatedAt;
private SubStatementData(BuilderImpl builder) {
this.createdAt = builder.createdAt;
this.duration = builder.duration;
this.error = builder.error;
this.hasResultSet = builder.hasResultSet;
this.id = builder.id;
this.queryString = builder.queryString;
this.redshiftQueryId = builder.redshiftQueryId;
this.resultRows = builder.resultRows;
this.resultSize = builder.resultSize;
this.status = builder.status;
this.updatedAt = builder.updatedAt;
}
/**
*
* The date and time (UTC) the statement was created.
*
*
* @return The date and time (UTC) the statement was created.
*/
public final Instant createdAt() {
return createdAt;
}
/**
*
* The amount of time in nanoseconds that the statement ran.
*
*
* @return The amount of time in nanoseconds that the statement ran.
*/
public final Long duration() {
return duration;
}
/**
*
* The error message from the cluster if the SQL statement encountered an error while running.
*
*
* @return The error message from the cluster if the SQL statement encountered an error while running.
*/
public final String error() {
return error;
}
/**
*
* A value that indicates whether the statement has a result set. The result set can be empty. The value is true for
* an empty result set.
*
*
* @return A value that indicates whether the statement has a result set. The result set can be empty. The value is
* true for an empty result set.
*/
public final Boolean hasResultSet() {
return hasResultSet;
}
/**
*
* The identifier of the SQL statement. This value is a universally unique identifier (UUID) generated by Amazon
* Redshift Data API. A suffix indicates the number of the SQL statement. For example,
* d9b6c0c9-0747-4bf4-b142-e8883122f766:2
has a suffix of :2
that indicates the second SQL
* statement of a batch query.
*
*
* @return The identifier of the SQL statement. This value is a universally unique identifier (UUID) generated by
* Amazon Redshift Data API. A suffix indicates the number of the SQL statement. For example,
* d9b6c0c9-0747-4bf4-b142-e8883122f766:2
has a suffix of :2
that indicates the
* second SQL statement of a batch query.
*/
public final String id() {
return id;
}
/**
*
* The SQL statement text.
*
*
* @return The SQL statement text.
*/
public final String queryString() {
return queryString;
}
/**
*
* The SQL statement identifier. This value is a universally unique identifier (UUID) generated by Amazon Redshift
* Data API.
*
*
* @return The SQL statement identifier. This value is a universally unique identifier (UUID) generated by Amazon
* Redshift Data API.
*/
public final Long redshiftQueryId() {
return redshiftQueryId;
}
/**
*
* Either the number of rows returned from the SQL statement or the number of rows affected. If result size is
* greater than zero, the result rows can be the number of rows affected by SQL statements such as INSERT, UPDATE,
* DELETE, COPY, and others. A -1
indicates the value is null.
*
*
* @return Either the number of rows returned from the SQL statement or the number of rows affected. If result size
* is greater than zero, the result rows can be the number of rows affected by SQL statements such as
* INSERT, UPDATE, DELETE, COPY, and others. A -1
indicates the value is null.
*/
public final Long resultRows() {
return resultRows;
}
/**
*
* The size in bytes of the returned results. A -1
indicates the value is null.
*
*
* @return The size in bytes of the returned results. A -1
indicates the value is null.
*/
public final Long resultSize() {
return resultSize;
}
/**
*
* The status of the SQL statement. An example is the that the SQL statement finished.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link StatementStatusString#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #statusAsString}.
*
*
* @return The status of the SQL statement. An example is the that the SQL statement finished.
* @see StatementStatusString
*/
public final StatementStatusString status() {
return StatementStatusString.fromValue(status);
}
/**
*
* The status of the SQL statement. An example is the that the SQL statement finished.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link StatementStatusString#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #statusAsString}.
*
*
* @return The status of the SQL statement. An example is the that the SQL statement finished.
* @see StatementStatusString
*/
public final String statusAsString() {
return status;
}
/**
*
* The date and time (UTC) that the statement metadata was last updated.
*
*
* @return The date and time (UTC) that the statement metadata was last updated.
*/
public final Instant updatedAt() {
return updatedAt;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(createdAt());
hashCode = 31 * hashCode + Objects.hashCode(duration());
hashCode = 31 * hashCode + Objects.hashCode(error());
hashCode = 31 * hashCode + Objects.hashCode(hasResultSet());
hashCode = 31 * hashCode + Objects.hashCode(id());
hashCode = 31 * hashCode + Objects.hashCode(queryString());
hashCode = 31 * hashCode + Objects.hashCode(redshiftQueryId());
hashCode = 31 * hashCode + Objects.hashCode(resultRows());
hashCode = 31 * hashCode + Objects.hashCode(resultSize());
hashCode = 31 * hashCode + Objects.hashCode(statusAsString());
hashCode = 31 * hashCode + Objects.hashCode(updatedAt());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof SubStatementData)) {
return false;
}
SubStatementData other = (SubStatementData) obj;
return Objects.equals(createdAt(), other.createdAt()) && Objects.equals(duration(), other.duration())
&& Objects.equals(error(), other.error()) && Objects.equals(hasResultSet(), other.hasResultSet())
&& Objects.equals(id(), other.id()) && Objects.equals(queryString(), other.queryString())
&& Objects.equals(redshiftQueryId(), other.redshiftQueryId()) && Objects.equals(resultRows(), other.resultRows())
&& Objects.equals(resultSize(), other.resultSize()) && Objects.equals(statusAsString(), other.statusAsString())
&& Objects.equals(updatedAt(), other.updatedAt());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("SubStatementData").add("CreatedAt", createdAt()).add("Duration", duration())
.add("Error", error()).add("HasResultSet", hasResultSet()).add("Id", id()).add("QueryString", queryString())
.add("RedshiftQueryId", redshiftQueryId()).add("ResultRows", resultRows()).add("ResultSize", resultSize())
.add("Status", statusAsString()).add("UpdatedAt", updatedAt()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "CreatedAt":
return Optional.ofNullable(clazz.cast(createdAt()));
case "Duration":
return Optional.ofNullable(clazz.cast(duration()));
case "Error":
return Optional.ofNullable(clazz.cast(error()));
case "HasResultSet":
return Optional.ofNullable(clazz.cast(hasResultSet()));
case "Id":
return Optional.ofNullable(clazz.cast(id()));
case "QueryString":
return Optional.ofNullable(clazz.cast(queryString()));
case "RedshiftQueryId":
return Optional.ofNullable(clazz.cast(redshiftQueryId()));
case "ResultRows":
return Optional.ofNullable(clazz.cast(resultRows()));
case "ResultSize":
return Optional.ofNullable(clazz.cast(resultSize()));
case "Status":
return Optional.ofNullable(clazz.cast(statusAsString()));
case "UpdatedAt":
return Optional.ofNullable(clazz.cast(updatedAt()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function