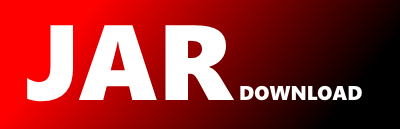
software.amazon.awssdk.services.redshiftdata.RedshiftDataClient Maven / Gradle / Ivy
Show all versions of redshiftdata Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.redshiftdata;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkPublicApi;
import software.amazon.awssdk.annotations.ThreadSafe;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.SdkClient;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.regions.ServiceMetadata;
import software.amazon.awssdk.services.redshiftdata.model.ActiveStatementsExceededException;
import software.amazon.awssdk.services.redshiftdata.model.BatchExecuteStatementException;
import software.amazon.awssdk.services.redshiftdata.model.BatchExecuteStatementRequest;
import software.amazon.awssdk.services.redshiftdata.model.BatchExecuteStatementResponse;
import software.amazon.awssdk.services.redshiftdata.model.CancelStatementRequest;
import software.amazon.awssdk.services.redshiftdata.model.CancelStatementResponse;
import software.amazon.awssdk.services.redshiftdata.model.DatabaseConnectionException;
import software.amazon.awssdk.services.redshiftdata.model.DescribeStatementRequest;
import software.amazon.awssdk.services.redshiftdata.model.DescribeStatementResponse;
import software.amazon.awssdk.services.redshiftdata.model.DescribeTableRequest;
import software.amazon.awssdk.services.redshiftdata.model.DescribeTableResponse;
import software.amazon.awssdk.services.redshiftdata.model.ExecuteStatementException;
import software.amazon.awssdk.services.redshiftdata.model.ExecuteStatementRequest;
import software.amazon.awssdk.services.redshiftdata.model.ExecuteStatementResponse;
import software.amazon.awssdk.services.redshiftdata.model.GetStatementResultRequest;
import software.amazon.awssdk.services.redshiftdata.model.GetStatementResultResponse;
import software.amazon.awssdk.services.redshiftdata.model.InternalServerException;
import software.amazon.awssdk.services.redshiftdata.model.ListDatabasesRequest;
import software.amazon.awssdk.services.redshiftdata.model.ListDatabasesResponse;
import software.amazon.awssdk.services.redshiftdata.model.ListSchemasRequest;
import software.amazon.awssdk.services.redshiftdata.model.ListSchemasResponse;
import software.amazon.awssdk.services.redshiftdata.model.ListStatementsRequest;
import software.amazon.awssdk.services.redshiftdata.model.ListStatementsResponse;
import software.amazon.awssdk.services.redshiftdata.model.ListTablesRequest;
import software.amazon.awssdk.services.redshiftdata.model.ListTablesResponse;
import software.amazon.awssdk.services.redshiftdata.model.RedshiftDataException;
import software.amazon.awssdk.services.redshiftdata.model.ResourceNotFoundException;
import software.amazon.awssdk.services.redshiftdata.model.ValidationException;
import software.amazon.awssdk.services.redshiftdata.paginators.DescribeTableIterable;
import software.amazon.awssdk.services.redshiftdata.paginators.GetStatementResultIterable;
import software.amazon.awssdk.services.redshiftdata.paginators.ListDatabasesIterable;
import software.amazon.awssdk.services.redshiftdata.paginators.ListSchemasIterable;
import software.amazon.awssdk.services.redshiftdata.paginators.ListStatementsIterable;
import software.amazon.awssdk.services.redshiftdata.paginators.ListTablesIterable;
/**
* Service client for accessing Redshift Data API Service. This can be created using the static {@link #builder()}
* method.
*
*
* You can use the Amazon Redshift Data API to run queries on Amazon Redshift tables. You can run SQL statements, which
* are committed if the statement succeeds.
*
*
* For more information about the Amazon Redshift Data API and CLI usage examples, see Using the Amazon Redshift Data API in the
* Amazon Redshift Management Guide.
*
*/
@Generated("software.amazon.awssdk:codegen")
@SdkPublicApi
@ThreadSafe
public interface RedshiftDataClient extends SdkClient {
String SERVICE_NAME = "redshift-data";
/**
* Value for looking up the service's metadata from the
* {@link software.amazon.awssdk.regions.ServiceMetadataProvider}.
*/
String SERVICE_METADATA_ID = "redshift-data";
/**
*
* Runs one or more SQL statements, which can be data manipulation language (DML) or data definition language (DDL).
* Depending on the authorization method, use one of the following combinations of request parameters:
*
*
* -
*
* Secrets Manager - when connecting to a cluster, specify the Amazon Resource Name (ARN) of the secret, the
* database name, and the cluster identifier that matches the cluster in the secret. When connecting to a serverless
* workgroup, specify the Amazon Resource Name (ARN) of the secret and the database name.
*
*
* -
*
* Temporary credentials - when connecting to a cluster, specify the cluster identifier, the database name, and the
* database user name. Also, permission to call the redshift:GetClusterCredentials
operation is
* required. When connecting to a serverless workgroup, specify the workgroup name and database name. Also,
* permission to call the redshift-serverless:GetCredentials
operation is required.
*
*
*
*
* For more information about the Amazon Redshift Data API and CLI usage examples, see Using the Amazon Redshift Data API in
* the Amazon Redshift Management Guide.
*
*
* @param batchExecuteStatementRequest
* @return Result of the BatchExecuteStatement operation returned by the service.
* @throws ValidationException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws ActiveStatementsExceededException
* The number of active statements exceeds the limit.
* @throws BatchExecuteStatementException
* An SQL statement encountered an environmental error while running.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws RedshiftDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample RedshiftDataClient.BatchExecuteStatement
* @see AWS API Documentation
*/
default BatchExecuteStatementResponse batchExecuteStatement(BatchExecuteStatementRequest batchExecuteStatementRequest)
throws ValidationException, ActiveStatementsExceededException, BatchExecuteStatementException, AwsServiceException,
SdkClientException, RedshiftDataException {
throw new UnsupportedOperationException();
}
/**
*
* Runs one or more SQL statements, which can be data manipulation language (DML) or data definition language (DDL).
* Depending on the authorization method, use one of the following combinations of request parameters:
*
*
* -
*
* Secrets Manager - when connecting to a cluster, specify the Amazon Resource Name (ARN) of the secret, the
* database name, and the cluster identifier that matches the cluster in the secret. When connecting to a serverless
* workgroup, specify the Amazon Resource Name (ARN) of the secret and the database name.
*
*
* -
*
* Temporary credentials - when connecting to a cluster, specify the cluster identifier, the database name, and the
* database user name. Also, permission to call the redshift:GetClusterCredentials
operation is
* required. When connecting to a serverless workgroup, specify the workgroup name and database name. Also,
* permission to call the redshift-serverless:GetCredentials
operation is required.
*
*
*
*
* For more information about the Amazon Redshift Data API and CLI usage examples, see Using the Amazon Redshift Data API in
* the Amazon Redshift Management Guide.
*
*
*
* This is a convenience which creates an instance of the {@link BatchExecuteStatementRequest.Builder} avoiding the
* need to create one manually via {@link BatchExecuteStatementRequest#builder()}
*
*
* @param batchExecuteStatementRequest
* A {@link Consumer} that will call methods on {@link BatchExecuteStatementInput.Builder} to create a
* request.
* @return Result of the BatchExecuteStatement operation returned by the service.
* @throws ValidationException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws ActiveStatementsExceededException
* The number of active statements exceeds the limit.
* @throws BatchExecuteStatementException
* An SQL statement encountered an environmental error while running.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws RedshiftDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample RedshiftDataClient.BatchExecuteStatement
* @see AWS API Documentation
*/
default BatchExecuteStatementResponse batchExecuteStatement(
Consumer batchExecuteStatementRequest) throws ValidationException,
ActiveStatementsExceededException, BatchExecuteStatementException, AwsServiceException, SdkClientException,
RedshiftDataException {
return batchExecuteStatement(BatchExecuteStatementRequest.builder().applyMutation(batchExecuteStatementRequest).build());
}
/**
*
* Cancels a running query. To be canceled, a query must be running.
*
*
* For more information about the Amazon Redshift Data API and CLI usage examples, see Using the Amazon Redshift Data API in
* the Amazon Redshift Management Guide.
*
*
* @param cancelStatementRequest
* @return Result of the CancelStatement operation returned by the service.
* @throws ValidationException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws ResourceNotFoundException
* The Amazon Redshift Data API operation failed due to a missing resource.
* @throws InternalServerException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws DatabaseConnectionException
* Connection to a database failed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws RedshiftDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample RedshiftDataClient.CancelStatement
* @see AWS
* API Documentation
*/
default CancelStatementResponse cancelStatement(CancelStatementRequest cancelStatementRequest) throws ValidationException,
ResourceNotFoundException, InternalServerException, DatabaseConnectionException, AwsServiceException,
SdkClientException, RedshiftDataException {
throw new UnsupportedOperationException();
}
/**
*
* Cancels a running query. To be canceled, a query must be running.
*
*
* For more information about the Amazon Redshift Data API and CLI usage examples, see Using the Amazon Redshift Data API in
* the Amazon Redshift Management Guide.
*
*
*
* This is a convenience which creates an instance of the {@link CancelStatementRequest.Builder} avoiding the need
* to create one manually via {@link CancelStatementRequest#builder()}
*
*
* @param cancelStatementRequest
* A {@link Consumer} that will call methods on {@link CancelStatementRequest.Builder} to create a request.
* @return Result of the CancelStatement operation returned by the service.
* @throws ValidationException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws ResourceNotFoundException
* The Amazon Redshift Data API operation failed due to a missing resource.
* @throws InternalServerException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws DatabaseConnectionException
* Connection to a database failed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws RedshiftDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample RedshiftDataClient.CancelStatement
* @see AWS
* API Documentation
*/
default CancelStatementResponse cancelStatement(Consumer cancelStatementRequest)
throws ValidationException, ResourceNotFoundException, InternalServerException, DatabaseConnectionException,
AwsServiceException, SdkClientException, RedshiftDataException {
return cancelStatement(CancelStatementRequest.builder().applyMutation(cancelStatementRequest).build());
}
/**
*
* Describes the details about a specific instance when a query was run by the Amazon Redshift Data API. The
* information includes when the query started, when it finished, the query status, the number of rows returned, and
* the SQL statement.
*
*
* For more information about the Amazon Redshift Data API and CLI usage examples, see Using the Amazon Redshift Data API in
* the Amazon Redshift Management Guide.
*
*
* @param describeStatementRequest
* @return Result of the DescribeStatement operation returned by the service.
* @throws ValidationException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws ResourceNotFoundException
* The Amazon Redshift Data API operation failed due to a missing resource.
* @throws InternalServerException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws RedshiftDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample RedshiftDataClient.DescribeStatement
* @see AWS API Documentation
*/
default DescribeStatementResponse describeStatement(DescribeStatementRequest describeStatementRequest)
throws ValidationException, ResourceNotFoundException, InternalServerException, AwsServiceException,
SdkClientException, RedshiftDataException {
throw new UnsupportedOperationException();
}
/**
*
* Describes the details about a specific instance when a query was run by the Amazon Redshift Data API. The
* information includes when the query started, when it finished, the query status, the number of rows returned, and
* the SQL statement.
*
*
* For more information about the Amazon Redshift Data API and CLI usage examples, see Using the Amazon Redshift Data API in
* the Amazon Redshift Management Guide.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeStatementRequest.Builder} avoiding the need
* to create one manually via {@link DescribeStatementRequest#builder()}
*
*
* @param describeStatementRequest
* A {@link Consumer} that will call methods on {@link DescribeStatementRequest.Builder} to create a request.
* @return Result of the DescribeStatement operation returned by the service.
* @throws ValidationException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws ResourceNotFoundException
* The Amazon Redshift Data API operation failed due to a missing resource.
* @throws InternalServerException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws RedshiftDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample RedshiftDataClient.DescribeStatement
* @see AWS API Documentation
*/
default DescribeStatementResponse describeStatement(Consumer describeStatementRequest)
throws ValidationException, ResourceNotFoundException, InternalServerException, AwsServiceException,
SdkClientException, RedshiftDataException {
return describeStatement(DescribeStatementRequest.builder().applyMutation(describeStatementRequest).build());
}
/**
*
* Describes the detailed information about a table from metadata in the cluster. The information includes its
* columns. A token is returned to page through the column list. Depending on the authorization method, use one of
* the following combinations of request parameters:
*
*
* -
*
* Secrets Manager - when connecting to a cluster, specify the Amazon Resource Name (ARN) of the secret, the
* database name, and the cluster identifier that matches the cluster in the secret. When connecting to a serverless
* workgroup, specify the Amazon Resource Name (ARN) of the secret and the database name.
*
*
* -
*
* Temporary credentials - when connecting to a cluster, specify the cluster identifier, the database name, and the
* database user name. Also, permission to call the redshift:GetClusterCredentials
operation is
* required. When connecting to a serverless workgroup, specify the workgroup name and database name. Also,
* permission to call the redshift-serverless:GetCredentials
operation is required.
*
*
*
*
* For more information about the Amazon Redshift Data API and CLI usage examples, see Using the Amazon Redshift Data API in
* the Amazon Redshift Management Guide.
*
*
* @param describeTableRequest
* @return Result of the DescribeTable operation returned by the service.
* @throws ValidationException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws InternalServerException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws DatabaseConnectionException
* Connection to a database failed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws RedshiftDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample RedshiftDataClient.DescribeTable
* @see AWS
* API Documentation
*/
default DescribeTableResponse describeTable(DescribeTableRequest describeTableRequest) throws ValidationException,
InternalServerException, DatabaseConnectionException, AwsServiceException, SdkClientException, RedshiftDataException {
throw new UnsupportedOperationException();
}
/**
*
* Describes the detailed information about a table from metadata in the cluster. The information includes its
* columns. A token is returned to page through the column list. Depending on the authorization method, use one of
* the following combinations of request parameters:
*
*
* -
*
* Secrets Manager - when connecting to a cluster, specify the Amazon Resource Name (ARN) of the secret, the
* database name, and the cluster identifier that matches the cluster in the secret. When connecting to a serverless
* workgroup, specify the Amazon Resource Name (ARN) of the secret and the database name.
*
*
* -
*
* Temporary credentials - when connecting to a cluster, specify the cluster identifier, the database name, and the
* database user name. Also, permission to call the redshift:GetClusterCredentials
operation is
* required. When connecting to a serverless workgroup, specify the workgroup name and database name. Also,
* permission to call the redshift-serverless:GetCredentials
operation is required.
*
*
*
*
* For more information about the Amazon Redshift Data API and CLI usage examples, see Using the Amazon Redshift Data API in
* the Amazon Redshift Management Guide.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeTableRequest.Builder} avoiding the need to
* create one manually via {@link DescribeTableRequest#builder()}
*
*
* @param describeTableRequest
* A {@link Consumer} that will call methods on {@link DescribeTableRequest.Builder} to create a request.
* @return Result of the DescribeTable operation returned by the service.
* @throws ValidationException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws InternalServerException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws DatabaseConnectionException
* Connection to a database failed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws RedshiftDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample RedshiftDataClient.DescribeTable
* @see AWS
* API Documentation
*/
default DescribeTableResponse describeTable(Consumer describeTableRequest)
throws ValidationException, InternalServerException, DatabaseConnectionException, AwsServiceException,
SdkClientException, RedshiftDataException {
return describeTable(DescribeTableRequest.builder().applyMutation(describeTableRequest).build());
}
/**
*
* Describes the detailed information about a table from metadata in the cluster. The information includes its
* columns. A token is returned to page through the column list. Depending on the authorization method, use one of
* the following combinations of request parameters:
*
*
* -
*
* Secrets Manager - when connecting to a cluster, specify the Amazon Resource Name (ARN) of the secret, the
* database name, and the cluster identifier that matches the cluster in the secret. When connecting to a serverless
* workgroup, specify the Amazon Resource Name (ARN) of the secret and the database name.
*
*
* -
*
* Temporary credentials - when connecting to a cluster, specify the cluster identifier, the database name, and the
* database user name. Also, permission to call the redshift:GetClusterCredentials
operation is
* required. When connecting to a serverless workgroup, specify the workgroup name and database name. Also,
* permission to call the redshift-serverless:GetCredentials
operation is required.
*
*
*
*
* For more information about the Amazon Redshift Data API and CLI usage examples, see Using the Amazon Redshift Data API in
* the Amazon Redshift Management Guide.
*
*
*
* This is a variant of
* {@link #describeTable(software.amazon.awssdk.services.redshiftdata.model.DescribeTableRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.redshiftdata.paginators.DescribeTableIterable responses = client.describeTablePaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.redshiftdata.paginators.DescribeTableIterable responses = client
* .describeTablePaginator(request);
* for (software.amazon.awssdk.services.redshiftdata.model.DescribeTableResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.redshiftdata.paginators.DescribeTableIterable responses = client.describeTablePaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeTable(software.amazon.awssdk.services.redshiftdata.model.DescribeTableRequest)} operation.
*
*
* @param describeTableRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ValidationException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws InternalServerException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws DatabaseConnectionException
* Connection to a database failed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws RedshiftDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample RedshiftDataClient.DescribeTable
* @see AWS
* API Documentation
*/
default DescribeTableIterable describeTablePaginator(DescribeTableRequest describeTableRequest) throws ValidationException,
InternalServerException, DatabaseConnectionException, AwsServiceException, SdkClientException, RedshiftDataException {
throw new UnsupportedOperationException();
}
/**
*
* Describes the detailed information about a table from metadata in the cluster. The information includes its
* columns. A token is returned to page through the column list. Depending on the authorization method, use one of
* the following combinations of request parameters:
*
*
* -
*
* Secrets Manager - when connecting to a cluster, specify the Amazon Resource Name (ARN) of the secret, the
* database name, and the cluster identifier that matches the cluster in the secret. When connecting to a serverless
* workgroup, specify the Amazon Resource Name (ARN) of the secret and the database name.
*
*
* -
*
* Temporary credentials - when connecting to a cluster, specify the cluster identifier, the database name, and the
* database user name. Also, permission to call the redshift:GetClusterCredentials
operation is
* required. When connecting to a serverless workgroup, specify the workgroup name and database name. Also,
* permission to call the redshift-serverless:GetCredentials
operation is required.
*
*
*
*
* For more information about the Amazon Redshift Data API and CLI usage examples, see Using the Amazon Redshift Data API in
* the Amazon Redshift Management Guide.
*
*
*
* This is a variant of
* {@link #describeTable(software.amazon.awssdk.services.redshiftdata.model.DescribeTableRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.redshiftdata.paginators.DescribeTableIterable responses = client.describeTablePaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.redshiftdata.paginators.DescribeTableIterable responses = client
* .describeTablePaginator(request);
* for (software.amazon.awssdk.services.redshiftdata.model.DescribeTableResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.redshiftdata.paginators.DescribeTableIterable responses = client.describeTablePaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeTable(software.amazon.awssdk.services.redshiftdata.model.DescribeTableRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link DescribeTableRequest.Builder} avoiding the need to
* create one manually via {@link DescribeTableRequest#builder()}
*
*
* @param describeTableRequest
* A {@link Consumer} that will call methods on {@link DescribeTableRequest.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ValidationException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws InternalServerException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws DatabaseConnectionException
* Connection to a database failed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws RedshiftDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample RedshiftDataClient.DescribeTable
* @see AWS
* API Documentation
*/
default DescribeTableIterable describeTablePaginator(Consumer describeTableRequest)
throws ValidationException, InternalServerException, DatabaseConnectionException, AwsServiceException,
SdkClientException, RedshiftDataException {
return describeTablePaginator(DescribeTableRequest.builder().applyMutation(describeTableRequest).build());
}
/**
*
* Runs an SQL statement, which can be data manipulation language (DML) or data definition language (DDL). This
* statement must be a single SQL statement. Depending on the authorization method, use one of the following
* combinations of request parameters:
*
*
* -
*
* Secrets Manager - when connecting to a cluster, specify the Amazon Resource Name (ARN) of the secret, the
* database name, and the cluster identifier that matches the cluster in the secret. When connecting to a serverless
* workgroup, specify the Amazon Resource Name (ARN) of the secret and the database name.
*
*
* -
*
* Temporary credentials - when connecting to a cluster, specify the cluster identifier, the database name, and the
* database user name. Also, permission to call the redshift:GetClusterCredentials
operation is
* required. When connecting to a serverless workgroup, specify the workgroup name and database name. Also,
* permission to call the redshift-serverless:GetCredentials
operation is required.
*
*
*
*
* For more information about the Amazon Redshift Data API and CLI usage examples, see Using the Amazon Redshift Data API in
* the Amazon Redshift Management Guide.
*
*
* @param executeStatementRequest
* @return Result of the ExecuteStatement operation returned by the service.
* @throws ValidationException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws ExecuteStatementException
* The SQL statement encountered an environmental error while running.
* @throws ActiveStatementsExceededException
* The number of active statements exceeds the limit.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws RedshiftDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample RedshiftDataClient.ExecuteStatement
* @see AWS API Documentation
*/
default ExecuteStatementResponse executeStatement(ExecuteStatementRequest executeStatementRequest)
throws ValidationException, ExecuteStatementException, ActiveStatementsExceededException, AwsServiceException,
SdkClientException, RedshiftDataException {
throw new UnsupportedOperationException();
}
/**
*
* Runs an SQL statement, which can be data manipulation language (DML) or data definition language (DDL). This
* statement must be a single SQL statement. Depending on the authorization method, use one of the following
* combinations of request parameters:
*
*
* -
*
* Secrets Manager - when connecting to a cluster, specify the Amazon Resource Name (ARN) of the secret, the
* database name, and the cluster identifier that matches the cluster in the secret. When connecting to a serverless
* workgroup, specify the Amazon Resource Name (ARN) of the secret and the database name.
*
*
* -
*
* Temporary credentials - when connecting to a cluster, specify the cluster identifier, the database name, and the
* database user name. Also, permission to call the redshift:GetClusterCredentials
operation is
* required. When connecting to a serverless workgroup, specify the workgroup name and database name. Also,
* permission to call the redshift-serverless:GetCredentials
operation is required.
*
*
*
*
* For more information about the Amazon Redshift Data API and CLI usage examples, see Using the Amazon Redshift Data API in
* the Amazon Redshift Management Guide.
*
*
*
* This is a convenience which creates an instance of the {@link ExecuteStatementRequest.Builder} avoiding the need
* to create one manually via {@link ExecuteStatementRequest#builder()}
*
*
* @param executeStatementRequest
* A {@link Consumer} that will call methods on {@link ExecuteStatementInput.Builder} to create a request.
* @return Result of the ExecuteStatement operation returned by the service.
* @throws ValidationException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws ExecuteStatementException
* The SQL statement encountered an environmental error while running.
* @throws ActiveStatementsExceededException
* The number of active statements exceeds the limit.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws RedshiftDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample RedshiftDataClient.ExecuteStatement
* @see AWS API Documentation
*/
default ExecuteStatementResponse executeStatement(Consumer executeStatementRequest)
throws ValidationException, ExecuteStatementException, ActiveStatementsExceededException, AwsServiceException,
SdkClientException, RedshiftDataException {
return executeStatement(ExecuteStatementRequest.builder().applyMutation(executeStatementRequest).build());
}
/**
*
* Fetches the temporarily cached result of an SQL statement. A token is returned to page through the statement
* results.
*
*
* For more information about the Amazon Redshift Data API and CLI usage examples, see Using the Amazon Redshift Data API in
* the Amazon Redshift Management Guide.
*
*
* @param getStatementResultRequest
* @return Result of the GetStatementResult operation returned by the service.
* @throws ValidationException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws ResourceNotFoundException
* The Amazon Redshift Data API operation failed due to a missing resource.
* @throws InternalServerException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws RedshiftDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample RedshiftDataClient.GetStatementResult
* @see AWS API Documentation
*/
default GetStatementResultResponse getStatementResult(GetStatementResultRequest getStatementResultRequest)
throws ValidationException, ResourceNotFoundException, InternalServerException, AwsServiceException,
SdkClientException, RedshiftDataException {
throw new UnsupportedOperationException();
}
/**
*
* Fetches the temporarily cached result of an SQL statement. A token is returned to page through the statement
* results.
*
*
* For more information about the Amazon Redshift Data API and CLI usage examples, see Using the Amazon Redshift Data API in
* the Amazon Redshift Management Guide.
*
*
*
* This is a convenience which creates an instance of the {@link GetStatementResultRequest.Builder} avoiding the
* need to create one manually via {@link GetStatementResultRequest#builder()}
*
*
* @param getStatementResultRequest
* A {@link Consumer} that will call methods on {@link GetStatementResultRequest.Builder} to create a
* request.
* @return Result of the GetStatementResult operation returned by the service.
* @throws ValidationException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws ResourceNotFoundException
* The Amazon Redshift Data API operation failed due to a missing resource.
* @throws InternalServerException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws RedshiftDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample RedshiftDataClient.GetStatementResult
* @see AWS API Documentation
*/
default GetStatementResultResponse getStatementResult(Consumer getStatementResultRequest)
throws ValidationException, ResourceNotFoundException, InternalServerException, AwsServiceException,
SdkClientException, RedshiftDataException {
return getStatementResult(GetStatementResultRequest.builder().applyMutation(getStatementResultRequest).build());
}
/**
*
* Fetches the temporarily cached result of an SQL statement. A token is returned to page through the statement
* results.
*
*
* For more information about the Amazon Redshift Data API and CLI usage examples, see Using the Amazon Redshift Data API in
* the Amazon Redshift Management Guide.
*
*
*
* This is a variant of
* {@link #getStatementResult(software.amazon.awssdk.services.redshiftdata.model.GetStatementResultRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.redshiftdata.paginators.GetStatementResultIterable responses = client.getStatementResultPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.redshiftdata.paginators.GetStatementResultIterable responses = client
* .getStatementResultPaginator(request);
* for (software.amazon.awssdk.services.redshiftdata.model.GetStatementResultResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.redshiftdata.paginators.GetStatementResultIterable responses = client.getStatementResultPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getStatementResult(software.amazon.awssdk.services.redshiftdata.model.GetStatementResultRequest)}
* operation.
*
*
* @param getStatementResultRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ValidationException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws ResourceNotFoundException
* The Amazon Redshift Data API operation failed due to a missing resource.
* @throws InternalServerException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws RedshiftDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample RedshiftDataClient.GetStatementResult
* @see AWS API Documentation
*/
default GetStatementResultIterable getStatementResultPaginator(GetStatementResultRequest getStatementResultRequest)
throws ValidationException, ResourceNotFoundException, InternalServerException, AwsServiceException,
SdkClientException, RedshiftDataException {
throw new UnsupportedOperationException();
}
/**
*
* Fetches the temporarily cached result of an SQL statement. A token is returned to page through the statement
* results.
*
*
* For more information about the Amazon Redshift Data API and CLI usage examples, see Using the Amazon Redshift Data API in
* the Amazon Redshift Management Guide.
*
*
*
* This is a variant of
* {@link #getStatementResult(software.amazon.awssdk.services.redshiftdata.model.GetStatementResultRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.redshiftdata.paginators.GetStatementResultIterable responses = client.getStatementResultPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.redshiftdata.paginators.GetStatementResultIterable responses = client
* .getStatementResultPaginator(request);
* for (software.amazon.awssdk.services.redshiftdata.model.GetStatementResultResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.redshiftdata.paginators.GetStatementResultIterable responses = client.getStatementResultPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getStatementResult(software.amazon.awssdk.services.redshiftdata.model.GetStatementResultRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link GetStatementResultRequest.Builder} avoiding the
* need to create one manually via {@link GetStatementResultRequest#builder()}
*
*
* @param getStatementResultRequest
* A {@link Consumer} that will call methods on {@link GetStatementResultRequest.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ValidationException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws ResourceNotFoundException
* The Amazon Redshift Data API operation failed due to a missing resource.
* @throws InternalServerException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws RedshiftDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample RedshiftDataClient.GetStatementResult
* @see AWS API Documentation
*/
default GetStatementResultIterable getStatementResultPaginator(
Consumer getStatementResultRequest) throws ValidationException,
ResourceNotFoundException, InternalServerException, AwsServiceException, SdkClientException, RedshiftDataException {
return getStatementResultPaginator(GetStatementResultRequest.builder().applyMutation(getStatementResultRequest).build());
}
/**
*
* List the databases in a cluster. A token is returned to page through the database list. Depending on the
* authorization method, use one of the following combinations of request parameters:
*
*
* -
*
* Secrets Manager - when connecting to a cluster, specify the Amazon Resource Name (ARN) of the secret, the
* database name, and the cluster identifier that matches the cluster in the secret. When connecting to a serverless
* workgroup, specify the Amazon Resource Name (ARN) of the secret and the database name.
*
*
* -
*
* Temporary credentials - when connecting to a cluster, specify the cluster identifier, the database name, and the
* database user name. Also, permission to call the redshift:GetClusterCredentials
operation is
* required. When connecting to a serverless workgroup, specify the workgroup name and database name. Also,
* permission to call the redshift-serverless:GetCredentials
operation is required.
*
*
*
*
* For more information about the Amazon Redshift Data API and CLI usage examples, see Using the Amazon Redshift Data API in
* the Amazon Redshift Management Guide.
*
*
* @param listDatabasesRequest
* @return Result of the ListDatabases operation returned by the service.
* @throws ValidationException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws InternalServerException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws DatabaseConnectionException
* Connection to a database failed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws RedshiftDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample RedshiftDataClient.ListDatabases
* @see AWS
* API Documentation
*/
default ListDatabasesResponse listDatabases(ListDatabasesRequest listDatabasesRequest) throws ValidationException,
InternalServerException, DatabaseConnectionException, AwsServiceException, SdkClientException, RedshiftDataException {
throw new UnsupportedOperationException();
}
/**
*
* List the databases in a cluster. A token is returned to page through the database list. Depending on the
* authorization method, use one of the following combinations of request parameters:
*
*
* -
*
* Secrets Manager - when connecting to a cluster, specify the Amazon Resource Name (ARN) of the secret, the
* database name, and the cluster identifier that matches the cluster in the secret. When connecting to a serverless
* workgroup, specify the Amazon Resource Name (ARN) of the secret and the database name.
*
*
* -
*
* Temporary credentials - when connecting to a cluster, specify the cluster identifier, the database name, and the
* database user name. Also, permission to call the redshift:GetClusterCredentials
operation is
* required. When connecting to a serverless workgroup, specify the workgroup name and database name. Also,
* permission to call the redshift-serverless:GetCredentials
operation is required.
*
*
*
*
* For more information about the Amazon Redshift Data API and CLI usage examples, see Using the Amazon Redshift Data API in
* the Amazon Redshift Management Guide.
*
*
*
* This is a convenience which creates an instance of the {@link ListDatabasesRequest.Builder} avoiding the need to
* create one manually via {@link ListDatabasesRequest#builder()}
*
*
* @param listDatabasesRequest
* A {@link Consumer} that will call methods on {@link ListDatabasesRequest.Builder} to create a request.
* @return Result of the ListDatabases operation returned by the service.
* @throws ValidationException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws InternalServerException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws DatabaseConnectionException
* Connection to a database failed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws RedshiftDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample RedshiftDataClient.ListDatabases
* @see AWS
* API Documentation
*/
default ListDatabasesResponse listDatabases(Consumer listDatabasesRequest)
throws ValidationException, InternalServerException, DatabaseConnectionException, AwsServiceException,
SdkClientException, RedshiftDataException {
return listDatabases(ListDatabasesRequest.builder().applyMutation(listDatabasesRequest).build());
}
/**
*
* List the databases in a cluster. A token is returned to page through the database list. Depending on the
* authorization method, use one of the following combinations of request parameters:
*
*
* -
*
* Secrets Manager - when connecting to a cluster, specify the Amazon Resource Name (ARN) of the secret, the
* database name, and the cluster identifier that matches the cluster in the secret. When connecting to a serverless
* workgroup, specify the Amazon Resource Name (ARN) of the secret and the database name.
*
*
* -
*
* Temporary credentials - when connecting to a cluster, specify the cluster identifier, the database name, and the
* database user name. Also, permission to call the redshift:GetClusterCredentials
operation is
* required. When connecting to a serverless workgroup, specify the workgroup name and database name. Also,
* permission to call the redshift-serverless:GetCredentials
operation is required.
*
*
*
*
* For more information about the Amazon Redshift Data API and CLI usage examples, see Using the Amazon Redshift Data API in
* the Amazon Redshift Management Guide.
*
*
*
* This is a variant of
* {@link #listDatabases(software.amazon.awssdk.services.redshiftdata.model.ListDatabasesRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.redshiftdata.paginators.ListDatabasesIterable responses = client.listDatabasesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.redshiftdata.paginators.ListDatabasesIterable responses = client
* .listDatabasesPaginator(request);
* for (software.amazon.awssdk.services.redshiftdata.model.ListDatabasesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.redshiftdata.paginators.ListDatabasesIterable responses = client.listDatabasesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listDatabases(software.amazon.awssdk.services.redshiftdata.model.ListDatabasesRequest)} operation.
*
*
* @param listDatabasesRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ValidationException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws InternalServerException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws DatabaseConnectionException
* Connection to a database failed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws RedshiftDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample RedshiftDataClient.ListDatabases
* @see AWS
* API Documentation
*/
default ListDatabasesIterable listDatabasesPaginator(ListDatabasesRequest listDatabasesRequest) throws ValidationException,
InternalServerException, DatabaseConnectionException, AwsServiceException, SdkClientException, RedshiftDataException {
throw new UnsupportedOperationException();
}
/**
*
* List the databases in a cluster. A token is returned to page through the database list. Depending on the
* authorization method, use one of the following combinations of request parameters:
*
*
* -
*
* Secrets Manager - when connecting to a cluster, specify the Amazon Resource Name (ARN) of the secret, the
* database name, and the cluster identifier that matches the cluster in the secret. When connecting to a serverless
* workgroup, specify the Amazon Resource Name (ARN) of the secret and the database name.
*
*
* -
*
* Temporary credentials - when connecting to a cluster, specify the cluster identifier, the database name, and the
* database user name. Also, permission to call the redshift:GetClusterCredentials
operation is
* required. When connecting to a serverless workgroup, specify the workgroup name and database name. Also,
* permission to call the redshift-serverless:GetCredentials
operation is required.
*
*
*
*
* For more information about the Amazon Redshift Data API and CLI usage examples, see Using the Amazon Redshift Data API in
* the Amazon Redshift Management Guide.
*
*
*
* This is a variant of
* {@link #listDatabases(software.amazon.awssdk.services.redshiftdata.model.ListDatabasesRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.redshiftdata.paginators.ListDatabasesIterable responses = client.listDatabasesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.redshiftdata.paginators.ListDatabasesIterable responses = client
* .listDatabasesPaginator(request);
* for (software.amazon.awssdk.services.redshiftdata.model.ListDatabasesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.redshiftdata.paginators.ListDatabasesIterable responses = client.listDatabasesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listDatabases(software.amazon.awssdk.services.redshiftdata.model.ListDatabasesRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListDatabasesRequest.Builder} avoiding the need to
* create one manually via {@link ListDatabasesRequest#builder()}
*
*
* @param listDatabasesRequest
* A {@link Consumer} that will call methods on {@link ListDatabasesRequest.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ValidationException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws InternalServerException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws DatabaseConnectionException
* Connection to a database failed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws RedshiftDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample RedshiftDataClient.ListDatabases
* @see AWS
* API Documentation
*/
default ListDatabasesIterable listDatabasesPaginator(Consumer listDatabasesRequest)
throws ValidationException, InternalServerException, DatabaseConnectionException, AwsServiceException,
SdkClientException, RedshiftDataException {
return listDatabasesPaginator(ListDatabasesRequest.builder().applyMutation(listDatabasesRequest).build());
}
/**
*
* Lists the schemas in a database. A token is returned to page through the schema list. Depending on the
* authorization method, use one of the following combinations of request parameters:
*
*
* -
*
* Secrets Manager - when connecting to a cluster, specify the Amazon Resource Name (ARN) of the secret, the
* database name, and the cluster identifier that matches the cluster in the secret. When connecting to a serverless
* workgroup, specify the Amazon Resource Name (ARN) of the secret and the database name.
*
*
* -
*
* Temporary credentials - when connecting to a cluster, specify the cluster identifier, the database name, and the
* database user name. Also, permission to call the redshift:GetClusterCredentials
operation is
* required. When connecting to a serverless workgroup, specify the workgroup name and database name. Also,
* permission to call the redshift-serverless:GetCredentials
operation is required.
*
*
*
*
* For more information about the Amazon Redshift Data API and CLI usage examples, see Using the Amazon Redshift Data API in
* the Amazon Redshift Management Guide.
*
*
* @param listSchemasRequest
* @return Result of the ListSchemas operation returned by the service.
* @throws ValidationException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws InternalServerException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws DatabaseConnectionException
* Connection to a database failed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws RedshiftDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample RedshiftDataClient.ListSchemas
* @see AWS API
* Documentation
*/
default ListSchemasResponse listSchemas(ListSchemasRequest listSchemasRequest) throws ValidationException,
InternalServerException, DatabaseConnectionException, AwsServiceException, SdkClientException, RedshiftDataException {
throw new UnsupportedOperationException();
}
/**
*
* Lists the schemas in a database. A token is returned to page through the schema list. Depending on the
* authorization method, use one of the following combinations of request parameters:
*
*
* -
*
* Secrets Manager - when connecting to a cluster, specify the Amazon Resource Name (ARN) of the secret, the
* database name, and the cluster identifier that matches the cluster in the secret. When connecting to a serverless
* workgroup, specify the Amazon Resource Name (ARN) of the secret and the database name.
*
*
* -
*
* Temporary credentials - when connecting to a cluster, specify the cluster identifier, the database name, and the
* database user name. Also, permission to call the redshift:GetClusterCredentials
operation is
* required. When connecting to a serverless workgroup, specify the workgroup name and database name. Also,
* permission to call the redshift-serverless:GetCredentials
operation is required.
*
*
*
*
* For more information about the Amazon Redshift Data API and CLI usage examples, see Using the Amazon Redshift Data API in
* the Amazon Redshift Management Guide.
*
*
*
* This is a convenience which creates an instance of the {@link ListSchemasRequest.Builder} avoiding the need to
* create one manually via {@link ListSchemasRequest#builder()}
*
*
* @param listSchemasRequest
* A {@link Consumer} that will call methods on {@link ListSchemasRequest.Builder} to create a request.
* @return Result of the ListSchemas operation returned by the service.
* @throws ValidationException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws InternalServerException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws DatabaseConnectionException
* Connection to a database failed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws RedshiftDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample RedshiftDataClient.ListSchemas
* @see AWS API
* Documentation
*/
default ListSchemasResponse listSchemas(Consumer listSchemasRequest) throws ValidationException,
InternalServerException, DatabaseConnectionException, AwsServiceException, SdkClientException, RedshiftDataException {
return listSchemas(ListSchemasRequest.builder().applyMutation(listSchemasRequest).build());
}
/**
*
* Lists the schemas in a database. A token is returned to page through the schema list. Depending on the
* authorization method, use one of the following combinations of request parameters:
*
*
* -
*
* Secrets Manager - when connecting to a cluster, specify the Amazon Resource Name (ARN) of the secret, the
* database name, and the cluster identifier that matches the cluster in the secret. When connecting to a serverless
* workgroup, specify the Amazon Resource Name (ARN) of the secret and the database name.
*
*
* -
*
* Temporary credentials - when connecting to a cluster, specify the cluster identifier, the database name, and the
* database user name. Also, permission to call the redshift:GetClusterCredentials
operation is
* required. When connecting to a serverless workgroup, specify the workgroup name and database name. Also,
* permission to call the redshift-serverless:GetCredentials
operation is required.
*
*
*
*
* For more information about the Amazon Redshift Data API and CLI usage examples, see Using the Amazon Redshift Data API in
* the Amazon Redshift Management Guide.
*
*
*
* This is a variant of {@link #listSchemas(software.amazon.awssdk.services.redshiftdata.model.ListSchemasRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.redshiftdata.paginators.ListSchemasIterable responses = client.listSchemasPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.redshiftdata.paginators.ListSchemasIterable responses = client.listSchemasPaginator(request);
* for (software.amazon.awssdk.services.redshiftdata.model.ListSchemasResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.redshiftdata.paginators.ListSchemasIterable responses = client.listSchemasPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listSchemas(software.amazon.awssdk.services.redshiftdata.model.ListSchemasRequest)} operation.
*
*
* @param listSchemasRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ValidationException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws InternalServerException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws DatabaseConnectionException
* Connection to a database failed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws RedshiftDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample RedshiftDataClient.ListSchemas
* @see AWS API
* Documentation
*/
default ListSchemasIterable listSchemasPaginator(ListSchemasRequest listSchemasRequest) throws ValidationException,
InternalServerException, DatabaseConnectionException, AwsServiceException, SdkClientException, RedshiftDataException {
throw new UnsupportedOperationException();
}
/**
*
* Lists the schemas in a database. A token is returned to page through the schema list. Depending on the
* authorization method, use one of the following combinations of request parameters:
*
*
* -
*
* Secrets Manager - when connecting to a cluster, specify the Amazon Resource Name (ARN) of the secret, the
* database name, and the cluster identifier that matches the cluster in the secret. When connecting to a serverless
* workgroup, specify the Amazon Resource Name (ARN) of the secret and the database name.
*
*
* -
*
* Temporary credentials - when connecting to a cluster, specify the cluster identifier, the database name, and the
* database user name. Also, permission to call the redshift:GetClusterCredentials
operation is
* required. When connecting to a serverless workgroup, specify the workgroup name and database name. Also,
* permission to call the redshift-serverless:GetCredentials
operation is required.
*
*
*
*
* For more information about the Amazon Redshift Data API and CLI usage examples, see Using the Amazon Redshift Data API in
* the Amazon Redshift Management Guide.
*
*
*
* This is a variant of {@link #listSchemas(software.amazon.awssdk.services.redshiftdata.model.ListSchemasRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.redshiftdata.paginators.ListSchemasIterable responses = client.listSchemasPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.redshiftdata.paginators.ListSchemasIterable responses = client.listSchemasPaginator(request);
* for (software.amazon.awssdk.services.redshiftdata.model.ListSchemasResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.redshiftdata.paginators.ListSchemasIterable responses = client.listSchemasPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listSchemas(software.amazon.awssdk.services.redshiftdata.model.ListSchemasRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListSchemasRequest.Builder} avoiding the need to
* create one manually via {@link ListSchemasRequest#builder()}
*
*
* @param listSchemasRequest
* A {@link Consumer} that will call methods on {@link ListSchemasRequest.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ValidationException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws InternalServerException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws DatabaseConnectionException
* Connection to a database failed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws RedshiftDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample RedshiftDataClient.ListSchemas
* @see AWS API
* Documentation
*/
default ListSchemasIterable listSchemasPaginator(Consumer listSchemasRequest)
throws ValidationException, InternalServerException, DatabaseConnectionException, AwsServiceException,
SdkClientException, RedshiftDataException {
return listSchemasPaginator(ListSchemasRequest.builder().applyMutation(listSchemasRequest).build());
}
/**
*
* List of SQL statements. By default, only finished statements are shown. A token is returned to page through the
* statement list.
*
*
* For more information about the Amazon Redshift Data API and CLI usage examples, see Using the Amazon Redshift Data API in
* the Amazon Redshift Management Guide.
*
*
* @param listStatementsRequest
* @return Result of the ListStatements operation returned by the service.
* @throws ValidationException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws InternalServerException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws RedshiftDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample RedshiftDataClient.ListStatements
* @see AWS
* API Documentation
*/
default ListStatementsResponse listStatements(ListStatementsRequest listStatementsRequest) throws ValidationException,
InternalServerException, AwsServiceException, SdkClientException, RedshiftDataException {
throw new UnsupportedOperationException();
}
/**
*
* List of SQL statements. By default, only finished statements are shown. A token is returned to page through the
* statement list.
*
*
* For more information about the Amazon Redshift Data API and CLI usage examples, see Using the Amazon Redshift Data API in
* the Amazon Redshift Management Guide.
*
*
*
* This is a convenience which creates an instance of the {@link ListStatementsRequest.Builder} avoiding the need to
* create one manually via {@link ListStatementsRequest#builder()}
*
*
* @param listStatementsRequest
* A {@link Consumer} that will call methods on {@link ListStatementsRequest.Builder} to create a request.
* @return Result of the ListStatements operation returned by the service.
* @throws ValidationException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws InternalServerException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws RedshiftDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample RedshiftDataClient.ListStatements
* @see AWS
* API Documentation
*/
default ListStatementsResponse listStatements(Consumer listStatementsRequest)
throws ValidationException, InternalServerException, AwsServiceException, SdkClientException, RedshiftDataException {
return listStatements(ListStatementsRequest.builder().applyMutation(listStatementsRequest).build());
}
/**
*
* List of SQL statements. By default, only finished statements are shown. A token is returned to page through the
* statement list.
*
*
* For more information about the Amazon Redshift Data API and CLI usage examples, see Using the Amazon Redshift Data API in
* the Amazon Redshift Management Guide.
*
*
*
* This is a variant of
* {@link #listStatements(software.amazon.awssdk.services.redshiftdata.model.ListStatementsRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.redshiftdata.paginators.ListStatementsIterable responses = client.listStatementsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.redshiftdata.paginators.ListStatementsIterable responses = client
* .listStatementsPaginator(request);
* for (software.amazon.awssdk.services.redshiftdata.model.ListStatementsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.redshiftdata.paginators.ListStatementsIterable responses = client.listStatementsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listStatements(software.amazon.awssdk.services.redshiftdata.model.ListStatementsRequest)} operation.
*
*
* @param listStatementsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ValidationException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws InternalServerException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws RedshiftDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample RedshiftDataClient.ListStatements
* @see AWS
* API Documentation
*/
default ListStatementsIterable listStatementsPaginator(ListStatementsRequest listStatementsRequest)
throws ValidationException, InternalServerException, AwsServiceException, SdkClientException, RedshiftDataException {
throw new UnsupportedOperationException();
}
/**
*
* List of SQL statements. By default, only finished statements are shown. A token is returned to page through the
* statement list.
*
*
* For more information about the Amazon Redshift Data API and CLI usage examples, see Using the Amazon Redshift Data API in
* the Amazon Redshift Management Guide.
*
*
*
* This is a variant of
* {@link #listStatements(software.amazon.awssdk.services.redshiftdata.model.ListStatementsRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.redshiftdata.paginators.ListStatementsIterable responses = client.listStatementsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.redshiftdata.paginators.ListStatementsIterable responses = client
* .listStatementsPaginator(request);
* for (software.amazon.awssdk.services.redshiftdata.model.ListStatementsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.redshiftdata.paginators.ListStatementsIterable responses = client.listStatementsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listStatements(software.amazon.awssdk.services.redshiftdata.model.ListStatementsRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListStatementsRequest.Builder} avoiding the need to
* create one manually via {@link ListStatementsRequest#builder()}
*
*
* @param listStatementsRequest
* A {@link Consumer} that will call methods on {@link ListStatementsRequest.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ValidationException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws InternalServerException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws RedshiftDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample RedshiftDataClient.ListStatements
* @see AWS
* API Documentation
*/
default ListStatementsIterable listStatementsPaginator(Consumer listStatementsRequest)
throws ValidationException, InternalServerException, AwsServiceException, SdkClientException, RedshiftDataException {
return listStatementsPaginator(ListStatementsRequest.builder().applyMutation(listStatementsRequest).build());
}
/**
*
* List the tables in a database. If neither SchemaPattern
nor TablePattern
are specified,
* then all tables in the database are returned. A token is returned to page through the table list. Depending on
* the authorization method, use one of the following combinations of request parameters:
*
*
* -
*
* Secrets Manager - when connecting to a cluster, specify the Amazon Resource Name (ARN) of the secret, the
* database name, and the cluster identifier that matches the cluster in the secret. When connecting to a serverless
* workgroup, specify the Amazon Resource Name (ARN) of the secret and the database name.
*
*
* -
*
* Temporary credentials - when connecting to a cluster, specify the cluster identifier, the database name, and the
* database user name. Also, permission to call the redshift:GetClusterCredentials
operation is
* required. When connecting to a serverless workgroup, specify the workgroup name and database name. Also,
* permission to call the redshift-serverless:GetCredentials
operation is required.
*
*
*
*
* For more information about the Amazon Redshift Data API and CLI usage examples, see Using the Amazon Redshift Data API in
* the Amazon Redshift Management Guide.
*
*
* @param listTablesRequest
* @return Result of the ListTables operation returned by the service.
* @throws ValidationException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws InternalServerException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws DatabaseConnectionException
* Connection to a database failed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws RedshiftDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample RedshiftDataClient.ListTables
* @see AWS API
* Documentation
*/
default ListTablesResponse listTables(ListTablesRequest listTablesRequest) throws ValidationException,
InternalServerException, DatabaseConnectionException, AwsServiceException, SdkClientException, RedshiftDataException {
throw new UnsupportedOperationException();
}
/**
*
* List the tables in a database. If neither SchemaPattern
nor TablePattern
are specified,
* then all tables in the database are returned. A token is returned to page through the table list. Depending on
* the authorization method, use one of the following combinations of request parameters:
*
*
* -
*
* Secrets Manager - when connecting to a cluster, specify the Amazon Resource Name (ARN) of the secret, the
* database name, and the cluster identifier that matches the cluster in the secret. When connecting to a serverless
* workgroup, specify the Amazon Resource Name (ARN) of the secret and the database name.
*
*
* -
*
* Temporary credentials - when connecting to a cluster, specify the cluster identifier, the database name, and the
* database user name. Also, permission to call the redshift:GetClusterCredentials
operation is
* required. When connecting to a serverless workgroup, specify the workgroup name and database name. Also,
* permission to call the redshift-serverless:GetCredentials
operation is required.
*
*
*
*
* For more information about the Amazon Redshift Data API and CLI usage examples, see Using the Amazon Redshift Data API in
* the Amazon Redshift Management Guide.
*
*
*
* This is a convenience which creates an instance of the {@link ListTablesRequest.Builder} avoiding the need to
* create one manually via {@link ListTablesRequest#builder()}
*
*
* @param listTablesRequest
* A {@link Consumer} that will call methods on {@link ListTablesRequest.Builder} to create a request.
* @return Result of the ListTables operation returned by the service.
* @throws ValidationException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws InternalServerException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws DatabaseConnectionException
* Connection to a database failed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws RedshiftDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample RedshiftDataClient.ListTables
* @see AWS API
* Documentation
*/
default ListTablesResponse listTables(Consumer listTablesRequest) throws ValidationException,
InternalServerException, DatabaseConnectionException, AwsServiceException, SdkClientException, RedshiftDataException {
return listTables(ListTablesRequest.builder().applyMutation(listTablesRequest).build());
}
/**
*
* List the tables in a database. If neither SchemaPattern
nor TablePattern
are specified,
* then all tables in the database are returned. A token is returned to page through the table list. Depending on
* the authorization method, use one of the following combinations of request parameters:
*
*
* -
*
* Secrets Manager - when connecting to a cluster, specify the Amazon Resource Name (ARN) of the secret, the
* database name, and the cluster identifier that matches the cluster in the secret. When connecting to a serverless
* workgroup, specify the Amazon Resource Name (ARN) of the secret and the database name.
*
*
* -
*
* Temporary credentials - when connecting to a cluster, specify the cluster identifier, the database name, and the
* database user name. Also, permission to call the redshift:GetClusterCredentials
operation is
* required. When connecting to a serverless workgroup, specify the workgroup name and database name. Also,
* permission to call the redshift-serverless:GetCredentials
operation is required.
*
*
*
*
* For more information about the Amazon Redshift Data API and CLI usage examples, see Using the Amazon Redshift Data API in
* the Amazon Redshift Management Guide.
*
*
*
* This is a variant of {@link #listTables(software.amazon.awssdk.services.redshiftdata.model.ListTablesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.redshiftdata.paginators.ListTablesIterable responses = client.listTablesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.redshiftdata.paginators.ListTablesIterable responses = client.listTablesPaginator(request);
* for (software.amazon.awssdk.services.redshiftdata.model.ListTablesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.redshiftdata.paginators.ListTablesIterable responses = client.listTablesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listTables(software.amazon.awssdk.services.redshiftdata.model.ListTablesRequest)} operation.
*
*
* @param listTablesRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ValidationException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws InternalServerException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws DatabaseConnectionException
* Connection to a database failed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws RedshiftDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample RedshiftDataClient.ListTables
* @see AWS API
* Documentation
*/
default ListTablesIterable listTablesPaginator(ListTablesRequest listTablesRequest) throws ValidationException,
InternalServerException, DatabaseConnectionException, AwsServiceException, SdkClientException, RedshiftDataException {
throw new UnsupportedOperationException();
}
/**
*
* List the tables in a database. If neither SchemaPattern
nor TablePattern
are specified,
* then all tables in the database are returned. A token is returned to page through the table list. Depending on
* the authorization method, use one of the following combinations of request parameters:
*
*
* -
*
* Secrets Manager - when connecting to a cluster, specify the Amazon Resource Name (ARN) of the secret, the
* database name, and the cluster identifier that matches the cluster in the secret. When connecting to a serverless
* workgroup, specify the Amazon Resource Name (ARN) of the secret and the database name.
*
*
* -
*
* Temporary credentials - when connecting to a cluster, specify the cluster identifier, the database name, and the
* database user name. Also, permission to call the redshift:GetClusterCredentials
operation is
* required. When connecting to a serverless workgroup, specify the workgroup name and database name. Also,
* permission to call the redshift-serverless:GetCredentials
operation is required.
*
*
*
*
* For more information about the Amazon Redshift Data API and CLI usage examples, see Using the Amazon Redshift Data API in
* the Amazon Redshift Management Guide.
*
*
*
* This is a variant of {@link #listTables(software.amazon.awssdk.services.redshiftdata.model.ListTablesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.redshiftdata.paginators.ListTablesIterable responses = client.listTablesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.redshiftdata.paginators.ListTablesIterable responses = client.listTablesPaginator(request);
* for (software.amazon.awssdk.services.redshiftdata.model.ListTablesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.redshiftdata.paginators.ListTablesIterable responses = client.listTablesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listTables(software.amazon.awssdk.services.redshiftdata.model.ListTablesRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListTablesRequest.Builder} avoiding the need to
* create one manually via {@link ListTablesRequest#builder()}
*
*
* @param listTablesRequest
* A {@link Consumer} that will call methods on {@link ListTablesRequest.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ValidationException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws InternalServerException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws DatabaseConnectionException
* Connection to a database failed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws RedshiftDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample RedshiftDataClient.ListTables
* @see AWS API
* Documentation
*/
default ListTablesIterable listTablesPaginator(Consumer listTablesRequest)
throws ValidationException, InternalServerException, DatabaseConnectionException, AwsServiceException,
SdkClientException, RedshiftDataException {
return listTablesPaginator(ListTablesRequest.builder().applyMutation(listTablesRequest).build());
}
/**
* Create a {@link RedshiftDataClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static RedshiftDataClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link RedshiftDataClient}.
*/
static RedshiftDataClientBuilder builder() {
return new DefaultRedshiftDataClientBuilder();
}
static ServiceMetadata serviceMetadata() {
return ServiceMetadata.of(SERVICE_METADATA_ID);
}
}