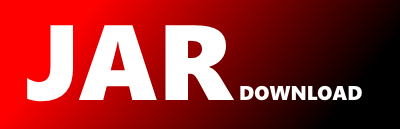
software.amazon.awssdk.services.redshiftdata.DefaultRedshiftDataAsyncClient Maven / Gradle / Ivy
Show all versions of redshiftdata Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.redshiftdata;
import java.util.Collections;
import java.util.List;
import java.util.concurrent.CompletableFuture;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.client.handler.AwsAsyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.awscore.internal.AwsProtocolMetadata;
import software.amazon.awssdk.awscore.internal.AwsServiceProtocol;
import software.amazon.awssdk.core.RequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkPlugin;
import software.amazon.awssdk.core.SdkRequest;
import software.amazon.awssdk.core.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientOption;
import software.amazon.awssdk.core.client.handler.AsyncClientHandler;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.metrics.CoreMetric;
import software.amazon.awssdk.metrics.MetricCollector;
import software.amazon.awssdk.metrics.MetricPublisher;
import software.amazon.awssdk.metrics.NoOpMetricCollector;
import software.amazon.awssdk.protocols.core.ExceptionMetadata;
import software.amazon.awssdk.protocols.json.AwsJsonProtocol;
import software.amazon.awssdk.protocols.json.AwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.BaseAwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.JsonOperationMetadata;
import software.amazon.awssdk.services.redshiftdata.internal.RedshiftDataServiceClientConfigurationBuilder;
import software.amazon.awssdk.services.redshiftdata.model.ActiveStatementsExceededException;
import software.amazon.awssdk.services.redshiftdata.model.BatchExecuteStatementException;
import software.amazon.awssdk.services.redshiftdata.model.BatchExecuteStatementRequest;
import software.amazon.awssdk.services.redshiftdata.model.BatchExecuteStatementResponse;
import software.amazon.awssdk.services.redshiftdata.model.CancelStatementRequest;
import software.amazon.awssdk.services.redshiftdata.model.CancelStatementResponse;
import software.amazon.awssdk.services.redshiftdata.model.DatabaseConnectionException;
import software.amazon.awssdk.services.redshiftdata.model.DescribeStatementRequest;
import software.amazon.awssdk.services.redshiftdata.model.DescribeStatementResponse;
import software.amazon.awssdk.services.redshiftdata.model.DescribeTableRequest;
import software.amazon.awssdk.services.redshiftdata.model.DescribeTableResponse;
import software.amazon.awssdk.services.redshiftdata.model.ExecuteStatementException;
import software.amazon.awssdk.services.redshiftdata.model.ExecuteStatementRequest;
import software.amazon.awssdk.services.redshiftdata.model.ExecuteStatementResponse;
import software.amazon.awssdk.services.redshiftdata.model.GetStatementResultRequest;
import software.amazon.awssdk.services.redshiftdata.model.GetStatementResultResponse;
import software.amazon.awssdk.services.redshiftdata.model.InternalServerException;
import software.amazon.awssdk.services.redshiftdata.model.ListDatabasesRequest;
import software.amazon.awssdk.services.redshiftdata.model.ListDatabasesResponse;
import software.amazon.awssdk.services.redshiftdata.model.ListSchemasRequest;
import software.amazon.awssdk.services.redshiftdata.model.ListSchemasResponse;
import software.amazon.awssdk.services.redshiftdata.model.ListStatementsRequest;
import software.amazon.awssdk.services.redshiftdata.model.ListStatementsResponse;
import software.amazon.awssdk.services.redshiftdata.model.ListTablesRequest;
import software.amazon.awssdk.services.redshiftdata.model.ListTablesResponse;
import software.amazon.awssdk.services.redshiftdata.model.RedshiftDataException;
import software.amazon.awssdk.services.redshiftdata.model.ResourceNotFoundException;
import software.amazon.awssdk.services.redshiftdata.model.ValidationException;
import software.amazon.awssdk.services.redshiftdata.transform.BatchExecuteStatementRequestMarshaller;
import software.amazon.awssdk.services.redshiftdata.transform.CancelStatementRequestMarshaller;
import software.amazon.awssdk.services.redshiftdata.transform.DescribeStatementRequestMarshaller;
import software.amazon.awssdk.services.redshiftdata.transform.DescribeTableRequestMarshaller;
import software.amazon.awssdk.services.redshiftdata.transform.ExecuteStatementRequestMarshaller;
import software.amazon.awssdk.services.redshiftdata.transform.GetStatementResultRequestMarshaller;
import software.amazon.awssdk.services.redshiftdata.transform.ListDatabasesRequestMarshaller;
import software.amazon.awssdk.services.redshiftdata.transform.ListSchemasRequestMarshaller;
import software.amazon.awssdk.services.redshiftdata.transform.ListStatementsRequestMarshaller;
import software.amazon.awssdk.services.redshiftdata.transform.ListTablesRequestMarshaller;
import software.amazon.awssdk.utils.CompletableFutureUtils;
/**
* Internal implementation of {@link RedshiftDataAsyncClient}.
*
* @see RedshiftDataAsyncClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultRedshiftDataAsyncClient implements RedshiftDataAsyncClient {
private static final Logger log = LoggerFactory.getLogger(DefaultRedshiftDataAsyncClient.class);
private static final AwsProtocolMetadata protocolMetadata = AwsProtocolMetadata.builder()
.serviceProtocol(AwsServiceProtocol.AWS_JSON).build();
private final AsyncClientHandler clientHandler;
private final AwsJsonProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
protected DefaultRedshiftDataAsyncClient(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsAsyncClientHandler(clientConfiguration);
this.clientConfiguration = clientConfiguration;
this.protocolFactory = init(AwsJsonProtocolFactory.builder()).build();
}
/**
*
* Runs one or more SQL statements, which can be data manipulation language (DML) or data definition language (DDL).
* Depending on the authorization method, use one of the following combinations of request parameters:
*
*
* -
*
* Secrets Manager - when connecting to a cluster, provide the secret-arn
of a secret stored in Secrets
* Manager which has username
and password
. The specified secret contains credentials to
* connect to the database
you specify. When you are connecting to a cluster, you also supply the
* database name, If you provide a cluster identifier (dbClusterIdentifier
), it must match the cluster
* identifier stored in the secret. When you are connecting to a serverless workgroup, you also supply the database
* name.
*
*
* -
*
* Temporary credentials - when connecting to your data warehouse, choose one of the following options:
*
*
* -
*
* When connecting to a serverless workgroup, specify the workgroup name and database name. The database user name
* is derived from the IAM identity. For example, arn:iam::123456789012:user:foo
has the database user
* name IAM:foo
. Also, permission to call the redshift-serverless:GetCredentials
operation
* is required.
*
*
* -
*
* When connecting to a cluster as an IAM identity, specify the cluster identifier and the database name. The
* database user name is derived from the IAM identity. For example, arn:iam::123456789012:user:foo
has
* the database user name IAM:foo
. Also, permission to call the
* redshift:GetClusterCredentialsWithIAM
operation is required.
*
*
* -
*
* When connecting to a cluster as a database user, specify the cluster identifier, the database name, and the
* database user name. Also, permission to call the redshift:GetClusterCredentials
operation is
* required.
*
*
*
*
*
*
* For more information about the Amazon Redshift Data API and CLI usage examples, see Using the Amazon Redshift Data API in
* the Amazon Redshift Management Guide.
*
*
* @param batchExecuteStatementRequest
* @return A Java Future containing the result of the BatchExecuteStatement operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The Amazon Redshift Data API operation failed due to invalid input.
* - ActiveStatementsExceededException The number of active statements exceeds the limit.
* - BatchExecuteStatementException An SQL statement encountered an environmental error while running.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RedshiftDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample RedshiftDataAsyncClient.BatchExecuteStatement
* @see AWS API Documentation
*/
@Override
public CompletableFuture batchExecuteStatement(
BatchExecuteStatementRequest batchExecuteStatementRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(batchExecuteStatementRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, batchExecuteStatementRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Redshift Data");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "BatchExecuteStatement");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, BatchExecuteStatementResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("BatchExecuteStatement").withProtocolMetadata(protocolMetadata)
.withMarshaller(new BatchExecuteStatementRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(batchExecuteStatementRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Cancels a running query. To be canceled, a query must be running.
*
*
* For more information about the Amazon Redshift Data API and CLI usage examples, see Using the Amazon Redshift Data API in
* the Amazon Redshift Management Guide.
*
*
* @param cancelStatementRequest
* @return A Java Future containing the result of the CancelStatement operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The Amazon Redshift Data API operation failed due to invalid input.
* - ResourceNotFoundException The Amazon Redshift Data API operation failed due to a missing resource.
* - InternalServerException The Amazon Redshift Data API operation failed due to invalid input.
* - DatabaseConnectionException Connection to a database failed.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RedshiftDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample RedshiftDataAsyncClient.CancelStatement
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture cancelStatement(CancelStatementRequest cancelStatementRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(cancelStatementRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, cancelStatementRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Redshift Data");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CancelStatement");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CancelStatementResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CancelStatement").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CancelStatementRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(cancelStatementRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Describes the details about a specific instance when a query was run by the Amazon Redshift Data API. The
* information includes when the query started, when it finished, the query status, the number of rows returned, and
* the SQL statement.
*
*
* For more information about the Amazon Redshift Data API and CLI usage examples, see Using the Amazon Redshift Data API in
* the Amazon Redshift Management Guide.
*
*
* @param describeStatementRequest
* @return A Java Future containing the result of the DescribeStatement operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The Amazon Redshift Data API operation failed due to invalid input.
* - ResourceNotFoundException The Amazon Redshift Data API operation failed due to a missing resource.
* - InternalServerException The Amazon Redshift Data API operation failed due to invalid input.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RedshiftDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample RedshiftDataAsyncClient.DescribeStatement
* @see AWS API Documentation
*/
@Override
public CompletableFuture describeStatement(DescribeStatementRequest describeStatementRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeStatementRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeStatementRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Redshift Data");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeStatement");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeStatementResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeStatement").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeStatementRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeStatementRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Describes the detailed information about a table from metadata in the cluster. The information includes its
* columns. A token is returned to page through the column list. Depending on the authorization method, use one of
* the following combinations of request parameters:
*
*
* -
*
* Secrets Manager - when connecting to a cluster, provide the secret-arn
of a secret stored in Secrets
* Manager which has username
and password
. The specified secret contains credentials to
* connect to the database
you specify. When you are connecting to a cluster, you also supply the
* database name, If you provide a cluster identifier (dbClusterIdentifier
), it must match the cluster
* identifier stored in the secret. When you are connecting to a serverless workgroup, you also supply the database
* name.
*
*
* -
*
* Temporary credentials - when connecting to your data warehouse, choose one of the following options:
*
*
* -
*
* When connecting to a serverless workgroup, specify the workgroup name and database name. The database user name
* is derived from the IAM identity. For example, arn:iam::123456789012:user:foo
has the database user
* name IAM:foo
. Also, permission to call the redshift-serverless:GetCredentials
operation
* is required.
*
*
* -
*
* When connecting to a cluster as an IAM identity, specify the cluster identifier and the database name. The
* database user name is derived from the IAM identity. For example, arn:iam::123456789012:user:foo
has
* the database user name IAM:foo
. Also, permission to call the
* redshift:GetClusterCredentialsWithIAM
operation is required.
*
*
* -
*
* When connecting to a cluster as a database user, specify the cluster identifier, the database name, and the
* database user name. Also, permission to call the redshift:GetClusterCredentials
operation is
* required.
*
*
*
*
*
*
* For more information about the Amazon Redshift Data API and CLI usage examples, see Using the Amazon Redshift Data API in
* the Amazon Redshift Management Guide.
*
*
* @param describeTableRequest
* @return A Java Future containing the result of the DescribeTable operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The Amazon Redshift Data API operation failed due to invalid input.
* - InternalServerException The Amazon Redshift Data API operation failed due to invalid input.
* - DatabaseConnectionException Connection to a database failed.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RedshiftDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample RedshiftDataAsyncClient.DescribeTable
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture describeTable(DescribeTableRequest describeTableRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeTableRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeTableRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Redshift Data");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeTable");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DescribeTableResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeTable").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeTableRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeTableRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Runs an SQL statement, which can be data manipulation language (DML) or data definition language (DDL). This
* statement must be a single SQL statement. Depending on the authorization method, use one of the following
* combinations of request parameters:
*
*
* -
*
* Secrets Manager - when connecting to a cluster, provide the secret-arn
of a secret stored in Secrets
* Manager which has username
and password
. The specified secret contains credentials to
* connect to the database
you specify. When you are connecting to a cluster, you also supply the
* database name, If you provide a cluster identifier (dbClusterIdentifier
), it must match the cluster
* identifier stored in the secret. When you are connecting to a serverless workgroup, you also supply the database
* name.
*
*
* -
*
* Temporary credentials - when connecting to your data warehouse, choose one of the following options:
*
*
* -
*
* When connecting to a serverless workgroup, specify the workgroup name and database name. The database user name
* is derived from the IAM identity. For example, arn:iam::123456789012:user:foo
has the database user
* name IAM:foo
. Also, permission to call the redshift-serverless:GetCredentials
operation
* is required.
*
*
* -
*
* When connecting to a cluster as an IAM identity, specify the cluster identifier and the database name. The
* database user name is derived from the IAM identity. For example, arn:iam::123456789012:user:foo
has
* the database user name IAM:foo
. Also, permission to call the
* redshift:GetClusterCredentialsWithIAM
operation is required.
*
*
* -
*
* When connecting to a cluster as a database user, specify the cluster identifier, the database name, and the
* database user name. Also, permission to call the redshift:GetClusterCredentials
operation is
* required.
*
*
*
*
*
*
* For more information about the Amazon Redshift Data API and CLI usage examples, see Using the Amazon Redshift Data API in
* the Amazon Redshift Management Guide.
*
*
* @param executeStatementRequest
* @return A Java Future containing the result of the ExecuteStatement operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The Amazon Redshift Data API operation failed due to invalid input.
* - ExecuteStatementException The SQL statement encountered an environmental error while running.
* - ActiveStatementsExceededException The number of active statements exceeds the limit.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RedshiftDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample RedshiftDataAsyncClient.ExecuteStatement
* @see AWS API Documentation
*/
@Override
public CompletableFuture executeStatement(ExecuteStatementRequest executeStatementRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(executeStatementRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, executeStatementRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Redshift Data");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ExecuteStatement");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ExecuteStatementResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ExecuteStatement").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ExecuteStatementRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(executeStatementRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Fetches the temporarily cached result of an SQL statement. A token is returned to page through the statement
* results.
*
*
* For more information about the Amazon Redshift Data API and CLI usage examples, see Using the Amazon Redshift Data API in
* the Amazon Redshift Management Guide.
*
*
* @param getStatementResultRequest
* @return A Java Future containing the result of the GetStatementResult operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The Amazon Redshift Data API operation failed due to invalid input.
* - ResourceNotFoundException The Amazon Redshift Data API operation failed due to a missing resource.
* - InternalServerException The Amazon Redshift Data API operation failed due to invalid input.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RedshiftDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample RedshiftDataAsyncClient.GetStatementResult
* @see AWS API Documentation
*/
@Override
public CompletableFuture getStatementResult(GetStatementResultRequest getStatementResultRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getStatementResultRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getStatementResultRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Redshift Data");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetStatementResult");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetStatementResultResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetStatementResult").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetStatementResultRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getStatementResultRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* List the databases in a cluster. A token is returned to page through the database list. Depending on the
* authorization method, use one of the following combinations of request parameters:
*
*
* -
*
* Secrets Manager - when connecting to a cluster, provide the secret-arn
of a secret stored in Secrets
* Manager which has username
and password
. The specified secret contains credentials to
* connect to the database
you specify. When you are connecting to a cluster, you also supply the
* database name, If you provide a cluster identifier (dbClusterIdentifier
), it must match the cluster
* identifier stored in the secret. When you are connecting to a serverless workgroup, you also supply the database
* name.
*
*
* -
*
* Temporary credentials - when connecting to your data warehouse, choose one of the following options:
*
*
* -
*
* When connecting to a serverless workgroup, specify the workgroup name and database name. The database user name
* is derived from the IAM identity. For example, arn:iam::123456789012:user:foo
has the database user
* name IAM:foo
. Also, permission to call the redshift-serverless:GetCredentials
operation
* is required.
*
*
* -
*
* When connecting to a cluster as an IAM identity, specify the cluster identifier and the database name. The
* database user name is derived from the IAM identity. For example, arn:iam::123456789012:user:foo
has
* the database user name IAM:foo
. Also, permission to call the
* redshift:GetClusterCredentialsWithIAM
operation is required.
*
*
* -
*
* When connecting to a cluster as a database user, specify the cluster identifier, the database name, and the
* database user name. Also, permission to call the redshift:GetClusterCredentials
operation is
* required.
*
*
*
*
*
*
* For more information about the Amazon Redshift Data API and CLI usage examples, see Using the Amazon Redshift Data API in
* the Amazon Redshift Management Guide.
*
*
* @param listDatabasesRequest
* @return A Java Future containing the result of the ListDatabases operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The Amazon Redshift Data API operation failed due to invalid input.
* - InternalServerException The Amazon Redshift Data API operation failed due to invalid input.
* - DatabaseConnectionException Connection to a database failed.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RedshiftDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample RedshiftDataAsyncClient.ListDatabases
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture listDatabases(ListDatabasesRequest listDatabasesRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listDatabasesRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listDatabasesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Redshift Data");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListDatabases");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListDatabasesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListDatabases").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListDatabasesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listDatabasesRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists the schemas in a database. A token is returned to page through the schema list. Depending on the
* authorization method, use one of the following combinations of request parameters:
*
*
* -
*
* Secrets Manager - when connecting to a cluster, provide the secret-arn
of a secret stored in Secrets
* Manager which has username
and password
. The specified secret contains credentials to
* connect to the database
you specify. When you are connecting to a cluster, you also supply the
* database name, If you provide a cluster identifier (dbClusterIdentifier
), it must match the cluster
* identifier stored in the secret. When you are connecting to a serverless workgroup, you also supply the database
* name.
*
*
* -
*
* Temporary credentials - when connecting to your data warehouse, choose one of the following options:
*
*
* -
*
* When connecting to a serverless workgroup, specify the workgroup name and database name. The database user name
* is derived from the IAM identity. For example, arn:iam::123456789012:user:foo
has the database user
* name IAM:foo
. Also, permission to call the redshift-serverless:GetCredentials
operation
* is required.
*
*
* -
*
* When connecting to a cluster as an IAM identity, specify the cluster identifier and the database name. The
* database user name is derived from the IAM identity. For example, arn:iam::123456789012:user:foo
has
* the database user name IAM:foo
. Also, permission to call the
* redshift:GetClusterCredentialsWithIAM
operation is required.
*
*
* -
*
* When connecting to a cluster as a database user, specify the cluster identifier, the database name, and the
* database user name. Also, permission to call the redshift:GetClusterCredentials
operation is
* required.
*
*
*
*
*
*
* For more information about the Amazon Redshift Data API and CLI usage examples, see Using the Amazon Redshift Data API in
* the Amazon Redshift Management Guide.
*
*
* @param listSchemasRequest
* @return A Java Future containing the result of the ListSchemas operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The Amazon Redshift Data API operation failed due to invalid input.
* - InternalServerException The Amazon Redshift Data API operation failed due to invalid input.
* - DatabaseConnectionException Connection to a database failed.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RedshiftDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample RedshiftDataAsyncClient.ListSchemas
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listSchemas(ListSchemasRequest listSchemasRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listSchemasRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listSchemasRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Redshift Data");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListSchemas");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListSchemasResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListSchemas").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListSchemasRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listSchemasRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* List of SQL statements. By default, only finished statements are shown. A token is returned to page through the
* statement list.
*
*
* For more information about the Amazon Redshift Data API and CLI usage examples, see Using the Amazon Redshift Data API in
* the Amazon Redshift Management Guide.
*
*
* @param listStatementsRequest
* @return A Java Future containing the result of the ListStatements operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The Amazon Redshift Data API operation failed due to invalid input.
* - InternalServerException The Amazon Redshift Data API operation failed due to invalid input.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RedshiftDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample RedshiftDataAsyncClient.ListStatements
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture listStatements(ListStatementsRequest listStatementsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listStatementsRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listStatementsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Redshift Data");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListStatements");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListStatementsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListStatements").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListStatementsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listStatementsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* List the tables in a database. If neither SchemaPattern
nor TablePattern
are specified,
* then all tables in the database are returned. A token is returned to page through the table list. Depending on
* the authorization method, use one of the following combinations of request parameters:
*
*
* -
*
* Secrets Manager - when connecting to a cluster, provide the secret-arn
of a secret stored in Secrets
* Manager which has username
and password
. The specified secret contains credentials to
* connect to the database
you specify. When you are connecting to a cluster, you also supply the
* database name, If you provide a cluster identifier (dbClusterIdentifier
), it must match the cluster
* identifier stored in the secret. When you are connecting to a serverless workgroup, you also supply the database
* name.
*
*
* -
*
* Temporary credentials - when connecting to your data warehouse, choose one of the following options:
*
*
* -
*
* When connecting to a serverless workgroup, specify the workgroup name and database name. The database user name
* is derived from the IAM identity. For example, arn:iam::123456789012:user:foo
has the database user
* name IAM:foo
. Also, permission to call the redshift-serverless:GetCredentials
operation
* is required.
*
*
* -
*
* When connecting to a cluster as an IAM identity, specify the cluster identifier and the database name. The
* database user name is derived from the IAM identity. For example, arn:iam::123456789012:user:foo
has
* the database user name IAM:foo
. Also, permission to call the
* redshift:GetClusterCredentialsWithIAM
operation is required.
*
*
* -
*
* When connecting to a cluster as a database user, specify the cluster identifier, the database name, and the
* database user name. Also, permission to call the redshift:GetClusterCredentials
operation is
* required.
*
*
*
*
*
*
* For more information about the Amazon Redshift Data API and CLI usage examples, see Using the Amazon Redshift Data API in
* the Amazon Redshift Management Guide.
*
*
* @param listTablesRequest
* @return A Java Future containing the result of the ListTables operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The Amazon Redshift Data API operation failed due to invalid input.
* - InternalServerException The Amazon Redshift Data API operation failed due to invalid input.
* - DatabaseConnectionException Connection to a database failed.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RedshiftDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample RedshiftDataAsyncClient.ListTables
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listTables(ListTablesRequest listTablesRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listTablesRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listTablesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Redshift Data");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListTables");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListTablesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("ListTables")
.withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListTablesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listTablesRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
@Override
public final RedshiftDataServiceClientConfiguration serviceClientConfiguration() {
return new RedshiftDataServiceClientConfigurationBuilder(this.clientConfiguration.toBuilder()).build();
}
@Override
public final String serviceName() {
return SERVICE_NAME;
}
private > T init(T builder) {
return builder
.clientConfiguration(clientConfiguration)
.defaultServiceExceptionSupplier(RedshiftDataException::builder)
.protocol(AwsJsonProtocol.AWS_JSON)
.protocolVersion("1.1")
.registerModeledException(
ExceptionMetadata.builder().errorCode("ActiveStatementsExceededException")
.exceptionBuilderSupplier(ActiveStatementsExceededException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ValidationException")
.exceptionBuilderSupplier(ValidationException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("DatabaseConnectionException")
.exceptionBuilderSupplier(DatabaseConnectionException::builder).httpStatusCode(500).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ResourceNotFoundException")
.exceptionBuilderSupplier(ResourceNotFoundException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InternalServerException")
.exceptionBuilderSupplier(InternalServerException::builder).httpStatusCode(500).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ExecuteStatementException")
.exceptionBuilderSupplier(ExecuteStatementException::builder).httpStatusCode(500).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("BatchExecuteStatementException")
.exceptionBuilderSupplier(BatchExecuteStatementException::builder).httpStatusCode(500).build());
}
private static List resolveMetricPublishers(SdkClientConfiguration clientConfiguration,
RequestOverrideConfiguration requestOverrideConfiguration) {
List publishers = null;
if (requestOverrideConfiguration != null) {
publishers = requestOverrideConfiguration.metricPublishers();
}
if (publishers == null || publishers.isEmpty()) {
publishers = clientConfiguration.option(SdkClientOption.METRIC_PUBLISHERS);
}
if (publishers == null) {
publishers = Collections.emptyList();
}
return publishers;
}
private SdkClientConfiguration updateSdkClientConfiguration(SdkRequest request, SdkClientConfiguration clientConfiguration) {
List plugins = request.overrideConfiguration().map(c -> c.plugins()).orElse(Collections.emptyList());
SdkClientConfiguration.Builder configuration = clientConfiguration.toBuilder();
if (plugins.isEmpty()) {
return configuration.build();
}
RedshiftDataServiceClientConfigurationBuilder serviceConfigBuilder = new RedshiftDataServiceClientConfigurationBuilder(
configuration);
for (SdkPlugin plugin : plugins) {
plugin.configureClient(serviceConfigBuilder);
}
return configuration.build();
}
private HttpResponseHandler createErrorResponseHandler(BaseAwsJsonProtocolFactory protocolFactory,
JsonOperationMetadata operationMetadata) {
return protocolFactory.createErrorResponseHandler(operationMetadata);
}
@Override
public void close() {
clientHandler.close();
}
}