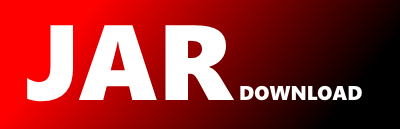
software.amazon.awssdk.services.redshiftdata.model.BatchExecuteStatementRequest Maven / Gradle / Ivy
Show all versions of redshiftdata Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.redshiftdata.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.DefaultValueTrait;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class BatchExecuteStatementRequest extends RedshiftDataRequest implements
ToCopyableBuilder {
private static final SdkField CLIENT_TOKEN_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("ClientToken")
.getter(getter(BatchExecuteStatementRequest::clientToken))
.setter(setter(Builder::clientToken))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ClientToken").build(),
DefaultValueTrait.idempotencyToken()).build();
private static final SdkField CLUSTER_IDENTIFIER_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ClusterIdentifier").getter(getter(BatchExecuteStatementRequest::clusterIdentifier))
.setter(setter(Builder::clusterIdentifier))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ClusterIdentifier").build()).build();
private static final SdkField DATABASE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Database").getter(getter(BatchExecuteStatementRequest::database)).setter(setter(Builder::database))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Database").build()).build();
private static final SdkField DB_USER_FIELD = SdkField. builder(MarshallingType.STRING).memberName("DbUser")
.getter(getter(BatchExecuteStatementRequest::dbUser)).setter(setter(Builder::dbUser))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DbUser").build()).build();
private static final SdkField SECRET_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SecretArn").getter(getter(BatchExecuteStatementRequest::secretArn)).setter(setter(Builder::secretArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SecretArn").build()).build();
private static final SdkField> SQLS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Sqls")
.getter(getter(BatchExecuteStatementRequest::sqls))
.setter(setter(Builder::sqls))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Sqls").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField STATEMENT_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("StatementName").getter(getter(BatchExecuteStatementRequest::statementName))
.setter(setter(Builder::statementName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StatementName").build()).build();
private static final SdkField WITH_EVENT_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("WithEvent").getter(getter(BatchExecuteStatementRequest::withEvent)).setter(setter(Builder::withEvent))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("WithEvent").build()).build();
private static final SdkField WORKGROUP_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("WorkgroupName").getter(getter(BatchExecuteStatementRequest::workgroupName))
.setter(setter(Builder::workgroupName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("WorkgroupName").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(CLIENT_TOKEN_FIELD,
CLUSTER_IDENTIFIER_FIELD, DATABASE_FIELD, DB_USER_FIELD, SECRET_ARN_FIELD, SQLS_FIELD, STATEMENT_NAME_FIELD,
WITH_EVENT_FIELD, WORKGROUP_NAME_FIELD));
private final String clientToken;
private final String clusterIdentifier;
private final String database;
private final String dbUser;
private final String secretArn;
private final List sqls;
private final String statementName;
private final Boolean withEvent;
private final String workgroupName;
private BatchExecuteStatementRequest(BuilderImpl builder) {
super(builder);
this.clientToken = builder.clientToken;
this.clusterIdentifier = builder.clusterIdentifier;
this.database = builder.database;
this.dbUser = builder.dbUser;
this.secretArn = builder.secretArn;
this.sqls = builder.sqls;
this.statementName = builder.statementName;
this.withEvent = builder.withEvent;
this.workgroupName = builder.workgroupName;
}
/**
*
* A unique, case-sensitive identifier that you provide to ensure the idempotency of the request.
*
*
* @return A unique, case-sensitive identifier that you provide to ensure the idempotency of the request.
*/
public final String clientToken() {
return clientToken;
}
/**
*
* The cluster identifier. This parameter is required when connecting to a cluster and authenticating using either
* Secrets Manager or temporary credentials.
*
*
* @return The cluster identifier. This parameter is required when connecting to a cluster and authenticating using
* either Secrets Manager or temporary credentials.
*/
public final String clusterIdentifier() {
return clusterIdentifier;
}
/**
*
* The name of the database. This parameter is required when authenticating using either Secrets Manager or
* temporary credentials.
*
*
* @return The name of the database. This parameter is required when authenticating using either Secrets Manager or
* temporary credentials.
*/
public final String database() {
return database;
}
/**
*
* The database user name. This parameter is required when connecting to a cluster as a database user and
* authenticating using temporary credentials.
*
*
* @return The database user name. This parameter is required when connecting to a cluster as a database user and
* authenticating using temporary credentials.
*/
public final String dbUser() {
return dbUser;
}
/**
*
* The name or ARN of the secret that enables access to the database. This parameter is required when authenticating
* using Secrets Manager.
*
*
* @return The name or ARN of the secret that enables access to the database. This parameter is required when
* authenticating using Secrets Manager.
*/
public final String secretArn() {
return secretArn;
}
/**
* For responses, this returns true if the service returned a value for the Sqls property. This DOES NOT check that
* the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is useful
* because the SDK will never return a null collection or map, but you may need to differentiate between the service
* returning nothing (or null) and the service returning an empty collection or map. For requests, this returns true
* if a value for the property was specified in the request builder, and false if a value was not specified.
*/
public final boolean hasSqls() {
return sqls != null && !(sqls instanceof SdkAutoConstructList);
}
/**
*
* One or more SQL statements to run.
*
*
* The SQL statements are run as a single transaction. They run serially in the order of the array. Subsequent SQL statements don't start until the previous statement in the array completes. If any SQL statement fails, then because they are run as one transaction, all work is rolled back.</p>
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasSqls} method.
*
*
* @return One or more SQL statements to run.
*
* The SQL statements are run as a single transaction. They run serially in the order of the array. Subsequent SQL statements don't start until the previous statement in the array completes. If any SQL statement fails, then because they are run as one transaction, all work is rolled back.</p>
*/
public final List sqls() {
return sqls;
}
/**
*
* The name of the SQL statements. You can name the SQL statements when you create them to identify the query.
*
*
* @return The name of the SQL statements. You can name the SQL statements when you create them to identify the
* query.
*/
public final String statementName() {
return statementName;
}
/**
*
* A value that indicates whether to send an event to the Amazon EventBridge event bus after the SQL statements run.
*
*
* @return A value that indicates whether to send an event to the Amazon EventBridge event bus after the SQL
* statements run.
*/
public final Boolean withEvent() {
return withEvent;
}
/**
*
* The serverless workgroup name or Amazon Resource Name (ARN). This parameter is required when connecting to a
* serverless workgroup and authenticating using either Secrets Manager or temporary credentials.
*
*
* @return The serverless workgroup name or Amazon Resource Name (ARN). This parameter is required when connecting
* to a serverless workgroup and authenticating using either Secrets Manager or temporary credentials.
*/
public final String workgroupName() {
return workgroupName;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(clientToken());
hashCode = 31 * hashCode + Objects.hashCode(clusterIdentifier());
hashCode = 31 * hashCode + Objects.hashCode(database());
hashCode = 31 * hashCode + Objects.hashCode(dbUser());
hashCode = 31 * hashCode + Objects.hashCode(secretArn());
hashCode = 31 * hashCode + Objects.hashCode(hasSqls() ? sqls() : null);
hashCode = 31 * hashCode + Objects.hashCode(statementName());
hashCode = 31 * hashCode + Objects.hashCode(withEvent());
hashCode = 31 * hashCode + Objects.hashCode(workgroupName());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof BatchExecuteStatementRequest)) {
return false;
}
BatchExecuteStatementRequest other = (BatchExecuteStatementRequest) obj;
return Objects.equals(clientToken(), other.clientToken())
&& Objects.equals(clusterIdentifier(), other.clusterIdentifier()) && Objects.equals(database(), other.database())
&& Objects.equals(dbUser(), other.dbUser()) && Objects.equals(secretArn(), other.secretArn())
&& hasSqls() == other.hasSqls() && Objects.equals(sqls(), other.sqls())
&& Objects.equals(statementName(), other.statementName()) && Objects.equals(withEvent(), other.withEvent())
&& Objects.equals(workgroupName(), other.workgroupName());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("BatchExecuteStatementRequest").add("ClientToken", clientToken())
.add("ClusterIdentifier", clusterIdentifier()).add("Database", database()).add("DbUser", dbUser())
.add("SecretArn", secretArn()).add("Sqls", hasSqls() ? sqls() : null).add("StatementName", statementName())
.add("WithEvent", withEvent()).add("WorkgroupName", workgroupName()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ClientToken":
return Optional.ofNullable(clazz.cast(clientToken()));
case "ClusterIdentifier":
return Optional.ofNullable(clazz.cast(clusterIdentifier()));
case "Database":
return Optional.ofNullable(clazz.cast(database()));
case "DbUser":
return Optional.ofNullable(clazz.cast(dbUser()));
case "SecretArn":
return Optional.ofNullable(clazz.cast(secretArn()));
case "Sqls":
return Optional.ofNullable(clazz.cast(sqls()));
case "StatementName":
return Optional.ofNullable(clazz.cast(statementName()));
case "WithEvent":
return Optional.ofNullable(clazz.cast(withEvent()));
case "WorkgroupName":
return Optional.ofNullable(clazz.cast(workgroupName()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function