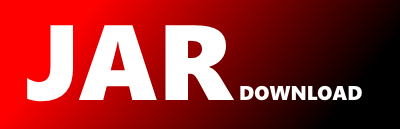
software.amazon.awssdk.services.redshiftdata.model.BatchExecuteStatementResponse Maven / Gradle / Ivy
Show all versions of redshiftdata Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.redshiftdata.model;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class BatchExecuteStatementResponse extends RedshiftDataResponse implements
ToCopyableBuilder {
private static final SdkField CLUSTER_IDENTIFIER_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ClusterIdentifier").getter(getter(BatchExecuteStatementResponse::clusterIdentifier))
.setter(setter(Builder::clusterIdentifier))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ClusterIdentifier").build()).build();
private static final SdkField CREATED_AT_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("CreatedAt").getter(getter(BatchExecuteStatementResponse::createdAt)).setter(setter(Builder::createdAt))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CreatedAt").build()).build();
private static final SdkField DATABASE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Database").getter(getter(BatchExecuteStatementResponse::database)).setter(setter(Builder::database))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Database").build()).build();
private static final SdkField> DB_GROUPS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("DbGroups")
.getter(getter(BatchExecuteStatementResponse::dbGroups))
.setter(setter(Builder::dbGroups))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DbGroups").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField DB_USER_FIELD = SdkField. builder(MarshallingType.STRING).memberName("DbUser")
.getter(getter(BatchExecuteStatementResponse::dbUser)).setter(setter(Builder::dbUser))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DbUser").build()).build();
private static final SdkField ID_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Id")
.getter(getter(BatchExecuteStatementResponse::id)).setter(setter(Builder::id))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Id").build()).build();
private static final SdkField SECRET_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SecretArn").getter(getter(BatchExecuteStatementResponse::secretArn)).setter(setter(Builder::secretArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SecretArn").build()).build();
private static final SdkField SESSION_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SessionId").getter(getter(BatchExecuteStatementResponse::sessionId)).setter(setter(Builder::sessionId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SessionId").build()).build();
private static final SdkField WORKGROUP_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("WorkgroupName").getter(getter(BatchExecuteStatementResponse::workgroupName))
.setter(setter(Builder::workgroupName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("WorkgroupName").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(CLUSTER_IDENTIFIER_FIELD,
CREATED_AT_FIELD, DATABASE_FIELD, DB_GROUPS_FIELD, DB_USER_FIELD, ID_FIELD, SECRET_ARN_FIELD, SESSION_ID_FIELD,
WORKGROUP_NAME_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private final String clusterIdentifier;
private final Instant createdAt;
private final String database;
private final List dbGroups;
private final String dbUser;
private final String id;
private final String secretArn;
private final String sessionId;
private final String workgroupName;
private BatchExecuteStatementResponse(BuilderImpl builder) {
super(builder);
this.clusterIdentifier = builder.clusterIdentifier;
this.createdAt = builder.createdAt;
this.database = builder.database;
this.dbGroups = builder.dbGroups;
this.dbUser = builder.dbUser;
this.id = builder.id;
this.secretArn = builder.secretArn;
this.sessionId = builder.sessionId;
this.workgroupName = builder.workgroupName;
}
/**
*
* The cluster identifier. This element is not returned when connecting to a serverless workgroup.
*
*
* @return The cluster identifier. This element is not returned when connecting to a serverless workgroup.
*/
public final String clusterIdentifier() {
return clusterIdentifier;
}
/**
*
* The date and time (UTC) the statement was created.
*
*
* @return The date and time (UTC) the statement was created.
*/
public final Instant createdAt() {
return createdAt;
}
/**
*
* The name of the database.
*
*
* @return The name of the database.
*/
public final String database() {
return database;
}
/**
* For responses, this returns true if the service returned a value for the DbGroups property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasDbGroups() {
return dbGroups != null && !(dbGroups instanceof SdkAutoConstructList);
}
/**
*
* A list of colon (:) separated names of database groups.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasDbGroups} method.
*
*
* @return A list of colon (:) separated names of database groups.
*/
public final List dbGroups() {
return dbGroups;
}
/**
*
* The database user name.
*
*
* @return The database user name.
*/
public final String dbUser() {
return dbUser;
}
/**
*
* The identifier of the SQL statement whose results are to be fetched. This value is a universally unique
* identifier (UUID) generated by Amazon Redshift Data API. This identifier is returned by
* BatchExecuteStatment
.
*
*
* @return The identifier of the SQL statement whose results are to be fetched. This value is a universally unique
* identifier (UUID) generated by Amazon Redshift Data API. This identifier is returned by
* BatchExecuteStatment
.
*/
public final String id() {
return id;
}
/**
*
* The name or ARN of the secret that enables access to the database.
*
*
* @return The name or ARN of the secret that enables access to the database.
*/
public final String secretArn() {
return secretArn;
}
/**
*
* The session identifier of the query.
*
*
* @return The session identifier of the query.
*/
public final String sessionId() {
return sessionId;
}
/**
*
* The serverless workgroup name or Amazon Resource Name (ARN). This element is not returned when connecting to a
* provisioned cluster.
*
*
* @return The serverless workgroup name or Amazon Resource Name (ARN). This element is not returned when connecting
* to a provisioned cluster.
*/
public final String workgroupName() {
return workgroupName;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(clusterIdentifier());
hashCode = 31 * hashCode + Objects.hashCode(createdAt());
hashCode = 31 * hashCode + Objects.hashCode(database());
hashCode = 31 * hashCode + Objects.hashCode(hasDbGroups() ? dbGroups() : null);
hashCode = 31 * hashCode + Objects.hashCode(dbUser());
hashCode = 31 * hashCode + Objects.hashCode(id());
hashCode = 31 * hashCode + Objects.hashCode(secretArn());
hashCode = 31 * hashCode + Objects.hashCode(sessionId());
hashCode = 31 * hashCode + Objects.hashCode(workgroupName());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof BatchExecuteStatementResponse)) {
return false;
}
BatchExecuteStatementResponse other = (BatchExecuteStatementResponse) obj;
return Objects.equals(clusterIdentifier(), other.clusterIdentifier()) && Objects.equals(createdAt(), other.createdAt())
&& Objects.equals(database(), other.database()) && hasDbGroups() == other.hasDbGroups()
&& Objects.equals(dbGroups(), other.dbGroups()) && Objects.equals(dbUser(), other.dbUser())
&& Objects.equals(id(), other.id()) && Objects.equals(secretArn(), other.secretArn())
&& Objects.equals(sessionId(), other.sessionId()) && Objects.equals(workgroupName(), other.workgroupName());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("BatchExecuteStatementResponse").add("ClusterIdentifier", clusterIdentifier())
.add("CreatedAt", createdAt()).add("Database", database()).add("DbGroups", hasDbGroups() ? dbGroups() : null)
.add("DbUser", dbUser()).add("Id", id()).add("SecretArn", secretArn()).add("SessionId", sessionId())
.add("WorkgroupName", workgroupName()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ClusterIdentifier":
return Optional.ofNullable(clazz.cast(clusterIdentifier()));
case "CreatedAt":
return Optional.ofNullable(clazz.cast(createdAt()));
case "Database":
return Optional.ofNullable(clazz.cast(database()));
case "DbGroups":
return Optional.ofNullable(clazz.cast(dbGroups()));
case "DbUser":
return Optional.ofNullable(clazz.cast(dbUser()));
case "Id":
return Optional.ofNullable(clazz.cast(id()));
case "SecretArn":
return Optional.ofNullable(clazz.cast(secretArn()));
case "SessionId":
return Optional.ofNullable(clazz.cast(sessionId()));
case "WorkgroupName":
return Optional.ofNullable(clazz.cast(workgroupName()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("ClusterIdentifier", CLUSTER_IDENTIFIER_FIELD);
map.put("CreatedAt", CREATED_AT_FIELD);
map.put("Database", DATABASE_FIELD);
map.put("DbGroups", DB_GROUPS_FIELD);
map.put("DbUser", DB_USER_FIELD);
map.put("Id", ID_FIELD);
map.put("SecretArn", SECRET_ARN_FIELD);
map.put("SessionId", SESSION_ID_FIELD);
map.put("WorkgroupName", WORKGROUP_NAME_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function