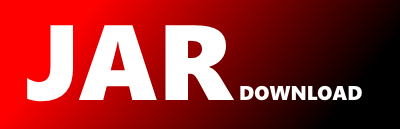
software.amazon.awssdk.services.redshiftserverless.model.UpdateNamespaceRequest Maven / Gradle / Ivy
Show all versions of redshiftserverless Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.redshiftserverless.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class UpdateNamespaceRequest extends RedshiftServerlessRequest implements
ToCopyableBuilder {
private static final SdkField ADMIN_PASSWORD_SECRET_KMS_KEY_ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("adminPasswordSecretKmsKeyId")
.getter(getter(UpdateNamespaceRequest::adminPasswordSecretKmsKeyId))
.setter(setter(Builder::adminPasswordSecretKmsKeyId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("adminPasswordSecretKmsKeyId")
.build()).build();
private static final SdkField ADMIN_USER_PASSWORD_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("adminUserPassword").getter(getter(UpdateNamespaceRequest::adminUserPassword))
.setter(setter(Builder::adminUserPassword))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("adminUserPassword").build()).build();
private static final SdkField ADMIN_USERNAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("adminUsername").getter(getter(UpdateNamespaceRequest::adminUsername))
.setter(setter(Builder::adminUsername))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("adminUsername").build()).build();
private static final SdkField DEFAULT_IAM_ROLE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("defaultIamRoleArn").getter(getter(UpdateNamespaceRequest::defaultIamRoleArn))
.setter(setter(Builder::defaultIamRoleArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("defaultIamRoleArn").build()).build();
private static final SdkField> IAM_ROLES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("iamRoles")
.getter(getter(UpdateNamespaceRequest::iamRoles))
.setter(setter(Builder::iamRoles))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("iamRoles").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField KMS_KEY_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("kmsKeyId").getter(getter(UpdateNamespaceRequest::kmsKeyId)).setter(setter(Builder::kmsKeyId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("kmsKeyId").build()).build();
private static final SdkField> LOG_EXPORTS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("logExports")
.getter(getter(UpdateNamespaceRequest::logExportsAsStrings))
.setter(setter(Builder::logExportsWithStrings))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("logExports").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField MANAGE_ADMIN_PASSWORD_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("manageAdminPassword").getter(getter(UpdateNamespaceRequest::manageAdminPassword))
.setter(setter(Builder::manageAdminPassword))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("manageAdminPassword").build())
.build();
private static final SdkField NAMESPACE_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("namespaceName").getter(getter(UpdateNamespaceRequest::namespaceName))
.setter(setter(Builder::namespaceName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("namespaceName").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(
ADMIN_PASSWORD_SECRET_KMS_KEY_ID_FIELD, ADMIN_USER_PASSWORD_FIELD, ADMIN_USERNAME_FIELD, DEFAULT_IAM_ROLE_ARN_FIELD,
IAM_ROLES_FIELD, KMS_KEY_ID_FIELD, LOG_EXPORTS_FIELD, MANAGE_ADMIN_PASSWORD_FIELD, NAMESPACE_NAME_FIELD));
private final String adminPasswordSecretKmsKeyId;
private final String adminUserPassword;
private final String adminUsername;
private final String defaultIamRoleArn;
private final List iamRoles;
private final String kmsKeyId;
private final List logExports;
private final Boolean manageAdminPassword;
private final String namespaceName;
private UpdateNamespaceRequest(BuilderImpl builder) {
super(builder);
this.adminPasswordSecretKmsKeyId = builder.adminPasswordSecretKmsKeyId;
this.adminUserPassword = builder.adminUserPassword;
this.adminUsername = builder.adminUsername;
this.defaultIamRoleArn = builder.defaultIamRoleArn;
this.iamRoles = builder.iamRoles;
this.kmsKeyId = builder.kmsKeyId;
this.logExports = builder.logExports;
this.manageAdminPassword = builder.manageAdminPassword;
this.namespaceName = builder.namespaceName;
}
/**
*
* The ID of the Key Management Service (KMS) key used to encrypt and store the namespace's admin credentials
* secret. You can only use this parameter if manageAdminPassword
is true.
*
*
* @return The ID of the Key Management Service (KMS) key used to encrypt and store the namespace's admin
* credentials secret. You can only use this parameter if manageAdminPassword
is true.
*/
public final String adminPasswordSecretKmsKeyId() {
return adminPasswordSecretKmsKeyId;
}
/**
*
* The password of the administrator for the first database created in the namespace. This parameter must be updated
* together with adminUsername
.
*
*
* You can't use adminUserPassword
if manageAdminPassword
is true.
*
*
* @return The password of the administrator for the first database created in the namespace. This parameter must be
* updated together with adminUsername
.
*
* You can't use adminUserPassword
if manageAdminPassword
is true.
*/
public final String adminUserPassword() {
return adminUserPassword;
}
/**
*
* The username of the administrator for the first database created in the namespace. This parameter must be updated
* together with adminUserPassword
.
*
*
* @return The username of the administrator for the first database created in the namespace. This parameter must be
* updated together with adminUserPassword
.
*/
public final String adminUsername() {
return adminUsername;
}
/**
*
* The Amazon Resource Name (ARN) of the IAM role to set as a default in the namespace. This parameter must be
* updated together with iamRoles
.
*
*
* @return The Amazon Resource Name (ARN) of the IAM role to set as a default in the namespace. This parameter must
* be updated together with iamRoles
.
*/
public final String defaultIamRoleArn() {
return defaultIamRoleArn;
}
/**
* For responses, this returns true if the service returned a value for the IamRoles property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasIamRoles() {
return iamRoles != null && !(iamRoles instanceof SdkAutoConstructList);
}
/**
*
* A list of IAM roles to associate with the namespace. This parameter must be updated together with
* defaultIamRoleArn
.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasIamRoles} method.
*
*
* @return A list of IAM roles to associate with the namespace. This parameter must be updated together with
* defaultIamRoleArn
.
*/
public final List iamRoles() {
return iamRoles;
}
/**
*
* The ID of the Amazon Web Services Key Management Service key used to encrypt your data.
*
*
* @return The ID of the Amazon Web Services Key Management Service key used to encrypt your data.
*/
public final String kmsKeyId() {
return kmsKeyId;
}
/**
*
* The types of logs the namespace can export. The export types are userlog
, connectionlog
* , and useractivitylog
.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasLogExports} method.
*
*
* @return The types of logs the namespace can export. The export types are userlog
,
* connectionlog
, and useractivitylog
.
*/
public final List logExports() {
return LogExportListCopier.copyStringToEnum(logExports);
}
/**
* For responses, this returns true if the service returned a value for the LogExports property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasLogExports() {
return logExports != null && !(logExports instanceof SdkAutoConstructList);
}
/**
*
* The types of logs the namespace can export. The export types are userlog
, connectionlog
* , and useractivitylog
.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasLogExports} method.
*
*
* @return The types of logs the namespace can export. The export types are userlog
,
* connectionlog
, and useractivitylog
.
*/
public final List logExportsAsStrings() {
return logExports;
}
/**
*
* If true
, Amazon Redshift uses Secrets Manager to manage the namespace's admin credentials. You can't
* use adminUserPassword
if manageAdminPassword
is true. If
* manageAdminPassword
is false or not set, Amazon Redshift uses adminUserPassword
for the
* admin user account's password.
*
*
* @return If true
, Amazon Redshift uses Secrets Manager to manage the namespace's admin credentials.
* You can't use adminUserPassword
if manageAdminPassword
is true. If
* manageAdminPassword
is false or not set, Amazon Redshift uses adminUserPassword
* for the admin user account's password.
*/
public final Boolean manageAdminPassword() {
return manageAdminPassword;
}
/**
*
* The name of the namespace to update. You can't update the name of a namespace once it is created.
*
*
* @return The name of the namespace to update. You can't update the name of a namespace once it is created.
*/
public final String namespaceName() {
return namespaceName;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(adminPasswordSecretKmsKeyId());
hashCode = 31 * hashCode + Objects.hashCode(adminUserPassword());
hashCode = 31 * hashCode + Objects.hashCode(adminUsername());
hashCode = 31 * hashCode + Objects.hashCode(defaultIamRoleArn());
hashCode = 31 * hashCode + Objects.hashCode(hasIamRoles() ? iamRoles() : null);
hashCode = 31 * hashCode + Objects.hashCode(kmsKeyId());
hashCode = 31 * hashCode + Objects.hashCode(hasLogExports() ? logExportsAsStrings() : null);
hashCode = 31 * hashCode + Objects.hashCode(manageAdminPassword());
hashCode = 31 * hashCode + Objects.hashCode(namespaceName());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof UpdateNamespaceRequest)) {
return false;
}
UpdateNamespaceRequest other = (UpdateNamespaceRequest) obj;
return Objects.equals(adminPasswordSecretKmsKeyId(), other.adminPasswordSecretKmsKeyId())
&& Objects.equals(adminUserPassword(), other.adminUserPassword())
&& Objects.equals(adminUsername(), other.adminUsername())
&& Objects.equals(defaultIamRoleArn(), other.defaultIamRoleArn()) && hasIamRoles() == other.hasIamRoles()
&& Objects.equals(iamRoles(), other.iamRoles()) && Objects.equals(kmsKeyId(), other.kmsKeyId())
&& hasLogExports() == other.hasLogExports() && Objects.equals(logExportsAsStrings(), other.logExportsAsStrings())
&& Objects.equals(manageAdminPassword(), other.manageAdminPassword())
&& Objects.equals(namespaceName(), other.namespaceName());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("UpdateNamespaceRequest").add("AdminPasswordSecretKmsKeyId", adminPasswordSecretKmsKeyId())
.add("AdminUserPassword", adminUserPassword() == null ? null : "*** Sensitive Data Redacted ***")
.add("AdminUsername", adminUsername() == null ? null : "*** Sensitive Data Redacted ***")
.add("DefaultIamRoleArn", defaultIamRoleArn()).add("IamRoles", hasIamRoles() ? iamRoles() : null)
.add("KmsKeyId", kmsKeyId()).add("LogExports", hasLogExports() ? logExportsAsStrings() : null)
.add("ManageAdminPassword", manageAdminPassword()).add("NamespaceName", namespaceName()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "adminPasswordSecretKmsKeyId":
return Optional.ofNullable(clazz.cast(adminPasswordSecretKmsKeyId()));
case "adminUserPassword":
return Optional.ofNullable(clazz.cast(adminUserPassword()));
case "adminUsername":
return Optional.ofNullable(clazz.cast(adminUsername()));
case "defaultIamRoleArn":
return Optional.ofNullable(clazz.cast(defaultIamRoleArn()));
case "iamRoles":
return Optional.ofNullable(clazz.cast(iamRoles()));
case "kmsKeyId":
return Optional.ofNullable(clazz.cast(kmsKeyId()));
case "logExports":
return Optional.ofNullable(clazz.cast(logExportsAsStrings()));
case "manageAdminPassword":
return Optional.ofNullable(clazz.cast(manageAdminPassword()));
case "namespaceName":
return Optional.ofNullable(clazz.cast(namespaceName()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function