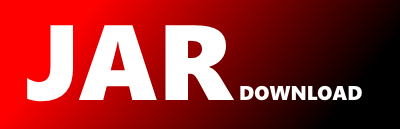
software.amazon.awssdk.services.resourcegroups.DefaultResourceGroupsAsyncClient Maven / Gradle / Ivy
/*
* Copyright 2013-2018 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.resourcegroups;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.awscore.client.handler.AwsAsyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.awscore.internal.protocol.json.AwsJsonProtocol;
import software.amazon.awssdk.awscore.protocol.json.AwsJsonProtocolFactory;
import software.amazon.awssdk.awscore.protocol.json.AwsJsonProtocolMetadata;
import software.amazon.awssdk.core.ApiName;
import software.amazon.awssdk.core.client.handler.AsyncClientHandler;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.internal.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.protocol.json.JsonClientMetadata;
import software.amazon.awssdk.core.protocol.json.JsonErrorResponseMetadata;
import software.amazon.awssdk.core.protocol.json.JsonErrorShapeMetadata;
import software.amazon.awssdk.core.protocol.json.JsonOperationMetadata;
import software.amazon.awssdk.core.util.VersionInfo;
import software.amazon.awssdk.services.resourcegroups.model.BadRequestException;
import software.amazon.awssdk.services.resourcegroups.model.CreateGroupRequest;
import software.amazon.awssdk.services.resourcegroups.model.CreateGroupResponse;
import software.amazon.awssdk.services.resourcegroups.model.DeleteGroupRequest;
import software.amazon.awssdk.services.resourcegroups.model.DeleteGroupResponse;
import software.amazon.awssdk.services.resourcegroups.model.ForbiddenException;
import software.amazon.awssdk.services.resourcegroups.model.GetGroupQueryRequest;
import software.amazon.awssdk.services.resourcegroups.model.GetGroupQueryResponse;
import software.amazon.awssdk.services.resourcegroups.model.GetGroupRequest;
import software.amazon.awssdk.services.resourcegroups.model.GetGroupResponse;
import software.amazon.awssdk.services.resourcegroups.model.GetTagsRequest;
import software.amazon.awssdk.services.resourcegroups.model.GetTagsResponse;
import software.amazon.awssdk.services.resourcegroups.model.InternalServerErrorException;
import software.amazon.awssdk.services.resourcegroups.model.ListGroupResourcesRequest;
import software.amazon.awssdk.services.resourcegroups.model.ListGroupResourcesResponse;
import software.amazon.awssdk.services.resourcegroups.model.ListGroupsRequest;
import software.amazon.awssdk.services.resourcegroups.model.ListGroupsResponse;
import software.amazon.awssdk.services.resourcegroups.model.MethodNotAllowedException;
import software.amazon.awssdk.services.resourcegroups.model.NotFoundException;
import software.amazon.awssdk.services.resourcegroups.model.ResourceGroupsRequest;
import software.amazon.awssdk.services.resourcegroups.model.SearchResourcesRequest;
import software.amazon.awssdk.services.resourcegroups.model.SearchResourcesResponse;
import software.amazon.awssdk.services.resourcegroups.model.TagRequest;
import software.amazon.awssdk.services.resourcegroups.model.TagResponse;
import software.amazon.awssdk.services.resourcegroups.model.TooManyRequestsException;
import software.amazon.awssdk.services.resourcegroups.model.UnauthorizedException;
import software.amazon.awssdk.services.resourcegroups.model.UntagRequest;
import software.amazon.awssdk.services.resourcegroups.model.UntagResponse;
import software.amazon.awssdk.services.resourcegroups.model.UpdateGroupQueryRequest;
import software.amazon.awssdk.services.resourcegroups.model.UpdateGroupQueryResponse;
import software.amazon.awssdk.services.resourcegroups.model.UpdateGroupRequest;
import software.amazon.awssdk.services.resourcegroups.model.UpdateGroupResponse;
import software.amazon.awssdk.services.resourcegroups.paginators.ListGroupResourcesPublisher;
import software.amazon.awssdk.services.resourcegroups.paginators.ListGroupsPublisher;
import software.amazon.awssdk.services.resourcegroups.paginators.SearchResourcesPublisher;
import software.amazon.awssdk.services.resourcegroups.transform.CreateGroupRequestMarshaller;
import software.amazon.awssdk.services.resourcegroups.transform.CreateGroupResponseUnmarshaller;
import software.amazon.awssdk.services.resourcegroups.transform.DeleteGroupRequestMarshaller;
import software.amazon.awssdk.services.resourcegroups.transform.DeleteGroupResponseUnmarshaller;
import software.amazon.awssdk.services.resourcegroups.transform.GetGroupQueryRequestMarshaller;
import software.amazon.awssdk.services.resourcegroups.transform.GetGroupQueryResponseUnmarshaller;
import software.amazon.awssdk.services.resourcegroups.transform.GetGroupRequestMarshaller;
import software.amazon.awssdk.services.resourcegroups.transform.GetGroupResponseUnmarshaller;
import software.amazon.awssdk.services.resourcegroups.transform.GetTagsRequestMarshaller;
import software.amazon.awssdk.services.resourcegroups.transform.GetTagsResponseUnmarshaller;
import software.amazon.awssdk.services.resourcegroups.transform.ListGroupResourcesRequestMarshaller;
import software.amazon.awssdk.services.resourcegroups.transform.ListGroupResourcesResponseUnmarshaller;
import software.amazon.awssdk.services.resourcegroups.transform.ListGroupsRequestMarshaller;
import software.amazon.awssdk.services.resourcegroups.transform.ListGroupsResponseUnmarshaller;
import software.amazon.awssdk.services.resourcegroups.transform.SearchResourcesRequestMarshaller;
import software.amazon.awssdk.services.resourcegroups.transform.SearchResourcesResponseUnmarshaller;
import software.amazon.awssdk.services.resourcegroups.transform.TagRequestMarshaller;
import software.amazon.awssdk.services.resourcegroups.transform.TagResponseUnmarshaller;
import software.amazon.awssdk.services.resourcegroups.transform.UntagRequestMarshaller;
import software.amazon.awssdk.services.resourcegroups.transform.UntagResponseUnmarshaller;
import software.amazon.awssdk.services.resourcegroups.transform.UpdateGroupQueryRequestMarshaller;
import software.amazon.awssdk.services.resourcegroups.transform.UpdateGroupQueryResponseUnmarshaller;
import software.amazon.awssdk.services.resourcegroups.transform.UpdateGroupRequestMarshaller;
import software.amazon.awssdk.services.resourcegroups.transform.UpdateGroupResponseUnmarshaller;
import software.amazon.awssdk.utils.CompletableFutureUtils;
/**
* Internal implementation of {@link ResourceGroupsAsyncClient}.
*
* @see ResourceGroupsAsyncClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultResourceGroupsAsyncClient implements ResourceGroupsAsyncClient {
private final AsyncClientHandler clientHandler;
private final AwsJsonProtocolFactory protocolFactory;
protected DefaultResourceGroupsAsyncClient(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsAsyncClientHandler(clientConfiguration);
this.protocolFactory = init();
}
@Override
public final String serviceName() {
return SERVICE_NAME;
}
/**
*
* Creates a group with a specified name, description, and resource query.
*
*
* @param createGroupRequest
* @return A Java Future containing the result of the CreateGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException The request does not comply with validation rules that are defined for the
* request parameters.
* - ForbiddenException The caller is not authorized to make the request.
* - MethodNotAllowedException The request uses an HTTP method which is not allowed for the specified
* resource.
* - TooManyRequestsException The caller has exceeded throttling limits.
* - InternalServerErrorException An internal error occurred while processing the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.CreateGroup
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture createGroup(CreateGroupRequest createGroupRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateGroupResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withMarshaller(new CreateGroupRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(createGroupRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes a specified resource group. Deleting a resource group does not delete resources that are members of the
* group; it only deletes the group structure.
*
*
* @param deleteGroupRequest
* @return A Java Future containing the result of the DeleteGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException The request does not comply with validation rules that are defined for the
* request parameters.
* - ForbiddenException The caller is not authorized to make the request.
* - NotFoundException One or more resources specified in the request do not exist.
* - MethodNotAllowedException The request uses an HTTP method which is not allowed for the specified
* resource.
* - TooManyRequestsException The caller has exceeded throttling limits.
* - InternalServerErrorException An internal error occurred while processing the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.DeleteGroup
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture deleteGroup(DeleteGroupRequest deleteGroupRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteGroupResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withMarshaller(new DeleteGroupRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(deleteGroupRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns information about a specified resource group.
*
*
* @param getGroupRequest
* @return A Java Future containing the result of the GetGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException The request does not comply with validation rules that are defined for the
* request parameters.
* - ForbiddenException The caller is not authorized to make the request.
* - NotFoundException One or more resources specified in the request do not exist.
* - MethodNotAllowedException The request uses an HTTP method which is not allowed for the specified
* resource.
* - TooManyRequestsException The caller has exceeded throttling limits.
* - InternalServerErrorException An internal error occurred while processing the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.GetGroup
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture getGroup(GetGroupRequest getGroupRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetGroupResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withMarshaller(new GetGroupRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getGroupRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns the resource query associated with the specified resource group.
*
*
* @param getGroupQueryRequest
* @return A Java Future containing the result of the GetGroupQuery operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException The request does not comply with validation rules that are defined for the
* request parameters.
* - ForbiddenException The caller is not authorized to make the request.
* - NotFoundException One or more resources specified in the request do not exist.
* - MethodNotAllowedException The request uses an HTTP method which is not allowed for the specified
* resource.
* - TooManyRequestsException The caller has exceeded throttling limits.
* - InternalServerErrorException An internal error occurred while processing the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.GetGroupQuery
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture getGroupQuery(GetGroupQueryRequest getGroupQueryRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetGroupQueryResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withMarshaller(new GetGroupQueryRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getGroupQueryRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns a list of tags that are associated with a resource, specified by an ARN.
*
*
* @param getTagsRequest
* @return A Java Future containing the result of the GetTags operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException The request does not comply with validation rules that are defined for the
* request parameters.
* - ForbiddenException The caller is not authorized to make the request.
* - NotFoundException One or more resources specified in the request do not exist.
* - MethodNotAllowedException The request uses an HTTP method which is not allowed for the specified
* resource.
* - TooManyRequestsException The caller has exceeded throttling limits.
* - InternalServerErrorException An internal error occurred while processing the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.GetTags
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture getTags(GetTagsRequest getTagsRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetTagsResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withMarshaller(new GetTagsRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getTagsRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns a list of ARNs of resources that are members of a specified resource group.
*
*
* @param listGroupResourcesRequest
* @return A Java Future containing the result of the ListGroupResources operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - UnauthorizedException The request has not been applied because it lacks valid authentication
* credentials for the target resource.
* - BadRequestException The request does not comply with validation rules that are defined for the
* request parameters.
* - ForbiddenException The caller is not authorized to make the request.
* - NotFoundException One or more resources specified in the request do not exist.
* - MethodNotAllowedException The request uses an HTTP method which is not allowed for the specified
* resource.
* - TooManyRequestsException The caller has exceeded throttling limits.
* - InternalServerErrorException An internal error occurred while processing the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.ListGroupResources
* @see AWS API Documentation
*/
@Override
public CompletableFuture listGroupResources(ListGroupResourcesRequest listGroupResourcesRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListGroupResourcesResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withMarshaller(new ListGroupResourcesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(listGroupResourcesRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns a list of ARNs of resources that are members of a specified resource group.
*
*
*
* This is a variant of
* {@link #listGroupResources(software.amazon.awssdk.services.resourcegroups.model.ListGroupResourcesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the forEach helper method
*
*
* {@code
* software.amazon.awssdk.services.resourcegroups.paginators.ListGroupResourcesPublisher publisher = client.listGroupResourcesPaginator(request);
* CompletableFuture future = publisher.forEach(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.resourcegroups.paginators.ListGroupResourcesPublisher publisher = client.listGroupResourcesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.resourcegroups.model.ListGroupResourcesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Note: If you prefer to have control on service calls, use the
* {@link #listGroupResources(software.amazon.awssdk.services.resourcegroups.model.ListGroupResourcesRequest)}
* operation.
*
*
* @param listGroupResourcesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - UnauthorizedException The request has not been applied because it lacks valid authentication
* credentials for the target resource.
* - BadRequestException The request does not comply with validation rules that are defined for the
* request parameters.
* - ForbiddenException The caller is not authorized to make the request.
* - NotFoundException One or more resources specified in the request do not exist.
* - MethodNotAllowedException The request uses an HTTP method which is not allowed for the specified
* resource.
* - TooManyRequestsException The caller has exceeded throttling limits.
* - InternalServerErrorException An internal error occurred while processing the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.ListGroupResources
* @see AWS API Documentation
*/
public ListGroupResourcesPublisher listGroupResourcesPaginator(ListGroupResourcesRequest listGroupResourcesRequest) {
return new ListGroupResourcesPublisher(this, applyPaginatorUserAgent(listGroupResourcesRequest));
}
/**
*
* Returns a list of existing resource groups in your account.
*
*
* @param listGroupsRequest
* @return A Java Future containing the result of the ListGroups operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException The request does not comply with validation rules that are defined for the
* request parameters.
* - ForbiddenException The caller is not authorized to make the request.
* - MethodNotAllowedException The request uses an HTTP method which is not allowed for the specified
* resource.
* - TooManyRequestsException The caller has exceeded throttling limits.
* - InternalServerErrorException An internal error occurred while processing the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.ListGroups
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listGroups(ListGroupsRequest listGroupsRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListGroupsResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withMarshaller(new ListGroupsRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(listGroupsRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns a list of existing resource groups in your account.
*
*
*
* This is a variant of {@link #listGroups(software.amazon.awssdk.services.resourcegroups.model.ListGroupsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the forEach helper method
*
*
* {@code
* software.amazon.awssdk.services.resourcegroups.paginators.ListGroupsPublisher publisher = client.listGroupsPaginator(request);
* CompletableFuture future = publisher.forEach(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.resourcegroups.paginators.ListGroupsPublisher publisher = client.listGroupsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.resourcegroups.model.ListGroupsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Note: If you prefer to have control on service calls, use the
* {@link #listGroups(software.amazon.awssdk.services.resourcegroups.model.ListGroupsRequest)} operation.
*
*
* @param listGroupsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException The request does not comply with validation rules that are defined for the
* request parameters.
* - ForbiddenException The caller is not authorized to make the request.
* - MethodNotAllowedException The request uses an HTTP method which is not allowed for the specified
* resource.
* - TooManyRequestsException The caller has exceeded throttling limits.
* - InternalServerErrorException An internal error occurred while processing the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.ListGroups
* @see AWS API
* Documentation
*/
public ListGroupsPublisher listGroupsPaginator(ListGroupsRequest listGroupsRequest) {
return new ListGroupsPublisher(this, applyPaginatorUserAgent(listGroupsRequest));
}
/**
*
* Returns a list of AWS resource identifiers that matches a specified query. The query uses the same format as a
* resource query in a CreateGroup or UpdateGroupQuery operation.
*
*
* @param searchResourcesRequest
* @return A Java Future containing the result of the SearchResources operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - UnauthorizedException The request has not been applied because it lacks valid authentication
* credentials for the target resource.
* - BadRequestException The request does not comply with validation rules that are defined for the
* request parameters.
* - ForbiddenException The caller is not authorized to make the request.
* - MethodNotAllowedException The request uses an HTTP method which is not allowed for the specified
* resource.
* - TooManyRequestsException The caller has exceeded throttling limits.
* - InternalServerErrorException An internal error occurred while processing the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.SearchResources
* @see AWS API Documentation
*/
@Override
public CompletableFuture searchResources(SearchResourcesRequest searchResourcesRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new SearchResourcesResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withMarshaller(new SearchResourcesRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(searchResourcesRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns a list of AWS resource identifiers that matches a specified query. The query uses the same format as a
* resource query in a CreateGroup or UpdateGroupQuery operation.
*
*
*
* This is a variant of
* {@link #searchResources(software.amazon.awssdk.services.resourcegroups.model.SearchResourcesRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the forEach helper method
*
*
* {@code
* software.amazon.awssdk.services.resourcegroups.paginators.SearchResourcesPublisher publisher = client.searchResourcesPaginator(request);
* CompletableFuture future = publisher.forEach(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.resourcegroups.paginators.SearchResourcesPublisher publisher = client.searchResourcesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.resourcegroups.model.SearchResourcesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Note: If you prefer to have control on service calls, use the
* {@link #searchResources(software.amazon.awssdk.services.resourcegroups.model.SearchResourcesRequest)}
* operation.
*
*
* @param searchResourcesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - UnauthorizedException The request has not been applied because it lacks valid authentication
* credentials for the target resource.
* - BadRequestException The request does not comply with validation rules that are defined for the
* request parameters.
* - ForbiddenException The caller is not authorized to make the request.
* - MethodNotAllowedException The request uses an HTTP method which is not allowed for the specified
* resource.
* - TooManyRequestsException The caller has exceeded throttling limits.
* - InternalServerErrorException An internal error occurred while processing the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.SearchResources
* @see AWS API Documentation
*/
public SearchResourcesPublisher searchResourcesPaginator(SearchResourcesRequest searchResourcesRequest) {
return new SearchResourcesPublisher(this, applyPaginatorUserAgent(searchResourcesRequest));
}
/**
*
* Adds specified tags to a resource with the specified ARN. Existing tags on a resource are not changed if they are
* not specified in the request parameters.
*
*
* @param tagRequest
* @return A Java Future containing the result of the Tag operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException The request does not comply with validation rules that are defined for the
* request parameters.
* - ForbiddenException The caller is not authorized to make the request.
* - NotFoundException One or more resources specified in the request do not exist.
* - MethodNotAllowedException The request uses an HTTP method which is not allowed for the specified
* resource.
* - TooManyRequestsException The caller has exceeded throttling limits.
* - InternalServerErrorException An internal error occurred while processing the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.Tag
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture tag(TagRequest tagRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new TagResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withMarshaller(new TagRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(tagRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes specified tags from a specified resource.
*
*
* @param untagRequest
* @return A Java Future containing the result of the Untag operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException The request does not comply with validation rules that are defined for the
* request parameters.
* - ForbiddenException The caller is not authorized to make the request.
* - NotFoundException One or more resources specified in the request do not exist.
* - MethodNotAllowedException The request uses an HTTP method which is not allowed for the specified
* resource.
* - TooManyRequestsException The caller has exceeded throttling limits.
* - InternalServerErrorException An internal error occurred while processing the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.Untag
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture untag(UntagRequest untagRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new UntagResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withMarshaller(new UntagRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(untagRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Updates an existing group with a new or changed description. You cannot update the name of a resource group.
*
*
* @param updateGroupRequest
* @return A Java Future containing the result of the UpdateGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException The request does not comply with validation rules that are defined for the
* request parameters.
* - ForbiddenException The caller is not authorized to make the request.
* - NotFoundException One or more resources specified in the request do not exist.
* - MethodNotAllowedException The request uses an HTTP method which is not allowed for the specified
* resource.
* - TooManyRequestsException The caller has exceeded throttling limits.
* - InternalServerErrorException An internal error occurred while processing the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.UpdateGroup
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture updateGroup(UpdateGroupRequest updateGroupRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new UpdateGroupResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withMarshaller(new UpdateGroupRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(updateGroupRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Updates the resource query of a group.
*
*
* @param updateGroupQueryRequest
* @return A Java Future containing the result of the UpdateGroupQuery operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException The request does not comply with validation rules that are defined for the
* request parameters.
* - ForbiddenException The caller is not authorized to make the request.
* - NotFoundException One or more resources specified in the request do not exist.
* - MethodNotAllowedException The request uses an HTTP method which is not allowed for the specified
* resource.
* - TooManyRequestsException The caller has exceeded throttling limits.
* - InternalServerErrorException An internal error occurred while processing the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.UpdateGroupQuery
* @see AWS API Documentation
*/
@Override
public CompletableFuture updateGroupQuery(UpdateGroupQueryRequest updateGroupQueryRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new UpdateGroupQueryResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withMarshaller(new UpdateGroupQueryRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(updateGroupQueryRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
@Override
public void close() {
clientHandler.close();
}
private software.amazon.awssdk.awscore.protocol.json.AwsJsonProtocolFactory init() {
return new AwsJsonProtocolFactory(
new JsonClientMetadata()
.withSupportsCbor(false)
.withSupportsIon(false)
.withBaseServiceExceptionClass(
software.amazon.awssdk.services.resourcegroups.model.ResourceGroupsException.class)
.withContentTypeOverride("")
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("NotFoundException").withModeledClass(
NotFoundException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("UnauthorizedException").withModeledClass(
UnauthorizedException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ForbiddenException").withModeledClass(
ForbiddenException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("MethodNotAllowedException").withModeledClass(
MethodNotAllowedException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("TooManyRequestsException").withModeledClass(
TooManyRequestsException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("BadRequestException").withModeledClass(
BadRequestException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InternalServerErrorException").withModeledClass(
InternalServerErrorException.class)), AwsJsonProtocolMetadata.builder()
.protocolVersion("1.1").protocol(AwsJsonProtocol.REST_JSON).build());
}
private T applyPaginatorUserAgent(T request) {
Consumer userAgentApplier = b -> b.addApiName(ApiName.builder()
.version(VersionInfo.SDK_VERSION).name("PAGINATED").build());
AwsRequestOverrideConfiguration overrideConfiguration = request.overrideConfiguration()
.map(c -> c.toBuilder().applyMutation(userAgentApplier).build())
.orElse((AwsRequestOverrideConfiguration.builder().applyMutation(userAgentApplier).build()));
return (T) request.toBuilder().overrideConfiguration(overrideConfiguration).build();
}
private HttpResponseHandler createErrorResponseHandler() {
return protocolFactory.createErrorResponseHandler(new JsonErrorResponseMetadata());
}
}