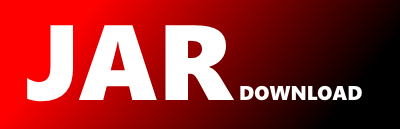
software.amazon.awssdk.services.resourcegroups.ResourceGroupsClient Maven / Gradle / Ivy
Show all versions of resource-groups Show documentation
/*
* Copyright 2013-2018 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.resourcegroups;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.SdkClient;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.regions.ServiceMetadata;
import software.amazon.awssdk.services.resourcegroups.model.BadRequestException;
import software.amazon.awssdk.services.resourcegroups.model.CreateGroupRequest;
import software.amazon.awssdk.services.resourcegroups.model.CreateGroupResponse;
import software.amazon.awssdk.services.resourcegroups.model.DeleteGroupRequest;
import software.amazon.awssdk.services.resourcegroups.model.DeleteGroupResponse;
import software.amazon.awssdk.services.resourcegroups.model.ForbiddenException;
import software.amazon.awssdk.services.resourcegroups.model.GetGroupQueryRequest;
import software.amazon.awssdk.services.resourcegroups.model.GetGroupQueryResponse;
import software.amazon.awssdk.services.resourcegroups.model.GetGroupRequest;
import software.amazon.awssdk.services.resourcegroups.model.GetGroupResponse;
import software.amazon.awssdk.services.resourcegroups.model.GetTagsRequest;
import software.amazon.awssdk.services.resourcegroups.model.GetTagsResponse;
import software.amazon.awssdk.services.resourcegroups.model.InternalServerErrorException;
import software.amazon.awssdk.services.resourcegroups.model.ListGroupResourcesRequest;
import software.amazon.awssdk.services.resourcegroups.model.ListGroupResourcesResponse;
import software.amazon.awssdk.services.resourcegroups.model.ListGroupsRequest;
import software.amazon.awssdk.services.resourcegroups.model.ListGroupsResponse;
import software.amazon.awssdk.services.resourcegroups.model.MethodNotAllowedException;
import software.amazon.awssdk.services.resourcegroups.model.NotFoundException;
import software.amazon.awssdk.services.resourcegroups.model.ResourceGroupsException;
import software.amazon.awssdk.services.resourcegroups.model.SearchResourcesRequest;
import software.amazon.awssdk.services.resourcegroups.model.SearchResourcesResponse;
import software.amazon.awssdk.services.resourcegroups.model.TagRequest;
import software.amazon.awssdk.services.resourcegroups.model.TagResponse;
import software.amazon.awssdk.services.resourcegroups.model.TooManyRequestsException;
import software.amazon.awssdk.services.resourcegroups.model.UnauthorizedException;
import software.amazon.awssdk.services.resourcegroups.model.UntagRequest;
import software.amazon.awssdk.services.resourcegroups.model.UntagResponse;
import software.amazon.awssdk.services.resourcegroups.model.UpdateGroupQueryRequest;
import software.amazon.awssdk.services.resourcegroups.model.UpdateGroupQueryResponse;
import software.amazon.awssdk.services.resourcegroups.model.UpdateGroupRequest;
import software.amazon.awssdk.services.resourcegroups.model.UpdateGroupResponse;
import software.amazon.awssdk.services.resourcegroups.paginators.ListGroupResourcesIterable;
import software.amazon.awssdk.services.resourcegroups.paginators.ListGroupsIterable;
import software.amazon.awssdk.services.resourcegroups.paginators.SearchResourcesIterable;
/**
* Service client for accessing Resource Groups. This can be created using the static {@link #builder()} method.
*
* AWS Resource Groups
*
* AWS Resource Groups lets you organize AWS resources such as Amazon EC2 instances, Amazon Relational Database Service
* databases, and Amazon S3 buckets into groups using criteria that you define as tags. A resource group is a collection
* of resources that match the resource types specified in a query, and share one or more tags or portions of tags. You
* can create a group of resources based on their roles in your cloud infrastructure, lifecycle stages, regions,
* application layers, or virtually any criteria. Resource groups enable you to automate management tasks, such as those
* in AWS Systems Manager Automation documents, on tag-related resources in AWS Systems Manager. Groups of tagged
* resources also let you quickly view a custom console in AWS Systems Manager that shows AWS Config compliance and
* other monitoring data about member resources.
*
*
* To create a resource group, build a resource query, and specify tags that identify the criteria that members of the
* group have in common. Tags are key-value pairs.
*
*
* For more information about Resource Groups, see the AWS Resource Groups User Guide.
*
*
* AWS Resource Groups uses a REST-compliant API that you can use to perform the following types of operations.
*
*
* -
*
* Create, Read, Update, and Delete (CRUD) operations on resource groups and resource query entities
*
*
* -
*
* Applying, editing, and removing tags from resource groups
*
*
* -
*
* Resolving resource group member ARNs so they can be returned as search results
*
*
* -
*
* Getting data about resources that are members of a group
*
*
* -
*
* Searching AWS resources based on a resource query
*
*
*
*/
@Generated("software.amazon.awssdk:codegen")
public interface ResourceGroupsClient extends SdkClient {
String SERVICE_NAME = "resource-groups";
/**
* Create a {@link ResourceGroupsClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static ResourceGroupsClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link ResourceGroupsClient}.
*/
static ResourceGroupsClientBuilder builder() {
return new DefaultResourceGroupsClientBuilder();
}
/**
*
* Creates a group with a specified name, description, and resource query.
*
*
* @param createGroupRequest
* @return Result of the CreateGroup operation returned by the service.
* @throws BadRequestException
* The request does not comply with validation rules that are defined for the request parameters.
* @throws ForbiddenException
* The caller is not authorized to make the request.
* @throws MethodNotAllowedException
* The request uses an HTTP method which is not allowed for the specified resource.
* @throws TooManyRequestsException
* The caller has exceeded throttling limits.
* @throws InternalServerErrorException
* An internal error occurred while processing the request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ResourceGroupsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ResourceGroupsClient.CreateGroup
* @see AWS
* API Documentation
*/
default CreateGroupResponse createGroup(CreateGroupRequest createGroupRequest) throws BadRequestException,
ForbiddenException, MethodNotAllowedException, TooManyRequestsException, InternalServerErrorException,
AwsServiceException, SdkClientException, ResourceGroupsException {
throw new UnsupportedOperationException();
}
/**
*
* Creates a group with a specified name, description, and resource query.
*
*
*
* This is a convenience which creates an instance of the {@link CreateGroupRequest.Builder} avoiding the need to
* create one manually via {@link CreateGroupRequest#builder()}
*
*
* @param createGroupRequest
* A {@link Consumer} that will call methods on {@link CreateGroupInput.Builder} to create a request.
* @return Result of the CreateGroup operation returned by the service.
* @throws BadRequestException
* The request does not comply with validation rules that are defined for the request parameters.
* @throws ForbiddenException
* The caller is not authorized to make the request.
* @throws MethodNotAllowedException
* The request uses an HTTP method which is not allowed for the specified resource.
* @throws TooManyRequestsException
* The caller has exceeded throttling limits.
* @throws InternalServerErrorException
* An internal error occurred while processing the request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ResourceGroupsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ResourceGroupsClient.CreateGroup
* @see AWS
* API Documentation
*/
default CreateGroupResponse createGroup(Consumer createGroupRequest) throws BadRequestException,
ForbiddenException, MethodNotAllowedException, TooManyRequestsException, InternalServerErrorException,
AwsServiceException, SdkClientException, ResourceGroupsException {
return createGroup(CreateGroupRequest.builder().applyMutation(createGroupRequest).build());
}
/**
*
* Deletes a specified resource group. Deleting a resource group does not delete resources that are members of the
* group; it only deletes the group structure.
*
*
* @param deleteGroupRequest
* @return Result of the DeleteGroup operation returned by the service.
* @throws BadRequestException
* The request does not comply with validation rules that are defined for the request parameters.
* @throws ForbiddenException
* The caller is not authorized to make the request.
* @throws NotFoundException
* One or more resources specified in the request do not exist.
* @throws MethodNotAllowedException
* The request uses an HTTP method which is not allowed for the specified resource.
* @throws TooManyRequestsException
* The caller has exceeded throttling limits.
* @throws InternalServerErrorException
* An internal error occurred while processing the request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ResourceGroupsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ResourceGroupsClient.DeleteGroup
* @see AWS
* API Documentation
*/
default DeleteGroupResponse deleteGroup(DeleteGroupRequest deleteGroupRequest) throws BadRequestException,
ForbiddenException, NotFoundException, MethodNotAllowedException, TooManyRequestsException,
InternalServerErrorException, AwsServiceException, SdkClientException, ResourceGroupsException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a specified resource group. Deleting a resource group does not delete resources that are members of the
* group; it only deletes the group structure.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteGroupRequest.Builder} avoiding the need to
* create one manually via {@link DeleteGroupRequest#builder()}
*
*
* @param deleteGroupRequest
* A {@link Consumer} that will call methods on {@link DeleteGroupInput.Builder} to create a request.
* @return Result of the DeleteGroup operation returned by the service.
* @throws BadRequestException
* The request does not comply with validation rules that are defined for the request parameters.
* @throws ForbiddenException
* The caller is not authorized to make the request.
* @throws NotFoundException
* One or more resources specified in the request do not exist.
* @throws MethodNotAllowedException
* The request uses an HTTP method which is not allowed for the specified resource.
* @throws TooManyRequestsException
* The caller has exceeded throttling limits.
* @throws InternalServerErrorException
* An internal error occurred while processing the request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ResourceGroupsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ResourceGroupsClient.DeleteGroup
* @see AWS
* API Documentation
*/
default DeleteGroupResponse deleteGroup(Consumer deleteGroupRequest) throws BadRequestException,
ForbiddenException, NotFoundException, MethodNotAllowedException, TooManyRequestsException,
InternalServerErrorException, AwsServiceException, SdkClientException, ResourceGroupsException {
return deleteGroup(DeleteGroupRequest.builder().applyMutation(deleteGroupRequest).build());
}
/**
*
* Returns information about a specified resource group.
*
*
* @param getGroupRequest
* @return Result of the GetGroup operation returned by the service.
* @throws BadRequestException
* The request does not comply with validation rules that are defined for the request parameters.
* @throws ForbiddenException
* The caller is not authorized to make the request.
* @throws NotFoundException
* One or more resources specified in the request do not exist.
* @throws MethodNotAllowedException
* The request uses an HTTP method which is not allowed for the specified resource.
* @throws TooManyRequestsException
* The caller has exceeded throttling limits.
* @throws InternalServerErrorException
* An internal error occurred while processing the request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ResourceGroupsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ResourceGroupsClient.GetGroup
* @see AWS API
* Documentation
*/
default GetGroupResponse getGroup(GetGroupRequest getGroupRequest) throws BadRequestException, ForbiddenException,
NotFoundException, MethodNotAllowedException, TooManyRequestsException, InternalServerErrorException,
AwsServiceException, SdkClientException, ResourceGroupsException {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about a specified resource group.
*
*
*
* This is a convenience which creates an instance of the {@link GetGroupRequest.Builder} avoiding the need to
* create one manually via {@link GetGroupRequest#builder()}
*
*
* @param getGroupRequest
* A {@link Consumer} that will call methods on {@link GetGroupInput.Builder} to create a request.
* @return Result of the GetGroup operation returned by the service.
* @throws BadRequestException
* The request does not comply with validation rules that are defined for the request parameters.
* @throws ForbiddenException
* The caller is not authorized to make the request.
* @throws NotFoundException
* One or more resources specified in the request do not exist.
* @throws MethodNotAllowedException
* The request uses an HTTP method which is not allowed for the specified resource.
* @throws TooManyRequestsException
* The caller has exceeded throttling limits.
* @throws InternalServerErrorException
* An internal error occurred while processing the request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ResourceGroupsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ResourceGroupsClient.GetGroup
* @see AWS API
* Documentation
*/
default GetGroupResponse getGroup(Consumer getGroupRequest) throws BadRequestException,
ForbiddenException, NotFoundException, MethodNotAllowedException, TooManyRequestsException,
InternalServerErrorException, AwsServiceException, SdkClientException, ResourceGroupsException {
return getGroup(GetGroupRequest.builder().applyMutation(getGroupRequest).build());
}
/**
*
* Returns the resource query associated with the specified resource group.
*
*
* @param getGroupQueryRequest
* @return Result of the GetGroupQuery operation returned by the service.
* @throws BadRequestException
* The request does not comply with validation rules that are defined for the request parameters.
* @throws ForbiddenException
* The caller is not authorized to make the request.
* @throws NotFoundException
* One or more resources specified in the request do not exist.
* @throws MethodNotAllowedException
* The request uses an HTTP method which is not allowed for the specified resource.
* @throws TooManyRequestsException
* The caller has exceeded throttling limits.
* @throws InternalServerErrorException
* An internal error occurred while processing the request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ResourceGroupsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ResourceGroupsClient.GetGroupQuery
* @see AWS
* API Documentation
*/
default GetGroupQueryResponse getGroupQuery(GetGroupQueryRequest getGroupQueryRequest) throws BadRequestException,
ForbiddenException, NotFoundException, MethodNotAllowedException, TooManyRequestsException,
InternalServerErrorException, AwsServiceException, SdkClientException, ResourceGroupsException {
throw new UnsupportedOperationException();
}
/**
*
* Returns the resource query associated with the specified resource group.
*
*
*
* This is a convenience which creates an instance of the {@link GetGroupQueryRequest.Builder} avoiding the need to
* create one manually via {@link GetGroupQueryRequest#builder()}
*
*
* @param getGroupQueryRequest
* A {@link Consumer} that will call methods on {@link GetGroupQueryInput.Builder} to create a request.
* @return Result of the GetGroupQuery operation returned by the service.
* @throws BadRequestException
* The request does not comply with validation rules that are defined for the request parameters.
* @throws ForbiddenException
* The caller is not authorized to make the request.
* @throws NotFoundException
* One or more resources specified in the request do not exist.
* @throws MethodNotAllowedException
* The request uses an HTTP method which is not allowed for the specified resource.
* @throws TooManyRequestsException
* The caller has exceeded throttling limits.
* @throws InternalServerErrorException
* An internal error occurred while processing the request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ResourceGroupsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ResourceGroupsClient.GetGroupQuery
* @see AWS
* API Documentation
*/
default GetGroupQueryResponse getGroupQuery(Consumer getGroupQueryRequest)
throws BadRequestException, ForbiddenException, NotFoundException, MethodNotAllowedException,
TooManyRequestsException, InternalServerErrorException, AwsServiceException, SdkClientException,
ResourceGroupsException {
return getGroupQuery(GetGroupQueryRequest.builder().applyMutation(getGroupQueryRequest).build());
}
/**
*
* Returns a list of tags that are associated with a resource, specified by an ARN.
*
*
* @param getTagsRequest
* @return Result of the GetTags operation returned by the service.
* @throws BadRequestException
* The request does not comply with validation rules that are defined for the request parameters.
* @throws ForbiddenException
* The caller is not authorized to make the request.
* @throws NotFoundException
* One or more resources specified in the request do not exist.
* @throws MethodNotAllowedException
* The request uses an HTTP method which is not allowed for the specified resource.
* @throws TooManyRequestsException
* The caller has exceeded throttling limits.
* @throws InternalServerErrorException
* An internal error occurred while processing the request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ResourceGroupsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ResourceGroupsClient.GetTags
* @see AWS API
* Documentation
*/
default GetTagsResponse getTags(GetTagsRequest getTagsRequest) throws BadRequestException, ForbiddenException,
NotFoundException, MethodNotAllowedException, TooManyRequestsException, InternalServerErrorException,
AwsServiceException, SdkClientException, ResourceGroupsException {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of tags that are associated with a resource, specified by an ARN.
*
*
*
* This is a convenience which creates an instance of the {@link GetTagsRequest.Builder} avoiding the need to create
* one manually via {@link GetTagsRequest#builder()}
*
*
* @param getTagsRequest
* A {@link Consumer} that will call methods on {@link GetTagsInput.Builder} to create a request.
* @return Result of the GetTags operation returned by the service.
* @throws BadRequestException
* The request does not comply with validation rules that are defined for the request parameters.
* @throws ForbiddenException
* The caller is not authorized to make the request.
* @throws NotFoundException
* One or more resources specified in the request do not exist.
* @throws MethodNotAllowedException
* The request uses an HTTP method which is not allowed for the specified resource.
* @throws TooManyRequestsException
* The caller has exceeded throttling limits.
* @throws InternalServerErrorException
* An internal error occurred while processing the request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ResourceGroupsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ResourceGroupsClient.GetTags
* @see AWS API
* Documentation
*/
default GetTagsResponse getTags(Consumer getTagsRequest) throws BadRequestException,
ForbiddenException, NotFoundException, MethodNotAllowedException, TooManyRequestsException,
InternalServerErrorException, AwsServiceException, SdkClientException, ResourceGroupsException {
return getTags(GetTagsRequest.builder().applyMutation(getTagsRequest).build());
}
/**
*
* Returns a list of ARNs of resources that are members of a specified resource group.
*
*
* @param listGroupResourcesRequest
* @return Result of the ListGroupResources operation returned by the service.
* @throws UnauthorizedException
* The request has not been applied because it lacks valid authentication credentials for the target
* resource.
* @throws BadRequestException
* The request does not comply with validation rules that are defined for the request parameters.
* @throws ForbiddenException
* The caller is not authorized to make the request.
* @throws NotFoundException
* One or more resources specified in the request do not exist.
* @throws MethodNotAllowedException
* The request uses an HTTP method which is not allowed for the specified resource.
* @throws TooManyRequestsException
* The caller has exceeded throttling limits.
* @throws InternalServerErrorException
* An internal error occurred while processing the request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ResourceGroupsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ResourceGroupsClient.ListGroupResources
* @see AWS API Documentation
*/
default ListGroupResourcesResponse listGroupResources(ListGroupResourcesRequest listGroupResourcesRequest)
throws UnauthorizedException, BadRequestException, ForbiddenException, NotFoundException, MethodNotAllowedException,
TooManyRequestsException, InternalServerErrorException, AwsServiceException, SdkClientException,
ResourceGroupsException {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of ARNs of resources that are members of a specified resource group.
*
*
*
* This is a convenience which creates an instance of the {@link ListGroupResourcesRequest.Builder} avoiding the
* need to create one manually via {@link ListGroupResourcesRequest#builder()}
*
*
* @param listGroupResourcesRequest
* A {@link Consumer} that will call methods on {@link ListGroupResourcesInput.Builder} to create a request.
* @return Result of the ListGroupResources operation returned by the service.
* @throws UnauthorizedException
* The request has not been applied because it lacks valid authentication credentials for the target
* resource.
* @throws BadRequestException
* The request does not comply with validation rules that are defined for the request parameters.
* @throws ForbiddenException
* The caller is not authorized to make the request.
* @throws NotFoundException
* One or more resources specified in the request do not exist.
* @throws MethodNotAllowedException
* The request uses an HTTP method which is not allowed for the specified resource.
* @throws TooManyRequestsException
* The caller has exceeded throttling limits.
* @throws InternalServerErrorException
* An internal error occurred while processing the request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ResourceGroupsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ResourceGroupsClient.ListGroupResources
* @see AWS API Documentation
*/
default ListGroupResourcesResponse listGroupResources(Consumer listGroupResourcesRequest)
throws UnauthorizedException, BadRequestException, ForbiddenException, NotFoundException, MethodNotAllowedException,
TooManyRequestsException, InternalServerErrorException, AwsServiceException, SdkClientException,
ResourceGroupsException {
return listGroupResources(ListGroupResourcesRequest.builder().applyMutation(listGroupResourcesRequest).build());
}
/**
*
* Returns a list of ARNs of resources that are members of a specified resource group.
*
*
*
* This is a variant of
* {@link #listGroupResources(software.amazon.awssdk.services.resourcegroups.model.ListGroupResourcesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.resourcegroups.paginators.ListGroupResourcesIterable responses = client.listGroupResourcesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.resourcegroups.paginators.ListGroupResourcesIterable responses = client
* .listGroupResourcesPaginator(request);
* for (software.amazon.awssdk.services.resourcegroups.model.ListGroupResourcesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.resourcegroups.paginators.ListGroupResourcesIterable responses = client.listGroupResourcesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listGroupResources(software.amazon.awssdk.services.resourcegroups.model.ListGroupResourcesRequest)}
* operation.
*
*
* @param listGroupResourcesRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws UnauthorizedException
* The request has not been applied because it lacks valid authentication credentials for the target
* resource.
* @throws BadRequestException
* The request does not comply with validation rules that are defined for the request parameters.
* @throws ForbiddenException
* The caller is not authorized to make the request.
* @throws NotFoundException
* One or more resources specified in the request do not exist.
* @throws MethodNotAllowedException
* The request uses an HTTP method which is not allowed for the specified resource.
* @throws TooManyRequestsException
* The caller has exceeded throttling limits.
* @throws InternalServerErrorException
* An internal error occurred while processing the request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ResourceGroupsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ResourceGroupsClient.ListGroupResources
* @see AWS API Documentation
*/
default ListGroupResourcesIterable listGroupResourcesPaginator(ListGroupResourcesRequest listGroupResourcesRequest)
throws UnauthorizedException, BadRequestException, ForbiddenException, NotFoundException, MethodNotAllowedException,
TooManyRequestsException, InternalServerErrorException, AwsServiceException, SdkClientException,
ResourceGroupsException {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of ARNs of resources that are members of a specified resource group.
*
*
*
* This is a variant of
* {@link #listGroupResources(software.amazon.awssdk.services.resourcegroups.model.ListGroupResourcesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.resourcegroups.paginators.ListGroupResourcesIterable responses = client.listGroupResourcesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.resourcegroups.paginators.ListGroupResourcesIterable responses = client
* .listGroupResourcesPaginator(request);
* for (software.amazon.awssdk.services.resourcegroups.model.ListGroupResourcesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.resourcegroups.paginators.ListGroupResourcesIterable responses = client.listGroupResourcesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listGroupResources(software.amazon.awssdk.services.resourcegroups.model.ListGroupResourcesRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListGroupResourcesRequest.Builder} avoiding the
* need to create one manually via {@link ListGroupResourcesRequest#builder()}
*
*
* @param listGroupResourcesRequest
* A {@link Consumer} that will call methods on {@link ListGroupResourcesInput.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws UnauthorizedException
* The request has not been applied because it lacks valid authentication credentials for the target
* resource.
* @throws BadRequestException
* The request does not comply with validation rules that are defined for the request parameters.
* @throws ForbiddenException
* The caller is not authorized to make the request.
* @throws NotFoundException
* One or more resources specified in the request do not exist.
* @throws MethodNotAllowedException
* The request uses an HTTP method which is not allowed for the specified resource.
* @throws TooManyRequestsException
* The caller has exceeded throttling limits.
* @throws InternalServerErrorException
* An internal error occurred while processing the request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ResourceGroupsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ResourceGroupsClient.ListGroupResources
* @see AWS API Documentation
*/
default ListGroupResourcesIterable listGroupResourcesPaginator(
Consumer listGroupResourcesRequest) throws UnauthorizedException,
BadRequestException, ForbiddenException, NotFoundException, MethodNotAllowedException, TooManyRequestsException,
InternalServerErrorException, AwsServiceException, SdkClientException, ResourceGroupsException {
return listGroupResourcesPaginator(ListGroupResourcesRequest.builder().applyMutation(listGroupResourcesRequest).build());
}
/**
*
* Returns a list of existing resource groups in your account.
*
*
* @return Result of the ListGroups operation returned by the service.
* @throws BadRequestException
* The request does not comply with validation rules that are defined for the request parameters.
* @throws ForbiddenException
* The caller is not authorized to make the request.
* @throws MethodNotAllowedException
* The request uses an HTTP method which is not allowed for the specified resource.
* @throws TooManyRequestsException
* The caller has exceeded throttling limits.
* @throws InternalServerErrorException
* An internal error occurred while processing the request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ResourceGroupsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ResourceGroupsClient.ListGroups
* @see #listGroups(ListGroupsRequest)
* @see AWS API
* Documentation
*/
default ListGroupsResponse listGroups() throws BadRequestException, ForbiddenException, MethodNotAllowedException,
TooManyRequestsException, InternalServerErrorException, AwsServiceException, SdkClientException,
ResourceGroupsException {
return listGroups(ListGroupsRequest.builder().build());
}
/**
*
* Returns a list of existing resource groups in your account.
*
*
* @param listGroupsRequest
* @return Result of the ListGroups operation returned by the service.
* @throws BadRequestException
* The request does not comply with validation rules that are defined for the request parameters.
* @throws ForbiddenException
* The caller is not authorized to make the request.
* @throws MethodNotAllowedException
* The request uses an HTTP method which is not allowed for the specified resource.
* @throws TooManyRequestsException
* The caller has exceeded throttling limits.
* @throws InternalServerErrorException
* An internal error occurred while processing the request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ResourceGroupsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ResourceGroupsClient.ListGroups
* @see AWS API
* Documentation
*/
default ListGroupsResponse listGroups(ListGroupsRequest listGroupsRequest) throws BadRequestException, ForbiddenException,
MethodNotAllowedException, TooManyRequestsException, InternalServerErrorException, AwsServiceException,
SdkClientException, ResourceGroupsException {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of existing resource groups in your account.
*
*
*
* This is a convenience which creates an instance of the {@link ListGroupsRequest.Builder} avoiding the need to
* create one manually via {@link ListGroupsRequest#builder()}
*
*
* @param listGroupsRequest
* A {@link Consumer} that will call methods on {@link ListGroupsInput.Builder} to create a request.
* @return Result of the ListGroups operation returned by the service.
* @throws BadRequestException
* The request does not comply with validation rules that are defined for the request parameters.
* @throws ForbiddenException
* The caller is not authorized to make the request.
* @throws MethodNotAllowedException
* The request uses an HTTP method which is not allowed for the specified resource.
* @throws TooManyRequestsException
* The caller has exceeded throttling limits.
* @throws InternalServerErrorException
* An internal error occurred while processing the request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ResourceGroupsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ResourceGroupsClient.ListGroups
* @see AWS API
* Documentation
*/
default ListGroupsResponse listGroups(Consumer listGroupsRequest) throws BadRequestException,
ForbiddenException, MethodNotAllowedException, TooManyRequestsException, InternalServerErrorException,
AwsServiceException, SdkClientException, ResourceGroupsException {
return listGroups(ListGroupsRequest.builder().applyMutation(listGroupsRequest).build());
}
/**
*
* Returns a list of existing resource groups in your account.
*
*
*
* This is a variant of {@link #listGroups(software.amazon.awssdk.services.resourcegroups.model.ListGroupsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.resourcegroups.paginators.ListGroupsIterable responses = client.listGroupsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.resourcegroups.paginators.ListGroupsIterable responses = client.listGroupsPaginator(request);
* for (software.amazon.awssdk.services.resourcegroups.model.ListGroupsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.resourcegroups.paginators.ListGroupsIterable responses = client.listGroupsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listGroups(software.amazon.awssdk.services.resourcegroups.model.ListGroupsRequest)} operation.
*
*
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws BadRequestException
* The request does not comply with validation rules that are defined for the request parameters.
* @throws ForbiddenException
* The caller is not authorized to make the request.
* @throws MethodNotAllowedException
* The request uses an HTTP method which is not allowed for the specified resource.
* @throws TooManyRequestsException
* The caller has exceeded throttling limits.
* @throws InternalServerErrorException
* An internal error occurred while processing the request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ResourceGroupsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ResourceGroupsClient.ListGroups
* @see #listGroupsPaginator(ListGroupsRequest)
* @see AWS API
* Documentation
*/
default ListGroupsIterable listGroupsPaginator() throws BadRequestException, ForbiddenException, MethodNotAllowedException,
TooManyRequestsException, InternalServerErrorException, AwsServiceException, SdkClientException,
ResourceGroupsException {
return listGroupsPaginator(ListGroupsRequest.builder().build());
}
/**
*
* Returns a list of existing resource groups in your account.
*
*
*
* This is a variant of {@link #listGroups(software.amazon.awssdk.services.resourcegroups.model.ListGroupsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.resourcegroups.paginators.ListGroupsIterable responses = client.listGroupsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.resourcegroups.paginators.ListGroupsIterable responses = client.listGroupsPaginator(request);
* for (software.amazon.awssdk.services.resourcegroups.model.ListGroupsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.resourcegroups.paginators.ListGroupsIterable responses = client.listGroupsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listGroups(software.amazon.awssdk.services.resourcegroups.model.ListGroupsRequest)} operation.
*
*
* @param listGroupsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws BadRequestException
* The request does not comply with validation rules that are defined for the request parameters.
* @throws ForbiddenException
* The caller is not authorized to make the request.
* @throws MethodNotAllowedException
* The request uses an HTTP method which is not allowed for the specified resource.
* @throws TooManyRequestsException
* The caller has exceeded throttling limits.
* @throws InternalServerErrorException
* An internal error occurred while processing the request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ResourceGroupsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ResourceGroupsClient.ListGroups
* @see AWS API
* Documentation
*/
default ListGroupsIterable listGroupsPaginator(ListGroupsRequest listGroupsRequest) throws BadRequestException,
ForbiddenException, MethodNotAllowedException, TooManyRequestsException, InternalServerErrorException,
AwsServiceException, SdkClientException, ResourceGroupsException {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of existing resource groups in your account.
*
*
*
* This is a variant of {@link #listGroups(software.amazon.awssdk.services.resourcegroups.model.ListGroupsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.resourcegroups.paginators.ListGroupsIterable responses = client.listGroupsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.resourcegroups.paginators.ListGroupsIterable responses = client.listGroupsPaginator(request);
* for (software.amazon.awssdk.services.resourcegroups.model.ListGroupsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.resourcegroups.paginators.ListGroupsIterable responses = client.listGroupsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listGroups(software.amazon.awssdk.services.resourcegroups.model.ListGroupsRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListGroupsRequest.Builder} avoiding the need to
* create one manually via {@link ListGroupsRequest#builder()}
*
*
* @param listGroupsRequest
* A {@link Consumer} that will call methods on {@link ListGroupsInput.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws BadRequestException
* The request does not comply with validation rules that are defined for the request parameters.
* @throws ForbiddenException
* The caller is not authorized to make the request.
* @throws MethodNotAllowedException
* The request uses an HTTP method which is not allowed for the specified resource.
* @throws TooManyRequestsException
* The caller has exceeded throttling limits.
* @throws InternalServerErrorException
* An internal error occurred while processing the request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ResourceGroupsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ResourceGroupsClient.ListGroups
* @see AWS API
* Documentation
*/
default ListGroupsIterable listGroupsPaginator(Consumer listGroupsRequest)
throws BadRequestException, ForbiddenException, MethodNotAllowedException, TooManyRequestsException,
InternalServerErrorException, AwsServiceException, SdkClientException, ResourceGroupsException {
return listGroupsPaginator(ListGroupsRequest.builder().applyMutation(listGroupsRequest).build());
}
/**
*
* Returns a list of AWS resource identifiers that matches a specified query. The query uses the same format as a
* resource query in a CreateGroup or UpdateGroupQuery operation.
*
*
* @param searchResourcesRequest
* @return Result of the SearchResources operation returned by the service.
* @throws UnauthorizedException
* The request has not been applied because it lacks valid authentication credentials for the target
* resource.
* @throws BadRequestException
* The request does not comply with validation rules that are defined for the request parameters.
* @throws ForbiddenException
* The caller is not authorized to make the request.
* @throws MethodNotAllowedException
* The request uses an HTTP method which is not allowed for the specified resource.
* @throws TooManyRequestsException
* The caller has exceeded throttling limits.
* @throws InternalServerErrorException
* An internal error occurred while processing the request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ResourceGroupsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ResourceGroupsClient.SearchResources
* @see AWS API Documentation
*/
default SearchResourcesResponse searchResources(SearchResourcesRequest searchResourcesRequest) throws UnauthorizedException,
BadRequestException, ForbiddenException, MethodNotAllowedException, TooManyRequestsException,
InternalServerErrorException, AwsServiceException, SdkClientException, ResourceGroupsException {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of AWS resource identifiers that matches a specified query. The query uses the same format as a
* resource query in a CreateGroup or UpdateGroupQuery operation.
*
*
*
* This is a convenience which creates an instance of the {@link SearchResourcesRequest.Builder} avoiding the need
* to create one manually via {@link SearchResourcesRequest#builder()}
*
*
* @param searchResourcesRequest
* A {@link Consumer} that will call methods on {@link SearchResourcesInput.Builder} to create a request.
* @return Result of the SearchResources operation returned by the service.
* @throws UnauthorizedException
* The request has not been applied because it lacks valid authentication credentials for the target
* resource.
* @throws BadRequestException
* The request does not comply with validation rules that are defined for the request parameters.
* @throws ForbiddenException
* The caller is not authorized to make the request.
* @throws MethodNotAllowedException
* The request uses an HTTP method which is not allowed for the specified resource.
* @throws TooManyRequestsException
* The caller has exceeded throttling limits.
* @throws InternalServerErrorException
* An internal error occurred while processing the request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ResourceGroupsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ResourceGroupsClient.SearchResources
* @see AWS API Documentation
*/
default SearchResourcesResponse searchResources(Consumer searchResourcesRequest)
throws UnauthorizedException, BadRequestException, ForbiddenException, MethodNotAllowedException,
TooManyRequestsException, InternalServerErrorException, AwsServiceException, SdkClientException,
ResourceGroupsException {
return searchResources(SearchResourcesRequest.builder().applyMutation(searchResourcesRequest).build());
}
/**
*
* Returns a list of AWS resource identifiers that matches a specified query. The query uses the same format as a
* resource query in a CreateGroup or UpdateGroupQuery operation.
*
*
*
* This is a variant of
* {@link #searchResources(software.amazon.awssdk.services.resourcegroups.model.SearchResourcesRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.resourcegroups.paginators.SearchResourcesIterable responses = client.searchResourcesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.resourcegroups.paginators.SearchResourcesIterable responses = client
* .searchResourcesPaginator(request);
* for (software.amazon.awssdk.services.resourcegroups.model.SearchResourcesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.resourcegroups.paginators.SearchResourcesIterable responses = client.searchResourcesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #searchResources(software.amazon.awssdk.services.resourcegroups.model.SearchResourcesRequest)}
* operation.
*
*
* @param searchResourcesRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws UnauthorizedException
* The request has not been applied because it lacks valid authentication credentials for the target
* resource.
* @throws BadRequestException
* The request does not comply with validation rules that are defined for the request parameters.
* @throws ForbiddenException
* The caller is not authorized to make the request.
* @throws MethodNotAllowedException
* The request uses an HTTP method which is not allowed for the specified resource.
* @throws TooManyRequestsException
* The caller has exceeded throttling limits.
* @throws InternalServerErrorException
* An internal error occurred while processing the request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ResourceGroupsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ResourceGroupsClient.SearchResources
* @see AWS API Documentation
*/
default SearchResourcesIterable searchResourcesPaginator(SearchResourcesRequest searchResourcesRequest)
throws UnauthorizedException, BadRequestException, ForbiddenException, MethodNotAllowedException,
TooManyRequestsException, InternalServerErrorException, AwsServiceException, SdkClientException,
ResourceGroupsException {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of AWS resource identifiers that matches a specified query. The query uses the same format as a
* resource query in a CreateGroup or UpdateGroupQuery operation.
*
*
*
* This is a variant of
* {@link #searchResources(software.amazon.awssdk.services.resourcegroups.model.SearchResourcesRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.resourcegroups.paginators.SearchResourcesIterable responses = client.searchResourcesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.resourcegroups.paginators.SearchResourcesIterable responses = client
* .searchResourcesPaginator(request);
* for (software.amazon.awssdk.services.resourcegroups.model.SearchResourcesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.resourcegroups.paginators.SearchResourcesIterable responses = client.searchResourcesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #searchResources(software.amazon.awssdk.services.resourcegroups.model.SearchResourcesRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link SearchResourcesRequest.Builder} avoiding the need
* to create one manually via {@link SearchResourcesRequest#builder()}
*
*
* @param searchResourcesRequest
* A {@link Consumer} that will call methods on {@link SearchResourcesInput.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws UnauthorizedException
* The request has not been applied because it lacks valid authentication credentials for the target
* resource.
* @throws BadRequestException
* The request does not comply with validation rules that are defined for the request parameters.
* @throws ForbiddenException
* The caller is not authorized to make the request.
* @throws MethodNotAllowedException
* The request uses an HTTP method which is not allowed for the specified resource.
* @throws TooManyRequestsException
* The caller has exceeded throttling limits.
* @throws InternalServerErrorException
* An internal error occurred while processing the request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ResourceGroupsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ResourceGroupsClient.SearchResources
* @see AWS API Documentation
*/
default SearchResourcesIterable searchResourcesPaginator(Consumer searchResourcesRequest)
throws UnauthorizedException, BadRequestException, ForbiddenException, MethodNotAllowedException,
TooManyRequestsException, InternalServerErrorException, AwsServiceException, SdkClientException,
ResourceGroupsException {
return searchResourcesPaginator(SearchResourcesRequest.builder().applyMutation(searchResourcesRequest).build());
}
/**
*
* Adds specified tags to a resource with the specified ARN. Existing tags on a resource are not changed if they are
* not specified in the request parameters.
*
*
* @param tagRequest
* @return Result of the Tag operation returned by the service.
* @throws BadRequestException
* The request does not comply with validation rules that are defined for the request parameters.
* @throws ForbiddenException
* The caller is not authorized to make the request.
* @throws NotFoundException
* One or more resources specified in the request do not exist.
* @throws MethodNotAllowedException
* The request uses an HTTP method which is not allowed for the specified resource.
* @throws TooManyRequestsException
* The caller has exceeded throttling limits.
* @throws InternalServerErrorException
* An internal error occurred while processing the request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ResourceGroupsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ResourceGroupsClient.Tag
* @see AWS API
* Documentation
*/
default TagResponse tag(TagRequest tagRequest) throws BadRequestException, ForbiddenException, NotFoundException,
MethodNotAllowedException, TooManyRequestsException, InternalServerErrorException, AwsServiceException,
SdkClientException, ResourceGroupsException {
throw new UnsupportedOperationException();
}
/**
*
* Adds specified tags to a resource with the specified ARN. Existing tags on a resource are not changed if they are
* not specified in the request parameters.
*
*
*
* This is a convenience which creates an instance of the {@link TagRequest.Builder} avoiding the need to create one
* manually via {@link TagRequest#builder()}
*
*
* @param tagRequest
* A {@link Consumer} that will call methods on {@link TagInput.Builder} to create a request.
* @return Result of the Tag operation returned by the service.
* @throws BadRequestException
* The request does not comply with validation rules that are defined for the request parameters.
* @throws ForbiddenException
* The caller is not authorized to make the request.
* @throws NotFoundException
* One or more resources specified in the request do not exist.
* @throws MethodNotAllowedException
* The request uses an HTTP method which is not allowed for the specified resource.
* @throws TooManyRequestsException
* The caller has exceeded throttling limits.
* @throws InternalServerErrorException
* An internal error occurred while processing the request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ResourceGroupsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ResourceGroupsClient.Tag
* @see AWS API
* Documentation
*/
default TagResponse tag(Consumer tagRequest) throws BadRequestException, ForbiddenException,
NotFoundException, MethodNotAllowedException, TooManyRequestsException, InternalServerErrorException,
AwsServiceException, SdkClientException, ResourceGroupsException {
return tag(TagRequest.builder().applyMutation(tagRequest).build());
}
/**
*
* Deletes specified tags from a specified resource.
*
*
* @param untagRequest
* @return Result of the Untag operation returned by the service.
* @throws BadRequestException
* The request does not comply with validation rules that are defined for the request parameters.
* @throws ForbiddenException
* The caller is not authorized to make the request.
* @throws NotFoundException
* One or more resources specified in the request do not exist.
* @throws MethodNotAllowedException
* The request uses an HTTP method which is not allowed for the specified resource.
* @throws TooManyRequestsException
* The caller has exceeded throttling limits.
* @throws InternalServerErrorException
* An internal error occurred while processing the request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ResourceGroupsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ResourceGroupsClient.Untag
* @see AWS API
* Documentation
*/
default UntagResponse untag(UntagRequest untagRequest) throws BadRequestException, ForbiddenException, NotFoundException,
MethodNotAllowedException, TooManyRequestsException, InternalServerErrorException, AwsServiceException,
SdkClientException, ResourceGroupsException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes specified tags from a specified resource.
*
*
*
* This is a convenience which creates an instance of the {@link UntagRequest.Builder} avoiding the need to create
* one manually via {@link UntagRequest#builder()}
*
*
* @param untagRequest
* A {@link Consumer} that will call methods on {@link UntagInput.Builder} to create a request.
* @return Result of the Untag operation returned by the service.
* @throws BadRequestException
* The request does not comply with validation rules that are defined for the request parameters.
* @throws ForbiddenException
* The caller is not authorized to make the request.
* @throws NotFoundException
* One or more resources specified in the request do not exist.
* @throws MethodNotAllowedException
* The request uses an HTTP method which is not allowed for the specified resource.
* @throws TooManyRequestsException
* The caller has exceeded throttling limits.
* @throws InternalServerErrorException
* An internal error occurred while processing the request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ResourceGroupsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ResourceGroupsClient.Untag
* @see AWS API
* Documentation
*/
default UntagResponse untag(Consumer untagRequest) throws BadRequestException, ForbiddenException,
NotFoundException, MethodNotAllowedException, TooManyRequestsException, InternalServerErrorException,
AwsServiceException, SdkClientException, ResourceGroupsException {
return untag(UntagRequest.builder().applyMutation(untagRequest).build());
}
/**
*
* Updates an existing group with a new or changed description. You cannot update the name of a resource group.
*
*
* @param updateGroupRequest
* @return Result of the UpdateGroup operation returned by the service.
* @throws BadRequestException
* The request does not comply with validation rules that are defined for the request parameters.
* @throws ForbiddenException
* The caller is not authorized to make the request.
* @throws NotFoundException
* One or more resources specified in the request do not exist.
* @throws MethodNotAllowedException
* The request uses an HTTP method which is not allowed for the specified resource.
* @throws TooManyRequestsException
* The caller has exceeded throttling limits.
* @throws InternalServerErrorException
* An internal error occurred while processing the request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ResourceGroupsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ResourceGroupsClient.UpdateGroup
* @see AWS
* API Documentation
*/
default UpdateGroupResponse updateGroup(UpdateGroupRequest updateGroupRequest) throws BadRequestException,
ForbiddenException, NotFoundException, MethodNotAllowedException, TooManyRequestsException,
InternalServerErrorException, AwsServiceException, SdkClientException, ResourceGroupsException {
throw new UnsupportedOperationException();
}
/**
*
* Updates an existing group with a new or changed description. You cannot update the name of a resource group.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateGroupRequest.Builder} avoiding the need to
* create one manually via {@link UpdateGroupRequest#builder()}
*
*
* @param updateGroupRequest
* A {@link Consumer} that will call methods on {@link UpdateGroupInput.Builder} to create a request.
* @return Result of the UpdateGroup operation returned by the service.
* @throws BadRequestException
* The request does not comply with validation rules that are defined for the request parameters.
* @throws ForbiddenException
* The caller is not authorized to make the request.
* @throws NotFoundException
* One or more resources specified in the request do not exist.
* @throws MethodNotAllowedException
* The request uses an HTTP method which is not allowed for the specified resource.
* @throws TooManyRequestsException
* The caller has exceeded throttling limits.
* @throws InternalServerErrorException
* An internal error occurred while processing the request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ResourceGroupsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ResourceGroupsClient.UpdateGroup
* @see AWS
* API Documentation
*/
default UpdateGroupResponse updateGroup(Consumer updateGroupRequest) throws BadRequestException,
ForbiddenException, NotFoundException, MethodNotAllowedException, TooManyRequestsException,
InternalServerErrorException, AwsServiceException, SdkClientException, ResourceGroupsException {
return updateGroup(UpdateGroupRequest.builder().applyMutation(updateGroupRequest).build());
}
/**
*
* Updates the resource query of a group.
*
*
* @param updateGroupQueryRequest
* @return Result of the UpdateGroupQuery operation returned by the service.
* @throws BadRequestException
* The request does not comply with validation rules that are defined for the request parameters.
* @throws ForbiddenException
* The caller is not authorized to make the request.
* @throws NotFoundException
* One or more resources specified in the request do not exist.
* @throws MethodNotAllowedException
* The request uses an HTTP method which is not allowed for the specified resource.
* @throws TooManyRequestsException
* The caller has exceeded throttling limits.
* @throws InternalServerErrorException
* An internal error occurred while processing the request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ResourceGroupsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ResourceGroupsClient.UpdateGroupQuery
* @see AWS API Documentation
*/
default UpdateGroupQueryResponse updateGroupQuery(UpdateGroupQueryRequest updateGroupQueryRequest)
throws BadRequestException, ForbiddenException, NotFoundException, MethodNotAllowedException,
TooManyRequestsException, InternalServerErrorException, AwsServiceException, SdkClientException,
ResourceGroupsException {
throw new UnsupportedOperationException();
}
/**
*
* Updates the resource query of a group.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateGroupQueryRequest.Builder} avoiding the need
* to create one manually via {@link UpdateGroupQueryRequest#builder()}
*
*
* @param updateGroupQueryRequest
* A {@link Consumer} that will call methods on {@link UpdateGroupQueryInput.Builder} to create a request.
* @return Result of the UpdateGroupQuery operation returned by the service.
* @throws BadRequestException
* The request does not comply with validation rules that are defined for the request parameters.
* @throws ForbiddenException
* The caller is not authorized to make the request.
* @throws NotFoundException
* One or more resources specified in the request do not exist.
* @throws MethodNotAllowedException
* The request uses an HTTP method which is not allowed for the specified resource.
* @throws TooManyRequestsException
* The caller has exceeded throttling limits.
* @throws InternalServerErrorException
* An internal error occurred while processing the request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ResourceGroupsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ResourceGroupsClient.UpdateGroupQuery
* @see AWS API Documentation
*/
default UpdateGroupQueryResponse updateGroupQuery(Consumer updateGroupQueryRequest)
throws BadRequestException, ForbiddenException, NotFoundException, MethodNotAllowedException,
TooManyRequestsException, InternalServerErrorException, AwsServiceException, SdkClientException,
ResourceGroupsException {
return updateGroupQuery(UpdateGroupQueryRequest.builder().applyMutation(updateGroupQueryRequest).build());
}
static ServiceMetadata serviceMetadata() {
return ServiceMetadata.of("resource-groups");
}
}