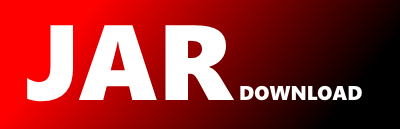
software.amazon.awssdk.services.resourcegroups.model.DeleteGroupResponse Maven / Gradle / Ivy
/*
* Copyright 2013-2018 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.resourcegroups.model;
import java.util.Objects;
import java.util.Optional;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class DeleteGroupResponse extends ResourceGroupsResponse implements
ToCopyableBuilder {
private final Group group;
private DeleteGroupResponse(BuilderImpl builder) {
super(builder);
this.group = builder.group;
}
/**
*
* A full description of the deleted resource group.
*
*
* @return A full description of the deleted resource group.
*/
public Group group() {
return group;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(group());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof DeleteGroupResponse)) {
return false;
}
DeleteGroupResponse other = (DeleteGroupResponse) obj;
return Objects.equals(group(), other.group());
}
@Override
public String toString() {
return ToString.builder("DeleteGroupResponse").add("Group", group()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Group":
return Optional.ofNullable(clazz.cast(group()));
default:
return Optional.empty();
}
}
public interface Builder extends ResourceGroupsResponse.Builder, CopyableBuilder {
/**
*
* A full description of the deleted resource group.
*
*
* @param group
* A full description of the deleted resource group.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder group(Group group);
/**
*
* A full description of the deleted resource group.
*
* This is a convenience that creates an instance of the {@link Group.Builder} avoiding the need to create one
* manually via {@link Group#builder()}.
*
* When the {@link Consumer} completes, {@link Group.Builder#build()} is called immediately and its result is
* passed to {@link #group(Group)}.
*
* @param group
* a consumer that will call methods on {@link Group.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #group(Group)
*/
default Builder group(Consumer group) {
return group(Group.builder().applyMutation(group).build());
}
}
static final class BuilderImpl extends ResourceGroupsResponse.BuilderImpl implements Builder {
private Group group;
private BuilderImpl() {
}
private BuilderImpl(DeleteGroupResponse model) {
super(model);
group(model.group);
}
public final Group.Builder getGroup() {
return group != null ? group.toBuilder() : null;
}
@Override
public final Builder group(Group group) {
this.group = group;
return this;
}
public final void setGroup(Group.BuilderImpl group) {
this.group = group != null ? group.build() : null;
}
@Override
public DeleteGroupResponse build() {
return new DeleteGroupResponse(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy