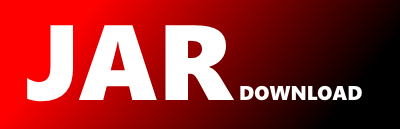
software.amazon.awssdk.services.resourcegroups.ResourceGroupsAsyncClient Maven / Gradle / Ivy
Show all versions of resourcegroups Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.resourcegroups;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkPublicApi;
import software.amazon.awssdk.annotations.ThreadSafe;
import software.amazon.awssdk.awscore.AwsClient;
import software.amazon.awssdk.services.resourcegroups.model.CancelTagSyncTaskRequest;
import software.amazon.awssdk.services.resourcegroups.model.CancelTagSyncTaskResponse;
import software.amazon.awssdk.services.resourcegroups.model.CreateGroupRequest;
import software.amazon.awssdk.services.resourcegroups.model.CreateGroupResponse;
import software.amazon.awssdk.services.resourcegroups.model.DeleteGroupRequest;
import software.amazon.awssdk.services.resourcegroups.model.DeleteGroupResponse;
import software.amazon.awssdk.services.resourcegroups.model.GetAccountSettingsRequest;
import software.amazon.awssdk.services.resourcegroups.model.GetAccountSettingsResponse;
import software.amazon.awssdk.services.resourcegroups.model.GetGroupConfigurationRequest;
import software.amazon.awssdk.services.resourcegroups.model.GetGroupConfigurationResponse;
import software.amazon.awssdk.services.resourcegroups.model.GetGroupQueryRequest;
import software.amazon.awssdk.services.resourcegroups.model.GetGroupQueryResponse;
import software.amazon.awssdk.services.resourcegroups.model.GetGroupRequest;
import software.amazon.awssdk.services.resourcegroups.model.GetGroupResponse;
import software.amazon.awssdk.services.resourcegroups.model.GetTagSyncTaskRequest;
import software.amazon.awssdk.services.resourcegroups.model.GetTagSyncTaskResponse;
import software.amazon.awssdk.services.resourcegroups.model.GetTagsRequest;
import software.amazon.awssdk.services.resourcegroups.model.GetTagsResponse;
import software.amazon.awssdk.services.resourcegroups.model.GroupResourcesRequest;
import software.amazon.awssdk.services.resourcegroups.model.GroupResourcesResponse;
import software.amazon.awssdk.services.resourcegroups.model.ListGroupResourcesRequest;
import software.amazon.awssdk.services.resourcegroups.model.ListGroupResourcesResponse;
import software.amazon.awssdk.services.resourcegroups.model.ListGroupingStatusesRequest;
import software.amazon.awssdk.services.resourcegroups.model.ListGroupingStatusesResponse;
import software.amazon.awssdk.services.resourcegroups.model.ListGroupsRequest;
import software.amazon.awssdk.services.resourcegroups.model.ListGroupsResponse;
import software.amazon.awssdk.services.resourcegroups.model.ListTagSyncTasksRequest;
import software.amazon.awssdk.services.resourcegroups.model.ListTagSyncTasksResponse;
import software.amazon.awssdk.services.resourcegroups.model.PutGroupConfigurationRequest;
import software.amazon.awssdk.services.resourcegroups.model.PutGroupConfigurationResponse;
import software.amazon.awssdk.services.resourcegroups.model.SearchResourcesRequest;
import software.amazon.awssdk.services.resourcegroups.model.SearchResourcesResponse;
import software.amazon.awssdk.services.resourcegroups.model.StartTagSyncTaskRequest;
import software.amazon.awssdk.services.resourcegroups.model.StartTagSyncTaskResponse;
import software.amazon.awssdk.services.resourcegroups.model.TagRequest;
import software.amazon.awssdk.services.resourcegroups.model.TagResponse;
import software.amazon.awssdk.services.resourcegroups.model.UngroupResourcesRequest;
import software.amazon.awssdk.services.resourcegroups.model.UngroupResourcesResponse;
import software.amazon.awssdk.services.resourcegroups.model.UntagRequest;
import software.amazon.awssdk.services.resourcegroups.model.UntagResponse;
import software.amazon.awssdk.services.resourcegroups.model.UpdateAccountSettingsRequest;
import software.amazon.awssdk.services.resourcegroups.model.UpdateAccountSettingsResponse;
import software.amazon.awssdk.services.resourcegroups.model.UpdateGroupQueryRequest;
import software.amazon.awssdk.services.resourcegroups.model.UpdateGroupQueryResponse;
import software.amazon.awssdk.services.resourcegroups.model.UpdateGroupRequest;
import software.amazon.awssdk.services.resourcegroups.model.UpdateGroupResponse;
import software.amazon.awssdk.services.resourcegroups.paginators.ListGroupResourcesPublisher;
import software.amazon.awssdk.services.resourcegroups.paginators.ListGroupingStatusesPublisher;
import software.amazon.awssdk.services.resourcegroups.paginators.ListGroupsPublisher;
import software.amazon.awssdk.services.resourcegroups.paginators.ListTagSyncTasksPublisher;
import software.amazon.awssdk.services.resourcegroups.paginators.SearchResourcesPublisher;
/**
* Service client for accessing Resource Groups asynchronously. This can be created using the static {@link #builder()}
* method.The asynchronous client performs non-blocking I/O when configured with any {@code SdkAsyncHttpClient}
* supported in the SDK. However, full non-blocking is not guaranteed as the async client may perform blocking calls in
* some cases such as credentials retrieval and endpoint discovery as part of the async API call.
*
*
* Resource Groups lets you organize Amazon Web Services resources such as Amazon Elastic Compute Cloud instances,
* Amazon Relational Database Service databases, and Amazon Simple Storage Service buckets into groups using criteria
* that you define as tags. A resource group is a collection of resources that match the resource types specified in a
* query, and share one or more tags or portions of tags. You can create a group of resources based on their roles in
* your cloud infrastructure, lifecycle stages, regions, application layers, or virtually any criteria. Resource Groups
* enable you to automate management tasks, such as those in Amazon Web Services Systems Manager Automation documents,
* on tag-related resources in Amazon Web Services Systems Manager. Groups of tagged resources also let you quickly view
* a custom console in Amazon Web Services Systems Manager that shows Config compliance and other monitoring data about
* member resources.
*
*
* To create a resource group, build a resource query, and specify tags that identify the criteria that members of the
* group have in common. Tags are key-value pairs.
*
*
* For more information about Resource Groups, see the Resource Groups User Guide.
*
*
* Resource Groups uses a REST-compliant API that you can use to perform the following types of operations.
*
*
* -
*
* Create, Read, Update, and Delete (CRUD) operations on resource groups and resource query entities
*
*
* -
*
* Applying, editing, and removing tags from resource groups
*
*
* -
*
* Resolving resource group member Amazon resource names (ARN)s so they can be returned as search results
*
*
* -
*
* Getting data about resources that are members of a group
*
*
* -
*
* Searching Amazon Web Services resources based on a resource query
*
*
*
*/
@Generated("software.amazon.awssdk:codegen")
@SdkPublicApi
@ThreadSafe
public interface ResourceGroupsAsyncClient extends AwsClient {
String SERVICE_NAME = "resource-groups";
/**
* Value for looking up the service's metadata from the
* {@link software.amazon.awssdk.regions.ServiceMetadataProvider}.
*/
String SERVICE_METADATA_ID = "resource-groups";
/**
*
* Cancels the specified tag-sync task.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:CancelTagSyncTask
on the application group
*
*
* -
*
* resource-groups:DeleteGroup
*
*
*
*
* @param cancelTagSyncTaskRequest
* @return A Java Future containing the result of the CancelTagSyncTask operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UnauthorizedException The request was rejected because it doesn't have valid credentials for the
* target resource.
* - BadRequestException The request includes one or more parameters that violate validation rules.
* - ForbiddenException The caller isn't authorized to make the request. Check permissions.
* - MethodNotAllowedException The request uses an HTTP method that isn't allowed for the specified
* resource.
* - TooManyRequestsException You've exceeded throttling limits by making too many requests in a period of
* time.
* - InternalServerErrorException An internal error occurred while processing the request. Try again
* later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.CancelTagSyncTask
* @see AWS API Documentation
*/
default CompletableFuture cancelTagSyncTask(CancelTagSyncTaskRequest cancelTagSyncTaskRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Cancels the specified tag-sync task.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:CancelTagSyncTask
on the application group
*
*
* -
*
* resource-groups:DeleteGroup
*
*
*
*
*
* This is a convenience which creates an instance of the {@link CancelTagSyncTaskRequest.Builder} avoiding the need
* to create one manually via {@link CancelTagSyncTaskRequest#builder()}
*
*
* @param cancelTagSyncTaskRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.resourcegroups.model.CancelTagSyncTaskRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the CancelTagSyncTask operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UnauthorizedException The request was rejected because it doesn't have valid credentials for the
* target resource.
* - BadRequestException The request includes one or more parameters that violate validation rules.
* - ForbiddenException The caller isn't authorized to make the request. Check permissions.
* - MethodNotAllowedException The request uses an HTTP method that isn't allowed for the specified
* resource.
* - TooManyRequestsException You've exceeded throttling limits by making too many requests in a period of
* time.
* - InternalServerErrorException An internal error occurred while processing the request. Try again
* later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.CancelTagSyncTask
* @see AWS API Documentation
*/
default CompletableFuture cancelTagSyncTask(
Consumer cancelTagSyncTaskRequest) {
return cancelTagSyncTask(CancelTagSyncTaskRequest.builder().applyMutation(cancelTagSyncTaskRequest).build());
}
/**
*
* Creates a resource group with the specified name and description. You can optionally include either a resource
* query or a service configuration. For more information about constructing a resource query, see Build queries and groups in
* Resource Groups in the Resource Groups User Guide. For more information about service-linked groups
* and service configurations, see Service configurations for Resource
* Groups.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:CreateGroup
*
*
*
*
* @param createGroupRequest
* @return A Java Future containing the result of the CreateGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BadRequestException The request includes one or more parameters that violate validation rules.
* - ForbiddenException The caller isn't authorized to make the request. Check permissions.
* - MethodNotAllowedException The request uses an HTTP method that isn't allowed for the specified
* resource.
* - TooManyRequestsException You've exceeded throttling limits by making too many requests in a period of
* time.
* - InternalServerErrorException An internal error occurred while processing the request. Try again
* later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.CreateGroup
* @see AWS
* API Documentation
*/
default CompletableFuture createGroup(CreateGroupRequest createGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a resource group with the specified name and description. You can optionally include either a resource
* query or a service configuration. For more information about constructing a resource query, see Build queries and groups in
* Resource Groups in the Resource Groups User Guide. For more information about service-linked groups
* and service configurations, see Service configurations for Resource
* Groups.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:CreateGroup
*
*
*
*
*
* This is a convenience which creates an instance of the {@link CreateGroupRequest.Builder} avoiding the need to
* create one manually via {@link CreateGroupRequest#builder()}
*
*
* @param createGroupRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.resourcegroups.model.CreateGroupRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the CreateGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BadRequestException The request includes one or more parameters that violate validation rules.
* - ForbiddenException The caller isn't authorized to make the request. Check permissions.
* - MethodNotAllowedException The request uses an HTTP method that isn't allowed for the specified
* resource.
* - TooManyRequestsException You've exceeded throttling limits by making too many requests in a period of
* time.
* - InternalServerErrorException An internal error occurred while processing the request. Try again
* later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.CreateGroup
* @see AWS
* API Documentation
*/
default CompletableFuture createGroup(Consumer createGroupRequest) {
return createGroup(CreateGroupRequest.builder().applyMutation(createGroupRequest).build());
}
/**
*
* Deletes the specified resource group. Deleting a resource group does not delete any resources that are members of
* the group; it only deletes the group structure.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:DeleteGroup
*
*
*
*
* @param deleteGroupRequest
* @return A Java Future containing the result of the DeleteGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BadRequestException The request includes one or more parameters that violate validation rules.
* - ForbiddenException The caller isn't authorized to make the request. Check permissions.
* - NotFoundException One or more of the specified resources don't exist.
* - MethodNotAllowedException The request uses an HTTP method that isn't allowed for the specified
* resource.
* - TooManyRequestsException You've exceeded throttling limits by making too many requests in a period of
* time.
* - InternalServerErrorException An internal error occurred while processing the request. Try again
* later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.DeleteGroup
* @see AWS
* API Documentation
*/
default CompletableFuture deleteGroup(DeleteGroupRequest deleteGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the specified resource group. Deleting a resource group does not delete any resources that are members of
* the group; it only deletes the group structure.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:DeleteGroup
*
*
*
*
*
* This is a convenience which creates an instance of the {@link DeleteGroupRequest.Builder} avoiding the need to
* create one manually via {@link DeleteGroupRequest#builder()}
*
*
* @param deleteGroupRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.resourcegroups.model.DeleteGroupRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeleteGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BadRequestException The request includes one or more parameters that violate validation rules.
* - ForbiddenException The caller isn't authorized to make the request. Check permissions.
* - NotFoundException One or more of the specified resources don't exist.
* - MethodNotAllowedException The request uses an HTTP method that isn't allowed for the specified
* resource.
* - TooManyRequestsException You've exceeded throttling limits by making too many requests in a period of
* time.
* - InternalServerErrorException An internal error occurred while processing the request. Try again
* later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.DeleteGroup
* @see AWS
* API Documentation
*/
default CompletableFuture deleteGroup(Consumer deleteGroupRequest) {
return deleteGroup(DeleteGroupRequest.builder().applyMutation(deleteGroupRequest).build());
}
/**
*
* Retrieves the current status of optional features in Resource Groups.
*
*
* @param getAccountSettingsRequest
* @return A Java Future containing the result of the GetAccountSettings operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BadRequestException The request includes one or more parameters that violate validation rules.
* - ForbiddenException The caller isn't authorized to make the request. Check permissions.
* - MethodNotAllowedException The request uses an HTTP method that isn't allowed for the specified
* resource.
* - TooManyRequestsException You've exceeded throttling limits by making too many requests in a period of
* time.
* - InternalServerErrorException An internal error occurred while processing the request. Try again
* later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.GetAccountSettings
* @see AWS API Documentation
*/
default CompletableFuture getAccountSettings(GetAccountSettingsRequest getAccountSettingsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the current status of optional features in Resource Groups.
*
*
*
* This is a convenience which creates an instance of the {@link GetAccountSettingsRequest.Builder} avoiding the
* need to create one manually via {@link GetAccountSettingsRequest#builder()}
*
*
* @param getAccountSettingsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.resourcegroups.model.GetAccountSettingsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetAccountSettings operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BadRequestException The request includes one or more parameters that violate validation rules.
* - ForbiddenException The caller isn't authorized to make the request. Check permissions.
* - MethodNotAllowedException The request uses an HTTP method that isn't allowed for the specified
* resource.
* - TooManyRequestsException You've exceeded throttling limits by making too many requests in a period of
* time.
* - InternalServerErrorException An internal error occurred while processing the request. Try again
* later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.GetAccountSettings
* @see AWS API Documentation
*/
default CompletableFuture getAccountSettings(
Consumer getAccountSettingsRequest) {
return getAccountSettings(GetAccountSettingsRequest.builder().applyMutation(getAccountSettingsRequest).build());
}
/**
*
* Returns information about a specified resource group.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:GetGroup
*
*
*
*
* @param getGroupRequest
* @return A Java Future containing the result of the GetGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BadRequestException The request includes one or more parameters that violate validation rules.
* - ForbiddenException The caller isn't authorized to make the request. Check permissions.
* - NotFoundException One or more of the specified resources don't exist.
* - MethodNotAllowedException The request uses an HTTP method that isn't allowed for the specified
* resource.
* - TooManyRequestsException You've exceeded throttling limits by making too many requests in a period of
* time.
* - InternalServerErrorException An internal error occurred while processing the request. Try again
* later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.GetGroup
* @see AWS API
* Documentation
*/
default CompletableFuture getGroup(GetGroupRequest getGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about a specified resource group.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:GetGroup
*
*
*
*
*
* This is a convenience which creates an instance of the {@link GetGroupRequest.Builder} avoiding the need to
* create one manually via {@link GetGroupRequest#builder()}
*
*
* @param getGroupRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.resourcegroups.model.GetGroupRequest.Builder} to create a request.
* @return A Java Future containing the result of the GetGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BadRequestException The request includes one or more parameters that violate validation rules.
* - ForbiddenException The caller isn't authorized to make the request. Check permissions.
* - NotFoundException One or more of the specified resources don't exist.
* - MethodNotAllowedException The request uses an HTTP method that isn't allowed for the specified
* resource.
* - TooManyRequestsException You've exceeded throttling limits by making too many requests in a period of
* time.
* - InternalServerErrorException An internal error occurred while processing the request. Try again
* later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.GetGroup
* @see AWS API
* Documentation
*/
default CompletableFuture getGroup(Consumer getGroupRequest) {
return getGroup(GetGroupRequest.builder().applyMutation(getGroupRequest).build());
}
/**
*
* Retrieves the service configuration associated with the specified resource group. For details about the service
* configuration syntax, see Service
* configurations for Resource Groups.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:GetGroupConfiguration
*
*
*
*
* @param getGroupConfigurationRequest
* @return A Java Future containing the result of the GetGroupConfiguration operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BadRequestException The request includes one or more parameters that violate validation rules.
* - ForbiddenException The caller isn't authorized to make the request. Check permissions.
* - NotFoundException One or more of the specified resources don't exist.
* - MethodNotAllowedException The request uses an HTTP method that isn't allowed for the specified
* resource.
* - TooManyRequestsException You've exceeded throttling limits by making too many requests in a period of
* time.
* - InternalServerErrorException An internal error occurred while processing the request. Try again
* later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.GetGroupConfiguration
* @see AWS API Documentation
*/
default CompletableFuture getGroupConfiguration(
GetGroupConfigurationRequest getGroupConfigurationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the service configuration associated with the specified resource group. For details about the service
* configuration syntax, see Service
* configurations for Resource Groups.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:GetGroupConfiguration
*
*
*
*
*
* This is a convenience which creates an instance of the {@link GetGroupConfigurationRequest.Builder} avoiding the
* need to create one manually via {@link GetGroupConfigurationRequest#builder()}
*
*
* @param getGroupConfigurationRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.resourcegroups.model.GetGroupConfigurationRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the GetGroupConfiguration operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BadRequestException The request includes one or more parameters that violate validation rules.
* - ForbiddenException The caller isn't authorized to make the request. Check permissions.
* - NotFoundException One or more of the specified resources don't exist.
* - MethodNotAllowedException The request uses an HTTP method that isn't allowed for the specified
* resource.
* - TooManyRequestsException You've exceeded throttling limits by making too many requests in a period of
* time.
* - InternalServerErrorException An internal error occurred while processing the request. Try again
* later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.GetGroupConfiguration
* @see AWS API Documentation
*/
default CompletableFuture getGroupConfiguration(
Consumer getGroupConfigurationRequest) {
return getGroupConfiguration(GetGroupConfigurationRequest.builder().applyMutation(getGroupConfigurationRequest).build());
}
/**
*
* Retrieves the resource query associated with the specified resource group. For more information about resource
* queries, see Create a tag-based group in Resource Groups.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:GetGroupQuery
*
*
*
*
* @param getGroupQueryRequest
* @return A Java Future containing the result of the GetGroupQuery operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BadRequestException The request includes one or more parameters that violate validation rules.
* - ForbiddenException The caller isn't authorized to make the request. Check permissions.
* - NotFoundException One or more of the specified resources don't exist.
* - MethodNotAllowedException The request uses an HTTP method that isn't allowed for the specified
* resource.
* - TooManyRequestsException You've exceeded throttling limits by making too many requests in a period of
* time.
* - InternalServerErrorException An internal error occurred while processing the request. Try again
* later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.GetGroupQuery
* @see AWS
* API Documentation
*/
default CompletableFuture getGroupQuery(GetGroupQueryRequest getGroupQueryRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the resource query associated with the specified resource group. For more information about resource
* queries, see Create a tag-based group in Resource Groups.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:GetGroupQuery
*
*
*
*
*
* This is a convenience which creates an instance of the {@link GetGroupQueryRequest.Builder} avoiding the need to
* create one manually via {@link GetGroupQueryRequest#builder()}
*
*
* @param getGroupQueryRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.resourcegroups.model.GetGroupQueryRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetGroupQuery operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BadRequestException The request includes one or more parameters that violate validation rules.
* - ForbiddenException The caller isn't authorized to make the request. Check permissions.
* - NotFoundException One or more of the specified resources don't exist.
* - MethodNotAllowedException The request uses an HTTP method that isn't allowed for the specified
* resource.
* - TooManyRequestsException You've exceeded throttling limits by making too many requests in a period of
* time.
* - InternalServerErrorException An internal error occurred while processing the request. Try again
* later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.GetGroupQuery
* @see AWS
* API Documentation
*/
default CompletableFuture getGroupQuery(Consumer getGroupQueryRequest) {
return getGroupQuery(GetGroupQueryRequest.builder().applyMutation(getGroupQueryRequest).build());
}
/**
*
* Returns information about a specified tag-sync task.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:GetTagSyncTask
on the application group
*
*
*
*
* @param getTagSyncTaskRequest
* @return A Java Future containing the result of the GetTagSyncTask operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UnauthorizedException The request was rejected because it doesn't have valid credentials for the
* target resource.
* - BadRequestException The request includes one or more parameters that violate validation rules.
* - ForbiddenException The caller isn't authorized to make the request. Check permissions.
* - NotFoundException One or more of the specified resources don't exist.
* - MethodNotAllowedException The request uses an HTTP method that isn't allowed for the specified
* resource.
* - TooManyRequestsException You've exceeded throttling limits by making too many requests in a period of
* time.
* - InternalServerErrorException An internal error occurred while processing the request. Try again
* later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.GetTagSyncTask
* @see AWS API Documentation
*/
default CompletableFuture getTagSyncTask(GetTagSyncTaskRequest getTagSyncTaskRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about a specified tag-sync task.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:GetTagSyncTask
on the application group
*
*
*
*
*
* This is a convenience which creates an instance of the {@link GetTagSyncTaskRequest.Builder} avoiding the need to
* create one manually via {@link GetTagSyncTaskRequest#builder()}
*
*
* @param getTagSyncTaskRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.resourcegroups.model.GetTagSyncTaskRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetTagSyncTask operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UnauthorizedException The request was rejected because it doesn't have valid credentials for the
* target resource.
* - BadRequestException The request includes one or more parameters that violate validation rules.
* - ForbiddenException The caller isn't authorized to make the request. Check permissions.
* - NotFoundException One or more of the specified resources don't exist.
* - MethodNotAllowedException The request uses an HTTP method that isn't allowed for the specified
* resource.
* - TooManyRequestsException You've exceeded throttling limits by making too many requests in a period of
* time.
* - InternalServerErrorException An internal error occurred while processing the request. Try again
* later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.GetTagSyncTask
* @see AWS API Documentation
*/
default CompletableFuture getTagSyncTask(Consumer getTagSyncTaskRequest) {
return getTagSyncTask(GetTagSyncTaskRequest.builder().applyMutation(getTagSyncTaskRequest).build());
}
/**
*
* Returns a list of tags that are associated with a resource group, specified by an Amazon resource name (ARN).
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:GetTags
*
*
*
*
* @param getTagsRequest
* @return A Java Future containing the result of the GetTags operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BadRequestException The request includes one or more parameters that violate validation rules.
* - ForbiddenException The caller isn't authorized to make the request. Check permissions.
* - NotFoundException One or more of the specified resources don't exist.
* - MethodNotAllowedException The request uses an HTTP method that isn't allowed for the specified
* resource.
* - TooManyRequestsException You've exceeded throttling limits by making too many requests in a period of
* time.
* - InternalServerErrorException An internal error occurred while processing the request. Try again
* later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.GetTags
* @see AWS API
* Documentation
*/
default CompletableFuture getTags(GetTagsRequest getTagsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of tags that are associated with a resource group, specified by an Amazon resource name (ARN).
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:GetTags
*
*
*
*
*
* This is a convenience which creates an instance of the {@link GetTagsRequest.Builder} avoiding the need to create
* one manually via {@link GetTagsRequest#builder()}
*
*
* @param getTagsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.resourcegroups.model.GetTagsRequest.Builder} to create a request.
* @return A Java Future containing the result of the GetTags operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BadRequestException The request includes one or more parameters that violate validation rules.
* - ForbiddenException The caller isn't authorized to make the request. Check permissions.
* - NotFoundException One or more of the specified resources don't exist.
* - MethodNotAllowedException The request uses an HTTP method that isn't allowed for the specified
* resource.
* - TooManyRequestsException You've exceeded throttling limits by making too many requests in a period of
* time.
* - InternalServerErrorException An internal error occurred while processing the request. Try again
* later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.GetTags
* @see AWS API
* Documentation
*/
default CompletableFuture getTags(Consumer getTagsRequest) {
return getTags(GetTagsRequest.builder().applyMutation(getTagsRequest).build());
}
/**
*
* Adds the specified resources to the specified group.
*
*
*
* You can only use this operation with the following groups:
*
*
* -
*
* AWS::EC2::HostManagement
*
*
* -
*
* AWS::EC2::CapacityReservationPool
*
*
* -
*
* AWS::ResourceGroups::ApplicationGroup
*
*
*
*
* Other resource group types and resource types are not currently supported by this operation.
*
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:GroupResources
*
*
*
*
* @param groupResourcesRequest
* @return A Java Future containing the result of the GroupResources operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BadRequestException The request includes one or more parameters that violate validation rules.
* - ForbiddenException The caller isn't authorized to make the request. Check permissions.
* - NotFoundException One or more of the specified resources don't exist.
* - MethodNotAllowedException The request uses an HTTP method that isn't allowed for the specified
* resource.
* - TooManyRequestsException You've exceeded throttling limits by making too many requests in a period of
* time.
* - InternalServerErrorException An internal error occurred while processing the request. Try again
* later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.GroupResources
* @see AWS API Documentation
*/
default CompletableFuture groupResources(GroupResourcesRequest groupResourcesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Adds the specified resources to the specified group.
*
*
*
* You can only use this operation with the following groups:
*
*
* -
*
* AWS::EC2::HostManagement
*
*
* -
*
* AWS::EC2::CapacityReservationPool
*
*
* -
*
* AWS::ResourceGroups::ApplicationGroup
*
*
*
*
* Other resource group types and resource types are not currently supported by this operation.
*
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:GroupResources
*
*
*
*
*
* This is a convenience which creates an instance of the {@link GroupResourcesRequest.Builder} avoiding the need to
* create one manually via {@link GroupResourcesRequest#builder()}
*
*
* @param groupResourcesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.resourcegroups.model.GroupResourcesRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GroupResources operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BadRequestException The request includes one or more parameters that violate validation rules.
* - ForbiddenException The caller isn't authorized to make the request. Check permissions.
* - NotFoundException One or more of the specified resources don't exist.
* - MethodNotAllowedException The request uses an HTTP method that isn't allowed for the specified
* resource.
* - TooManyRequestsException You've exceeded throttling limits by making too many requests in a period of
* time.
* - InternalServerErrorException An internal error occurred while processing the request. Try again
* later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.GroupResources
* @see AWS API Documentation
*/
default CompletableFuture groupResources(Consumer groupResourcesRequest) {
return groupResources(GroupResourcesRequest.builder().applyMutation(groupResourcesRequest).build());
}
/**
*
* Returns a list of Amazon resource names (ARNs) of the resources that are members of a specified resource group.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:ListGroupResources
*
*
* -
*
* cloudformation:DescribeStacks
*
*
* -
*
* cloudformation:ListStackResources
*
*
* -
*
* tag:GetResources
*
*
*
*
* @param listGroupResourcesRequest
* @return A Java Future containing the result of the ListGroupResources operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UnauthorizedException The request was rejected because it doesn't have valid credentials for the
* target resource.
* - BadRequestException The request includes one or more parameters that violate validation rules.
* - ForbiddenException The caller isn't authorized to make the request. Check permissions.
* - NotFoundException One or more of the specified resources don't exist.
* - MethodNotAllowedException The request uses an HTTP method that isn't allowed for the specified
* resource.
* - TooManyRequestsException You've exceeded throttling limits by making too many requests in a period of
* time.
* - InternalServerErrorException An internal error occurred while processing the request. Try again
* later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.ListGroupResources
* @see AWS API Documentation
*/
default CompletableFuture listGroupResources(ListGroupResourcesRequest listGroupResourcesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of Amazon resource names (ARNs) of the resources that are members of a specified resource group.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:ListGroupResources
*
*
* -
*
* cloudformation:DescribeStacks
*
*
* -
*
* cloudformation:ListStackResources
*
*
* -
*
* tag:GetResources
*
*
*
*
*
* This is a convenience which creates an instance of the {@link ListGroupResourcesRequest.Builder} avoiding the
* need to create one manually via {@link ListGroupResourcesRequest#builder()}
*
*
* @param listGroupResourcesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.resourcegroups.model.ListGroupResourcesRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListGroupResources operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UnauthorizedException The request was rejected because it doesn't have valid credentials for the
* target resource.
* - BadRequestException The request includes one or more parameters that violate validation rules.
* - ForbiddenException The caller isn't authorized to make the request. Check permissions.
* - NotFoundException One or more of the specified resources don't exist.
* - MethodNotAllowedException The request uses an HTTP method that isn't allowed for the specified
* resource.
* - TooManyRequestsException You've exceeded throttling limits by making too many requests in a period of
* time.
* - InternalServerErrorException An internal error occurred while processing the request. Try again
* later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.ListGroupResources
* @see AWS API Documentation
*/
default CompletableFuture listGroupResources(
Consumer listGroupResourcesRequest) {
return listGroupResources(ListGroupResourcesRequest.builder().applyMutation(listGroupResourcesRequest).build());
}
/**
*
* This is a variant of
* {@link #listGroupResources(software.amazon.awssdk.services.resourcegroups.model.ListGroupResourcesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.resourcegroups.paginators.ListGroupResourcesPublisher publisher = client.listGroupResourcesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.resourcegroups.paginators.ListGroupResourcesPublisher publisher = client.listGroupResourcesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.resourcegroups.model.ListGroupResourcesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listGroupResources(software.amazon.awssdk.services.resourcegroups.model.ListGroupResourcesRequest)}
* operation.
*
*
* @param listGroupResourcesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UnauthorizedException The request was rejected because it doesn't have valid credentials for the
* target resource.
* - BadRequestException The request includes one or more parameters that violate validation rules.
* - ForbiddenException The caller isn't authorized to make the request. Check permissions.
* - NotFoundException One or more of the specified resources don't exist.
* - MethodNotAllowedException The request uses an HTTP method that isn't allowed for the specified
* resource.
* - TooManyRequestsException You've exceeded throttling limits by making too many requests in a period of
* time.
* - InternalServerErrorException An internal error occurred while processing the request. Try again
* later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.ListGroupResources
* @see AWS API Documentation
*/
default ListGroupResourcesPublisher listGroupResourcesPaginator(ListGroupResourcesRequest listGroupResourcesRequest) {
return new ListGroupResourcesPublisher(this, listGroupResourcesRequest);
}
/**
*
* This is a variant of
* {@link #listGroupResources(software.amazon.awssdk.services.resourcegroups.model.ListGroupResourcesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.resourcegroups.paginators.ListGroupResourcesPublisher publisher = client.listGroupResourcesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.resourcegroups.paginators.ListGroupResourcesPublisher publisher = client.listGroupResourcesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.resourcegroups.model.ListGroupResourcesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listGroupResources(software.amazon.awssdk.services.resourcegroups.model.ListGroupResourcesRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListGroupResourcesRequest.Builder} avoiding the
* need to create one manually via {@link ListGroupResourcesRequest#builder()}
*
*
* @param listGroupResourcesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.resourcegroups.model.ListGroupResourcesRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UnauthorizedException The request was rejected because it doesn't have valid credentials for the
* target resource.
* - BadRequestException The request includes one or more parameters that violate validation rules.
* - ForbiddenException The caller isn't authorized to make the request. Check permissions.
* - NotFoundException One or more of the specified resources don't exist.
* - MethodNotAllowedException The request uses an HTTP method that isn't allowed for the specified
* resource.
* - TooManyRequestsException You've exceeded throttling limits by making too many requests in a period of
* time.
* - InternalServerErrorException An internal error occurred while processing the request. Try again
* later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.ListGroupResources
* @see AWS API Documentation
*/
default ListGroupResourcesPublisher listGroupResourcesPaginator(
Consumer listGroupResourcesRequest) {
return listGroupResourcesPaginator(ListGroupResourcesRequest.builder().applyMutation(listGroupResourcesRequest).build());
}
/**
*
* Returns the status of the last grouping or ungrouping action for each resource in the specified application
* group.
*
*
* @param listGroupingStatusesRequest
* @return A Java Future containing the result of the ListGroupingStatuses operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BadRequestException The request includes one or more parameters that violate validation rules.
* - ForbiddenException The caller isn't authorized to make the request. Check permissions.
* - MethodNotAllowedException The request uses an HTTP method that isn't allowed for the specified
* resource.
* - TooManyRequestsException You've exceeded throttling limits by making too many requests in a period of
* time.
* - InternalServerErrorException An internal error occurred while processing the request. Try again
* later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.ListGroupingStatuses
* @see AWS API Documentation
*/
default CompletableFuture listGroupingStatuses(
ListGroupingStatusesRequest listGroupingStatusesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns the status of the last grouping or ungrouping action for each resource in the specified application
* group.
*
*
*
* This is a convenience which creates an instance of the {@link ListGroupingStatusesRequest.Builder} avoiding the
* need to create one manually via {@link ListGroupingStatusesRequest#builder()}
*
*
* @param listGroupingStatusesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.resourcegroups.model.ListGroupingStatusesRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the ListGroupingStatuses operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BadRequestException The request includes one or more parameters that violate validation rules.
* - ForbiddenException The caller isn't authorized to make the request. Check permissions.
* - MethodNotAllowedException The request uses an HTTP method that isn't allowed for the specified
* resource.
* - TooManyRequestsException You've exceeded throttling limits by making too many requests in a period of
* time.
* - InternalServerErrorException An internal error occurred while processing the request. Try again
* later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.ListGroupingStatuses
* @see AWS API Documentation
*/
default CompletableFuture listGroupingStatuses(
Consumer listGroupingStatusesRequest) {
return listGroupingStatuses(ListGroupingStatusesRequest.builder().applyMutation(listGroupingStatusesRequest).build());
}
/**
*
* This is a variant of
* {@link #listGroupingStatuses(software.amazon.awssdk.services.resourcegroups.model.ListGroupingStatusesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.resourcegroups.paginators.ListGroupingStatusesPublisher publisher = client.listGroupingStatusesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.resourcegroups.paginators.ListGroupingStatusesPublisher publisher = client.listGroupingStatusesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.resourcegroups.model.ListGroupingStatusesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listGroupingStatuses(software.amazon.awssdk.services.resourcegroups.model.ListGroupingStatusesRequest)}
* operation.
*
*
* @param listGroupingStatusesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BadRequestException The request includes one or more parameters that violate validation rules.
* - ForbiddenException The caller isn't authorized to make the request. Check permissions.
* - MethodNotAllowedException The request uses an HTTP method that isn't allowed for the specified
* resource.
* - TooManyRequestsException You've exceeded throttling limits by making too many requests in a period of
* time.
* - InternalServerErrorException An internal error occurred while processing the request. Try again
* later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.ListGroupingStatuses
* @see AWS API Documentation
*/
default ListGroupingStatusesPublisher listGroupingStatusesPaginator(ListGroupingStatusesRequest listGroupingStatusesRequest) {
return new ListGroupingStatusesPublisher(this, listGroupingStatusesRequest);
}
/**
*
* This is a variant of
* {@link #listGroupingStatuses(software.amazon.awssdk.services.resourcegroups.model.ListGroupingStatusesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.resourcegroups.paginators.ListGroupingStatusesPublisher publisher = client.listGroupingStatusesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.resourcegroups.paginators.ListGroupingStatusesPublisher publisher = client.listGroupingStatusesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.resourcegroups.model.ListGroupingStatusesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listGroupingStatuses(software.amazon.awssdk.services.resourcegroups.model.ListGroupingStatusesRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListGroupingStatusesRequest.Builder} avoiding the
* need to create one manually via {@link ListGroupingStatusesRequest#builder()}
*
*
* @param listGroupingStatusesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.resourcegroups.model.ListGroupingStatusesRequest.Builder} to create
* a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BadRequestException The request includes one or more parameters that violate validation rules.
* - ForbiddenException The caller isn't authorized to make the request. Check permissions.
* - MethodNotAllowedException The request uses an HTTP method that isn't allowed for the specified
* resource.
* - TooManyRequestsException You've exceeded throttling limits by making too many requests in a period of
* time.
* - InternalServerErrorException An internal error occurred while processing the request. Try again
* later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.ListGroupingStatuses
* @see AWS API Documentation
*/
default ListGroupingStatusesPublisher listGroupingStatusesPaginator(
Consumer listGroupingStatusesRequest) {
return listGroupingStatusesPaginator(ListGroupingStatusesRequest.builder().applyMutation(listGroupingStatusesRequest)
.build());
}
/**
*
* Returns a list of existing Resource Groups in your account.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:ListGroups
*
*
*
*
* @param listGroupsRequest
* @return A Java Future containing the result of the ListGroups operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BadRequestException The request includes one or more parameters that violate validation rules.
* - ForbiddenException The caller isn't authorized to make the request. Check permissions.
* - MethodNotAllowedException The request uses an HTTP method that isn't allowed for the specified
* resource.
* - TooManyRequestsException You've exceeded throttling limits by making too many requests in a period of
* time.
* - InternalServerErrorException An internal error occurred while processing the request. Try again
* later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.ListGroups
* @see AWS
* API Documentation
*/
default CompletableFuture listGroups(ListGroupsRequest listGroupsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of existing Resource Groups in your account.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:ListGroups
*
*
*
*
*
* This is a convenience which creates an instance of the {@link ListGroupsRequest.Builder} avoiding the need to
* create one manually via {@link ListGroupsRequest#builder()}
*
*
* @param listGroupsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.resourcegroups.model.ListGroupsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListGroups operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BadRequestException The request includes one or more parameters that violate validation rules.
* - ForbiddenException The caller isn't authorized to make the request. Check permissions.
* - MethodNotAllowedException The request uses an HTTP method that isn't allowed for the specified
* resource.
* - TooManyRequestsException You've exceeded throttling limits by making too many requests in a period of
* time.
* - InternalServerErrorException An internal error occurred while processing the request. Try again
* later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.ListGroups
* @see AWS
* API Documentation
*/
default CompletableFuture listGroups(Consumer listGroupsRequest) {
return listGroups(ListGroupsRequest.builder().applyMutation(listGroupsRequest).build());
}
/**
*
* Returns a list of existing Resource Groups in your account.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:ListGroups
*
*
*
*
* @return A Java Future containing the result of the ListGroups operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BadRequestException The request includes one or more parameters that violate validation rules.
* - ForbiddenException The caller isn't authorized to make the request. Check permissions.
* - MethodNotAllowedException The request uses an HTTP method that isn't allowed for the specified
* resource.
* - TooManyRequestsException You've exceeded throttling limits by making too many requests in a period of
* time.
* - InternalServerErrorException An internal error occurred while processing the request. Try again
* later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.ListGroups
* @see AWS
* API Documentation
*/
default CompletableFuture listGroups() {
return listGroups(ListGroupsRequest.builder().build());
}
/**
*
* This is a variant of {@link #listGroups(software.amazon.awssdk.services.resourcegroups.model.ListGroupsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.resourcegroups.paginators.ListGroupsPublisher publisher = client.listGroupsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.resourcegroups.paginators.ListGroupsPublisher publisher = client.listGroupsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.resourcegroups.model.ListGroupsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listGroups(software.amazon.awssdk.services.resourcegroups.model.ListGroupsRequest)} operation.
*
*
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BadRequestException The request includes one or more parameters that violate validation rules.
* - ForbiddenException The caller isn't authorized to make the request. Check permissions.
* - MethodNotAllowedException The request uses an HTTP method that isn't allowed for the specified
* resource.
* - TooManyRequestsException You've exceeded throttling limits by making too many requests in a period of
* time.
* - InternalServerErrorException An internal error occurred while processing the request. Try again
* later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.ListGroups
* @see AWS
* API Documentation
*/
default ListGroupsPublisher listGroupsPaginator() {
return listGroupsPaginator(ListGroupsRequest.builder().build());
}
/**
*
* This is a variant of {@link #listGroups(software.amazon.awssdk.services.resourcegroups.model.ListGroupsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.resourcegroups.paginators.ListGroupsPublisher publisher = client.listGroupsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.resourcegroups.paginators.ListGroupsPublisher publisher = client.listGroupsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.resourcegroups.model.ListGroupsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listGroups(software.amazon.awssdk.services.resourcegroups.model.ListGroupsRequest)} operation.
*
*
* @param listGroupsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BadRequestException The request includes one or more parameters that violate validation rules.
* - ForbiddenException The caller isn't authorized to make the request. Check permissions.
* - MethodNotAllowedException The request uses an HTTP method that isn't allowed for the specified
* resource.
* - TooManyRequestsException You've exceeded throttling limits by making too many requests in a period of
* time.
* - InternalServerErrorException An internal error occurred while processing the request. Try again
* later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.ListGroups
* @see AWS
* API Documentation
*/
default ListGroupsPublisher listGroupsPaginator(ListGroupsRequest listGroupsRequest) {
return new ListGroupsPublisher(this, listGroupsRequest);
}
/**
*
* This is a variant of {@link #listGroups(software.amazon.awssdk.services.resourcegroups.model.ListGroupsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.resourcegroups.paginators.ListGroupsPublisher publisher = client.listGroupsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.resourcegroups.paginators.ListGroupsPublisher publisher = client.listGroupsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.resourcegroups.model.ListGroupsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listGroups(software.amazon.awssdk.services.resourcegroups.model.ListGroupsRequest)} operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListGroupsRequest.Builder} avoiding the need to
* create one manually via {@link ListGroupsRequest#builder()}
*
*
* @param listGroupsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.resourcegroups.model.ListGroupsRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BadRequestException The request includes one or more parameters that violate validation rules.
* - ForbiddenException The caller isn't authorized to make the request. Check permissions.
* - MethodNotAllowedException The request uses an HTTP method that isn't allowed for the specified
* resource.
* - TooManyRequestsException You've exceeded throttling limits by making too many requests in a period of
* time.
* - InternalServerErrorException An internal error occurred while processing the request. Try again
* later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.ListGroups
* @see AWS
* API Documentation
*/
default ListGroupsPublisher listGroupsPaginator(Consumer listGroupsRequest) {
return listGroupsPaginator(ListGroupsRequest.builder().applyMutation(listGroupsRequest).build());
}
/**
*
* Returns a list of tag-sync tasks.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:ListTagSyncTasks
with the group passed in the filters as the resource or * if using
* no filters
*
*
*
*
* @param listTagSyncTasksRequest
* @return A Java Future containing the result of the ListTagSyncTasks operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UnauthorizedException The request was rejected because it doesn't have valid credentials for the
* target resource.
* - BadRequestException The request includes one or more parameters that violate validation rules.
* - ForbiddenException The caller isn't authorized to make the request. Check permissions.
* - MethodNotAllowedException The request uses an HTTP method that isn't allowed for the specified
* resource.
* - TooManyRequestsException You've exceeded throttling limits by making too many requests in a period of
* time.
* - InternalServerErrorException An internal error occurred while processing the request. Try again
* later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.ListTagSyncTasks
* @see AWS API Documentation
*/
default CompletableFuture listTagSyncTasks(ListTagSyncTasksRequest listTagSyncTasksRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of tag-sync tasks.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:ListTagSyncTasks
with the group passed in the filters as the resource or * if using
* no filters
*
*
*
*
*
* This is a convenience which creates an instance of the {@link ListTagSyncTasksRequest.Builder} avoiding the need
* to create one manually via {@link ListTagSyncTasksRequest#builder()}
*
*
* @param listTagSyncTasksRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.resourcegroups.model.ListTagSyncTasksRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListTagSyncTasks operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UnauthorizedException The request was rejected because it doesn't have valid credentials for the
* target resource.
* - BadRequestException The request includes one or more parameters that violate validation rules.
* - ForbiddenException The caller isn't authorized to make the request. Check permissions.
* - MethodNotAllowedException The request uses an HTTP method that isn't allowed for the specified
* resource.
* - TooManyRequestsException You've exceeded throttling limits by making too many requests in a period of
* time.
* - InternalServerErrorException An internal error occurred while processing the request. Try again
* later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.ListTagSyncTasks
* @see AWS API Documentation
*/
default CompletableFuture listTagSyncTasks(
Consumer listTagSyncTasksRequest) {
return listTagSyncTasks(ListTagSyncTasksRequest.builder().applyMutation(listTagSyncTasksRequest).build());
}
/**
*
* This is a variant of
* {@link #listTagSyncTasks(software.amazon.awssdk.services.resourcegroups.model.ListTagSyncTasksRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.resourcegroups.paginators.ListTagSyncTasksPublisher publisher = client.listTagSyncTasksPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.resourcegroups.paginators.ListTagSyncTasksPublisher publisher = client.listTagSyncTasksPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.resourcegroups.model.ListTagSyncTasksResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listTagSyncTasks(software.amazon.awssdk.services.resourcegroups.model.ListTagSyncTasksRequest)}
* operation.
*
*
* @param listTagSyncTasksRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UnauthorizedException The request was rejected because it doesn't have valid credentials for the
* target resource.
* - BadRequestException The request includes one or more parameters that violate validation rules.
* - ForbiddenException The caller isn't authorized to make the request. Check permissions.
* - MethodNotAllowedException The request uses an HTTP method that isn't allowed for the specified
* resource.
* - TooManyRequestsException You've exceeded throttling limits by making too many requests in a period of
* time.
* - InternalServerErrorException An internal error occurred while processing the request. Try again
* later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.ListTagSyncTasks
* @see AWS API Documentation
*/
default ListTagSyncTasksPublisher listTagSyncTasksPaginator(ListTagSyncTasksRequest listTagSyncTasksRequest) {
return new ListTagSyncTasksPublisher(this, listTagSyncTasksRequest);
}
/**
*
* This is a variant of
* {@link #listTagSyncTasks(software.amazon.awssdk.services.resourcegroups.model.ListTagSyncTasksRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.resourcegroups.paginators.ListTagSyncTasksPublisher publisher = client.listTagSyncTasksPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.resourcegroups.paginators.ListTagSyncTasksPublisher publisher = client.listTagSyncTasksPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.resourcegroups.model.ListTagSyncTasksResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listTagSyncTasks(software.amazon.awssdk.services.resourcegroups.model.ListTagSyncTasksRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListTagSyncTasksRequest.Builder} avoiding the need
* to create one manually via {@link ListTagSyncTasksRequest#builder()}
*
*
* @param listTagSyncTasksRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.resourcegroups.model.ListTagSyncTasksRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UnauthorizedException The request was rejected because it doesn't have valid credentials for the
* target resource.
* - BadRequestException The request includes one or more parameters that violate validation rules.
* - ForbiddenException The caller isn't authorized to make the request. Check permissions.
* - MethodNotAllowedException The request uses an HTTP method that isn't allowed for the specified
* resource.
* - TooManyRequestsException You've exceeded throttling limits by making too many requests in a period of
* time.
* - InternalServerErrorException An internal error occurred while processing the request. Try again
* later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.ListTagSyncTasks
* @see AWS API Documentation
*/
default ListTagSyncTasksPublisher listTagSyncTasksPaginator(Consumer listTagSyncTasksRequest) {
return listTagSyncTasksPaginator(ListTagSyncTasksRequest.builder().applyMutation(listTagSyncTasksRequest).build());
}
/**
*
* Attaches a service configuration to the specified group. This occurs asynchronously, and can take time to
* complete. You can use GetGroupConfiguration to check the status of the update.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:PutGroupConfiguration
*
*
*
*
* @param putGroupConfigurationRequest
* @return A Java Future containing the result of the PutGroupConfiguration operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BadRequestException The request includes one or more parameters that violate validation rules.
* - ForbiddenException The caller isn't authorized to make the request. Check permissions.
* - NotFoundException One or more of the specified resources don't exist.
* - MethodNotAllowedException The request uses an HTTP method that isn't allowed for the specified
* resource.
* - TooManyRequestsException You've exceeded throttling limits by making too many requests in a period of
* time.
* - InternalServerErrorException An internal error occurred while processing the request. Try again
* later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.PutGroupConfiguration
* @see AWS API Documentation
*/
default CompletableFuture putGroupConfiguration(
PutGroupConfigurationRequest putGroupConfigurationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Attaches a service configuration to the specified group. This occurs asynchronously, and can take time to
* complete. You can use GetGroupConfiguration to check the status of the update.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:PutGroupConfiguration
*
*
*
*
*
* This is a convenience which creates an instance of the {@link PutGroupConfigurationRequest.Builder} avoiding the
* need to create one manually via {@link PutGroupConfigurationRequest#builder()}
*
*
* @param putGroupConfigurationRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.resourcegroups.model.PutGroupConfigurationRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the PutGroupConfiguration operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BadRequestException The request includes one or more parameters that violate validation rules.
* - ForbiddenException The caller isn't authorized to make the request. Check permissions.
* - NotFoundException One or more of the specified resources don't exist.
* - MethodNotAllowedException The request uses an HTTP method that isn't allowed for the specified
* resource.
* - TooManyRequestsException You've exceeded throttling limits by making too many requests in a period of
* time.
* - InternalServerErrorException An internal error occurred while processing the request. Try again
* later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.PutGroupConfiguration
* @see AWS API Documentation
*/
default CompletableFuture putGroupConfiguration(
Consumer putGroupConfigurationRequest) {
return putGroupConfiguration(PutGroupConfigurationRequest.builder().applyMutation(putGroupConfigurationRequest).build());
}
/**
*
* Returns a list of Amazon Web Services resource identifiers that matches the specified query. The query uses the
* same format as a resource query in a CreateGroup or UpdateGroupQuery operation.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:SearchResources
*
*
* -
*
* cloudformation:DescribeStacks
*
*
* -
*
* cloudformation:ListStackResources
*
*
* -
*
* tag:GetResources
*
*
*
*
* @param searchResourcesRequest
* @return A Java Future containing the result of the SearchResources operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UnauthorizedException The request was rejected because it doesn't have valid credentials for the
* target resource.
* - BadRequestException The request includes one or more parameters that violate validation rules.
* - ForbiddenException The caller isn't authorized to make the request. Check permissions.
* - MethodNotAllowedException The request uses an HTTP method that isn't allowed for the specified
* resource.
* - TooManyRequestsException You've exceeded throttling limits by making too many requests in a period of
* time.
* - InternalServerErrorException An internal error occurred while processing the request. Try again
* later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.SearchResources
* @see AWS API Documentation
*/
default CompletableFuture searchResources(SearchResourcesRequest searchResourcesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of Amazon Web Services resource identifiers that matches the specified query. The query uses the
* same format as a resource query in a CreateGroup or UpdateGroupQuery operation.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:SearchResources
*
*
* -
*
* cloudformation:DescribeStacks
*
*
* -
*
* cloudformation:ListStackResources
*
*
* -
*
* tag:GetResources
*
*
*
*
*
* This is a convenience which creates an instance of the {@link SearchResourcesRequest.Builder} avoiding the need
* to create one manually via {@link SearchResourcesRequest#builder()}
*
*
* @param searchResourcesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.resourcegroups.model.SearchResourcesRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the SearchResources operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UnauthorizedException The request was rejected because it doesn't have valid credentials for the
* target resource.
* - BadRequestException The request includes one or more parameters that violate validation rules.
* - ForbiddenException The caller isn't authorized to make the request. Check permissions.
* - MethodNotAllowedException The request uses an HTTP method that isn't allowed for the specified
* resource.
* - TooManyRequestsException You've exceeded throttling limits by making too many requests in a period of
* time.
* - InternalServerErrorException An internal error occurred while processing the request. Try again
* later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.SearchResources
* @see AWS API Documentation
*/
default CompletableFuture searchResources(
Consumer searchResourcesRequest) {
return searchResources(SearchResourcesRequest.builder().applyMutation(searchResourcesRequest).build());
}
/**
*
* This is a variant of
* {@link #searchResources(software.amazon.awssdk.services.resourcegroups.model.SearchResourcesRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.resourcegroups.paginators.SearchResourcesPublisher publisher = client.searchResourcesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.resourcegroups.paginators.SearchResourcesPublisher publisher = client.searchResourcesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.resourcegroups.model.SearchResourcesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #searchResources(software.amazon.awssdk.services.resourcegroups.model.SearchResourcesRequest)}
* operation.
*
*
* @param searchResourcesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UnauthorizedException The request was rejected because it doesn't have valid credentials for the
* target resource.
* - BadRequestException The request includes one or more parameters that violate validation rules.
* - ForbiddenException The caller isn't authorized to make the request. Check permissions.
* - MethodNotAllowedException The request uses an HTTP method that isn't allowed for the specified
* resource.
* - TooManyRequestsException You've exceeded throttling limits by making too many requests in a period of
* time.
* - InternalServerErrorException An internal error occurred while processing the request. Try again
* later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.SearchResources
* @see AWS API Documentation
*/
default SearchResourcesPublisher searchResourcesPaginator(SearchResourcesRequest searchResourcesRequest) {
return new SearchResourcesPublisher(this, searchResourcesRequest);
}
/**
*
* This is a variant of
* {@link #searchResources(software.amazon.awssdk.services.resourcegroups.model.SearchResourcesRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.resourcegroups.paginators.SearchResourcesPublisher publisher = client.searchResourcesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.resourcegroups.paginators.SearchResourcesPublisher publisher = client.searchResourcesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.resourcegroups.model.SearchResourcesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #searchResources(software.amazon.awssdk.services.resourcegroups.model.SearchResourcesRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link SearchResourcesRequest.Builder} avoiding the need
* to create one manually via {@link SearchResourcesRequest#builder()}
*
*
* @param searchResourcesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.resourcegroups.model.SearchResourcesRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UnauthorizedException The request was rejected because it doesn't have valid credentials for the
* target resource.
* - BadRequestException The request includes one or more parameters that violate validation rules.
* - ForbiddenException The caller isn't authorized to make the request. Check permissions.
* - MethodNotAllowedException The request uses an HTTP method that isn't allowed for the specified
* resource.
* - TooManyRequestsException You've exceeded throttling limits by making too many requests in a period of
* time.
* - InternalServerErrorException An internal error occurred while processing the request. Try again
* later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.SearchResources
* @see AWS API Documentation
*/
default SearchResourcesPublisher searchResourcesPaginator(Consumer searchResourcesRequest) {
return searchResourcesPaginator(SearchResourcesRequest.builder().applyMutation(searchResourcesRequest).build());
}
/**
*
* Creates a new tag-sync task to onboard and sync resources tagged with a specific tag key-value pair to an
* application.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:StartTagSyncTask
on the application group
*
*
* -
*
* resource-groups:CreateGroup
*
*
* -
*
* iam:PassRole
on the role provided in the request
*
*
*
*
* @param startTagSyncTaskRequest
* @return A Java Future containing the result of the StartTagSyncTask operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UnauthorizedException The request was rejected because it doesn't have valid credentials for the
* target resource.
* - BadRequestException The request includes one or more parameters that violate validation rules.
* - ForbiddenException The caller isn't authorized to make the request. Check permissions.
* - NotFoundException One or more of the specified resources don't exist.
* - MethodNotAllowedException The request uses an HTTP method that isn't allowed for the specified
* resource.
* - TooManyRequestsException You've exceeded throttling limits by making too many requests in a period of
* time.
* - InternalServerErrorException An internal error occurred while processing the request. Try again
* later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.StartTagSyncTask
* @see AWS API Documentation
*/
default CompletableFuture startTagSyncTask(StartTagSyncTaskRequest startTagSyncTaskRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new tag-sync task to onboard and sync resources tagged with a specific tag key-value pair to an
* application.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:StartTagSyncTask
on the application group
*
*
* -
*
* resource-groups:CreateGroup
*
*
* -
*
* iam:PassRole
on the role provided in the request
*
*
*
*
*
* This is a convenience which creates an instance of the {@link StartTagSyncTaskRequest.Builder} avoiding the need
* to create one manually via {@link StartTagSyncTaskRequest#builder()}
*
*
* @param startTagSyncTaskRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.resourcegroups.model.StartTagSyncTaskRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the StartTagSyncTask operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UnauthorizedException The request was rejected because it doesn't have valid credentials for the
* target resource.
* - BadRequestException The request includes one or more parameters that violate validation rules.
* - ForbiddenException The caller isn't authorized to make the request. Check permissions.
* - NotFoundException One or more of the specified resources don't exist.
* - MethodNotAllowedException The request uses an HTTP method that isn't allowed for the specified
* resource.
* - TooManyRequestsException You've exceeded throttling limits by making too many requests in a period of
* time.
* - InternalServerErrorException An internal error occurred while processing the request. Try again
* later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.StartTagSyncTask
* @see AWS API Documentation
*/
default CompletableFuture startTagSyncTask(
Consumer startTagSyncTaskRequest) {
return startTagSyncTask(StartTagSyncTaskRequest.builder().applyMutation(startTagSyncTaskRequest).build());
}
/**
*
* Adds tags to a resource group with the specified Amazon resource name (ARN). Existing tags on a resource group
* are not changed if they are not specified in the request parameters.
*
*
*
* Do not store personally identifiable information (PII) or other confidential or sensitive information in tags. We
* use tags to provide you with billing and administration services. Tags are not intended to be used for private or
* sensitive data.
*
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:Tag
*
*
*
*
* @param tagRequest
* @return A Java Future containing the result of the Tag operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BadRequestException The request includes one or more parameters that violate validation rules.
* - ForbiddenException The caller isn't authorized to make the request. Check permissions.
* - NotFoundException One or more of the specified resources don't exist.
* - MethodNotAllowedException The request uses an HTTP method that isn't allowed for the specified
* resource.
* - TooManyRequestsException You've exceeded throttling limits by making too many requests in a period of
* time.
* - InternalServerErrorException An internal error occurred while processing the request. Try again
* later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.Tag
* @see AWS API
* Documentation
*/
default CompletableFuture tag(TagRequest tagRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Adds tags to a resource group with the specified Amazon resource name (ARN). Existing tags on a resource group
* are not changed if they are not specified in the request parameters.
*
*
*
* Do not store personally identifiable information (PII) or other confidential or sensitive information in tags. We
* use tags to provide you with billing and administration services. Tags are not intended to be used for private or
* sensitive data.
*
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:Tag
*
*
*
*
*
* This is a convenience which creates an instance of the {@link TagRequest.Builder} avoiding the need to create one
* manually via {@link TagRequest#builder()}
*
*
* @param tagRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.resourcegroups.model.TagRequest.Builder} to create a request.
* @return A Java Future containing the result of the Tag operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BadRequestException The request includes one or more parameters that violate validation rules.
* - ForbiddenException The caller isn't authorized to make the request. Check permissions.
* - NotFoundException One or more of the specified resources don't exist.
* - MethodNotAllowedException The request uses an HTTP method that isn't allowed for the specified
* resource.
* - TooManyRequestsException You've exceeded throttling limits by making too many requests in a period of
* time.
* - InternalServerErrorException An internal error occurred while processing the request. Try again
* later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.Tag
* @see AWS API
* Documentation
*/
default CompletableFuture tag(Consumer tagRequest) {
return tag(TagRequest.builder().applyMutation(tagRequest).build());
}
/**
*
* Removes the specified resources from the specified group. This operation works only with static groups that you
* populated using the GroupResources operation. It doesn't work with any resource groups that are
* automatically populated by tag-based or CloudFormation stack-based queries.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:UngroupResources
*
*
*
*
* @param ungroupResourcesRequest
* @return A Java Future containing the result of the UngroupResources operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BadRequestException The request includes one or more parameters that violate validation rules.
* - ForbiddenException The caller isn't authorized to make the request. Check permissions.
* - NotFoundException One or more of the specified resources don't exist.
* - MethodNotAllowedException The request uses an HTTP method that isn't allowed for the specified
* resource.
* - TooManyRequestsException You've exceeded throttling limits by making too many requests in a period of
* time.
* - InternalServerErrorException An internal error occurred while processing the request. Try again
* later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.UngroupResources
* @see AWS API Documentation
*/
default CompletableFuture ungroupResources(UngroupResourcesRequest ungroupResourcesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Removes the specified resources from the specified group. This operation works only with static groups that you
* populated using the GroupResources operation. It doesn't work with any resource groups that are
* automatically populated by tag-based or CloudFormation stack-based queries.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:UngroupResources
*
*
*
*
*
* This is a convenience which creates an instance of the {@link UngroupResourcesRequest.Builder} avoiding the need
* to create one manually via {@link UngroupResourcesRequest#builder()}
*
*
* @param ungroupResourcesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.resourcegroups.model.UngroupResourcesRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the UngroupResources operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BadRequestException The request includes one or more parameters that violate validation rules.
* - ForbiddenException The caller isn't authorized to make the request. Check permissions.
* - NotFoundException One or more of the specified resources don't exist.
* - MethodNotAllowedException The request uses an HTTP method that isn't allowed for the specified
* resource.
* - TooManyRequestsException You've exceeded throttling limits by making too many requests in a period of
* time.
* - InternalServerErrorException An internal error occurred while processing the request. Try again
* later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.UngroupResources
* @see AWS API Documentation
*/
default CompletableFuture ungroupResources(
Consumer ungroupResourcesRequest) {
return ungroupResources(UngroupResourcesRequest.builder().applyMutation(ungroupResourcesRequest).build());
}
/**
*
* Deletes tags from a specified resource group.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:Untag
*
*
*
*
* @param untagRequest
* @return A Java Future containing the result of the Untag operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BadRequestException The request includes one or more parameters that violate validation rules.
* - ForbiddenException The caller isn't authorized to make the request. Check permissions.
* - NotFoundException One or more of the specified resources don't exist.
* - MethodNotAllowedException The request uses an HTTP method that isn't allowed for the specified
* resource.
* - TooManyRequestsException You've exceeded throttling limits by making too many requests in a period of
* time.
* - InternalServerErrorException An internal error occurred while processing the request. Try again
* later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.Untag
* @see AWS API
* Documentation
*/
default CompletableFuture untag(UntagRequest untagRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes tags from a specified resource group.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:Untag
*
*
*
*
*
* This is a convenience which creates an instance of the {@link UntagRequest.Builder} avoiding the need to create
* one manually via {@link UntagRequest#builder()}
*
*
* @param untagRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.resourcegroups.model.UntagRequest.Builder} to create a request.
* @return A Java Future containing the result of the Untag operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BadRequestException The request includes one or more parameters that violate validation rules.
* - ForbiddenException The caller isn't authorized to make the request. Check permissions.
* - NotFoundException One or more of the specified resources don't exist.
* - MethodNotAllowedException The request uses an HTTP method that isn't allowed for the specified
* resource.
* - TooManyRequestsException You've exceeded throttling limits by making too many requests in a period of
* time.
* - InternalServerErrorException An internal error occurred while processing the request. Try again
* later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.Untag
* @see AWS API
* Documentation
*/
default CompletableFuture untag(Consumer untagRequest) {
return untag(UntagRequest.builder().applyMutation(untagRequest).build());
}
/**
*
* Turns on or turns off optional features in Resource Groups.
*
*
* The preceding example shows that the request to turn on group lifecycle events is IN_PROGRESS
. You
* can call the GetAccountSettings operation to check for completion by looking for
* GroupLifecycleEventsStatus
to change to ACTIVE
.
*
*
* @param updateAccountSettingsRequest
* @return A Java Future containing the result of the UpdateAccountSettings operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BadRequestException The request includes one or more parameters that violate validation rules.
* - ForbiddenException The caller isn't authorized to make the request. Check permissions.
* - MethodNotAllowedException The request uses an HTTP method that isn't allowed for the specified
* resource.
* - TooManyRequestsException You've exceeded throttling limits by making too many requests in a period of
* time.
* - InternalServerErrorException An internal error occurred while processing the request. Try again
* later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.UpdateAccountSettings
* @see AWS API Documentation
*/
default CompletableFuture updateAccountSettings(
UpdateAccountSettingsRequest updateAccountSettingsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Turns on or turns off optional features in Resource Groups.
*
*
* The preceding example shows that the request to turn on group lifecycle events is IN_PROGRESS
. You
* can call the GetAccountSettings operation to check for completion by looking for
* GroupLifecycleEventsStatus
to change to ACTIVE
.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateAccountSettingsRequest.Builder} avoiding the
* need to create one manually via {@link UpdateAccountSettingsRequest#builder()}
*
*
* @param updateAccountSettingsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.resourcegroups.model.UpdateAccountSettingsRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the UpdateAccountSettings operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BadRequestException The request includes one or more parameters that violate validation rules.
* - ForbiddenException The caller isn't authorized to make the request. Check permissions.
* - MethodNotAllowedException The request uses an HTTP method that isn't allowed for the specified
* resource.
* - TooManyRequestsException You've exceeded throttling limits by making too many requests in a period of
* time.
* - InternalServerErrorException An internal error occurred while processing the request. Try again
* later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.UpdateAccountSettings
* @see AWS API Documentation
*/
default CompletableFuture updateAccountSettings(
Consumer updateAccountSettingsRequest) {
return updateAccountSettings(UpdateAccountSettingsRequest.builder().applyMutation(updateAccountSettingsRequest).build());
}
/**
*
* Updates the description for an existing group. You cannot update the name of a resource group.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:UpdateGroup
*
*
*
*
* @param updateGroupRequest
* @return A Java Future containing the result of the UpdateGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BadRequestException The request includes one or more parameters that violate validation rules.
* - ForbiddenException The caller isn't authorized to make the request. Check permissions.
* - NotFoundException One or more of the specified resources don't exist.
* - MethodNotAllowedException The request uses an HTTP method that isn't allowed for the specified
* resource.
* - TooManyRequestsException You've exceeded throttling limits by making too many requests in a period of
* time.
* - InternalServerErrorException An internal error occurred while processing the request. Try again
* later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.UpdateGroup
* @see AWS
* API Documentation
*/
default CompletableFuture updateGroup(UpdateGroupRequest updateGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates the description for an existing group. You cannot update the name of a resource group.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:UpdateGroup
*
*
*
*
*
* This is a convenience which creates an instance of the {@link UpdateGroupRequest.Builder} avoiding the need to
* create one manually via {@link UpdateGroupRequest#builder()}
*
*
* @param updateGroupRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.resourcegroups.model.UpdateGroupRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the UpdateGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BadRequestException The request includes one or more parameters that violate validation rules.
* - ForbiddenException The caller isn't authorized to make the request. Check permissions.
* - NotFoundException One or more of the specified resources don't exist.
* - MethodNotAllowedException The request uses an HTTP method that isn't allowed for the specified
* resource.
* - TooManyRequestsException You've exceeded throttling limits by making too many requests in a period of
* time.
* - InternalServerErrorException An internal error occurred while processing the request. Try again
* later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.UpdateGroup
* @see AWS
* API Documentation
*/
default CompletableFuture updateGroup(Consumer updateGroupRequest) {
return updateGroup(UpdateGroupRequest.builder().applyMutation(updateGroupRequest).build());
}
/**
*
* Updates the resource query of a group. For more information about resource queries, see Create a tag-based group in Resource Groups.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:UpdateGroupQuery
*
*
*
*
* @param updateGroupQueryRequest
* @return A Java Future containing the result of the UpdateGroupQuery operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BadRequestException The request includes one or more parameters that violate validation rules.
* - ForbiddenException The caller isn't authorized to make the request. Check permissions.
* - NotFoundException One or more of the specified resources don't exist.
* - MethodNotAllowedException The request uses an HTTP method that isn't allowed for the specified
* resource.
* - TooManyRequestsException You've exceeded throttling limits by making too many requests in a period of
* time.
* - InternalServerErrorException An internal error occurred while processing the request. Try again
* later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.UpdateGroupQuery
* @see AWS API Documentation
*/
default CompletableFuture updateGroupQuery(UpdateGroupQueryRequest updateGroupQueryRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates the resource query of a group. For more information about resource queries, see Create a tag-based group in Resource Groups.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:UpdateGroupQuery
*
*
*
*
*
* This is a convenience which creates an instance of the {@link UpdateGroupQueryRequest.Builder} avoiding the need
* to create one manually via {@link UpdateGroupQueryRequest#builder()}
*
*
* @param updateGroupQueryRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.resourcegroups.model.UpdateGroupQueryRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the UpdateGroupQuery operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BadRequestException The request includes one or more parameters that violate validation rules.
* - ForbiddenException The caller isn't authorized to make the request. Check permissions.
* - NotFoundException One or more of the specified resources don't exist.
* - MethodNotAllowedException The request uses an HTTP method that isn't allowed for the specified
* resource.
* - TooManyRequestsException You've exceeded throttling limits by making too many requests in a period of
* time.
* - InternalServerErrorException An internal error occurred while processing the request. Try again
* later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ResourceGroupsException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ResourceGroupsAsyncClient.UpdateGroupQuery
* @see AWS API Documentation
*/
default CompletableFuture updateGroupQuery(
Consumer updateGroupQueryRequest) {
return updateGroupQuery(UpdateGroupQueryRequest.builder().applyMutation(updateGroupQueryRequest).build());
}
@Override
default ResourceGroupsServiceClientConfiguration serviceClientConfiguration() {
throw new UnsupportedOperationException();
}
/**
* Create a {@link ResourceGroupsAsyncClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static ResourceGroupsAsyncClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link ResourceGroupsAsyncClient}.
*/
static ResourceGroupsAsyncClientBuilder builder() {
return new DefaultResourceGroupsAsyncClientBuilder();
}
}