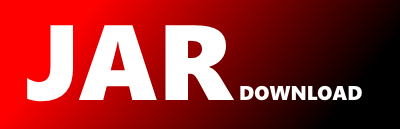
software.amazon.awssdk.retries.LegacyRetryStrategy Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package software.amazon.awssdk.retries;
import java.util.function.Predicate;
import software.amazon.awssdk.annotations.SdkPublicApi;
import software.amazon.awssdk.annotations.ThreadSafe;
import software.amazon.awssdk.retries.api.BackoffStrategy;
import software.amazon.awssdk.retries.api.RetryStrategy;
import software.amazon.awssdk.retries.internal.DefaultLegacyRetryStrategy;
import software.amazon.awssdk.retries.internal.circuitbreaker.TokenBucketStore;
/**
* The legacy retry strategy is a {@link RetryStrategy} for normal use-cases.
*
* The legacy retry strategy by default:
*
* - Retries on the conditions configured in the {@link Builder}.
*
- Retries 3 times (4 total attempts). Adjust with {@link Builder#maxAttempts(int)}
*
- For non-throttling exceptions uses the {@link BackoffStrategy#exponentialDelay} backoff strategy, with a base delay
* of 100 milliseconds and max delay of 20 seconds. Adjust with {@link Builder#backoffStrategy}
*
- For throttling exceptions uses the {@link BackoffStrategy#exponentialDelayHalfJitter} backoff strategy, with a base
* delay of 500 milliseconds and max delay of 20 seconds. Adjust with
* {@link LegacyRetryStrategy.Builder#throttlingBackoffStrategy}
*
- Circuit breaking (disabling retries) in the event of high downstream failures across the scope of
* the strategy. The circuit breaking will never prevent a successful first attempt. Adjust with
* {@link Builder#circuitBreakerEnabled}
*
- The state of the circuit breaker is not affected by throttling exceptions
*
*
* @see StandardRetryStrategy
* @see AdaptiveRetryStrategy
*/
@SdkPublicApi
@ThreadSafe
public interface LegacyRetryStrategy extends RetryStrategy {
/**
* Create a new {@link LegacyRetryStrategy.Builder}.
*
* Example Usage
*
* LegacyRetryStrategy retryStrategy =
* LegacyRetryStrategy.builder()
* .retryOnExceptionInstanceOf(IllegalArgumentException.class)
* .retryOnExceptionInstanceOf(IllegalStateException.class)
* .build();
*
*/
static Builder builder() {
return DefaultLegacyRetryStrategy
.builder()
.maxAttempts(DefaultRetryStrategy.Legacy.MAX_ATTEMPTS)
.tokenBucketStore(TokenBucketStore
.builder()
.tokenBucketMaxCapacity(DefaultRetryStrategy.Legacy.TOKEN_BUCKET_SIZE)
.build())
.tokenBucketExceptionCost(DefaultRetryStrategy.Legacy.DEFAULT_EXCEPTION_TOKEN_COST)
.tokenBucketThrottlingExceptionCost(DefaultRetryStrategy.Legacy.THROTTLE_EXCEPTION_TOKEN_COST);
}
@Override
Builder toBuilder();
interface Builder extends RetryStrategy.Builder {
/**
* Configure the backoff strategy used for throttling exceptions by this strategy.
*
* By default, this uses jittered exponential backoff.
*/
Builder throttlingBackoffStrategy(BackoffStrategy throttlingBackoffStrategy);
/**
* Whether circuit breaking is enabled for this strategy.
*
*
The circuit breaker will prevent attempts (even below the {@link #maxAttempts(int)}) if a large number of
* failures are observed by this executor.
*
*
Note: The circuit breaker scope is local to the created {@link RetryStrategy},
* and will therefore not be effective unless the {@link RetryStrategy} is used for more than one call. It's recommended
* that a {@link RetryStrategy} be reused for all calls to a single unreliable resource. It's also recommended that
* separate {@link RetryStrategy}s be used for calls to unrelated resources.
*
*
By default, this is {@code true}.
*/
Builder circuitBreakerEnabled(Boolean circuitBreakerEnabled);
/**
* Configure the predicate to allow the strategy categorize a Throwable as throttling exception.
*/
Builder treatAsThrottling(Predicate treatAsThrottling);
@Override
LegacyRetryStrategy build();
}
}