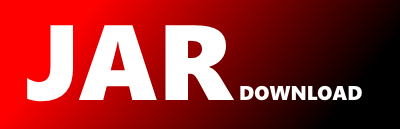
software.amazon.awssdk.services.route53.model.CreateHostedZoneResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of route53 Show documentation
Show all versions of route53 Show documentation
The AWS Java SDK for Amazon Route53 module holds the client classes that are used for communicating
with Amazon Route53
Service
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.route53.model;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* A complex type containing the response information for the hosted zone.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class CreateHostedZoneResponse extends Route53Response implements
ToCopyableBuilder {
private static final SdkField HOSTED_ZONE_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("HostedZone")
.getter(getter(CreateHostedZoneResponse::hostedZone))
.setter(setter(Builder::hostedZone))
.constructor(HostedZone::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HostedZone")
.unmarshallLocationName("HostedZone").build()).build();
private static final SdkField CHANGE_INFO_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("ChangeInfo")
.getter(getter(CreateHostedZoneResponse::changeInfo))
.setter(setter(Builder::changeInfo))
.constructor(ChangeInfo::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ChangeInfo")
.unmarshallLocationName("ChangeInfo").build()).build();
private static final SdkField DELEGATION_SET_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("DelegationSet")
.getter(getter(CreateHostedZoneResponse::delegationSet))
.setter(setter(Builder::delegationSet))
.constructor(DelegationSet::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DelegationSet")
.unmarshallLocationName("DelegationSet").build()).build();
private static final SdkField VPC_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("VPC")
.getter(getter(CreateHostedZoneResponse::vpc))
.setter(setter(Builder::vpc))
.constructor(VPC::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("VPC").unmarshallLocationName("VPC")
.build()).build();
private static final SdkField LOCATION_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("Location")
.getter(getter(CreateHostedZoneResponse::location))
.setter(setter(Builder::location))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("Location")
.unmarshallLocationName("Location").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(HOSTED_ZONE_FIELD,
CHANGE_INFO_FIELD, DELEGATION_SET_FIELD, VPC_FIELD, LOCATION_FIELD));
private final HostedZone hostedZone;
private final ChangeInfo changeInfo;
private final DelegationSet delegationSet;
private final VPC vpc;
private final String location;
private CreateHostedZoneResponse(BuilderImpl builder) {
super(builder);
this.hostedZone = builder.hostedZone;
this.changeInfo = builder.changeInfo;
this.delegationSet = builder.delegationSet;
this.vpc = builder.vpc;
this.location = builder.location;
}
/**
*
* A complex type that contains general information about the hosted zone.
*
*
* @return A complex type that contains general information about the hosted zone.
*/
public final HostedZone hostedZone() {
return hostedZone;
}
/**
*
* A complex type that contains information about the CreateHostedZone
request.
*
*
* @return A complex type that contains information about the CreateHostedZone
request.
*/
public final ChangeInfo changeInfo() {
return changeInfo;
}
/**
*
* A complex type that describes the name servers for this hosted zone.
*
*
* @return A complex type that describes the name servers for this hosted zone.
*/
public final DelegationSet delegationSet() {
return delegationSet;
}
/**
*
* A complex type that contains information about an Amazon VPC that you associated with this hosted zone.
*
*
* @return A complex type that contains information about an Amazon VPC that you associated with this hosted zone.
*/
public final VPC vpc() {
return vpc;
}
/**
*
* The unique URL representing the new hosted zone.
*
*
* @return The unique URL representing the new hosted zone.
*/
public final String location() {
return location;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(hostedZone());
hashCode = 31 * hashCode + Objects.hashCode(changeInfo());
hashCode = 31 * hashCode + Objects.hashCode(delegationSet());
hashCode = 31 * hashCode + Objects.hashCode(vpc());
hashCode = 31 * hashCode + Objects.hashCode(location());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CreateHostedZoneResponse)) {
return false;
}
CreateHostedZoneResponse other = (CreateHostedZoneResponse) obj;
return Objects.equals(hostedZone(), other.hostedZone()) && Objects.equals(changeInfo(), other.changeInfo())
&& Objects.equals(delegationSet(), other.delegationSet()) && Objects.equals(vpc(), other.vpc())
&& Objects.equals(location(), other.location());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("CreateHostedZoneResponse").add("HostedZone", hostedZone()).add("ChangeInfo", changeInfo())
.add("DelegationSet", delegationSet()).add("VPC", vpc()).add("Location", location()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "HostedZone":
return Optional.ofNullable(clazz.cast(hostedZone()));
case "ChangeInfo":
return Optional.ofNullable(clazz.cast(changeInfo()));
case "DelegationSet":
return Optional.ofNullable(clazz.cast(delegationSet()));
case "VPC":
return Optional.ofNullable(clazz.cast(vpc()));
case "Location":
return Optional.ofNullable(clazz.cast(location()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
© 2015 - 2025 Weber Informatics LLC | Privacy Policy