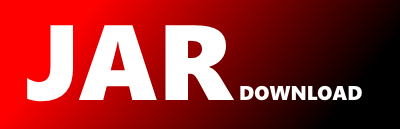
software.amazon.awssdk.services.route53.model.HostedZone Maven / Gradle / Ivy
Show all versions of route53 Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.route53.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* A complex type that contains general information about the hosted zone.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class HostedZone implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("Id")
.getter(getter(HostedZone::id))
.setter(setter(Builder::id))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Id").unmarshallLocationName("Id")
.build()).build();
private static final SdkField NAME_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("Name")
.getter(getter(HostedZone::name))
.setter(setter(Builder::name))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Name")
.unmarshallLocationName("Name").build()).build();
private static final SdkField CALLER_REFERENCE_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("CallerReference")
.getter(getter(HostedZone::callerReference))
.setter(setter(Builder::callerReference))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CallerReference")
.unmarshallLocationName("CallerReference").build()).build();
private static final SdkField CONFIG_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("Config")
.getter(getter(HostedZone::config))
.setter(setter(Builder::config))
.constructor(HostedZoneConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Config")
.unmarshallLocationName("Config").build()).build();
private static final SdkField RESOURCE_RECORD_SET_COUNT_FIELD = SdkField
. builder(MarshallingType.LONG)
.memberName("ResourceRecordSetCount")
.getter(getter(HostedZone::resourceRecordSetCount))
.setter(setter(Builder::resourceRecordSetCount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ResourceRecordSetCount")
.unmarshallLocationName("ResourceRecordSetCount").build()).build();
private static final SdkField LINKED_SERVICE_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("LinkedService")
.getter(getter(HostedZone::linkedService))
.setter(setter(Builder::linkedService))
.constructor(LinkedService::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LinkedService")
.unmarshallLocationName("LinkedService").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ID_FIELD, NAME_FIELD,
CALLER_REFERENCE_FIELD, CONFIG_FIELD, RESOURCE_RECORD_SET_COUNT_FIELD, LINKED_SERVICE_FIELD));
private static final long serialVersionUID = 1L;
private final String id;
private final String name;
private final String callerReference;
private final HostedZoneConfig config;
private final Long resourceRecordSetCount;
private final LinkedService linkedService;
private HostedZone(BuilderImpl builder) {
this.id = builder.id;
this.name = builder.name;
this.callerReference = builder.callerReference;
this.config = builder.config;
this.resourceRecordSetCount = builder.resourceRecordSetCount;
this.linkedService = builder.linkedService;
}
/**
*
* The ID that Amazon Route 53 assigned to the hosted zone when you created it.
*
*
* @return The ID that Amazon Route 53 assigned to the hosted zone when you created it.
*/
public final String id() {
return id;
}
/**
*
* The name of the domain. For public hosted zones, this is the name that you have registered with your DNS
* registrar.
*
*
* For information about how to specify characters other than a-z
, 0-9
, and -
* (hyphen) and how to specify internationalized domain names, see CreateHostedZone.
*
*
* @return The name of the domain. For public hosted zones, this is the name that you have registered with your DNS
* registrar.
*
* For information about how to specify characters other than a-z
, 0-9
, and
* -
(hyphen) and how to specify internationalized domain names, see CreateHostedZone.
*/
public final String name() {
return name;
}
/**
*
* The value that you specified for CallerReference
when you created the hosted zone.
*
*
* @return The value that you specified for CallerReference
when you created the hosted zone.
*/
public final String callerReference() {
return callerReference;
}
/**
*
* A complex type that includes the Comment
and PrivateZone
elements. If you omitted the
* HostedZoneConfig
and Comment
elements from the request, the Config
and
* Comment
elements don't appear in the response.
*
*
* @return A complex type that includes the Comment
and PrivateZone
elements. If you
* omitted the HostedZoneConfig
and Comment
elements from the request, the
* Config
and Comment
elements don't appear in the response.
*/
public final HostedZoneConfig config() {
return config;
}
/**
*
* The number of resource record sets in the hosted zone.
*
*
* @return The number of resource record sets in the hosted zone.
*/
public final Long resourceRecordSetCount() {
return resourceRecordSetCount;
}
/**
*
* If the hosted zone was created by another service, the service that created the hosted zone. When a hosted zone
* is created by another service, you can't edit or delete it using Route 53.
*
*
* @return If the hosted zone was created by another service, the service that created the hosted zone. When a
* hosted zone is created by another service, you can't edit or delete it using Route 53.
*/
public final LinkedService linkedService() {
return linkedService;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(id());
hashCode = 31 * hashCode + Objects.hashCode(name());
hashCode = 31 * hashCode + Objects.hashCode(callerReference());
hashCode = 31 * hashCode + Objects.hashCode(config());
hashCode = 31 * hashCode + Objects.hashCode(resourceRecordSetCount());
hashCode = 31 * hashCode + Objects.hashCode(linkedService());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof HostedZone)) {
return false;
}
HostedZone other = (HostedZone) obj;
return Objects.equals(id(), other.id()) && Objects.equals(name(), other.name())
&& Objects.equals(callerReference(), other.callerReference()) && Objects.equals(config(), other.config())
&& Objects.equals(resourceRecordSetCount(), other.resourceRecordSetCount())
&& Objects.equals(linkedService(), other.linkedService());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("HostedZone").add("Id", id()).add("Name", name()).add("CallerReference", callerReference())
.add("Config", config()).add("ResourceRecordSetCount", resourceRecordSetCount())
.add("LinkedService", linkedService()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Id":
return Optional.ofNullable(clazz.cast(id()));
case "Name":
return Optional.ofNullable(clazz.cast(name()));
case "CallerReference":
return Optional.ofNullable(clazz.cast(callerReference()));
case "Config":
return Optional.ofNullable(clazz.cast(config()));
case "ResourceRecordSetCount":
return Optional.ofNullable(clazz.cast(resourceRecordSetCount()));
case "LinkedService":
return Optional.ofNullable(clazz.cast(linkedService()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function