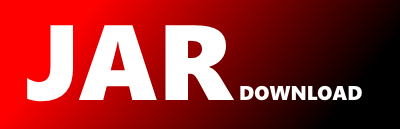
software.amazon.awssdk.services.route53profiles.Route53ProfilesClient Maven / Gradle / Ivy
Show all versions of route53profiles Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.route53profiles;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkPublicApi;
import software.amazon.awssdk.annotations.ThreadSafe;
import software.amazon.awssdk.awscore.AwsClient;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.regions.ServiceMetadata;
import software.amazon.awssdk.services.route53profiles.model.AccessDeniedException;
import software.amazon.awssdk.services.route53profiles.model.AssociateProfileRequest;
import software.amazon.awssdk.services.route53profiles.model.AssociateProfileResponse;
import software.amazon.awssdk.services.route53profiles.model.AssociateResourceToProfileRequest;
import software.amazon.awssdk.services.route53profiles.model.AssociateResourceToProfileResponse;
import software.amazon.awssdk.services.route53profiles.model.ConflictException;
import software.amazon.awssdk.services.route53profiles.model.CreateProfileRequest;
import software.amazon.awssdk.services.route53profiles.model.CreateProfileResponse;
import software.amazon.awssdk.services.route53profiles.model.DeleteProfileRequest;
import software.amazon.awssdk.services.route53profiles.model.DeleteProfileResponse;
import software.amazon.awssdk.services.route53profiles.model.DisassociateProfileRequest;
import software.amazon.awssdk.services.route53profiles.model.DisassociateProfileResponse;
import software.amazon.awssdk.services.route53profiles.model.DisassociateResourceFromProfileRequest;
import software.amazon.awssdk.services.route53profiles.model.DisassociateResourceFromProfileResponse;
import software.amazon.awssdk.services.route53profiles.model.GetProfileAssociationRequest;
import software.amazon.awssdk.services.route53profiles.model.GetProfileAssociationResponse;
import software.amazon.awssdk.services.route53profiles.model.GetProfileRequest;
import software.amazon.awssdk.services.route53profiles.model.GetProfileResourceAssociationRequest;
import software.amazon.awssdk.services.route53profiles.model.GetProfileResourceAssociationResponse;
import software.amazon.awssdk.services.route53profiles.model.GetProfileResponse;
import software.amazon.awssdk.services.route53profiles.model.InternalServiceErrorException;
import software.amazon.awssdk.services.route53profiles.model.InvalidNextTokenException;
import software.amazon.awssdk.services.route53profiles.model.InvalidParameterException;
import software.amazon.awssdk.services.route53profiles.model.LimitExceededException;
import software.amazon.awssdk.services.route53profiles.model.ListProfileAssociationsRequest;
import software.amazon.awssdk.services.route53profiles.model.ListProfileAssociationsResponse;
import software.amazon.awssdk.services.route53profiles.model.ListProfileResourceAssociationsRequest;
import software.amazon.awssdk.services.route53profiles.model.ListProfileResourceAssociationsResponse;
import software.amazon.awssdk.services.route53profiles.model.ListProfilesRequest;
import software.amazon.awssdk.services.route53profiles.model.ListProfilesResponse;
import software.amazon.awssdk.services.route53profiles.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.route53profiles.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.route53profiles.model.ResourceExistsException;
import software.amazon.awssdk.services.route53profiles.model.ResourceNotFoundException;
import software.amazon.awssdk.services.route53profiles.model.Route53ProfilesException;
import software.amazon.awssdk.services.route53profiles.model.TagResourceRequest;
import software.amazon.awssdk.services.route53profiles.model.TagResourceResponse;
import software.amazon.awssdk.services.route53profiles.model.ThrottlingException;
import software.amazon.awssdk.services.route53profiles.model.UntagResourceRequest;
import software.amazon.awssdk.services.route53profiles.model.UntagResourceResponse;
import software.amazon.awssdk.services.route53profiles.model.UpdateProfileResourceAssociationRequest;
import software.amazon.awssdk.services.route53profiles.model.UpdateProfileResourceAssociationResponse;
import software.amazon.awssdk.services.route53profiles.model.ValidationException;
import software.amazon.awssdk.services.route53profiles.paginators.ListProfileAssociationsIterable;
import software.amazon.awssdk.services.route53profiles.paginators.ListProfileResourceAssociationsIterable;
import software.amazon.awssdk.services.route53profiles.paginators.ListProfilesIterable;
/**
* Service client for accessing Route 53 Profiles. This can be created using the static {@link #builder()} method.
*
*
* With Amazon Route 53 Profiles you can share Route 53 configurations with VPCs and AWS accounts
*
*/
@Generated("software.amazon.awssdk:codegen")
@SdkPublicApi
@ThreadSafe
public interface Route53ProfilesClient extends AwsClient {
String SERVICE_NAME = "route53profiles";
/**
* Value for looking up the service's metadata from the
* {@link software.amazon.awssdk.regions.ServiceMetadataProvider}.
*/
String SERVICE_METADATA_ID = "route53profiles";
/**
*
* Associates a Route 53 Profiles profile with a VPC. A VPC can have only one Profile associated with it, but a
* Profile can be associated with 1000 of VPCs (and you can request a higher quota). For more information, see https://
* docs.aws.amazon.com/Route53/latest/DeveloperGuide/DNSLimitations.html#limits-api-entities.
*
*
* @param associateProfileRequest
* @return Result of the AssociateProfile operation returned by the service.
* @throws ResourceNotFoundException
* The resource you are associating is not found.
* @throws ResourceExistsException
* The resource you are trying to associate, has already been associated.
* @throws LimitExceededException
* The request caused one or more limits to be exceeded.
* @throws InvalidParameterException
* One or more parameters in this request are not valid.
* @throws ThrottlingException
* The request was throttled. Try again in a few minutes.
* @throws ValidationException
* You have provided an invalid command.
* @throws AccessDeniedException
* The current account doesn't have the IAM permissions required to perform the specified operation.
* @throws ConflictException
* The request you submitted conflicts with an existing request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws Route53ProfilesException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample Route53ProfilesClient.AssociateProfile
* @see AWS API Documentation
*/
default AssociateProfileResponse associateProfile(AssociateProfileRequest associateProfileRequest)
throws ResourceNotFoundException, ResourceExistsException, LimitExceededException, InvalidParameterException,
ThrottlingException, ValidationException, AccessDeniedException, ConflictException, AwsServiceException,
SdkClientException, Route53ProfilesException {
throw new UnsupportedOperationException();
}
/**
*
* Associates a Route 53 Profiles profile with a VPC. A VPC can have only one Profile associated with it, but a
* Profile can be associated with 1000 of VPCs (and you can request a higher quota). For more information, see https://
* docs.aws.amazon.com/Route53/latest/DeveloperGuide/DNSLimitations.html#limits-api-entities.
*
*
*
* This is a convenience which creates an instance of the {@link AssociateProfileRequest.Builder} avoiding the need
* to create one manually via {@link AssociateProfileRequest#builder()}
*
*
* @param associateProfileRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.route53profiles.model.AssociateProfileRequest.Builder} to create a
* request.
* @return Result of the AssociateProfile operation returned by the service.
* @throws ResourceNotFoundException
* The resource you are associating is not found.
* @throws ResourceExistsException
* The resource you are trying to associate, has already been associated.
* @throws LimitExceededException
* The request caused one or more limits to be exceeded.
* @throws InvalidParameterException
* One or more parameters in this request are not valid.
* @throws ThrottlingException
* The request was throttled. Try again in a few minutes.
* @throws ValidationException
* You have provided an invalid command.
* @throws AccessDeniedException
* The current account doesn't have the IAM permissions required to perform the specified operation.
* @throws ConflictException
* The request you submitted conflicts with an existing request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws Route53ProfilesException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample Route53ProfilesClient.AssociateProfile
* @see AWS API Documentation
*/
default AssociateProfileResponse associateProfile(Consumer associateProfileRequest)
throws ResourceNotFoundException, ResourceExistsException, LimitExceededException, InvalidParameterException,
ThrottlingException, ValidationException, AccessDeniedException, ConflictException, AwsServiceException,
SdkClientException, Route53ProfilesException {
return associateProfile(AssociateProfileRequest.builder().applyMutation(associateProfileRequest).build());
}
/**
*
* Associates a DNS reource configuration to a Route 53 Profile.
*
*
* @param associateResourceToProfileRequest
* @return Result of the AssociateResourceToProfile operation returned by the service.
* @throws ResourceNotFoundException
* The resource you are associating is not found.
* @throws LimitExceededException
* The request caused one or more limits to be exceeded.
* @throws InternalServiceErrorException
* An internal server error occured. Retry your request.
* @throws InvalidParameterException
* One or more parameters in this request are not valid.
* @throws ThrottlingException
* The request was throttled. Try again in a few minutes.
* @throws ValidationException
* You have provided an invalid command.
* @throws AccessDeniedException
* The current account doesn't have the IAM permissions required to perform the specified operation.
* @throws ConflictException
* The request you submitted conflicts with an existing request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws Route53ProfilesException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample Route53ProfilesClient.AssociateResourceToProfile
* @see AWS API Documentation
*/
default AssociateResourceToProfileResponse associateResourceToProfile(
AssociateResourceToProfileRequest associateResourceToProfileRequest) throws ResourceNotFoundException,
LimitExceededException, InternalServiceErrorException, InvalidParameterException, ThrottlingException,
ValidationException, AccessDeniedException, ConflictException, AwsServiceException, SdkClientException,
Route53ProfilesException {
throw new UnsupportedOperationException();
}
/**
*
* Associates a DNS reource configuration to a Route 53 Profile.
*
*
*
* This is a convenience which creates an instance of the {@link AssociateResourceToProfileRequest.Builder} avoiding
* the need to create one manually via {@link AssociateResourceToProfileRequest#builder()}
*
*
* @param associateResourceToProfileRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.route53profiles.model.AssociateResourceToProfileRequest.Builder} to
* create a request.
* @return Result of the AssociateResourceToProfile operation returned by the service.
* @throws ResourceNotFoundException
* The resource you are associating is not found.
* @throws LimitExceededException
* The request caused one or more limits to be exceeded.
* @throws InternalServiceErrorException
* An internal server error occured. Retry your request.
* @throws InvalidParameterException
* One or more parameters in this request are not valid.
* @throws ThrottlingException
* The request was throttled. Try again in a few minutes.
* @throws ValidationException
* You have provided an invalid command.
* @throws AccessDeniedException
* The current account doesn't have the IAM permissions required to perform the specified operation.
* @throws ConflictException
* The request you submitted conflicts with an existing request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws Route53ProfilesException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample Route53ProfilesClient.AssociateResourceToProfile
* @see AWS API Documentation
*/
default AssociateResourceToProfileResponse associateResourceToProfile(
Consumer associateResourceToProfileRequest)
throws ResourceNotFoundException, LimitExceededException, InternalServiceErrorException, InvalidParameterException,
ThrottlingException, ValidationException, AccessDeniedException, ConflictException, AwsServiceException,
SdkClientException, Route53ProfilesException {
return associateResourceToProfile(AssociateResourceToProfileRequest.builder()
.applyMutation(associateResourceToProfileRequest).build());
}
/**
*
* Creates an empty Route 53 Profile.
*
*
* @param createProfileRequest
* @return Result of the CreateProfile operation returned by the service.
* @throws LimitExceededException
* The request caused one or more limits to be exceeded.
* @throws InvalidParameterException
* One or more parameters in this request are not valid.
* @throws ThrottlingException
* The request was throttled. Try again in a few minutes.
* @throws ValidationException
* You have provided an invalid command.
* @throws AccessDeniedException
* The current account doesn't have the IAM permissions required to perform the specified operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws Route53ProfilesException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample Route53ProfilesClient.CreateProfile
* @see AWS
* API Documentation
*/
default CreateProfileResponse createProfile(CreateProfileRequest createProfileRequest) throws LimitExceededException,
InvalidParameterException, ThrottlingException, ValidationException, AccessDeniedException, AwsServiceException,
SdkClientException, Route53ProfilesException {
throw new UnsupportedOperationException();
}
/**
*
* Creates an empty Route 53 Profile.
*
*
*
* This is a convenience which creates an instance of the {@link CreateProfileRequest.Builder} avoiding the need to
* create one manually via {@link CreateProfileRequest#builder()}
*
*
* @param createProfileRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.route53profiles.model.CreateProfileRequest.Builder} to create a
* request.
* @return Result of the CreateProfile operation returned by the service.
* @throws LimitExceededException
* The request caused one or more limits to be exceeded.
* @throws InvalidParameterException
* One or more parameters in this request are not valid.
* @throws ThrottlingException
* The request was throttled. Try again in a few minutes.
* @throws ValidationException
* You have provided an invalid command.
* @throws AccessDeniedException
* The current account doesn't have the IAM permissions required to perform the specified operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws Route53ProfilesException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample Route53ProfilesClient.CreateProfile
* @see AWS
* API Documentation
*/
default CreateProfileResponse createProfile(Consumer createProfileRequest)
throws LimitExceededException, InvalidParameterException, ThrottlingException, ValidationException,
AccessDeniedException, AwsServiceException, SdkClientException, Route53ProfilesException {
return createProfile(CreateProfileRequest.builder().applyMutation(createProfileRequest).build());
}
/**
*
* Deletes the specified Route 53 Profile. Before you can delete a profile, you must first disassociate it from all
* VPCs.
*
*
* @param deleteProfileRequest
* @return Result of the DeleteProfile operation returned by the service.
* @throws ResourceNotFoundException
* The resource you are associating is not found.
* @throws ThrottlingException
* The request was throttled. Try again in a few minutes.
* @throws ValidationException
* You have provided an invalid command.
* @throws AccessDeniedException
* The current account doesn't have the IAM permissions required to perform the specified operation.
* @throws ConflictException
* The request you submitted conflicts with an existing request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws Route53ProfilesException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample Route53ProfilesClient.DeleteProfile
* @see AWS
* API Documentation
*/
default DeleteProfileResponse deleteProfile(DeleteProfileRequest deleteProfileRequest) throws ResourceNotFoundException,
ThrottlingException, ValidationException, AccessDeniedException, ConflictException, AwsServiceException,
SdkClientException, Route53ProfilesException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the specified Route 53 Profile. Before you can delete a profile, you must first disassociate it from all
* VPCs.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteProfileRequest.Builder} avoiding the need to
* create one manually via {@link DeleteProfileRequest#builder()}
*
*
* @param deleteProfileRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.route53profiles.model.DeleteProfileRequest.Builder} to create a
* request.
* @return Result of the DeleteProfile operation returned by the service.
* @throws ResourceNotFoundException
* The resource you are associating is not found.
* @throws ThrottlingException
* The request was throttled. Try again in a few minutes.
* @throws ValidationException
* You have provided an invalid command.
* @throws AccessDeniedException
* The current account doesn't have the IAM permissions required to perform the specified operation.
* @throws ConflictException
* The request you submitted conflicts with an existing request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws Route53ProfilesException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample Route53ProfilesClient.DeleteProfile
* @see AWS
* API Documentation
*/
default DeleteProfileResponse deleteProfile(Consumer deleteProfileRequest)
throws ResourceNotFoundException, ThrottlingException, ValidationException, AccessDeniedException, ConflictException,
AwsServiceException, SdkClientException, Route53ProfilesException {
return deleteProfile(DeleteProfileRequest.builder().applyMutation(deleteProfileRequest).build());
}
/**
*
* Dissociates a specified Route 53 Profile from the specified VPC.
*
*
* @param disassociateProfileRequest
* @return Result of the DisassociateProfile operation returned by the service.
* @throws ResourceNotFoundException
* The resource you are associating is not found.
* @throws LimitExceededException
* The request caused one or more limits to be exceeded.
* @throws InvalidParameterException
* One or more parameters in this request are not valid.
* @throws ThrottlingException
* The request was throttled. Try again in a few minutes.
* @throws ValidationException
* You have provided an invalid command.
* @throws AccessDeniedException
* The current account doesn't have the IAM permissions required to perform the specified operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws Route53ProfilesException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample Route53ProfilesClient.DisassociateProfile
* @see AWS API Documentation
*/
default DisassociateProfileResponse disassociateProfile(DisassociateProfileRequest disassociateProfileRequest)
throws ResourceNotFoundException, LimitExceededException, InvalidParameterException, ThrottlingException,
ValidationException, AccessDeniedException, AwsServiceException, SdkClientException, Route53ProfilesException {
throw new UnsupportedOperationException();
}
/**
*
* Dissociates a specified Route 53 Profile from the specified VPC.
*
*
*
* This is a convenience which creates an instance of the {@link DisassociateProfileRequest.Builder} avoiding the
* need to create one manually via {@link DisassociateProfileRequest#builder()}
*
*
* @param disassociateProfileRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.route53profiles.model.DisassociateProfileRequest.Builder} to create
* a request.
* @return Result of the DisassociateProfile operation returned by the service.
* @throws ResourceNotFoundException
* The resource you are associating is not found.
* @throws LimitExceededException
* The request caused one or more limits to be exceeded.
* @throws InvalidParameterException
* One or more parameters in this request are not valid.
* @throws ThrottlingException
* The request was throttled. Try again in a few minutes.
* @throws ValidationException
* You have provided an invalid command.
* @throws AccessDeniedException
* The current account doesn't have the IAM permissions required to perform the specified operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws Route53ProfilesException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample Route53ProfilesClient.DisassociateProfile
* @see AWS API Documentation
*/
default DisassociateProfileResponse disassociateProfile(
Consumer disassociateProfileRequest) throws ResourceNotFoundException,
LimitExceededException, InvalidParameterException, ThrottlingException, ValidationException, AccessDeniedException,
AwsServiceException, SdkClientException, Route53ProfilesException {
return disassociateProfile(DisassociateProfileRequest.builder().applyMutation(disassociateProfileRequest).build());
}
/**
*
* Dissoaciated a specified resource, from the Route 53 Profile.
*
*
* @param disassociateResourceFromProfileRequest
* @return Result of the DisassociateResourceFromProfile operation returned by the service.
* @throws ResourceNotFoundException
* The resource you are associating is not found.
* @throws LimitExceededException
* The request caused one or more limits to be exceeded.
* @throws InternalServiceErrorException
* An internal server error occured. Retry your request.
* @throws InvalidParameterException
* One or more parameters in this request are not valid.
* @throws ThrottlingException
* The request was throttled. Try again in a few minutes.
* @throws ValidationException
* You have provided an invalid command.
* @throws AccessDeniedException
* The current account doesn't have the IAM permissions required to perform the specified operation.
* @throws ConflictException
* The request you submitted conflicts with an existing request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws Route53ProfilesException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample Route53ProfilesClient.DisassociateResourceFromProfile
* @see AWS API Documentation
*/
default DisassociateResourceFromProfileResponse disassociateResourceFromProfile(
DisassociateResourceFromProfileRequest disassociateResourceFromProfileRequest) throws ResourceNotFoundException,
LimitExceededException, InternalServiceErrorException, InvalidParameterException, ThrottlingException,
ValidationException, AccessDeniedException, ConflictException, AwsServiceException, SdkClientException,
Route53ProfilesException {
throw new UnsupportedOperationException();
}
/**
*
* Dissoaciated a specified resource, from the Route 53 Profile.
*
*
*
* This is a convenience which creates an instance of the {@link DisassociateResourceFromProfileRequest.Builder}
* avoiding the need to create one manually via {@link DisassociateResourceFromProfileRequest#builder()}
*
*
* @param disassociateResourceFromProfileRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.route53profiles.model.DisassociateResourceFromProfileRequest.Builder}
* to create a request.
* @return Result of the DisassociateResourceFromProfile operation returned by the service.
* @throws ResourceNotFoundException
* The resource you are associating is not found.
* @throws LimitExceededException
* The request caused one or more limits to be exceeded.
* @throws InternalServiceErrorException
* An internal server error occured. Retry your request.
* @throws InvalidParameterException
* One or more parameters in this request are not valid.
* @throws ThrottlingException
* The request was throttled. Try again in a few minutes.
* @throws ValidationException
* You have provided an invalid command.
* @throws AccessDeniedException
* The current account doesn't have the IAM permissions required to perform the specified operation.
* @throws ConflictException
* The request you submitted conflicts with an existing request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws Route53ProfilesException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample Route53ProfilesClient.DisassociateResourceFromProfile
* @see AWS API Documentation
*/
default DisassociateResourceFromProfileResponse disassociateResourceFromProfile(
Consumer disassociateResourceFromProfileRequest)
throws ResourceNotFoundException, LimitExceededException, InternalServiceErrorException, InvalidParameterException,
ThrottlingException, ValidationException, AccessDeniedException, ConflictException, AwsServiceException,
SdkClientException, Route53ProfilesException {
return disassociateResourceFromProfile(DisassociateResourceFromProfileRequest.builder()
.applyMutation(disassociateResourceFromProfileRequest).build());
}
/**
*
* Returns information about a specified Route 53 Profile, such as whether whether the Profile is shared, and the
* current status of the Profile.
*
*
* @param getProfileRequest
* @return Result of the GetProfile operation returned by the service.
* @throws ResourceNotFoundException
* The resource you are associating is not found.
* @throws ThrottlingException
* The request was throttled. Try again in a few minutes.
* @throws ValidationException
* You have provided an invalid command.
* @throws AccessDeniedException
* The current account doesn't have the IAM permissions required to perform the specified operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws Route53ProfilesException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample Route53ProfilesClient.GetProfile
* @see AWS
* API Documentation
*/
default GetProfileResponse getProfile(GetProfileRequest getProfileRequest) throws ResourceNotFoundException,
ThrottlingException, ValidationException, AccessDeniedException, AwsServiceException, SdkClientException,
Route53ProfilesException {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about a specified Route 53 Profile, such as whether whether the Profile is shared, and the
* current status of the Profile.
*
*
*
* This is a convenience which creates an instance of the {@link GetProfileRequest.Builder} avoiding the need to
* create one manually via {@link GetProfileRequest#builder()}
*
*
* @param getProfileRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.route53profiles.model.GetProfileRequest.Builder} to create a
* request.
* @return Result of the GetProfile operation returned by the service.
* @throws ResourceNotFoundException
* The resource you are associating is not found.
* @throws ThrottlingException
* The request was throttled. Try again in a few minutes.
* @throws ValidationException
* You have provided an invalid command.
* @throws AccessDeniedException
* The current account doesn't have the IAM permissions required to perform the specified operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws Route53ProfilesException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample Route53ProfilesClient.GetProfile
* @see AWS
* API Documentation
*/
default GetProfileResponse getProfile(Consumer getProfileRequest)
throws ResourceNotFoundException, ThrottlingException, ValidationException, AccessDeniedException,
AwsServiceException, SdkClientException, Route53ProfilesException {
return getProfile(GetProfileRequest.builder().applyMutation(getProfileRequest).build());
}
/**
*
* Retrieves a Route 53 Profile association for a VPC. A VPC can have only one Profile association, but a Profile
* can be associated with up to 5000 VPCs.
*
*
* @param getProfileAssociationRequest
* @return Result of the GetProfileAssociation operation returned by the service.
* @throws ResourceNotFoundException
* The resource you are associating is not found.
* @throws ThrottlingException
* The request was throttled. Try again in a few minutes.
* @throws ValidationException
* You have provided an invalid command.
* @throws AccessDeniedException
* The current account doesn't have the IAM permissions required to perform the specified operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws Route53ProfilesException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample Route53ProfilesClient.GetProfileAssociation
* @see AWS API Documentation
*/
default GetProfileAssociationResponse getProfileAssociation(GetProfileAssociationRequest getProfileAssociationRequest)
throws ResourceNotFoundException, ThrottlingException, ValidationException, AccessDeniedException,
AwsServiceException, SdkClientException, Route53ProfilesException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves a Route 53 Profile association for a VPC. A VPC can have only one Profile association, but a Profile
* can be associated with up to 5000 VPCs.
*
*
*
* This is a convenience which creates an instance of the {@link GetProfileAssociationRequest.Builder} avoiding the
* need to create one manually via {@link GetProfileAssociationRequest#builder()}
*
*
* @param getProfileAssociationRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.route53profiles.model.GetProfileAssociationRequest.Builder} to
* create a request.
* @return Result of the GetProfileAssociation operation returned by the service.
* @throws ResourceNotFoundException
* The resource you are associating is not found.
* @throws ThrottlingException
* The request was throttled. Try again in a few minutes.
* @throws ValidationException
* You have provided an invalid command.
* @throws AccessDeniedException
* The current account doesn't have the IAM permissions required to perform the specified operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws Route53ProfilesException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample Route53ProfilesClient.GetProfileAssociation
* @see AWS API Documentation
*/
default GetProfileAssociationResponse getProfileAssociation(
Consumer getProfileAssociationRequest) throws ResourceNotFoundException,
ThrottlingException, ValidationException, AccessDeniedException, AwsServiceException, SdkClientException,
Route53ProfilesException {
return getProfileAssociation(GetProfileAssociationRequest.builder().applyMutation(getProfileAssociationRequest).build());
}
/**
*
* Returns information about a specified Route 53 Profile resource association.
*
*
* @param getProfileResourceAssociationRequest
* @return Result of the GetProfileResourceAssociation operation returned by the service.
* @throws ResourceNotFoundException
* The resource you are associating is not found.
* @throws InvalidParameterException
* One or more parameters in this request are not valid.
* @throws ThrottlingException
* The request was throttled. Try again in a few minutes.
* @throws ValidationException
* You have provided an invalid command.
* @throws AccessDeniedException
* The current account doesn't have the IAM permissions required to perform the specified operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws Route53ProfilesException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample Route53ProfilesClient.GetProfileResourceAssociation
* @see AWS API Documentation
*/
default GetProfileResourceAssociationResponse getProfileResourceAssociation(
GetProfileResourceAssociationRequest getProfileResourceAssociationRequest) throws ResourceNotFoundException,
InvalidParameterException, ThrottlingException, ValidationException, AccessDeniedException, AwsServiceException,
SdkClientException, Route53ProfilesException {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about a specified Route 53 Profile resource association.
*
*
*
* This is a convenience which creates an instance of the {@link GetProfileResourceAssociationRequest.Builder}
* avoiding the need to create one manually via {@link GetProfileResourceAssociationRequest#builder()}
*
*
* @param getProfileResourceAssociationRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.route53profiles.model.GetProfileResourceAssociationRequest.Builder}
* to create a request.
* @return Result of the GetProfileResourceAssociation operation returned by the service.
* @throws ResourceNotFoundException
* The resource you are associating is not found.
* @throws InvalidParameterException
* One or more parameters in this request are not valid.
* @throws ThrottlingException
* The request was throttled. Try again in a few minutes.
* @throws ValidationException
* You have provided an invalid command.
* @throws AccessDeniedException
* The current account doesn't have the IAM permissions required to perform the specified operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws Route53ProfilesException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample Route53ProfilesClient.GetProfileResourceAssociation
* @see AWS API Documentation
*/
default GetProfileResourceAssociationResponse getProfileResourceAssociation(
Consumer getProfileResourceAssociationRequest)
throws ResourceNotFoundException, InvalidParameterException, ThrottlingException, ValidationException,
AccessDeniedException, AwsServiceException, SdkClientException, Route53ProfilesException {
return getProfileResourceAssociation(GetProfileResourceAssociationRequest.builder()
.applyMutation(getProfileResourceAssociationRequest).build());
}
/**
*
* Lists all the VPCs that the specified Route 53 Profile is associated with.
*
*
* @param listProfileAssociationsRequest
* @return Result of the ListProfileAssociations operation returned by the service.
* @throws InvalidParameterException
* One or more parameters in this request are not valid.
* @throws ThrottlingException
* The request was throttled. Try again in a few minutes.
* @throws ValidationException
* You have provided an invalid command.
* @throws AccessDeniedException
* The current account doesn't have the IAM permissions required to perform the specified operation.
* @throws InvalidNextTokenException
* The NextToken
you provided isn;t valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws Route53ProfilesException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample Route53ProfilesClient.ListProfileAssociations
* @see AWS API Documentation
*/
default ListProfileAssociationsResponse listProfileAssociations(ListProfileAssociationsRequest listProfileAssociationsRequest)
throws InvalidParameterException, ThrottlingException, ValidationException, AccessDeniedException,
InvalidNextTokenException, AwsServiceException, SdkClientException, Route53ProfilesException {
throw new UnsupportedOperationException();
}
/**
*
* Lists all the VPCs that the specified Route 53 Profile is associated with.
*
*
*
* This is a convenience which creates an instance of the {@link ListProfileAssociationsRequest.Builder} avoiding
* the need to create one manually via {@link ListProfileAssociationsRequest#builder()}
*
*
* @param listProfileAssociationsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.route53profiles.model.ListProfileAssociationsRequest.Builder} to
* create a request.
* @return Result of the ListProfileAssociations operation returned by the service.
* @throws InvalidParameterException
* One or more parameters in this request are not valid.
* @throws ThrottlingException
* The request was throttled. Try again in a few minutes.
* @throws ValidationException
* You have provided an invalid command.
* @throws AccessDeniedException
* The current account doesn't have the IAM permissions required to perform the specified operation.
* @throws InvalidNextTokenException
* The NextToken
you provided isn;t valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws Route53ProfilesException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample Route53ProfilesClient.ListProfileAssociations
* @see AWS API Documentation
*/
default ListProfileAssociationsResponse listProfileAssociations(
Consumer listProfileAssociationsRequest) throws InvalidParameterException,
ThrottlingException, ValidationException, AccessDeniedException, InvalidNextTokenException, AwsServiceException,
SdkClientException, Route53ProfilesException {
return listProfileAssociations(ListProfileAssociationsRequest.builder().applyMutation(listProfileAssociationsRequest)
.build());
}
/**
*
* This is a variant of
* {@link #listProfileAssociations(software.amazon.awssdk.services.route53profiles.model.ListProfileAssociationsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.route53profiles.paginators.ListProfileAssociationsIterable responses = client.listProfileAssociationsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.route53profiles.paginators.ListProfileAssociationsIterable responses = client
* .listProfileAssociationsPaginator(request);
* for (software.amazon.awssdk.services.route53profiles.model.ListProfileAssociationsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.route53profiles.paginators.ListProfileAssociationsIterable responses = client.listProfileAssociationsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listProfileAssociations(software.amazon.awssdk.services.route53profiles.model.ListProfileAssociationsRequest)}
* operation.
*
*
* @param listProfileAssociationsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidParameterException
* One or more parameters in this request are not valid.
* @throws ThrottlingException
* The request was throttled. Try again in a few minutes.
* @throws ValidationException
* You have provided an invalid command.
* @throws AccessDeniedException
* The current account doesn't have the IAM permissions required to perform the specified operation.
* @throws InvalidNextTokenException
* The NextToken
you provided isn;t valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws Route53ProfilesException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample Route53ProfilesClient.ListProfileAssociations
* @see AWS API Documentation
*/
default ListProfileAssociationsIterable listProfileAssociationsPaginator(
ListProfileAssociationsRequest listProfileAssociationsRequest) throws InvalidParameterException, ThrottlingException,
ValidationException, AccessDeniedException, InvalidNextTokenException, AwsServiceException, SdkClientException,
Route53ProfilesException {
return new ListProfileAssociationsIterable(this, listProfileAssociationsRequest);
}
/**
*
* This is a variant of
* {@link #listProfileAssociations(software.amazon.awssdk.services.route53profiles.model.ListProfileAssociationsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.route53profiles.paginators.ListProfileAssociationsIterable responses = client.listProfileAssociationsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.route53profiles.paginators.ListProfileAssociationsIterable responses = client
* .listProfileAssociationsPaginator(request);
* for (software.amazon.awssdk.services.route53profiles.model.ListProfileAssociationsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.route53profiles.paginators.ListProfileAssociationsIterable responses = client.listProfileAssociationsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listProfileAssociations(software.amazon.awssdk.services.route53profiles.model.ListProfileAssociationsRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListProfileAssociationsRequest.Builder} avoiding
* the need to create one manually via {@link ListProfileAssociationsRequest#builder()}
*
*
* @param listProfileAssociationsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.route53profiles.model.ListProfileAssociationsRequest.Builder} to
* create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidParameterException
* One or more parameters in this request are not valid.
* @throws ThrottlingException
* The request was throttled. Try again in a few minutes.
* @throws ValidationException
* You have provided an invalid command.
* @throws AccessDeniedException
* The current account doesn't have the IAM permissions required to perform the specified operation.
* @throws InvalidNextTokenException
* The NextToken
you provided isn;t valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws Route53ProfilesException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample Route53ProfilesClient.ListProfileAssociations
* @see AWS API Documentation
*/
default ListProfileAssociationsIterable listProfileAssociationsPaginator(
Consumer listProfileAssociationsRequest) throws InvalidParameterException,
ThrottlingException, ValidationException, AccessDeniedException, InvalidNextTokenException, AwsServiceException,
SdkClientException, Route53ProfilesException {
return listProfileAssociationsPaginator(ListProfileAssociationsRequest.builder()
.applyMutation(listProfileAssociationsRequest).build());
}
/**
*
* Lists all the resource associations for the specified Route 53 Profile.
*
*
* @param listProfileResourceAssociationsRequest
* @return Result of the ListProfileResourceAssociations operation returned by the service.
* @throws ResourceNotFoundException
* The resource you are associating is not found.
* @throws InternalServiceErrorException
* An internal server error occured. Retry your request.
* @throws InvalidParameterException
* One or more parameters in this request are not valid.
* @throws ThrottlingException
* The request was throttled. Try again in a few minutes.
* @throws ValidationException
* You have provided an invalid command.
* @throws AccessDeniedException
* The current account doesn't have the IAM permissions required to perform the specified operation.
* @throws InvalidNextTokenException
* The NextToken
you provided isn;t valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws Route53ProfilesException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample Route53ProfilesClient.ListProfileResourceAssociations
* @see AWS API Documentation
*/
default ListProfileResourceAssociationsResponse listProfileResourceAssociations(
ListProfileResourceAssociationsRequest listProfileResourceAssociationsRequest) throws ResourceNotFoundException,
InternalServiceErrorException, InvalidParameterException, ThrottlingException, ValidationException,
AccessDeniedException, InvalidNextTokenException, AwsServiceException, SdkClientException, Route53ProfilesException {
throw new UnsupportedOperationException();
}
/**
*
* Lists all the resource associations for the specified Route 53 Profile.
*
*
*
* This is a convenience which creates an instance of the {@link ListProfileResourceAssociationsRequest.Builder}
* avoiding the need to create one manually via {@link ListProfileResourceAssociationsRequest#builder()}
*
*
* @param listProfileResourceAssociationsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.route53profiles.model.ListProfileResourceAssociationsRequest.Builder}
* to create a request.
* @return Result of the ListProfileResourceAssociations operation returned by the service.
* @throws ResourceNotFoundException
* The resource you are associating is not found.
* @throws InternalServiceErrorException
* An internal server error occured. Retry your request.
* @throws InvalidParameterException
* One or more parameters in this request are not valid.
* @throws ThrottlingException
* The request was throttled. Try again in a few minutes.
* @throws ValidationException
* You have provided an invalid command.
* @throws AccessDeniedException
* The current account doesn't have the IAM permissions required to perform the specified operation.
* @throws InvalidNextTokenException
* The NextToken
you provided isn;t valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws Route53ProfilesException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample Route53ProfilesClient.ListProfileResourceAssociations
* @see AWS API Documentation
*/
default ListProfileResourceAssociationsResponse listProfileResourceAssociations(
Consumer listProfileResourceAssociationsRequest)
throws ResourceNotFoundException, InternalServiceErrorException, InvalidParameterException, ThrottlingException,
ValidationException, AccessDeniedException, InvalidNextTokenException, AwsServiceException, SdkClientException,
Route53ProfilesException {
return listProfileResourceAssociations(ListProfileResourceAssociationsRequest.builder()
.applyMutation(listProfileResourceAssociationsRequest).build());
}
/**
*
* This is a variant of
* {@link #listProfileResourceAssociations(software.amazon.awssdk.services.route53profiles.model.ListProfileResourceAssociationsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.route53profiles.paginators.ListProfileResourceAssociationsIterable responses = client.listProfileResourceAssociationsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.route53profiles.paginators.ListProfileResourceAssociationsIterable responses = client
* .listProfileResourceAssociationsPaginator(request);
* for (software.amazon.awssdk.services.route53profiles.model.ListProfileResourceAssociationsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.route53profiles.paginators.ListProfileResourceAssociationsIterable responses = client.listProfileResourceAssociationsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listProfileResourceAssociations(software.amazon.awssdk.services.route53profiles.model.ListProfileResourceAssociationsRequest)}
* operation.
*
*
* @param listProfileResourceAssociationsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ResourceNotFoundException
* The resource you are associating is not found.
* @throws InternalServiceErrorException
* An internal server error occured. Retry your request.
* @throws InvalidParameterException
* One or more parameters in this request are not valid.
* @throws ThrottlingException
* The request was throttled. Try again in a few minutes.
* @throws ValidationException
* You have provided an invalid command.
* @throws AccessDeniedException
* The current account doesn't have the IAM permissions required to perform the specified operation.
* @throws InvalidNextTokenException
* The NextToken
you provided isn;t valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws Route53ProfilesException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample Route53ProfilesClient.ListProfileResourceAssociations
* @see AWS API Documentation
*/
default ListProfileResourceAssociationsIterable listProfileResourceAssociationsPaginator(
ListProfileResourceAssociationsRequest listProfileResourceAssociationsRequest) throws ResourceNotFoundException,
InternalServiceErrorException, InvalidParameterException, ThrottlingException, ValidationException,
AccessDeniedException, InvalidNextTokenException, AwsServiceException, SdkClientException, Route53ProfilesException {
return new ListProfileResourceAssociationsIterable(this, listProfileResourceAssociationsRequest);
}
/**
*
* This is a variant of
* {@link #listProfileResourceAssociations(software.amazon.awssdk.services.route53profiles.model.ListProfileResourceAssociationsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.route53profiles.paginators.ListProfileResourceAssociationsIterable responses = client.listProfileResourceAssociationsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.route53profiles.paginators.ListProfileResourceAssociationsIterable responses = client
* .listProfileResourceAssociationsPaginator(request);
* for (software.amazon.awssdk.services.route53profiles.model.ListProfileResourceAssociationsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.route53profiles.paginators.ListProfileResourceAssociationsIterable responses = client.listProfileResourceAssociationsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listProfileResourceAssociations(software.amazon.awssdk.services.route53profiles.model.ListProfileResourceAssociationsRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListProfileResourceAssociationsRequest.Builder}
* avoiding the need to create one manually via {@link ListProfileResourceAssociationsRequest#builder()}
*
*
* @param listProfileResourceAssociationsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.route53profiles.model.ListProfileResourceAssociationsRequest.Builder}
* to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ResourceNotFoundException
* The resource you are associating is not found.
* @throws InternalServiceErrorException
* An internal server error occured. Retry your request.
* @throws InvalidParameterException
* One or more parameters in this request are not valid.
* @throws ThrottlingException
* The request was throttled. Try again in a few minutes.
* @throws ValidationException
* You have provided an invalid command.
* @throws AccessDeniedException
* The current account doesn't have the IAM permissions required to perform the specified operation.
* @throws InvalidNextTokenException
* The NextToken
you provided isn;t valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws Route53ProfilesException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample Route53ProfilesClient.ListProfileResourceAssociations
* @see AWS API Documentation
*/
default ListProfileResourceAssociationsIterable listProfileResourceAssociationsPaginator(
Consumer listProfileResourceAssociationsRequest)
throws ResourceNotFoundException, InternalServiceErrorException, InvalidParameterException, ThrottlingException,
ValidationException, AccessDeniedException, InvalidNextTokenException, AwsServiceException, SdkClientException,
Route53ProfilesException {
return listProfileResourceAssociationsPaginator(ListProfileResourceAssociationsRequest.builder()
.applyMutation(listProfileResourceAssociationsRequest).build());
}
/**
*
* Lists all the Route 53 Profiles associated with your Amazon Web Services account.
*
*
* @param listProfilesRequest
* @return Result of the ListProfiles operation returned by the service.
* @throws InvalidParameterException
* One or more parameters in this request are not valid.
* @throws ThrottlingException
* The request was throttled. Try again in a few minutes.
* @throws ValidationException
* You have provided an invalid command.
* @throws AccessDeniedException
* The current account doesn't have the IAM permissions required to perform the specified operation.
* @throws InvalidNextTokenException
* The NextToken
you provided isn;t valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws Route53ProfilesException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample Route53ProfilesClient.ListProfiles
* @see AWS
* API Documentation
*/
default ListProfilesResponse listProfiles(ListProfilesRequest listProfilesRequest) throws InvalidParameterException,
ThrottlingException, ValidationException, AccessDeniedException, InvalidNextTokenException, AwsServiceException,
SdkClientException, Route53ProfilesException {
throw new UnsupportedOperationException();
}
/**
*
* Lists all the Route 53 Profiles associated with your Amazon Web Services account.
*
*
*
* This is a convenience which creates an instance of the {@link ListProfilesRequest.Builder} avoiding the need to
* create one manually via {@link ListProfilesRequest#builder()}
*
*
* @param listProfilesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.route53profiles.model.ListProfilesRequest.Builder} to create a
* request.
* @return Result of the ListProfiles operation returned by the service.
* @throws InvalidParameterException
* One or more parameters in this request are not valid.
* @throws ThrottlingException
* The request was throttled. Try again in a few minutes.
* @throws ValidationException
* You have provided an invalid command.
* @throws AccessDeniedException
* The current account doesn't have the IAM permissions required to perform the specified operation.
* @throws InvalidNextTokenException
* The NextToken
you provided isn;t valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws Route53ProfilesException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample Route53ProfilesClient.ListProfiles
* @see AWS
* API Documentation
*/
default ListProfilesResponse listProfiles(Consumer listProfilesRequest)
throws InvalidParameterException, ThrottlingException, ValidationException, AccessDeniedException,
InvalidNextTokenException, AwsServiceException, SdkClientException, Route53ProfilesException {
return listProfiles(ListProfilesRequest.builder().applyMutation(listProfilesRequest).build());
}
/**
*
* This is a variant of
* {@link #listProfiles(software.amazon.awssdk.services.route53profiles.model.ListProfilesRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.route53profiles.paginators.ListProfilesIterable responses = client.listProfilesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.route53profiles.paginators.ListProfilesIterable responses = client
* .listProfilesPaginator(request);
* for (software.amazon.awssdk.services.route53profiles.model.ListProfilesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.route53profiles.paginators.ListProfilesIterable responses = client.listProfilesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listProfiles(software.amazon.awssdk.services.route53profiles.model.ListProfilesRequest)} operation.
*
*
* @param listProfilesRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidParameterException
* One or more parameters in this request are not valid.
* @throws ThrottlingException
* The request was throttled. Try again in a few minutes.
* @throws ValidationException
* You have provided an invalid command.
* @throws AccessDeniedException
* The current account doesn't have the IAM permissions required to perform the specified operation.
* @throws InvalidNextTokenException
* The NextToken
you provided isn;t valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws Route53ProfilesException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample Route53ProfilesClient.ListProfiles
* @see AWS
* API Documentation
*/
default ListProfilesIterable listProfilesPaginator(ListProfilesRequest listProfilesRequest) throws InvalidParameterException,
ThrottlingException, ValidationException, AccessDeniedException, InvalidNextTokenException, AwsServiceException,
SdkClientException, Route53ProfilesException {
return new ListProfilesIterable(this, listProfilesRequest);
}
/**
*
* This is a variant of
* {@link #listProfiles(software.amazon.awssdk.services.route53profiles.model.ListProfilesRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.route53profiles.paginators.ListProfilesIterable responses = client.listProfilesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.route53profiles.paginators.ListProfilesIterable responses = client
* .listProfilesPaginator(request);
* for (software.amazon.awssdk.services.route53profiles.model.ListProfilesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.route53profiles.paginators.ListProfilesIterable responses = client.listProfilesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listProfiles(software.amazon.awssdk.services.route53profiles.model.ListProfilesRequest)} operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListProfilesRequest.Builder} avoiding the need to
* create one manually via {@link ListProfilesRequest#builder()}
*
*
* @param listProfilesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.route53profiles.model.ListProfilesRequest.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidParameterException
* One or more parameters in this request are not valid.
* @throws ThrottlingException
* The request was throttled. Try again in a few minutes.
* @throws ValidationException
* You have provided an invalid command.
* @throws AccessDeniedException
* The current account doesn't have the IAM permissions required to perform the specified operation.
* @throws InvalidNextTokenException
* The NextToken
you provided isn;t valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws Route53ProfilesException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample Route53ProfilesClient.ListProfiles
* @see AWS
* API Documentation
*/
default ListProfilesIterable listProfilesPaginator(Consumer listProfilesRequest)
throws InvalidParameterException, ThrottlingException, ValidationException, AccessDeniedException,
InvalidNextTokenException, AwsServiceException, SdkClientException, Route53ProfilesException {
return listProfilesPaginator(ListProfilesRequest.builder().applyMutation(listProfilesRequest).build());
}
/**
*
* Lists the tags that you associated with the specified resource.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws ResourceNotFoundException
* The resource you are associating is not found.
* @throws ThrottlingException
* The request was throttled. Try again in a few minutes.
* @throws ValidationException
* You have provided an invalid command.
* @throws AccessDeniedException
* The current account doesn't have the IAM permissions required to perform the specified operation.
* @throws ConflictException
* The request you submitted conflicts with an existing request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws Route53ProfilesException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample Route53ProfilesClient.ListTagsForResource
* @see AWS API Documentation
*/
default ListTagsForResourceResponse listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest)
throws ResourceNotFoundException, ThrottlingException, ValidationException, AccessDeniedException, ConflictException,
AwsServiceException, SdkClientException, Route53ProfilesException {
throw new UnsupportedOperationException();
}
/**
*
* Lists the tags that you associated with the specified resource.
*
*
*
* This is a convenience which creates an instance of the {@link ListTagsForResourceRequest.Builder} avoiding the
* need to create one manually via {@link ListTagsForResourceRequest#builder()}
*
*
* @param listTagsForResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.route53profiles.model.ListTagsForResourceRequest.Builder} to create
* a request.
* @return Result of the ListTagsForResource operation returned by the service.
* @throws ResourceNotFoundException
* The resource you are associating is not found.
* @throws ThrottlingException
* The request was throttled. Try again in a few minutes.
* @throws ValidationException
* You have provided an invalid command.
* @throws AccessDeniedException
* The current account doesn't have the IAM permissions required to perform the specified operation.
* @throws ConflictException
* The request you submitted conflicts with an existing request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws Route53ProfilesException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample Route53ProfilesClient.ListTagsForResource
* @see AWS API Documentation
*/
default ListTagsForResourceResponse listTagsForResource(
Consumer listTagsForResourceRequest) throws ResourceNotFoundException,
ThrottlingException, ValidationException, AccessDeniedException, ConflictException, AwsServiceException,
SdkClientException, Route53ProfilesException {
return listTagsForResource(ListTagsForResourceRequest.builder().applyMutation(listTagsForResourceRequest).build());
}
/**
*
* Adds one or more tags to a specified resource.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws ResourceNotFoundException
* The resource you are associating is not found.
* @throws ThrottlingException
* The request was throttled. Try again in a few minutes.
* @throws ValidationException
* You have provided an invalid command.
* @throws AccessDeniedException
* The current account doesn't have the IAM permissions required to perform the specified operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws Route53ProfilesException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample Route53ProfilesClient.TagResource
* @see AWS
* API Documentation
*/
default TagResourceResponse tagResource(TagResourceRequest tagResourceRequest) throws ResourceNotFoundException,
ThrottlingException, ValidationException, AccessDeniedException, AwsServiceException, SdkClientException,
Route53ProfilesException {
throw new UnsupportedOperationException();
}
/**
*
* Adds one or more tags to a specified resource.
*
*
*
* This is a convenience which creates an instance of the {@link TagResourceRequest.Builder} avoiding the need to
* create one manually via {@link TagResourceRequest#builder()}
*
*
* @param tagResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.route53profiles.model.TagResourceRequest.Builder} to create a
* request.
* @return Result of the TagResource operation returned by the service.
* @throws ResourceNotFoundException
* The resource you are associating is not found.
* @throws ThrottlingException
* The request was throttled. Try again in a few minutes.
* @throws ValidationException
* You have provided an invalid command.
* @throws AccessDeniedException
* The current account doesn't have the IAM permissions required to perform the specified operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws Route53ProfilesException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample Route53ProfilesClient.TagResource
* @see AWS
* API Documentation
*/
default TagResourceResponse tagResource(Consumer tagResourceRequest)
throws ResourceNotFoundException, ThrottlingException, ValidationException, AccessDeniedException,
AwsServiceException, SdkClientException, Route53ProfilesException {
return tagResource(TagResourceRequest.builder().applyMutation(tagResourceRequest).build());
}
/**
*
* Removes one or more tags from a specified resource.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws ResourceNotFoundException
* The resource you are associating is not found.
* @throws ThrottlingException
* The request was throttled. Try again in a few minutes.
* @throws ValidationException
* You have provided an invalid command.
* @throws AccessDeniedException
* The current account doesn't have the IAM permissions required to perform the specified operation.
* @throws ConflictException
* The request you submitted conflicts with an existing request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws Route53ProfilesException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample Route53ProfilesClient.UntagResource
* @see AWS
* API Documentation
*/
default UntagResourceResponse untagResource(UntagResourceRequest untagResourceRequest) throws ResourceNotFoundException,
ThrottlingException, ValidationException, AccessDeniedException, ConflictException, AwsServiceException,
SdkClientException, Route53ProfilesException {
throw new UnsupportedOperationException();
}
/**
*
* Removes one or more tags from a specified resource.
*
*
*
* This is a convenience which creates an instance of the {@link UntagResourceRequest.Builder} avoiding the need to
* create one manually via {@link UntagResourceRequest#builder()}
*
*
* @param untagResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.route53profiles.model.UntagResourceRequest.Builder} to create a
* request.
* @return Result of the UntagResource operation returned by the service.
* @throws ResourceNotFoundException
* The resource you are associating is not found.
* @throws ThrottlingException
* The request was throttled. Try again in a few minutes.
* @throws ValidationException
* You have provided an invalid command.
* @throws AccessDeniedException
* The current account doesn't have the IAM permissions required to perform the specified operation.
* @throws ConflictException
* The request you submitted conflicts with an existing request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws Route53ProfilesException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample Route53ProfilesClient.UntagResource
* @see AWS
* API Documentation
*/
default UntagResourceResponse untagResource(Consumer untagResourceRequest)
throws ResourceNotFoundException, ThrottlingException, ValidationException, AccessDeniedException, ConflictException,
AwsServiceException, SdkClientException, Route53ProfilesException {
return untagResource(UntagResourceRequest.builder().applyMutation(untagResourceRequest).build());
}
/**
*
* Updates the specified Route 53 Profile resourse association.
*
*
* @param updateProfileResourceAssociationRequest
* @return Result of the UpdateProfileResourceAssociation operation returned by the service.
* @throws ResourceNotFoundException
* The resource you are associating is not found.
* @throws LimitExceededException
* The request caused one or more limits to be exceeded.
* @throws InternalServiceErrorException
* An internal server error occured. Retry your request.
* @throws InvalidParameterException
* One or more parameters in this request are not valid.
* @throws ThrottlingException
* The request was throttled. Try again in a few minutes.
* @throws ValidationException
* You have provided an invalid command.
* @throws AccessDeniedException
* The current account doesn't have the IAM permissions required to perform the specified operation.
* @throws ConflictException
* The request you submitted conflicts with an existing request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws Route53ProfilesException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample Route53ProfilesClient.UpdateProfileResourceAssociation
* @see AWS API Documentation
*/
default UpdateProfileResourceAssociationResponse updateProfileResourceAssociation(
UpdateProfileResourceAssociationRequest updateProfileResourceAssociationRequest) throws ResourceNotFoundException,
LimitExceededException, InternalServiceErrorException, InvalidParameterException, ThrottlingException,
ValidationException, AccessDeniedException, ConflictException, AwsServiceException, SdkClientException,
Route53ProfilesException {
throw new UnsupportedOperationException();
}
/**
*
* Updates the specified Route 53 Profile resourse association.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateProfileResourceAssociationRequest.Builder}
* avoiding the need to create one manually via {@link UpdateProfileResourceAssociationRequest#builder()}
*
*
* @param updateProfileResourceAssociationRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.route53profiles.model.UpdateProfileResourceAssociationRequest.Builder}
* to create a request.
* @return Result of the UpdateProfileResourceAssociation operation returned by the service.
* @throws ResourceNotFoundException
* The resource you are associating is not found.
* @throws LimitExceededException
* The request caused one or more limits to be exceeded.
* @throws InternalServiceErrorException
* An internal server error occured. Retry your request.
* @throws InvalidParameterException
* One or more parameters in this request are not valid.
* @throws ThrottlingException
* The request was throttled. Try again in a few minutes.
* @throws ValidationException
* You have provided an invalid command.
* @throws AccessDeniedException
* The current account doesn't have the IAM permissions required to perform the specified operation.
* @throws ConflictException
* The request you submitted conflicts with an existing request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws Route53ProfilesException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample Route53ProfilesClient.UpdateProfileResourceAssociation
* @see AWS API Documentation
*/
default UpdateProfileResourceAssociationResponse updateProfileResourceAssociation(
Consumer updateProfileResourceAssociationRequest)
throws ResourceNotFoundException, LimitExceededException, InternalServiceErrorException, InvalidParameterException,
ThrottlingException, ValidationException, AccessDeniedException, ConflictException, AwsServiceException,
SdkClientException, Route53ProfilesException {
return updateProfileResourceAssociation(UpdateProfileResourceAssociationRequest.builder()
.applyMutation(updateProfileResourceAssociationRequest).build());
}
/**
* Create a {@link Route53ProfilesClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static Route53ProfilesClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link Route53ProfilesClient}.
*/
static Route53ProfilesClientBuilder builder() {
return new DefaultRoute53ProfilesClientBuilder();
}
static ServiceMetadata serviceMetadata() {
return ServiceMetadata.of(SERVICE_METADATA_ID);
}
@Override
default Route53ProfilesServiceClientConfiguration serviceClientConfiguration() {
throw new UnsupportedOperationException();
}
}