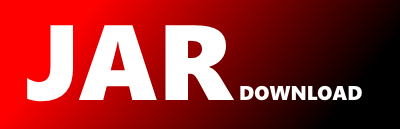
software.amazon.awssdk.services.route53recoveryreadiness.model.DNSTargetResource Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of route53recoveryreadiness Show documentation
Show all versions of route53recoveryreadiness Show documentation
The AWS Java SDK for Route53 Recovery Readiness module holds the client classes that are used for
communicating with Route53 Recovery Readiness.
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.route53recoveryreadiness.model;
import java.beans.Transient;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
* A component for DNS/Routing Control Readiness Checks
*/
@Generated("software.amazon.awssdk:codegen")
public final class DNSTargetResource implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField DOMAIN_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DomainName").getter(getter(DNSTargetResource::domainName)).setter(setter(Builder::domainName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("domainName").build()).build();
private static final SdkField HOSTED_ZONE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("HostedZoneArn").getter(getter(DNSTargetResource::hostedZoneArn)).setter(setter(Builder::hostedZoneArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("hostedZoneArn").build()).build();
private static final SdkField RECORD_SET_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("RecordSetId").getter(getter(DNSTargetResource::recordSetId)).setter(setter(Builder::recordSetId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("recordSetId").build()).build();
private static final SdkField RECORD_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("RecordType").getter(getter(DNSTargetResource::recordType)).setter(setter(Builder::recordType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("recordType").build()).build();
private static final SdkField TARGET_RESOURCE_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("TargetResource")
.getter(getter(DNSTargetResource::targetResource)).setter(setter(Builder::targetResource))
.constructor(TargetResource::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("targetResource").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(DOMAIN_NAME_FIELD,
HOSTED_ZONE_ARN_FIELD, RECORD_SET_ID_FIELD, RECORD_TYPE_FIELD, TARGET_RESOURCE_FIELD));
private static final long serialVersionUID = 1L;
private final String domainName;
private final String hostedZoneArn;
private final String recordSetId;
private final String recordType;
private final TargetResource targetResource;
private DNSTargetResource(BuilderImpl builder) {
this.domainName = builder.domainName;
this.hostedZoneArn = builder.hostedZoneArn;
this.recordSetId = builder.recordSetId;
this.recordType = builder.recordType;
this.targetResource = builder.targetResource;
}
/**
* The DNS Name that acts as ingress point to a portion of application
*
* @return The DNS Name that acts as ingress point to a portion of application
*/
public final String domainName() {
return domainName;
}
/**
* The Hosted Zone ARN that contains the DNS record with the provided name of target resource.
*
* @return The Hosted Zone ARN that contains the DNS record with the provided name of target resource.
*/
public final String hostedZoneArn() {
return hostedZoneArn;
}
/**
* The R53 Set Id to uniquely identify a record given a Name and a Type
*
* @return The R53 Set Id to uniquely identify a record given a Name and a Type
*/
public final String recordSetId() {
return recordSetId;
}
/**
* The Type of DNS Record of target resource
*
* @return The Type of DNS Record of target resource
*/
public final String recordType() {
return recordType;
}
/**
* Returns the value of the TargetResource property for this object.
*
* @return The value of the TargetResource property for this object.
*/
public final TargetResource targetResource() {
return targetResource;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(domainName());
hashCode = 31 * hashCode + Objects.hashCode(hostedZoneArn());
hashCode = 31 * hashCode + Objects.hashCode(recordSetId());
hashCode = 31 * hashCode + Objects.hashCode(recordType());
hashCode = 31 * hashCode + Objects.hashCode(targetResource());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof DNSTargetResource)) {
return false;
}
DNSTargetResource other = (DNSTargetResource) obj;
return Objects.equals(domainName(), other.domainName()) && Objects.equals(hostedZoneArn(), other.hostedZoneArn())
&& Objects.equals(recordSetId(), other.recordSetId()) && Objects.equals(recordType(), other.recordType())
&& Objects.equals(targetResource(), other.targetResource());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("DNSTargetResource").add("DomainName", domainName()).add("HostedZoneArn", hostedZoneArn())
.add("RecordSetId", recordSetId()).add("RecordType", recordType()).add("TargetResource", targetResource())
.build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "DomainName":
return Optional.ofNullable(clazz.cast(domainName()));
case "HostedZoneArn":
return Optional.ofNullable(clazz.cast(hostedZoneArn()));
case "RecordSetId":
return Optional.ofNullable(clazz.cast(recordSetId()));
case "RecordType":
return Optional.ofNullable(clazz.cast(recordType()));
case "TargetResource":
return Optional.ofNullable(clazz.cast(targetResource()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
© 2015 - 2025 Weber Informatics LLC | Privacy Policy