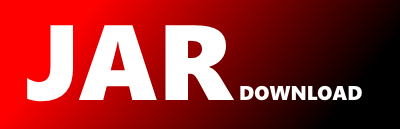
software.amazon.awssdk.services.route53recoveryreadiness.endpoints.internal.EndpointRuleset Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.route53recoveryreadiness.endpoints.internal;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.protocols.jsoncore.JsonNode;
/**
* The set of rules that are used to compute the endpoint to use for a request.
*/
@SdkInternalApi
public final class EndpointRuleset {
private static final String SERVICE_ID = "serviceId";
private static final String VERSION = "version";
private static final String PARAMETERS = "parameters";
private static final String RULES = "rules";
private final String serviceId;
private final List rules;
private final String version;
private final Parameters parameters;
private EndpointRuleset(Builder b) {
this.serviceId = b.serviceId;
this.rules = b.rules;
this.version = b.version;
this.parameters = b.parameters;
}
public String getServiceId() {
return serviceId;
}
public List getRules() {
return rules;
}
public String getVersion() {
return version;
}
public Parameters getParameters() {
return parameters;
}
public static Builder builder() {
return new Builder();
}
public static EndpointRuleset fromNode(JsonNode node) {
Builder b = builder();
Map obj = node.asObject();
JsonNode serviceIdNode = obj.get(SERVICE_ID);
if (serviceIdNode != null) {
b.serviceId(serviceIdNode.asString());
}
JsonNode versionNode = obj.get(VERSION);
if (versionNode != null) {
b.version(versionNode.asString());
}
b.parameters(Parameters.fromNode(obj.get(PARAMETERS)));
obj.get(RULES).asArray().forEach(rn -> b.addRule(Rule.fromNode(rn)));
return b.build();
}
@Override
public String toString() {
return "EndpointRuleset{" + "serviceId='" + serviceId + '\'' + ", rules=" + rules + ", version='" + version + '\''
+ ", parameters=" + parameters + '}';
}
public static class Builder {
private String serviceId;
private final List rules = new ArrayList<>();
private String version;
private Parameters parameters;
public Builder serviceId(String serviceId) {
this.serviceId = serviceId;
return this;
}
public Builder withDefaultVersion() {
this.version = "1.0";
return this;
}
public Builder version(String version) {
this.version = version;
return this;
}
public Builder addRule(Rule rule) {
rules.add(rule);
return this;
}
public Builder parameters(Parameters parameters) {
this.parameters = parameters;
return this;
}
public EndpointRuleset build() {
return new EndpointRuleset(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy