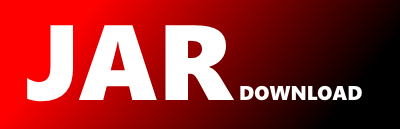
software.amazon.awssdk.services.s3.model.BucketLoggingStatus Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of s3 Show documentation
Show all versions of s3 Show documentation
The AWS Java SDK for Amazon S3 module holds the client classes that are used for communicating with
Amazon Simple Storage Service
/*
* Copyright 2012-2017 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.s3.model;
import javax.annotation.Generated;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public class BucketLoggingStatus implements ToCopyableBuilder {
private final LoggingEnabled loggingEnabled;
private BucketLoggingStatus(BuilderImpl builder) {
this.loggingEnabled = builder.loggingEnabled;
}
/**
* Returns the value of the LoggingEnabled property for this object.
*
* @return The value of the LoggingEnabled property for this object.
*/
public LoggingEnabled loggingEnabled() {
return loggingEnabled;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + ((loggingEnabled() == null) ? 0 : loggingEnabled().hashCode());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof BucketLoggingStatus)) {
return false;
}
BucketLoggingStatus other = (BucketLoggingStatus) obj;
if (other.loggingEnabled() == null ^ this.loggingEnabled() == null) {
return false;
}
if (other.loggingEnabled() != null && !other.loggingEnabled().equals(this.loggingEnabled())) {
return false;
}
return true;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (loggingEnabled() != null) {
sb.append("LoggingEnabled: ").append(loggingEnabled()).append(",");
}
sb.append("}");
return sb.toString();
}
public interface Builder extends CopyableBuilder {
/**
* Sets the value of the LoggingEnabled property for this object.
*
* @param loggingEnabled
* The new value for the LoggingEnabled property for this object.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder loggingEnabled(LoggingEnabled loggingEnabled);
}
private static final class BuilderImpl implements Builder {
private LoggingEnabled loggingEnabled;
private BuilderImpl() {
}
private BuilderImpl(BucketLoggingStatus model) {
setLoggingEnabled(model.loggingEnabled);
}
public final LoggingEnabled getLoggingEnabled() {
return loggingEnabled;
}
@Override
public final Builder loggingEnabled(LoggingEnabled loggingEnabled) {
this.loggingEnabled = loggingEnabled;
return this;
}
public final void setLoggingEnabled(LoggingEnabled loggingEnabled) {
this.loggingEnabled = loggingEnabled;
}
@Override
public BucketLoggingStatus build() {
return new BucketLoggingStatus(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy