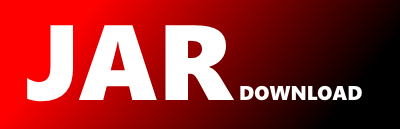
software.amazon.awssdk.services.s3.model.NoncurrentVersionTransition Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of s3 Show documentation
Show all versions of s3 Show documentation
The AWS Java SDK for Amazon S3 module holds the client classes that are used for communicating with
Amazon Simple Storage Service
/*
* Copyright 2012-2017 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.s3.model;
import javax.annotation.Generated;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
* Container for the transition rule that describes when noncurrent objects transition to the STANDARD_IA or GLACIER
* storage class. If your bucket is versioning-enabled (or versioning is suspended), you can set this action to request
* that Amazon S3 transition noncurrent object versions to the STANDARD_IA or GLACIER storage class at a specific period
* in the object's lifetime.
*/
@Generated("software.amazon.awssdk:codegen")
public class NoncurrentVersionTransition implements
ToCopyableBuilder {
private final Integer noncurrentDays;
private final String storageClass;
private NoncurrentVersionTransition(BuilderImpl builder) {
this.noncurrentDays = builder.noncurrentDays;
this.storageClass = builder.storageClass;
}
/**
* Specifies the number of days an object is noncurrent before Amazon S3 can perform the associated action. For
* information about the noncurrent days calculations, see How Amazon S3 Calculates When an
* Object Became Noncurrent in the Amazon Simple Storage Service Developer Guide.
*
* @return Specifies the number of days an object is noncurrent before Amazon S3 can perform the associated action.
* For information about the noncurrent days calculations, see How Amazon S3 Calculates
* When an Object Became Noncurrent in the Amazon Simple Storage Service Developer Guide.
*/
public Integer noncurrentDays() {
return noncurrentDays;
}
/**
* The class of storage used to store the object.
*
* @return The class of storage used to store the object.
* @see TransitionStorageClass
*/
public String storageClass() {
return storageClass;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + ((noncurrentDays() == null) ? 0 : noncurrentDays().hashCode());
hashCode = 31 * hashCode + ((storageClass() == null) ? 0 : storageClass().hashCode());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof NoncurrentVersionTransition)) {
return false;
}
NoncurrentVersionTransition other = (NoncurrentVersionTransition) obj;
if (other.noncurrentDays() == null ^ this.noncurrentDays() == null) {
return false;
}
if (other.noncurrentDays() != null && !other.noncurrentDays().equals(this.noncurrentDays())) {
return false;
}
if (other.storageClass() == null ^ this.storageClass() == null) {
return false;
}
if (other.storageClass() != null && !other.storageClass().equals(this.storageClass())) {
return false;
}
return true;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (noncurrentDays() != null) {
sb.append("NoncurrentDays: ").append(noncurrentDays()).append(",");
}
if (storageClass() != null) {
sb.append("StorageClass: ").append(storageClass()).append(",");
}
sb.append("}");
return sb.toString();
}
public interface Builder extends CopyableBuilder {
/**
* Specifies the number of days an object is noncurrent before Amazon S3 can perform the associated action. For
* information about the noncurrent days calculations, see How Amazon S3 Calculates When an
* Object Became Noncurrent in the Amazon Simple Storage Service Developer Guide.
*
* @param noncurrentDays
* Specifies the number of days an object is noncurrent before Amazon S3 can perform the associated
* action. For information about the noncurrent days calculations, see How Amazon S3 Calculates
* When an Object Became Noncurrent in the Amazon Simple Storage Service Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder noncurrentDays(Integer noncurrentDays);
/**
* The class of storage used to store the object.
*
* @param storageClass
* The class of storage used to store the object.
* @see TransitionStorageClass
* @return Returns a reference to this object so that method calls can be chained together.
* @see TransitionStorageClass
*/
Builder storageClass(String storageClass);
/**
* The class of storage used to store the object.
*
* @param storageClass
* The class of storage used to store the object.
* @see TransitionStorageClass
* @return Returns a reference to this object so that method calls can be chained together.
* @see TransitionStorageClass
*/
Builder storageClass(TransitionStorageClass storageClass);
}
private static final class BuilderImpl implements Builder {
private Integer noncurrentDays;
private String storageClass;
private BuilderImpl() {
}
private BuilderImpl(NoncurrentVersionTransition model) {
setNoncurrentDays(model.noncurrentDays);
setStorageClass(model.storageClass);
}
public final Integer getNoncurrentDays() {
return noncurrentDays;
}
@Override
public final Builder noncurrentDays(Integer noncurrentDays) {
this.noncurrentDays = noncurrentDays;
return this;
}
public final void setNoncurrentDays(Integer noncurrentDays) {
this.noncurrentDays = noncurrentDays;
}
public final String getStorageClass() {
return storageClass;
}
@Override
public final Builder storageClass(String storageClass) {
this.storageClass = storageClass;
return this;
}
@Override
public final Builder storageClass(TransitionStorageClass storageClass) {
this.storageClass(storageClass.toString());
return this;
}
public final void setStorageClass(String storageClass) {
this.storageClass = storageClass;
}
public final void setStorageClass(TransitionStorageClass storageClass) {
this.storageClass(storageClass.toString());
}
@Override
public NoncurrentVersionTransition build() {
return new NoncurrentVersionTransition(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy