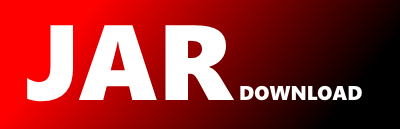
software.amazon.awssdk.services.s3.model.NotificationConfiguration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of s3 Show documentation
Show all versions of s3 Show documentation
The AWS Java SDK for Amazon S3 module holds the client classes that are used for communicating with
Amazon Simple Storage Service
/*
* Copyright 2012-2017 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.s3.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.List;
import javax.annotation.Generated;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
* Container for specifying the notification configuration of the bucket. If this element is empty, notifications are
* turned off on the bucket.
*/
@Generated("software.amazon.awssdk:codegen")
public class NotificationConfiguration implements ToCopyableBuilder {
private final List topicConfigurations;
private final List queueConfigurations;
private final List lambdaFunctionConfigurations;
private NotificationConfiguration(BuilderImpl builder) {
this.topicConfigurations = builder.topicConfigurations;
this.queueConfigurations = builder.queueConfigurations;
this.lambdaFunctionConfigurations = builder.lambdaFunctionConfigurations;
}
/**
* Returns the value of the TopicConfigurations property for this object.
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return The value of the TopicConfigurations property for this object.
*/
public List topicConfigurations() {
return topicConfigurations;
}
/**
* Returns the value of the QueueConfigurations property for this object.
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return The value of the QueueConfigurations property for this object.
*/
public List queueConfigurations() {
return queueConfigurations;
}
/**
* Returns the value of the LambdaFunctionConfigurations property for this object.
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return The value of the LambdaFunctionConfigurations property for this object.
*/
public List lambdaFunctionConfigurations() {
return lambdaFunctionConfigurations;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + ((topicConfigurations() == null) ? 0 : topicConfigurations().hashCode());
hashCode = 31 * hashCode + ((queueConfigurations() == null) ? 0 : queueConfigurations().hashCode());
hashCode = 31 * hashCode + ((lambdaFunctionConfigurations() == null) ? 0 : lambdaFunctionConfigurations().hashCode());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof NotificationConfiguration)) {
return false;
}
NotificationConfiguration other = (NotificationConfiguration) obj;
if (other.topicConfigurations() == null ^ this.topicConfigurations() == null) {
return false;
}
if (other.topicConfigurations() != null && !other.topicConfigurations().equals(this.topicConfigurations())) {
return false;
}
if (other.queueConfigurations() == null ^ this.queueConfigurations() == null) {
return false;
}
if (other.queueConfigurations() != null && !other.queueConfigurations().equals(this.queueConfigurations())) {
return false;
}
if (other.lambdaFunctionConfigurations() == null ^ this.lambdaFunctionConfigurations() == null) {
return false;
}
if (other.lambdaFunctionConfigurations() != null
&& !other.lambdaFunctionConfigurations().equals(this.lambdaFunctionConfigurations())) {
return false;
}
return true;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (topicConfigurations() != null) {
sb.append("TopicConfigurations: ").append(topicConfigurations()).append(",");
}
if (queueConfigurations() != null) {
sb.append("QueueConfigurations: ").append(queueConfigurations()).append(",");
}
if (lambdaFunctionConfigurations() != null) {
sb.append("LambdaFunctionConfigurations: ").append(lambdaFunctionConfigurations()).append(",");
}
sb.append("}");
return sb.toString();
}
public interface Builder extends CopyableBuilder {
/**
* Sets the value of the TopicConfigurations property for this object.
*
* @param topicConfigurations
* The new value for the TopicConfigurations property for this object.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder topicConfigurations(Collection topicConfigurations);
/**
* Sets the value of the TopicConfigurations property for this object.
*
* @param topicConfigurations
* The new value for the TopicConfigurations property for this object.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder topicConfigurations(TopicConfiguration... topicConfigurations);
/**
* Sets the value of the QueueConfigurations property for this object.
*
* @param queueConfigurations
* The new value for the QueueConfigurations property for this object.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder queueConfigurations(Collection queueConfigurations);
/**
* Sets the value of the QueueConfigurations property for this object.
*
* @param queueConfigurations
* The new value for the QueueConfigurations property for this object.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder queueConfigurations(QueueConfiguration... queueConfigurations);
/**
* Sets the value of the LambdaFunctionConfigurations property for this object.
*
* @param lambdaFunctionConfigurations
* The new value for the LambdaFunctionConfigurations property for this object.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder lambdaFunctionConfigurations(Collection lambdaFunctionConfigurations);
/**
* Sets the value of the LambdaFunctionConfigurations property for this object.
*
* @param lambdaFunctionConfigurations
* The new value for the LambdaFunctionConfigurations property for this object.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder lambdaFunctionConfigurations(LambdaFunctionConfiguration... lambdaFunctionConfigurations);
}
private static final class BuilderImpl implements Builder {
private List topicConfigurations;
private List queueConfigurations;
private List lambdaFunctionConfigurations;
private BuilderImpl() {
}
private BuilderImpl(NotificationConfiguration model) {
setTopicConfigurations(model.topicConfigurations);
setQueueConfigurations(model.queueConfigurations);
setLambdaFunctionConfigurations(model.lambdaFunctionConfigurations);
}
public final Collection getTopicConfigurations() {
return topicConfigurations;
}
@Override
public final Builder topicConfigurations(Collection topicConfigurations) {
this.topicConfigurations = TopicConfigurationListCopier.copy(topicConfigurations);
return this;
}
@Override
@SafeVarargs
public final Builder topicConfigurations(TopicConfiguration... topicConfigurations) {
topicConfigurations(Arrays.asList(topicConfigurations));
return this;
}
public final void setTopicConfigurations(Collection topicConfigurations) {
this.topicConfigurations = TopicConfigurationListCopier.copy(topicConfigurations);
}
@SafeVarargs
public final void setTopicConfigurations(TopicConfiguration... topicConfigurations) {
topicConfigurations(Arrays.asList(topicConfigurations));
}
public final Collection getQueueConfigurations() {
return queueConfigurations;
}
@Override
public final Builder queueConfigurations(Collection queueConfigurations) {
this.queueConfigurations = QueueConfigurationListCopier.copy(queueConfigurations);
return this;
}
@Override
@SafeVarargs
public final Builder queueConfigurations(QueueConfiguration... queueConfigurations) {
queueConfigurations(Arrays.asList(queueConfigurations));
return this;
}
public final void setQueueConfigurations(Collection queueConfigurations) {
this.queueConfigurations = QueueConfigurationListCopier.copy(queueConfigurations);
}
@SafeVarargs
public final void setQueueConfigurations(QueueConfiguration... queueConfigurations) {
queueConfigurations(Arrays.asList(queueConfigurations));
}
public final Collection getLambdaFunctionConfigurations() {
return lambdaFunctionConfigurations;
}
@Override
public final Builder lambdaFunctionConfigurations(Collection lambdaFunctionConfigurations) {
this.lambdaFunctionConfigurations = LambdaFunctionConfigurationListCopier.copy(lambdaFunctionConfigurations);
return this;
}
@Override
@SafeVarargs
public final Builder lambdaFunctionConfigurations(LambdaFunctionConfiguration... lambdaFunctionConfigurations) {
lambdaFunctionConfigurations(Arrays.asList(lambdaFunctionConfigurations));
return this;
}
public final void setLambdaFunctionConfigurations(Collection lambdaFunctionConfigurations) {
this.lambdaFunctionConfigurations = LambdaFunctionConfigurationListCopier.copy(lambdaFunctionConfigurations);
}
@SafeVarargs
public final void setLambdaFunctionConfigurations(LambdaFunctionConfiguration... lambdaFunctionConfigurations) {
lambdaFunctionConfigurations(Arrays.asList(lambdaFunctionConfigurations));
}
@Override
public NotificationConfiguration build() {
return new NotificationConfiguration(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy