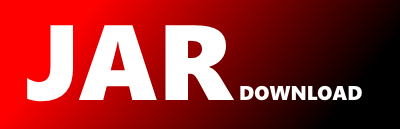
software.amazon.awssdk.services.s3.model.QueueConfiguration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of s3 Show documentation
Show all versions of s3 Show documentation
The AWS Java SDK for Amazon S3 module holds the client classes that are used for communicating with
Amazon Simple Storage Service
/*
* Copyright 2012-2017 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.s3.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.List;
import java.util.stream.Collectors;
import javax.annotation.Generated;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
* Container for specifying an configuration when you want Amazon S3 to publish events to an Amazon Simple Queue Service
* (Amazon SQS) queue.
*/
@Generated("software.amazon.awssdk:codegen")
public class QueueConfiguration implements ToCopyableBuilder {
private final String id;
private final String queueArn;
private final List events;
private final NotificationConfigurationFilter filter;
private QueueConfiguration(BuilderImpl builder) {
this.id = builder.id;
this.queueArn = builder.queueArn;
this.events = builder.events;
this.filter = builder.filter;
}
/**
* Returns the value of the Id property for this object.
*
* @return The value of the Id property for this object.
*/
public String id() {
return id;
}
/**
* Amazon SQS queue ARN to which Amazon S3 will publish a message when it detects events of specified type.
*
* @return Amazon SQS queue ARN to which Amazon S3 will publish a message when it detects events of specified type.
*/
public String queueArn() {
return queueArn;
}
/**
* Returns the value of the Events property for this object.
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return The value of the Events property for this object.
* @see Event
*/
public List events() {
return events;
}
/**
* Returns the value of the Filter property for this object.
*
* @return The value of the Filter property for this object.
*/
public NotificationConfigurationFilter filter() {
return filter;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + ((id() == null) ? 0 : id().hashCode());
hashCode = 31 * hashCode + ((queueArn() == null) ? 0 : queueArn().hashCode());
hashCode = 31 * hashCode + ((events() == null) ? 0 : events().hashCode());
hashCode = 31 * hashCode + ((filter() == null) ? 0 : filter().hashCode());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof QueueConfiguration)) {
return false;
}
QueueConfiguration other = (QueueConfiguration) obj;
if (other.id() == null ^ this.id() == null) {
return false;
}
if (other.id() != null && !other.id().equals(this.id())) {
return false;
}
if (other.queueArn() == null ^ this.queueArn() == null) {
return false;
}
if (other.queueArn() != null && !other.queueArn().equals(this.queueArn())) {
return false;
}
if (other.events() == null ^ this.events() == null) {
return false;
}
if (other.events() != null && !other.events().equals(this.events())) {
return false;
}
if (other.filter() == null ^ this.filter() == null) {
return false;
}
if (other.filter() != null && !other.filter().equals(this.filter())) {
return false;
}
return true;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (id() != null) {
sb.append("Id: ").append(id()).append(",");
}
if (queueArn() != null) {
sb.append("QueueArn: ").append(queueArn()).append(",");
}
if (events() != null) {
sb.append("Events: ").append(events()).append(",");
}
if (filter() != null) {
sb.append("Filter: ").append(filter()).append(",");
}
sb.append("}");
return sb.toString();
}
public interface Builder extends CopyableBuilder {
/**
* Sets the value of the Id property for this object.
*
* @param id
* The new value for the Id property for this object.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder id(String id);
/**
* Amazon SQS queue ARN to which Amazon S3 will publish a message when it detects events of specified type.
*
* @param queueArn
* Amazon SQS queue ARN to which Amazon S3 will publish a message when it detects events of specified
* type.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder queueArn(String queueArn);
/**
* Sets the value of the Events property for this object.
*
* @param events
* The new value for the Events property for this object.
* @see Event
* @return Returns a reference to this object so that method calls can be chained together.
* @see Event
*/
Builder events(Collection events);
/**
* Sets the value of the Events property for this object.
*
* @param events
* The new value for the Events property for this object.
* @see Event
* @return Returns a reference to this object so that method calls can be chained together.
* @see Event
*/
Builder events(String... events);
/**
* Sets the value of the Events property for this object.
*
* @param events
* The new value for the Events property for this object.
* @see Event
* @return Returns a reference to this object so that method calls can be chained together.
* @see Event
*/
Builder events(Event... events);
/**
* Sets the value of the Filter property for this object.
*
* @param filter
* The new value for the Filter property for this object.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder filter(NotificationConfigurationFilter filter);
}
private static final class BuilderImpl implements Builder {
private String id;
private String queueArn;
private List events;
private NotificationConfigurationFilter filter;
private BuilderImpl() {
}
private BuilderImpl(QueueConfiguration model) {
setId(model.id);
setQueueArn(model.queueArn);
setEvents(model.events);
setFilter(model.filter);
}
public final String getId() {
return id;
}
@Override
public final Builder id(String id) {
this.id = id;
return this;
}
public final void setId(String id) {
this.id = id;
}
public final String getQueueArn() {
return queueArn;
}
@Override
public final Builder queueArn(String queueArn) {
this.queueArn = queueArn;
return this;
}
public final void setQueueArn(String queueArn) {
this.queueArn = queueArn;
}
public final Collection getEvents() {
return events;
}
@Override
public final Builder events(Collection events) {
this.events = EventListCopier.copy(events);
return this;
}
@Override
@SafeVarargs
public final Builder events(String... events) {
events(Arrays.asList(events));
return this;
}
@Override
@SafeVarargs
public final Builder events(Event... events) {
events(Arrays.asList(events).stream().map(Object::toString).collect(Collectors.toList()));
return this;
}
public final void setEvents(Collection events) {
this.events = EventListCopier.copy(events);
}
@SafeVarargs
public final void setEvents(String... events) {
events(Arrays.asList(events));
}
@SafeVarargs
public final void setEvents(Event... events) {
events(Arrays.asList(events).stream().map(Object::toString).collect(Collectors.toList()));
}
public final NotificationConfigurationFilter getFilter() {
return filter;
}
@Override
public final Builder filter(NotificationConfigurationFilter filter) {
this.filter = filter;
return this;
}
public final void setFilter(NotificationConfigurationFilter filter) {
this.filter = filter;
}
@Override
public QueueConfiguration build() {
return new QueueConfiguration(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy