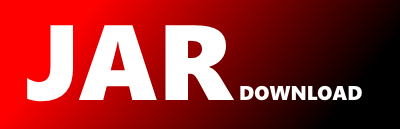
software.amazon.awssdk.services.s3.model.HeadObjectRequest Maven / Gradle / Ivy
Show all versions of s3 Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.s3.model;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class HeadObjectRequest extends S3Request implements ToCopyableBuilder {
private static final SdkField BUCKET_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("Bucket")
.getter(getter(HeadObjectRequest::bucket))
.setter(setter(Builder::bucket))
.traits(LocationTrait.builder().location(MarshallLocation.PATH).locationName("Bucket")
.unmarshallLocationName("Bucket").build()).build();
private static final SdkField IF_MATCH_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("IfMatch")
.getter(getter(HeadObjectRequest::ifMatch))
.setter(setter(Builder::ifMatch))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("If-Match")
.unmarshallLocationName("If-Match").build()).build();
private static final SdkField IF_MODIFIED_SINCE_FIELD = SdkField
. builder(MarshallingType.INSTANT)
.memberName("IfModifiedSince")
.getter(getter(HeadObjectRequest::ifModifiedSince))
.setter(setter(Builder::ifModifiedSince))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("If-Modified-Since")
.unmarshallLocationName("If-Modified-Since").build()).build();
private static final SdkField IF_NONE_MATCH_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("IfNoneMatch")
.getter(getter(HeadObjectRequest::ifNoneMatch))
.setter(setter(Builder::ifNoneMatch))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("If-None-Match")
.unmarshallLocationName("If-None-Match").build()).build();
private static final SdkField IF_UNMODIFIED_SINCE_FIELD = SdkField
. builder(MarshallingType.INSTANT)
.memberName("IfUnmodifiedSince")
.getter(getter(HeadObjectRequest::ifUnmodifiedSince))
.setter(setter(Builder::ifUnmodifiedSince))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("If-Unmodified-Since")
.unmarshallLocationName("If-Unmodified-Since").build()).build();
private static final SdkField KEY_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("Key")
.getter(getter(HeadObjectRequest::key))
.setter(setter(Builder::key))
.traits(LocationTrait.builder().location(MarshallLocation.GREEDY_PATH).locationName("Key")
.unmarshallLocationName("Key").build()).build();
private static final SdkField RANGE_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("Range")
.getter(getter(HeadObjectRequest::range))
.setter(setter(Builder::range))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("Range")
.unmarshallLocationName("Range").build()).build();
private static final SdkField VERSION_ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("VersionId")
.getter(getter(HeadObjectRequest::versionId))
.setter(setter(Builder::versionId))
.traits(LocationTrait.builder().location(MarshallLocation.QUERY_PARAM).locationName("versionId")
.unmarshallLocationName("versionId").build()).build();
private static final SdkField SSE_CUSTOMER_ALGORITHM_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("SSECustomerAlgorithm")
.getter(getter(HeadObjectRequest::sseCustomerAlgorithm))
.setter(setter(Builder::sseCustomerAlgorithm))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER)
.locationName("x-amz-server-side-encryption-customer-algorithm")
.unmarshallLocationName("x-amz-server-side-encryption-customer-algorithm").build()).build();
private static final SdkField SSE_CUSTOMER_KEY_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("SSECustomerKey")
.getter(getter(HeadObjectRequest::sseCustomerKey))
.setter(setter(Builder::sseCustomerKey))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER)
.locationName("x-amz-server-side-encryption-customer-key")
.unmarshallLocationName("x-amz-server-side-encryption-customer-key").build()).build();
private static final SdkField SSE_CUSTOMER_KEY_MD5_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("SSECustomerKeyMD5")
.getter(getter(HeadObjectRequest::sseCustomerKeyMD5))
.setter(setter(Builder::sseCustomerKeyMD5))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER)
.locationName("x-amz-server-side-encryption-customer-key-MD5")
.unmarshallLocationName("x-amz-server-side-encryption-customer-key-MD5").build()).build();
private static final SdkField REQUEST_PAYER_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("RequestPayer")
.getter(getter(HeadObjectRequest::requestPayerAsString))
.setter(setter(Builder::requestPayer))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("x-amz-request-payer")
.unmarshallLocationName("x-amz-request-payer").build()).build();
private static final SdkField PART_NUMBER_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("PartNumber")
.getter(getter(HeadObjectRequest::partNumber))
.setter(setter(Builder::partNumber))
.traits(LocationTrait.builder().location(MarshallLocation.QUERY_PARAM).locationName("partNumber")
.unmarshallLocationName("partNumber").build()).build();
private static final SdkField EXPECTED_BUCKET_OWNER_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("ExpectedBucketOwner")
.getter(getter(HeadObjectRequest::expectedBucketOwner))
.setter(setter(Builder::expectedBucketOwner))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("x-amz-expected-bucket-owner")
.unmarshallLocationName("x-amz-expected-bucket-owner").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(BUCKET_FIELD, IF_MATCH_FIELD,
IF_MODIFIED_SINCE_FIELD, IF_NONE_MATCH_FIELD, IF_UNMODIFIED_SINCE_FIELD, KEY_FIELD, RANGE_FIELD, VERSION_ID_FIELD,
SSE_CUSTOMER_ALGORITHM_FIELD, SSE_CUSTOMER_KEY_FIELD, SSE_CUSTOMER_KEY_MD5_FIELD, REQUEST_PAYER_FIELD,
PART_NUMBER_FIELD, EXPECTED_BUCKET_OWNER_FIELD));
private final String bucket;
private final String ifMatch;
private final Instant ifModifiedSince;
private final String ifNoneMatch;
private final Instant ifUnmodifiedSince;
private final String key;
private final String range;
private final String versionId;
private final String sseCustomerAlgorithm;
private final String sseCustomerKey;
private final String sseCustomerKeyMD5;
private final String requestPayer;
private final Integer partNumber;
private final String expectedBucketOwner;
private HeadObjectRequest(BuilderImpl builder) {
super(builder);
this.bucket = builder.bucket;
this.ifMatch = builder.ifMatch;
this.ifModifiedSince = builder.ifModifiedSince;
this.ifNoneMatch = builder.ifNoneMatch;
this.ifUnmodifiedSince = builder.ifUnmodifiedSince;
this.key = builder.key;
this.range = builder.range;
this.versionId = builder.versionId;
this.sseCustomerAlgorithm = builder.sseCustomerAlgorithm;
this.sseCustomerKey = builder.sseCustomerKey;
this.sseCustomerKeyMD5 = builder.sseCustomerKeyMD5;
this.requestPayer = builder.requestPayer;
this.partNumber = builder.partNumber;
this.expectedBucketOwner = builder.expectedBucketOwner;
}
/**
*
* The name of the bucket containing the object.
*
*
* When using this API with an access point, you must direct requests to the access point hostname. The access point
* hostname takes the form AccessPointName-AccountId.s3-accesspoint.Region.amazonaws.com. When
* using this operation with an access point through the AWS SDKs, you provide the access point ARN in place of the
* bucket name. For more information about access point ARNs, see Using Access Points in the
* Amazon Simple Storage Service Developer Guide.
*
*
* When using this API with Amazon S3 on Outposts, you must direct requests to the S3 on Outposts hostname. The S3
* on Outposts hostname takes the form
* AccessPointName-AccountId.outpostID.s3-outposts.Region.amazonaws.com. When using this
* operation using S3 on Outposts through the AWS SDKs, you provide the Outposts bucket ARN in place of the bucket
* name. For more information about S3 on Outposts ARNs, see Using S3 on Outposts in the
* Amazon Simple Storage Service Developer Guide.
*
*
* @return The name of the bucket containing the object.
*
* When using this API with an access point, you must direct requests to the access point hostname. The
* access point hostname takes the form
* AccessPointName-AccountId.s3-accesspoint.Region.amazonaws.com. When using this
* operation with an access point through the AWS SDKs, you provide the access point ARN in place of the
* bucket name. For more information about access point ARNs, see Using Access Points
* in the Amazon Simple Storage Service Developer Guide.
*
*
* When using this API with Amazon S3 on Outposts, you must direct requests to the S3 on Outposts hostname.
* The S3 on Outposts hostname takes the form
* AccessPointName-AccountId.outpostID.s3-outposts.Region.amazonaws.com. When
* using this operation using S3 on Outposts through the AWS SDKs, you provide the Outposts bucket ARN in
* place of the bucket name. For more information about S3 on Outposts ARNs, see Using S3 on Outposts in the
* Amazon Simple Storage Service Developer Guide.
*/
public String bucket() {
return bucket;
}
/**
*
* Return the object only if its entity tag (ETag) is the same as the one specified, otherwise return a 412
* (precondition failed).
*
*
* @return Return the object only if its entity tag (ETag) is the same as the one specified, otherwise return a 412
* (precondition failed).
*/
public String ifMatch() {
return ifMatch;
}
/**
*
* Return the object only if it has been modified since the specified time, otherwise return a 304 (not modified).
*
*
* @return Return the object only if it has been modified since the specified time, otherwise return a 304 (not
* modified).
*/
public Instant ifModifiedSince() {
return ifModifiedSince;
}
/**
*
* Return the object only if its entity tag (ETag) is different from the one specified, otherwise return a 304 (not
* modified).
*
*
* @return Return the object only if its entity tag (ETag) is different from the one specified, otherwise return a
* 304 (not modified).
*/
public String ifNoneMatch() {
return ifNoneMatch;
}
/**
*
* Return the object only if it has not been modified since the specified time, otherwise return a 412 (precondition
* failed).
*
*
* @return Return the object only if it has not been modified since the specified time, otherwise return a 412
* (precondition failed).
*/
public Instant ifUnmodifiedSince() {
return ifUnmodifiedSince;
}
/**
*
* The object key.
*
*
* @return The object key.
*/
public String key() {
return key;
}
/**
*
* Downloads the specified range bytes of an object. For more information about the HTTP Range header, see http://www.w3.org/Protocols/rfc2616/rfc2616-sec14.html#sec14.35.
*
*
*
* Amazon S3 doesn't support retrieving multiple ranges of data per GET
request.
*
*
*
* @return Downloads the specified range bytes of an object. For more information about the HTTP Range header, see
* http://www.w3.org/Protocols/
* rfc2616/rfc2616-sec14.html#sec14.35.
*
* Amazon S3 doesn't support retrieving multiple ranges of data per GET
request.
*
*/
public String range() {
return range;
}
/**
*
* VersionId used to reference a specific version of the object.
*
*
* @return VersionId used to reference a specific version of the object.
*/
public String versionId() {
return versionId;
}
/**
*
* Specifies the algorithm to use to when encrypting the object (for example, AES256).
*
*
* @return Specifies the algorithm to use to when encrypting the object (for example, AES256).
*/
public String sseCustomerAlgorithm() {
return sseCustomerAlgorithm;
}
/**
*
* Specifies the customer-provided encryption key for Amazon S3 to use in encrypting data. This value is used to
* store the object and then it is discarded; Amazon S3 does not store the encryption key. The key must be
* appropriate for use with the algorithm specified in the
* x-amz-server-side-encryption-customer-algorithm
header.
*
*
* @return Specifies the customer-provided encryption key for Amazon S3 to use in encrypting data. This value is
* used to store the object and then it is discarded; Amazon S3 does not store the encryption key. The key
* must be appropriate for use with the algorithm specified in the
* x-amz-server-side-encryption-customer-algorithm
header.
*/
public String sseCustomerKey() {
return sseCustomerKey;
}
/**
*
* Specifies the 128-bit MD5 digest of the encryption key according to RFC 1321. Amazon S3 uses this header for a
* message integrity check to ensure that the encryption key was transmitted without error.
*
*
* @return Specifies the 128-bit MD5 digest of the encryption key according to RFC 1321. Amazon S3 uses this header
* for a message integrity check to ensure that the encryption key was transmitted without error.
*/
public String sseCustomerKeyMD5() {
return sseCustomerKeyMD5;
}
/**
* Returns the value of the RequestPayer property for this object.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #requestPayer} will
* return {@link RequestPayer#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #requestPayerAsString}.
*
*
* @return The value of the RequestPayer property for this object.
* @see RequestPayer
*/
public RequestPayer requestPayer() {
return RequestPayer.fromValue(requestPayer);
}
/**
* Returns the value of the RequestPayer property for this object.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #requestPayer} will
* return {@link RequestPayer#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #requestPayerAsString}.
*
*
* @return The value of the RequestPayer property for this object.
* @see RequestPayer
*/
public String requestPayerAsString() {
return requestPayer;
}
/**
*
* Part number of the object being read. This is a positive integer between 1 and 10,000. Effectively performs a
* 'ranged' HEAD request for the part specified. Useful querying about the size of the part and the number of parts
* in this object.
*
*
* @return Part number of the object being read. This is a positive integer between 1 and 10,000. Effectively
* performs a 'ranged' HEAD request for the part specified. Useful querying about the size of the part and
* the number of parts in this object.
*/
public Integer partNumber() {
return partNumber;
}
/**
*
* The account id of the expected bucket owner. If the bucket is owned by a different account, the request will fail
* with an HTTP 403 (Access Denied)
error.
*
*
* @return The account id of the expected bucket owner. If the bucket is owned by a different account, the request
* will fail with an HTTP 403 (Access Denied)
error.
*/
public String expectedBucketOwner() {
return expectedBucketOwner;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(bucket());
hashCode = 31 * hashCode + Objects.hashCode(ifMatch());
hashCode = 31 * hashCode + Objects.hashCode(ifModifiedSince());
hashCode = 31 * hashCode + Objects.hashCode(ifNoneMatch());
hashCode = 31 * hashCode + Objects.hashCode(ifUnmodifiedSince());
hashCode = 31 * hashCode + Objects.hashCode(key());
hashCode = 31 * hashCode + Objects.hashCode(range());
hashCode = 31 * hashCode + Objects.hashCode(versionId());
hashCode = 31 * hashCode + Objects.hashCode(sseCustomerAlgorithm());
hashCode = 31 * hashCode + Objects.hashCode(sseCustomerKey());
hashCode = 31 * hashCode + Objects.hashCode(sseCustomerKeyMD5());
hashCode = 31 * hashCode + Objects.hashCode(requestPayerAsString());
hashCode = 31 * hashCode + Objects.hashCode(partNumber());
hashCode = 31 * hashCode + Objects.hashCode(expectedBucketOwner());
return hashCode;
}
@Override
public boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof HeadObjectRequest)) {
return false;
}
HeadObjectRequest other = (HeadObjectRequest) obj;
return Objects.equals(bucket(), other.bucket()) && Objects.equals(ifMatch(), other.ifMatch())
&& Objects.equals(ifModifiedSince(), other.ifModifiedSince())
&& Objects.equals(ifNoneMatch(), other.ifNoneMatch())
&& Objects.equals(ifUnmodifiedSince(), other.ifUnmodifiedSince()) && Objects.equals(key(), other.key())
&& Objects.equals(range(), other.range()) && Objects.equals(versionId(), other.versionId())
&& Objects.equals(sseCustomerAlgorithm(), other.sseCustomerAlgorithm())
&& Objects.equals(sseCustomerKey(), other.sseCustomerKey())
&& Objects.equals(sseCustomerKeyMD5(), other.sseCustomerKeyMD5())
&& Objects.equals(requestPayerAsString(), other.requestPayerAsString())
&& Objects.equals(partNumber(), other.partNumber())
&& Objects.equals(expectedBucketOwner(), other.expectedBucketOwner());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("HeadObjectRequest").add("Bucket", bucket()).add("IfMatch", ifMatch())
.add("IfModifiedSince", ifModifiedSince()).add("IfNoneMatch", ifNoneMatch())
.add("IfUnmodifiedSince", ifUnmodifiedSince()).add("Key", key()).add("Range", range())
.add("VersionId", versionId()).add("SSECustomerAlgorithm", sseCustomerAlgorithm())
.add("SSECustomerKey", sseCustomerKey() == null ? null : "*** Sensitive Data Redacted ***")
.add("SSECustomerKeyMD5", sseCustomerKeyMD5()).add("RequestPayer", requestPayerAsString())
.add("PartNumber", partNumber()).add("ExpectedBucketOwner", expectedBucketOwner()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Bucket":
return Optional.ofNullable(clazz.cast(bucket()));
case "IfMatch":
return Optional.ofNullable(clazz.cast(ifMatch()));
case "IfModifiedSince":
return Optional.ofNullable(clazz.cast(ifModifiedSince()));
case "IfNoneMatch":
return Optional.ofNullable(clazz.cast(ifNoneMatch()));
case "IfUnmodifiedSince":
return Optional.ofNullable(clazz.cast(ifUnmodifiedSince()));
case "Key":
return Optional.ofNullable(clazz.cast(key()));
case "Range":
return Optional.ofNullable(clazz.cast(range()));
case "VersionId":
return Optional.ofNullable(clazz.cast(versionId()));
case "SSECustomerAlgorithm":
return Optional.ofNullable(clazz.cast(sseCustomerAlgorithm()));
case "SSECustomerKey":
return Optional.ofNullable(clazz.cast(sseCustomerKey()));
case "SSECustomerKeyMD5":
return Optional.ofNullable(clazz.cast(sseCustomerKeyMD5()));
case "RequestPayer":
return Optional.ofNullable(clazz.cast(requestPayerAsString()));
case "PartNumber":
return Optional.ofNullable(clazz.cast(partNumber()));
case "ExpectedBucketOwner":
return Optional.ofNullable(clazz.cast(expectedBucketOwner()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function