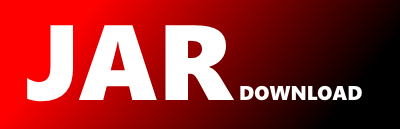
software.amazon.awssdk.services.s3.model.ListObjectVersionsResponse Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.s3.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class ListObjectVersionsResponse extends S3Response implements
ToCopyableBuilder {
private static final SdkField IS_TRUNCATED_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("IsTruncated")
.getter(getter(ListObjectVersionsResponse::isTruncated))
.setter(setter(Builder::isTruncated))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IsTruncated")
.unmarshallLocationName("IsTruncated").build()).build();
private static final SdkField KEY_MARKER_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("KeyMarker")
.getter(getter(ListObjectVersionsResponse::keyMarker))
.setter(setter(Builder::keyMarker))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("KeyMarker")
.unmarshallLocationName("KeyMarker").build()).build();
private static final SdkField VERSION_ID_MARKER_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("VersionIdMarker")
.getter(getter(ListObjectVersionsResponse::versionIdMarker))
.setter(setter(Builder::versionIdMarker))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("VersionIdMarker")
.unmarshallLocationName("VersionIdMarker").build()).build();
private static final SdkField NEXT_KEY_MARKER_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("NextKeyMarker")
.getter(getter(ListObjectVersionsResponse::nextKeyMarker))
.setter(setter(Builder::nextKeyMarker))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NextKeyMarker")
.unmarshallLocationName("NextKeyMarker").build()).build();
private static final SdkField NEXT_VERSION_ID_MARKER_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("NextVersionIdMarker")
.getter(getter(ListObjectVersionsResponse::nextVersionIdMarker))
.setter(setter(Builder::nextVersionIdMarker))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NextVersionIdMarker")
.unmarshallLocationName("NextVersionIdMarker").build()).build();
private static final SdkField> VERSIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Versions")
.getter(getter(ListObjectVersionsResponse::versions))
.setter(setter(Builder::versions))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Version")
.unmarshallLocationName("Version").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(ObjectVersion::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").unmarshallLocationName("member").build()).build())
.isFlattened(true).build()).build();
private static final SdkField> DELETE_MARKERS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("DeleteMarkers")
.getter(getter(ListObjectVersionsResponse::deleteMarkers))
.setter(setter(Builder::deleteMarkers))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DeleteMarker")
.unmarshallLocationName("DeleteMarker").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(DeleteMarkerEntry::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").unmarshallLocationName("member").build()).build())
.isFlattened(true).build()).build();
private static final SdkField NAME_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("Name")
.getter(getter(ListObjectVersionsResponse::name))
.setter(setter(Builder::name))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Name")
.unmarshallLocationName("Name").build()).build();
private static final SdkField PREFIX_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("Prefix")
.getter(getter(ListObjectVersionsResponse::prefix))
.setter(setter(Builder::prefix))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Prefix")
.unmarshallLocationName("Prefix").build()).build();
private static final SdkField DELIMITER_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("Delimiter")
.getter(getter(ListObjectVersionsResponse::delimiter))
.setter(setter(Builder::delimiter))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Delimiter")
.unmarshallLocationName("Delimiter").build()).build();
private static final SdkField MAX_KEYS_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("MaxKeys")
.getter(getter(ListObjectVersionsResponse::maxKeys))
.setter(setter(Builder::maxKeys))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MaxKeys")
.unmarshallLocationName("MaxKeys").build()).build();
private static final SdkField> COMMON_PREFIXES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("CommonPrefixes")
.getter(getter(ListObjectVersionsResponse::commonPrefixes))
.setter(setter(Builder::commonPrefixes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CommonPrefixes")
.unmarshallLocationName("CommonPrefixes").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(CommonPrefix::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").unmarshallLocationName("member").build()).build())
.isFlattened(true).build()).build();
private static final SdkField ENCODING_TYPE_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("EncodingType")
.getter(getter(ListObjectVersionsResponse::encodingTypeAsString))
.setter(setter(Builder::encodingType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EncodingType")
.unmarshallLocationName("EncodingType").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(IS_TRUNCATED_FIELD,
KEY_MARKER_FIELD, VERSION_ID_MARKER_FIELD, NEXT_KEY_MARKER_FIELD, NEXT_VERSION_ID_MARKER_FIELD, VERSIONS_FIELD,
DELETE_MARKERS_FIELD, NAME_FIELD, PREFIX_FIELD, DELIMITER_FIELD, MAX_KEYS_FIELD, COMMON_PREFIXES_FIELD,
ENCODING_TYPE_FIELD));
private final Boolean isTruncated;
private final String keyMarker;
private final String versionIdMarker;
private final String nextKeyMarker;
private final String nextVersionIdMarker;
private final List versions;
private final List deleteMarkers;
private final String name;
private final String prefix;
private final String delimiter;
private final Integer maxKeys;
private final List commonPrefixes;
private final String encodingType;
private ListObjectVersionsResponse(BuilderImpl builder) {
super(builder);
this.isTruncated = builder.isTruncated;
this.keyMarker = builder.keyMarker;
this.versionIdMarker = builder.versionIdMarker;
this.nextKeyMarker = builder.nextKeyMarker;
this.nextVersionIdMarker = builder.nextVersionIdMarker;
this.versions = builder.versions;
this.deleteMarkers = builder.deleteMarkers;
this.name = builder.name;
this.prefix = builder.prefix;
this.delimiter = builder.delimiter;
this.maxKeys = builder.maxKeys;
this.commonPrefixes = builder.commonPrefixes;
this.encodingType = builder.encodingType;
}
/**
*
* A flag that indicates whether Amazon S3 returned all of the results that satisfied the search criteria. If your
* results were truncated, you can make a follow-up paginated request using the NextKeyMarker and
* NextVersionIdMarker response parameters as a starting place in another request to return the rest of the results.
*
*
* @return A flag that indicates whether Amazon S3 returned all of the results that satisfied the search criteria.
* If your results were truncated, you can make a follow-up paginated request using the NextKeyMarker and
* NextVersionIdMarker response parameters as a starting place in another request to return the rest of the
* results.
*/
public Boolean isTruncated() {
return isTruncated;
}
/**
*
* Marks the last key returned in a truncated response.
*
*
* @return Marks the last key returned in a truncated response.
*/
public String keyMarker() {
return keyMarker;
}
/**
*
* Marks the last version of the key returned in a truncated response.
*
*
* @return Marks the last version of the key returned in a truncated response.
*/
public String versionIdMarker() {
return versionIdMarker;
}
/**
*
* When the number of responses exceeds the value of MaxKeys
, NextKeyMarker
specifies the
* first key not returned that satisfies the search criteria. Use this value for the key-marker request parameter in
* a subsequent request.
*
*
* @return When the number of responses exceeds the value of MaxKeys
, NextKeyMarker
* specifies the first key not returned that satisfies the search criteria. Use this value for the
* key-marker request parameter in a subsequent request.
*/
public String nextKeyMarker() {
return nextKeyMarker;
}
/**
*
* When the number of responses exceeds the value of MaxKeys
, NextVersionIdMarker
* specifies the first object version not returned that satisfies the search criteria. Use this value for the
* version-id-marker request parameter in a subsequent request.
*
*
* @return When the number of responses exceeds the value of MaxKeys
, NextVersionIdMarker
* specifies the first object version not returned that satisfies the search criteria. Use this value for
* the version-id-marker request parameter in a subsequent request.
*/
public String nextVersionIdMarker() {
return nextVersionIdMarker;
}
/**
* Returns true if the Versions property was specified by the sender (it may be empty), or false if the sender did
* not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS service.
*/
public boolean hasVersions() {
return versions != null && !(versions instanceof SdkAutoConstructList);
}
/**
*
* Container for version information.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasVersions()} to see if a value was sent in this field.
*
*
* @return Container for version information.
*/
public List versions() {
return versions;
}
/**
* Returns true if the DeleteMarkers property was specified by the sender (it may be empty), or false if the sender
* did not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS service.
*/
public boolean hasDeleteMarkers() {
return deleteMarkers != null && !(deleteMarkers instanceof SdkAutoConstructList);
}
/**
*
* Container for an object that is a delete marker.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasDeleteMarkers()} to see if a value was sent in this field.
*
*
* @return Container for an object that is a delete marker.
*/
public List deleteMarkers() {
return deleteMarkers;
}
/**
*
* The bucket name.
*
*
* @return The bucket name.
*/
public String name() {
return name;
}
/**
*
* Selects objects that start with the value supplied by this parameter.
*
*
* @return Selects objects that start with the value supplied by this parameter.
*/
public String prefix() {
return prefix;
}
/**
*
* The delimiter grouping the included keys. A delimiter is a character that you specify to group keys. All keys
* that contain the same string between the prefix and the first occurrence of the delimiter are grouped under a
* single result element in CommonPrefixes
. These groups are counted as one result against the max-keys
* limitation. These keys are not returned elsewhere in the response.
*
*
* @return The delimiter grouping the included keys. A delimiter is a character that you specify to group keys. All
* keys that contain the same string between the prefix and the first occurrence of the delimiter are
* grouped under a single result element in CommonPrefixes
. These groups are counted as one
* result against the max-keys limitation. These keys are not returned elsewhere in the response.
*/
public String delimiter() {
return delimiter;
}
/**
*
* Specifies the maximum number of objects to return.
*
*
* @return Specifies the maximum number of objects to return.
*/
public Integer maxKeys() {
return maxKeys;
}
/**
* Returns true if the CommonPrefixes property was specified by the sender (it may be empty), or false if the sender
* did not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS service.
*/
public boolean hasCommonPrefixes() {
return commonPrefixes != null && !(commonPrefixes instanceof SdkAutoConstructList);
}
/**
*
* All of the keys rolled up into a common prefix count as a single return when calculating the number of returns.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasCommonPrefixes()} to see if a value was sent in this field.
*
*
* @return All of the keys rolled up into a common prefix count as a single return when calculating the number of
* returns.
*/
public List commonPrefixes() {
return commonPrefixes;
}
/**
*
* Encoding type used by Amazon S3 to encode object key names in the XML response.
*
*
* If you specify encoding-type request parameter, Amazon S3 includes this element in the response, and returns
* encoded key name values in the following response elements:
*
*
* KeyMarker, NextKeyMarker, Prefix, Key
, and Delimiter
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #encodingType} will
* return {@link EncodingType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #encodingTypeAsString}.
*
*
* @return Encoding type used by Amazon S3 to encode object key names in the XML response.
*
* If you specify encoding-type request parameter, Amazon S3 includes this element in the response, and
* returns encoded key name values in the following response elements:
*
*
* KeyMarker, NextKeyMarker, Prefix, Key
, and Delimiter
.
* @see EncodingType
*/
public EncodingType encodingType() {
return EncodingType.fromValue(encodingType);
}
/**
*
* Encoding type used by Amazon S3 to encode object key names in the XML response.
*
*
* If you specify encoding-type request parameter, Amazon S3 includes this element in the response, and returns
* encoded key name values in the following response elements:
*
*
* KeyMarker, NextKeyMarker, Prefix, Key
, and Delimiter
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #encodingType} will
* return {@link EncodingType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #encodingTypeAsString}.
*
*
* @return Encoding type used by Amazon S3 to encode object key names in the XML response.
*
* If you specify encoding-type request parameter, Amazon S3 includes this element in the response, and
* returns encoded key name values in the following response elements:
*
*
* KeyMarker, NextKeyMarker, Prefix, Key
, and Delimiter
.
* @see EncodingType
*/
public String encodingTypeAsString() {
return encodingType;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(isTruncated());
hashCode = 31 * hashCode + Objects.hashCode(keyMarker());
hashCode = 31 * hashCode + Objects.hashCode(versionIdMarker());
hashCode = 31 * hashCode + Objects.hashCode(nextKeyMarker());
hashCode = 31 * hashCode + Objects.hashCode(nextVersionIdMarker());
hashCode = 31 * hashCode + Objects.hashCode(hasVersions() ? versions() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasDeleteMarkers() ? deleteMarkers() : null);
hashCode = 31 * hashCode + Objects.hashCode(name());
hashCode = 31 * hashCode + Objects.hashCode(prefix());
hashCode = 31 * hashCode + Objects.hashCode(delimiter());
hashCode = 31 * hashCode + Objects.hashCode(maxKeys());
hashCode = 31 * hashCode + Objects.hashCode(hasCommonPrefixes() ? commonPrefixes() : null);
hashCode = 31 * hashCode + Objects.hashCode(encodingTypeAsString());
return hashCode;
}
@Override
public boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ListObjectVersionsResponse)) {
return false;
}
ListObjectVersionsResponse other = (ListObjectVersionsResponse) obj;
return Objects.equals(isTruncated(), other.isTruncated()) && Objects.equals(keyMarker(), other.keyMarker())
&& Objects.equals(versionIdMarker(), other.versionIdMarker())
&& Objects.equals(nextKeyMarker(), other.nextKeyMarker())
&& Objects.equals(nextVersionIdMarker(), other.nextVersionIdMarker()) && hasVersions() == other.hasVersions()
&& Objects.equals(versions(), other.versions()) && hasDeleteMarkers() == other.hasDeleteMarkers()
&& Objects.equals(deleteMarkers(), other.deleteMarkers()) && Objects.equals(name(), other.name())
&& Objects.equals(prefix(), other.prefix()) && Objects.equals(delimiter(), other.delimiter())
&& Objects.equals(maxKeys(), other.maxKeys()) && hasCommonPrefixes() == other.hasCommonPrefixes()
&& Objects.equals(commonPrefixes(), other.commonPrefixes())
&& Objects.equals(encodingTypeAsString(), other.encodingTypeAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("ListObjectVersionsResponse").add("IsTruncated", isTruncated()).add("KeyMarker", keyMarker())
.add("VersionIdMarker", versionIdMarker()).add("NextKeyMarker", nextKeyMarker())
.add("NextVersionIdMarker", nextVersionIdMarker()).add("Versions", hasVersions() ? versions() : null)
.add("DeleteMarkers", hasDeleteMarkers() ? deleteMarkers() : null).add("Name", name()).add("Prefix", prefix())
.add("Delimiter", delimiter()).add("MaxKeys", maxKeys())
.add("CommonPrefixes", hasCommonPrefixes() ? commonPrefixes() : null).add("EncodingType", encodingTypeAsString())
.build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "IsTruncated":
return Optional.ofNullable(clazz.cast(isTruncated()));
case "KeyMarker":
return Optional.ofNullable(clazz.cast(keyMarker()));
case "VersionIdMarker":
return Optional.ofNullable(clazz.cast(versionIdMarker()));
case "NextKeyMarker":
return Optional.ofNullable(clazz.cast(nextKeyMarker()));
case "NextVersionIdMarker":
return Optional.ofNullable(clazz.cast(nextVersionIdMarker()));
case "Versions":
return Optional.ofNullable(clazz.cast(versions()));
case "DeleteMarkers":
return Optional.ofNullable(clazz.cast(deleteMarkers()));
case "Name":
return Optional.ofNullable(clazz.cast(name()));
case "Prefix":
return Optional.ofNullable(clazz.cast(prefix()));
case "Delimiter":
return Optional.ofNullable(clazz.cast(delimiter()));
case "MaxKeys":
return Optional.ofNullable(clazz.cast(maxKeys()));
case "CommonPrefixes":
return Optional.ofNullable(clazz.cast(commonPrefixes()));
case "EncodingType":
return Optional.ofNullable(clazz.cast(encodingTypeAsString()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function
*
* If you specify encoding-type request parameter, Amazon S3 includes this element in the response, and
* returns encoded key name values in the following response elements:
*
*
* KeyMarker, NextKeyMarker, Prefix, Key
, and Delimiter
.
* @see EncodingType
* @return Returns a reference to this object so that method calls can be chained together.
* @see EncodingType
*/
Builder encodingType(String encodingType);
/**
*
* Encoding type used by Amazon S3 to encode object key names in the XML response.
*
*
* If you specify encoding-type request parameter, Amazon S3 includes this element in the response, and returns
* encoded key name values in the following response elements:
*
*
* KeyMarker, NextKeyMarker, Prefix, Key
, and Delimiter
.
*
*
* @param encodingType
* Encoding type used by Amazon S3 to encode object key names in the XML response.
*
* If you specify encoding-type request parameter, Amazon S3 includes this element in the response, and
* returns encoded key name values in the following response elements:
*
*
* KeyMarker, NextKeyMarker, Prefix, Key
, and Delimiter
.
* @see EncodingType
* @return Returns a reference to this object so that method calls can be chained together.
* @see EncodingType
*/
Builder encodingType(EncodingType encodingType);
}
static final class BuilderImpl extends S3Response.BuilderImpl implements Builder {
private Boolean isTruncated;
private String keyMarker;
private String versionIdMarker;
private String nextKeyMarker;
private String nextVersionIdMarker;
private List versions = DefaultSdkAutoConstructList.getInstance();
private List deleteMarkers = DefaultSdkAutoConstructList.getInstance();
private String name;
private String prefix;
private String delimiter;
private Integer maxKeys;
private List commonPrefixes = DefaultSdkAutoConstructList.getInstance();
private String encodingType;
private BuilderImpl() {
}
private BuilderImpl(ListObjectVersionsResponse model) {
super(model);
isTruncated(model.isTruncated);
keyMarker(model.keyMarker);
versionIdMarker(model.versionIdMarker);
nextKeyMarker(model.nextKeyMarker);
nextVersionIdMarker(model.nextVersionIdMarker);
versions(model.versions);
deleteMarkers(model.deleteMarkers);
name(model.name);
prefix(model.prefix);
delimiter(model.delimiter);
maxKeys(model.maxKeys);
commonPrefixes(model.commonPrefixes);
encodingType(model.encodingType);
}
public final Boolean getIsTruncated() {
return isTruncated;
}
@Override
public final Builder isTruncated(Boolean isTruncated) {
this.isTruncated = isTruncated;
return this;
}
public final void setIsTruncated(Boolean isTruncated) {
this.isTruncated = isTruncated;
}
public final String getKeyMarker() {
return keyMarker;
}
@Override
public final Builder keyMarker(String keyMarker) {
this.keyMarker = keyMarker;
return this;
}
public final void setKeyMarker(String keyMarker) {
this.keyMarker = keyMarker;
}
public final String getVersionIdMarker() {
return versionIdMarker;
}
@Override
public final Builder versionIdMarker(String versionIdMarker) {
this.versionIdMarker = versionIdMarker;
return this;
}
public final void setVersionIdMarker(String versionIdMarker) {
this.versionIdMarker = versionIdMarker;
}
public final String getNextKeyMarker() {
return nextKeyMarker;
}
@Override
public final Builder nextKeyMarker(String nextKeyMarker) {
this.nextKeyMarker = nextKeyMarker;
return this;
}
public final void setNextKeyMarker(String nextKeyMarker) {
this.nextKeyMarker = nextKeyMarker;
}
public final String getNextVersionIdMarker() {
return nextVersionIdMarker;
}
@Override
public final Builder nextVersionIdMarker(String nextVersionIdMarker) {
this.nextVersionIdMarker = nextVersionIdMarker;
return this;
}
public final void setNextVersionIdMarker(String nextVersionIdMarker) {
this.nextVersionIdMarker = nextVersionIdMarker;
}
public final Collection getVersions() {
if (versions instanceof SdkAutoConstructList) {
return null;
}
return versions != null ? versions.stream().map(ObjectVersion::toBuilder).collect(Collectors.toList()) : null;
}
@Override
public final Builder versions(Collection versions) {
this.versions = ObjectVersionListCopier.copy(versions);
return this;
}
@Override
@SafeVarargs
public final Builder versions(ObjectVersion... versions) {
versions(Arrays.asList(versions));
return this;
}
@Override
@SafeVarargs
public final Builder versions(Consumer... versions) {
versions(Stream.of(versions).map(c -> ObjectVersion.builder().applyMutation(c).build()).collect(Collectors.toList()));
return this;
}
public final void setVersions(Collection versions) {
this.versions = ObjectVersionListCopier.copyFromBuilder(versions);
}
public final Collection getDeleteMarkers() {
if (deleteMarkers instanceof SdkAutoConstructList) {
return null;
}
return deleteMarkers != null ? deleteMarkers.stream().map(DeleteMarkerEntry::toBuilder).collect(Collectors.toList())
: null;
}
@Override
public final Builder deleteMarkers(Collection deleteMarkers) {
this.deleteMarkers = DeleteMarkersCopier.copy(deleteMarkers);
return this;
}
@Override
@SafeVarargs
public final Builder deleteMarkers(DeleteMarkerEntry... deleteMarkers) {
deleteMarkers(Arrays.asList(deleteMarkers));
return this;
}
@Override
@SafeVarargs
public final Builder deleteMarkers(Consumer... deleteMarkers) {
deleteMarkers(Stream.of(deleteMarkers).map(c -> DeleteMarkerEntry.builder().applyMutation(c).build())
.collect(Collectors.toList()));
return this;
}
public final void setDeleteMarkers(Collection deleteMarkers) {
this.deleteMarkers = DeleteMarkersCopier.copyFromBuilder(deleteMarkers);
}
public final String getName() {
return name;
}
@Override
public final Builder name(String name) {
this.name = name;
return this;
}
public final void setName(String name) {
this.name = name;
}
public final String getPrefix() {
return prefix;
}
@Override
public final Builder prefix(String prefix) {
this.prefix = prefix;
return this;
}
public final void setPrefix(String prefix) {
this.prefix = prefix;
}
public final String getDelimiter() {
return delimiter;
}
@Override
public final Builder delimiter(String delimiter) {
this.delimiter = delimiter;
return this;
}
public final void setDelimiter(String delimiter) {
this.delimiter = delimiter;
}
public final Integer getMaxKeys() {
return maxKeys;
}
@Override
public final Builder maxKeys(Integer maxKeys) {
this.maxKeys = maxKeys;
return this;
}
public final void setMaxKeys(Integer maxKeys) {
this.maxKeys = maxKeys;
}
public final Collection getCommonPrefixes() {
if (commonPrefixes instanceof SdkAutoConstructList) {
return null;
}
return commonPrefixes != null ? commonPrefixes.stream().map(CommonPrefix::toBuilder).collect(Collectors.toList())
: null;
}
@Override
public final Builder commonPrefixes(Collection commonPrefixes) {
this.commonPrefixes = CommonPrefixListCopier.copy(commonPrefixes);
return this;
}
@Override
@SafeVarargs
public final Builder commonPrefixes(CommonPrefix... commonPrefixes) {
commonPrefixes(Arrays.asList(commonPrefixes));
return this;
}
@Override
@SafeVarargs
public final Builder commonPrefixes(Consumer... commonPrefixes) {
commonPrefixes(Stream.of(commonPrefixes).map(c -> CommonPrefix.builder().applyMutation(c).build())
.collect(Collectors.toList()));
return this;
}
public final void setCommonPrefixes(Collection commonPrefixes) {
this.commonPrefixes = CommonPrefixListCopier.copyFromBuilder(commonPrefixes);
}
public final String getEncodingType() {
return encodingType;
}
@Override
public final Builder encodingType(String encodingType) {
this.encodingType = encodingType;
return this;
}
@Override
public final Builder encodingType(EncodingType encodingType) {
this.encodingType(encodingType == null ? null : encodingType.toString());
return this;
}
public final void setEncodingType(String encodingType) {
this.encodingType = encodingType;
}
@Override
public ListObjectVersionsResponse build() {
return new ListObjectVersionsResponse(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
}
}