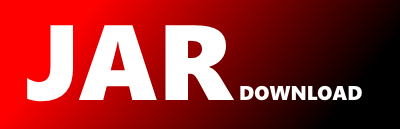
software.amazon.awssdk.services.s3.DefaultS3AsyncClient Maven / Gradle / Ivy
Show all versions of s3 Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.s3;
import static software.amazon.awssdk.utils.FunctionalUtils.runAndLogError;
import java.util.Collections;
import java.util.List;
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.ScheduledExecutorService;
import java.util.function.Consumer;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.awscore.client.handler.AwsAsyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.ApiName;
import software.amazon.awssdk.core.RequestOverrideConfiguration;
import software.amazon.awssdk.core.Response;
import software.amazon.awssdk.core.async.AsyncRequestBody;
import software.amazon.awssdk.core.async.AsyncResponseTransformer;
import software.amazon.awssdk.core.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientOption;
import software.amazon.awssdk.core.client.handler.AsyncClientHandler;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.metrics.CoreMetric;
import software.amazon.awssdk.core.runtime.transform.AsyncStreamingRequestMarshaller;
import software.amazon.awssdk.core.util.VersionInfo;
import software.amazon.awssdk.metrics.MetricCollector;
import software.amazon.awssdk.metrics.MetricPublisher;
import software.amazon.awssdk.metrics.NoOpMetricCollector;
import software.amazon.awssdk.protocols.core.ExceptionMetadata;
import software.amazon.awssdk.protocols.xml.AwsS3ProtocolFactory;
import software.amazon.awssdk.protocols.xml.XmlOperationMetadata;
import software.amazon.awssdk.services.s3.model.AbortMultipartUploadRequest;
import software.amazon.awssdk.services.s3.model.AbortMultipartUploadResponse;
import software.amazon.awssdk.services.s3.model.BucketAlreadyExistsException;
import software.amazon.awssdk.services.s3.model.BucketAlreadyOwnedByYouException;
import software.amazon.awssdk.services.s3.model.CompleteMultipartUploadRequest;
import software.amazon.awssdk.services.s3.model.CompleteMultipartUploadResponse;
import software.amazon.awssdk.services.s3.model.CopyObjectRequest;
import software.amazon.awssdk.services.s3.model.CopyObjectResponse;
import software.amazon.awssdk.services.s3.model.CreateBucketRequest;
import software.amazon.awssdk.services.s3.model.CreateBucketResponse;
import software.amazon.awssdk.services.s3.model.CreateMultipartUploadRequest;
import software.amazon.awssdk.services.s3.model.CreateMultipartUploadResponse;
import software.amazon.awssdk.services.s3.model.DeleteBucketAnalyticsConfigurationRequest;
import software.amazon.awssdk.services.s3.model.DeleteBucketAnalyticsConfigurationResponse;
import software.amazon.awssdk.services.s3.model.DeleteBucketCorsRequest;
import software.amazon.awssdk.services.s3.model.DeleteBucketCorsResponse;
import software.amazon.awssdk.services.s3.model.DeleteBucketEncryptionRequest;
import software.amazon.awssdk.services.s3.model.DeleteBucketEncryptionResponse;
import software.amazon.awssdk.services.s3.model.DeleteBucketInventoryConfigurationRequest;
import software.amazon.awssdk.services.s3.model.DeleteBucketInventoryConfigurationResponse;
import software.amazon.awssdk.services.s3.model.DeleteBucketLifecycleRequest;
import software.amazon.awssdk.services.s3.model.DeleteBucketLifecycleResponse;
import software.amazon.awssdk.services.s3.model.DeleteBucketMetricsConfigurationRequest;
import software.amazon.awssdk.services.s3.model.DeleteBucketMetricsConfigurationResponse;
import software.amazon.awssdk.services.s3.model.DeleteBucketOwnershipControlsRequest;
import software.amazon.awssdk.services.s3.model.DeleteBucketOwnershipControlsResponse;
import software.amazon.awssdk.services.s3.model.DeleteBucketPolicyRequest;
import software.amazon.awssdk.services.s3.model.DeleteBucketPolicyResponse;
import software.amazon.awssdk.services.s3.model.DeleteBucketReplicationRequest;
import software.amazon.awssdk.services.s3.model.DeleteBucketReplicationResponse;
import software.amazon.awssdk.services.s3.model.DeleteBucketRequest;
import software.amazon.awssdk.services.s3.model.DeleteBucketResponse;
import software.amazon.awssdk.services.s3.model.DeleteBucketTaggingRequest;
import software.amazon.awssdk.services.s3.model.DeleteBucketTaggingResponse;
import software.amazon.awssdk.services.s3.model.DeleteBucketWebsiteRequest;
import software.amazon.awssdk.services.s3.model.DeleteBucketWebsiteResponse;
import software.amazon.awssdk.services.s3.model.DeleteObjectRequest;
import software.amazon.awssdk.services.s3.model.DeleteObjectResponse;
import software.amazon.awssdk.services.s3.model.DeleteObjectTaggingRequest;
import software.amazon.awssdk.services.s3.model.DeleteObjectTaggingResponse;
import software.amazon.awssdk.services.s3.model.DeleteObjectsRequest;
import software.amazon.awssdk.services.s3.model.DeleteObjectsResponse;
import software.amazon.awssdk.services.s3.model.DeletePublicAccessBlockRequest;
import software.amazon.awssdk.services.s3.model.DeletePublicAccessBlockResponse;
import software.amazon.awssdk.services.s3.model.GetBucketAccelerateConfigurationRequest;
import software.amazon.awssdk.services.s3.model.GetBucketAccelerateConfigurationResponse;
import software.amazon.awssdk.services.s3.model.GetBucketAclRequest;
import software.amazon.awssdk.services.s3.model.GetBucketAclResponse;
import software.amazon.awssdk.services.s3.model.GetBucketAnalyticsConfigurationRequest;
import software.amazon.awssdk.services.s3.model.GetBucketAnalyticsConfigurationResponse;
import software.amazon.awssdk.services.s3.model.GetBucketCorsRequest;
import software.amazon.awssdk.services.s3.model.GetBucketCorsResponse;
import software.amazon.awssdk.services.s3.model.GetBucketEncryptionRequest;
import software.amazon.awssdk.services.s3.model.GetBucketEncryptionResponse;
import software.amazon.awssdk.services.s3.model.GetBucketInventoryConfigurationRequest;
import software.amazon.awssdk.services.s3.model.GetBucketInventoryConfigurationResponse;
import software.amazon.awssdk.services.s3.model.GetBucketLifecycleConfigurationRequest;
import software.amazon.awssdk.services.s3.model.GetBucketLifecycleConfigurationResponse;
import software.amazon.awssdk.services.s3.model.GetBucketLocationRequest;
import software.amazon.awssdk.services.s3.model.GetBucketLocationResponse;
import software.amazon.awssdk.services.s3.model.GetBucketLoggingRequest;
import software.amazon.awssdk.services.s3.model.GetBucketLoggingResponse;
import software.amazon.awssdk.services.s3.model.GetBucketMetricsConfigurationRequest;
import software.amazon.awssdk.services.s3.model.GetBucketMetricsConfigurationResponse;
import software.amazon.awssdk.services.s3.model.GetBucketNotificationConfigurationRequest;
import software.amazon.awssdk.services.s3.model.GetBucketNotificationConfigurationResponse;
import software.amazon.awssdk.services.s3.model.GetBucketOwnershipControlsRequest;
import software.amazon.awssdk.services.s3.model.GetBucketOwnershipControlsResponse;
import software.amazon.awssdk.services.s3.model.GetBucketPolicyRequest;
import software.amazon.awssdk.services.s3.model.GetBucketPolicyResponse;
import software.amazon.awssdk.services.s3.model.GetBucketPolicyStatusRequest;
import software.amazon.awssdk.services.s3.model.GetBucketPolicyStatusResponse;
import software.amazon.awssdk.services.s3.model.GetBucketReplicationRequest;
import software.amazon.awssdk.services.s3.model.GetBucketReplicationResponse;
import software.amazon.awssdk.services.s3.model.GetBucketRequestPaymentRequest;
import software.amazon.awssdk.services.s3.model.GetBucketRequestPaymentResponse;
import software.amazon.awssdk.services.s3.model.GetBucketTaggingRequest;
import software.amazon.awssdk.services.s3.model.GetBucketTaggingResponse;
import software.amazon.awssdk.services.s3.model.GetBucketVersioningRequest;
import software.amazon.awssdk.services.s3.model.GetBucketVersioningResponse;
import software.amazon.awssdk.services.s3.model.GetBucketWebsiteRequest;
import software.amazon.awssdk.services.s3.model.GetBucketWebsiteResponse;
import software.amazon.awssdk.services.s3.model.GetObjectAclRequest;
import software.amazon.awssdk.services.s3.model.GetObjectAclResponse;
import software.amazon.awssdk.services.s3.model.GetObjectLegalHoldRequest;
import software.amazon.awssdk.services.s3.model.GetObjectLegalHoldResponse;
import software.amazon.awssdk.services.s3.model.GetObjectLockConfigurationRequest;
import software.amazon.awssdk.services.s3.model.GetObjectLockConfigurationResponse;
import software.amazon.awssdk.services.s3.model.GetObjectRequest;
import software.amazon.awssdk.services.s3.model.GetObjectResponse;
import software.amazon.awssdk.services.s3.model.GetObjectRetentionRequest;
import software.amazon.awssdk.services.s3.model.GetObjectRetentionResponse;
import software.amazon.awssdk.services.s3.model.GetObjectTaggingRequest;
import software.amazon.awssdk.services.s3.model.GetObjectTaggingResponse;
import software.amazon.awssdk.services.s3.model.GetObjectTorrentRequest;
import software.amazon.awssdk.services.s3.model.GetObjectTorrentResponse;
import software.amazon.awssdk.services.s3.model.GetPublicAccessBlockRequest;
import software.amazon.awssdk.services.s3.model.GetPublicAccessBlockResponse;
import software.amazon.awssdk.services.s3.model.HeadBucketRequest;
import software.amazon.awssdk.services.s3.model.HeadBucketResponse;
import software.amazon.awssdk.services.s3.model.HeadObjectRequest;
import software.amazon.awssdk.services.s3.model.HeadObjectResponse;
import software.amazon.awssdk.services.s3.model.ListBucketAnalyticsConfigurationsRequest;
import software.amazon.awssdk.services.s3.model.ListBucketAnalyticsConfigurationsResponse;
import software.amazon.awssdk.services.s3.model.ListBucketInventoryConfigurationsRequest;
import software.amazon.awssdk.services.s3.model.ListBucketInventoryConfigurationsResponse;
import software.amazon.awssdk.services.s3.model.ListBucketMetricsConfigurationsRequest;
import software.amazon.awssdk.services.s3.model.ListBucketMetricsConfigurationsResponse;
import software.amazon.awssdk.services.s3.model.ListBucketsRequest;
import software.amazon.awssdk.services.s3.model.ListBucketsResponse;
import software.amazon.awssdk.services.s3.model.ListMultipartUploadsRequest;
import software.amazon.awssdk.services.s3.model.ListMultipartUploadsResponse;
import software.amazon.awssdk.services.s3.model.ListObjectVersionsRequest;
import software.amazon.awssdk.services.s3.model.ListObjectVersionsResponse;
import software.amazon.awssdk.services.s3.model.ListObjectsRequest;
import software.amazon.awssdk.services.s3.model.ListObjectsResponse;
import software.amazon.awssdk.services.s3.model.ListObjectsV2Request;
import software.amazon.awssdk.services.s3.model.ListObjectsV2Response;
import software.amazon.awssdk.services.s3.model.ListPartsRequest;
import software.amazon.awssdk.services.s3.model.ListPartsResponse;
import software.amazon.awssdk.services.s3.model.NoSuchBucketException;
import software.amazon.awssdk.services.s3.model.NoSuchKeyException;
import software.amazon.awssdk.services.s3.model.NoSuchUploadException;
import software.amazon.awssdk.services.s3.model.ObjectAlreadyInActiveTierErrorException;
import software.amazon.awssdk.services.s3.model.ObjectNotInActiveTierErrorException;
import software.amazon.awssdk.services.s3.model.PutBucketAccelerateConfigurationRequest;
import software.amazon.awssdk.services.s3.model.PutBucketAccelerateConfigurationResponse;
import software.amazon.awssdk.services.s3.model.PutBucketAclRequest;
import software.amazon.awssdk.services.s3.model.PutBucketAclResponse;
import software.amazon.awssdk.services.s3.model.PutBucketAnalyticsConfigurationRequest;
import software.amazon.awssdk.services.s3.model.PutBucketAnalyticsConfigurationResponse;
import software.amazon.awssdk.services.s3.model.PutBucketCorsRequest;
import software.amazon.awssdk.services.s3.model.PutBucketCorsResponse;
import software.amazon.awssdk.services.s3.model.PutBucketEncryptionRequest;
import software.amazon.awssdk.services.s3.model.PutBucketEncryptionResponse;
import software.amazon.awssdk.services.s3.model.PutBucketInventoryConfigurationRequest;
import software.amazon.awssdk.services.s3.model.PutBucketInventoryConfigurationResponse;
import software.amazon.awssdk.services.s3.model.PutBucketLifecycleConfigurationRequest;
import software.amazon.awssdk.services.s3.model.PutBucketLifecycleConfigurationResponse;
import software.amazon.awssdk.services.s3.model.PutBucketLoggingRequest;
import software.amazon.awssdk.services.s3.model.PutBucketLoggingResponse;
import software.amazon.awssdk.services.s3.model.PutBucketMetricsConfigurationRequest;
import software.amazon.awssdk.services.s3.model.PutBucketMetricsConfigurationResponse;
import software.amazon.awssdk.services.s3.model.PutBucketNotificationConfigurationRequest;
import software.amazon.awssdk.services.s3.model.PutBucketNotificationConfigurationResponse;
import software.amazon.awssdk.services.s3.model.PutBucketOwnershipControlsRequest;
import software.amazon.awssdk.services.s3.model.PutBucketOwnershipControlsResponse;
import software.amazon.awssdk.services.s3.model.PutBucketPolicyRequest;
import software.amazon.awssdk.services.s3.model.PutBucketPolicyResponse;
import software.amazon.awssdk.services.s3.model.PutBucketReplicationRequest;
import software.amazon.awssdk.services.s3.model.PutBucketReplicationResponse;
import software.amazon.awssdk.services.s3.model.PutBucketRequestPaymentRequest;
import software.amazon.awssdk.services.s3.model.PutBucketRequestPaymentResponse;
import software.amazon.awssdk.services.s3.model.PutBucketTaggingRequest;
import software.amazon.awssdk.services.s3.model.PutBucketTaggingResponse;
import software.amazon.awssdk.services.s3.model.PutBucketVersioningRequest;
import software.amazon.awssdk.services.s3.model.PutBucketVersioningResponse;
import software.amazon.awssdk.services.s3.model.PutBucketWebsiteRequest;
import software.amazon.awssdk.services.s3.model.PutBucketWebsiteResponse;
import software.amazon.awssdk.services.s3.model.PutObjectAclRequest;
import software.amazon.awssdk.services.s3.model.PutObjectAclResponse;
import software.amazon.awssdk.services.s3.model.PutObjectLegalHoldRequest;
import software.amazon.awssdk.services.s3.model.PutObjectLegalHoldResponse;
import software.amazon.awssdk.services.s3.model.PutObjectLockConfigurationRequest;
import software.amazon.awssdk.services.s3.model.PutObjectLockConfigurationResponse;
import software.amazon.awssdk.services.s3.model.PutObjectRequest;
import software.amazon.awssdk.services.s3.model.PutObjectResponse;
import software.amazon.awssdk.services.s3.model.PutObjectRetentionRequest;
import software.amazon.awssdk.services.s3.model.PutObjectRetentionResponse;
import software.amazon.awssdk.services.s3.model.PutObjectTaggingRequest;
import software.amazon.awssdk.services.s3.model.PutObjectTaggingResponse;
import software.amazon.awssdk.services.s3.model.PutPublicAccessBlockRequest;
import software.amazon.awssdk.services.s3.model.PutPublicAccessBlockResponse;
import software.amazon.awssdk.services.s3.model.RestoreObjectRequest;
import software.amazon.awssdk.services.s3.model.RestoreObjectResponse;
import software.amazon.awssdk.services.s3.model.S3Exception;
import software.amazon.awssdk.services.s3.model.S3Request;
import software.amazon.awssdk.services.s3.model.UploadPartCopyRequest;
import software.amazon.awssdk.services.s3.model.UploadPartCopyResponse;
import software.amazon.awssdk.services.s3.model.UploadPartRequest;
import software.amazon.awssdk.services.s3.model.UploadPartResponse;
import software.amazon.awssdk.services.s3.paginators.ListMultipartUploadsPublisher;
import software.amazon.awssdk.services.s3.paginators.ListObjectVersionsPublisher;
import software.amazon.awssdk.services.s3.paginators.ListObjectsV2Publisher;
import software.amazon.awssdk.services.s3.paginators.ListPartsPublisher;
import software.amazon.awssdk.services.s3.transform.AbortMultipartUploadRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.CompleteMultipartUploadRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.CopyObjectRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.CreateBucketRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.CreateMultipartUploadRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.DeleteBucketAnalyticsConfigurationRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.DeleteBucketCorsRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.DeleteBucketEncryptionRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.DeleteBucketInventoryConfigurationRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.DeleteBucketLifecycleRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.DeleteBucketMetricsConfigurationRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.DeleteBucketOwnershipControlsRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.DeleteBucketPolicyRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.DeleteBucketReplicationRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.DeleteBucketRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.DeleteBucketTaggingRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.DeleteBucketWebsiteRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.DeleteObjectRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.DeleteObjectTaggingRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.DeleteObjectsRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.DeletePublicAccessBlockRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.GetBucketAccelerateConfigurationRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.GetBucketAclRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.GetBucketAnalyticsConfigurationRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.GetBucketCorsRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.GetBucketEncryptionRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.GetBucketInventoryConfigurationRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.GetBucketLifecycleConfigurationRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.GetBucketLocationRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.GetBucketLoggingRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.GetBucketMetricsConfigurationRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.GetBucketNotificationConfigurationRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.GetBucketOwnershipControlsRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.GetBucketPolicyRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.GetBucketPolicyStatusRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.GetBucketReplicationRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.GetBucketRequestPaymentRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.GetBucketTaggingRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.GetBucketVersioningRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.GetBucketWebsiteRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.GetObjectAclRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.GetObjectLegalHoldRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.GetObjectLockConfigurationRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.GetObjectRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.GetObjectRetentionRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.GetObjectTaggingRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.GetObjectTorrentRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.GetPublicAccessBlockRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.HeadBucketRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.HeadObjectRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.ListBucketAnalyticsConfigurationsRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.ListBucketInventoryConfigurationsRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.ListBucketMetricsConfigurationsRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.ListBucketsRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.ListMultipartUploadsRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.ListObjectVersionsRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.ListObjectsRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.ListObjectsV2RequestMarshaller;
import software.amazon.awssdk.services.s3.transform.ListPartsRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.PutBucketAccelerateConfigurationRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.PutBucketAclRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.PutBucketAnalyticsConfigurationRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.PutBucketCorsRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.PutBucketEncryptionRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.PutBucketInventoryConfigurationRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.PutBucketLifecycleConfigurationRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.PutBucketLoggingRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.PutBucketMetricsConfigurationRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.PutBucketNotificationConfigurationRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.PutBucketOwnershipControlsRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.PutBucketPolicyRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.PutBucketReplicationRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.PutBucketRequestPaymentRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.PutBucketTaggingRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.PutBucketVersioningRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.PutBucketWebsiteRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.PutObjectAclRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.PutObjectLegalHoldRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.PutObjectLockConfigurationRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.PutObjectRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.PutObjectRetentionRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.PutObjectTaggingRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.PutPublicAccessBlockRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.RestoreObjectRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.UploadPartCopyRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.UploadPartRequestMarshaller;
import software.amazon.awssdk.services.s3.waiters.S3AsyncWaiter;
import software.amazon.awssdk.utils.CompletableFutureUtils;
/**
* Internal implementation of {@link S3AsyncClient}.
*
* @see S3AsyncClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultS3AsyncClient implements S3AsyncClient {
private static final Logger log = LoggerFactory.getLogger(DefaultS3AsyncClient.class);
private final AsyncClientHandler clientHandler;
private final AwsS3ProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
private final ScheduledExecutorService executorService;
protected DefaultS3AsyncClient(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsAsyncClientHandler(clientConfiguration);
this.clientConfiguration = clientConfiguration;
this.protocolFactory = init();
this.executorService = clientConfiguration.option(SdkClientOption.SCHEDULED_EXECUTOR_SERVICE);
}
@Override
public final String serviceName() {
return SERVICE_NAME;
}
/**
*
* This operation aborts a multipart upload. After a multipart upload is aborted, no additional parts can be
* uploaded using that upload ID. The storage consumed by any previously uploaded parts will be freed. However, if
* any part uploads are currently in progress, those part uploads might or might not succeed. As a result, it might
* be necessary to abort a given multipart upload multiple times in order to completely free all storage consumed by
* all parts.
*
*
* To verify that all parts have been removed, so you don't get charged for the part storage, you should call the ListParts operation and ensure that
* the parts list is empty.
*
*
* For information about permissions required to use the multipart upload API, see Multipart Upload API and
* Permissions.
*
*
* The following operations are related to AbortMultipartUpload
:
*
*
* -
*
*
* -
*
* UploadPart
*
*
* -
*
*
* -
*
* ListParts
*
*
* -
*
*
*
*
* @param abortMultipartUploadRequest
* @return A Java Future containing the result of the AbortMultipartUpload operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NoSuchUploadException The specified multipart upload does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.AbortMultipartUpload
*/
@Override
public CompletableFuture abortMultipartUpload(
AbortMultipartUploadRequest abortMultipartUploadRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, abortMultipartUploadRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AbortMultipartUpload");
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(AbortMultipartUploadResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("AbortMultipartUpload")
.withMarshaller(new AbortMultipartUploadRequestMarshaller(protocolFactory))
.withCombinedResponseHandler(responseHandler).withInput(abortMultipartUploadRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = abortMultipartUploadRequest.overrideConfiguration().orElse(
null);
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Completes a multipart upload by assembling previously uploaded parts.
*
*
* You first initiate the multipart upload and then upload all parts using the UploadPart operation. After
* successfully uploading all relevant parts of an upload, you call this operation to complete the upload. Upon
* receiving this request, Amazon S3 concatenates all the parts in ascending order by part number to create a new
* object. In the Complete Multipart Upload request, you must provide the parts list. You must ensure that the parts
* list is complete. This operation concatenates the parts that you provide in the list. For each part in the list,
* you must provide the part number and the ETag
value, returned after that part was uploaded.
*
*
* Processing of a Complete Multipart Upload request could take several minutes to complete. After Amazon S3 begins
* processing the request, it sends an HTTP response header that specifies a 200 OK response. While processing is in
* progress, Amazon S3 periodically sends white space characters to keep the connection from timing out. Because a
* request could fail after the initial 200 OK response has been sent, it is important that you check the response
* body to determine whether the request succeeded.
*
*
* Note that if CompleteMultipartUpload
fails, applications should be prepared to retry the failed
* requests. For more information, see Amazon S3 Error Best
* Practices.
*
*
* For more information about multipart uploads, see Uploading Objects Using Multipart
* Upload.
*
*
* For information about permissions required to use the multipart upload API, see Multipart Upload API and
* Permissions.
*
*
* CompleteMultipartUpload
has the following special errors:
*
*
* -
*
* Error code: EntityTooSmall
*
*
* -
*
* Description: Your proposed upload is smaller than the minimum allowed object size. Each part must be at least 5
* MB in size, except the last part.
*
*
* -
*
* 400 Bad Request
*
*
*
*
* -
*
* Error code: InvalidPart
*
*
* -
*
* Description: One or more of the specified parts could not be found. The part might not have been uploaded, or the
* specified entity tag might not have matched the part's entity tag.
*
*
* -
*
* 400 Bad Request
*
*
*
*
* -
*
* Error code: InvalidPartOrder
*
*
* -
*
* Description: The list of parts was not in ascending order. The parts list must be specified in order by part
* number.
*
*
* -
*
* 400 Bad Request
*
*
*
*
* -
*
* Error code: NoSuchUpload
*
*
* -
*
* Description: The specified multipart upload does not exist. The upload ID might be invalid, or the multipart
* upload might have been aborted or completed.
*
*
* -
*
* 404 Not Found
*
*
*
*
*
*
* The following operations are related to CompleteMultipartUpload
:
*
*
* -
*
*
* -
*
* UploadPart
*
*
* -
*
*
* -
*
* ListParts
*
*
* -
*
*
*
*
* @param completeMultipartUploadRequest
* @return A Java Future containing the result of the CompleteMultipartUpload operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.CompleteMultipartUpload
*/
@Override
public CompletableFuture completeMultipartUpload(
CompleteMultipartUploadRequest completeMultipartUploadRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, completeMultipartUploadRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CompleteMultipartUpload");
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(CompleteMultipartUploadResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CompleteMultipartUpload")
.withMarshaller(new CompleteMultipartUploadRequestMarshaller(protocolFactory))
.withCombinedResponseHandler(responseHandler).withInput(completeMultipartUploadRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = completeMultipartUploadRequest.overrideConfiguration()
.orElse(null);
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a copy of an object that is already stored in Amazon S3.
*
*
*
* You can store individual objects of up to 5 TB in Amazon S3. You create a copy of your object up to 5 GB in size
* in a single atomic operation using this API. However, to copy an object greater than 5 GB, you must use the
* multipart upload Upload Part - Copy API. For more information, see Copy Object Using the
* REST Multipart Upload API.
*
*
*
* All copy requests must be authenticated. Additionally, you must have read access to the source object and
* write access to the destination bucket. For more information, see REST Authentication. Both the
* Region that you want to copy the object from and the Region that you want to copy the object to must be enabled
* for your account.
*
*
* A copy request might return an error when Amazon S3 receives the copy request or while Amazon S3 is copying the
* files. If the error occurs before the copy operation starts, you receive a standard Amazon S3 error. If the error
* occurs during the copy operation, the error response is embedded in the 200 OK
response. This means
* that a 200 OK
response can contain either a success or an error. Design your application to parse
* the contents of the response and handle it appropriately.
*
*
* If the copy is successful, you receive a response with information about the copied object.
*
*
*
* If the request is an HTTP 1.1 request, the response is chunk encoded. If it were not, it would not contain the
* content-length, and you would need to read the entire body.
*
*
*
* The copy request charge is based on the storage class and Region that you specify for the destination object. For
* pricing information, see Amazon S3 pricing.
*
*
*
* Amazon S3 transfer acceleration does not support cross-Region copies. If you request a cross-Region copy using a
* transfer acceleration endpoint, you get a 400 Bad Request
error. For more information, see Transfer Acceleration.
*
*
*
* Metadata
*
*
* When copying an object, you can preserve all metadata (default) or specify new metadata. However, the ACL is not
* preserved and is set to private for the user making the request. To override the default ACL setting, specify a
* new ACL when generating a copy request. For more information, see Using ACLs.
*
*
* To specify whether you want the object metadata copied from the source object or replaced with metadata provided
* in the request, you can optionally add the x-amz-metadata-directive
header. When you grant
* permissions, you can use the s3:x-amz-metadata-directive
condition key to enforce certain metadata
* behavior when objects are uploaded. For more information, see Specifying Conditions in a
* Policy in the Amazon S3 Developer Guide. For a complete list of Amazon S3-specific condition keys, see
* Actions, Resources, and Condition
* Keys for Amazon S3.
*
*
* x-amz-copy-source-if
Headers
*
*
* To only copy an object under certain conditions, such as whether the Etag
matches or whether the
* object was modified before or after a specified date, use the following request parameters:
*
*
* -
*
* x-amz-copy-source-if-match
*
*
* -
*
* x-amz-copy-source-if-none-match
*
*
* -
*
* x-amz-copy-source-if-unmodified-since
*
*
* -
*
* x-amz-copy-source-if-modified-since
*
*
*
*
* If both the x-amz-copy-source-if-match
and x-amz-copy-source-if-unmodified-since
* headers are present in the request and evaluate as follows, Amazon S3 returns 200 OK
and copies the
* data:
*
*
* -
*
* x-amz-copy-source-if-match
condition evaluates to true
*
*
* -
*
* x-amz-copy-source-if-unmodified-since
condition evaluates to false
*
*
*
*
* If both the x-amz-copy-source-if-none-match
and x-amz-copy-source-if-modified-since
* headers are present in the request and evaluate as follows, Amazon S3 returns the
* 412 Precondition Failed
response code:
*
*
* -
*
* x-amz-copy-source-if-none-match
condition evaluates to false
*
*
* -
*
* x-amz-copy-source-if-modified-since
condition evaluates to true
*
*
*
*
*
* All headers with the x-amz-
prefix, including x-amz-copy-source
, must be signed.
*
*
*
* Encryption
*
*
* The source object that you are copying can be encrypted or unencrypted. The source object can be encrypted with
* server-side encryption using AWS managed encryption keys (SSE-S3 or SSE-KMS) or by using a customer-provided
* encryption key. With server-side encryption, Amazon S3 encrypts your data as it writes it to disks in its data
* centers and decrypts the data when you access it.
*
*
* You can optionally use the appropriate encryption-related headers to request server-side encryption for the
* target object. You have the option to provide your own encryption key or use SSE-S3 or SSE-KMS, regardless of the
* form of server-side encryption that was used to encrypt the source object. You can even request encryption if the
* source object was not encrypted. For more information about server-side encryption, see Using Server-Side
* Encryption.
*
*
* Access Control List (ACL)-Specific Request Headers
*
*
* When copying an object, you can optionally use headers to grant ACL-based permissions. By default, all objects
* are private. Only the owner has full access control. When adding a new object, you can grant permissions to
* individual AWS accounts or to predefined groups defined by Amazon S3. These permissions are then added to the ACL
* on the object. For more information, see Access Control List (ACL) Overview
* and Managing ACLs Using the
* REST API.
*
*
* Storage Class Options
*
*
* You can use the CopyObject
operation to change the storage class of an object that is already stored
* in Amazon S3 using the StorageClass
parameter. For more information, see Storage Classes in the
* Amazon S3 Service Developer Guide.
*
*
* Versioning
*
*
* By default, x-amz-copy-source
identifies the current version of an object to copy. If the current
* version is a delete marker, Amazon S3 behaves as if the object was deleted. To copy a different version, use the
* versionId
subresource.
*
*
* If you enable versioning on the target bucket, Amazon S3 generates a unique version ID for the object being
* copied. This version ID is different from the version ID of the source object. Amazon S3 returns the version ID
* of the copied object in the x-amz-version-id
response header in the response.
*
*
* If you do not enable versioning or suspend it on the target bucket, the version ID that Amazon S3 generates is
* always null.
*
*
* If the source object's storage class is GLACIER, you must restore a copy of this object before you can use it as
* a source object for the copy operation. For more information, see RestoreObject.
*
*
* The following operations are related to CopyObject
:
*
*
*
* For more information, see Copying Objects.
*
*
* @param copyObjectRequest
* @return A Java Future containing the result of the CopyObject operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ObjectNotInActiveTierErrorException The source object of the COPY operation is not in the active tier
* and is only stored in Amazon S3 Glacier.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.CopyObject
*/
@Override
public CompletableFuture copyObject(CopyObjectRequest copyObjectRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, copyObjectRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CopyObject");
HttpResponseHandler> responseHandler = protocolFactory.createCombinedResponseHandler(
CopyObjectResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("CopyObject")
.withMarshaller(new CopyObjectRequestMarshaller(protocolFactory))
.withCombinedResponseHandler(responseHandler).withInput(copyObjectRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = copyObjectRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a new S3 bucket. To create a bucket, you must register with Amazon S3 and have a valid AWS Access Key ID
* to authenticate requests. Anonymous requests are never allowed to create buckets. By creating the bucket, you
* become the bucket owner.
*
*
* Not every string is an acceptable bucket name. For information about bucket naming restrictions, see Working with Amazon S3 buckets.
*
*
* If you want to create an Amazon S3 on Outposts bucket, see Create Bucket.
*
*
* By default, the bucket is created in the US East (N. Virginia) Region. You can optionally specify a Region in the
* request body. You might choose a Region to optimize latency, minimize costs, or address regulatory requirements.
* For example, if you reside in Europe, you will probably find it advantageous to create buckets in the Europe
* (Ireland) Region. For more information, see Accessing a
* bucket.
*
*
*
* If you send your create bucket request to the s3.amazonaws.com
endpoint, the request goes to the
* us-east-1 Region. Accordingly, the signature calculations in Signature Version 4 must use us-east-1 as the
* Region, even if the location constraint in the request specifies another Region where the bucket is to be
* created. If you create a bucket in a Region other than US East (N. Virginia), your application must be able to
* handle 307 redirect. For more information, see Virtual hosting of buckets.
*
*
*
* When creating a bucket using this operation, you can optionally specify the accounts or groups that should be
* granted specific permissions on the bucket. There are two ways to grant the appropriate permissions using the
* request headers.
*
*
* -
*
* Specify a canned ACL using the x-amz-acl
request header. Amazon S3 supports a set of predefined
* ACLs, known as canned ACLs. Each canned ACL has a predefined set of grantees and permissions. For more
* information, see Canned
* ACL.
*
*
* -
*
* Specify access permissions explicitly using the x-amz-grant-read
, x-amz-grant-write
,
* x-amz-grant-read-acp
, x-amz-grant-write-acp
, and x-amz-grant-full-control
* headers. These headers map to the set of permissions Amazon S3 supports in an ACL. For more information, see Access control list (ACL) overview.
*
*
* You specify each grantee as a type=value pair, where the type is one of the following:
*
*
* -
*
* id
– if the value specified is the canonical user ID of an AWS account
*
*
* -
*
* uri
– if you are granting permissions to a predefined group
*
*
* -
*
* emailAddress
– if the value specified is the email address of an AWS account
*
*
*
* Using email addresses to specify a grantee is only supported in the following AWS Regions:
*
*
* -
*
* US East (N. Virginia)
*
*
* -
*
* US West (N. California)
*
*
* -
*
* US West (Oregon)
*
*
* -
*
* Asia Pacific (Singapore)
*
*
* -
*
* Asia Pacific (Sydney)
*
*
* -
*
* Asia Pacific (Tokyo)
*
*
* -
*
* Europe (Ireland)
*
*
* -
*
* South America (São Paulo)
*
*
*
*
* For a list of all the Amazon S3 supported Regions and endpoints, see Regions and Endpoints in the AWS
* General Reference.
*
*
*
*
* For example, the following x-amz-grant-read
header grants the AWS accounts identified by account IDs
* permissions to read object data and its metadata:
*
*
* x-amz-grant-read: id="11112222333", id="444455556666"
*
*
*
*
*
* You can use either a canned ACL or specify access permissions explicitly. You cannot do both.
*
*
*
* The following operations are related to CreateBucket
:
*
*
* -
*
* PutObject
*
*
* -
*
* DeleteBucket
*
*
*
*
* @param createBucketRequest
* @return A Java Future containing the result of the CreateBucket operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BucketAlreadyExistsException The requested bucket name is not available. The bucket namespace is
* shared by all users of the system. Select a different name and try again.
* - BucketAlreadyOwnedByYouException The bucket you tried to create already exists, and you own it.
* Amazon S3 returns this error in all AWS Regions except in the North Virginia Region. For legacy
* compatibility, if you re-create an existing bucket that you already own in the North Virginia Region,
* Amazon S3 returns 200 OK and resets the bucket access control lists (ACLs).
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.CreateBucket
*/
@Override
public CompletableFuture createBucket(CreateBucketRequest createBucketRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, createBucketRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateBucket");
HttpResponseHandler> responseHandler = protocolFactory.createCombinedResponseHandler(
CreateBucketResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateBucket").withMarshaller(new CreateBucketRequestMarshaller(protocolFactory))
.withCombinedResponseHandler(responseHandler).withInput(createBucketRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = createBucketRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* This operation initiates a multipart upload and returns an upload ID. This upload ID is used to associate all of
* the parts in the specific multipart upload. You specify this upload ID in each of your subsequent upload part
* requests (see UploadPart). You
* also include this upload ID in the final request to either complete or abort the multipart upload request.
*
*
* For more information about multipart uploads, see Multipart Upload Overview.
*
*
* If you have configured a lifecycle rule to abort incomplete multipart uploads, the upload must complete within
* the number of days specified in the bucket lifecycle configuration. Otherwise, the incomplete multipart upload
* becomes eligible for an abort operation and Amazon S3 aborts the multipart upload. For more information, see
* Aborting Incomplete Multipart Uploads Using a Bucket Lifecycle Policy.
*
*
* For information about the permissions required to use the multipart upload API, see Multipart Upload API and
* Permissions.
*
*
* For request signing, multipart upload is just a series of regular requests. You initiate a multipart upload, send
* one or more requests to upload parts, and then complete the multipart upload process. You sign each request
* individually. There is nothing special about signing multipart upload requests. For more information about
* signing, see Authenticating
* Requests (AWS Signature Version 4).
*
*
*
* After you initiate a multipart upload and upload one or more parts, to stop being charged for storing the
* uploaded parts, you must either complete or abort the multipart upload. Amazon S3 frees up the space used to
* store the parts and stop charging you for storing them only after you either complete or abort a multipart
* upload.
*
*
*
* You can optionally request server-side encryption. For server-side encryption, Amazon S3 encrypts your data as it
* writes it to disks in its data centers and decrypts it when you access it. You can provide your own encryption
* key, or use AWS Key Management Service (AWS KMS) customer master keys (CMKs) or Amazon S3-managed encryption
* keys. If you choose to provide your own encryption key, the request headers you provide in UploadPart and UploadPartCopy requests must
* match the headers you used in the request to initiate the upload by using CreateMultipartUpload
.
*
*
* To perform a multipart upload with encryption using an AWS KMS CMK, the requester must have permission to the
* kms:Encrypt
, kms:Decrypt
, kms:ReEncrypt*
,
* kms:GenerateDataKey*
, and kms:DescribeKey
actions on the key. These permissions are
* required because Amazon S3 must decrypt and read data from the encrypted file parts before it completes the
* multipart upload.
*
*
* If your AWS Identity and Access Management (IAM) user or role is in the same AWS account as the AWS KMS CMK, then
* you must have these permissions on the key policy. If your IAM user or role belongs to a different account than
* the key, then you must have the permissions on both the key policy and your IAM user or role.
*
*
* For more information, see Protecting Data Using
* Server-Side Encryption.
*
*
* - Access Permissions
* -
*
* When copying an object, you can optionally specify the accounts or groups that should be granted specific
* permissions on the new object. There are two ways to grant the permissions using the request headers:
*
*
* -
*
* Specify a canned ACL with the x-amz-acl
request header. For more information, see Canned ACL.
*
*
* -
*
* Specify access permissions explicitly with the x-amz-grant-read
, x-amz-grant-read-acp
,
* x-amz-grant-write-acp
, and x-amz-grant-full-control
headers. These parameters map to
* the set of permissions that Amazon S3 supports in an ACL. For more information, see Access Control List (ACL) Overview.
*
*
*
*
* You can use either a canned ACL or specify access permissions explicitly. You cannot do both.
*
*
* - Server-Side- Encryption-Specific Request Headers
* -
*
* You can optionally tell Amazon S3 to encrypt data at rest using server-side encryption. Server-side encryption is
* for data encryption at rest. Amazon S3 encrypts your data as it writes it to disks in its data centers and
* decrypts it when you access it. The option you use depends on whether you want to use AWS managed encryption keys
* or provide your own encryption key.
*
*
* -
*
* Use encryption keys managed by Amazon S3 or customer master keys (CMKs) stored in AWS Key Management Service (AWS
* KMS) – If you want AWS to manage the keys used to encrypt data, specify the following headers in the request.
*
*
* -
*
* x-amz-server-side-encryption
*
*
* -
*
* x-amz-server-side-encryption-aws-kms-key-id
*
*
* -
*
* x-amz-server-side-encryption-context
*
*
*
*
*
* If you specify x-amz-server-side-encryption:aws:kms
, but don't provide
* x-amz-server-side-encryption-aws-kms-key-id
, Amazon S3 uses the AWS managed CMK in AWS KMS to
* protect the data.
*
*
*
* All GET and PUT requests for an object protected by AWS KMS fail if you don't make them with SSL or by using
* SigV4.
*
*
*
* For more information about server-side encryption with CMKs stored in AWS KMS (SSE-KMS), see Protecting Data Using Server-Side
* Encryption with CMKs stored in AWS KMS.
*
*
* -
*
* Use customer-provided encryption keys – If you want to manage your own encryption keys, provide all the following
* headers in the request.
*
*
* -
*
* x-amz-server-side-encryption-customer-algorithm
*
*
* -
*
* x-amz-server-side-encryption-customer-key
*
*
* -
*
* x-amz-server-side-encryption-customer-key-MD5
*
*
*
*
* For more information about server-side encryption with CMKs stored in AWS KMS (SSE-KMS), see Protecting Data Using Server-Side
* Encryption with CMKs stored in AWS KMS.
*
*
*
*
* - Access-Control-List (ACL)-Specific Request Headers
* -
*
* You also can use the following access control–related headers with this operation. By default, all objects are
* private. Only the owner has full access control. When adding a new object, you can grant permissions to
* individual AWS accounts or to predefined groups defined by Amazon S3. These permissions are then added to the
* access control list (ACL) on the object. For more information, see Using ACLs. With this
* operation, you can grant access permissions using one of the following two methods:
*
*
* -
*
* Specify a canned ACL (x-amz-acl
) — Amazon S3 supports a set of predefined ACLs, known as canned
* ACLs. Each canned ACL has a predefined set of grantees and permissions. For more information, see Canned ACL.
*
*
* -
*
* Specify access permissions explicitly — To explicitly grant access permissions to specific AWS accounts or
* groups, use the following headers. Each header maps to specific permissions that Amazon S3 supports in an ACL.
* For more information, see Access
* Control List (ACL) Overview. In the header, you specify a list of grantees who get the specific permission.
* To grant permissions explicitly, use:
*
*
* -
*
* x-amz-grant-read
*
*
* -
*
* x-amz-grant-write
*
*
* -
*
* x-amz-grant-read-acp
*
*
* -
*
* x-amz-grant-write-acp
*
*
* -
*
* x-amz-grant-full-control
*
*
*
*
* You specify each grantee as a type=value pair, where the type is one of the following:
*
*
* -
*
* id
– if the value specified is the canonical user ID of an AWS account
*
*
* -
*
* uri
– if you are granting permissions to a predefined group
*
*
* -
*
* emailAddress
– if the value specified is the email address of an AWS account
*
*
*
* Using email addresses to specify a grantee is only supported in the following AWS Regions:
*
*
* -
*
* US East (N. Virginia)
*
*
* -
*
* US West (N. California)
*
*
* -
*
* US West (Oregon)
*
*
* -
*
* Asia Pacific (Singapore)
*
*
* -
*
* Asia Pacific (Sydney)
*
*
* -
*
* Asia Pacific (Tokyo)
*
*
* -
*
* Europe (Ireland)
*
*
* -
*
* South America (São Paulo)
*
*
*
*
* For a list of all the Amazon S3 supported Regions and endpoints, see Regions and Endpoints in the AWS
* General Reference.
*
*
*
*
* For example, the following x-amz-grant-read
header grants the AWS accounts identified by account IDs
* permissions to read object data and its metadata:
*
*
* x-amz-grant-read: id="11112222333", id="444455556666"
*
*
*
*
*
*
* The following operations are related to CreateMultipartUpload
:
*
*
* -
*
* UploadPart
*
*
* -
*
*
* -
*
*
* -
*
* ListParts
*
*
* -
*
*
*
*
* @param createMultipartUploadRequest
* @return A Java Future containing the result of the CreateMultipartUpload operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.CreateMultipartUpload
*/
@Override
public CompletableFuture createMultipartUpload(
CreateMultipartUploadRequest createMultipartUploadRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, createMultipartUploadRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateMultipartUpload");
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(CreateMultipartUploadResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateMultipartUpload")
.withMarshaller(new CreateMultipartUploadRequestMarshaller(protocolFactory))
.withCombinedResponseHandler(responseHandler).withInput(createMultipartUploadRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = createMultipartUploadRequest.overrideConfiguration().orElse(
null);
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the S3 bucket. All objects (including all object versions and delete markers) in the bucket must be
* deleted before the bucket itself can be deleted.
*
*
* Related Resources
*
*
* -
*
* CreateBucket
*
*
* -
*
* DeleteObject
*
*
*
*
* @param deleteBucketRequest
* @return A Java Future containing the result of the DeleteBucket operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.DeleteBucket
*/
@Override
public CompletableFuture deleteBucket(DeleteBucketRequest deleteBucketRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteBucketRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteBucket");
HttpResponseHandler> responseHandler = protocolFactory.createCombinedResponseHandler(
DeleteBucketResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteBucket").withMarshaller(new DeleteBucketRequestMarshaller(protocolFactory))
.withCombinedResponseHandler(responseHandler).withInput(deleteBucketRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = deleteBucketRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes an analytics configuration for the bucket (specified by the analytics configuration ID).
*
*
* To use this operation, you must have permissions to perform the s3:PutAnalyticsConfiguration
action.
* The bucket owner has this permission by default. The bucket owner can grant this permission to others. For more
* information about permissions, see Permissions Related to Bucket Subresource Operations and Managing Access Permissions to Your
* Amazon S3 Resources.
*
*
* For information about the Amazon S3 analytics feature, see Amazon S3 Analytics – Storage
* Class Analysis.
*
*
* The following operations are related to DeleteBucketAnalyticsConfiguration
:
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* @param deleteBucketAnalyticsConfigurationRequest
* @return A Java Future containing the result of the DeleteBucketAnalyticsConfiguration operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.DeleteBucketAnalyticsConfiguration
*/
@Override
public CompletableFuture deleteBucketAnalyticsConfiguration(
DeleteBucketAnalyticsConfigurationRequest deleteBucketAnalyticsConfigurationRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration,
deleteBucketAnalyticsConfigurationRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteBucketAnalyticsConfiguration");
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(DeleteBucketAnalyticsConfigurationResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteBucketAnalyticsConfiguration")
.withMarshaller(new DeleteBucketAnalyticsConfigurationRequestMarshaller(protocolFactory))
.withCombinedResponseHandler(responseHandler).withInput(deleteBucketAnalyticsConfigurationRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = deleteBucketAnalyticsConfigurationRequest
.overrideConfiguration().orElse(null);
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the cors
configuration information set for the bucket.
*
*
* To use this operation, you must have permission to perform the s3:PutBucketCORS
action. The bucket
* owner has this permission by default and can grant this permission to others.
*
*
* For information about cors
, see Enabling Cross-Origin Resource Sharing in
* the Amazon Simple Storage Service Developer Guide.
*
*
* Related Resources:
*
*
* -
*
* PutBucketCors
*
*
* -
*
*
*
*
* @param deleteBucketCorsRequest
* @return A Java Future containing the result of the DeleteBucketCors operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.DeleteBucketCors
*/
@Override
public CompletableFuture deleteBucketCors(DeleteBucketCorsRequest deleteBucketCorsRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteBucketCorsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteBucketCors");
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(DeleteBucketCorsResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteBucketCors")
.withMarshaller(new DeleteBucketCorsRequestMarshaller(protocolFactory))
.withCombinedResponseHandler(responseHandler).withInput(deleteBucketCorsRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = deleteBucketCorsRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* This implementation of the DELETE operation removes default encryption from the bucket. For information about the
* Amazon S3 default encryption feature, see Amazon S3 Default Bucket
* Encryption in the Amazon Simple Storage Service Developer Guide.
*
*
* To use this operation, you must have permissions to perform the s3:PutEncryptionConfiguration
* action. The bucket owner has this permission by default. The bucket owner can grant this permission to others.
* For more information about permissions, see Permissions Related to Bucket Subresource Operations and Managing Access Permissions to your
* Amazon S3 Resources in the Amazon Simple Storage Service Developer Guide.
*
*
* Related Resources
*
*
* -
*
*
* -
*
*
*
*
* @param deleteBucketEncryptionRequest
* @return A Java Future containing the result of the DeleteBucketEncryption operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.DeleteBucketEncryption
*/
@Override
public CompletableFuture deleteBucketEncryption(
DeleteBucketEncryptionRequest deleteBucketEncryptionRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteBucketEncryptionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteBucketEncryption");
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(DeleteBucketEncryptionResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteBucketEncryption")
.withMarshaller(new DeleteBucketEncryptionRequestMarshaller(protocolFactory))
.withCombinedResponseHandler(responseHandler).withInput(deleteBucketEncryptionRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = deleteBucketEncryptionRequest.overrideConfiguration().orElse(
null);
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes an inventory configuration (identified by the inventory ID) from the bucket.
*
*
* To use this operation, you must have permissions to perform the s3:PutInventoryConfiguration
action.
* The bucket owner has this permission by default. The bucket owner can grant this permission to others. For more
* information about permissions, see Permissions Related to Bucket Subresource Operations and Managing Access Permissions to Your
* Amazon S3 Resources.
*
*
* For information about the Amazon S3 inventory feature, see Amazon S3 Inventory.
*
*
* Operations related to DeleteBucketInventoryConfiguration
include:
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* @param deleteBucketInventoryConfigurationRequest
* @return A Java Future containing the result of the DeleteBucketInventoryConfiguration operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.DeleteBucketInventoryConfiguration
*/
@Override
public CompletableFuture deleteBucketInventoryConfiguration(
DeleteBucketInventoryConfigurationRequest deleteBucketInventoryConfigurationRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration,
deleteBucketInventoryConfigurationRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteBucketInventoryConfiguration");
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(DeleteBucketInventoryConfigurationResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteBucketInventoryConfiguration")
.withMarshaller(new DeleteBucketInventoryConfigurationRequestMarshaller(protocolFactory))
.withCombinedResponseHandler(responseHandler).withInput(deleteBucketInventoryConfigurationRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = deleteBucketInventoryConfigurationRequest
.overrideConfiguration().orElse(null);
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the lifecycle configuration from the specified bucket. Amazon S3 removes all the lifecycle configuration
* rules in the lifecycle subresource associated with the bucket. Your objects never expire, and Amazon S3 no longer
* automatically deletes any objects on the basis of rules contained in the deleted lifecycle configuration.
*
*
* To use this operation, you must have permission to perform the s3:PutLifecycleConfiguration
action.
* By default, the bucket owner has this permission and the bucket owner can grant this permission to others.
*
*
* There is usually some time lag before lifecycle configuration deletion is fully propagated to all the Amazon S3
* systems.
*
*
* For more information about the object expiration, see Elements to Describe Lifecycle Actions.
*
*
* Related actions include:
*
*
* -
*
*
* -
*
*
*
*
* @param deleteBucketLifecycleRequest
* @return A Java Future containing the result of the DeleteBucketLifecycle operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.DeleteBucketLifecycle
*/
@Override
public CompletableFuture deleteBucketLifecycle(
DeleteBucketLifecycleRequest deleteBucketLifecycleRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteBucketLifecycleRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteBucketLifecycle");
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(DeleteBucketLifecycleResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteBucketLifecycle")
.withMarshaller(new DeleteBucketLifecycleRequestMarshaller(protocolFactory))
.withCombinedResponseHandler(responseHandler).withInput(deleteBucketLifecycleRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = deleteBucketLifecycleRequest.overrideConfiguration().orElse(
null);
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes a metrics configuration for the Amazon CloudWatch request metrics (specified by the metrics configuration
* ID) from the bucket. Note that this doesn't include the daily storage metrics.
*
*
* To use this operation, you must have permissions to perform the s3:PutMetricsConfiguration
action.
* The bucket owner has this permission by default. The bucket owner can grant this permission to others. For more
* information about permissions, see Permissions Related to Bucket Subresource Operations and Managing Access Permissions to Your
* Amazon S3 Resources.
*
*
* For information about CloudWatch request metrics for Amazon S3, see Monitoring Metrics with Amazon
* CloudWatch.
*
*
* The following operations are related to DeleteBucketMetricsConfiguration
:
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* @param deleteBucketMetricsConfigurationRequest
* @return A Java Future containing the result of the DeleteBucketMetricsConfiguration operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.DeleteBucketMetricsConfiguration
*/
@Override
public CompletableFuture deleteBucketMetricsConfiguration(
DeleteBucketMetricsConfigurationRequest deleteBucketMetricsConfigurationRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration,
deleteBucketMetricsConfigurationRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteBucketMetricsConfiguration");
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(DeleteBucketMetricsConfigurationResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteBucketMetricsConfiguration")
.withMarshaller(new DeleteBucketMetricsConfigurationRequestMarshaller(protocolFactory))
.withCombinedResponseHandler(responseHandler).withInput(deleteBucketMetricsConfigurationRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = deleteBucketMetricsConfigurationRequest
.overrideConfiguration().orElse(null);
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Removes OwnershipControls
for an Amazon S3 bucket. To use this operation, you must have the
* s3:PutBucketOwnershipControls
permission. For more information about Amazon S3 permissions, see Specifying Permissions in a
* Policy.
*
*
* For information about Amazon S3 Object Ownership, see Using Object Ownership.
*
*
* The following operations are related to DeleteBucketOwnershipControls
:
*
*
* -
*
*
* -
*
*
*
*
* @param deleteBucketOwnershipControlsRequest
* @return A Java Future containing the result of the DeleteBucketOwnershipControls operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.DeleteBucketOwnershipControls
*/
@Override
public CompletableFuture deleteBucketOwnershipControls(
DeleteBucketOwnershipControlsRequest deleteBucketOwnershipControlsRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration,
deleteBucketOwnershipControlsRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteBucketOwnershipControls");
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(DeleteBucketOwnershipControlsResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteBucketOwnershipControls")
.withMarshaller(new DeleteBucketOwnershipControlsRequestMarshaller(protocolFactory))
.withCombinedResponseHandler(responseHandler).withInput(deleteBucketOwnershipControlsRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = deleteBucketOwnershipControlsRequest.overrideConfiguration()
.orElse(null);
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* This implementation of the DELETE operation uses the policy subresource to delete the policy of a specified
* bucket. If you are using an identity other than the root user of the AWS account that owns the bucket, the
* calling identity must have the DeleteBucketPolicy
permissions on the specified bucket and belong to
* the bucket owner's account to use this operation.
*
*
* If you don't have DeleteBucketPolicy
permissions, Amazon S3 returns a 403 Access Denied
* error. If you have the correct permissions, but you're not using an identity that belongs to the bucket owner's
* account, Amazon S3 returns a 405 Method Not Allowed
error.
*
*
*
* As a security precaution, the root user of the AWS account that owns a bucket can always use this operation, even
* if the policy explicitly denies the root user the ability to perform this action.
*
*
*
* For more information about bucket policies, see Using Bucket Policies and
* UserPolicies.
*
*
* The following operations are related to DeleteBucketPolicy
*
*
* -
*
* CreateBucket
*
*
* -
*
* DeleteObject
*
*
*
*
* @param deleteBucketPolicyRequest
* @return A Java Future containing the result of the DeleteBucketPolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.DeleteBucketPolicy
*/
@Override
public CompletableFuture deleteBucketPolicy(DeleteBucketPolicyRequest deleteBucketPolicyRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteBucketPolicyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteBucketPolicy");
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(DeleteBucketPolicyResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteBucketPolicy")
.withMarshaller(new DeleteBucketPolicyRequestMarshaller(protocolFactory))
.withCombinedResponseHandler(responseHandler).withInput(deleteBucketPolicyRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = deleteBucketPolicyRequest.overrideConfiguration()
.orElse(null);
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the replication configuration from the bucket.
*
*
* To use this operation, you must have permissions to perform the s3:PutReplicationConfiguration
* action. The bucket owner has these permissions by default and can grant it to others. For more information about
* permissions, see Permissions Related to Bucket Subresource Operations and Managing Access Permissions to Your
* Amazon S3 Resources.
*
*
*
* It can take a while for the deletion of a replication configuration to fully propagate.
*
*
*
* For information about replication configuration, see Replication in the Amazon S3
* Developer Guide.
*
*
* The following operations are related to DeleteBucketReplication
:
*
*
* -
*
*
* -
*
*
*
*
* @param deleteBucketReplicationRequest
* @return A Java Future containing the result of the DeleteBucketReplication operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.DeleteBucketReplication
*/
@Override
public CompletableFuture deleteBucketReplication(
DeleteBucketReplicationRequest deleteBucketReplicationRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteBucketReplicationRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteBucketReplication");
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(DeleteBucketReplicationResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteBucketReplication")
.withMarshaller(new DeleteBucketReplicationRequestMarshaller(protocolFactory))
.withCombinedResponseHandler(responseHandler).withInput(deleteBucketReplicationRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = deleteBucketReplicationRequest.overrideConfiguration()
.orElse(null);
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the tags from the bucket.
*
*
* To use this operation, you must have permission to perform the s3:PutBucketTagging
action. By
* default, the bucket owner has this permission and can grant this permission to others.
*
*
* The following operations are related to DeleteBucketTagging
:
*
*
* -
*
* GetBucketTagging
*
*
* -
*
* PutBucketTagging
*
*
*
*
* @param deleteBucketTaggingRequest
* @return A Java Future containing the result of the DeleteBucketTagging operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.DeleteBucketTagging
*/
@Override
public CompletableFuture deleteBucketTagging(
DeleteBucketTaggingRequest deleteBucketTaggingRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteBucketTaggingRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteBucketTagging");
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(DeleteBucketTaggingResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteBucketTagging")
.withMarshaller(new DeleteBucketTaggingRequestMarshaller(protocolFactory))
.withCombinedResponseHandler(responseHandler).withInput(deleteBucketTaggingRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = deleteBucketTaggingRequest.overrideConfiguration().orElse(
null);
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* This operation removes the website configuration for a bucket. Amazon S3 returns a 200 OK
response
* upon successfully deleting a website configuration on the specified bucket. You will get a 200 OK
* response if the website configuration you are trying to delete does not exist on the bucket. Amazon S3 returns a
* 404
response if the bucket specified in the request does not exist.
*
*
* This DELETE operation requires the S3:DeleteBucketWebsite
permission. By default, only the bucket
* owner can delete the website configuration attached to a bucket. However, bucket owners can grant other users
* permission to delete the website configuration by writing a bucket policy granting them the
* S3:DeleteBucketWebsite
permission.
*
*
* For more information about hosting websites, see Hosting Websites on Amazon S3.
*
*
* The following operations are related to DeleteBucketWebsite
:
*
*
* -
*
* GetBucketWebsite
*
*
* -
*
* PutBucketWebsite
*
*
*
*
* @param deleteBucketWebsiteRequest
* @return A Java Future containing the result of the DeleteBucketWebsite operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.DeleteBucketWebsite
*/
@Override
public CompletableFuture deleteBucketWebsite(
DeleteBucketWebsiteRequest deleteBucketWebsiteRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteBucketWebsiteRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteBucketWebsite");
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(DeleteBucketWebsiteResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteBucketWebsite")
.withMarshaller(new DeleteBucketWebsiteRequestMarshaller(protocolFactory))
.withCombinedResponseHandler(responseHandler).withInput(deleteBucketWebsiteRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = deleteBucketWebsiteRequest.overrideConfiguration().orElse(
null);
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Removes the null version (if there is one) of an object and inserts a delete marker, which becomes the latest
* version of the object. If there isn't a null version, Amazon S3 does not remove any objects.
*
*
* To remove a specific version, you must be the bucket owner and you must use the version Id subresource. Using
* this subresource permanently deletes the version. If the object deleted is a delete marker, Amazon S3 sets the
* response header, x-amz-delete-marker
, to true.
*
*
* If the object you want to delete is in a bucket where the bucket versioning configuration is MFA Delete enabled,
* you must include the x-amz-mfa
request header in the DELETE versionId
request. Requests
* that include x-amz-mfa
must use HTTPS.
*
*
* For more information about MFA Delete, see Using MFA Delete. To see sample
* requests that use versioning, see Sample
* Request.
*
*
* You can delete objects by explicitly calling the DELETE Object API or configure its lifecycle (PutBucketLifecycle) to
* enable Amazon S3 to remove them for you. If you want to block users or accounts from removing or deleting objects
* from your bucket, you must deny them the s3:DeleteObject
, s3:DeleteObjectVersion
, and
* s3:PutLifeCycleConfiguration
actions.
*
*
* The following operation is related to DeleteObject
:
*
*
* -
*
* PutObject
*
*
*
*
* @param deleteObjectRequest
* @return A Java Future containing the result of the DeleteObject operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.DeleteObject
*/
@Override
public CompletableFuture deleteObject(DeleteObjectRequest deleteObjectRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteObjectRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteObject");
HttpResponseHandler> responseHandler = protocolFactory.createCombinedResponseHandler(
DeleteObjectResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteObject").withMarshaller(new DeleteObjectRequestMarshaller(protocolFactory))
.withCombinedResponseHandler(responseHandler).withInput(deleteObjectRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = deleteObjectRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Removes the entire tag set from the specified object. For more information about managing object tags, see Object Tagging.
*
*
* To use this operation, you must have permission to perform the s3:DeleteObjectTagging
action.
*
*
* To delete tags of a specific object version, add the versionId
query parameter in the request. You
* will need permission for the s3:DeleteObjectVersionTagging
action.
*
*
* The following operations are related to DeleteBucketMetricsConfiguration
:
*
*
* -
*
* PutObjectTagging
*
*
* -
*
* GetObjectTagging
*
*
*
*
* @param deleteObjectTaggingRequest
* @return A Java Future containing the result of the DeleteObjectTagging operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.DeleteObjectTagging
*/
@Override
public CompletableFuture deleteObjectTagging(
DeleteObjectTaggingRequest deleteObjectTaggingRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteObjectTaggingRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteObjectTagging");
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(DeleteObjectTaggingResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteObjectTagging")
.withMarshaller(new DeleteObjectTaggingRequestMarshaller(protocolFactory))
.withCombinedResponseHandler(responseHandler).withInput(deleteObjectTaggingRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = deleteObjectTaggingRequest.overrideConfiguration().orElse(
null);
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* This operation enables you to delete multiple objects from a bucket using a single HTTP request. If you know the
* object keys that you want to delete, then this operation provides a suitable alternative to sending individual
* delete requests, reducing per-request overhead.
*
*
* The request contains a list of up to 1000 keys that you want to delete. In the XML, you provide the object key
* names, and optionally, version IDs if you want to delete a specific version of the object from a
* versioning-enabled bucket. For each key, Amazon S3 performs a delete operation and returns the result of that
* delete, success, or failure, in the response. Note that if the object specified in the request is not found,
* Amazon S3 returns the result as deleted.
*
*
* The operation supports two modes for the response: verbose and quiet. By default, the operation uses verbose mode
* in which the response includes the result of deletion of each key in your request. In quiet mode the response
* includes only keys where the delete operation encountered an error. For a successful deletion, the operation does
* not return any information about the delete in the response body.
*
*
* When performing this operation on an MFA Delete enabled bucket, that attempts to delete any versioned objects,
* you must include an MFA token. If you do not provide one, the entire request will fail, even if there are
* non-versioned objects you are trying to delete. If you provide an invalid token, whether there are versioned keys
* in the request or not, the entire Multi-Object Delete request will fail. For information about MFA Delete, see MFA
* Delete.
*
*
* Finally, the Content-MD5 header is required for all Multi-Object Delete requests. Amazon S3 uses the header value
* to ensure that your request body has not been altered in transit.
*
*
* The following operations are related to DeleteObjects
:
*
*
* -
*
*
* -
*
* UploadPart
*
*
* -
*
*
* -
*
* ListParts
*
*
* -
*
*
*
*
* @param deleteObjectsRequest
* @return A Java Future containing the result of the DeleteObjects operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.DeleteObjects
*/
@Override
public CompletableFuture deleteObjects(DeleteObjectsRequest deleteObjectsRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteObjectsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteObjects");
HttpResponseHandler> responseHandler = protocolFactory.createCombinedResponseHandler(
DeleteObjectsResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteObjects")
.withMarshaller(new DeleteObjectsRequestMarshaller(protocolFactory))
.withCombinedResponseHandler(responseHandler).withInput(deleteObjectsRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = deleteObjectsRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Removes the PublicAccessBlock
configuration for an Amazon S3 bucket. To use this operation, you must
* have the s3:PutBucketPublicAccessBlock
permission. For more information about permissions, see Permissions Related to Bucket Subresource Operations and Managing Access Permissions to Your
* Amazon S3 Resources.
*
*
* The following operations are related to DeletePublicAccessBlock
:
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* @param deletePublicAccessBlockRequest
* @return A Java Future containing the result of the DeletePublicAccessBlock operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.DeletePublicAccessBlock
*/
@Override
public CompletableFuture deletePublicAccessBlock(
DeletePublicAccessBlockRequest deletePublicAccessBlockRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deletePublicAccessBlockRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeletePublicAccessBlock");
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(DeletePublicAccessBlockResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeletePublicAccessBlock")
.withMarshaller(new DeletePublicAccessBlockRequestMarshaller(protocolFactory))
.withCombinedResponseHandler(responseHandler).withInput(deletePublicAccessBlockRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = deletePublicAccessBlockRequest.overrideConfiguration()
.orElse(null);
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* This implementation of the GET operation uses the accelerate
subresource to return the Transfer
* Acceleration state of a bucket, which is either Enabled
or Suspended
. Amazon S3
* Transfer Acceleration is a bucket-level feature that enables you to perform faster data transfers to and from
* Amazon S3.
*
*
* To use this operation, you must have permission to perform the s3:GetAccelerateConfiguration
action.
* The bucket owner has this permission by default. The bucket owner can grant this permission to others. For more
* information about permissions, see Permissions Related to Bucket Subresource Operations and Managing Access Permissions to your
* Amazon S3 Resources in the Amazon Simple Storage Service Developer Guide.
*
*
* You set the Transfer Acceleration state of an existing bucket to Enabled
or Suspended
* by using the
* PutBucketAccelerateConfiguration operation.
*
*
* A GET accelerate
request does not return a state value for a bucket that has no transfer
* acceleration state. A bucket has no Transfer Acceleration state if a state has never been set on the bucket.
*
*
* For more information about transfer acceleration, see Transfer Acceleration in
* the Amazon Simple Storage Service Developer Guide.
*
*
* Related Resources
*
*
* -
*
*
*
*
* @param getBucketAccelerateConfigurationRequest
* @return A Java Future containing the result of the GetBucketAccelerateConfiguration operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.GetBucketAccelerateConfiguration
*/
@Override
public CompletableFuture getBucketAccelerateConfiguration(
GetBucketAccelerateConfigurationRequest getBucketAccelerateConfigurationRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration,
getBucketAccelerateConfigurationRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetBucketAccelerateConfiguration");
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(GetBucketAccelerateConfigurationResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetBucketAccelerateConfiguration")
.withMarshaller(new GetBucketAccelerateConfigurationRequestMarshaller(protocolFactory))
.withCombinedResponseHandler(responseHandler).withInput(getBucketAccelerateConfigurationRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = getBucketAccelerateConfigurationRequest
.overrideConfiguration().orElse(null);
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* This implementation of the GET
operation uses the acl
subresource to return the access
* control list (ACL) of a bucket. To use GET
to return the ACL of the bucket, you must have
* READ_ACP
access to the bucket. If READ_ACP
permission is granted to the anonymous user,
* you can return the ACL of the bucket without using an authorization header.
*
*
* Related Resources
*
*
* -
*
* ListObjects
*
*
*
*
* @param getBucketAclRequest
* @return A Java Future containing the result of the GetBucketAcl operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.GetBucketAcl
*/
@Override
public CompletableFuture getBucketAcl(GetBucketAclRequest getBucketAclRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, getBucketAclRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetBucketAcl");
HttpResponseHandler> responseHandler = protocolFactory.createCombinedResponseHandler(
GetBucketAclResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetBucketAcl").withMarshaller(new GetBucketAclRequestMarshaller(protocolFactory))
.withCombinedResponseHandler(responseHandler).withInput(getBucketAclRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = getBucketAclRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* This implementation of the GET operation returns an analytics configuration (identified by the analytics
* configuration ID) from the bucket.
*
*
* To use this operation, you must have permissions to perform the s3:GetAnalyticsConfiguration
action.
* The bucket owner has this permission by default. The bucket owner can grant this permission to others. For more
* information about permissions, see Permissions Related to Bucket Subresource Operations and Managing Access Permissions to Your
* Amazon S3 Resources in the Amazon Simple Storage Service Developer Guide.
*
*
* For information about Amazon S3 analytics feature, see Amazon S3 Analytics – Storage
* Class Analysis in the Amazon Simple Storage Service Developer Guide.
*
*
* Related Resources
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* @param getBucketAnalyticsConfigurationRequest
* @return A Java Future containing the result of the GetBucketAnalyticsConfiguration operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.GetBucketAnalyticsConfiguration
*/
@Override
public CompletableFuture getBucketAnalyticsConfiguration(
GetBucketAnalyticsConfigurationRequest getBucketAnalyticsConfigurationRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration,
getBucketAnalyticsConfigurationRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetBucketAnalyticsConfiguration");
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(GetBucketAnalyticsConfigurationResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetBucketAnalyticsConfiguration")
.withMarshaller(new GetBucketAnalyticsConfigurationRequestMarshaller(protocolFactory))
.withCombinedResponseHandler(responseHandler).withInput(getBucketAnalyticsConfigurationRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = getBucketAnalyticsConfigurationRequest
.overrideConfiguration().orElse(null);
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns the cors configuration information set for the bucket.
*
*
* To use this operation, you must have permission to perform the s3:GetBucketCORS action. By default, the bucket
* owner has this permission and can grant it to others.
*
*
* For more information about cors, see
* Enabling Cross-Origin Resource Sharing.
*
*
* The following operations are related to GetBucketCors
:
*
*
* -
*
* PutBucketCors
*
*
* -
*
* DeleteBucketCors
*
*
*
*
* @param getBucketCorsRequest
* @return A Java Future containing the result of the GetBucketCors operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.GetBucketCors
*/
@Override
public CompletableFuture getBucketCors(GetBucketCorsRequest getBucketCorsRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, getBucketCorsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetBucketCors");
HttpResponseHandler> responseHandler = protocolFactory.createCombinedResponseHandler(
GetBucketCorsResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetBucketCors")
.withMarshaller(new GetBucketCorsRequestMarshaller(protocolFactory))
.withCombinedResponseHandler(responseHandler).withInput(getBucketCorsRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = getBucketCorsRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns the default encryption configuration for an Amazon S3 bucket. For information about the Amazon S3 default
* encryption feature, see Amazon
* S3 Default Bucket Encryption.
*
*
* To use this operation, you must have permission to perform the s3:GetEncryptionConfiguration
action.
* The bucket owner has this permission by default. The bucket owner can grant this permission to others. For more
* information about permissions, see Permissions Related to Bucket Subresource Operations and Managing Access Permissions to Your
* Amazon S3 Resources.
*
*
* The following operations are related to GetBucketEncryption
:
*
*
* -
*
*
* -
*
*
*
*
* @param getBucketEncryptionRequest
* @return A Java Future containing the result of the GetBucketEncryption operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.GetBucketEncryption
*/
@Override
public CompletableFuture getBucketEncryption(
GetBucketEncryptionRequest getBucketEncryptionRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, getBucketEncryptionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetBucketEncryption");
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(GetBucketEncryptionResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetBucketEncryption")
.withMarshaller(new GetBucketEncryptionRequestMarshaller(protocolFactory))
.withCombinedResponseHandler(responseHandler).withInput(getBucketEncryptionRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = getBucketEncryptionRequest.overrideConfiguration().orElse(
null);
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns an inventory configuration (identified by the inventory configuration ID) from the bucket.
*
*
* To use this operation, you must have permissions to perform the s3:GetInventoryConfiguration
action.
* The bucket owner has this permission by default and can grant this permission to others. For more information
* about permissions, see Permissions Related to Bucket Subresource Operations and Managing Access Permissions to Your
* Amazon S3 Resources.
*
*
* For information about the Amazon S3 inventory feature, see Amazon S3 Inventory.
*
*
* The following operations are related to GetBucketInventoryConfiguration
:
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* @param getBucketInventoryConfigurationRequest
* @return A Java Future containing the result of the GetBucketInventoryConfiguration operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.GetBucketInventoryConfiguration
*/
@Override
public CompletableFuture getBucketInventoryConfiguration(
GetBucketInventoryConfigurationRequest getBucketInventoryConfigurationRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration,
getBucketInventoryConfigurationRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetBucketInventoryConfiguration");
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(GetBucketInventoryConfigurationResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetBucketInventoryConfiguration")
.withMarshaller(new GetBucketInventoryConfigurationRequestMarshaller(protocolFactory))
.withCombinedResponseHandler(responseHandler).withInput(getBucketInventoryConfigurationRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = getBucketInventoryConfigurationRequest
.overrideConfiguration().orElse(null);
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
*
* Bucket lifecycle configuration now supports specifying a lifecycle rule using an object key name prefix, one or
* more object tags, or a combination of both. Accordingly, this section describes the latest API. The response
* describes the new filter element that you can use to specify a filter to select a subset of objects to which the
* rule applies. If you are using a previous version of the lifecycle configuration, it still works. For the earlier
* API description, see GetBucketLifecycle.
*
*
*
* Returns the lifecycle configuration information set on the bucket. For information about lifecycle configuration,
* see Object Lifecycle
* Management.
*
*
* To use this operation, you must have permission to perform the s3:GetLifecycleConfiguration
action.
* The bucket owner has this permission, by default. The bucket owner can grant this permission to others. For more
* information about permissions, see Permissions Related to Bucket Subresource Operations and Managing Access Permissions to Your
* Amazon S3 Resources.
*
*
* GetBucketLifecycleConfiguration
has the following special error:
*
*
* -
*
* Error code: NoSuchLifecycleConfiguration
*
*
* -
*
* Description: The lifecycle configuration does not exist.
*
*
* -
*
* HTTP Status Code: 404 Not Found
*
*
* -
*
* SOAP Fault Code Prefix: Client
*
*
*
*
*
*
* The following operations are related to GetBucketLifecycleConfiguration
:
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* @param getBucketLifecycleConfigurationRequest
* @return A Java Future containing the result of the GetBucketLifecycleConfiguration operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.GetBucketLifecycleConfiguration
*/
@Override
public CompletableFuture getBucketLifecycleConfiguration(
GetBucketLifecycleConfigurationRequest getBucketLifecycleConfigurationRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration,
getBucketLifecycleConfigurationRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetBucketLifecycleConfiguration");
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(GetBucketLifecycleConfigurationResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetBucketLifecycleConfiguration")
.withMarshaller(new GetBucketLifecycleConfigurationRequestMarshaller(protocolFactory))
.withCombinedResponseHandler(responseHandler).withInput(getBucketLifecycleConfigurationRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = getBucketLifecycleConfigurationRequest
.overrideConfiguration().orElse(null);
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns the Region the bucket resides in. You set the bucket's Region using the LocationConstraint
* request parameter in a CreateBucket
request. For more information, see CreateBucket.
*
*
* To use this implementation of the operation, you must be the bucket owner.
*
*
* The following operations are related to GetBucketLocation
:
*
*
* -
*
* GetObject
*
*
* -
*
* CreateBucket
*
*
*
*
* @param getBucketLocationRequest
* @return A Java Future containing the result of the GetBucketLocation operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.GetBucketLocation
*/
@Override
public CompletableFuture getBucketLocation(GetBucketLocationRequest getBucketLocationRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, getBucketLocationRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetBucketLocation");
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(GetBucketLocationResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetBucketLocation")
.withMarshaller(new GetBucketLocationRequestMarshaller(protocolFactory))
.withCombinedResponseHandler(responseHandler).withInput(getBucketLocationRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = getBucketLocationRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns the logging status of a bucket and the permissions users have to view and modify that status. To use GET,
* you must be the bucket owner.
*
*
* The following operations are related to GetBucketLogging
:
*
*
* -
*
* CreateBucket
*
*
* -
*
* PutBucketLogging
*
*
*
*
* @param getBucketLoggingRequest
* @return A Java Future containing the result of the GetBucketLogging operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.GetBucketLogging
*/
@Override
public CompletableFuture getBucketLogging(GetBucketLoggingRequest getBucketLoggingRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, getBucketLoggingRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetBucketLogging");
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(GetBucketLoggingResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetBucketLogging")
.withMarshaller(new GetBucketLoggingRequestMarshaller(protocolFactory))
.withCombinedResponseHandler(responseHandler).withInput(getBucketLoggingRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = getBucketLoggingRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Gets a metrics configuration (specified by the metrics configuration ID) from the bucket. Note that this doesn't
* include the daily storage metrics.
*
*
* To use this operation, you must have permissions to perform the s3:GetMetricsConfiguration
action.
* The bucket owner has this permission by default. The bucket owner can grant this permission to others. For more
* information about permissions, see Permissions Related to Bucket Subresource Operations and Managing Access Permissions to Your
* Amazon S3 Resources.
*
*
* For information about CloudWatch request metrics for Amazon S3, see Monitoring Metrics with Amazon
* CloudWatch.
*
*
* The following operations are related to GetBucketMetricsConfiguration
:
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* @param getBucketMetricsConfigurationRequest
* @return A Java Future containing the result of the GetBucketMetricsConfiguration operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.GetBucketMetricsConfiguration
*/
@Override
public CompletableFuture getBucketMetricsConfiguration(
GetBucketMetricsConfigurationRequest getBucketMetricsConfigurationRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration,
getBucketMetricsConfigurationRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetBucketMetricsConfiguration");
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(GetBucketMetricsConfigurationResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetBucketMetricsConfiguration")
.withMarshaller(new GetBucketMetricsConfigurationRequestMarshaller(protocolFactory))
.withCombinedResponseHandler(responseHandler).withInput(getBucketMetricsConfigurationRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = getBucketMetricsConfigurationRequest.overrideConfiguration()
.orElse(null);
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns the notification configuration of a bucket.
*
*
* If notifications are not enabled on the bucket, the operation returns an empty
* NotificationConfiguration
element.
*
*
* By default, you must be the bucket owner to read the notification configuration of a bucket. However, the bucket
* owner can use a bucket policy to grant permission to other users to read this configuration with the
* s3:GetBucketNotification
permission.
*
*
* For more information about setting and reading the notification configuration on a bucket, see Setting Up Notification of Bucket
* Events. For more information about bucket policies, see Using Bucket Policies.
*
*
* The following operation is related to GetBucketNotification
:
*
*
* -
*
*
*
*
* @param getBucketNotificationConfigurationRequest
* @return A Java Future containing the result of the GetBucketNotificationConfiguration operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.GetBucketNotificationConfiguration
*/
@Override
public CompletableFuture getBucketNotificationConfiguration(
GetBucketNotificationConfigurationRequest getBucketNotificationConfigurationRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration,
getBucketNotificationConfigurationRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetBucketNotificationConfiguration");
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(GetBucketNotificationConfigurationResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetBucketNotificationConfiguration")
.withMarshaller(new GetBucketNotificationConfigurationRequestMarshaller(protocolFactory))
.withCombinedResponseHandler(responseHandler).withInput(getBucketNotificationConfigurationRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = getBucketNotificationConfigurationRequest
.overrideConfiguration().orElse(null);
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieves OwnershipControls
for an Amazon S3 bucket. To use this operation, you must have the
* s3:GetBucketOwnershipControls
permission. For more information about Amazon S3 permissions, see Specifying Permissions in a
* Policy.
*
*
* For information about Amazon S3 Object Ownership, see Using Object Ownership.
*
*
* The following operations are related to GetBucketOwnershipControls
:
*
*
* -
*
*
* -
*
*
*
*
* @param getBucketOwnershipControlsRequest
* @return A Java Future containing the result of the GetBucketOwnershipControls operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.GetBucketOwnershipControls
*/
@Override
public CompletableFuture getBucketOwnershipControls(
GetBucketOwnershipControlsRequest getBucketOwnershipControlsRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, getBucketOwnershipControlsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetBucketOwnershipControls");
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(GetBucketOwnershipControlsResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetBucketOwnershipControls")
.withMarshaller(new GetBucketOwnershipControlsRequestMarshaller(protocolFactory))
.withCombinedResponseHandler(responseHandler).withInput(getBucketOwnershipControlsRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = getBucketOwnershipControlsRequest.overrideConfiguration()
.orElse(null);
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns the policy of a specified bucket. If you are using an identity other than the root user of the AWS
* account that owns the bucket, the calling identity must have the GetBucketPolicy
permissions on the
* specified bucket and belong to the bucket owner's account in order to use this operation.
*
*
* If you don't have GetBucketPolicy
permissions, Amazon S3 returns a 403 Access Denied
* error. If you have the correct permissions, but you're not using an identity that belongs to the bucket owner's
* account, Amazon S3 returns a 405 Method Not Allowed
error.
*
*
*
* As a security precaution, the root user of the AWS account that owns a bucket can always use this operation, even
* if the policy explicitly denies the root user the ability to perform this action.
*
*
*
* For more information about bucket policies, see Using Bucket Policies and User
* Policies.
*
*
* The following operation is related to GetBucketPolicy
:
*
*
* -
*
* GetObject
*
*
*
*
* @param getBucketPolicyRequest
* @return A Java Future containing the result of the GetBucketPolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.GetBucketPolicy
*/
@Override
public CompletableFuture getBucketPolicy(GetBucketPolicyRequest getBucketPolicyRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, getBucketPolicyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetBucketPolicy");
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(GetBucketPolicyResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetBucketPolicy")
.withMarshaller(new GetBucketPolicyRequestMarshaller(protocolFactory))
.withCombinedResponseHandler(responseHandler).withInput(getBucketPolicyRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = getBucketPolicyRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieves the policy status for an Amazon S3 bucket, indicating whether the bucket is public. In order to use
* this operation, you must have the s3:GetBucketPolicyStatus
permission. For more information about
* Amazon S3 permissions, see Specifying Permissions in a
* Policy.
*
*
* For more information about when Amazon S3 considers a bucket public, see The Meaning of "Public".
*
*
* The following operations are related to GetBucketPolicyStatus
:
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* @param getBucketPolicyStatusRequest
* @return A Java Future containing the result of the GetBucketPolicyStatus operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.GetBucketPolicyStatus
*/
@Override
public CompletableFuture getBucketPolicyStatus(
GetBucketPolicyStatusRequest getBucketPolicyStatusRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, getBucketPolicyStatusRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetBucketPolicyStatus");
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(GetBucketPolicyStatusResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetBucketPolicyStatus")
.withMarshaller(new GetBucketPolicyStatusRequestMarshaller(protocolFactory))
.withCombinedResponseHandler(responseHandler).withInput(getBucketPolicyStatusRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = getBucketPolicyStatusRequest.overrideConfiguration().orElse(
null);
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns the replication configuration of a bucket.
*
*
*
* It can take a while to propagate the put or delete a replication configuration to all Amazon S3 systems.
* Therefore, a get request soon after put or delete can return a wrong result.
*
*
*
* For information about replication configuration, see Replication in the Amazon Simple
* Storage Service Developer Guide.
*
*
* This operation requires permissions for the s3:GetReplicationConfiguration
action. For more
* information about permissions, see Using Bucket Policies and User
* Policies.
*
*
* If you include the Filter
element in a replication configuration, you must also include the
* DeleteMarkerReplication
and Priority
elements. The response also returns those
* elements.
*
*
* For information about GetBucketReplication
errors, see List of
* replication-related error codes
*
*
* The following operations are related to GetBucketReplication
:
*
*
* -
*
*
* -
*
*
*
*
* @param getBucketReplicationRequest
* @return A Java Future containing the result of the GetBucketReplication operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.GetBucketReplication
*/
@Override
public CompletableFuture getBucketReplication(
GetBucketReplicationRequest getBucketReplicationRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, getBucketReplicationRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetBucketReplication");
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(GetBucketReplicationResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetBucketReplication")
.withMarshaller(new GetBucketReplicationRequestMarshaller(protocolFactory))
.withCombinedResponseHandler(responseHandler).withInput(getBucketReplicationRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = getBucketReplicationRequest.overrideConfiguration().orElse(
null);
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns the request payment configuration of a bucket. To use this version of the operation, you must be the
* bucket owner. For more information, see Requester Pays Buckets.
*
*
* The following operations are related to GetBucketRequestPayment
:
*
*
* -
*
* ListObjects
*
*
*
*
* @param getBucketRequestPaymentRequest
* @return A Java Future containing the result of the GetBucketRequestPayment operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.GetBucketRequestPayment
*/
@Override
public CompletableFuture getBucketRequestPayment(
GetBucketRequestPaymentRequest getBucketRequestPaymentRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, getBucketRequestPaymentRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetBucketRequestPayment");
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(GetBucketRequestPaymentResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetBucketRequestPayment")
.withMarshaller(new GetBucketRequestPaymentRequestMarshaller(protocolFactory))
.withCombinedResponseHandler(responseHandler).withInput(getBucketRequestPaymentRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = getBucketRequestPaymentRequest.overrideConfiguration()
.orElse(null);
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns the tag set associated with the bucket.
*
*
* To use this operation, you must have permission to perform the s3:GetBucketTagging
action. By
* default, the bucket owner has this permission and can grant this permission to others.
*
*
* GetBucketTagging
has the following special error:
*
*
* -
*
* Error code: NoSuchTagSetError
*
*
* -
*
* Description: There is no tag set associated with the bucket.
*
*
*
*
*
*
* The following operations are related to GetBucketTagging
:
*
*
* -
*
* PutBucketTagging
*
*
* -
*
*
*
*
* @param getBucketTaggingRequest
* @return A Java Future containing the result of the GetBucketTagging operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.GetBucketTagging
*/
@Override
public CompletableFuture getBucketTagging(GetBucketTaggingRequest getBucketTaggingRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, getBucketTaggingRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetBucketTagging");
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(GetBucketTaggingResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetBucketTagging")
.withMarshaller(new GetBucketTaggingRequestMarshaller(protocolFactory))
.withCombinedResponseHandler(responseHandler).withInput(getBucketTaggingRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = getBucketTaggingRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns the versioning state of a bucket.
*
*
* To retrieve the versioning state of a bucket, you must be the bucket owner.
*
*
* This implementation also returns the MFA Delete status of the versioning state. If the MFA Delete status is
* enabled
, the bucket owner must use an authentication device to change the versioning state of the
* bucket.
*
*
* The following operations are related to GetBucketVersioning
:
*
*
* -
*
* GetObject
*
*
* -
*
* PutObject
*
*
* -
*
* DeleteObject
*
*
*
*
* @param getBucketVersioningRequest
* @return A Java Future containing the result of the GetBucketVersioning operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.GetBucketVersioning
*/
@Override
public CompletableFuture getBucketVersioning(
GetBucketVersioningRequest getBucketVersioningRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, getBucketVersioningRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetBucketVersioning");
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(GetBucketVersioningResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetBucketVersioning")
.withMarshaller(new GetBucketVersioningRequestMarshaller(protocolFactory))
.withCombinedResponseHandler(responseHandler).withInput(getBucketVersioningRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = getBucketVersioningRequest.overrideConfiguration().orElse(
null);
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns the website configuration for a bucket. To host website on Amazon S3, you can configure a bucket as
* website by adding a website configuration. For more information about hosting websites, see Hosting Websites on Amazon S3.
*
*
* This GET operation requires the S3:GetBucketWebsite
permission. By default, only the bucket owner
* can read the bucket website configuration. However, bucket owners can allow other users to read the website
* configuration by writing a bucket policy granting them the S3:GetBucketWebsite
permission.
*
*
* The following operations are related to DeleteBucketWebsite
:
*
*
* -
*
*
* -
*
* PutBucketWebsite
*
*
*
*
* @param getBucketWebsiteRequest
* @return A Java Future containing the result of the GetBucketWebsite operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.GetBucketWebsite
*/
@Override
public CompletableFuture getBucketWebsite(GetBucketWebsiteRequest getBucketWebsiteRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, getBucketWebsiteRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetBucketWebsite");
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(GetBucketWebsiteResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetBucketWebsite")
.withMarshaller(new GetBucketWebsiteRequestMarshaller(protocolFactory))
.withCombinedResponseHandler(responseHandler).withInput(getBucketWebsiteRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = getBucketWebsiteRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieves objects from Amazon S3. To use GET
, you must have READ
access to the object.
* If you grant READ
access to the anonymous user, you can return the object without using an
* authorization header.
*
*
* An Amazon S3 bucket has no directory hierarchy such as you would find in a typical computer file system. You can,
* however, create a logical hierarchy by using object key names that imply a folder structure. For example, instead
* of naming an object sample.jpg
, you can name it photos/2006/February/sample.jpg
.
*
*
* To get an object from such a logical hierarchy, specify the full key name for the object in the GET
* operation. For a virtual hosted-style request example, if you have the object
* photos/2006/February/sample.jpg
, specify the resource as
* /photos/2006/February/sample.jpg
. For a path-style request example, if you have the object
* photos/2006/February/sample.jpg
in the bucket named examplebucket
, specify the resource
* as /examplebucket/photos/2006/February/sample.jpg
. For more information about request types, see HTTP Host
* Header Bucket Specification.
*
*
* To distribute large files to many people, you can save bandwidth costs by using BitTorrent. For more information,
* see Amazon S3 Torrent. For more
* information about returning the ACL of an object, see GetObjectAcl.
*
*
* If the object you are retrieving is stored in the GLACIER or DEEP_ARCHIVE storage classes, before you can
* retrieve the object you must first restore a copy using RestoreObject. Otherwise, this
* operation returns an InvalidObjectStateError
error. For information about restoring archived
* objects, see Restoring Archived
* Objects.
*
*
* Encryption request headers, like x-amz-server-side-encryption
, should not be sent for GET requests
* if your object uses server-side encryption with CMKs stored in AWS KMS (SSE-KMS) or server-side encryption with
* Amazon S3–managed encryption keys (SSE-S3). If your object does use these types of keys, you’ll get an HTTP 400
* BadRequest error.
*
*
* If you encrypt an object by using server-side encryption with customer-provided encryption keys (SSE-C) when you
* store the object in Amazon S3, then when you GET the object, you must use the following headers:
*
*
* -
*
* x-amz-server-side-encryption-customer-algorithm
*
*
* -
*
* x-amz-server-side-encryption-customer-key
*
*
* -
*
* x-amz-server-side-encryption-customer-key-MD5
*
*
*
*
* For more information about SSE-C, see Server-Side
* Encryption (Using Customer-Provided Encryption Keys).
*
*
* Assuming you have permission to read object tags (permission for the s3:GetObjectVersionTagging
* action), the response also returns the x-amz-tagging-count
header that provides the count of number
* of tags associated with the object. You can use GetObjectTagging to retrieve
* the tag set associated with an object.
*
*
* Permissions
*
*
* You need the s3:GetObject
permission for this operation. For more information, see Specifying Permissions in a
* Policy. If the object you request does not exist, the error Amazon S3 returns depends on whether you also
* have the s3:ListBucket
permission.
*
*
* -
*
* If you have the s3:ListBucket
permission on the bucket, Amazon S3 will return an HTTP status code
* 404 ("no such key") error.
*
*
* -
*
* If you don’t have the s3:ListBucket
permission, Amazon S3 will return an HTTP status code 403
* ("access denied") error.
*
*
*
*
* Versioning
*
*
* By default, the GET operation returns the current version of an object. To return a different version, use the
* versionId
subresource.
*
*
*
* If the current version of the object is a delete marker, Amazon S3 behaves as if the object was deleted and
* includes x-amz-delete-marker: true
in the response.
*
*
*
* For more information about versioning, see PutBucketVersioning.
*
*
* Overriding Response Header Values
*
*
* There are times when you want to override certain response header values in a GET response. For example, you
* might override the Content-Disposition response header value in your GET request.
*
*
* You can override values for a set of response headers using the following query parameters. These response header
* values are sent only on a successful request, that is, when status code 200 OK is returned. The set of headers
* you can override using these parameters is a subset of the headers that Amazon S3 accepts when you create an
* object. The response headers that you can override for the GET response are Content-Type
,
* Content-Language
, Expires
, Cache-Control
, Content-Disposition
* , and Content-Encoding
. To override these header values in the GET response, you use the following
* request parameters.
*
*
*
* You must sign the request, either using an Authorization header or a presigned URL, when using these parameters.
* They cannot be used with an unsigned (anonymous) request.
*
*
*
* -
*
* response-content-type
*
*
* -
*
* response-content-language
*
*
* -
*
* response-expires
*
*
* -
*
* response-cache-control
*
*
* -
*
* response-content-disposition
*
*
* -
*
* response-content-encoding
*
*
*
*
* Additional Considerations about Request Headers
*
*
* If both of the If-Match
and If-Unmodified-Since
headers are present in the request as
* follows: If-Match
condition evaluates to true
, and; If-Unmodified-Since
* condition evaluates to false
; then, S3 returns 200 OK and the data requested.
*
*
* If both of the If-None-Match
and If-Modified-Since
headers are present in the request
* as follows: If-None-Match
condition evaluates to false
, and;
* If-Modified-Since
condition evaluates to true
; then, S3 returns 304 Not Modified
* response code.
*
*
* For more information about conditional requests, see RFC 7232.
*
*
* The following operations are related to GetObject
:
*
*
* -
*
* ListBuckets
*
*
* -
*
* GetObjectAcl
*
*
*
*
* @param getObjectRequest
* @param asyncResponseTransformer
* The response transformer for processing the streaming response in a non-blocking manner. See
* {@link AsyncResponseTransformer} for details on how this callback should be implemented and for links to
* precanned implementations for common scenarios like downloading to a file. The service documentation for
* the response content is as follows '
*
* Object data.
*
* '.
* @return A future to the transformed result of the AsyncResponseTransformer.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NoSuchKeyException The specified key does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.GetObject
*/
@Override
public CompletableFuture getObject(GetObjectRequest getObjectRequest,
AsyncResponseTransformer asyncResponseTransformer) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, getObjectRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetObject");
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
GetObjectResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(true));
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler.execute(
new ClientExecutionParams().withOperationName("GetObject")
.withMarshaller(new GetObjectRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withMetricCollector(apiCallMetricCollector)
.withInput(getObjectRequest), asyncResponseTransformer);
AwsRequestOverrideConfiguration requestOverrideConfig = getObjectRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
if (e != null) {
runAndLogError(log, "Exception thrown in exceptionOccurred callback, ignoring",
() -> asyncResponseTransformer.exceptionOccurred(e));
}
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
runAndLogError(log, "Exception thrown in exceptionOccurred callback, ignoring",
() -> asyncResponseTransformer.exceptionOccurred(t));
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns the access control list (ACL) of an object. To use this operation, you must have