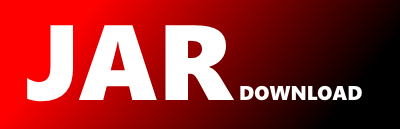
software.amazon.awssdk.services.s3.model.ListPartsResponse Maven / Gradle / Ivy
Show all versions of s3 Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.s3.model;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class ListPartsResponse extends S3Response implements
ToCopyableBuilder {
private static final SdkField ABORT_DATE_FIELD = SdkField
. builder(MarshallingType.INSTANT)
.memberName("AbortDate")
.getter(getter(ListPartsResponse::abortDate))
.setter(setter(Builder::abortDate))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("x-amz-abort-date")
.unmarshallLocationName("x-amz-abort-date").build()).build();
private static final SdkField ABORT_RULE_ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("AbortRuleId")
.getter(getter(ListPartsResponse::abortRuleId))
.setter(setter(Builder::abortRuleId))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("x-amz-abort-rule-id")
.unmarshallLocationName("x-amz-abort-rule-id").build()).build();
private static final SdkField BUCKET_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("Bucket")
.getter(getter(ListPartsResponse::bucket))
.setter(setter(Builder::bucket))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Bucket")
.unmarshallLocationName("Bucket").build()).build();
private static final SdkField KEY_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("Key")
.getter(getter(ListPartsResponse::key))
.setter(setter(Builder::key))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Key").unmarshallLocationName("Key")
.build()).build();
private static final SdkField UPLOAD_ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("UploadId")
.getter(getter(ListPartsResponse::uploadId))
.setter(setter(Builder::uploadId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("UploadId")
.unmarshallLocationName("UploadId").build()).build();
private static final SdkField PART_NUMBER_MARKER_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("PartNumberMarker")
.getter(getter(ListPartsResponse::partNumberMarker))
.setter(setter(Builder::partNumberMarker))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PartNumberMarker")
.unmarshallLocationName("PartNumberMarker").build()).build();
private static final SdkField NEXT_PART_NUMBER_MARKER_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("NextPartNumberMarker")
.getter(getter(ListPartsResponse::nextPartNumberMarker))
.setter(setter(Builder::nextPartNumberMarker))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NextPartNumberMarker")
.unmarshallLocationName("NextPartNumberMarker").build()).build();
private static final SdkField MAX_PARTS_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("MaxParts")
.getter(getter(ListPartsResponse::maxParts))
.setter(setter(Builder::maxParts))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MaxParts")
.unmarshallLocationName("MaxParts").build()).build();
private static final SdkField IS_TRUNCATED_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("IsTruncated")
.getter(getter(ListPartsResponse::isTruncated))
.setter(setter(Builder::isTruncated))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IsTruncated")
.unmarshallLocationName("IsTruncated").build()).build();
private static final SdkField> PARTS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Parts")
.getter(getter(ListPartsResponse::parts))
.setter(setter(Builder::parts))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Part")
.unmarshallLocationName("Part").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Part::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").unmarshallLocationName("member").build()).build())
.isFlattened(true).build()).build();
private static final SdkField INITIATOR_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("Initiator")
.getter(getter(ListPartsResponse::initiator))
.setter(setter(Builder::initiator))
.constructor(Initiator::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Initiator")
.unmarshallLocationName("Initiator").build()).build();
private static final SdkField OWNER_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("Owner")
.getter(getter(ListPartsResponse::owner))
.setter(setter(Builder::owner))
.constructor(Owner::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Owner")
.unmarshallLocationName("Owner").build()).build();
private static final SdkField STORAGE_CLASS_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("StorageClass")
.getter(getter(ListPartsResponse::storageClassAsString))
.setter(setter(Builder::storageClass))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StorageClass")
.unmarshallLocationName("StorageClass").build()).build();
private static final SdkField REQUEST_CHARGED_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("RequestCharged")
.getter(getter(ListPartsResponse::requestChargedAsString))
.setter(setter(Builder::requestCharged))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("x-amz-request-charged")
.unmarshallLocationName("x-amz-request-charged").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ABORT_DATE_FIELD,
ABORT_RULE_ID_FIELD, BUCKET_FIELD, KEY_FIELD, UPLOAD_ID_FIELD, PART_NUMBER_MARKER_FIELD,
NEXT_PART_NUMBER_MARKER_FIELD, MAX_PARTS_FIELD, IS_TRUNCATED_FIELD, PARTS_FIELD, INITIATOR_FIELD, OWNER_FIELD,
STORAGE_CLASS_FIELD, REQUEST_CHARGED_FIELD));
private final Instant abortDate;
private final String abortRuleId;
private final String bucket;
private final String key;
private final String uploadId;
private final Integer partNumberMarker;
private final Integer nextPartNumberMarker;
private final Integer maxParts;
private final Boolean isTruncated;
private final List parts;
private final Initiator initiator;
private final Owner owner;
private final String storageClass;
private final String requestCharged;
private ListPartsResponse(BuilderImpl builder) {
super(builder);
this.abortDate = builder.abortDate;
this.abortRuleId = builder.abortRuleId;
this.bucket = builder.bucket;
this.key = builder.key;
this.uploadId = builder.uploadId;
this.partNumberMarker = builder.partNumberMarker;
this.nextPartNumberMarker = builder.nextPartNumberMarker;
this.maxParts = builder.maxParts;
this.isTruncated = builder.isTruncated;
this.parts = builder.parts;
this.initiator = builder.initiator;
this.owner = builder.owner;
this.storageClass = builder.storageClass;
this.requestCharged = builder.requestCharged;
}
/**
*
* If the bucket has a lifecycle rule configured with an action to abort incomplete multipart uploads and the prefix
* in the lifecycle rule matches the object name in the request, then the response includes this header indicating
* when the initiated multipart upload will become eligible for abort operation. For more information, see Aborting Incomplete Multipart Uploads Using a Bucket Lifecycle Policy.
*
*
* The response will also include the x-amz-abort-rule-id
header that will provide the ID of the
* lifecycle configuration rule that defines this action.
*
*
* @return If the bucket has a lifecycle rule configured with an action to abort incomplete multipart uploads and
* the prefix in the lifecycle rule matches the object name in the request, then the response includes this
* header indicating when the initiated multipart upload will become eligible for abort operation. For more
* information, see Aborting Incomplete Multipart Uploads Using a Bucket Lifecycle Policy.
*
* The response will also include the x-amz-abort-rule-id
header that will provide the ID of
* the lifecycle configuration rule that defines this action.
*/
public final Instant abortDate() {
return abortDate;
}
/**
*
* This header is returned along with the x-amz-abort-date
header. It identifies applicable lifecycle
* configuration rule that defines the action to abort incomplete multipart uploads.
*
*
* @return This header is returned along with the x-amz-abort-date
header. It identifies applicable
* lifecycle configuration rule that defines the action to abort incomplete multipart uploads.
*/
public final String abortRuleId() {
return abortRuleId;
}
/**
*
* The name of the bucket to which the multipart upload was initiated.
*
*
* @return The name of the bucket to which the multipart upload was initiated.
*/
public final String bucket() {
return bucket;
}
/**
*
* Object key for which the multipart upload was initiated.
*
*
* @return Object key for which the multipart upload was initiated.
*/
public final String key() {
return key;
}
/**
*
* Upload ID identifying the multipart upload whose parts are being listed.
*
*
* @return Upload ID identifying the multipart upload whose parts are being listed.
*/
public final String uploadId() {
return uploadId;
}
/**
*
* When a list is truncated, this element specifies the last part in the list, as well as the value to use for the
* part-number-marker request parameter in a subsequent request.
*
*
* @return When a list is truncated, this element specifies the last part in the list, as well as the value to use
* for the part-number-marker request parameter in a subsequent request.
*/
public final Integer partNumberMarker() {
return partNumberMarker;
}
/**
*
* When a list is truncated, this element specifies the last part in the list, as well as the value to use for the
* part-number-marker request parameter in a subsequent request.
*
*
* @return When a list is truncated, this element specifies the last part in the list, as well as the value to use
* for the part-number-marker request parameter in a subsequent request.
*/
public final Integer nextPartNumberMarker() {
return nextPartNumberMarker;
}
/**
*
* Maximum number of parts that were allowed in the response.
*
*
* @return Maximum number of parts that were allowed in the response.
*/
public final Integer maxParts() {
return maxParts;
}
/**
*
* Indicates whether the returned list of parts is truncated. A true value indicates that the list was truncated. A
* list can be truncated if the number of parts exceeds the limit returned in the MaxParts element.
*
*
* @return Indicates whether the returned list of parts is truncated. A true value indicates that the list was
* truncated. A list can be truncated if the number of parts exceeds the limit returned in the MaxParts
* element.
*/
public final Boolean isTruncated() {
return isTruncated;
}
/**
* Returns true if the Parts property was specified by the sender (it may be empty), or false if the sender did not
* specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS service.
*/
public final boolean hasParts() {
return parts != null && !(parts instanceof SdkAutoConstructList);
}
/**
*
* Container for elements related to a particular part. A response can contain zero or more Part
* elements.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasParts()} to see if a value was sent in this field.
*
*
* @return Container for elements related to a particular part. A response can contain zero or more
* Part
elements.
*/
public final List parts() {
return parts;
}
/**
*
* Container element that identifies who initiated the multipart upload. If the initiator is an AWS account, this
* element provides the same information as the Owner
element. If the initiator is an IAM User, this
* element provides the user ARN and display name.
*
*
* @return Container element that identifies who initiated the multipart upload. If the initiator is an AWS account,
* this element provides the same information as the Owner
element. If the initiator is an IAM
* User, this element provides the user ARN and display name.
*/
public final Initiator initiator() {
return initiator;
}
/**
*
* Container element that identifies the object owner, after the object is created. If multipart upload is initiated
* by an IAM user, this element provides the parent account ID and display name.
*
*
* @return Container element that identifies the object owner, after the object is created. If multipart upload is
* initiated by an IAM user, this element provides the parent account ID and display name.
*/
public final Owner owner() {
return owner;
}
/**
*
* Class of storage (STANDARD or REDUCED_REDUNDANCY) used to store the uploaded object.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #storageClass} will
* return {@link StorageClass#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #storageClassAsString}.
*
*
* @return Class of storage (STANDARD or REDUCED_REDUNDANCY) used to store the uploaded object.
* @see StorageClass
*/
public final StorageClass storageClass() {
return StorageClass.fromValue(storageClass);
}
/**
*
* Class of storage (STANDARD or REDUCED_REDUNDANCY) used to store the uploaded object.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #storageClass} will
* return {@link StorageClass#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #storageClassAsString}.
*
*
* @return Class of storage (STANDARD or REDUCED_REDUNDANCY) used to store the uploaded object.
* @see StorageClass
*/
public final String storageClassAsString() {
return storageClass;
}
/**
* Returns the value of the RequestCharged property for this object.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #requestCharged}
* will return {@link RequestCharged#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #requestChargedAsString}.
*
*
* @return The value of the RequestCharged property for this object.
* @see RequestCharged
*/
public final RequestCharged requestCharged() {
return RequestCharged.fromValue(requestCharged);
}
/**
* Returns the value of the RequestCharged property for this object.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #requestCharged}
* will return {@link RequestCharged#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #requestChargedAsString}.
*
*
* @return The value of the RequestCharged property for this object.
* @see RequestCharged
*/
public final String requestChargedAsString() {
return requestCharged;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(abortDate());
hashCode = 31 * hashCode + Objects.hashCode(abortRuleId());
hashCode = 31 * hashCode + Objects.hashCode(bucket());
hashCode = 31 * hashCode + Objects.hashCode(key());
hashCode = 31 * hashCode + Objects.hashCode(uploadId());
hashCode = 31 * hashCode + Objects.hashCode(partNumberMarker());
hashCode = 31 * hashCode + Objects.hashCode(nextPartNumberMarker());
hashCode = 31 * hashCode + Objects.hashCode(maxParts());
hashCode = 31 * hashCode + Objects.hashCode(isTruncated());
hashCode = 31 * hashCode + Objects.hashCode(hasParts() ? parts() : null);
hashCode = 31 * hashCode + Objects.hashCode(initiator());
hashCode = 31 * hashCode + Objects.hashCode(owner());
hashCode = 31 * hashCode + Objects.hashCode(storageClassAsString());
hashCode = 31 * hashCode + Objects.hashCode(requestChargedAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ListPartsResponse)) {
return false;
}
ListPartsResponse other = (ListPartsResponse) obj;
return Objects.equals(abortDate(), other.abortDate()) && Objects.equals(abortRuleId(), other.abortRuleId())
&& Objects.equals(bucket(), other.bucket()) && Objects.equals(key(), other.key())
&& Objects.equals(uploadId(), other.uploadId()) && Objects.equals(partNumberMarker(), other.partNumberMarker())
&& Objects.equals(nextPartNumberMarker(), other.nextPartNumberMarker())
&& Objects.equals(maxParts(), other.maxParts()) && Objects.equals(isTruncated(), other.isTruncated())
&& hasParts() == other.hasParts() && Objects.equals(parts(), other.parts())
&& Objects.equals(initiator(), other.initiator()) && Objects.equals(owner(), other.owner())
&& Objects.equals(storageClassAsString(), other.storageClassAsString())
&& Objects.equals(requestChargedAsString(), other.requestChargedAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ListPartsResponse").add("AbortDate", abortDate()).add("AbortRuleId", abortRuleId())
.add("Bucket", bucket()).add("Key", key()).add("UploadId", uploadId())
.add("PartNumberMarker", partNumberMarker()).add("NextPartNumberMarker", nextPartNumberMarker())
.add("MaxParts", maxParts()).add("IsTruncated", isTruncated()).add("Parts", hasParts() ? parts() : null)
.add("Initiator", initiator()).add("Owner", owner()).add("StorageClass", storageClassAsString())
.add("RequestCharged", requestChargedAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "AbortDate":
return Optional.ofNullable(clazz.cast(abortDate()));
case "AbortRuleId":
return Optional.ofNullable(clazz.cast(abortRuleId()));
case "Bucket":
return Optional.ofNullable(clazz.cast(bucket()));
case "Key":
return Optional.ofNullable(clazz.cast(key()));
case "UploadId":
return Optional.ofNullable(clazz.cast(uploadId()));
case "PartNumberMarker":
return Optional.ofNullable(clazz.cast(partNumberMarker()));
case "NextPartNumberMarker":
return Optional.ofNullable(clazz.cast(nextPartNumberMarker()));
case "MaxParts":
return Optional.ofNullable(clazz.cast(maxParts()));
case "IsTruncated":
return Optional.ofNullable(clazz.cast(isTruncated()));
case "Parts":
return Optional.ofNullable(clazz.cast(parts()));
case "Initiator":
return Optional.ofNullable(clazz.cast(initiator()));
case "Owner":
return Optional.ofNullable(clazz.cast(owner()));
case "StorageClass":
return Optional.ofNullable(clazz.cast(storageClassAsString()));
case "RequestCharged":
return Optional.ofNullable(clazz.cast(requestChargedAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function