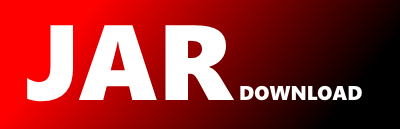
software.amazon.awssdk.services.s3.model.MultipartUpload Maven / Gradle / Ivy
Show all versions of s3 Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.s3.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Container for the MultipartUpload
for the Amazon S3 object.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class MultipartUpload implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField UPLOAD_ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("UploadId")
.getter(getter(MultipartUpload::uploadId))
.setter(setter(Builder::uploadId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("UploadId")
.unmarshallLocationName("UploadId").build()).build();
private static final SdkField KEY_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("Key")
.getter(getter(MultipartUpload::key))
.setter(setter(Builder::key))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Key").unmarshallLocationName("Key")
.build()).build();
private static final SdkField INITIATED_FIELD = SdkField
. builder(MarshallingType.INSTANT)
.memberName("Initiated")
.getter(getter(MultipartUpload::initiated))
.setter(setter(Builder::initiated))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Initiated")
.unmarshallLocationName("Initiated").build()).build();
private static final SdkField STORAGE_CLASS_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("StorageClass")
.getter(getter(MultipartUpload::storageClassAsString))
.setter(setter(Builder::storageClass))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StorageClass")
.unmarshallLocationName("StorageClass").build()).build();
private static final SdkField OWNER_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("Owner")
.getter(getter(MultipartUpload::owner))
.setter(setter(Builder::owner))
.constructor(Owner::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Owner")
.unmarshallLocationName("Owner").build()).build();
private static final SdkField INITIATOR_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("Initiator")
.getter(getter(MultipartUpload::initiator))
.setter(setter(Builder::initiator))
.constructor(Initiator::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Initiator")
.unmarshallLocationName("Initiator").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(UPLOAD_ID_FIELD, KEY_FIELD,
INITIATED_FIELD, STORAGE_CLASS_FIELD, OWNER_FIELD, INITIATOR_FIELD));
private static final long serialVersionUID = 1L;
private final String uploadId;
private final String key;
private final Instant initiated;
private final String storageClass;
private final Owner owner;
private final Initiator initiator;
private MultipartUpload(BuilderImpl builder) {
this.uploadId = builder.uploadId;
this.key = builder.key;
this.initiated = builder.initiated;
this.storageClass = builder.storageClass;
this.owner = builder.owner;
this.initiator = builder.initiator;
}
/**
*
* Upload ID that identifies the multipart upload.
*
*
* @return Upload ID that identifies the multipart upload.
*/
public final String uploadId() {
return uploadId;
}
/**
*
* Key of the object for which the multipart upload was initiated.
*
*
* @return Key of the object for which the multipart upload was initiated.
*/
public final String key() {
return key;
}
/**
*
* Date and time at which the multipart upload was initiated.
*
*
* @return Date and time at which the multipart upload was initiated.
*/
public final Instant initiated() {
return initiated;
}
/**
*
* The class of storage used to store the object.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #storageClass} will
* return {@link StorageClass#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #storageClassAsString}.
*
*
* @return The class of storage used to store the object.
* @see StorageClass
*/
public final StorageClass storageClass() {
return StorageClass.fromValue(storageClass);
}
/**
*
* The class of storage used to store the object.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #storageClass} will
* return {@link StorageClass#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #storageClassAsString}.
*
*
* @return The class of storage used to store the object.
* @see StorageClass
*/
public final String storageClassAsString() {
return storageClass;
}
/**
*
* Specifies the owner of the object that is part of the multipart upload.
*
*
* @return Specifies the owner of the object that is part of the multipart upload.
*/
public final Owner owner() {
return owner;
}
/**
*
* Identifies who initiated the multipart upload.
*
*
* @return Identifies who initiated the multipart upload.
*/
public final Initiator initiator() {
return initiator;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(uploadId());
hashCode = 31 * hashCode + Objects.hashCode(key());
hashCode = 31 * hashCode + Objects.hashCode(initiated());
hashCode = 31 * hashCode + Objects.hashCode(storageClassAsString());
hashCode = 31 * hashCode + Objects.hashCode(owner());
hashCode = 31 * hashCode + Objects.hashCode(initiator());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof MultipartUpload)) {
return false;
}
MultipartUpload other = (MultipartUpload) obj;
return Objects.equals(uploadId(), other.uploadId()) && Objects.equals(key(), other.key())
&& Objects.equals(initiated(), other.initiated())
&& Objects.equals(storageClassAsString(), other.storageClassAsString()) && Objects.equals(owner(), other.owner())
&& Objects.equals(initiator(), other.initiator());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("MultipartUpload").add("UploadId", uploadId()).add("Key", key()).add("Initiated", initiated())
.add("StorageClass", storageClassAsString()).add("Owner", owner()).add("Initiator", initiator()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "UploadId":
return Optional.ofNullable(clazz.cast(uploadId()));
case "Key":
return Optional.ofNullable(clazz.cast(key()));
case "Initiated":
return Optional.ofNullable(clazz.cast(initiated()));
case "StorageClass":
return Optional.ofNullable(clazz.cast(storageClassAsString()));
case "Owner":
return Optional.ofNullable(clazz.cast(owner()));
case "Initiator":
return Optional.ofNullable(clazz.cast(initiator()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function