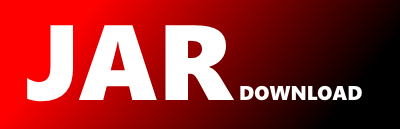
software.amazon.awssdk.services.s3.model.PutObjectResponse Maven / Gradle / Ivy
Show all versions of s3 Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.s3.model;
import java.beans.Transient;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class PutObjectResponse extends S3Response implements
ToCopyableBuilder {
private static final SdkField EXPIRATION_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("Expiration")
.getter(getter(PutObjectResponse::expiration))
.setter(setter(Builder::expiration))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("x-amz-expiration")
.unmarshallLocationName("x-amz-expiration").build()).build();
private static final SdkField E_TAG_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("ETag")
.getter(getter(PutObjectResponse::eTag))
.setter(setter(Builder::eTag))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("ETag").unmarshallLocationName("ETag")
.build()).build();
private static final SdkField SERVER_SIDE_ENCRYPTION_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("ServerSideEncryption")
.getter(getter(PutObjectResponse::serverSideEncryptionAsString))
.setter(setter(Builder::serverSideEncryption))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("x-amz-server-side-encryption")
.unmarshallLocationName("x-amz-server-side-encryption").build()).build();
private static final SdkField VERSION_ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("VersionId")
.getter(getter(PutObjectResponse::versionId))
.setter(setter(Builder::versionId))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("x-amz-version-id")
.unmarshallLocationName("x-amz-version-id").build()).build();
private static final SdkField SSE_CUSTOMER_ALGORITHM_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("SSECustomerAlgorithm")
.getter(getter(PutObjectResponse::sseCustomerAlgorithm))
.setter(setter(Builder::sseCustomerAlgorithm))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER)
.locationName("x-amz-server-side-encryption-customer-algorithm")
.unmarshallLocationName("x-amz-server-side-encryption-customer-algorithm").build()).build();
private static final SdkField SSE_CUSTOMER_KEY_MD5_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("SSECustomerKeyMD5")
.getter(getter(PutObjectResponse::sseCustomerKeyMD5))
.setter(setter(Builder::sseCustomerKeyMD5))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER)
.locationName("x-amz-server-side-encryption-customer-key-MD5")
.unmarshallLocationName("x-amz-server-side-encryption-customer-key-MD5").build()).build();
private static final SdkField SSEKMS_KEY_ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("SSEKMSKeyId")
.getter(getter(PutObjectResponse::ssekmsKeyId))
.setter(setter(Builder::ssekmsKeyId))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER)
.locationName("x-amz-server-side-encryption-aws-kms-key-id")
.unmarshallLocationName("x-amz-server-side-encryption-aws-kms-key-id").build()).build();
private static final SdkField SSEKMS_ENCRYPTION_CONTEXT_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("SSEKMSEncryptionContext")
.getter(getter(PutObjectResponse::ssekmsEncryptionContext))
.setter(setter(Builder::ssekmsEncryptionContext))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER)
.locationName("x-amz-server-side-encryption-context")
.unmarshallLocationName("x-amz-server-side-encryption-context").build()).build();
private static final SdkField BUCKET_KEY_ENABLED_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("BucketKeyEnabled")
.getter(getter(PutObjectResponse::bucketKeyEnabled))
.setter(setter(Builder::bucketKeyEnabled))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER)
.locationName("x-amz-server-side-encryption-bucket-key-enabled")
.unmarshallLocationName("x-amz-server-side-encryption-bucket-key-enabled").build()).build();
private static final SdkField REQUEST_CHARGED_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("RequestCharged")
.getter(getter(PutObjectResponse::requestChargedAsString))
.setter(setter(Builder::requestCharged))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("x-amz-request-charged")
.unmarshallLocationName("x-amz-request-charged").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(EXPIRATION_FIELD, E_TAG_FIELD,
SERVER_SIDE_ENCRYPTION_FIELD, VERSION_ID_FIELD, SSE_CUSTOMER_ALGORITHM_FIELD, SSE_CUSTOMER_KEY_MD5_FIELD,
SSEKMS_KEY_ID_FIELD, SSEKMS_ENCRYPTION_CONTEXT_FIELD, BUCKET_KEY_ENABLED_FIELD, REQUEST_CHARGED_FIELD));
private final String expiration;
private final String eTag;
private final String serverSideEncryption;
private final String versionId;
private final String sseCustomerAlgorithm;
private final String sseCustomerKeyMD5;
private final String ssekmsKeyId;
private final String ssekmsEncryptionContext;
private final Boolean bucketKeyEnabled;
private final String requestCharged;
private PutObjectResponse(BuilderImpl builder) {
super(builder);
this.expiration = builder.expiration;
this.eTag = builder.eTag;
this.serverSideEncryption = builder.serverSideEncryption;
this.versionId = builder.versionId;
this.sseCustomerAlgorithm = builder.sseCustomerAlgorithm;
this.sseCustomerKeyMD5 = builder.sseCustomerKeyMD5;
this.ssekmsKeyId = builder.ssekmsKeyId;
this.ssekmsEncryptionContext = builder.ssekmsEncryptionContext;
this.bucketKeyEnabled = builder.bucketKeyEnabled;
this.requestCharged = builder.requestCharged;
}
/**
*
* If the expiration is configured for the object (see PutBucketLifecycleConfiguration), the response includes this header. It includes the expiry-date and rule-id
* key-value pairs that provide information about object expiration. The value of the rule-id is URL encoded.
*
*
* @return If the expiration is configured for the object (see PutBucketLifecycleConfiguration), the response includes this header. It includes the expiry-date and
* rule-id key-value pairs that provide information about object expiration. The value of the rule-id is URL
* encoded.
*/
public final String expiration() {
return expiration;
}
/**
*
* Entity tag for the uploaded object.
*
*
* @return Entity tag for the uploaded object.
*/
public final String eTag() {
return eTag;
}
/**
*
* If you specified server-side encryption either with an Amazon Web Services KMS key or Amazon S3-managed
* encryption key in your PUT request, the response includes this header. It confirms the encryption algorithm that
* Amazon S3 used to encrypt the object.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #serverSideEncryption} will return {@link ServerSideEncryption#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #serverSideEncryptionAsString}.
*
*
* @return If you specified server-side encryption either with an Amazon Web Services KMS key or Amazon S3-managed
* encryption key in your PUT request, the response includes this header. It confirms the encryption
* algorithm that Amazon S3 used to encrypt the object.
* @see ServerSideEncryption
*/
public final ServerSideEncryption serverSideEncryption() {
return ServerSideEncryption.fromValue(serverSideEncryption);
}
/**
*
* If you specified server-side encryption either with an Amazon Web Services KMS key or Amazon S3-managed
* encryption key in your PUT request, the response includes this header. It confirms the encryption algorithm that
* Amazon S3 used to encrypt the object.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #serverSideEncryption} will return {@link ServerSideEncryption#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #serverSideEncryptionAsString}.
*
*
* @return If you specified server-side encryption either with an Amazon Web Services KMS key or Amazon S3-managed
* encryption key in your PUT request, the response includes this header. It confirms the encryption
* algorithm that Amazon S3 used to encrypt the object.
* @see ServerSideEncryption
*/
public final String serverSideEncryptionAsString() {
return serverSideEncryption;
}
/**
*
* Version of the object.
*
*
* @return Version of the object.
*/
public final String versionId() {
return versionId;
}
/**
*
* If server-side encryption with a customer-provided encryption key was requested, the response will include this
* header confirming the encryption algorithm used.
*
*
* @return If server-side encryption with a customer-provided encryption key was requested, the response will
* include this header confirming the encryption algorithm used.
*/
public final String sseCustomerAlgorithm() {
return sseCustomerAlgorithm;
}
/**
*
* If server-side encryption with a customer-provided encryption key was requested, the response will include this
* header to provide round-trip message integrity verification of the customer-provided encryption key.
*
*
* @return If server-side encryption with a customer-provided encryption key was requested, the response will
* include this header to provide round-trip message integrity verification of the customer-provided
* encryption key.
*/
public final String sseCustomerKeyMD5() {
return sseCustomerKeyMD5;
}
/**
*
* If x-amz-server-side-encryption
is present and has the value of aws:kms
, this header
* specifies the ID of the Amazon Web Services Key Management Service (Amazon Web Services KMS) symmetric customer
* managed key that was used for the object.
*
*
* @return If x-amz-server-side-encryption
is present and has the value of aws:kms
, this
* header specifies the ID of the Amazon Web Services Key Management Service (Amazon Web Services KMS)
* symmetric customer managed key that was used for the object.
*/
public final String ssekmsKeyId() {
return ssekmsKeyId;
}
/**
*
* If present, specifies the Amazon Web Services KMS Encryption Context to use for object encryption. The value of
* this header is a base64-encoded UTF-8 string holding JSON with the encryption context key-value pairs.
*
*
* @return If present, specifies the Amazon Web Services KMS Encryption Context to use for object encryption. The
* value of this header is a base64-encoded UTF-8 string holding JSON with the encryption context key-value
* pairs.
*/
public final String ssekmsEncryptionContext() {
return ssekmsEncryptionContext;
}
/**
*
* Indicates whether the uploaded object uses an S3 Bucket Key for server-side encryption with Amazon Web Services
* KMS (SSE-KMS).
*
*
* @return Indicates whether the uploaded object uses an S3 Bucket Key for server-side encryption with Amazon Web
* Services KMS (SSE-KMS).
*/
public final Boolean bucketKeyEnabled() {
return bucketKeyEnabled;
}
/**
* Returns the value of the RequestCharged property for this object.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #requestCharged}
* will return {@link RequestCharged#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #requestChargedAsString}.
*
*
* @return The value of the RequestCharged property for this object.
* @see RequestCharged
*/
public final RequestCharged requestCharged() {
return RequestCharged.fromValue(requestCharged);
}
/**
* Returns the value of the RequestCharged property for this object.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #requestCharged}
* will return {@link RequestCharged#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #requestChargedAsString}.
*
*
* @return The value of the RequestCharged property for this object.
* @see RequestCharged
*/
public final String requestChargedAsString() {
return requestCharged;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(expiration());
hashCode = 31 * hashCode + Objects.hashCode(eTag());
hashCode = 31 * hashCode + Objects.hashCode(serverSideEncryptionAsString());
hashCode = 31 * hashCode + Objects.hashCode(versionId());
hashCode = 31 * hashCode + Objects.hashCode(sseCustomerAlgorithm());
hashCode = 31 * hashCode + Objects.hashCode(sseCustomerKeyMD5());
hashCode = 31 * hashCode + Objects.hashCode(ssekmsKeyId());
hashCode = 31 * hashCode + Objects.hashCode(ssekmsEncryptionContext());
hashCode = 31 * hashCode + Objects.hashCode(bucketKeyEnabled());
hashCode = 31 * hashCode + Objects.hashCode(requestChargedAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof PutObjectResponse)) {
return false;
}
PutObjectResponse other = (PutObjectResponse) obj;
return Objects.equals(expiration(), other.expiration()) && Objects.equals(eTag(), other.eTag())
&& Objects.equals(serverSideEncryptionAsString(), other.serverSideEncryptionAsString())
&& Objects.equals(versionId(), other.versionId())
&& Objects.equals(sseCustomerAlgorithm(), other.sseCustomerAlgorithm())
&& Objects.equals(sseCustomerKeyMD5(), other.sseCustomerKeyMD5())
&& Objects.equals(ssekmsKeyId(), other.ssekmsKeyId())
&& Objects.equals(ssekmsEncryptionContext(), other.ssekmsEncryptionContext())
&& Objects.equals(bucketKeyEnabled(), other.bucketKeyEnabled())
&& Objects.equals(requestChargedAsString(), other.requestChargedAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("PutObjectResponse").add("Expiration", expiration()).add("ETag", eTag())
.add("ServerSideEncryption", serverSideEncryptionAsString()).add("VersionId", versionId())
.add("SSECustomerAlgorithm", sseCustomerAlgorithm()).add("SSECustomerKeyMD5", sseCustomerKeyMD5())
.add("SSEKMSKeyId", ssekmsKeyId() == null ? null : "*** Sensitive Data Redacted ***")
.add("SSEKMSEncryptionContext", ssekmsEncryptionContext() == null ? null : "*** Sensitive Data Redacted ***")
.add("BucketKeyEnabled", bucketKeyEnabled()).add("RequestCharged", requestChargedAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Expiration":
return Optional.ofNullable(clazz.cast(expiration()));
case "ETag":
return Optional.ofNullable(clazz.cast(eTag()));
case "ServerSideEncryption":
return Optional.ofNullable(clazz.cast(serverSideEncryptionAsString()));
case "VersionId":
return Optional.ofNullable(clazz.cast(versionId()));
case "SSECustomerAlgorithm":
return Optional.ofNullable(clazz.cast(sseCustomerAlgorithm()));
case "SSECustomerKeyMD5":
return Optional.ofNullable(clazz.cast(sseCustomerKeyMD5()));
case "SSEKMSKeyId":
return Optional.ofNullable(clazz.cast(ssekmsKeyId()));
case "SSEKMSEncryptionContext":
return Optional.ofNullable(clazz.cast(ssekmsEncryptionContext()));
case "BucketKeyEnabled":
return Optional.ofNullable(clazz.cast(bucketKeyEnabled()));
case "RequestCharged":
return Optional.ofNullable(clazz.cast(requestChargedAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function