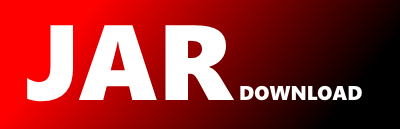
software.amazon.awssdk.services.s3.model.InventoryConfiguration Maven / Gradle / Ivy
Show all versions of s3 Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.s3.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.traits.RequiredTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Specifies the inventory configuration for an Amazon S3 bucket. For more information, see GET Bucket inventory in
* the Amazon S3 API Reference.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class InventoryConfiguration implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField DESTINATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("Destination")
.getter(getter(InventoryConfiguration::destination))
.setter(setter(Builder::destination))
.constructor(InventoryDestination::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Destination")
.unmarshallLocationName("Destination").build(), RequiredTrait.create()).build();
private static final SdkField IS_ENABLED_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("IsEnabled")
.getter(getter(InventoryConfiguration::isEnabled))
.setter(setter(Builder::isEnabled))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IsEnabled")
.unmarshallLocationName("IsEnabled").build(), RequiredTrait.create()).build();
private static final SdkField FILTER_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("Filter")
.getter(getter(InventoryConfiguration::filter))
.setter(setter(Builder::filter))
.constructor(InventoryFilter::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Filter")
.unmarshallLocationName("Filter").build()).build();
private static final SdkField ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("Id")
.getter(getter(InventoryConfiguration::id))
.setter(setter(Builder::id))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Id").unmarshallLocationName("Id")
.build(), RequiredTrait.create()).build();
private static final SdkField INCLUDED_OBJECT_VERSIONS_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("IncludedObjectVersions")
.getter(getter(InventoryConfiguration::includedObjectVersionsAsString))
.setter(setter(Builder::includedObjectVersions))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IncludedObjectVersions")
.unmarshallLocationName("IncludedObjectVersions").build(), RequiredTrait.create()).build();
private static final SdkField> OPTIONAL_FIELDS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("OptionalFields")
.getter(getter(InventoryConfiguration::optionalFieldsAsStrings))
.setter(setter(Builder::optionalFieldsWithStrings))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OptionalFields")
.unmarshallLocationName("OptionalFields").build(),
ListTrait
.builder()
.memberLocationName("Field")
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("Field").unmarshallLocationName("Field").build()).build())
.build()).build();
private static final SdkField SCHEDULE_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("Schedule")
.getter(getter(InventoryConfiguration::schedule))
.setter(setter(Builder::schedule))
.constructor(InventorySchedule::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Schedule")
.unmarshallLocationName("Schedule").build(), RequiredTrait.create()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(DESTINATION_FIELD,
IS_ENABLED_FIELD, FILTER_FIELD, ID_FIELD, INCLUDED_OBJECT_VERSIONS_FIELD, OPTIONAL_FIELDS_FIELD, SCHEDULE_FIELD));
private static final long serialVersionUID = 1L;
private final InventoryDestination destination;
private final Boolean isEnabled;
private final InventoryFilter filter;
private final String id;
private final String includedObjectVersions;
private final List optionalFields;
private final InventorySchedule schedule;
private InventoryConfiguration(BuilderImpl builder) {
this.destination = builder.destination;
this.isEnabled = builder.isEnabled;
this.filter = builder.filter;
this.id = builder.id;
this.includedObjectVersions = builder.includedObjectVersions;
this.optionalFields = builder.optionalFields;
this.schedule = builder.schedule;
}
/**
*
* Contains information about where to publish the inventory results.
*
*
* @return Contains information about where to publish the inventory results.
*/
public final InventoryDestination destination() {
return destination;
}
/**
*
* Specifies whether the inventory is enabled or disabled. If set to True
, an inventory list is
* generated. If set to False
, no inventory list is generated.
*
*
* @return Specifies whether the inventory is enabled or disabled. If set to True
, an inventory list is
* generated. If set to False
, no inventory list is generated.
*/
public final Boolean isEnabled() {
return isEnabled;
}
/**
*
* Specifies an inventory filter. The inventory only includes objects that meet the filter's criteria.
*
*
* @return Specifies an inventory filter. The inventory only includes objects that meet the filter's criteria.
*/
public final InventoryFilter filter() {
return filter;
}
/**
*
* The ID used to identify the inventory configuration.
*
*
* @return The ID used to identify the inventory configuration.
*/
public final String id() {
return id;
}
/**
*
* Object versions to include in the inventory list. If set to All
, the list includes all the object
* versions, which adds the version-related fields VersionId
, IsLatest
, and
* DeleteMarker
to the list. If set to Current
, the list does not contain these
* version-related fields.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #includedObjectVersions} will return {@link InventoryIncludedObjectVersions#UNKNOWN_TO_SDK_VERSION}. The
* raw value returned by the service is available from {@link #includedObjectVersionsAsString}.
*
*
* @return Object versions to include in the inventory list. If set to All
, the list includes all the
* object versions, which adds the version-related fields VersionId
, IsLatest
, and
* DeleteMarker
to the list. If set to Current
, the list does not contain these
* version-related fields.
* @see InventoryIncludedObjectVersions
*/
public final InventoryIncludedObjectVersions includedObjectVersions() {
return InventoryIncludedObjectVersions.fromValue(includedObjectVersions);
}
/**
*
* Object versions to include in the inventory list. If set to All
, the list includes all the object
* versions, which adds the version-related fields VersionId
, IsLatest
, and
* DeleteMarker
to the list. If set to Current
, the list does not contain these
* version-related fields.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #includedObjectVersions} will return {@link InventoryIncludedObjectVersions#UNKNOWN_TO_SDK_VERSION}. The
* raw value returned by the service is available from {@link #includedObjectVersionsAsString}.
*
*
* @return Object versions to include in the inventory list. If set to All
, the list includes all the
* object versions, which adds the version-related fields VersionId
, IsLatest
, and
* DeleteMarker
to the list. If set to Current
, the list does not contain these
* version-related fields.
* @see InventoryIncludedObjectVersions
*/
public final String includedObjectVersionsAsString() {
return includedObjectVersions;
}
/**
*
* Contains the optional fields that are included in the inventory results.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasOptionalFields} method.
*
*
* @return Contains the optional fields that are included in the inventory results.
*/
public final List optionalFields() {
return InventoryOptionalFieldsCopier.copyStringToEnum(optionalFields);
}
/**
* For responses, this returns true if the service returned a value for the OptionalFields property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasOptionalFields() {
return optionalFields != null && !(optionalFields instanceof SdkAutoConstructList);
}
/**
*
* Contains the optional fields that are included in the inventory results.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasOptionalFields} method.
*
*
* @return Contains the optional fields that are included in the inventory results.
*/
public final List optionalFieldsAsStrings() {
return optionalFields;
}
/**
*
* Specifies the schedule for generating inventory results.
*
*
* @return Specifies the schedule for generating inventory results.
*/
public final InventorySchedule schedule() {
return schedule;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(destination());
hashCode = 31 * hashCode + Objects.hashCode(isEnabled());
hashCode = 31 * hashCode + Objects.hashCode(filter());
hashCode = 31 * hashCode + Objects.hashCode(id());
hashCode = 31 * hashCode + Objects.hashCode(includedObjectVersionsAsString());
hashCode = 31 * hashCode + Objects.hashCode(hasOptionalFields() ? optionalFieldsAsStrings() : null);
hashCode = 31 * hashCode + Objects.hashCode(schedule());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof InventoryConfiguration)) {
return false;
}
InventoryConfiguration other = (InventoryConfiguration) obj;
return Objects.equals(destination(), other.destination()) && Objects.equals(isEnabled(), other.isEnabled())
&& Objects.equals(filter(), other.filter()) && Objects.equals(id(), other.id())
&& Objects.equals(includedObjectVersionsAsString(), other.includedObjectVersionsAsString())
&& hasOptionalFields() == other.hasOptionalFields()
&& Objects.equals(optionalFieldsAsStrings(), other.optionalFieldsAsStrings())
&& Objects.equals(schedule(), other.schedule());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("InventoryConfiguration").add("Destination", destination()).add("IsEnabled", isEnabled())
.add("Filter", filter()).add("Id", id()).add("IncludedObjectVersions", includedObjectVersionsAsString())
.add("OptionalFields", hasOptionalFields() ? optionalFieldsAsStrings() : null).add("Schedule", schedule())
.build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Destination":
return Optional.ofNullable(clazz.cast(destination()));
case "IsEnabled":
return Optional.ofNullable(clazz.cast(isEnabled()));
case "Filter":
return Optional.ofNullable(clazz.cast(filter()));
case "Id":
return Optional.ofNullable(clazz.cast(id()));
case "IncludedObjectVersions":
return Optional.ofNullable(clazz.cast(includedObjectVersionsAsString()));
case "OptionalFields":
return Optional.ofNullable(clazz.cast(optionalFieldsAsStrings()));
case "Schedule":
return Optional.ofNullable(clazz.cast(schedule()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function