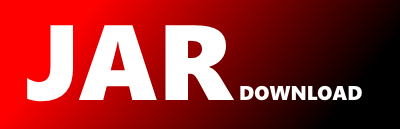
software.amazon.awssdk.services.s3.model.ListMultipartUploadsRequest Maven / Gradle / Ivy
Show all versions of s3 Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.s3.model;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.traits.RequiredTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class ListMultipartUploadsRequest extends S3Request implements
ToCopyableBuilder {
private static final SdkField BUCKET_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("Bucket")
.getter(getter(ListMultipartUploadsRequest::bucket))
.setter(setter(Builder::bucket))
.traits(LocationTrait.builder().location(MarshallLocation.PATH).locationName("Bucket")
.unmarshallLocationName("Bucket").build(), RequiredTrait.create()).build();
private static final SdkField DELIMITER_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("Delimiter")
.getter(getter(ListMultipartUploadsRequest::delimiter))
.setter(setter(Builder::delimiter))
.traits(LocationTrait.builder().location(MarshallLocation.QUERY_PARAM).locationName("delimiter")
.unmarshallLocationName("delimiter").build()).build();
private static final SdkField ENCODING_TYPE_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("EncodingType")
.getter(getter(ListMultipartUploadsRequest::encodingTypeAsString))
.setter(setter(Builder::encodingType))
.traits(LocationTrait.builder().location(MarshallLocation.QUERY_PARAM).locationName("encoding-type")
.unmarshallLocationName("encoding-type").build()).build();
private static final SdkField KEY_MARKER_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("KeyMarker")
.getter(getter(ListMultipartUploadsRequest::keyMarker))
.setter(setter(Builder::keyMarker))
.traits(LocationTrait.builder().location(MarshallLocation.QUERY_PARAM).locationName("key-marker")
.unmarshallLocationName("key-marker").build()).build();
private static final SdkField MAX_UPLOADS_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("MaxUploads")
.getter(getter(ListMultipartUploadsRequest::maxUploads))
.setter(setter(Builder::maxUploads))
.traits(LocationTrait.builder().location(MarshallLocation.QUERY_PARAM).locationName("max-uploads")
.unmarshallLocationName("max-uploads").build()).build();
private static final SdkField PREFIX_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("Prefix")
.getter(getter(ListMultipartUploadsRequest::prefix))
.setter(setter(Builder::prefix))
.traits(LocationTrait.builder().location(MarshallLocation.QUERY_PARAM).locationName("prefix")
.unmarshallLocationName("prefix").build()).build();
private static final SdkField UPLOAD_ID_MARKER_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("UploadIdMarker")
.getter(getter(ListMultipartUploadsRequest::uploadIdMarker))
.setter(setter(Builder::uploadIdMarker))
.traits(LocationTrait.builder().location(MarshallLocation.QUERY_PARAM).locationName("upload-id-marker")
.unmarshallLocationName("upload-id-marker").build()).build();
private static final SdkField EXPECTED_BUCKET_OWNER_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("ExpectedBucketOwner")
.getter(getter(ListMultipartUploadsRequest::expectedBucketOwner))
.setter(setter(Builder::expectedBucketOwner))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("x-amz-expected-bucket-owner")
.unmarshallLocationName("x-amz-expected-bucket-owner").build()).build();
private static final SdkField REQUEST_PAYER_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("RequestPayer")
.getter(getter(ListMultipartUploadsRequest::requestPayerAsString))
.setter(setter(Builder::requestPayer))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("x-amz-request-payer")
.unmarshallLocationName("x-amz-request-payer").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(BUCKET_FIELD, DELIMITER_FIELD,
ENCODING_TYPE_FIELD, KEY_MARKER_FIELD, MAX_UPLOADS_FIELD, PREFIX_FIELD, UPLOAD_ID_MARKER_FIELD,
EXPECTED_BUCKET_OWNER_FIELD, REQUEST_PAYER_FIELD));
private final String bucket;
private final String delimiter;
private final String encodingType;
private final String keyMarker;
private final Integer maxUploads;
private final String prefix;
private final String uploadIdMarker;
private final String expectedBucketOwner;
private final String requestPayer;
private ListMultipartUploadsRequest(BuilderImpl builder) {
super(builder);
this.bucket = builder.bucket;
this.delimiter = builder.delimiter;
this.encodingType = builder.encodingType;
this.keyMarker = builder.keyMarker;
this.maxUploads = builder.maxUploads;
this.prefix = builder.prefix;
this.uploadIdMarker = builder.uploadIdMarker;
this.expectedBucketOwner = builder.expectedBucketOwner;
this.requestPayer = builder.requestPayer;
}
/**
*
* The name of the bucket to which the multipart upload was initiated.
*
*
* Directory buckets - When you use this operation with a directory bucket, you must use virtual-hosted-style
* requests in the format Bucket_name.s3express-az_id.region.amazonaws.com
.
* Path-style requests are not supported. Directory bucket names must be unique in the chosen Availability Zone.
* Bucket names must follow the format bucket_base_name--az-id--x-s3
(for example,
* DOC-EXAMPLE-BUCKET--usw2-az2--x-s3
). For information about bucket naming
* restrictions, see Directory bucket
* naming rules in the Amazon S3 User Guide.
*
*
* Access points - When you use this action with an access point, you must provide the alias of the access
* point in place of the bucket name or specify the access point ARN. When using the access point ARN, you must
* direct requests to the access point hostname. The access point hostname takes the form
* AccessPointName-AccountId.s3-accesspoint.Region.amazonaws.com. When using this action with
* an access point through the Amazon Web Services SDKs, you provide the access point ARN in place of the bucket
* name. For more information about access point ARNs, see Using access points in
* the Amazon S3 User Guide.
*
*
*
* Access points and Object Lambda access points are not supported by directory buckets.
*
*
*
* S3 on Outposts - When you use this action with Amazon S3 on Outposts, you must direct requests to the S3
* on Outposts hostname. The S3 on Outposts hostname takes the form
* AccessPointName-AccountId.outpostID.s3-outposts.Region.amazonaws.com
.
* When you use this action with S3 on Outposts through the Amazon Web Services SDKs, you provide the Outposts
* access point ARN in place of the bucket name. For more information about S3 on Outposts ARNs, see What is S3 on Outposts? in the
* Amazon S3 User Guide.
*
*
* @return The name of the bucket to which the multipart upload was initiated.
*
* Directory buckets - When you use this operation with a directory bucket, you must use
* virtual-hosted-style requests in the format
* Bucket_name.s3express-az_id.region.amazonaws.com
. Path-style requests
* are not supported. Directory bucket names must be unique in the chosen Availability Zone. Bucket names
* must follow the format bucket_base_name--az-id--x-s3
(for example,
* DOC-EXAMPLE-BUCKET--usw2-az2--x-s3
). For information about bucket naming
* restrictions, see Directory
* bucket naming rules in the Amazon S3 User Guide.
*
*
* Access points - When you use this action with an access point, you must provide the alias of the
* access point in place of the bucket name or specify the access point ARN. When using the access point
* ARN, you must direct requests to the access point hostname. The access point hostname takes the form
* AccessPointName-AccountId.s3-accesspoint.Region.amazonaws.com. When using this
* action with an access point through the Amazon Web Services SDKs, you provide the access point ARN in
* place of the bucket name. For more information about access point ARNs, see Using access
* points in the Amazon S3 User Guide.
*
*
*
* Access points and Object Lambda access points are not supported by directory buckets.
*
*
*
* S3 on Outposts - When you use this action with Amazon S3 on Outposts, you must direct requests to
* the S3 on Outposts hostname. The S3 on Outposts hostname takes the form
* AccessPointName-AccountId.outpostID.s3-outposts.Region.amazonaws.com
* . When you use this action with S3 on Outposts through the Amazon Web Services SDKs, you provide the
* Outposts access point ARN in place of the bucket name. For more information about S3 on Outposts ARNs,
* see What is S3 on
* Outposts? in the Amazon S3 User Guide.
*/
public final String bucket() {
return bucket;
}
/**
*
* Character you use to group keys.
*
*
* All keys that contain the same string between the prefix, if specified, and the first occurrence of the delimiter
* after the prefix are grouped under a single result element, CommonPrefixes
. If you don't specify the
* prefix parameter, then the substring starts at the beginning of the key. The keys that are grouped under
* CommonPrefixes
result element are not returned elsewhere in the response.
*
*
*
* Directory buckets - For directory buckets, /
is the only supported delimiter.
*
*
*
* @return Character you use to group keys.
*
* All keys that contain the same string between the prefix, if specified, and the first occurrence of the
* delimiter after the prefix are grouped under a single result element, CommonPrefixes
. If you
* don't specify the prefix parameter, then the substring starts at the beginning of the key. The keys that
* are grouped under CommonPrefixes
result element are not returned elsewhere in the response.
*
*
*
* Directory buckets - For directory buckets, /
is the only supported delimiter.
*
*/
public final String delimiter() {
return delimiter;
}
/**
* Returns the value of the EncodingType property for this object.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #encodingType} will
* return {@link EncodingType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #encodingTypeAsString}.
*
*
* @return The value of the EncodingType property for this object.
* @see EncodingType
*/
public final EncodingType encodingType() {
return EncodingType.fromValue(encodingType);
}
/**
* Returns the value of the EncodingType property for this object.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #encodingType} will
* return {@link EncodingType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #encodingTypeAsString}.
*
*
* @return The value of the EncodingType property for this object.
* @see EncodingType
*/
public final String encodingTypeAsString() {
return encodingType;
}
/**
*
* Specifies the multipart upload after which listing should begin.
*
*
*
* -
*
* General purpose buckets - For general purpose buckets, key-marker
is an object key. Together
* with upload-id-marker
, this parameter specifies the multipart upload after which listing should
* begin.
*
*
* If upload-id-marker
is not specified, only the keys lexicographically greater than the specified
* key-marker
will be included in the list.
*
*
* If upload-id-marker
is specified, any multipart uploads for a key equal to the
* key-marker
might also be included, provided those multipart uploads have upload IDs
* lexicographically greater than the specified upload-id-marker
.
*
*
* -
*
* Directory buckets - For directory buckets, key-marker
is obfuscated and isn't a real object
* key. The upload-id-marker
parameter isn't supported by directory buckets. To list the additional
* multipart uploads, you only need to set the value of key-marker
to the NextKeyMarker
* value from the previous response.
*
*
* In the ListMultipartUploads
response, the multipart uploads aren't sorted lexicographically based on
* the object keys.
*
*
*
*
*
* @return Specifies the multipart upload after which listing should begin.
*
* -
*
* General purpose buckets - For general purpose buckets, key-marker
is an object key.
* Together with upload-id-marker
, this parameter specifies the multipart upload after which
* listing should begin.
*
*
* If upload-id-marker
is not specified, only the keys lexicographically greater than the
* specified key-marker
will be included in the list.
*
*
* If upload-id-marker
is specified, any multipart uploads for a key equal to the
* key-marker
might also be included, provided those multipart uploads have upload IDs
* lexicographically greater than the specified upload-id-marker
.
*
*
* -
*
* Directory buckets - For directory buckets, key-marker
is obfuscated and isn't a real
* object key. The upload-id-marker
parameter isn't supported by directory buckets. To list the
* additional multipart uploads, you only need to set the value of key-marker
to the
* NextKeyMarker
value from the previous response.
*
*
* In the ListMultipartUploads
response, the multipart uploads aren't sorted lexicographically
* based on the object keys.
*
*
*
*/
public final String keyMarker() {
return keyMarker;
}
/**
*
* Sets the maximum number of multipart uploads, from 1 to 1,000, to return in the response body. 1,000 is the
* maximum number of uploads that can be returned in a response.
*
*
* @return Sets the maximum number of multipart uploads, from 1 to 1,000, to return in the response body. 1,000 is
* the maximum number of uploads that can be returned in a response.
*/
public final Integer maxUploads() {
return maxUploads;
}
/**
*
* Lists in-progress uploads only for those keys that begin with the specified prefix. You can use prefixes to
* separate a bucket into different grouping of keys. (You can think of using prefix
to make groups in
* the same way that you'd use a folder in a file system.)
*
*
*
* Directory buckets - For directory buckets, only prefixes that end in a delimiter (/
) are
* supported.
*
*
*
* @return Lists in-progress uploads only for those keys that begin with the specified prefix. You can use prefixes
* to separate a bucket into different grouping of keys. (You can think of using prefix
to make
* groups in the same way that you'd use a folder in a file system.)
*
* Directory buckets - For directory buckets, only prefixes that end in a delimiter (/
)
* are supported.
*
*/
public final String prefix() {
return prefix;
}
/**
*
* Together with key-marker, specifies the multipart upload after which listing should begin. If key-marker is not
* specified, the upload-id-marker parameter is ignored. Otherwise, any multipart uploads for a key equal to the
* key-marker might be included in the list only if they have an upload ID lexicographically greater than the
* specified upload-id-marker
.
*
*
*
* This functionality is not supported for directory buckets.
*
*
*
* @return Together with key-marker, specifies the multipart upload after which listing should begin. If key-marker
* is not specified, the upload-id-marker parameter is ignored. Otherwise, any multipart uploads for a key
* equal to the key-marker might be included in the list only if they have an upload ID lexicographically
* greater than the specified upload-id-marker
.
*
* This functionality is not supported for directory buckets.
*
*/
public final String uploadIdMarker() {
return uploadIdMarker;
}
/**
*
* The account ID of the expected bucket owner. If the account ID that you provide does not match the actual owner
* of the bucket, the request fails with the HTTP status code 403 Forbidden
(access denied).
*
*
* @return The account ID of the expected bucket owner. If the account ID that you provide does not match the actual
* owner of the bucket, the request fails with the HTTP status code 403 Forbidden
(access
* denied).
*/
public final String expectedBucketOwner() {
return expectedBucketOwner;
}
/**
* Returns the value of the RequestPayer property for this object.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #requestPayer} will
* return {@link RequestPayer#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #requestPayerAsString}.
*
*
* @return The value of the RequestPayer property for this object.
* @see RequestPayer
*/
public final RequestPayer requestPayer() {
return RequestPayer.fromValue(requestPayer);
}
/**
* Returns the value of the RequestPayer property for this object.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #requestPayer} will
* return {@link RequestPayer#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #requestPayerAsString}.
*
*
* @return The value of the RequestPayer property for this object.
* @see RequestPayer
*/
public final String requestPayerAsString() {
return requestPayer;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(bucket());
hashCode = 31 * hashCode + Objects.hashCode(delimiter());
hashCode = 31 * hashCode + Objects.hashCode(encodingTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(keyMarker());
hashCode = 31 * hashCode + Objects.hashCode(maxUploads());
hashCode = 31 * hashCode + Objects.hashCode(prefix());
hashCode = 31 * hashCode + Objects.hashCode(uploadIdMarker());
hashCode = 31 * hashCode + Objects.hashCode(expectedBucketOwner());
hashCode = 31 * hashCode + Objects.hashCode(requestPayerAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ListMultipartUploadsRequest)) {
return false;
}
ListMultipartUploadsRequest other = (ListMultipartUploadsRequest) obj;
return Objects.equals(bucket(), other.bucket()) && Objects.equals(delimiter(), other.delimiter())
&& Objects.equals(encodingTypeAsString(), other.encodingTypeAsString())
&& Objects.equals(keyMarker(), other.keyMarker()) && Objects.equals(maxUploads(), other.maxUploads())
&& Objects.equals(prefix(), other.prefix()) && Objects.equals(uploadIdMarker(), other.uploadIdMarker())
&& Objects.equals(expectedBucketOwner(), other.expectedBucketOwner())
&& Objects.equals(requestPayerAsString(), other.requestPayerAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ListMultipartUploadsRequest").add("Bucket", bucket()).add("Delimiter", delimiter())
.add("EncodingType", encodingTypeAsString()).add("KeyMarker", keyMarker()).add("MaxUploads", maxUploads())
.add("Prefix", prefix()).add("UploadIdMarker", uploadIdMarker())
.add("ExpectedBucketOwner", expectedBucketOwner()).add("RequestPayer", requestPayerAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Bucket":
return Optional.ofNullable(clazz.cast(bucket()));
case "Delimiter":
return Optional.ofNullable(clazz.cast(delimiter()));
case "EncodingType":
return Optional.ofNullable(clazz.cast(encodingTypeAsString()));
case "KeyMarker":
return Optional.ofNullable(clazz.cast(keyMarker()));
case "MaxUploads":
return Optional.ofNullable(clazz.cast(maxUploads()));
case "Prefix":
return Optional.ofNullable(clazz.cast(prefix()));
case "UploadIdMarker":
return Optional.ofNullable(clazz.cast(uploadIdMarker()));
case "ExpectedBucketOwner":
return Optional.ofNullable(clazz.cast(expectedBucketOwner()));
case "RequestPayer":
return Optional.ofNullable(clazz.cast(requestPayerAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function