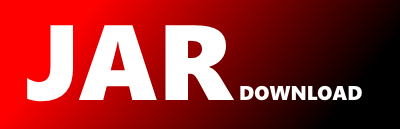
software.amazon.awssdk.services.s3.model.DeleteObjectsRequest Maven / Gradle / Ivy
Show all versions of s3 Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.s3.model;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.traits.PayloadTrait;
import software.amazon.awssdk.core.traits.RequiredTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class DeleteObjectsRequest extends S3Request implements
ToCopyableBuilder {
private static final SdkField BUCKET_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("Bucket")
.getter(getter(DeleteObjectsRequest::bucket))
.setter(setter(Builder::bucket))
.traits(LocationTrait.builder().location(MarshallLocation.PATH).locationName("Bucket")
.unmarshallLocationName("Bucket").build(), RequiredTrait.create()).build();
private static final SdkField DELETE_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("Delete")
.getter(getter(DeleteObjectsRequest::delete))
.setter(setter(Builder::delete))
.constructor(Delete::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Delete")
.unmarshallLocationName("Delete").build(), PayloadTrait.create(), RequiredTrait.create()).build();
private static final SdkField MFA_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("MFA")
.getter(getter(DeleteObjectsRequest::mfa))
.setter(setter(Builder::mfa))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("x-amz-mfa")
.unmarshallLocationName("x-amz-mfa").build()).build();
private static final SdkField REQUEST_PAYER_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("RequestPayer")
.getter(getter(DeleteObjectsRequest::requestPayerAsString))
.setter(setter(Builder::requestPayer))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("x-amz-request-payer")
.unmarshallLocationName("x-amz-request-payer").build()).build();
private static final SdkField BYPASS_GOVERNANCE_RETENTION_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("BypassGovernanceRetention")
.getter(getter(DeleteObjectsRequest::bypassGovernanceRetention))
.setter(setter(Builder::bypassGovernanceRetention))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("x-amz-bypass-governance-retention")
.unmarshallLocationName("x-amz-bypass-governance-retention").build()).build();
private static final SdkField EXPECTED_BUCKET_OWNER_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("ExpectedBucketOwner")
.getter(getter(DeleteObjectsRequest::expectedBucketOwner))
.setter(setter(Builder::expectedBucketOwner))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("x-amz-expected-bucket-owner")
.unmarshallLocationName("x-amz-expected-bucket-owner").build()).build();
private static final SdkField CHECKSUM_ALGORITHM_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("ChecksumAlgorithm")
.getter(getter(DeleteObjectsRequest::checksumAlgorithmAsString))
.setter(setter(Builder::checksumAlgorithm))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("x-amz-sdk-checksum-algorithm")
.unmarshallLocationName("x-amz-sdk-checksum-algorithm").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(BUCKET_FIELD, DELETE_FIELD,
MFA_FIELD, REQUEST_PAYER_FIELD, BYPASS_GOVERNANCE_RETENTION_FIELD, EXPECTED_BUCKET_OWNER_FIELD,
CHECKSUM_ALGORITHM_FIELD));
private final String bucket;
private final Delete delete;
private final String mfa;
private final String requestPayer;
private final Boolean bypassGovernanceRetention;
private final String expectedBucketOwner;
private final String checksumAlgorithm;
private DeleteObjectsRequest(BuilderImpl builder) {
super(builder);
this.bucket = builder.bucket;
this.delete = builder.delete;
this.mfa = builder.mfa;
this.requestPayer = builder.requestPayer;
this.bypassGovernanceRetention = builder.bypassGovernanceRetention;
this.expectedBucketOwner = builder.expectedBucketOwner;
this.checksumAlgorithm = builder.checksumAlgorithm;
}
/**
*
* The bucket name containing the objects to delete.
*
*
* Directory buckets - When you use this operation with a directory bucket, you must use virtual-hosted-style
* requests in the format Bucket_name.s3express-az_id.region.amazonaws.com
.
* Path-style requests are not supported. Directory bucket names must be unique in the chosen Availability Zone.
* Bucket names must follow the format bucket_base_name--az-id--x-s3
(for example,
* DOC-EXAMPLE-BUCKET--usw2-az1--x-s3
). For information about bucket naming
* restrictions, see Directory bucket
* naming rules in the Amazon S3 User Guide.
*
*
* Access points - When you use this action with an access point, you must provide the alias of the access
* point in place of the bucket name or specify the access point ARN. When using the access point ARN, you must
* direct requests to the access point hostname. The access point hostname takes the form
* AccessPointName-AccountId.s3-accesspoint.Region.amazonaws.com. When using this action with
* an access point through the Amazon Web Services SDKs, you provide the access point ARN in place of the bucket
* name. For more information about access point ARNs, see Using access points in
* the Amazon S3 User Guide.
*
*
*
* Access points and Object Lambda access points are not supported by directory buckets.
*
*
*
* S3 on Outposts - When you use this action with Amazon S3 on Outposts, you must direct requests to the S3
* on Outposts hostname. The S3 on Outposts hostname takes the form
* AccessPointName-AccountId.outpostID.s3-outposts.Region.amazonaws.com
.
* When you use this action with S3 on Outposts through the Amazon Web Services SDKs, you provide the Outposts
* access point ARN in place of the bucket name. For more information about S3 on Outposts ARNs, see What is S3 on Outposts? in the
* Amazon S3 User Guide.
*
*
* @return The bucket name containing the objects to delete.
*
* Directory buckets - When you use this operation with a directory bucket, you must use
* virtual-hosted-style requests in the format
* Bucket_name.s3express-az_id.region.amazonaws.com
. Path-style requests
* are not supported. Directory bucket names must be unique in the chosen Availability Zone. Bucket names
* must follow the format bucket_base_name--az-id--x-s3
(for example,
* DOC-EXAMPLE-BUCKET--usw2-az1--x-s3
). For information about bucket naming
* restrictions, see Directory
* bucket naming rules in the Amazon S3 User Guide.
*
*
* Access points - When you use this action with an access point, you must provide the alias of the
* access point in place of the bucket name or specify the access point ARN. When using the access point
* ARN, you must direct requests to the access point hostname. The access point hostname takes the form
* AccessPointName-AccountId.s3-accesspoint.Region.amazonaws.com. When using this
* action with an access point through the Amazon Web Services SDKs, you provide the access point ARN in
* place of the bucket name. For more information about access point ARNs, see Using access
* points in the Amazon S3 User Guide.
*
*
*
* Access points and Object Lambda access points are not supported by directory buckets.
*
*
*
* S3 on Outposts - When you use this action with Amazon S3 on Outposts, you must direct requests to
* the S3 on Outposts hostname. The S3 on Outposts hostname takes the form
* AccessPointName-AccountId.outpostID.s3-outposts.Region.amazonaws.com
* . When you use this action with S3 on Outposts through the Amazon Web Services SDKs, you provide the
* Outposts access point ARN in place of the bucket name. For more information about S3 on Outposts ARNs,
* see What is S3 on
* Outposts? in the Amazon S3 User Guide.
*/
public final String bucket() {
return bucket;
}
/**
*
* Container for the request.
*
*
* @return Container for the request.
*/
public final Delete delete() {
return delete;
}
/**
*
* The concatenation of the authentication device's serial number, a space, and the value that is displayed on your
* authentication device. Required to permanently delete a versioned object if versioning is configured with MFA
* delete enabled.
*
*
* When performing the DeleteObjects
operation on an MFA delete enabled bucket, which attempts to
* delete the specified versioned objects, you must include an MFA token. If you don't provide an MFA token, the
* entire request will fail, even if there are non-versioned objects that you are trying to delete. If you provide
* an invalid token, whether there are versioned object keys in the request or not, the entire Multi-Object Delete
* request will fail. For information about MFA Delete, see MFA
* Delete in the Amazon S3 User Guide.
*
*
*
* This functionality is not supported for directory buckets.
*
*
*
* @return The concatenation of the authentication device's serial number, a space, and the value that is displayed
* on your authentication device. Required to permanently delete a versioned object if versioning is
* configured with MFA delete enabled.
*
* When performing the DeleteObjects
operation on an MFA delete enabled bucket, which attempts
* to delete the specified versioned objects, you must include an MFA token. If you don't provide an MFA
* token, the entire request will fail, even if there are non-versioned objects that you are trying to
* delete. If you provide an invalid token, whether there are versioned object keys in the request or not,
* the entire Multi-Object Delete request will fail. For information about MFA Delete, see
* MFA Delete in the Amazon S3 User Guide.
*
*
*
* This functionality is not supported for directory buckets.
*
*/
public final String mfa() {
return mfa;
}
/**
* Returns the value of the RequestPayer property for this object.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #requestPayer} will
* return {@link RequestPayer#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #requestPayerAsString}.
*
*
* @return The value of the RequestPayer property for this object.
* @see RequestPayer
*/
public final RequestPayer requestPayer() {
return RequestPayer.fromValue(requestPayer);
}
/**
* Returns the value of the RequestPayer property for this object.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #requestPayer} will
* return {@link RequestPayer#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #requestPayerAsString}.
*
*
* @return The value of the RequestPayer property for this object.
* @see RequestPayer
*/
public final String requestPayerAsString() {
return requestPayer;
}
/**
*
* Specifies whether you want to delete this object even if it has a Governance-type Object Lock in place. To use
* this header, you must have the s3:BypassGovernanceRetention
permission.
*
*
*
* This functionality is not supported for directory buckets.
*
*
*
* @return Specifies whether you want to delete this object even if it has a Governance-type Object Lock in place.
* To use this header, you must have the s3:BypassGovernanceRetention
permission.
*
* This functionality is not supported for directory buckets.
*
*/
public final Boolean bypassGovernanceRetention() {
return bypassGovernanceRetention;
}
/**
*
* The account ID of the expected bucket owner. If the account ID that you provide does not match the actual owner
* of the bucket, the request fails with the HTTP status code 403 Forbidden
(access denied).
*
*
* @return The account ID of the expected bucket owner. If the account ID that you provide does not match the actual
* owner of the bucket, the request fails with the HTTP status code 403 Forbidden
(access
* denied).
*/
public final String expectedBucketOwner() {
return expectedBucketOwner;
}
/**
*
* Indicates the algorithm used to create the checksum for the object when you use the SDK. This header will not
* provide any additional functionality if you don't use the SDK. When you send this header, there must be a
* corresponding x-amz-checksum-algorithm
or x-amz-trailer
header sent. Otherwise,
* Amazon S3 fails the request with the HTTP status code 400 Bad Request
.
*
*
* For the x-amz-checksum-algorithm
header, replace algorithm
with the
* supported algorithm from the following list:
*
*
* -
*
* CRC32
*
*
* -
*
* CRC32C
*
*
* -
*
* SHA1
*
*
* -
*
* SHA256
*
*
*
*
* For more information, see Checking object
* integrity in the Amazon S3 User Guide.
*
*
* If the individual checksum value you provide through x-amz-checksum-algorithm
doesn't match
* the checksum algorithm you set through x-amz-sdk-checksum-algorithm
, Amazon S3 ignores any provided
* ChecksumAlgorithm
parameter and uses the checksum algorithm that matches the provided value in
* x-amz-checksum-algorithm
.
*
*
* If you provide an individual checksum, Amazon S3 ignores any provided ChecksumAlgorithm
parameter.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #checksumAlgorithm}
* will return {@link ChecksumAlgorithm#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #checksumAlgorithmAsString}.
*
*
* @return Indicates the algorithm used to create the checksum for the object when you use the SDK. This header will
* not provide any additional functionality if you don't use the SDK. When you send this header, there must
* be a corresponding x-amz-checksum-algorithm
or x-amz-trailer
header
* sent. Otherwise, Amazon S3 fails the request with the HTTP status code 400 Bad Request
.
*
* For the x-amz-checksum-algorithm
header, replace algorithm
* with the supported algorithm from the following list:
*
*
* -
*
* CRC32
*
*
* -
*
* CRC32C
*
*
* -
*
* SHA1
*
*
* -
*
* SHA256
*
*
*
*
* For more information, see Checking
* object integrity in the Amazon S3 User Guide.
*
*
* If the individual checksum value you provide through x-amz-checksum-algorithm
* doesn't match the checksum algorithm you set through x-amz-sdk-checksum-algorithm
, Amazon S3
* ignores any provided ChecksumAlgorithm
parameter and uses the checksum algorithm that
* matches the provided value in x-amz-checksum-algorithm
.
*
*
* If you provide an individual checksum, Amazon S3 ignores any provided ChecksumAlgorithm
* parameter.
* @see ChecksumAlgorithm
*/
public final ChecksumAlgorithm checksumAlgorithm() {
return ChecksumAlgorithm.fromValue(checksumAlgorithm);
}
/**
*
* Indicates the algorithm used to create the checksum for the object when you use the SDK. This header will not
* provide any additional functionality if you don't use the SDK. When you send this header, there must be a
* corresponding x-amz-checksum-algorithm
or x-amz-trailer
header sent. Otherwise,
* Amazon S3 fails the request with the HTTP status code 400 Bad Request
.
*
*
* For the x-amz-checksum-algorithm
header, replace algorithm
with the
* supported algorithm from the following list:
*
*
* -
*
* CRC32
*
*
* -
*
* CRC32C
*
*
* -
*
* SHA1
*
*
* -
*
* SHA256
*
*
*
*
* For more information, see Checking object
* integrity in the Amazon S3 User Guide.
*
*
* If the individual checksum value you provide through x-amz-checksum-algorithm
doesn't match
* the checksum algorithm you set through x-amz-sdk-checksum-algorithm
, Amazon S3 ignores any provided
* ChecksumAlgorithm
parameter and uses the checksum algorithm that matches the provided value in
* x-amz-checksum-algorithm
.
*
*
* If you provide an individual checksum, Amazon S3 ignores any provided ChecksumAlgorithm
parameter.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #checksumAlgorithm}
* will return {@link ChecksumAlgorithm#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #checksumAlgorithmAsString}.
*
*
* @return Indicates the algorithm used to create the checksum for the object when you use the SDK. This header will
* not provide any additional functionality if you don't use the SDK. When you send this header, there must
* be a corresponding x-amz-checksum-algorithm
or x-amz-trailer
header
* sent. Otherwise, Amazon S3 fails the request with the HTTP status code 400 Bad Request
.
*
* For the x-amz-checksum-algorithm
header, replace algorithm
* with the supported algorithm from the following list:
*
*
* -
*
* CRC32
*
*
* -
*
* CRC32C
*
*
* -
*
* SHA1
*
*
* -
*
* SHA256
*
*
*
*
* For more information, see Checking
* object integrity in the Amazon S3 User Guide.
*
*
* If the individual checksum value you provide through x-amz-checksum-algorithm
* doesn't match the checksum algorithm you set through x-amz-sdk-checksum-algorithm
, Amazon S3
* ignores any provided ChecksumAlgorithm
parameter and uses the checksum algorithm that
* matches the provided value in x-amz-checksum-algorithm
.
*
*
* If you provide an individual checksum, Amazon S3 ignores any provided ChecksumAlgorithm
* parameter.
* @see ChecksumAlgorithm
*/
public final String checksumAlgorithmAsString() {
return checksumAlgorithm;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(bucket());
hashCode = 31 * hashCode + Objects.hashCode(delete());
hashCode = 31 * hashCode + Objects.hashCode(mfa());
hashCode = 31 * hashCode + Objects.hashCode(requestPayerAsString());
hashCode = 31 * hashCode + Objects.hashCode(bypassGovernanceRetention());
hashCode = 31 * hashCode + Objects.hashCode(expectedBucketOwner());
hashCode = 31 * hashCode + Objects.hashCode(checksumAlgorithmAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof DeleteObjectsRequest)) {
return false;
}
DeleteObjectsRequest other = (DeleteObjectsRequest) obj;
return Objects.equals(bucket(), other.bucket()) && Objects.equals(delete(), other.delete())
&& Objects.equals(mfa(), other.mfa()) && Objects.equals(requestPayerAsString(), other.requestPayerAsString())
&& Objects.equals(bypassGovernanceRetention(), other.bypassGovernanceRetention())
&& Objects.equals(expectedBucketOwner(), other.expectedBucketOwner())
&& Objects.equals(checksumAlgorithmAsString(), other.checksumAlgorithmAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("DeleteObjectsRequest").add("Bucket", bucket()).add("Delete", delete()).add("MFA", mfa())
.add("RequestPayer", requestPayerAsString()).add("BypassGovernanceRetention", bypassGovernanceRetention())
.add("ExpectedBucketOwner", expectedBucketOwner()).add("ChecksumAlgorithm", checksumAlgorithmAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Bucket":
return Optional.ofNullable(clazz.cast(bucket()));
case "Delete":
return Optional.ofNullable(clazz.cast(delete()));
case "MFA":
return Optional.ofNullable(clazz.cast(mfa()));
case "RequestPayer":
return Optional.ofNullable(clazz.cast(requestPayerAsString()));
case "BypassGovernanceRetention":
return Optional.ofNullable(clazz.cast(bypassGovernanceRetention()));
case "ExpectedBucketOwner":
return Optional.ofNullable(clazz.cast(expectedBucketOwner()));
case "ChecksumAlgorithm":
return Optional.ofNullable(clazz.cast(checksumAlgorithmAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
*
* Directory buckets - When you use this operation with a directory bucket, you must use
* virtual-hosted-style requests in the format
* Bucket_name.s3express-az_id.region.amazonaws.com
. Path-style
* requests are not supported. Directory bucket names must be unique in the chosen Availability Zone.
* Bucket names must follow the format bucket_base_name--az-id--x-s3
(for
* example, DOC-EXAMPLE-BUCKET--usw2-az1--x-s3
). For information about bucket
* naming restrictions, see Directory bucket naming rules in the Amazon S3 User Guide.
*
*
* Access points - When you use this action with an access point, you must provide the alias of
* the access point in place of the bucket name or specify the access point ARN. When using the access
* point ARN, you must direct requests to the access point hostname. The access point hostname takes the
* form AccessPointName-AccountId.s3-accesspoint.Region.amazonaws.com. When using
* this action with an access point through the Amazon Web Services SDKs, you provide the access point
* ARN in place of the bucket name. For more information about access point ARNs, see Using access
* points in the Amazon S3 User Guide.
*
*
*
* Access points and Object Lambda access points are not supported by directory buckets.
*
*
*
* S3 on Outposts - When you use this action with Amazon S3 on Outposts, you must direct requests
* to the S3 on Outposts hostname. The S3 on Outposts hostname takes the form
* AccessPointName-AccountId.outpostID.s3-outposts.Region.amazonaws.com
* . When you use this action with S3 on Outposts through the Amazon Web Services SDKs, you provide the
* Outposts access point ARN in place of the bucket name. For more information about S3 on Outposts ARNs,
* see What is S3 on
* Outposts? in the Amazon S3 User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder bucket(String bucket);
/**
*
* Container for the request.
*
*
* @param delete
* Container for the request.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder delete(Delete delete);
/**
*
* Container for the request.
*
* This is a convenience method that creates an instance of the {@link Delete.Builder} avoiding the need to
* create one manually via {@link Delete#builder()}.
*
*
* When the {@link Consumer} completes, {@link Delete.Builder#build()} is called immediately and its result is
* passed to {@link #delete(Delete)}.
*
* @param delete
* a consumer that will call methods on {@link Delete.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #delete(Delete)
*/
default Builder delete(Consumer delete) {
return delete(Delete.builder().applyMutation(delete).build());
}
/**
*
* The concatenation of the authentication device's serial number, a space, and the value that is displayed on
* your authentication device. Required to permanently delete a versioned object if versioning is configured
* with MFA delete enabled.
*
*
* When performing the DeleteObjects
operation on an MFA delete enabled bucket, which attempts to
* delete the specified versioned objects, you must include an MFA token. If you don't provide an MFA token, the
* entire request will fail, even if there are non-versioned objects that you are trying to delete. If you
* provide an invalid token, whether there are versioned object keys in the request or not, the entire
* Multi-Object Delete request will fail. For information about MFA Delete, see MFA
* Delete in the Amazon S3 User Guide.
*
*
*
* This functionality is not supported for directory buckets.
*
*
*
* @param mfa
* The concatenation of the authentication device's serial number, a space, and the value that is
* displayed on your authentication device. Required to permanently delete a versioned object if
* versioning is configured with MFA delete enabled.
*
* When performing the DeleteObjects
operation on an MFA delete enabled bucket, which
* attempts to delete the specified versioned objects, you must include an MFA token. If you don't
* provide an MFA token, the entire request will fail, even if there are non-versioned objects that you
* are trying to delete. If you provide an invalid token, whether there are versioned object keys in the
* request or not, the entire Multi-Object Delete request will fail. For information about MFA Delete,
* see MFA
* Delete in the Amazon S3 User Guide.
*
*
*
* This functionality is not supported for directory buckets.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder mfa(String mfa);
/**
* Sets the value of the RequestPayer property for this object.
*
* @param requestPayer
* The new value for the RequestPayer property for this object.
* @see RequestPayer
* @return Returns a reference to this object so that method calls can be chained together.
* @see RequestPayer
*/
Builder requestPayer(String requestPayer);
/**
* Sets the value of the RequestPayer property for this object.
*
* @param requestPayer
* The new value for the RequestPayer property for this object.
* @see RequestPayer
* @return Returns a reference to this object so that method calls can be chained together.
* @see RequestPayer
*/
Builder requestPayer(RequestPayer requestPayer);
/**
*
* Specifies whether you want to delete this object even if it has a Governance-type Object Lock in place. To
* use this header, you must have the s3:BypassGovernanceRetention
permission.
*
*
*
* This functionality is not supported for directory buckets.
*
*
*
* @param bypassGovernanceRetention
* Specifies whether you want to delete this object even if it has a Governance-type Object Lock in
* place. To use this header, you must have the s3:BypassGovernanceRetention
permission.
*
*
* This functionality is not supported for directory buckets.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder bypassGovernanceRetention(Boolean bypassGovernanceRetention);
/**
*
* The account ID of the expected bucket owner. If the account ID that you provide does not match the actual
* owner of the bucket, the request fails with the HTTP status code 403 Forbidden
(access denied).
*
*
* @param expectedBucketOwner
* The account ID of the expected bucket owner. If the account ID that you provide does not match the
* actual owner of the bucket, the request fails with the HTTP status code 403 Forbidden
* (access denied).
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder expectedBucketOwner(String expectedBucketOwner);
/**
*
* Indicates the algorithm used to create the checksum for the object when you use the SDK. This header will not
* provide any additional functionality if you don't use the SDK. When you send this header, there must be a
* corresponding x-amz-checksum-algorithm
or x-amz-trailer
header sent.
* Otherwise, Amazon S3 fails the request with the HTTP status code 400 Bad Request
.
*
*
* For the x-amz-checksum-algorithm
header, replace algorithm
with
* the supported algorithm from the following list:
*
*
* -
*
* CRC32
*
*
* -
*
* CRC32C
*
*
* -
*
* SHA1
*
*
* -
*
* SHA256
*
*
*
*
* For more information, see Checking object
* integrity in the Amazon S3 User Guide.
*
*
* If the individual checksum value you provide through x-amz-checksum-algorithm
doesn't
* match the checksum algorithm you set through x-amz-sdk-checksum-algorithm
, Amazon S3 ignores any
* provided ChecksumAlgorithm
parameter and uses the checksum algorithm that matches the provided
* value in x-amz-checksum-algorithm
.
*
*
* If you provide an individual checksum, Amazon S3 ignores any provided ChecksumAlgorithm
* parameter.
*
*
* @param checksumAlgorithm
* Indicates the algorithm used to create the checksum for the object when you use the SDK. This header
* will not provide any additional functionality if you don't use the SDK. When you send this header,
* there must be a corresponding x-amz-checksum-algorithm
or
* x-amz-trailer
header sent. Otherwise, Amazon S3 fails the request with the HTTP status
* code 400 Bad Request
.
*
* For the x-amz-checksum-algorithm
header, replace algorithm
* with the supported algorithm from the following list:
*
*
* -
*
* CRC32
*
*
* -
*
* CRC32C
*
*
* -
*
* SHA1
*
*
* -
*
* SHA256
*
*
*
*
* For more information, see Checking
* object integrity in the Amazon S3 User Guide.
*
*
* If the individual checksum value you provide through x-amz-checksum-algorithm
* doesn't match the checksum algorithm you set through x-amz-sdk-checksum-algorithm
, Amazon
* S3 ignores any provided ChecksumAlgorithm
parameter and uses the checksum algorithm that
* matches the provided value in x-amz-checksum-algorithm
.
*
*
* If you provide an individual checksum, Amazon S3 ignores any provided ChecksumAlgorithm
* parameter.
* @see ChecksumAlgorithm
* @return Returns a reference to this object so that method calls can be chained together.
* @see ChecksumAlgorithm
*/
Builder checksumAlgorithm(String checksumAlgorithm);
/**
*
* Indicates the algorithm used to create the checksum for the object when you use the SDK. This header will not
* provide any additional functionality if you don't use the SDK. When you send this header, there must be a
* corresponding x-amz-checksum-algorithm
or x-amz-trailer
header sent.
* Otherwise, Amazon S3 fails the request with the HTTP status code 400 Bad Request
.
*
*
* For the x-amz-checksum-algorithm
header, replace algorithm
with
* the supported algorithm from the following list:
*
*
* -
*
* CRC32
*
*
* -
*
* CRC32C
*
*
* -
*
* SHA1
*
*
* -
*
* SHA256
*
*
*
*
* For more information, see Checking object
* integrity in the Amazon S3 User Guide.
*
*
* If the individual checksum value you provide through x-amz-checksum-algorithm
doesn't
* match the checksum algorithm you set through x-amz-sdk-checksum-algorithm
, Amazon S3 ignores any
* provided ChecksumAlgorithm
parameter and uses the checksum algorithm that matches the provided
* value in x-amz-checksum-algorithm
.
*
*
* If you provide an individual checksum, Amazon S3 ignores any provided ChecksumAlgorithm
* parameter.
*
*
* @param checksumAlgorithm
* Indicates the algorithm used to create the checksum for the object when you use the SDK. This header
* will not provide any additional functionality if you don't use the SDK. When you send this header,
* there must be a corresponding x-amz-checksum-algorithm
or
* x-amz-trailer
header sent. Otherwise, Amazon S3 fails the request with the HTTP status
* code 400 Bad Request
.
*
* For the x-amz-checksum-algorithm
header, replace algorithm
* with the supported algorithm from the following list:
*
*
* -
*
* CRC32
*
*
* -
*
* CRC32C
*
*
* -
*
* SHA1
*
*
* -
*
* SHA256
*
*
*
*
* For more information, see Checking
* object integrity in the Amazon S3 User Guide.
*
*
* If the individual checksum value you provide through x-amz-checksum-algorithm
* doesn't match the checksum algorithm you set through x-amz-sdk-checksum-algorithm
, Amazon
* S3 ignores any provided ChecksumAlgorithm
parameter and uses the checksum algorithm that
* matches the provided value in x-amz-checksum-algorithm
.
*
*
* If you provide an individual checksum, Amazon S3 ignores any provided ChecksumAlgorithm
* parameter.
* @see ChecksumAlgorithm
* @return Returns a reference to this object so that method calls can be chained together.
* @see ChecksumAlgorithm
*/
Builder checksumAlgorithm(ChecksumAlgorithm checksumAlgorithm);
@Override
Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration);
@Override
Builder overrideConfiguration(Consumer builderConsumer);
}
static final class BuilderImpl extends S3Request.BuilderImpl implements Builder {
private String bucket;
private Delete delete;
private String mfa;
private String requestPayer;
private Boolean bypassGovernanceRetention;
private String expectedBucketOwner;
private String checksumAlgorithm;
private BuilderImpl() {
}
private BuilderImpl(DeleteObjectsRequest model) {
super(model);
bucket(model.bucket);
delete(model.delete);
mfa(model.mfa);
requestPayer(model.requestPayer);
bypassGovernanceRetention(model.bypassGovernanceRetention);
expectedBucketOwner(model.expectedBucketOwner);
checksumAlgorithm(model.checksumAlgorithm);
}
public final String getBucket() {
return bucket;
}
public final void setBucket(String bucket) {
this.bucket = bucket;
}
@Override
public final Builder bucket(String bucket) {
this.bucket = bucket;
return this;
}
public final Delete.Builder getDelete() {
return delete != null ? delete.toBuilder() : null;
}
public final void setDelete(Delete.BuilderImpl delete) {
this.delete = delete != null ? delete.build() : null;
}
@Override
public final Builder delete(Delete delete) {
this.delete = delete;
return this;
}
public final String getMfa() {
return mfa;
}
public final void setMfa(String mfa) {
this.mfa = mfa;
}
@Override
public final Builder mfa(String mfa) {
this.mfa = mfa;
return this;
}
public final String getRequestPayer() {
return requestPayer;
}
public final void setRequestPayer(String requestPayer) {
this.requestPayer = requestPayer;
}
@Override
public final Builder requestPayer(String requestPayer) {
this.requestPayer = requestPayer;
return this;
}
@Override
public final Builder requestPayer(RequestPayer requestPayer) {
this.requestPayer(requestPayer == null ? null : requestPayer.toString());
return this;
}
public final Boolean getBypassGovernanceRetention() {
return bypassGovernanceRetention;
}
public final void setBypassGovernanceRetention(Boolean bypassGovernanceRetention) {
this.bypassGovernanceRetention = bypassGovernanceRetention;
}
@Override
public final Builder bypassGovernanceRetention(Boolean bypassGovernanceRetention) {
this.bypassGovernanceRetention = bypassGovernanceRetention;
return this;
}
public final String getExpectedBucketOwner() {
return expectedBucketOwner;
}
public final void setExpectedBucketOwner(String expectedBucketOwner) {
this.expectedBucketOwner = expectedBucketOwner;
}
@Override
public final Builder expectedBucketOwner(String expectedBucketOwner) {
this.expectedBucketOwner = expectedBucketOwner;
return this;
}
public final String getChecksumAlgorithm() {
return checksumAlgorithm;
}
public final void setChecksumAlgorithm(String checksumAlgorithm) {
this.checksumAlgorithm = checksumAlgorithm;
}
@Override
public final Builder checksumAlgorithm(String checksumAlgorithm) {
this.checksumAlgorithm = checksumAlgorithm;
return this;
}
@Override
public final Builder checksumAlgorithm(ChecksumAlgorithm checksumAlgorithm) {
this.checksumAlgorithm(checksumAlgorithm == null ? null : checksumAlgorithm.toString());
return this;
}
@Override
public Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration) {
super.overrideConfiguration(overrideConfiguration);
return this;
}
@Override
public Builder overrideConfiguration(Consumer builderConsumer) {
super.overrideConfiguration(builderConsumer);
return this;
}
@Override
public DeleteObjectsRequest build() {
return new DeleteObjectsRequest(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
}
}