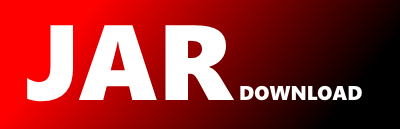
software.amazon.awssdk.services.s3.model.InputSerialization Maven / Gradle / Ivy
Show all versions of s3 Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.s3.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Describes the serialization format of the object.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class InputSerialization implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField CSV_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("CSV")
.getter(getter(InputSerialization::csv))
.setter(setter(Builder::csv))
.constructor(CSVInput::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CSV").unmarshallLocationName("CSV")
.build()).build();
private static final SdkField COMPRESSION_TYPE_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("CompressionType")
.getter(getter(InputSerialization::compressionTypeAsString))
.setter(setter(Builder::compressionType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CompressionType")
.unmarshallLocationName("CompressionType").build()).build();
private static final SdkField JSON_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("JSON")
.getter(getter(InputSerialization::json))
.setter(setter(Builder::json))
.constructor(JSONInput::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("JSON")
.unmarshallLocationName("JSON").build()).build();
private static final SdkField PARQUET_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("Parquet")
.getter(getter(InputSerialization::parquet))
.setter(setter(Builder::parquet))
.constructor(ParquetInput::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Parquet")
.unmarshallLocationName("Parquet").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(CSV_FIELD,
COMPRESSION_TYPE_FIELD, JSON_FIELD, PARQUET_FIELD));
private static final long serialVersionUID = 1L;
private final CSVInput csv;
private final String compressionType;
private final JSONInput json;
private final ParquetInput parquet;
private InputSerialization(BuilderImpl builder) {
this.csv = builder.csv;
this.compressionType = builder.compressionType;
this.json = builder.json;
this.parquet = builder.parquet;
}
/**
*
* Describes the serialization of a CSV-encoded object.
*
*
* @return Describes the serialization of a CSV-encoded object.
*/
public final CSVInput csv() {
return csv;
}
/**
*
* Specifies object's compression format. Valid values: NONE, GZIP, BZIP2. Default Value: NONE.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #compressionType}
* will return {@link CompressionType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #compressionTypeAsString}.
*
*
* @return Specifies object's compression format. Valid values: NONE, GZIP, BZIP2. Default Value: NONE.
* @see CompressionType
*/
public final CompressionType compressionType() {
return CompressionType.fromValue(compressionType);
}
/**
*
* Specifies object's compression format. Valid values: NONE, GZIP, BZIP2. Default Value: NONE.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #compressionType}
* will return {@link CompressionType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #compressionTypeAsString}.
*
*
* @return Specifies object's compression format. Valid values: NONE, GZIP, BZIP2. Default Value: NONE.
* @see CompressionType
*/
public final String compressionTypeAsString() {
return compressionType;
}
/**
*
* Specifies JSON as object's input serialization format.
*
*
* @return Specifies JSON as object's input serialization format.
*/
public final JSONInput json() {
return json;
}
/**
*
* Specifies Parquet as object's input serialization format.
*
*
* @return Specifies Parquet as object's input serialization format.
*/
public final ParquetInput parquet() {
return parquet;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(csv());
hashCode = 31 * hashCode + Objects.hashCode(compressionTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(json());
hashCode = 31 * hashCode + Objects.hashCode(parquet());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof InputSerialization)) {
return false;
}
InputSerialization other = (InputSerialization) obj;
return Objects.equals(csv(), other.csv()) && Objects.equals(compressionTypeAsString(), other.compressionTypeAsString())
&& Objects.equals(json(), other.json()) && Objects.equals(parquet(), other.parquet());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("InputSerialization").add("CSV", csv()).add("CompressionType", compressionTypeAsString())
.add("JSON", json()).add("Parquet", parquet()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "CSV":
return Optional.ofNullable(clazz.cast(csv()));
case "CompressionType":
return Optional.ofNullable(clazz.cast(compressionTypeAsString()));
case "JSON":
return Optional.ofNullable(clazz.cast(json()));
case "Parquet":
return Optional.ofNullable(clazz.cast(parquet()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function