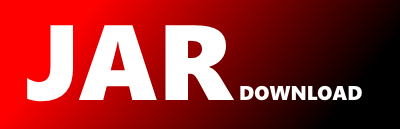
software.amazon.awssdk.services.s3.model.ObjectVersion Maven / Gradle / Ivy
Show all versions of s3 Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.s3.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The version of an object.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ObjectVersion implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField E_TAG_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("ETag")
.getter(getter(ObjectVersion::eTag))
.setter(setter(Builder::eTag))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ETag")
.unmarshallLocationName("ETag").build()).build();
private static final SdkField> CHECKSUM_ALGORITHM_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("ChecksumAlgorithm")
.getter(getter(ObjectVersion::checksumAlgorithmAsStrings))
.setter(setter(Builder::checksumAlgorithmWithStrings))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ChecksumAlgorithm")
.unmarshallLocationName("ChecksumAlgorithm").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").unmarshallLocationName("member").build()).build())
.isFlattened(true).build()).build();
private static final SdkField SIZE_FIELD = SdkField
. builder(MarshallingType.LONG)
.memberName("Size")
.getter(getter(ObjectVersion::size))
.setter(setter(Builder::size))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Size")
.unmarshallLocationName("Size").build()).build();
private static final SdkField STORAGE_CLASS_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("StorageClass")
.getter(getter(ObjectVersion::storageClassAsString))
.setter(setter(Builder::storageClass))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StorageClass")
.unmarshallLocationName("StorageClass").build()).build();
private static final SdkField KEY_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("Key")
.getter(getter(ObjectVersion::key))
.setter(setter(Builder::key))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Key").unmarshallLocationName("Key")
.build()).build();
private static final SdkField VERSION_ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("VersionId")
.getter(getter(ObjectVersion::versionId))
.setter(setter(Builder::versionId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("VersionId")
.unmarshallLocationName("VersionId").build()).build();
private static final SdkField IS_LATEST_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("IsLatest")
.getter(getter(ObjectVersion::isLatest))
.setter(setter(Builder::isLatest))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IsLatest")
.unmarshallLocationName("IsLatest").build()).build();
private static final SdkField LAST_MODIFIED_FIELD = SdkField
. builder(MarshallingType.INSTANT)
.memberName("LastModified")
.getter(getter(ObjectVersion::lastModified))
.setter(setter(Builder::lastModified))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LastModified")
.unmarshallLocationName("LastModified").build()).build();
private static final SdkField OWNER_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("Owner")
.getter(getter(ObjectVersion::owner))
.setter(setter(Builder::owner))
.constructor(Owner::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Owner")
.unmarshallLocationName("Owner").build()).build();
private static final SdkField RESTORE_STATUS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("RestoreStatus")
.getter(getter(ObjectVersion::restoreStatus))
.setter(setter(Builder::restoreStatus))
.constructor(RestoreStatus::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RestoreStatus")
.unmarshallLocationName("RestoreStatus").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(E_TAG_FIELD,
CHECKSUM_ALGORITHM_FIELD, SIZE_FIELD, STORAGE_CLASS_FIELD, KEY_FIELD, VERSION_ID_FIELD, IS_LATEST_FIELD,
LAST_MODIFIED_FIELD, OWNER_FIELD, RESTORE_STATUS_FIELD));
private static final long serialVersionUID = 1L;
private final String eTag;
private final List checksumAlgorithm;
private final Long size;
private final String storageClass;
private final String key;
private final String versionId;
private final Boolean isLatest;
private final Instant lastModified;
private final Owner owner;
private final RestoreStatus restoreStatus;
private ObjectVersion(BuilderImpl builder) {
this.eTag = builder.eTag;
this.checksumAlgorithm = builder.checksumAlgorithm;
this.size = builder.size;
this.storageClass = builder.storageClass;
this.key = builder.key;
this.versionId = builder.versionId;
this.isLatest = builder.isLatest;
this.lastModified = builder.lastModified;
this.owner = builder.owner;
this.restoreStatus = builder.restoreStatus;
}
/**
*
* The entity tag is an MD5 hash of that version of the object.
*
*
* @return The entity tag is an MD5 hash of that version of the object.
*/
public final String eTag() {
return eTag;
}
/**
*
* The algorithm that was used to create a checksum of the object.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasChecksumAlgorithm} method.
*
*
* @return The algorithm that was used to create a checksum of the object.
*/
public final List checksumAlgorithm() {
return ChecksumAlgorithmListCopier.copyStringToEnum(checksumAlgorithm);
}
/**
* For responses, this returns true if the service returned a value for the ChecksumAlgorithm property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasChecksumAlgorithm() {
return checksumAlgorithm != null && !(checksumAlgorithm instanceof SdkAutoConstructList);
}
/**
*
* The algorithm that was used to create a checksum of the object.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasChecksumAlgorithm} method.
*
*
* @return The algorithm that was used to create a checksum of the object.
*/
public final List checksumAlgorithmAsStrings() {
return checksumAlgorithm;
}
/**
*
* Size in bytes of the object.
*
*
* @return Size in bytes of the object.
*/
public final Long size() {
return size;
}
/**
*
* The class of storage used to store the object.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #storageClass} will
* return {@link ObjectVersionStorageClass#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #storageClassAsString}.
*
*
* @return The class of storage used to store the object.
* @see ObjectVersionStorageClass
*/
public final ObjectVersionStorageClass storageClass() {
return ObjectVersionStorageClass.fromValue(storageClass);
}
/**
*
* The class of storage used to store the object.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #storageClass} will
* return {@link ObjectVersionStorageClass#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #storageClassAsString}.
*
*
* @return The class of storage used to store the object.
* @see ObjectVersionStorageClass
*/
public final String storageClassAsString() {
return storageClass;
}
/**
*
* The object key.
*
*
* @return The object key.
*/
public final String key() {
return key;
}
/**
*
* Version ID of an object.
*
*
* @return Version ID of an object.
*/
public final String versionId() {
return versionId;
}
/**
*
* Specifies whether the object is (true) or is not (false) the latest version of an object.
*
*
* @return Specifies whether the object is (true) or is not (false) the latest version of an object.
*/
public final Boolean isLatest() {
return isLatest;
}
/**
*
* Date and time when the object was last modified.
*
*
* @return Date and time when the object was last modified.
*/
public final Instant lastModified() {
return lastModified;
}
/**
*
* Specifies the owner of the object.
*
*
* @return Specifies the owner of the object.
*/
public final Owner owner() {
return owner;
}
/**
*
* Specifies the restoration status of an object. Objects in certain storage classes must be restored before they
* can be retrieved. For more information about these storage classes and how to work with archived objects, see Working with archived
* objects in the Amazon S3 User Guide.
*
*
* @return Specifies the restoration status of an object. Objects in certain storage classes must be restored before
* they can be retrieved. For more information about these storage classes and how to work with archived
* objects, see
* Working with archived objects in the Amazon S3 User Guide.
*/
public final RestoreStatus restoreStatus() {
return restoreStatus;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(eTag());
hashCode = 31 * hashCode + Objects.hashCode(hasChecksumAlgorithm() ? checksumAlgorithmAsStrings() : null);
hashCode = 31 * hashCode + Objects.hashCode(size());
hashCode = 31 * hashCode + Objects.hashCode(storageClassAsString());
hashCode = 31 * hashCode + Objects.hashCode(key());
hashCode = 31 * hashCode + Objects.hashCode(versionId());
hashCode = 31 * hashCode + Objects.hashCode(isLatest());
hashCode = 31 * hashCode + Objects.hashCode(lastModified());
hashCode = 31 * hashCode + Objects.hashCode(owner());
hashCode = 31 * hashCode + Objects.hashCode(restoreStatus());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ObjectVersion)) {
return false;
}
ObjectVersion other = (ObjectVersion) obj;
return Objects.equals(eTag(), other.eTag()) && hasChecksumAlgorithm() == other.hasChecksumAlgorithm()
&& Objects.equals(checksumAlgorithmAsStrings(), other.checksumAlgorithmAsStrings())
&& Objects.equals(size(), other.size()) && Objects.equals(storageClassAsString(), other.storageClassAsString())
&& Objects.equals(key(), other.key()) && Objects.equals(versionId(), other.versionId())
&& Objects.equals(isLatest(), other.isLatest()) && Objects.equals(lastModified(), other.lastModified())
&& Objects.equals(owner(), other.owner()) && Objects.equals(restoreStatus(), other.restoreStatus());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ObjectVersion").add("ETag", eTag())
.add("ChecksumAlgorithm", hasChecksumAlgorithm() ? checksumAlgorithmAsStrings() : null).add("Size", size())
.add("StorageClass", storageClassAsString()).add("Key", key()).add("VersionId", versionId())
.add("IsLatest", isLatest()).add("LastModified", lastModified()).add("Owner", owner())
.add("RestoreStatus", restoreStatus()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ETag":
return Optional.ofNullable(clazz.cast(eTag()));
case "ChecksumAlgorithm":
return Optional.ofNullable(clazz.cast(checksumAlgorithmAsStrings()));
case "Size":
return Optional.ofNullable(clazz.cast(size()));
case "StorageClass":
return Optional.ofNullable(clazz.cast(storageClassAsString()));
case "Key":
return Optional.ofNullable(clazz.cast(key()));
case "VersionId":
return Optional.ofNullable(clazz.cast(versionId()));
case "IsLatest":
return Optional.ofNullable(clazz.cast(isLatest()));
case "LastModified":
return Optional.ofNullable(clazz.cast(lastModified()));
case "Owner":
return Optional.ofNullable(clazz.cast(owner()));
case "RestoreStatus":
return Optional.ofNullable(clazz.cast(restoreStatus()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function