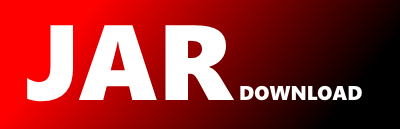
software.amazon.awssdk.services.s3.model.NotificationConfiguration Maven / Gradle / Ivy
Show all versions of s3 Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.s3.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* A container for specifying the notification configuration of the bucket. If this element is empty, notifications are
* turned off for the bucket.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class NotificationConfiguration implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField> TOPIC_CONFIGURATIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("TopicConfigurations")
.getter(getter(NotificationConfiguration::topicConfigurations))
.setter(setter(Builder::topicConfigurations))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TopicConfiguration")
.unmarshallLocationName("TopicConfiguration").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(TopicConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").unmarshallLocationName("member").build()).build())
.isFlattened(true).build()).build();
private static final SdkField> QUEUE_CONFIGURATIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("QueueConfigurations")
.getter(getter(NotificationConfiguration::queueConfigurations))
.setter(setter(Builder::queueConfigurations))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("QueueConfiguration")
.unmarshallLocationName("QueueConfiguration").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(QueueConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").unmarshallLocationName("member").build()).build())
.isFlattened(true).build()).build();
private static final SdkField> LAMBDA_FUNCTION_CONFIGURATIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("LambdaFunctionConfigurations")
.getter(getter(NotificationConfiguration::lambdaFunctionConfigurations))
.setter(setter(Builder::lambdaFunctionConfigurations))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CloudFunctionConfiguration")
.unmarshallLocationName("CloudFunctionConfiguration").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(LambdaFunctionConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").unmarshallLocationName("member").build()).build())
.isFlattened(true).build()).build();
private static final SdkField EVENT_BRIDGE_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("EventBridgeConfiguration")
.getter(getter(NotificationConfiguration::eventBridgeConfiguration))
.setter(setter(Builder::eventBridgeConfiguration))
.constructor(EventBridgeConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EventBridgeConfiguration")
.unmarshallLocationName("EventBridgeConfiguration").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(TOPIC_CONFIGURATIONS_FIELD,
QUEUE_CONFIGURATIONS_FIELD, LAMBDA_FUNCTION_CONFIGURATIONS_FIELD, EVENT_BRIDGE_CONFIGURATION_FIELD));
private static final long serialVersionUID = 1L;
private final List topicConfigurations;
private final List queueConfigurations;
private final List lambdaFunctionConfigurations;
private final EventBridgeConfiguration eventBridgeConfiguration;
private NotificationConfiguration(BuilderImpl builder) {
this.topicConfigurations = builder.topicConfigurations;
this.queueConfigurations = builder.queueConfigurations;
this.lambdaFunctionConfigurations = builder.lambdaFunctionConfigurations;
this.eventBridgeConfiguration = builder.eventBridgeConfiguration;
}
/**
* For responses, this returns true if the service returned a value for the TopicConfigurations property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasTopicConfigurations() {
return topicConfigurations != null && !(topicConfigurations instanceof SdkAutoConstructList);
}
/**
*
* The topic to which notifications are sent and the events for which notifications are generated.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasTopicConfigurations} method.
*
*
* @return The topic to which notifications are sent and the events for which notifications are generated.
*/
public final List topicConfigurations() {
return topicConfigurations;
}
/**
* For responses, this returns true if the service returned a value for the QueueConfigurations property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasQueueConfigurations() {
return queueConfigurations != null && !(queueConfigurations instanceof SdkAutoConstructList);
}
/**
*
* The Amazon Simple Queue Service queues to publish messages to and the events for which to publish messages.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasQueueConfigurations} method.
*
*
* @return The Amazon Simple Queue Service queues to publish messages to and the events for which to publish
* messages.
*/
public final List queueConfigurations() {
return queueConfigurations;
}
/**
* For responses, this returns true if the service returned a value for the LambdaFunctionConfigurations property.
* This DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasLambdaFunctionConfigurations() {
return lambdaFunctionConfigurations != null && !(lambdaFunctionConfigurations instanceof SdkAutoConstructList);
}
/**
*
* Describes the Lambda functions to invoke and the events for which to invoke them.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasLambdaFunctionConfigurations} method.
*
*
* @return Describes the Lambda functions to invoke and the events for which to invoke them.
*/
public final List lambdaFunctionConfigurations() {
return lambdaFunctionConfigurations;
}
/**
*
* Enables delivery of events to Amazon EventBridge.
*
*
* @return Enables delivery of events to Amazon EventBridge.
*/
public final EventBridgeConfiguration eventBridgeConfiguration() {
return eventBridgeConfiguration;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(hasTopicConfigurations() ? topicConfigurations() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasQueueConfigurations() ? queueConfigurations() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasLambdaFunctionConfigurations() ? lambdaFunctionConfigurations() : null);
hashCode = 31 * hashCode + Objects.hashCode(eventBridgeConfiguration());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof NotificationConfiguration)) {
return false;
}
NotificationConfiguration other = (NotificationConfiguration) obj;
return hasTopicConfigurations() == other.hasTopicConfigurations()
&& Objects.equals(topicConfigurations(), other.topicConfigurations())
&& hasQueueConfigurations() == other.hasQueueConfigurations()
&& Objects.equals(queueConfigurations(), other.queueConfigurations())
&& hasLambdaFunctionConfigurations() == other.hasLambdaFunctionConfigurations()
&& Objects.equals(lambdaFunctionConfigurations(), other.lambdaFunctionConfigurations())
&& Objects.equals(eventBridgeConfiguration(), other.eventBridgeConfiguration());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("NotificationConfiguration")
.add("TopicConfigurations", hasTopicConfigurations() ? topicConfigurations() : null)
.add("QueueConfigurations", hasQueueConfigurations() ? queueConfigurations() : null)
.add("LambdaFunctionConfigurations", hasLambdaFunctionConfigurations() ? lambdaFunctionConfigurations() : null)
.add("EventBridgeConfiguration", eventBridgeConfiguration()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "TopicConfigurations":
return Optional.ofNullable(clazz.cast(topicConfigurations()));
case "QueueConfigurations":
return Optional.ofNullable(clazz.cast(queueConfigurations()));
case "LambdaFunctionConfigurations":
return Optional.ofNullable(clazz.cast(lambdaFunctionConfigurations()));
case "EventBridgeConfiguration":
return Optional.ofNullable(clazz.cast(eventBridgeConfiguration()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function