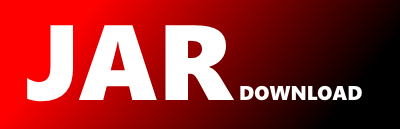
software.amazon.awssdk.services.s3.model.LifecycleRule Maven / Gradle / Ivy
Show all versions of s3 Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.s3.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.traits.RequiredTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* A lifecycle rule for individual objects in an Amazon S3 bucket.
*
*
* For more information see, Managing your storage
* lifecycle in the Amazon S3 User Guide.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class LifecycleRule implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField EXPIRATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("Expiration")
.getter(getter(LifecycleRule::expiration))
.setter(setter(Builder::expiration))
.constructor(LifecycleExpiration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Expiration")
.unmarshallLocationName("Expiration").build()).build();
private static final SdkField ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("ID")
.getter(getter(LifecycleRule::id))
.setter(setter(Builder::id))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ID").unmarshallLocationName("ID")
.build()).build();
private static final SdkField PREFIX_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("Prefix")
.getter(getter(LifecycleRule::prefix))
.setter(setter(Builder::prefix))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Prefix")
.unmarshallLocationName("Prefix").build()).build();
private static final SdkField FILTER_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("Filter")
.getter(getter(LifecycleRule::filter))
.setter(setter(Builder::filter))
.constructor(LifecycleRuleFilter::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Filter")
.unmarshallLocationName("Filter").build()).build();
private static final SdkField STATUS_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("Status")
.getter(getter(LifecycleRule::statusAsString))
.setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Status")
.unmarshallLocationName("Status").build(), RequiredTrait.create()).build();
private static final SdkField> TRANSITIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Transitions")
.getter(getter(LifecycleRule::transitions))
.setter(setter(Builder::transitions))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Transition")
.unmarshallLocationName("Transition").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Transition::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").unmarshallLocationName("member").build()).build())
.isFlattened(true).build()).build();
private static final SdkField> NONCURRENT_VERSION_TRANSITIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("NoncurrentVersionTransitions")
.getter(getter(LifecycleRule::noncurrentVersionTransitions))
.setter(setter(Builder::noncurrentVersionTransitions))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NoncurrentVersionTransition")
.unmarshallLocationName("NoncurrentVersionTransition").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(NoncurrentVersionTransition::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").unmarshallLocationName("member").build()).build())
.isFlattened(true).build()).build();
private static final SdkField NONCURRENT_VERSION_EXPIRATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("NoncurrentVersionExpiration")
.getter(getter(LifecycleRule::noncurrentVersionExpiration))
.setter(setter(Builder::noncurrentVersionExpiration))
.constructor(NoncurrentVersionExpiration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NoncurrentVersionExpiration")
.unmarshallLocationName("NoncurrentVersionExpiration").build()).build();
private static final SdkField ABORT_INCOMPLETE_MULTIPART_UPLOAD_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("AbortIncompleteMultipartUpload")
.getter(getter(LifecycleRule::abortIncompleteMultipartUpload))
.setter(setter(Builder::abortIncompleteMultipartUpload))
.constructor(AbortIncompleteMultipartUpload::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AbortIncompleteMultipartUpload")
.unmarshallLocationName("AbortIncompleteMultipartUpload").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(EXPIRATION_FIELD, ID_FIELD,
PREFIX_FIELD, FILTER_FIELD, STATUS_FIELD, TRANSITIONS_FIELD, NONCURRENT_VERSION_TRANSITIONS_FIELD,
NONCURRENT_VERSION_EXPIRATION_FIELD, ABORT_INCOMPLETE_MULTIPART_UPLOAD_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private static final long serialVersionUID = 1L;
private final LifecycleExpiration expiration;
private final String id;
private final String prefix;
private final LifecycleRuleFilter filter;
private final String status;
private final List transitions;
private final List noncurrentVersionTransitions;
private final NoncurrentVersionExpiration noncurrentVersionExpiration;
private final AbortIncompleteMultipartUpload abortIncompleteMultipartUpload;
private LifecycleRule(BuilderImpl builder) {
this.expiration = builder.expiration;
this.id = builder.id;
this.prefix = builder.prefix;
this.filter = builder.filter;
this.status = builder.status;
this.transitions = builder.transitions;
this.noncurrentVersionTransitions = builder.noncurrentVersionTransitions;
this.noncurrentVersionExpiration = builder.noncurrentVersionExpiration;
this.abortIncompleteMultipartUpload = builder.abortIncompleteMultipartUpload;
}
/**
*
* Specifies the expiration for the lifecycle of the object in the form of date, days and, whether the object has a
* delete marker.
*
*
* @return Specifies the expiration for the lifecycle of the object in the form of date, days and, whether the
* object has a delete marker.
*/
public final LifecycleExpiration expiration() {
return expiration;
}
/**
*
* Unique identifier for the rule. The value cannot be longer than 255 characters.
*
*
* @return Unique identifier for the rule. The value cannot be longer than 255 characters.
*/
public final String id() {
return id;
}
/**
*
* Prefix identifying one or more objects to which the rule applies. This is no longer used; use Filter
* instead.
*
*
*
* Replacement must be made for object keys containing special characters (such as carriage returns) when using XML
* requests. For more information, see XML
* related object key constraints.
*
*
*
* @return Prefix identifying one or more objects to which the rule applies. This is no longer used; use
* Filter
instead.
*
* Replacement must be made for object keys containing special characters (such as carriage returns) when
* using XML requests. For more information, see XML related object key constraints.
*
* @deprecated
*/
@Deprecated
public final String prefix() {
return prefix;
}
/**
*
* The Filter
is used to identify objects that a Lifecycle Rule applies to. A Filter
must
* have exactly one of Prefix
, Tag
, or And
specified. Filter
is
* required if the LifecycleRule
does not contain a Prefix
element.
*
*
*
* Tag
filters are not supported for directory buckets.
*
*
*
* @return The Filter
is used to identify objects that a Lifecycle Rule applies to. A
* Filter
must have exactly one of Prefix
, Tag
, or And
* specified. Filter
is required if the LifecycleRule
does not contain a
* Prefix
element.
*
* Tag
filters are not supported for directory buckets.
*
*/
public final LifecycleRuleFilter filter() {
return filter;
}
/**
*
* If 'Enabled', the rule is currently being applied. If 'Disabled', the rule is not currently being applied.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link ExpirationStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return If 'Enabled', the rule is currently being applied. If 'Disabled', the rule is not currently being
* applied.
* @see ExpirationStatus
*/
public final ExpirationStatus status() {
return ExpirationStatus.fromValue(status);
}
/**
*
* If 'Enabled', the rule is currently being applied. If 'Disabled', the rule is not currently being applied.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link ExpirationStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return If 'Enabled', the rule is currently being applied. If 'Disabled', the rule is not currently being
* applied.
* @see ExpirationStatus
*/
public final String statusAsString() {
return status;
}
/**
* For responses, this returns true if the service returned a value for the Transitions property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasTransitions() {
return transitions != null && !(transitions instanceof SdkAutoConstructList);
}
/**
*
* Specifies when an Amazon S3 object transitions to a specified storage class.
*
*
*
* This parameter applies to general purpose buckets only. It is not supported for directory bucket lifecycle
* configurations.
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasTransitions} method.
*
*
* @return Specifies when an Amazon S3 object transitions to a specified storage class.
*
* This parameter applies to general purpose buckets only. It is not supported for directory bucket
* lifecycle configurations.
*
*/
public final List transitions() {
return transitions;
}
/**
* For responses, this returns true if the service returned a value for the NoncurrentVersionTransitions property.
* This DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasNoncurrentVersionTransitions() {
return noncurrentVersionTransitions != null && !(noncurrentVersionTransitions instanceof SdkAutoConstructList);
}
/**
*
* Specifies the transition rule for the lifecycle rule that describes when noncurrent objects transition to a
* specific storage class. If your bucket is versioning-enabled (or versioning is suspended), you can set this
* action to request that Amazon S3 transition noncurrent object versions to a specific storage class at a set
* period in the object's lifetime.
*
*
*
* This parameter applies to general purpose buckets only. It is not supported for directory bucket lifecycle
* configurations.
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasNoncurrentVersionTransitions} method.
*
*
* @return Specifies the transition rule for the lifecycle rule that describes when noncurrent objects transition to
* a specific storage class. If your bucket is versioning-enabled (or versioning is suspended), you can set
* this action to request that Amazon S3 transition noncurrent object versions to a specific storage class
* at a set period in the object's lifetime.
*
* This parameter applies to general purpose buckets only. It is not supported for directory bucket
* lifecycle configurations.
*
*/
public final List noncurrentVersionTransitions() {
return noncurrentVersionTransitions;
}
/**
* Returns the value of the NoncurrentVersionExpiration property for this object.
*
* @return The value of the NoncurrentVersionExpiration property for this object.
*/
public final NoncurrentVersionExpiration noncurrentVersionExpiration() {
return noncurrentVersionExpiration;
}
/**
* Returns the value of the AbortIncompleteMultipartUpload property for this object.
*
* @return The value of the AbortIncompleteMultipartUpload property for this object.
*/
public final AbortIncompleteMultipartUpload abortIncompleteMultipartUpload() {
return abortIncompleteMultipartUpload;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(expiration());
hashCode = 31 * hashCode + Objects.hashCode(id());
hashCode = 31 * hashCode + Objects.hashCode(prefix());
hashCode = 31 * hashCode + Objects.hashCode(filter());
hashCode = 31 * hashCode + Objects.hashCode(statusAsString());
hashCode = 31 * hashCode + Objects.hashCode(hasTransitions() ? transitions() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasNoncurrentVersionTransitions() ? noncurrentVersionTransitions() : null);
hashCode = 31 * hashCode + Objects.hashCode(noncurrentVersionExpiration());
hashCode = 31 * hashCode + Objects.hashCode(abortIncompleteMultipartUpload());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof LifecycleRule)) {
return false;
}
LifecycleRule other = (LifecycleRule) obj;
return Objects.equals(expiration(), other.expiration()) && Objects.equals(id(), other.id())
&& Objects.equals(prefix(), other.prefix()) && Objects.equals(filter(), other.filter())
&& Objects.equals(statusAsString(), other.statusAsString()) && hasTransitions() == other.hasTransitions()
&& Objects.equals(transitions(), other.transitions())
&& hasNoncurrentVersionTransitions() == other.hasNoncurrentVersionTransitions()
&& Objects.equals(noncurrentVersionTransitions(), other.noncurrentVersionTransitions())
&& Objects.equals(noncurrentVersionExpiration(), other.noncurrentVersionExpiration())
&& Objects.equals(abortIncompleteMultipartUpload(), other.abortIncompleteMultipartUpload());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("LifecycleRule").add("Expiration", expiration()).add("ID", id()).add("Prefix", prefix())
.add("Filter", filter()).add("Status", statusAsString())
.add("Transitions", hasTransitions() ? transitions() : null)
.add("NoncurrentVersionTransitions", hasNoncurrentVersionTransitions() ? noncurrentVersionTransitions() : null)
.add("NoncurrentVersionExpiration", noncurrentVersionExpiration())
.add("AbortIncompleteMultipartUpload", abortIncompleteMultipartUpload()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Expiration":
return Optional.ofNullable(clazz.cast(expiration()));
case "ID":
return Optional.ofNullable(clazz.cast(id()));
case "Prefix":
return Optional.ofNullable(clazz.cast(prefix()));
case "Filter":
return Optional.ofNullable(clazz.cast(filter()));
case "Status":
return Optional.ofNullable(clazz.cast(statusAsString()));
case "Transitions":
return Optional.ofNullable(clazz.cast(transitions()));
case "NoncurrentVersionTransitions":
return Optional.ofNullable(clazz.cast(noncurrentVersionTransitions()));
case "NoncurrentVersionExpiration":
return Optional.ofNullable(clazz.cast(noncurrentVersionExpiration()));
case "AbortIncompleteMultipartUpload":
return Optional.ofNullable(clazz.cast(abortIncompleteMultipartUpload()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("Expiration", EXPIRATION_FIELD);
map.put("ID", ID_FIELD);
map.put("Prefix", PREFIX_FIELD);
map.put("Filter", FILTER_FIELD);
map.put("Status", STATUS_FIELD);
map.put("Transition", TRANSITIONS_FIELD);
map.put("NoncurrentVersionTransition", NONCURRENT_VERSION_TRANSITIONS_FIELD);
map.put("NoncurrentVersionExpiration", NONCURRENT_VERSION_EXPIRATION_FIELD);
map.put("AbortIncompleteMultipartUpload", ABORT_INCOMPLETE_MULTIPART_UPLOAD_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function