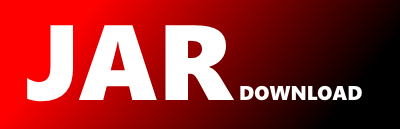
software.amazon.awssdk.services.s3.model.RestoreRequest Maven / Gradle / Ivy
Show all versions of s3 Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.s3.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Container for restore job parameters.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class RestoreRequest implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField DAYS_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("Days")
.getter(getter(RestoreRequest::days))
.setter(setter(Builder::days))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Days")
.unmarshallLocationName("Days").build()).build();
private static final SdkField GLACIER_JOB_PARAMETERS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("GlacierJobParameters")
.getter(getter(RestoreRequest::glacierJobParameters))
.setter(setter(Builder::glacierJobParameters))
.constructor(GlacierJobParameters::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("GlacierJobParameters")
.unmarshallLocationName("GlacierJobParameters").build()).build();
private static final SdkField TYPE_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("Type")
.getter(getter(RestoreRequest::typeAsString))
.setter(setter(Builder::type))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Type")
.unmarshallLocationName("Type").build()).build();
private static final SdkField TIER_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("Tier")
.getter(getter(RestoreRequest::tierAsString))
.setter(setter(Builder::tier))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Tier")
.unmarshallLocationName("Tier").build()).build();
private static final SdkField DESCRIPTION_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("Description")
.getter(getter(RestoreRequest::description))
.setter(setter(Builder::description))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Description")
.unmarshallLocationName("Description").build()).build();
private static final SdkField SELECT_PARAMETERS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("SelectParameters")
.getter(getter(RestoreRequest::selectParameters))
.setter(setter(Builder::selectParameters))
.constructor(SelectParameters::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SelectParameters")
.unmarshallLocationName("SelectParameters").build()).build();
private static final SdkField OUTPUT_LOCATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("OutputLocation")
.getter(getter(RestoreRequest::outputLocation))
.setter(setter(Builder::outputLocation))
.constructor(OutputLocation::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OutputLocation")
.unmarshallLocationName("OutputLocation").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(DAYS_FIELD,
GLACIER_JOB_PARAMETERS_FIELD, TYPE_FIELD, TIER_FIELD, DESCRIPTION_FIELD, SELECT_PARAMETERS_FIELD,
OUTPUT_LOCATION_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private static final long serialVersionUID = 1L;
private final Integer days;
private final GlacierJobParameters glacierJobParameters;
private final String type;
private final String tier;
private final String description;
private final SelectParameters selectParameters;
private final OutputLocation outputLocation;
private RestoreRequest(BuilderImpl builder) {
this.days = builder.days;
this.glacierJobParameters = builder.glacierJobParameters;
this.type = builder.type;
this.tier = builder.tier;
this.description = builder.description;
this.selectParameters = builder.selectParameters;
this.outputLocation = builder.outputLocation;
}
/**
*
* Lifetime of the active copy in days. Do not use with restores that specify OutputLocation
.
*
*
* The Days element is required for regular restores, and must not be provided for select requests.
*
*
* @return Lifetime of the active copy in days. Do not use with restores that specify OutputLocation
* .
*
* The Days element is required for regular restores, and must not be provided for select requests.
*/
public final Integer days() {
return days;
}
/**
*
* S3 Glacier related parameters pertaining to this job. Do not use with restores that specify
* OutputLocation
.
*
*
* @return S3 Glacier related parameters pertaining to this job. Do not use with restores that specify
* OutputLocation
.
*/
public final GlacierJobParameters glacierJobParameters() {
return glacierJobParameters;
}
/**
*
*
* Amazon S3 Select is no longer available to new customers. Existing customers of Amazon S3 Select can continue to
* use the feature as usual. Learn more
*
*
*
* Type of restore request.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #type} will return
* {@link RestoreRequestType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #typeAsString}.
*
*
* @return
* Amazon S3 Select is no longer available to new customers. Existing customers of Amazon S3 Select can
* continue to use the feature as usual. Learn
* more
*
*
*
* Type of restore request.
* @see RestoreRequestType
*/
public final RestoreRequestType type() {
return RestoreRequestType.fromValue(type);
}
/**
*
*
* Amazon S3 Select is no longer available to new customers. Existing customers of Amazon S3 Select can continue to
* use the feature as usual. Learn more
*
*
*
* Type of restore request.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #type} will return
* {@link RestoreRequestType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #typeAsString}.
*
*
* @return
* Amazon S3 Select is no longer available to new customers. Existing customers of Amazon S3 Select can
* continue to use the feature as usual. Learn
* more
*
*
*
* Type of restore request.
* @see RestoreRequestType
*/
public final String typeAsString() {
return type;
}
/**
*
* Retrieval tier at which the restore will be processed.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #tier} will return
* {@link Tier#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #tierAsString}.
*
*
* @return Retrieval tier at which the restore will be processed.
* @see Tier
*/
public final Tier tier() {
return Tier.fromValue(tier);
}
/**
*
* Retrieval tier at which the restore will be processed.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #tier} will return
* {@link Tier#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #tierAsString}.
*
*
* @return Retrieval tier at which the restore will be processed.
* @see Tier
*/
public final String tierAsString() {
return tier;
}
/**
*
* The optional description for the job.
*
*
* @return The optional description for the job.
*/
public final String description() {
return description;
}
/**
*
*
* Amazon S3 Select is no longer available to new customers. Existing customers of Amazon S3 Select can continue to
* use the feature as usual. Learn more
*
*
*
* Describes the parameters for Select job types.
*
*
* @return
* Amazon S3 Select is no longer available to new customers. Existing customers of Amazon S3 Select can
* continue to use the feature as usual. Learn
* more
*
*
*
* Describes the parameters for Select job types.
*/
public final SelectParameters selectParameters() {
return selectParameters;
}
/**
*
* Describes the location where the restore job's output is stored.
*
*
* @return Describes the location where the restore job's output is stored.
*/
public final OutputLocation outputLocation() {
return outputLocation;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(days());
hashCode = 31 * hashCode + Objects.hashCode(glacierJobParameters());
hashCode = 31 * hashCode + Objects.hashCode(typeAsString());
hashCode = 31 * hashCode + Objects.hashCode(tierAsString());
hashCode = 31 * hashCode + Objects.hashCode(description());
hashCode = 31 * hashCode + Objects.hashCode(selectParameters());
hashCode = 31 * hashCode + Objects.hashCode(outputLocation());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof RestoreRequest)) {
return false;
}
RestoreRequest other = (RestoreRequest) obj;
return Objects.equals(days(), other.days()) && Objects.equals(glacierJobParameters(), other.glacierJobParameters())
&& Objects.equals(typeAsString(), other.typeAsString()) && Objects.equals(tierAsString(), other.tierAsString())
&& Objects.equals(description(), other.description())
&& Objects.equals(selectParameters(), other.selectParameters())
&& Objects.equals(outputLocation(), other.outputLocation());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("RestoreRequest").add("Days", days()).add("GlacierJobParameters", glacierJobParameters())
.add("Type", typeAsString()).add("Tier", tierAsString()).add("Description", description())
.add("SelectParameters", selectParameters()).add("OutputLocation", outputLocation()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Days":
return Optional.ofNullable(clazz.cast(days()));
case "GlacierJobParameters":
return Optional.ofNullable(clazz.cast(glacierJobParameters()));
case "Type":
return Optional.ofNullable(clazz.cast(typeAsString()));
case "Tier":
return Optional.ofNullable(clazz.cast(tierAsString()));
case "Description":
return Optional.ofNullable(clazz.cast(description()));
case "SelectParameters":
return Optional.ofNullable(clazz.cast(selectParameters()));
case "OutputLocation":
return Optional.ofNullable(clazz.cast(outputLocation()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("Days", DAYS_FIELD);
map.put("GlacierJobParameters", GLACIER_JOB_PARAMETERS_FIELD);
map.put("Type", TYPE_FIELD);
map.put("Tier", TIER_FIELD);
map.put("Description", DESCRIPTION_FIELD);
map.put("SelectParameters", SELECT_PARAMETERS_FIELD);
map.put("OutputLocation", OUTPUT_LOCATION_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function