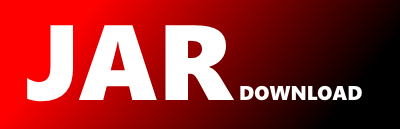
software.amazon.awssdk.services.s3.model.SelectObjectContentRequest Maven / Gradle / Ivy
Show all versions of s3 Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.s3.model;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.traits.RequiredTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
*
* Learn Amazon S3 Select is no longer available to new customers. Existing customers of Amazon S3 Select can continue
* to use the feature as usual. Learn more
*
*
*
* Request to filter the contents of an Amazon S3 object based on a simple Structured Query Language (SQL) statement. In
* the request, along with the SQL expression, you must specify a data serialization format (JSON or CSV) of the object.
* Amazon S3 uses this to parse object data into records. It returns only records that match the specified SQL
* expression. You must also specify the data serialization format for the response. For more information, see S3Select API Documentation.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class SelectObjectContentRequest extends S3Request implements
ToCopyableBuilder {
private static final SdkField BUCKET_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("Bucket")
.getter(getter(SelectObjectContentRequest::bucket))
.setter(setter(Builder::bucket))
.traits(LocationTrait.builder().location(MarshallLocation.PATH).locationName("Bucket")
.unmarshallLocationName("Bucket").build(), RequiredTrait.create()).build();
private static final SdkField KEY_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("Key")
.getter(getter(SelectObjectContentRequest::key))
.setter(setter(Builder::key))
.traits(LocationTrait.builder().location(MarshallLocation.GREEDY_PATH).locationName("Key")
.unmarshallLocationName("Key").build(), RequiredTrait.create()).build();
private static final SdkField SSE_CUSTOMER_ALGORITHM_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("SSECustomerAlgorithm")
.getter(getter(SelectObjectContentRequest::sseCustomerAlgorithm))
.setter(setter(Builder::sseCustomerAlgorithm))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER)
.locationName("x-amz-server-side-encryption-customer-algorithm")
.unmarshallLocationName("x-amz-server-side-encryption-customer-algorithm").build()).build();
private static final SdkField SSE_CUSTOMER_KEY_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("SSECustomerKey")
.getter(getter(SelectObjectContentRequest::sseCustomerKey))
.setter(setter(Builder::sseCustomerKey))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER)
.locationName("x-amz-server-side-encryption-customer-key")
.unmarshallLocationName("x-amz-server-side-encryption-customer-key").build()).build();
private static final SdkField SSE_CUSTOMER_KEY_MD5_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("SSECustomerKeyMD5")
.getter(getter(SelectObjectContentRequest::sseCustomerKeyMD5))
.setter(setter(Builder::sseCustomerKeyMD5))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER)
.locationName("x-amz-server-side-encryption-customer-key-MD5")
.unmarshallLocationName("x-amz-server-side-encryption-customer-key-MD5").build()).build();
private static final SdkField EXPRESSION_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("Expression")
.getter(getter(SelectObjectContentRequest::expression))
.setter(setter(Builder::expression))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Expression")
.unmarshallLocationName("Expression").build(), RequiredTrait.create()).build();
private static final SdkField EXPRESSION_TYPE_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("ExpressionType")
.getter(getter(SelectObjectContentRequest::expressionTypeAsString))
.setter(setter(Builder::expressionType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ExpressionType")
.unmarshallLocationName("ExpressionType").build(), RequiredTrait.create()).build();
private static final SdkField REQUEST_PROGRESS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("RequestProgress")
.getter(getter(SelectObjectContentRequest::requestProgress))
.setter(setter(Builder::requestProgress))
.constructor(RequestProgress::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RequestProgress")
.unmarshallLocationName("RequestProgress").build()).build();
private static final SdkField INPUT_SERIALIZATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("InputSerialization")
.getter(getter(SelectObjectContentRequest::inputSerialization))
.setter(setter(Builder::inputSerialization))
.constructor(InputSerialization::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InputSerialization")
.unmarshallLocationName("InputSerialization").build(), RequiredTrait.create()).build();
private static final SdkField OUTPUT_SERIALIZATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("OutputSerialization")
.getter(getter(SelectObjectContentRequest::outputSerialization))
.setter(setter(Builder::outputSerialization))
.constructor(OutputSerialization::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OutputSerialization")
.unmarshallLocationName("OutputSerialization").build(), RequiredTrait.create()).build();
private static final SdkField SCAN_RANGE_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("ScanRange")
.getter(getter(SelectObjectContentRequest::scanRange))
.setter(setter(Builder::scanRange))
.constructor(ScanRange::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ScanRange")
.unmarshallLocationName("ScanRange").build()).build();
private static final SdkField EXPECTED_BUCKET_OWNER_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("ExpectedBucketOwner")
.getter(getter(SelectObjectContentRequest::expectedBucketOwner))
.setter(setter(Builder::expectedBucketOwner))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("x-amz-expected-bucket-owner")
.unmarshallLocationName("x-amz-expected-bucket-owner").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(BUCKET_FIELD, KEY_FIELD,
SSE_CUSTOMER_ALGORITHM_FIELD, SSE_CUSTOMER_KEY_FIELD, SSE_CUSTOMER_KEY_MD5_FIELD, EXPRESSION_FIELD,
EXPRESSION_TYPE_FIELD, REQUEST_PROGRESS_FIELD, INPUT_SERIALIZATION_FIELD, OUTPUT_SERIALIZATION_FIELD,
SCAN_RANGE_FIELD, EXPECTED_BUCKET_OWNER_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private final String bucket;
private final String key;
private final String sseCustomerAlgorithm;
private final String sseCustomerKey;
private final String sseCustomerKeyMD5;
private final String expression;
private final String expressionType;
private final RequestProgress requestProgress;
private final InputSerialization inputSerialization;
private final OutputSerialization outputSerialization;
private final ScanRange scanRange;
private final String expectedBucketOwner;
private SelectObjectContentRequest(BuilderImpl builder) {
super(builder);
this.bucket = builder.bucket;
this.key = builder.key;
this.sseCustomerAlgorithm = builder.sseCustomerAlgorithm;
this.sseCustomerKey = builder.sseCustomerKey;
this.sseCustomerKeyMD5 = builder.sseCustomerKeyMD5;
this.expression = builder.expression;
this.expressionType = builder.expressionType;
this.requestProgress = builder.requestProgress;
this.inputSerialization = builder.inputSerialization;
this.outputSerialization = builder.outputSerialization;
this.scanRange = builder.scanRange;
this.expectedBucketOwner = builder.expectedBucketOwner;
}
/**
*
* The S3 bucket.
*
*
* @return The S3 bucket.
*/
public final String bucket() {
return bucket;
}
/**
*
* The object key.
*
*
* @return The object key.
*/
public final String key() {
return key;
}
/**
*
* The server-side encryption (SSE) algorithm used to encrypt the object. This parameter is needed only when the
* object was created using a checksum algorithm. For more information, see Protecting data
* using SSE-C keys in the Amazon S3 User Guide.
*
*
* @return The server-side encryption (SSE) algorithm used to encrypt the object. This parameter is needed only when
* the object was created using a checksum algorithm. For more information, see Protecting
* data using SSE-C keys in the Amazon S3 User Guide.
*/
public final String sseCustomerAlgorithm() {
return sseCustomerAlgorithm;
}
/**
*
* The server-side encryption (SSE) customer managed key. This parameter is needed only when the object was created
* using a checksum algorithm. For more information, see Protecting data
* using SSE-C keys in the Amazon S3 User Guide.
*
*
* @return The server-side encryption (SSE) customer managed key. This parameter is needed only when the object was
* created using a checksum algorithm. For more information, see Protecting
* data using SSE-C keys in the Amazon S3 User Guide.
*/
public final String sseCustomerKey() {
return sseCustomerKey;
}
/**
*
* The MD5 server-side encryption (SSE) customer managed key. This parameter is needed only when the object was
* created using a checksum algorithm. For more information, see Protecting data
* using SSE-C keys in the Amazon S3 User Guide.
*
*
* @return The MD5 server-side encryption (SSE) customer managed key. This parameter is needed only when the object
* was created using a checksum algorithm. For more information, see Protecting
* data using SSE-C keys in the Amazon S3 User Guide.
*/
public final String sseCustomerKeyMD5() {
return sseCustomerKeyMD5;
}
/**
*
* The expression that is used to query the object.
*
*
* @return The expression that is used to query the object.
*/
public final String expression() {
return expression;
}
/**
*
* The type of the provided expression (for example, SQL).
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #expressionType}
* will return {@link ExpressionType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #expressionTypeAsString}.
*
*
* @return The type of the provided expression (for example, SQL).
* @see ExpressionType
*/
public final ExpressionType expressionType() {
return ExpressionType.fromValue(expressionType);
}
/**
*
* The type of the provided expression (for example, SQL).
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #expressionType}
* will return {@link ExpressionType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #expressionTypeAsString}.
*
*
* @return The type of the provided expression (for example, SQL).
* @see ExpressionType
*/
public final String expressionTypeAsString() {
return expressionType;
}
/**
*
* Specifies if periodic request progress information should be enabled.
*
*
* @return Specifies if periodic request progress information should be enabled.
*/
public final RequestProgress requestProgress() {
return requestProgress;
}
/**
*
* Describes the format of the data in the object that is being queried.
*
*
* @return Describes the format of the data in the object that is being queried.
*/
public final InputSerialization inputSerialization() {
return inputSerialization;
}
/**
*
* Describes the format of the data that you want Amazon S3 to return in response.
*
*
* @return Describes the format of the data that you want Amazon S3 to return in response.
*/
public final OutputSerialization outputSerialization() {
return outputSerialization;
}
/**
*
* Specifies the byte range of the object to get the records from. A record is processed when its first byte is
* contained by the range. This parameter is optional, but when specified, it must not be empty. See RFC 2616,
* Section 14.35.1 about how to specify the start and end of the range.
*
*
* ScanRange
may be used in the following ways:
*
*
* -
*
* <scanrange><start>50</start><end>100</end></scanrange>
- process
* only the records starting between the bytes 50 and 100 (inclusive, counting from zero)
*
*
* -
*
* <scanrange><start>50</start></scanrange>
- process only the records starting
* after the byte 50
*
*
* -
*
* <scanrange><end>50</end></scanrange>
- process only the records within the
* last 50 bytes of the file.
*
*
*
*
* @return Specifies the byte range of the object to get the records from. A record is processed when its first byte
* is contained by the range. This parameter is optional, but when specified, it must not be empty. See RFC
* 2616, Section 14.35.1 about how to specify the start and end of the range.
*
* ScanRange
may be used in the following ways:
*
*
* -
*
* <scanrange><start>50</start><end>100</end></scanrange>
-
* process only the records starting between the bytes 50 and 100 (inclusive, counting from zero)
*
*
* -
*
* <scanrange><start>50</start></scanrange>
- process only the records
* starting after the byte 50
*
*
* -
*
* <scanrange><end>50</end></scanrange>
- process only the records
* within the last 50 bytes of the file.
*
*
*/
public final ScanRange scanRange() {
return scanRange;
}
/**
*
* The account ID of the expected bucket owner. If the account ID that you provide does not match the actual owner
* of the bucket, the request fails with the HTTP status code 403 Forbidden
(access denied).
*
*
* @return The account ID of the expected bucket owner. If the account ID that you provide does not match the actual
* owner of the bucket, the request fails with the HTTP status code 403 Forbidden
(access
* denied).
*/
public final String expectedBucketOwner() {
return expectedBucketOwner;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(bucket());
hashCode = 31 * hashCode + Objects.hashCode(key());
hashCode = 31 * hashCode + Objects.hashCode(sseCustomerAlgorithm());
hashCode = 31 * hashCode + Objects.hashCode(sseCustomerKey());
hashCode = 31 * hashCode + Objects.hashCode(sseCustomerKeyMD5());
hashCode = 31 * hashCode + Objects.hashCode(expression());
hashCode = 31 * hashCode + Objects.hashCode(expressionTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(requestProgress());
hashCode = 31 * hashCode + Objects.hashCode(inputSerialization());
hashCode = 31 * hashCode + Objects.hashCode(outputSerialization());
hashCode = 31 * hashCode + Objects.hashCode(scanRange());
hashCode = 31 * hashCode + Objects.hashCode(expectedBucketOwner());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof SelectObjectContentRequest)) {
return false;
}
SelectObjectContentRequest other = (SelectObjectContentRequest) obj;
return Objects.equals(bucket(), other.bucket()) && Objects.equals(key(), other.key())
&& Objects.equals(sseCustomerAlgorithm(), other.sseCustomerAlgorithm())
&& Objects.equals(sseCustomerKey(), other.sseCustomerKey())
&& Objects.equals(sseCustomerKeyMD5(), other.sseCustomerKeyMD5())
&& Objects.equals(expression(), other.expression())
&& Objects.equals(expressionTypeAsString(), other.expressionTypeAsString())
&& Objects.equals(requestProgress(), other.requestProgress())
&& Objects.equals(inputSerialization(), other.inputSerialization())
&& Objects.equals(outputSerialization(), other.outputSerialization())
&& Objects.equals(scanRange(), other.scanRange())
&& Objects.equals(expectedBucketOwner(), other.expectedBucketOwner());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("SelectObjectContentRequest").add("Bucket", bucket()).add("Key", key())
.add("SSECustomerAlgorithm", sseCustomerAlgorithm())
.add("SSECustomerKey", sseCustomerKey() == null ? null : "*** Sensitive Data Redacted ***")
.add("SSECustomerKeyMD5", sseCustomerKeyMD5()).add("Expression", expression())
.add("ExpressionType", expressionTypeAsString()).add("RequestProgress", requestProgress())
.add("InputSerialization", inputSerialization()).add("OutputSerialization", outputSerialization())
.add("ScanRange", scanRange()).add("ExpectedBucketOwner", expectedBucketOwner()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Bucket":
return Optional.ofNullable(clazz.cast(bucket()));
case "Key":
return Optional.ofNullable(clazz.cast(key()));
case "SSECustomerAlgorithm":
return Optional.ofNullable(clazz.cast(sseCustomerAlgorithm()));
case "SSECustomerKey":
return Optional.ofNullable(clazz.cast(sseCustomerKey()));
case "SSECustomerKeyMD5":
return Optional.ofNullable(clazz.cast(sseCustomerKeyMD5()));
case "Expression":
return Optional.ofNullable(clazz.cast(expression()));
case "ExpressionType":
return Optional.ofNullable(clazz.cast(expressionTypeAsString()));
case "RequestProgress":
return Optional.ofNullable(clazz.cast(requestProgress()));
case "InputSerialization":
return Optional.ofNullable(clazz.cast(inputSerialization()));
case "OutputSerialization":
return Optional.ofNullable(clazz.cast(outputSerialization()));
case "ScanRange":
return Optional.ofNullable(clazz.cast(scanRange()));
case "ExpectedBucketOwner":
return Optional.ofNullable(clazz.cast(expectedBucketOwner()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("Bucket", BUCKET_FIELD);
map.put("Key", KEY_FIELD);
map.put("x-amz-server-side-encryption-customer-algorithm", SSE_CUSTOMER_ALGORITHM_FIELD);
map.put("x-amz-server-side-encryption-customer-key", SSE_CUSTOMER_KEY_FIELD);
map.put("x-amz-server-side-encryption-customer-key-MD5", SSE_CUSTOMER_KEY_MD5_FIELD);
map.put("Expression", EXPRESSION_FIELD);
map.put("ExpressionType", EXPRESSION_TYPE_FIELD);
map.put("RequestProgress", REQUEST_PROGRESS_FIELD);
map.put("InputSerialization", INPUT_SERIALIZATION_FIELD);
map.put("OutputSerialization", OUTPUT_SERIALIZATION_FIELD);
map.put("ScanRange", SCAN_RANGE_FIELD);
map.put("x-amz-expected-bucket-owner", EXPECTED_BUCKET_OWNER_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function