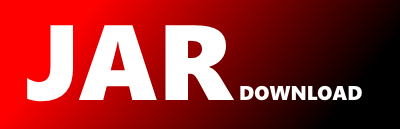
software.amazon.awssdk.services.s3.DefaultS3AsyncClient Maven / Gradle / Ivy
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.s3;
import static software.amazon.awssdk.utils.FunctionalUtils.runAndLogError;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.awscore.client.handler.AwsAsyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.ApiName;
import software.amazon.awssdk.core.async.AsyncRequestBody;
import software.amazon.awssdk.core.async.AsyncResponseTransformer;
import software.amazon.awssdk.core.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.client.handler.AsyncClientHandler;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.util.VersionInfo;
import software.amazon.awssdk.protocols.core.ExceptionMetadata;
import software.amazon.awssdk.protocols.xml.AwsS3ProtocolFactory;
import software.amazon.awssdk.protocols.xml.XmlOperationMetadata;
import software.amazon.awssdk.services.s3.model.AbortMultipartUploadRequest;
import software.amazon.awssdk.services.s3.model.AbortMultipartUploadResponse;
import software.amazon.awssdk.services.s3.model.BucketAlreadyExistsException;
import software.amazon.awssdk.services.s3.model.BucketAlreadyOwnedByYouException;
import software.amazon.awssdk.services.s3.model.CompleteMultipartUploadRequest;
import software.amazon.awssdk.services.s3.model.CompleteMultipartUploadResponse;
import software.amazon.awssdk.services.s3.model.CopyObjectRequest;
import software.amazon.awssdk.services.s3.model.CopyObjectResponse;
import software.amazon.awssdk.services.s3.model.CreateBucketRequest;
import software.amazon.awssdk.services.s3.model.CreateBucketResponse;
import software.amazon.awssdk.services.s3.model.CreateMultipartUploadRequest;
import software.amazon.awssdk.services.s3.model.CreateMultipartUploadResponse;
import software.amazon.awssdk.services.s3.model.DeleteBucketAnalyticsConfigurationRequest;
import software.amazon.awssdk.services.s3.model.DeleteBucketAnalyticsConfigurationResponse;
import software.amazon.awssdk.services.s3.model.DeleteBucketCorsRequest;
import software.amazon.awssdk.services.s3.model.DeleteBucketCorsResponse;
import software.amazon.awssdk.services.s3.model.DeleteBucketEncryptionRequest;
import software.amazon.awssdk.services.s3.model.DeleteBucketEncryptionResponse;
import software.amazon.awssdk.services.s3.model.DeleteBucketInventoryConfigurationRequest;
import software.amazon.awssdk.services.s3.model.DeleteBucketInventoryConfigurationResponse;
import software.amazon.awssdk.services.s3.model.DeleteBucketLifecycleRequest;
import software.amazon.awssdk.services.s3.model.DeleteBucketLifecycleResponse;
import software.amazon.awssdk.services.s3.model.DeleteBucketMetricsConfigurationRequest;
import software.amazon.awssdk.services.s3.model.DeleteBucketMetricsConfigurationResponse;
import software.amazon.awssdk.services.s3.model.DeleteBucketPolicyRequest;
import software.amazon.awssdk.services.s3.model.DeleteBucketPolicyResponse;
import software.amazon.awssdk.services.s3.model.DeleteBucketReplicationRequest;
import software.amazon.awssdk.services.s3.model.DeleteBucketReplicationResponse;
import software.amazon.awssdk.services.s3.model.DeleteBucketRequest;
import software.amazon.awssdk.services.s3.model.DeleteBucketResponse;
import software.amazon.awssdk.services.s3.model.DeleteBucketTaggingRequest;
import software.amazon.awssdk.services.s3.model.DeleteBucketTaggingResponse;
import software.amazon.awssdk.services.s3.model.DeleteBucketWebsiteRequest;
import software.amazon.awssdk.services.s3.model.DeleteBucketWebsiteResponse;
import software.amazon.awssdk.services.s3.model.DeleteObjectRequest;
import software.amazon.awssdk.services.s3.model.DeleteObjectResponse;
import software.amazon.awssdk.services.s3.model.DeleteObjectTaggingRequest;
import software.amazon.awssdk.services.s3.model.DeleteObjectTaggingResponse;
import software.amazon.awssdk.services.s3.model.DeleteObjectsRequest;
import software.amazon.awssdk.services.s3.model.DeleteObjectsResponse;
import software.amazon.awssdk.services.s3.model.DeletePublicAccessBlockRequest;
import software.amazon.awssdk.services.s3.model.DeletePublicAccessBlockResponse;
import software.amazon.awssdk.services.s3.model.GetBucketAccelerateConfigurationRequest;
import software.amazon.awssdk.services.s3.model.GetBucketAccelerateConfigurationResponse;
import software.amazon.awssdk.services.s3.model.GetBucketAclRequest;
import software.amazon.awssdk.services.s3.model.GetBucketAclResponse;
import software.amazon.awssdk.services.s3.model.GetBucketAnalyticsConfigurationRequest;
import software.amazon.awssdk.services.s3.model.GetBucketAnalyticsConfigurationResponse;
import software.amazon.awssdk.services.s3.model.GetBucketCorsRequest;
import software.amazon.awssdk.services.s3.model.GetBucketCorsResponse;
import software.amazon.awssdk.services.s3.model.GetBucketEncryptionRequest;
import software.amazon.awssdk.services.s3.model.GetBucketEncryptionResponse;
import software.amazon.awssdk.services.s3.model.GetBucketInventoryConfigurationRequest;
import software.amazon.awssdk.services.s3.model.GetBucketInventoryConfigurationResponse;
import software.amazon.awssdk.services.s3.model.GetBucketLifecycleConfigurationRequest;
import software.amazon.awssdk.services.s3.model.GetBucketLifecycleConfigurationResponse;
import software.amazon.awssdk.services.s3.model.GetBucketLocationRequest;
import software.amazon.awssdk.services.s3.model.GetBucketLocationResponse;
import software.amazon.awssdk.services.s3.model.GetBucketLoggingRequest;
import software.amazon.awssdk.services.s3.model.GetBucketLoggingResponse;
import software.amazon.awssdk.services.s3.model.GetBucketMetricsConfigurationRequest;
import software.amazon.awssdk.services.s3.model.GetBucketMetricsConfigurationResponse;
import software.amazon.awssdk.services.s3.model.GetBucketNotificationConfigurationRequest;
import software.amazon.awssdk.services.s3.model.GetBucketNotificationConfigurationResponse;
import software.amazon.awssdk.services.s3.model.GetBucketPolicyRequest;
import software.amazon.awssdk.services.s3.model.GetBucketPolicyResponse;
import software.amazon.awssdk.services.s3.model.GetBucketPolicyStatusRequest;
import software.amazon.awssdk.services.s3.model.GetBucketPolicyStatusResponse;
import software.amazon.awssdk.services.s3.model.GetBucketReplicationRequest;
import software.amazon.awssdk.services.s3.model.GetBucketReplicationResponse;
import software.amazon.awssdk.services.s3.model.GetBucketRequestPaymentRequest;
import software.amazon.awssdk.services.s3.model.GetBucketRequestPaymentResponse;
import software.amazon.awssdk.services.s3.model.GetBucketTaggingRequest;
import software.amazon.awssdk.services.s3.model.GetBucketTaggingResponse;
import software.amazon.awssdk.services.s3.model.GetBucketVersioningRequest;
import software.amazon.awssdk.services.s3.model.GetBucketVersioningResponse;
import software.amazon.awssdk.services.s3.model.GetBucketWebsiteRequest;
import software.amazon.awssdk.services.s3.model.GetBucketWebsiteResponse;
import software.amazon.awssdk.services.s3.model.GetObjectAclRequest;
import software.amazon.awssdk.services.s3.model.GetObjectAclResponse;
import software.amazon.awssdk.services.s3.model.GetObjectLegalHoldRequest;
import software.amazon.awssdk.services.s3.model.GetObjectLegalHoldResponse;
import software.amazon.awssdk.services.s3.model.GetObjectLockConfigurationRequest;
import software.amazon.awssdk.services.s3.model.GetObjectLockConfigurationResponse;
import software.amazon.awssdk.services.s3.model.GetObjectRequest;
import software.amazon.awssdk.services.s3.model.GetObjectResponse;
import software.amazon.awssdk.services.s3.model.GetObjectRetentionRequest;
import software.amazon.awssdk.services.s3.model.GetObjectRetentionResponse;
import software.amazon.awssdk.services.s3.model.GetObjectTaggingRequest;
import software.amazon.awssdk.services.s3.model.GetObjectTaggingResponse;
import software.amazon.awssdk.services.s3.model.GetObjectTorrentRequest;
import software.amazon.awssdk.services.s3.model.GetObjectTorrentResponse;
import software.amazon.awssdk.services.s3.model.GetPublicAccessBlockRequest;
import software.amazon.awssdk.services.s3.model.GetPublicAccessBlockResponse;
import software.amazon.awssdk.services.s3.model.HeadBucketRequest;
import software.amazon.awssdk.services.s3.model.HeadBucketResponse;
import software.amazon.awssdk.services.s3.model.HeadObjectRequest;
import software.amazon.awssdk.services.s3.model.HeadObjectResponse;
import software.amazon.awssdk.services.s3.model.ListBucketAnalyticsConfigurationsRequest;
import software.amazon.awssdk.services.s3.model.ListBucketAnalyticsConfigurationsResponse;
import software.amazon.awssdk.services.s3.model.ListBucketInventoryConfigurationsRequest;
import software.amazon.awssdk.services.s3.model.ListBucketInventoryConfigurationsResponse;
import software.amazon.awssdk.services.s3.model.ListBucketMetricsConfigurationsRequest;
import software.amazon.awssdk.services.s3.model.ListBucketMetricsConfigurationsResponse;
import software.amazon.awssdk.services.s3.model.ListBucketsRequest;
import software.amazon.awssdk.services.s3.model.ListBucketsResponse;
import software.amazon.awssdk.services.s3.model.ListMultipartUploadsRequest;
import software.amazon.awssdk.services.s3.model.ListMultipartUploadsResponse;
import software.amazon.awssdk.services.s3.model.ListObjectVersionsRequest;
import software.amazon.awssdk.services.s3.model.ListObjectVersionsResponse;
import software.amazon.awssdk.services.s3.model.ListObjectsRequest;
import software.amazon.awssdk.services.s3.model.ListObjectsResponse;
import software.amazon.awssdk.services.s3.model.ListObjectsV2Request;
import software.amazon.awssdk.services.s3.model.ListObjectsV2Response;
import software.amazon.awssdk.services.s3.model.ListPartsRequest;
import software.amazon.awssdk.services.s3.model.ListPartsResponse;
import software.amazon.awssdk.services.s3.model.NoSuchBucketException;
import software.amazon.awssdk.services.s3.model.NoSuchKeyException;
import software.amazon.awssdk.services.s3.model.NoSuchUploadException;
import software.amazon.awssdk.services.s3.model.ObjectAlreadyInActiveTierErrorException;
import software.amazon.awssdk.services.s3.model.ObjectNotInActiveTierErrorException;
import software.amazon.awssdk.services.s3.model.PutBucketAccelerateConfigurationRequest;
import software.amazon.awssdk.services.s3.model.PutBucketAccelerateConfigurationResponse;
import software.amazon.awssdk.services.s3.model.PutBucketAclRequest;
import software.amazon.awssdk.services.s3.model.PutBucketAclResponse;
import software.amazon.awssdk.services.s3.model.PutBucketAnalyticsConfigurationRequest;
import software.amazon.awssdk.services.s3.model.PutBucketAnalyticsConfigurationResponse;
import software.amazon.awssdk.services.s3.model.PutBucketCorsRequest;
import software.amazon.awssdk.services.s3.model.PutBucketCorsResponse;
import software.amazon.awssdk.services.s3.model.PutBucketEncryptionRequest;
import software.amazon.awssdk.services.s3.model.PutBucketEncryptionResponse;
import software.amazon.awssdk.services.s3.model.PutBucketInventoryConfigurationRequest;
import software.amazon.awssdk.services.s3.model.PutBucketInventoryConfigurationResponse;
import software.amazon.awssdk.services.s3.model.PutBucketLifecycleConfigurationRequest;
import software.amazon.awssdk.services.s3.model.PutBucketLifecycleConfigurationResponse;
import software.amazon.awssdk.services.s3.model.PutBucketLoggingRequest;
import software.amazon.awssdk.services.s3.model.PutBucketLoggingResponse;
import software.amazon.awssdk.services.s3.model.PutBucketMetricsConfigurationRequest;
import software.amazon.awssdk.services.s3.model.PutBucketMetricsConfigurationResponse;
import software.amazon.awssdk.services.s3.model.PutBucketNotificationConfigurationRequest;
import software.amazon.awssdk.services.s3.model.PutBucketNotificationConfigurationResponse;
import software.amazon.awssdk.services.s3.model.PutBucketPolicyRequest;
import software.amazon.awssdk.services.s3.model.PutBucketPolicyResponse;
import software.amazon.awssdk.services.s3.model.PutBucketReplicationRequest;
import software.amazon.awssdk.services.s3.model.PutBucketReplicationResponse;
import software.amazon.awssdk.services.s3.model.PutBucketRequestPaymentRequest;
import software.amazon.awssdk.services.s3.model.PutBucketRequestPaymentResponse;
import software.amazon.awssdk.services.s3.model.PutBucketTaggingRequest;
import software.amazon.awssdk.services.s3.model.PutBucketTaggingResponse;
import software.amazon.awssdk.services.s3.model.PutBucketVersioningRequest;
import software.amazon.awssdk.services.s3.model.PutBucketVersioningResponse;
import software.amazon.awssdk.services.s3.model.PutBucketWebsiteRequest;
import software.amazon.awssdk.services.s3.model.PutBucketWebsiteResponse;
import software.amazon.awssdk.services.s3.model.PutObjectAclRequest;
import software.amazon.awssdk.services.s3.model.PutObjectAclResponse;
import software.amazon.awssdk.services.s3.model.PutObjectLegalHoldRequest;
import software.amazon.awssdk.services.s3.model.PutObjectLegalHoldResponse;
import software.amazon.awssdk.services.s3.model.PutObjectLockConfigurationRequest;
import software.amazon.awssdk.services.s3.model.PutObjectLockConfigurationResponse;
import software.amazon.awssdk.services.s3.model.PutObjectRequest;
import software.amazon.awssdk.services.s3.model.PutObjectResponse;
import software.amazon.awssdk.services.s3.model.PutObjectRetentionRequest;
import software.amazon.awssdk.services.s3.model.PutObjectRetentionResponse;
import software.amazon.awssdk.services.s3.model.PutObjectTaggingRequest;
import software.amazon.awssdk.services.s3.model.PutObjectTaggingResponse;
import software.amazon.awssdk.services.s3.model.PutPublicAccessBlockRequest;
import software.amazon.awssdk.services.s3.model.PutPublicAccessBlockResponse;
import software.amazon.awssdk.services.s3.model.RestoreObjectRequest;
import software.amazon.awssdk.services.s3.model.RestoreObjectResponse;
import software.amazon.awssdk.services.s3.model.S3Exception;
import software.amazon.awssdk.services.s3.model.S3Request;
import software.amazon.awssdk.services.s3.model.UploadPartCopyRequest;
import software.amazon.awssdk.services.s3.model.UploadPartCopyResponse;
import software.amazon.awssdk.services.s3.model.UploadPartRequest;
import software.amazon.awssdk.services.s3.model.UploadPartResponse;
import software.amazon.awssdk.services.s3.paginators.ListMultipartUploadsPublisher;
import software.amazon.awssdk.services.s3.paginators.ListObjectVersionsPublisher;
import software.amazon.awssdk.services.s3.paginators.ListObjectsV2Publisher;
import software.amazon.awssdk.services.s3.paginators.ListPartsPublisher;
import software.amazon.awssdk.services.s3.transform.AbortMultipartUploadRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.CompleteMultipartUploadRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.CopyObjectRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.CreateBucketRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.CreateMultipartUploadRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.DeleteBucketAnalyticsConfigurationRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.DeleteBucketCorsRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.DeleteBucketEncryptionRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.DeleteBucketInventoryConfigurationRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.DeleteBucketLifecycleRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.DeleteBucketMetricsConfigurationRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.DeleteBucketPolicyRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.DeleteBucketReplicationRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.DeleteBucketRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.DeleteBucketTaggingRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.DeleteBucketWebsiteRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.DeleteObjectRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.DeleteObjectTaggingRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.DeleteObjectsRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.DeletePublicAccessBlockRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.GetBucketAccelerateConfigurationRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.GetBucketAclRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.GetBucketAnalyticsConfigurationRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.GetBucketCorsRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.GetBucketEncryptionRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.GetBucketInventoryConfigurationRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.GetBucketLifecycleConfigurationRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.GetBucketLocationRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.GetBucketLoggingRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.GetBucketMetricsConfigurationRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.GetBucketNotificationConfigurationRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.GetBucketPolicyRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.GetBucketPolicyStatusRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.GetBucketReplicationRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.GetBucketRequestPaymentRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.GetBucketTaggingRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.GetBucketVersioningRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.GetBucketWebsiteRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.GetObjectAclRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.GetObjectLegalHoldRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.GetObjectLockConfigurationRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.GetObjectRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.GetObjectRetentionRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.GetObjectTaggingRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.GetObjectTorrentRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.GetPublicAccessBlockRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.HeadBucketRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.HeadObjectRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.ListBucketAnalyticsConfigurationsRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.ListBucketInventoryConfigurationsRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.ListBucketMetricsConfigurationsRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.ListBucketsRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.ListMultipartUploadsRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.ListObjectVersionsRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.ListObjectsRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.ListObjectsV2RequestMarshaller;
import software.amazon.awssdk.services.s3.transform.ListPartsRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.PutBucketAccelerateConfigurationRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.PutBucketAclRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.PutBucketAnalyticsConfigurationRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.PutBucketCorsRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.PutBucketEncryptionRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.PutBucketInventoryConfigurationRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.PutBucketLifecycleConfigurationRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.PutBucketLoggingRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.PutBucketMetricsConfigurationRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.PutBucketNotificationConfigurationRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.PutBucketPolicyRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.PutBucketReplicationRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.PutBucketRequestPaymentRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.PutBucketTaggingRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.PutBucketVersioningRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.PutBucketWebsiteRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.PutObjectAclRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.PutObjectLegalHoldRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.PutObjectLockConfigurationRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.PutObjectRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.PutObjectRetentionRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.PutObjectTaggingRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.PutPublicAccessBlockRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.RestoreObjectRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.UploadPartCopyRequestMarshaller;
import software.amazon.awssdk.services.s3.transform.UploadPartRequestMarshaller;
import software.amazon.awssdk.utils.CompletableFutureUtils;
/**
* Internal implementation of {@link S3AsyncClient}.
*
* @see S3AsyncClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultS3AsyncClient implements S3AsyncClient {
private static final Logger log = LoggerFactory.getLogger(DefaultS3AsyncClient.class);
private final AsyncClientHandler clientHandler;
private final AwsS3ProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
protected DefaultS3AsyncClient(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsAsyncClientHandler(clientConfiguration);
this.clientConfiguration = clientConfiguration;
this.protocolFactory = init();
}
@Override
public final String serviceName() {
return SERVICE_NAME;
}
/**
*
* Aborts a multipart upload.
*
*
* To verify that all parts have been removed, so you don't get charged for the part storage, you should call the
* List Parts operation and ensure the parts list is empty.
*
*
* @param abortMultipartUploadRequest
* @return A Java Future containing the result of the AbortMultipartUpload operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NoSuchUploadException The specified multipart upload does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.AbortMultipartUpload
*/
@Override
public CompletableFuture abortMultipartUpload(
AbortMultipartUploadRequest abortMultipartUploadRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
AbortMultipartUploadResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("AbortMultipartUpload")
.withMarshaller(new AbortMultipartUploadRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(abortMultipartUploadRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Completes a multipart upload by assembling previously uploaded parts.
*
*
* @param completeMultipartUploadRequest
* @return A Java Future containing the result of the CompleteMultipartUpload operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.CompleteMultipartUpload
*/
@Override
public CompletableFuture completeMultipartUpload(
CompleteMultipartUploadRequest completeMultipartUploadRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
CompleteMultipartUploadResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CompleteMultipartUpload")
.withMarshaller(new CompleteMultipartUploadRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(completeMultipartUploadRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a copy of an object that is already stored in Amazon S3.
*
*
* @param copyObjectRequest
* @return A Java Future containing the result of the CopyObject operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ObjectNotInActiveTierErrorException The source object of the COPY operation is not in the active tier
* and is only stored in Amazon Glacier.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.CopyObject
*/
@Override
public CompletableFuture copyObject(CopyObjectRequest copyObjectRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
CopyObjectResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CopyObject").withMarshaller(new CopyObjectRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(copyObjectRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a new bucket.
*
*
* @param createBucketRequest
* @return A Java Future containing the result of the CreateBucket operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BucketAlreadyExistsException The requested bucket name is not available. The bucket namespace is
* shared by all users of the system. Please select a different name and try again.
* - BucketAlreadyOwnedByYouException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.CreateBucket
*/
@Override
public CompletableFuture createBucket(CreateBucketRequest createBucketRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
CreateBucketResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateBucket").withMarshaller(new CreateBucketRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(createBucketRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Initiates a multipart upload and returns an upload ID.
*
*
* Note: After you initiate multipart upload and upload one or more parts, you must either complete or abort
* multipart upload in order to stop getting charged for storage of the uploaded parts. Only after you either
* complete or abort multipart upload, Amazon S3 frees up the parts storage and stops charging you for the parts
* storage.
*
*
* @param createMultipartUploadRequest
* @return A Java Future containing the result of the CreateMultipartUpload operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.CreateMultipartUpload
*/
@Override
public CompletableFuture createMultipartUpload(
CreateMultipartUploadRequest createMultipartUploadRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
CreateMultipartUploadResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateMultipartUpload")
.withMarshaller(new CreateMultipartUploadRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(createMultipartUploadRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the bucket. All objects (including all object versions and Delete Markers) in the bucket must be deleted
* before the bucket itself can be deleted.
*
*
* @param deleteBucketRequest
* @return A Java Future containing the result of the DeleteBucket operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.DeleteBucket
*/
@Override
public CompletableFuture deleteBucket(DeleteBucketRequest deleteBucketRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
DeleteBucketResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteBucket").withMarshaller(new DeleteBucketRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(deleteBucketRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes an analytics configuration for the bucket (specified by the analytics configuration ID).
*
*
* @param deleteBucketAnalyticsConfigurationRequest
* @return A Java Future containing the result of the DeleteBucketAnalyticsConfiguration operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.DeleteBucketAnalyticsConfiguration
*/
@Override
public CompletableFuture deleteBucketAnalyticsConfiguration(
DeleteBucketAnalyticsConfigurationRequest deleteBucketAnalyticsConfigurationRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DeleteBucketAnalyticsConfigurationResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteBucketAnalyticsConfiguration")
.withMarshaller(new DeleteBucketAnalyticsConfigurationRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(deleteBucketAnalyticsConfigurationRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the CORS configuration information set for the bucket.
*
*
* @param deleteBucketCorsRequest
* @return A Java Future containing the result of the DeleteBucketCors operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.DeleteBucketCors
*/
@Override
public CompletableFuture deleteBucketCors(DeleteBucketCorsRequest deleteBucketCorsRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
DeleteBucketCorsResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteBucketCors").withMarshaller(new DeleteBucketCorsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(deleteBucketCorsRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the server-side encryption configuration from the bucket.
*
*
* @param deleteBucketEncryptionRequest
* @return A Java Future containing the result of the DeleteBucketEncryption operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.DeleteBucketEncryption
*/
@Override
public CompletableFuture deleteBucketEncryption(
DeleteBucketEncryptionRequest deleteBucketEncryptionRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
DeleteBucketEncryptionResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteBucketEncryption")
.withMarshaller(new DeleteBucketEncryptionRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(deleteBucketEncryptionRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes an inventory configuration (identified by the inventory ID) from the bucket.
*
*
* @param deleteBucketInventoryConfigurationRequest
* @return A Java Future containing the result of the DeleteBucketInventoryConfiguration operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.DeleteBucketInventoryConfiguration
*/
@Override
public CompletableFuture deleteBucketInventoryConfiguration(
DeleteBucketInventoryConfigurationRequest deleteBucketInventoryConfigurationRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DeleteBucketInventoryConfigurationResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteBucketInventoryConfiguration")
.withMarshaller(new DeleteBucketInventoryConfigurationRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(deleteBucketInventoryConfigurationRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the lifecycle configuration from the bucket.
*
*
* @param deleteBucketLifecycleRequest
* @return A Java Future containing the result of the DeleteBucketLifecycle operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.DeleteBucketLifecycle
*/
@Override
public CompletableFuture deleteBucketLifecycle(
DeleteBucketLifecycleRequest deleteBucketLifecycleRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
DeleteBucketLifecycleResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteBucketLifecycle")
.withMarshaller(new DeleteBucketLifecycleRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(deleteBucketLifecycleRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes a metrics configuration (specified by the metrics configuration ID) from the bucket.
*
*
* @param deleteBucketMetricsConfigurationRequest
* @return A Java Future containing the result of the DeleteBucketMetricsConfiguration operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.DeleteBucketMetricsConfiguration
*/
@Override
public CompletableFuture deleteBucketMetricsConfiguration(
DeleteBucketMetricsConfigurationRequest deleteBucketMetricsConfigurationRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DeleteBucketMetricsConfigurationResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteBucketMetricsConfiguration")
.withMarshaller(new DeleteBucketMetricsConfigurationRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(deleteBucketMetricsConfigurationRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the policy from the bucket.
*
*
* @param deleteBucketPolicyRequest
* @return A Java Future containing the result of the DeleteBucketPolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.DeleteBucketPolicy
*/
@Override
public CompletableFuture deleteBucketPolicy(DeleteBucketPolicyRequest deleteBucketPolicyRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
DeleteBucketPolicyResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteBucketPolicy")
.withMarshaller(new DeleteBucketPolicyRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(deleteBucketPolicyRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the replication configuration from the bucket. For information about replication configuration, see Cross-Region Replication (CRR) in the
* Amazon S3 Developer Guide.
*
*
* @param deleteBucketReplicationRequest
* @return A Java Future containing the result of the DeleteBucketReplication operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.DeleteBucketReplication
*/
@Override
public CompletableFuture deleteBucketReplication(
DeleteBucketReplicationRequest deleteBucketReplicationRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
DeleteBucketReplicationResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteBucketReplication")
.withMarshaller(new DeleteBucketReplicationRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(deleteBucketReplicationRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the tags from the bucket.
*
*
* @param deleteBucketTaggingRequest
* @return A Java Future containing the result of the DeleteBucketTagging operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.DeleteBucketTagging
*/
@Override
public CompletableFuture deleteBucketTagging(
DeleteBucketTaggingRequest deleteBucketTaggingRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
DeleteBucketTaggingResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteBucketTagging")
.withMarshaller(new DeleteBucketTaggingRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(deleteBucketTaggingRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* This operation removes the website configuration from the bucket.
*
*
* @param deleteBucketWebsiteRequest
* @return A Java Future containing the result of the DeleteBucketWebsite operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.DeleteBucketWebsite
*/
@Override
public CompletableFuture deleteBucketWebsite(
DeleteBucketWebsiteRequest deleteBucketWebsiteRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
DeleteBucketWebsiteResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteBucketWebsite")
.withMarshaller(new DeleteBucketWebsiteRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(deleteBucketWebsiteRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Removes the null version (if there is one) of an object and inserts a delete marker, which becomes the latest
* version of the object. If there isn't a null version, Amazon S3 does not remove any objects.
*
*
* @param deleteObjectRequest
* @return A Java Future containing the result of the DeleteObject operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.DeleteObject
*/
@Override
public CompletableFuture deleteObject(DeleteObjectRequest deleteObjectRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
DeleteObjectResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteObject").withMarshaller(new DeleteObjectRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(deleteObjectRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Removes the tag-set from an existing object.
*
*
* @param deleteObjectTaggingRequest
* @return A Java Future containing the result of the DeleteObjectTagging operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.DeleteObjectTagging
*/
@Override
public CompletableFuture deleteObjectTagging(
DeleteObjectTaggingRequest deleteObjectTaggingRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
DeleteObjectTaggingResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteObjectTagging")
.withMarshaller(new DeleteObjectTaggingRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(deleteObjectTaggingRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* This operation enables you to delete multiple objects from a bucket using a single HTTP request. You may specify
* up to 1000 keys.
*
*
* @param deleteObjectsRequest
* @return A Java Future containing the result of the DeleteObjects operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.DeleteObjects
*/
@Override
public CompletableFuture deleteObjects(DeleteObjectsRequest deleteObjectsRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
DeleteObjectsResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteObjects").withMarshaller(new DeleteObjectsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(deleteObjectsRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Removes the PublicAccessBlock
configuration from an Amazon S3 bucket.
*
*
* @param deletePublicAccessBlockRequest
* @return A Java Future containing the result of the DeletePublicAccessBlock operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.DeletePublicAccessBlock
*/
@Override
public CompletableFuture deletePublicAccessBlock(
DeletePublicAccessBlockRequest deletePublicAccessBlockRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
DeletePublicAccessBlockResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeletePublicAccessBlock")
.withMarshaller(new DeletePublicAccessBlockRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(deletePublicAccessBlockRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns the accelerate configuration of a bucket.
*
*
* @param getBucketAccelerateConfigurationRequest
* @return A Java Future containing the result of the GetBucketAccelerateConfiguration operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.GetBucketAccelerateConfiguration
*/
@Override
public CompletableFuture getBucketAccelerateConfiguration(
GetBucketAccelerateConfigurationRequest getBucketAccelerateConfigurationRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(GetBucketAccelerateConfigurationResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetBucketAccelerateConfiguration")
.withMarshaller(new GetBucketAccelerateConfigurationRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(getBucketAccelerateConfigurationRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Gets the access control policy for the bucket.
*
*
* @param getBucketAclRequest
* @return A Java Future containing the result of the GetBucketAcl operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.GetBucketAcl
*/
@Override
public CompletableFuture getBucketAcl(GetBucketAclRequest getBucketAclRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
GetBucketAclResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetBucketAcl").withMarshaller(new GetBucketAclRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(getBucketAclRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Gets an analytics configuration for the bucket (specified by the analytics configuration ID).
*
*
* @param getBucketAnalyticsConfigurationRequest
* @return A Java Future containing the result of the GetBucketAnalyticsConfiguration operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.GetBucketAnalyticsConfiguration
*/
@Override
public CompletableFuture getBucketAnalyticsConfiguration(
GetBucketAnalyticsConfigurationRequest getBucketAnalyticsConfigurationRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
GetBucketAnalyticsConfigurationResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetBucketAnalyticsConfiguration")
.withMarshaller(new GetBucketAnalyticsConfigurationRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(getBucketAnalyticsConfigurationRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns the CORS configuration for the bucket.
*
*
* @param getBucketCorsRequest
* @return A Java Future containing the result of the GetBucketCors operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.GetBucketCors
*/
@Override
public CompletableFuture getBucketCors(GetBucketCorsRequest getBucketCorsRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
GetBucketCorsResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetBucketCors").withMarshaller(new GetBucketCorsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(getBucketCorsRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns the server-side encryption configuration of a bucket.
*
*
* @param getBucketEncryptionRequest
* @return A Java Future containing the result of the GetBucketEncryption operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.GetBucketEncryption
*/
@Override
public CompletableFuture getBucketEncryption(
GetBucketEncryptionRequest getBucketEncryptionRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
GetBucketEncryptionResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetBucketEncryption")
.withMarshaller(new GetBucketEncryptionRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(getBucketEncryptionRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns an inventory configuration (identified by the inventory ID) from the bucket.
*
*
* @param getBucketInventoryConfigurationRequest
* @return A Java Future containing the result of the GetBucketInventoryConfiguration operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.GetBucketInventoryConfiguration
*/
@Override
public CompletableFuture getBucketInventoryConfiguration(
GetBucketInventoryConfigurationRequest getBucketInventoryConfigurationRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
GetBucketInventoryConfigurationResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetBucketInventoryConfiguration")
.withMarshaller(new GetBucketInventoryConfigurationRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(getBucketInventoryConfigurationRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns the lifecycle configuration information set on the bucket.
*
*
* @param getBucketLifecycleConfigurationRequest
* @return A Java Future containing the result of the GetBucketLifecycleConfiguration operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.GetBucketLifecycleConfiguration
*/
@Override
public CompletableFuture getBucketLifecycleConfiguration(
GetBucketLifecycleConfigurationRequest getBucketLifecycleConfigurationRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
GetBucketLifecycleConfigurationResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetBucketLifecycleConfiguration")
.withMarshaller(new GetBucketLifecycleConfigurationRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(getBucketLifecycleConfigurationRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns the region the bucket resides in.
*
*
* @param getBucketLocationRequest
* @return A Java Future containing the result of the GetBucketLocation operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.GetBucketLocation
*/
@Override
public CompletableFuture getBucketLocation(GetBucketLocationRequest getBucketLocationRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
GetBucketLocationResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetBucketLocation")
.withMarshaller(new GetBucketLocationRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getBucketLocationRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns the logging status of a bucket and the permissions users have to view and modify that status. To use GET,
* you must be the bucket owner.
*
*
* @param getBucketLoggingRequest
* @return A Java Future containing the result of the GetBucketLogging operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.GetBucketLogging
*/
@Override
public CompletableFuture getBucketLogging(GetBucketLoggingRequest getBucketLoggingRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
GetBucketLoggingResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetBucketLogging").withMarshaller(new GetBucketLoggingRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(getBucketLoggingRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Gets a metrics configuration (specified by the metrics configuration ID) from the bucket.
*
*
* @param getBucketMetricsConfigurationRequest
* @return A Java Future containing the result of the GetBucketMetricsConfiguration operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.GetBucketMetricsConfiguration
*/
@Override
public CompletableFuture getBucketMetricsConfiguration(
GetBucketMetricsConfigurationRequest getBucketMetricsConfigurationRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
GetBucketMetricsConfigurationResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetBucketMetricsConfiguration")
.withMarshaller(new GetBucketMetricsConfigurationRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(getBucketMetricsConfigurationRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns the notification configuration of a bucket.
*
*
* @param getBucketNotificationConfigurationRequest
* @return A Java Future containing the result of the GetBucketNotificationConfiguration operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.GetBucketNotificationConfiguration
*/
@Override
public CompletableFuture getBucketNotificationConfiguration(
GetBucketNotificationConfigurationRequest getBucketNotificationConfigurationRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(GetBucketNotificationConfigurationResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetBucketNotificationConfiguration")
.withMarshaller(new GetBucketNotificationConfigurationRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(getBucketNotificationConfigurationRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns the policy of a specified bucket.
*
*
* @param getBucketPolicyRequest
* @return A Java Future containing the result of the GetBucketPolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.GetBucketPolicy
*/
@Override
public CompletableFuture getBucketPolicy(GetBucketPolicyRequest getBucketPolicyRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
GetBucketPolicyResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetBucketPolicy").withMarshaller(new GetBucketPolicyRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(getBucketPolicyRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieves the policy status for an Amazon S3 bucket, indicating whether the bucket is public.
*
*
* @param getBucketPolicyStatusRequest
* @return A Java Future containing the result of the GetBucketPolicyStatus operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.GetBucketPolicyStatus
*/
@Override
public CompletableFuture getBucketPolicyStatus(
GetBucketPolicyStatusRequest getBucketPolicyStatusRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
GetBucketPolicyStatusResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetBucketPolicyStatus")
.withMarshaller(new GetBucketPolicyStatusRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(getBucketPolicyStatusRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns the replication configuration of a bucket.
*
*
*
* It can take a while to propagate the put or delete a replication configuration to all Amazon S3 systems.
* Therefore, a get request soon after put or delete can return a wrong result.
*
*
*
* @param getBucketReplicationRequest
* @return A Java Future containing the result of the GetBucketReplication operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.GetBucketReplication
*/
@Override
public CompletableFuture getBucketReplication(
GetBucketReplicationRequest getBucketReplicationRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
GetBucketReplicationResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetBucketReplication")
.withMarshaller(new GetBucketReplicationRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(getBucketReplicationRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns the request payment configuration of a bucket.
*
*
* @param getBucketRequestPaymentRequest
* @return A Java Future containing the result of the GetBucketRequestPayment operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.GetBucketRequestPayment
*/
@Override
public CompletableFuture getBucketRequestPayment(
GetBucketRequestPaymentRequest getBucketRequestPaymentRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
GetBucketRequestPaymentResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetBucketRequestPayment")
.withMarshaller(new GetBucketRequestPaymentRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(getBucketRequestPaymentRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns the tag set associated with the bucket.
*
*
* @param getBucketTaggingRequest
* @return A Java Future containing the result of the GetBucketTagging operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.GetBucketTagging
*/
@Override
public CompletableFuture getBucketTagging(GetBucketTaggingRequest getBucketTaggingRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
GetBucketTaggingResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetBucketTagging").withMarshaller(new GetBucketTaggingRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(getBucketTaggingRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns the versioning state of a bucket.
*
*
* @param getBucketVersioningRequest
* @return A Java Future containing the result of the GetBucketVersioning operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.GetBucketVersioning
*/
@Override
public CompletableFuture getBucketVersioning(
GetBucketVersioningRequest getBucketVersioningRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
GetBucketVersioningResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetBucketVersioning")
.withMarshaller(new GetBucketVersioningRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(getBucketVersioningRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns the website configuration for a bucket.
*
*
* @param getBucketWebsiteRequest
* @return A Java Future containing the result of the GetBucketWebsite operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.GetBucketWebsite
*/
@Override
public CompletableFuture getBucketWebsite(GetBucketWebsiteRequest getBucketWebsiteRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
GetBucketWebsiteResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetBucketWebsite").withMarshaller(new GetBucketWebsiteRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(getBucketWebsiteRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieves objects from Amazon S3.
*
*
* @param getObjectRequest
* @param asyncResponseTransformer
* The response transformer for processing the streaming response in a non-blocking manner. See
* {@link AsyncResponseTransformer} for details on how this callback should be implemented and for links to
* precanned implementations for common scenarios like downloading to a file. The service documentation for
* the response content is as follows '
*
* Object data.
*
* '.
* @return A future to the transformed result of the AsyncResponseTransformer.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NoSuchKeyException The specified key does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.GetObject
*/
@Override
public CompletableFuture getObject(GetObjectRequest getObjectRequest,
AsyncResponseTransformer asyncResponseTransformer) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
GetObjectResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(true));
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler
.execute(new ClientExecutionParams().withOperationName("GetObject")
.withMarshaller(new GetObjectRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getObjectRequest), asyncResponseTransformer);
} catch (Throwable t) {
runAndLogError(log, "Exception thrown in exceptionOccurred callback, ignoring",
() -> asyncResponseTransformer.exceptionOccurred(t));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns the access control list (ACL) of an object.
*
*
* @param getObjectAclRequest
* @return A Java Future containing the result of the GetObjectAcl operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NoSuchKeyException The specified key does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.GetObjectAcl
*/
@Override
public CompletableFuture getObjectAcl(GetObjectAclRequest getObjectAclRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
GetObjectAclResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetObjectAcl").withMarshaller(new GetObjectAclRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(getObjectAclRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Gets an object's current Legal Hold status.
*
*
* @param getObjectLegalHoldRequest
* @return A Java Future containing the result of the GetObjectLegalHold operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.GetObjectLegalHold
*/
@Override
public CompletableFuture getObjectLegalHold(GetObjectLegalHoldRequest getObjectLegalHoldRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
GetObjectLegalHoldResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetObjectLegalHold")
.withMarshaller(new GetObjectLegalHoldRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(getObjectLegalHoldRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Gets the Object Lock configuration for a bucket. The rule specified in the Object Lock configuration will be
* applied by default to every new object placed in the specified bucket.
*
*
* @param getObjectLockConfigurationRequest
* @return A Java Future containing the result of the GetObjectLockConfiguration operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.GetObjectLockConfiguration
*/
@Override
public CompletableFuture getObjectLockConfiguration(
GetObjectLockConfigurationRequest getObjectLockConfigurationRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
GetObjectLockConfigurationResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetObjectLockConfiguration")
.withMarshaller(new GetObjectLockConfigurationRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(getObjectLockConfigurationRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieves an object's retention settings.
*
*
* @param getObjectRetentionRequest
* @return A Java Future containing the result of the GetObjectRetention operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.GetObjectRetention
*/
@Override
public CompletableFuture getObjectRetention(GetObjectRetentionRequest getObjectRetentionRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
GetObjectRetentionResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetObjectRetention")
.withMarshaller(new GetObjectRetentionRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(getObjectRetentionRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns the tag-set of an object.
*
*
* @param getObjectTaggingRequest
* @return A Java Future containing the result of the GetObjectTagging operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.GetObjectTagging
*/
@Override
public CompletableFuture getObjectTagging(GetObjectTaggingRequest getObjectTaggingRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
GetObjectTaggingResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetObjectTagging").withMarshaller(new GetObjectTaggingRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(getObjectTaggingRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Return torrent files from a bucket.
*
*
* @param getObjectTorrentRequest
* @param asyncResponseTransformer
* The response transformer for processing the streaming response in a non-blocking manner. See
* {@link AsyncResponseTransformer} for details on how this callback should be implemented and for links to
* precanned implementations for common scenarios like downloading to a file. The service documentation for
* the response content is as follows ''.
* @return A future to the transformed result of the AsyncResponseTransformer.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.GetObjectTorrent
*/
@Override
public CompletableFuture getObjectTorrent(GetObjectTorrentRequest getObjectTorrentRequest,
AsyncResponseTransformer asyncResponseTransformer) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
GetObjectTorrentResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(true));
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler.execute(
new ClientExecutionParams()
.withOperationName("GetObjectTorrent")
.withMarshaller(new GetObjectTorrentRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(getObjectTorrentRequest), asyncResponseTransformer);
} catch (Throwable t) {
runAndLogError(log, "Exception thrown in exceptionOccurred callback, ignoring",
() -> asyncResponseTransformer.exceptionOccurred(t));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieves the PublicAccessBlock
configuration for an Amazon S3 bucket.
*
*
* @param getPublicAccessBlockRequest
* @return A Java Future containing the result of the GetPublicAccessBlock operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.GetPublicAccessBlock
*/
@Override
public CompletableFuture getPublicAccessBlock(
GetPublicAccessBlockRequest getPublicAccessBlockRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
GetPublicAccessBlockResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetPublicAccessBlock")
.withMarshaller(new GetPublicAccessBlockRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(getPublicAccessBlockRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* This operation is useful to determine if a bucket exists and you have permission to access it.
*
*
* @param headBucketRequest
* @return A Java Future containing the result of the HeadBucket operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NoSuchBucketException The specified bucket does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.HeadBucket
*/
@Override
public CompletableFuture headBucket(HeadBucketRequest headBucketRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
HeadBucketResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("HeadBucket").withMarshaller(new HeadBucketRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(headBucketRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* The HEAD operation retrieves metadata from an object without returning the object itself. This operation is
* useful if you're only interested in an object's metadata. To use HEAD, you must have READ access to the object.
*
*
* @param headObjectRequest
* @return A Java Future containing the result of the HeadObject operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NoSuchKeyException The specified key does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.HeadObject
*/
@Override
public CompletableFuture headObject(HeadObjectRequest headObjectRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
HeadObjectResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("HeadObject").withMarshaller(new HeadObjectRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(headObjectRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists the analytics configurations for the bucket.
*
*
* @param listBucketAnalyticsConfigurationsRequest
* @return A Java Future containing the result of the ListBucketAnalyticsConfigurations operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.ListBucketAnalyticsConfigurations
*/
@Override
public CompletableFuture listBucketAnalyticsConfigurations(
ListBucketAnalyticsConfigurationsRequest listBucketAnalyticsConfigurationsRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(ListBucketAnalyticsConfigurationsResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListBucketAnalyticsConfigurations")
.withMarshaller(new ListBucketAnalyticsConfigurationsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(listBucketAnalyticsConfigurationsRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns a list of inventory configurations for the bucket.
*
*
* @param listBucketInventoryConfigurationsRequest
* @return A Java Future containing the result of the ListBucketInventoryConfigurations operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.ListBucketInventoryConfigurations
*/
@Override
public CompletableFuture listBucketInventoryConfigurations(
ListBucketInventoryConfigurationsRequest listBucketInventoryConfigurationsRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(ListBucketInventoryConfigurationsResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListBucketInventoryConfigurations")
.withMarshaller(new ListBucketInventoryConfigurationsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(listBucketInventoryConfigurationsRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists the metrics configurations for the bucket.
*
*
* @param listBucketMetricsConfigurationsRequest
* @return A Java Future containing the result of the ListBucketMetricsConfigurations operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.ListBucketMetricsConfigurations
*/
@Override
public CompletableFuture listBucketMetricsConfigurations(
ListBucketMetricsConfigurationsRequest listBucketMetricsConfigurationsRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
ListBucketMetricsConfigurationsResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListBucketMetricsConfigurations")
.withMarshaller(new ListBucketMetricsConfigurationsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(listBucketMetricsConfigurationsRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns a list of all buckets owned by the authenticated sender of the request.
*
*
* @param listBucketsRequest
* @return A Java Future containing the result of the ListBuckets operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.ListBuckets
*/
@Override
public CompletableFuture listBuckets(ListBucketsRequest listBucketsRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
ListBucketsResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListBuckets").withMarshaller(new ListBucketsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(listBucketsRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* This operation lists in-progress multipart uploads.
*
*
* @param listMultipartUploadsRequest
* @return A Java Future containing the result of the ListMultipartUploads operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.ListMultipartUploads
*/
@Override
public CompletableFuture listMultipartUploads(
ListMultipartUploadsRequest listMultipartUploadsRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
ListMultipartUploadsResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListMultipartUploads")
.withMarshaller(new ListMultipartUploadsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(listMultipartUploadsRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* This operation lists in-progress multipart uploads.
*
*
*
* This is a variant of
* {@link #listMultipartUploads(software.amazon.awssdk.services.s3.model.ListMultipartUploadsRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.s3.paginators.ListMultipartUploadsPublisher publisher = client.listMultipartUploadsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.s3.paginators.ListMultipartUploadsPublisher publisher = client.listMultipartUploadsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.s3.model.ListMultipartUploadsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Note: If you prefer to have control on service calls, use the
* {@link #listMultipartUploads(software.amazon.awssdk.services.s3.model.ListMultipartUploadsRequest)}
* operation.
*
*
* @param listMultipartUploadsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.ListMultipartUploads
*/
public ListMultipartUploadsPublisher listMultipartUploadsPaginator(ListMultipartUploadsRequest listMultipartUploadsRequest) {
return new ListMultipartUploadsPublisher(this, applyPaginatorUserAgent(listMultipartUploadsRequest));
}
/**
*
* Returns metadata about all of the versions of objects in a bucket.
*
*
* @param listObjectVersionsRequest
* @return A Java Future containing the result of the ListObjectVersions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.ListObjectVersions
*/
@Override
public CompletableFuture listObjectVersions(ListObjectVersionsRequest listObjectVersionsRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
ListObjectVersionsResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListObjectVersions")
.withMarshaller(new ListObjectVersionsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(listObjectVersionsRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns metadata about all of the versions of objects in a bucket.
*
*
*
* This is a variant of
* {@link #listObjectVersions(software.amazon.awssdk.services.s3.model.ListObjectVersionsRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.s3.paginators.ListObjectVersionsPublisher publisher = client.listObjectVersionsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.s3.paginators.ListObjectVersionsPublisher publisher = client.listObjectVersionsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.s3.model.ListObjectVersionsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Note: If you prefer to have control on service calls, use the
* {@link #listObjectVersions(software.amazon.awssdk.services.s3.model.ListObjectVersionsRequest)} operation.
*
*
* @param listObjectVersionsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.ListObjectVersions
*/
public ListObjectVersionsPublisher listObjectVersionsPaginator(ListObjectVersionsRequest listObjectVersionsRequest) {
return new ListObjectVersionsPublisher(this, applyPaginatorUserAgent(listObjectVersionsRequest));
}
/**
*
* Returns some or all (up to 1000) of the objects in a bucket. You can use the request parameters as selection
* criteria to return a subset of the objects in a bucket.
*
*
* @param listObjectsRequest
* @return A Java Future containing the result of the ListObjects operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NoSuchBucketException The specified bucket does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.ListObjects
*/
@Override
public CompletableFuture listObjects(ListObjectsRequest listObjectsRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
ListObjectsResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListObjects").withMarshaller(new ListObjectsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(listObjectsRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns some or all (up to 1000) of the objects in a bucket. You can use the request parameters as selection
* criteria to return a subset of the objects in a bucket. Note: ListObjectsV2 is the revised List Objects API and
* we recommend you use this revised API for new application development.
*
*
* @param listObjectsV2Request
* @return A Java Future containing the result of the ListObjectsV2 operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NoSuchBucketException The specified bucket does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.ListObjectsV2
*/
@Override
public CompletableFuture listObjectsV2(ListObjectsV2Request listObjectsV2Request) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
ListObjectsV2Response::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListObjectsV2").withMarshaller(new ListObjectsV2RequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(listObjectsV2Request));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns some or all (up to 1000) of the objects in a bucket. You can use the request parameters as selection
* criteria to return a subset of the objects in a bucket. Note: ListObjectsV2 is the revised List Objects API and
* we recommend you use this revised API for new application development.
*
*
*
* This is a variant of {@link #listObjectsV2(software.amazon.awssdk.services.s3.model.ListObjectsV2Request)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.s3.paginators.ListObjectsV2Publisher publisher = client.listObjectsV2Paginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.s3.paginators.ListObjectsV2Publisher publisher = client.listObjectsV2Paginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.s3.model.ListObjectsV2Response response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Note: If you prefer to have control on service calls, use the
* {@link #listObjectsV2(software.amazon.awssdk.services.s3.model.ListObjectsV2Request)} operation.
*
*
* @param listObjectsV2Request
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NoSuchBucketException The specified bucket does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.ListObjectsV2
*/
public ListObjectsV2Publisher listObjectsV2Paginator(ListObjectsV2Request listObjectsV2Request) {
return new ListObjectsV2Publisher(this, applyPaginatorUserAgent(listObjectsV2Request));
}
/**
*
* Lists the parts that have been uploaded for a specific multipart upload.
*
*
* @param listPartsRequest
* @return A Java Future containing the result of the ListParts operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.ListParts
*/
@Override
public CompletableFuture listParts(ListPartsRequest listPartsRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
ListPartsResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListParts").withMarshaller(new ListPartsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(listPartsRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists the parts that have been uploaded for a specific multipart upload.
*
*
*
* This is a variant of {@link #listParts(software.amazon.awssdk.services.s3.model.ListPartsRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.s3.paginators.ListPartsPublisher publisher = client.listPartsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.s3.paginators.ListPartsPublisher publisher = client.listPartsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.s3.model.ListPartsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Note: If you prefer to have control on service calls, use the
* {@link #listParts(software.amazon.awssdk.services.s3.model.ListPartsRequest)} operation.
*
*
* @param listPartsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.ListParts
*/
public ListPartsPublisher listPartsPaginator(ListPartsRequest listPartsRequest) {
return new ListPartsPublisher(this, applyPaginatorUserAgent(listPartsRequest));
}
/**
*
* Sets the accelerate configuration of an existing bucket.
*
*
* @param putBucketAccelerateConfigurationRequest
* @return A Java Future containing the result of the PutBucketAccelerateConfiguration operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.PutBucketAccelerateConfiguration
*/
@Override
public CompletableFuture putBucketAccelerateConfiguration(
PutBucketAccelerateConfigurationRequest putBucketAccelerateConfigurationRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(PutBucketAccelerateConfigurationResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("PutBucketAccelerateConfiguration")
.withMarshaller(new PutBucketAccelerateConfigurationRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(putBucketAccelerateConfigurationRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Sets the permissions on a bucket using access control lists (ACL).
*
*
* @param putBucketAclRequest
* @return A Java Future containing the result of the PutBucketAcl operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.PutBucketAcl
*/
@Override
public CompletableFuture putBucketAcl(PutBucketAclRequest putBucketAclRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
PutBucketAclResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("PutBucketAcl").withMarshaller(new PutBucketAclRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(putBucketAclRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Sets an analytics configuration for the bucket (specified by the analytics configuration ID).
*
*
* @param putBucketAnalyticsConfigurationRequest
* @return A Java Future containing the result of the PutBucketAnalyticsConfiguration operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.PutBucketAnalyticsConfiguration
*/
@Override
public CompletableFuture putBucketAnalyticsConfiguration(
PutBucketAnalyticsConfigurationRequest putBucketAnalyticsConfigurationRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
PutBucketAnalyticsConfigurationResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("PutBucketAnalyticsConfiguration")
.withMarshaller(new PutBucketAnalyticsConfigurationRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(putBucketAnalyticsConfigurationRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Sets the CORS configuration for a bucket.
*
*
* @param putBucketCorsRequest
* @return A Java Future containing the result of the PutBucketCors operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.PutBucketCors
*/
@Override
public CompletableFuture putBucketCors(PutBucketCorsRequest putBucketCorsRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
PutBucketCorsResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("PutBucketCors").withMarshaller(new PutBucketCorsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(putBucketCorsRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a new server-side encryption configuration (or replaces an existing one, if present).
*
*
* @param putBucketEncryptionRequest
* @return A Java Future containing the result of the PutBucketEncryption operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - S3Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample S3AsyncClient.PutBucketEncryption
*/
@Override
public CompletableFuture putBucketEncryption(
PutBucketEncryptionRequest putBucketEncryptionRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
PutBucketEncryptionResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
HttpResponseHandler