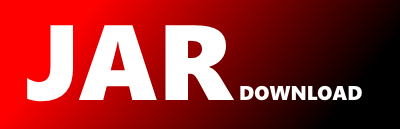
software.amazon.awssdk.services.s3.model.CSVOutput Maven / Gradle / Ivy
Show all versions of s3 Show documentation
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.s3.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Describes how CSV-formatted results are formatted.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class CSVOutput implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField QUOTE_FIELDS_FIELD = SdkField
. builder(MarshallingType.STRING)
.getter(getter(CSVOutput::quoteFieldsAsString))
.setter(setter(Builder::quoteFields))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("QuoteFields")
.unmarshallLocationName("QuoteFields").build()).build();
private static final SdkField QUOTE_ESCAPE_CHARACTER_FIELD = SdkField
. builder(MarshallingType.STRING)
.getter(getter(CSVOutput::quoteEscapeCharacter))
.setter(setter(Builder::quoteEscapeCharacter))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("QuoteEscapeCharacter")
.unmarshallLocationName("QuoteEscapeCharacter").build()).build();
private static final SdkField RECORD_DELIMITER_FIELD = SdkField
. builder(MarshallingType.STRING)
.getter(getter(CSVOutput::recordDelimiter))
.setter(setter(Builder::recordDelimiter))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RecordDelimiter")
.unmarshallLocationName("RecordDelimiter").build()).build();
private static final SdkField FIELD_DELIMITER_FIELD = SdkField
. builder(MarshallingType.STRING)
.getter(getter(CSVOutput::fieldDelimiter))
.setter(setter(Builder::fieldDelimiter))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("FieldDelimiter")
.unmarshallLocationName("FieldDelimiter").build()).build();
private static final SdkField QUOTE_CHARACTER_FIELD = SdkField
. builder(MarshallingType.STRING)
.getter(getter(CSVOutput::quoteCharacter))
.setter(setter(Builder::quoteCharacter))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("QuoteCharacter")
.unmarshallLocationName("QuoteCharacter").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(QUOTE_FIELDS_FIELD,
QUOTE_ESCAPE_CHARACTER_FIELD, RECORD_DELIMITER_FIELD, FIELD_DELIMITER_FIELD, QUOTE_CHARACTER_FIELD));
private static final long serialVersionUID = 1L;
private final String quoteFields;
private final String quoteEscapeCharacter;
private final String recordDelimiter;
private final String fieldDelimiter;
private final String quoteCharacter;
private CSVOutput(BuilderImpl builder) {
this.quoteFields = builder.quoteFields;
this.quoteEscapeCharacter = builder.quoteEscapeCharacter;
this.recordDelimiter = builder.recordDelimiter;
this.fieldDelimiter = builder.fieldDelimiter;
this.quoteCharacter = builder.quoteCharacter;
}
/**
*
* Indicates whether or not all output fields should be quoted.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #quoteFields} will
* return {@link QuoteFields#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #quoteFieldsAsString}.
*
*
* @return Indicates whether or not all output fields should be quoted.
* @see QuoteFields
*/
public QuoteFields quoteFields() {
return QuoteFields.fromValue(quoteFields);
}
/**
*
* Indicates whether or not all output fields should be quoted.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #quoteFields} will
* return {@link QuoteFields#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #quoteFieldsAsString}.
*
*
* @return Indicates whether or not all output fields should be quoted.
* @see QuoteFields
*/
public String quoteFieldsAsString() {
return quoteFields;
}
/**
*
* Th single character used for escaping the quote character inside an already escaped value.
*
*
* @return Th single character used for escaping the quote character inside an already escaped value.
*/
public String quoteEscapeCharacter() {
return quoteEscapeCharacter;
}
/**
*
* The value used to separate individual records.
*
*
* @return The value used to separate individual records.
*/
public String recordDelimiter() {
return recordDelimiter;
}
/**
*
* The value used to separate individual fields in a record.
*
*
* @return The value used to separate individual fields in a record.
*/
public String fieldDelimiter() {
return fieldDelimiter;
}
/**
*
* The value used for escaping where the field delimiter is part of the value.
*
*
* @return The value used for escaping where the field delimiter is part of the value.
*/
public String quoteCharacter() {
return quoteCharacter;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(quoteFieldsAsString());
hashCode = 31 * hashCode + Objects.hashCode(quoteEscapeCharacter());
hashCode = 31 * hashCode + Objects.hashCode(recordDelimiter());
hashCode = 31 * hashCode + Objects.hashCode(fieldDelimiter());
hashCode = 31 * hashCode + Objects.hashCode(quoteCharacter());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CSVOutput)) {
return false;
}
CSVOutput other = (CSVOutput) obj;
return Objects.equals(quoteFieldsAsString(), other.quoteFieldsAsString())
&& Objects.equals(quoteEscapeCharacter(), other.quoteEscapeCharacter())
&& Objects.equals(recordDelimiter(), other.recordDelimiter())
&& Objects.equals(fieldDelimiter(), other.fieldDelimiter())
&& Objects.equals(quoteCharacter(), other.quoteCharacter());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("CSVOutput").add("QuoteFields", quoteFieldsAsString())
.add("QuoteEscapeCharacter", quoteEscapeCharacter()).add("RecordDelimiter", recordDelimiter())
.add("FieldDelimiter", fieldDelimiter()).add("QuoteCharacter", quoteCharacter()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "QuoteFields":
return Optional.ofNullable(clazz.cast(quoteFieldsAsString()));
case "QuoteEscapeCharacter":
return Optional.ofNullable(clazz.cast(quoteEscapeCharacter()));
case "RecordDelimiter":
return Optional.ofNullable(clazz.cast(recordDelimiter()));
case "FieldDelimiter":
return Optional.ofNullable(clazz.cast(fieldDelimiter()));
case "QuoteCharacter":
return Optional.ofNullable(clazz.cast(quoteCharacter()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function