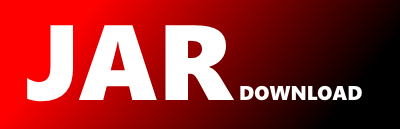
software.amazon.awssdk.services.s3.model.PutBucketAclRequest Maven / Gradle / Ivy
Show all versions of s3 Show documentation
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.s3.model;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.traits.PayloadTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class PutBucketAclRequest extends S3Request implements
ToCopyableBuilder {
private static final SdkField ACL_FIELD = SdkField
. builder(MarshallingType.STRING)
.getter(getter(PutBucketAclRequest::aclAsString))
.setter(setter(Builder::acl))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("x-amz-acl")
.unmarshallLocationName("x-amz-acl").build()).build();
private static final SdkField ACCESS_CONTROL_POLICY_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.getter(getter(PutBucketAclRequest::accessControlPolicy))
.setter(setter(Builder::accessControlPolicy))
.constructor(AccessControlPolicy::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AccessControlPolicy")
.unmarshallLocationName("AccessControlPolicy").build(), PayloadTrait.create()).build();
private static final SdkField BUCKET_FIELD = SdkField
. builder(MarshallingType.STRING)
.getter(getter(PutBucketAclRequest::bucket))
.setter(setter(Builder::bucket))
.traits(LocationTrait.builder().location(MarshallLocation.PATH).locationName("Bucket")
.unmarshallLocationName("Bucket").build()).build();
private static final SdkField CONTENT_MD5_FIELD = SdkField
. builder(MarshallingType.STRING)
.getter(getter(PutBucketAclRequest::contentMD5))
.setter(setter(Builder::contentMD5))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("Content-MD5")
.unmarshallLocationName("Content-MD5").build()).build();
private static final SdkField GRANT_FULL_CONTROL_FIELD = SdkField
. builder(MarshallingType.STRING)
.getter(getter(PutBucketAclRequest::grantFullControl))
.setter(setter(Builder::grantFullControl))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("x-amz-grant-full-control")
.unmarshallLocationName("x-amz-grant-full-control").build()).build();
private static final SdkField GRANT_READ_FIELD = SdkField
. builder(MarshallingType.STRING)
.getter(getter(PutBucketAclRequest::grantRead))
.setter(setter(Builder::grantRead))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("x-amz-grant-read")
.unmarshallLocationName("x-amz-grant-read").build()).build();
private static final SdkField GRANT_READ_ACP_FIELD = SdkField
. builder(MarshallingType.STRING)
.getter(getter(PutBucketAclRequest::grantReadACP))
.setter(setter(Builder::grantReadACP))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("x-amz-grant-read-acp")
.unmarshallLocationName("x-amz-grant-read-acp").build()).build();
private static final SdkField GRANT_WRITE_FIELD = SdkField
. builder(MarshallingType.STRING)
.getter(getter(PutBucketAclRequest::grantWrite))
.setter(setter(Builder::grantWrite))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("x-amz-grant-write")
.unmarshallLocationName("x-amz-grant-write").build()).build();
private static final SdkField GRANT_WRITE_ACP_FIELD = SdkField
. builder(MarshallingType.STRING)
.getter(getter(PutBucketAclRequest::grantWriteACP))
.setter(setter(Builder::grantWriteACP))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("x-amz-grant-write-acp")
.unmarshallLocationName("x-amz-grant-write-acp").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ACL_FIELD,
ACCESS_CONTROL_POLICY_FIELD, BUCKET_FIELD, CONTENT_MD5_FIELD, GRANT_FULL_CONTROL_FIELD, GRANT_READ_FIELD,
GRANT_READ_ACP_FIELD, GRANT_WRITE_FIELD, GRANT_WRITE_ACP_FIELD));
private final String acl;
private final AccessControlPolicy accessControlPolicy;
private final String bucket;
private final String contentMD5;
private final String grantFullControl;
private final String grantRead;
private final String grantReadACP;
private final String grantWrite;
private final String grantWriteACP;
private PutBucketAclRequest(BuilderImpl builder) {
super(builder);
this.acl = builder.acl;
this.accessControlPolicy = builder.accessControlPolicy;
this.bucket = builder.bucket;
this.contentMD5 = builder.contentMD5;
this.grantFullControl = builder.grantFullControl;
this.grantRead = builder.grantRead;
this.grantReadACP = builder.grantReadACP;
this.grantWrite = builder.grantWrite;
this.grantWriteACP = builder.grantWriteACP;
}
/**
*
* The canned ACL to apply to the bucket.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #acl} will return
* {@link BucketCannedACL#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #aclAsString}.
*
*
* @return The canned ACL to apply to the bucket.
* @see BucketCannedACL
*/
public BucketCannedACL acl() {
return BucketCannedACL.fromValue(acl);
}
/**
*
* The canned ACL to apply to the bucket.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #acl} will return
* {@link BucketCannedACL#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #aclAsString}.
*
*
* @return The canned ACL to apply to the bucket.
* @see BucketCannedACL
*/
public String aclAsString() {
return acl;
}
/**
*
*
* @return
*/
public AccessControlPolicy accessControlPolicy() {
return accessControlPolicy;
}
/**
*
*
* @return
*/
public String bucket() {
return bucket;
}
/**
*
*
* @return
*/
public String contentMD5() {
return contentMD5;
}
/**
*
* Allows grantee the read, write, read ACP, and write ACP permissions on the bucket.
*
*
* @return Allows grantee the read, write, read ACP, and write ACP permissions on the bucket.
*/
public String grantFullControl() {
return grantFullControl;
}
/**
*
* Allows grantee to list the objects in the bucket.
*
*
* @return Allows grantee to list the objects in the bucket.
*/
public String grantRead() {
return grantRead;
}
/**
*
* Allows grantee to read the bucket ACL.
*
*
* @return Allows grantee to read the bucket ACL.
*/
public String grantReadACP() {
return grantReadACP;
}
/**
*
* Allows grantee to create, overwrite, and delete any object in the bucket.
*
*
* @return Allows grantee to create, overwrite, and delete any object in the bucket.
*/
public String grantWrite() {
return grantWrite;
}
/**
*
* Allows grantee to write the ACL for the applicable bucket.
*
*
* @return Allows grantee to write the ACL for the applicable bucket.
*/
public String grantWriteACP() {
return grantWriteACP;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(aclAsString());
hashCode = 31 * hashCode + Objects.hashCode(accessControlPolicy());
hashCode = 31 * hashCode + Objects.hashCode(bucket());
hashCode = 31 * hashCode + Objects.hashCode(contentMD5());
hashCode = 31 * hashCode + Objects.hashCode(grantFullControl());
hashCode = 31 * hashCode + Objects.hashCode(grantRead());
hashCode = 31 * hashCode + Objects.hashCode(grantReadACP());
hashCode = 31 * hashCode + Objects.hashCode(grantWrite());
hashCode = 31 * hashCode + Objects.hashCode(grantWriteACP());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof PutBucketAclRequest)) {
return false;
}
PutBucketAclRequest other = (PutBucketAclRequest) obj;
return Objects.equals(aclAsString(), other.aclAsString())
&& Objects.equals(accessControlPolicy(), other.accessControlPolicy()) && Objects.equals(bucket(), other.bucket())
&& Objects.equals(contentMD5(), other.contentMD5())
&& Objects.equals(grantFullControl(), other.grantFullControl()) && Objects.equals(grantRead(), other.grantRead())
&& Objects.equals(grantReadACP(), other.grantReadACP()) && Objects.equals(grantWrite(), other.grantWrite())
&& Objects.equals(grantWriteACP(), other.grantWriteACP());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("PutBucketAclRequest").add("ACL", aclAsString())
.add("AccessControlPolicy", accessControlPolicy()).add("Bucket", bucket()).add("ContentMD5", contentMD5())
.add("GrantFullControl", grantFullControl()).add("GrantRead", grantRead()).add("GrantReadACP", grantReadACP())
.add("GrantWrite", grantWrite()).add("GrantWriteACP", grantWriteACP()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ACL":
return Optional.ofNullable(clazz.cast(aclAsString()));
case "AccessControlPolicy":
return Optional.ofNullable(clazz.cast(accessControlPolicy()));
case "Bucket":
return Optional.ofNullable(clazz.cast(bucket()));
case "ContentMD5":
return Optional.ofNullable(clazz.cast(contentMD5()));
case "GrantFullControl":
return Optional.ofNullable(clazz.cast(grantFullControl()));
case "GrantRead":
return Optional.ofNullable(clazz.cast(grantRead()));
case "GrantReadACP":
return Optional.ofNullable(clazz.cast(grantReadACP()));
case "GrantWrite":
return Optional.ofNullable(clazz.cast(grantWrite()));
case "GrantWriteACP":
return Optional.ofNullable(clazz.cast(grantWriteACP()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function