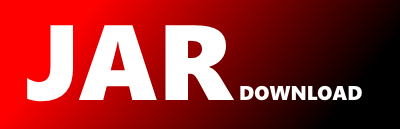
software.amazon.awssdk.services.s3control.model.JobReport Maven / Gradle / Ivy
Show all versions of s3control Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.s3control.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Contains the configuration parameters for a job-completion report.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class JobReport implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField BUCKET_FIELD = SdkField
. builder(MarshallingType.STRING)
.getter(getter(JobReport::bucket))
.setter(setter(Builder::bucket))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Bucket")
.unmarshallLocationName("Bucket").build()).build();
private static final SdkField FORMAT_FIELD = SdkField
. builder(MarshallingType.STRING)
.getter(getter(JobReport::formatAsString))
.setter(setter(Builder::format))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Format")
.unmarshallLocationName("Format").build()).build();
private static final SdkField ENABLED_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.getter(getter(JobReport::enabled))
.setter(setter(Builder::enabled))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Enabled")
.unmarshallLocationName("Enabled").build()).build();
private static final SdkField PREFIX_FIELD = SdkField
. builder(MarshallingType.STRING)
.getter(getter(JobReport::prefix))
.setter(setter(Builder::prefix))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Prefix")
.unmarshallLocationName("Prefix").build()).build();
private static final SdkField REPORT_SCOPE_FIELD = SdkField
. builder(MarshallingType.STRING)
.getter(getter(JobReport::reportScopeAsString))
.setter(setter(Builder::reportScope))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReportScope")
.unmarshallLocationName("ReportScope").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(BUCKET_FIELD, FORMAT_FIELD,
ENABLED_FIELD, PREFIX_FIELD, REPORT_SCOPE_FIELD));
private static final long serialVersionUID = 1L;
private final String bucket;
private final String format;
private final Boolean enabled;
private final String prefix;
private final String reportScope;
private JobReport(BuilderImpl builder) {
this.bucket = builder.bucket;
this.format = builder.format;
this.enabled = builder.enabled;
this.prefix = builder.prefix;
this.reportScope = builder.reportScope;
}
/**
*
* The Amazon Resource Name (ARN) for the bucket where specified job-completion report will be stored.
*
*
* @return The Amazon Resource Name (ARN) for the bucket where specified job-completion report will be stored.
*/
public String bucket() {
return bucket;
}
/**
*
* The format of the specified job-completion report.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #format} will
* return {@link JobReportFormat#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #formatAsString}.
*
*
* @return The format of the specified job-completion report.
* @see JobReportFormat
*/
public JobReportFormat format() {
return JobReportFormat.fromValue(format);
}
/**
*
* The format of the specified job-completion report.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #format} will
* return {@link JobReportFormat#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #formatAsString}.
*
*
* @return The format of the specified job-completion report.
* @see JobReportFormat
*/
public String formatAsString() {
return format;
}
/**
*
* Indicates whether the specified job will generate a job-completion report.
*
*
* @return Indicates whether the specified job will generate a job-completion report.
*/
public Boolean enabled() {
return enabled;
}
/**
*
* An optional prefix to describe where in the specified bucket the job-completion report will be stored. Amazon S3
* will store the job-completion report at <prefix>/job-<job-id>/report.json.
*
*
* @return An optional prefix to describe where in the specified bucket the job-completion report will be stored.
* Amazon S3 will store the job-completion report at <prefix>/job-<job-id>/report.json.
*/
public String prefix() {
return prefix;
}
/**
*
* Indicates whether the job-completion report will include details of all tasks or only failed tasks.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #reportScope} will
* return {@link JobReportScope#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #reportScopeAsString}.
*
*
* @return Indicates whether the job-completion report will include details of all tasks or only failed tasks.
* @see JobReportScope
*/
public JobReportScope reportScope() {
return JobReportScope.fromValue(reportScope);
}
/**
*
* Indicates whether the job-completion report will include details of all tasks or only failed tasks.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #reportScope} will
* return {@link JobReportScope#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #reportScopeAsString}.
*
*
* @return Indicates whether the job-completion report will include details of all tasks or only failed tasks.
* @see JobReportScope
*/
public String reportScopeAsString() {
return reportScope;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(bucket());
hashCode = 31 * hashCode + Objects.hashCode(formatAsString());
hashCode = 31 * hashCode + Objects.hashCode(enabled());
hashCode = 31 * hashCode + Objects.hashCode(prefix());
hashCode = 31 * hashCode + Objects.hashCode(reportScopeAsString());
return hashCode;
}
@Override
public boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof JobReport)) {
return false;
}
JobReport other = (JobReport) obj;
return Objects.equals(bucket(), other.bucket()) && Objects.equals(formatAsString(), other.formatAsString())
&& Objects.equals(enabled(), other.enabled()) && Objects.equals(prefix(), other.prefix())
&& Objects.equals(reportScopeAsString(), other.reportScopeAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("JobReport").add("Bucket", bucket()).add("Format", formatAsString()).add("Enabled", enabled())
.add("Prefix", prefix()).add("ReportScope", reportScopeAsString()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Bucket":
return Optional.ofNullable(clazz.cast(bucket()));
case "Format":
return Optional.ofNullable(clazz.cast(formatAsString()));
case "Enabled":
return Optional.ofNullable(clazz.cast(enabled()));
case "Prefix":
return Optional.ofNullable(clazz.cast(prefix()));
case "ReportScope":
return Optional.ofNullable(clazz.cast(reportScopeAsString()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function