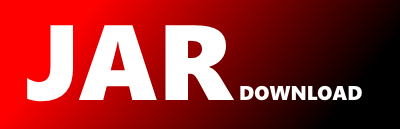
software.amazon.awssdk.services.s3control.model.CreateBucketRequest Maven / Gradle / Ivy
Show all versions of s3control Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.s3control.model;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.traits.PayloadTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class CreateBucketRequest extends S3ControlRequest implements
ToCopyableBuilder {
private static final SdkField ACL_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("ACL")
.getter(getter(CreateBucketRequest::aclAsString))
.setter(setter(Builder::acl))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("x-amz-acl")
.unmarshallLocationName("x-amz-acl").build()).build();
private static final SdkField BUCKET_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("Bucket")
.getter(getter(CreateBucketRequest::bucket))
.setter(setter(Builder::bucket))
.traits(LocationTrait.builder().location(MarshallLocation.PATH).locationName("name").unmarshallLocationName("name")
.build()).build();
private static final SdkField CREATE_BUCKET_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("CreateBucketConfiguration")
.getter(getter(CreateBucketRequest::createBucketConfiguration))
.setter(setter(Builder::createBucketConfiguration))
.constructor(CreateBucketConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CreateBucketConfiguration")
.unmarshallLocationName("CreateBucketConfiguration").build(), PayloadTrait.create()).build();
private static final SdkField GRANT_FULL_CONTROL_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("GrantFullControl")
.getter(getter(CreateBucketRequest::grantFullControl))
.setter(setter(Builder::grantFullControl))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("x-amz-grant-full-control")
.unmarshallLocationName("x-amz-grant-full-control").build()).build();
private static final SdkField GRANT_READ_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("GrantRead")
.getter(getter(CreateBucketRequest::grantRead))
.setter(setter(Builder::grantRead))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("x-amz-grant-read")
.unmarshallLocationName("x-amz-grant-read").build()).build();
private static final SdkField GRANT_READ_ACP_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("GrantReadACP")
.getter(getter(CreateBucketRequest::grantReadACP))
.setter(setter(Builder::grantReadACP))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("x-amz-grant-read-acp")
.unmarshallLocationName("x-amz-grant-read-acp").build()).build();
private static final SdkField GRANT_WRITE_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("GrantWrite")
.getter(getter(CreateBucketRequest::grantWrite))
.setter(setter(Builder::grantWrite))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("x-amz-grant-write")
.unmarshallLocationName("x-amz-grant-write").build()).build();
private static final SdkField GRANT_WRITE_ACP_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("GrantWriteACP")
.getter(getter(CreateBucketRequest::grantWriteACP))
.setter(setter(Builder::grantWriteACP))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("x-amz-grant-write-acp")
.unmarshallLocationName("x-amz-grant-write-acp").build()).build();
private static final SdkField OBJECT_LOCK_ENABLED_FOR_BUCKET_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("ObjectLockEnabledForBucket")
.getter(getter(CreateBucketRequest::objectLockEnabledForBucket))
.setter(setter(Builder::objectLockEnabledForBucket))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("x-amz-bucket-object-lock-enabled")
.unmarshallLocationName("x-amz-bucket-object-lock-enabled").build()).build();
private static final SdkField OUTPOST_ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("OutpostId")
.getter(getter(CreateBucketRequest::outpostId))
.setter(setter(Builder::outpostId))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("x-amz-outpost-id")
.unmarshallLocationName("x-amz-outpost-id").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ACL_FIELD, BUCKET_FIELD,
CREATE_BUCKET_CONFIGURATION_FIELD, GRANT_FULL_CONTROL_FIELD, GRANT_READ_FIELD, GRANT_READ_ACP_FIELD,
GRANT_WRITE_FIELD, GRANT_WRITE_ACP_FIELD, OBJECT_LOCK_ENABLED_FOR_BUCKET_FIELD, OUTPOST_ID_FIELD));
private final String acl;
private final String bucket;
private final CreateBucketConfiguration createBucketConfiguration;
private final String grantFullControl;
private final String grantRead;
private final String grantReadACP;
private final String grantWrite;
private final String grantWriteACP;
private final Boolean objectLockEnabledForBucket;
private final String outpostId;
private CreateBucketRequest(BuilderImpl builder) {
super(builder);
this.acl = builder.acl;
this.bucket = builder.bucket;
this.createBucketConfiguration = builder.createBucketConfiguration;
this.grantFullControl = builder.grantFullControl;
this.grantRead = builder.grantRead;
this.grantReadACP = builder.grantReadACP;
this.grantWrite = builder.grantWrite;
this.grantWriteACP = builder.grantWriteACP;
this.objectLockEnabledForBucket = builder.objectLockEnabledForBucket;
this.outpostId = builder.outpostId;
}
/**
*
* The canned ACL to apply to the bucket.
*
*
*
* This is not supported by Amazon S3 on Outposts buckets.
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #acl} will return
* {@link BucketCannedACL#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #aclAsString}.
*
*
* @return The canned ACL to apply to the bucket.
*
* This is not supported by Amazon S3 on Outposts buckets.
*
* @see BucketCannedACL
*/
public final BucketCannedACL acl() {
return BucketCannedACL.fromValue(acl);
}
/**
*
* The canned ACL to apply to the bucket.
*
*
*
* This is not supported by Amazon S3 on Outposts buckets.
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #acl} will return
* {@link BucketCannedACL#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #aclAsString}.
*
*
* @return The canned ACL to apply to the bucket.
*
* This is not supported by Amazon S3 on Outposts buckets.
*
* @see BucketCannedACL
*/
public final String aclAsString() {
return acl;
}
/**
*
* The name of the bucket.
*
*
* @return The name of the bucket.
*/
public final String bucket() {
return bucket;
}
/**
*
* The configuration information for the bucket.
*
*
*
* This is not supported by Amazon S3 on Outposts buckets.
*
*
*
* @return The configuration information for the bucket.
*
* This is not supported by Amazon S3 on Outposts buckets.
*
*/
public final CreateBucketConfiguration createBucketConfiguration() {
return createBucketConfiguration;
}
/**
*
* Allows grantee the read, write, read ACP, and write ACP permissions on the bucket.
*
*
*
* This is not supported by Amazon S3 on Outposts buckets.
*
*
*
* @return Allows grantee the read, write, read ACP, and write ACP permissions on the bucket.
*
* This is not supported by Amazon S3 on Outposts buckets.
*
*/
public final String grantFullControl() {
return grantFullControl;
}
/**
*
* Allows grantee to list the objects in the bucket.
*
*
*
* This is not supported by Amazon S3 on Outposts buckets.
*
*
*
* @return Allows grantee to list the objects in the bucket.
*
* This is not supported by Amazon S3 on Outposts buckets.
*
*/
public final String grantRead() {
return grantRead;
}
/**
*
* Allows grantee to read the bucket ACL.
*
*
*
* This is not supported by Amazon S3 on Outposts buckets.
*
*
*
* @return Allows grantee to read the bucket ACL.
*
* This is not supported by Amazon S3 on Outposts buckets.
*
*/
public final String grantReadACP() {
return grantReadACP;
}
/**
*
* Allows grantee to create, overwrite, and delete any object in the bucket.
*
*
*
* This is not supported by Amazon S3 on Outposts buckets.
*
*
*
* @return Allows grantee to create, overwrite, and delete any object in the bucket.
*
* This is not supported by Amazon S3 on Outposts buckets.
*
*/
public final String grantWrite() {
return grantWrite;
}
/**
*
* Allows grantee to write the ACL for the applicable bucket.
*
*
*
* This is not supported by Amazon S3 on Outposts buckets.
*
*
*
* @return Allows grantee to write the ACL for the applicable bucket.
*
* This is not supported by Amazon S3 on Outposts buckets.
*
*/
public final String grantWriteACP() {
return grantWriteACP;
}
/**
*
* Specifies whether you want S3 Object Lock to be enabled for the new bucket.
*
*
*
* This is not supported by Amazon S3 on Outposts buckets.
*
*
*
* @return Specifies whether you want S3 Object Lock to be enabled for the new bucket.
*
* This is not supported by Amazon S3 on Outposts buckets.
*
*/
public final Boolean objectLockEnabledForBucket() {
return objectLockEnabledForBucket;
}
/**
*
* The ID of the Outposts where the bucket is being created.
*
*
*
* This is required by Amazon S3 on Outposts buckets.
*
*
*
* @return The ID of the Outposts where the bucket is being created.
*
* This is required by Amazon S3 on Outposts buckets.
*
*/
public final String outpostId() {
return outpostId;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(aclAsString());
hashCode = 31 * hashCode + Objects.hashCode(bucket());
hashCode = 31 * hashCode + Objects.hashCode(createBucketConfiguration());
hashCode = 31 * hashCode + Objects.hashCode(grantFullControl());
hashCode = 31 * hashCode + Objects.hashCode(grantRead());
hashCode = 31 * hashCode + Objects.hashCode(grantReadACP());
hashCode = 31 * hashCode + Objects.hashCode(grantWrite());
hashCode = 31 * hashCode + Objects.hashCode(grantWriteACP());
hashCode = 31 * hashCode + Objects.hashCode(objectLockEnabledForBucket());
hashCode = 31 * hashCode + Objects.hashCode(outpostId());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CreateBucketRequest)) {
return false;
}
CreateBucketRequest other = (CreateBucketRequest) obj;
return Objects.equals(aclAsString(), other.aclAsString()) && Objects.equals(bucket(), other.bucket())
&& Objects.equals(createBucketConfiguration(), other.createBucketConfiguration())
&& Objects.equals(grantFullControl(), other.grantFullControl()) && Objects.equals(grantRead(), other.grantRead())
&& Objects.equals(grantReadACP(), other.grantReadACP()) && Objects.equals(grantWrite(), other.grantWrite())
&& Objects.equals(grantWriteACP(), other.grantWriteACP())
&& Objects.equals(objectLockEnabledForBucket(), other.objectLockEnabledForBucket())
&& Objects.equals(outpostId(), other.outpostId());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("CreateBucketRequest").add("ACL", aclAsString()).add("Bucket", bucket())
.add("CreateBucketConfiguration", createBucketConfiguration()).add("GrantFullControl", grantFullControl())
.add("GrantRead", grantRead()).add("GrantReadACP", grantReadACP()).add("GrantWrite", grantWrite())
.add("GrantWriteACP", grantWriteACP()).add("ObjectLockEnabledForBucket", objectLockEnabledForBucket())
.add("OutpostId", outpostId()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ACL":
return Optional.ofNullable(clazz.cast(aclAsString()));
case "Bucket":
return Optional.ofNullable(clazz.cast(bucket()));
case "CreateBucketConfiguration":
return Optional.ofNullable(clazz.cast(createBucketConfiguration()));
case "GrantFullControl":
return Optional.ofNullable(clazz.cast(grantFullControl()));
case "GrantRead":
return Optional.ofNullable(clazz.cast(grantRead()));
case "GrantReadACP":
return Optional.ofNullable(clazz.cast(grantReadACP()));
case "GrantWrite":
return Optional.ofNullable(clazz.cast(grantWrite()));
case "GrantWriteACP":
return Optional.ofNullable(clazz.cast(grantWriteACP()));
case "ObjectLockEnabledForBucket":
return Optional.ofNullable(clazz.cast(objectLockEnabledForBucket()));
case "OutpostId":
return Optional.ofNullable(clazz.cast(outpostId()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function