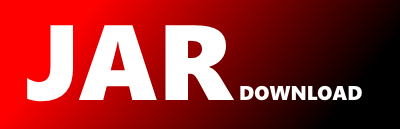
software.amazon.awssdk.services.s3control.model.JobDescriptor Maven / Gradle / Ivy
Show all versions of s3control Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.s3control.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* A container element for the job configuration and status information returned by a Describe Job
request.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class JobDescriptor implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField JOB_ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("JobId")
.getter(getter(JobDescriptor::jobId))
.setter(setter(Builder::jobId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("JobId")
.unmarshallLocationName("JobId").build()).build();
private static final SdkField CONFIRMATION_REQUIRED_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("ConfirmationRequired")
.getter(getter(JobDescriptor::confirmationRequired))
.setter(setter(Builder::confirmationRequired))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ConfirmationRequired")
.unmarshallLocationName("ConfirmationRequired").build()).build();
private static final SdkField DESCRIPTION_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("Description")
.getter(getter(JobDescriptor::description))
.setter(setter(Builder::description))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Description")
.unmarshallLocationName("Description").build()).build();
private static final SdkField JOB_ARN_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("JobArn")
.getter(getter(JobDescriptor::jobArn))
.setter(setter(Builder::jobArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("JobArn")
.unmarshallLocationName("JobArn").build()).build();
private static final SdkField STATUS_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("Status")
.getter(getter(JobDescriptor::statusAsString))
.setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Status")
.unmarshallLocationName("Status").build()).build();
private static final SdkField MANIFEST_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("Manifest")
.getter(getter(JobDescriptor::manifest))
.setter(setter(Builder::manifest))
.constructor(JobManifest::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Manifest")
.unmarshallLocationName("Manifest").build()).build();
private static final SdkField OPERATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("Operation")
.getter(getter(JobDescriptor::operation))
.setter(setter(Builder::operation))
.constructor(JobOperation::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Operation")
.unmarshallLocationName("Operation").build()).build();
private static final SdkField PRIORITY_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("Priority")
.getter(getter(JobDescriptor::priority))
.setter(setter(Builder::priority))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Priority")
.unmarshallLocationName("Priority").build()).build();
private static final SdkField PROGRESS_SUMMARY_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("ProgressSummary")
.getter(getter(JobDescriptor::progressSummary))
.setter(setter(Builder::progressSummary))
.constructor(JobProgressSummary::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ProgressSummary")
.unmarshallLocationName("ProgressSummary").build()).build();
private static final SdkField STATUS_UPDATE_REASON_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("StatusUpdateReason")
.getter(getter(JobDescriptor::statusUpdateReason))
.setter(setter(Builder::statusUpdateReason))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StatusUpdateReason")
.unmarshallLocationName("StatusUpdateReason").build()).build();
private static final SdkField> FAILURE_REASONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("FailureReasons")
.getter(getter(JobDescriptor::failureReasons))
.setter(setter(Builder::failureReasons))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("FailureReasons")
.unmarshallLocationName("FailureReasons").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(JobFailure::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").unmarshallLocationName("member").build()).build())
.build()).build();
private static final SdkField REPORT_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("Report")
.getter(getter(JobDescriptor::report))
.setter(setter(Builder::report))
.constructor(JobReport::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Report")
.unmarshallLocationName("Report").build()).build();
private static final SdkField CREATION_TIME_FIELD = SdkField
. builder(MarshallingType.INSTANT)
.memberName("CreationTime")
.getter(getter(JobDescriptor::creationTime))
.setter(setter(Builder::creationTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CreationTime")
.unmarshallLocationName("CreationTime").build()).build();
private static final SdkField TERMINATION_DATE_FIELD = SdkField
. builder(MarshallingType.INSTANT)
.memberName("TerminationDate")
.getter(getter(JobDescriptor::terminationDate))
.setter(setter(Builder::terminationDate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TerminationDate")
.unmarshallLocationName("TerminationDate").build()).build();
private static final SdkField ROLE_ARN_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("RoleArn")
.getter(getter(JobDescriptor::roleArn))
.setter(setter(Builder::roleArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RoleArn")
.unmarshallLocationName("RoleArn").build()).build();
private static final SdkField SUSPENDED_DATE_FIELD = SdkField
. builder(MarshallingType.INSTANT)
.memberName("SuspendedDate")
.getter(getter(JobDescriptor::suspendedDate))
.setter(setter(Builder::suspendedDate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SuspendedDate")
.unmarshallLocationName("SuspendedDate").build()).build();
private static final SdkField SUSPENDED_CAUSE_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("SuspendedCause")
.getter(getter(JobDescriptor::suspendedCause))
.setter(setter(Builder::suspendedCause))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SuspendedCause")
.unmarshallLocationName("SuspendedCause").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(JOB_ID_FIELD,
CONFIRMATION_REQUIRED_FIELD, DESCRIPTION_FIELD, JOB_ARN_FIELD, STATUS_FIELD, MANIFEST_FIELD, OPERATION_FIELD,
PRIORITY_FIELD, PROGRESS_SUMMARY_FIELD, STATUS_UPDATE_REASON_FIELD, FAILURE_REASONS_FIELD, REPORT_FIELD,
CREATION_TIME_FIELD, TERMINATION_DATE_FIELD, ROLE_ARN_FIELD, SUSPENDED_DATE_FIELD, SUSPENDED_CAUSE_FIELD));
private static final long serialVersionUID = 1L;
private final String jobId;
private final Boolean confirmationRequired;
private final String description;
private final String jobArn;
private final String status;
private final JobManifest manifest;
private final JobOperation operation;
private final Integer priority;
private final JobProgressSummary progressSummary;
private final String statusUpdateReason;
private final List failureReasons;
private final JobReport report;
private final Instant creationTime;
private final Instant terminationDate;
private final String roleArn;
private final Instant suspendedDate;
private final String suspendedCause;
private JobDescriptor(BuilderImpl builder) {
this.jobId = builder.jobId;
this.confirmationRequired = builder.confirmationRequired;
this.description = builder.description;
this.jobArn = builder.jobArn;
this.status = builder.status;
this.manifest = builder.manifest;
this.operation = builder.operation;
this.priority = builder.priority;
this.progressSummary = builder.progressSummary;
this.statusUpdateReason = builder.statusUpdateReason;
this.failureReasons = builder.failureReasons;
this.report = builder.report;
this.creationTime = builder.creationTime;
this.terminationDate = builder.terminationDate;
this.roleArn = builder.roleArn;
this.suspendedDate = builder.suspendedDate;
this.suspendedCause = builder.suspendedCause;
}
/**
*
* The ID for the specified job.
*
*
* @return The ID for the specified job.
*/
public final String jobId() {
return jobId;
}
/**
*
* Indicates whether confirmation is required before Amazon S3 begins running the specified job. Confirmation is
* required only for jobs created through the Amazon S3 console.
*
*
* @return Indicates whether confirmation is required before Amazon S3 begins running the specified job.
* Confirmation is required only for jobs created through the Amazon S3 console.
*/
public final Boolean confirmationRequired() {
return confirmationRequired;
}
/**
*
* The description for this job, if one was provided in this job's Create Job
request.
*
*
* @return The description for this job, if one was provided in this job's Create Job
request.
*/
public final String description() {
return description;
}
/**
*
* The Amazon Resource Name (ARN) for this job.
*
*
* @return The Amazon Resource Name (ARN) for this job.
*/
public final String jobArn() {
return jobArn;
}
/**
*
* The current status of the specified job.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link JobStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return The current status of the specified job.
* @see JobStatus
*/
public final JobStatus status() {
return JobStatus.fromValue(status);
}
/**
*
* The current status of the specified job.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link JobStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return The current status of the specified job.
* @see JobStatus
*/
public final String statusAsString() {
return status;
}
/**
*
* The configuration information for the specified job's manifest object.
*
*
* @return The configuration information for the specified job's manifest object.
*/
public final JobManifest manifest() {
return manifest;
}
/**
*
* The operation that the specified job is configured to run on the objects listed in the manifest.
*
*
* @return The operation that the specified job is configured to run on the objects listed in the manifest.
*/
public final JobOperation operation() {
return operation;
}
/**
*
* The priority of the specified job.
*
*
* @return The priority of the specified job.
*/
public final Integer priority() {
return priority;
}
/**
*
* Describes the total number of tasks that the specified job has run, the number of tasks that succeeded, and the
* number of tasks that failed.
*
*
* @return Describes the total number of tasks that the specified job has run, the number of tasks that succeeded,
* and the number of tasks that failed.
*/
public final JobProgressSummary progressSummary() {
return progressSummary;
}
/**
*
* The reason for updating the job.
*
*
* @return The reason for updating the job.
*/
public final String statusUpdateReason() {
return statusUpdateReason;
}
/**
* Returns true if the FailureReasons property was specified by the sender (it may be empty), or false if the sender
* did not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS service.
*/
public final boolean hasFailureReasons() {
return failureReasons != null && !(failureReasons instanceof SdkAutoConstructList);
}
/**
*
* If the specified job failed, this field contains information describing the failure.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasFailureReasons()} to see if a value was sent in this field.
*
*
* @return If the specified job failed, this field contains information describing the failure.
*/
public final List failureReasons() {
return failureReasons;
}
/**
*
* Contains the configuration information for the job-completion report if you requested one in the
* Create Job
request.
*
*
* @return Contains the configuration information for the job-completion report if you requested one in the
* Create Job
request.
*/
public final JobReport report() {
return report;
}
/**
*
* A timestamp indicating when this job was created.
*
*
* @return A timestamp indicating when this job was created.
*/
public final Instant creationTime() {
return creationTime;
}
/**
*
* A timestamp indicating when this job terminated. A job's termination date is the date and time when it succeeded,
* failed, or was canceled.
*
*
* @return A timestamp indicating when this job terminated. A job's termination date is the date and time when it
* succeeded, failed, or was canceled.
*/
public final Instant terminationDate() {
return terminationDate;
}
/**
*
* The Amazon Resource Name (ARN) for the AWS Identity and Access Management (IAM) role assigned to run the tasks
* for this job.
*
*
* @return The Amazon Resource Name (ARN) for the AWS Identity and Access Management (IAM) role assigned to run the
* tasks for this job.
*/
public final String roleArn() {
return roleArn;
}
/**
*
* The timestamp when this job was suspended, if it has been suspended.
*
*
* @return The timestamp when this job was suspended, if it has been suspended.
*/
public final Instant suspendedDate() {
return suspendedDate;
}
/**
*
* The reason why the specified job was suspended. A job is only suspended if you create it through the Amazon S3
* console. When you create the job, it enters the Suspended
state to await confirmation before
* running. After you confirm the job, it automatically exits the Suspended
state.
*
*
* @return The reason why the specified job was suspended. A job is only suspended if you create it through the
* Amazon S3 console. When you create the job, it enters the Suspended
state to await
* confirmation before running. After you confirm the job, it automatically exits the Suspended
* state.
*/
public final String suspendedCause() {
return suspendedCause;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(jobId());
hashCode = 31 * hashCode + Objects.hashCode(confirmationRequired());
hashCode = 31 * hashCode + Objects.hashCode(description());
hashCode = 31 * hashCode + Objects.hashCode(jobArn());
hashCode = 31 * hashCode + Objects.hashCode(statusAsString());
hashCode = 31 * hashCode + Objects.hashCode(manifest());
hashCode = 31 * hashCode + Objects.hashCode(operation());
hashCode = 31 * hashCode + Objects.hashCode(priority());
hashCode = 31 * hashCode + Objects.hashCode(progressSummary());
hashCode = 31 * hashCode + Objects.hashCode(statusUpdateReason());
hashCode = 31 * hashCode + Objects.hashCode(hasFailureReasons() ? failureReasons() : null);
hashCode = 31 * hashCode + Objects.hashCode(report());
hashCode = 31 * hashCode + Objects.hashCode(creationTime());
hashCode = 31 * hashCode + Objects.hashCode(terminationDate());
hashCode = 31 * hashCode + Objects.hashCode(roleArn());
hashCode = 31 * hashCode + Objects.hashCode(suspendedDate());
hashCode = 31 * hashCode + Objects.hashCode(suspendedCause());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof JobDescriptor)) {
return false;
}
JobDescriptor other = (JobDescriptor) obj;
return Objects.equals(jobId(), other.jobId()) && Objects.equals(confirmationRequired(), other.confirmationRequired())
&& Objects.equals(description(), other.description()) && Objects.equals(jobArn(), other.jobArn())
&& Objects.equals(statusAsString(), other.statusAsString()) && Objects.equals(manifest(), other.manifest())
&& Objects.equals(operation(), other.operation()) && Objects.equals(priority(), other.priority())
&& Objects.equals(progressSummary(), other.progressSummary())
&& Objects.equals(statusUpdateReason(), other.statusUpdateReason())
&& hasFailureReasons() == other.hasFailureReasons() && Objects.equals(failureReasons(), other.failureReasons())
&& Objects.equals(report(), other.report()) && Objects.equals(creationTime(), other.creationTime())
&& Objects.equals(terminationDate(), other.terminationDate()) && Objects.equals(roleArn(), other.roleArn())
&& Objects.equals(suspendedDate(), other.suspendedDate())
&& Objects.equals(suspendedCause(), other.suspendedCause());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("JobDescriptor").add("JobId", jobId()).add("ConfirmationRequired", confirmationRequired())
.add("Description", description()).add("JobArn", jobArn()).add("Status", statusAsString())
.add("Manifest", manifest()).add("Operation", operation()).add("Priority", priority())
.add("ProgressSummary", progressSummary()).add("StatusUpdateReason", statusUpdateReason())
.add("FailureReasons", hasFailureReasons() ? failureReasons() : null).add("Report", report())
.add("CreationTime", creationTime()).add("TerminationDate", terminationDate()).add("RoleArn", roleArn())
.add("SuspendedDate", suspendedDate()).add("SuspendedCause", suspendedCause()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "JobId":
return Optional.ofNullable(clazz.cast(jobId()));
case "ConfirmationRequired":
return Optional.ofNullable(clazz.cast(confirmationRequired()));
case "Description":
return Optional.ofNullable(clazz.cast(description()));
case "JobArn":
return Optional.ofNullable(clazz.cast(jobArn()));
case "Status":
return Optional.ofNullable(clazz.cast(statusAsString()));
case "Manifest":
return Optional.ofNullable(clazz.cast(manifest()));
case "Operation":
return Optional.ofNullable(clazz.cast(operation()));
case "Priority":
return Optional.ofNullable(clazz.cast(priority()));
case "ProgressSummary":
return Optional.ofNullable(clazz.cast(progressSummary()));
case "StatusUpdateReason":
return Optional.ofNullable(clazz.cast(statusUpdateReason()));
case "FailureReasons":
return Optional.ofNullable(clazz.cast(failureReasons()));
case "Report":
return Optional.ofNullable(clazz.cast(report()));
case "CreationTime":
return Optional.ofNullable(clazz.cast(creationTime()));
case "TerminationDate":
return Optional.ofNullable(clazz.cast(terminationDate()));
case "RoleArn":
return Optional.ofNullable(clazz.cast(roleArn()));
case "SuspendedDate":
return Optional.ofNullable(clazz.cast(suspendedDate()));
case "SuspendedCause":
return Optional.ofNullable(clazz.cast(suspendedCause()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function