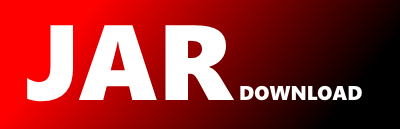
software.amazon.awssdk.services.s3control.model.JobOperation Maven / Gradle / Ivy
Show all versions of s3control Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.s3control.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The operation that you want this job to perform on every object listed in the manifest. For more information about
* the available operations, see Operations in the Amazon
* Simple Storage Service User Guide.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class JobOperation implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField LAMBDA_INVOKE_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("LambdaInvoke")
.getter(getter(JobOperation::lambdaInvoke))
.setter(setter(Builder::lambdaInvoke))
.constructor(LambdaInvokeOperation::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LambdaInvoke")
.unmarshallLocationName("LambdaInvoke").build()).build();
private static final SdkField S3_PUT_OBJECT_COPY_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("S3PutObjectCopy")
.getter(getter(JobOperation::s3PutObjectCopy))
.setter(setter(Builder::s3PutObjectCopy))
.constructor(S3CopyObjectOperation::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("S3PutObjectCopy")
.unmarshallLocationName("S3PutObjectCopy").build()).build();
private static final SdkField S3_PUT_OBJECT_ACL_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("S3PutObjectAcl")
.getter(getter(JobOperation::s3PutObjectAcl))
.setter(setter(Builder::s3PutObjectAcl))
.constructor(S3SetObjectAclOperation::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("S3PutObjectAcl")
.unmarshallLocationName("S3PutObjectAcl").build()).build();
private static final SdkField S3_PUT_OBJECT_TAGGING_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("S3PutObjectTagging")
.getter(getter(JobOperation::s3PutObjectTagging))
.setter(setter(Builder::s3PutObjectTagging))
.constructor(S3SetObjectTaggingOperation::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("S3PutObjectTagging")
.unmarshallLocationName("S3PutObjectTagging").build()).build();
private static final SdkField S3_DELETE_OBJECT_TAGGING_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("S3DeleteObjectTagging")
.getter(getter(JobOperation::s3DeleteObjectTagging))
.setter(setter(Builder::s3DeleteObjectTagging))
.constructor(S3DeleteObjectTaggingOperation::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("S3DeleteObjectTagging")
.unmarshallLocationName("S3DeleteObjectTagging").build()).build();
private static final SdkField S3_INITIATE_RESTORE_OBJECT_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("S3InitiateRestoreObject")
.getter(getter(JobOperation::s3InitiateRestoreObject))
.setter(setter(Builder::s3InitiateRestoreObject))
.constructor(S3InitiateRestoreObjectOperation::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("S3InitiateRestoreObject")
.unmarshallLocationName("S3InitiateRestoreObject").build()).build();
private static final SdkField S3_PUT_OBJECT_LEGAL_HOLD_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("S3PutObjectLegalHold")
.getter(getter(JobOperation::s3PutObjectLegalHold))
.setter(setter(Builder::s3PutObjectLegalHold))
.constructor(S3SetObjectLegalHoldOperation::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("S3PutObjectLegalHold")
.unmarshallLocationName("S3PutObjectLegalHold").build()).build();
private static final SdkField S3_PUT_OBJECT_RETENTION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("S3PutObjectRetention")
.getter(getter(JobOperation::s3PutObjectRetention))
.setter(setter(Builder::s3PutObjectRetention))
.constructor(S3SetObjectRetentionOperation::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("S3PutObjectRetention")
.unmarshallLocationName("S3PutObjectRetention").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(LAMBDA_INVOKE_FIELD,
S3_PUT_OBJECT_COPY_FIELD, S3_PUT_OBJECT_ACL_FIELD, S3_PUT_OBJECT_TAGGING_FIELD, S3_DELETE_OBJECT_TAGGING_FIELD,
S3_INITIATE_RESTORE_OBJECT_FIELD, S3_PUT_OBJECT_LEGAL_HOLD_FIELD, S3_PUT_OBJECT_RETENTION_FIELD));
private static final long serialVersionUID = 1L;
private final LambdaInvokeOperation lambdaInvoke;
private final S3CopyObjectOperation s3PutObjectCopy;
private final S3SetObjectAclOperation s3PutObjectAcl;
private final S3SetObjectTaggingOperation s3PutObjectTagging;
private final S3DeleteObjectTaggingOperation s3DeleteObjectTagging;
private final S3InitiateRestoreObjectOperation s3InitiateRestoreObject;
private final S3SetObjectLegalHoldOperation s3PutObjectLegalHold;
private final S3SetObjectRetentionOperation s3PutObjectRetention;
private JobOperation(BuilderImpl builder) {
this.lambdaInvoke = builder.lambdaInvoke;
this.s3PutObjectCopy = builder.s3PutObjectCopy;
this.s3PutObjectAcl = builder.s3PutObjectAcl;
this.s3PutObjectTagging = builder.s3PutObjectTagging;
this.s3DeleteObjectTagging = builder.s3DeleteObjectTagging;
this.s3InitiateRestoreObject = builder.s3InitiateRestoreObject;
this.s3PutObjectLegalHold = builder.s3PutObjectLegalHold;
this.s3PutObjectRetention = builder.s3PutObjectRetention;
}
/**
*
* Directs the specified job to invoke an AWS Lambda function on every object in the manifest.
*
*
* @return Directs the specified job to invoke an AWS Lambda function on every object in the manifest.
*/
public final LambdaInvokeOperation lambdaInvoke() {
return lambdaInvoke;
}
/**
*
* Directs the specified job to run a PUT Copy object call on every object in the manifest.
*
*
* @return Directs the specified job to run a PUT Copy object call on every object in the manifest.
*/
public final S3CopyObjectOperation s3PutObjectCopy() {
return s3PutObjectCopy;
}
/**
*
* Directs the specified job to run a PUT Object acl call on every object in the manifest.
*
*
* @return Directs the specified job to run a PUT Object acl call on every object in the manifest.
*/
public final S3SetObjectAclOperation s3PutObjectAcl() {
return s3PutObjectAcl;
}
/**
*
* Directs the specified job to run a PUT Object tagging call on every object in the manifest.
*
*
* @return Directs the specified job to run a PUT Object tagging call on every object in the manifest.
*/
public final S3SetObjectTaggingOperation s3PutObjectTagging() {
return s3PutObjectTagging;
}
/**
*
* Directs the specified job to execute a DELETE Object tagging call on every object in the manifest.
*
*
* @return Directs the specified job to execute a DELETE Object tagging call on every object in the manifest.
*/
public final S3DeleteObjectTaggingOperation s3DeleteObjectTagging() {
return s3DeleteObjectTagging;
}
/**
*
* Directs the specified job to initiate restore requests for every archived object in the manifest.
*
*
* @return Directs the specified job to initiate restore requests for every archived object in the manifest.
*/
public final S3InitiateRestoreObjectOperation s3InitiateRestoreObject() {
return s3InitiateRestoreObject;
}
/**
* Returns the value of the S3PutObjectLegalHold property for this object.
*
* @return The value of the S3PutObjectLegalHold property for this object.
*/
public final S3SetObjectLegalHoldOperation s3PutObjectLegalHold() {
return s3PutObjectLegalHold;
}
/**
* Returns the value of the S3PutObjectRetention property for this object.
*
* @return The value of the S3PutObjectRetention property for this object.
*/
public final S3SetObjectRetentionOperation s3PutObjectRetention() {
return s3PutObjectRetention;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(lambdaInvoke());
hashCode = 31 * hashCode + Objects.hashCode(s3PutObjectCopy());
hashCode = 31 * hashCode + Objects.hashCode(s3PutObjectAcl());
hashCode = 31 * hashCode + Objects.hashCode(s3PutObjectTagging());
hashCode = 31 * hashCode + Objects.hashCode(s3DeleteObjectTagging());
hashCode = 31 * hashCode + Objects.hashCode(s3InitiateRestoreObject());
hashCode = 31 * hashCode + Objects.hashCode(s3PutObjectLegalHold());
hashCode = 31 * hashCode + Objects.hashCode(s3PutObjectRetention());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof JobOperation)) {
return false;
}
JobOperation other = (JobOperation) obj;
return Objects.equals(lambdaInvoke(), other.lambdaInvoke()) && Objects.equals(s3PutObjectCopy(), other.s3PutObjectCopy())
&& Objects.equals(s3PutObjectAcl(), other.s3PutObjectAcl())
&& Objects.equals(s3PutObjectTagging(), other.s3PutObjectTagging())
&& Objects.equals(s3DeleteObjectTagging(), other.s3DeleteObjectTagging())
&& Objects.equals(s3InitiateRestoreObject(), other.s3InitiateRestoreObject())
&& Objects.equals(s3PutObjectLegalHold(), other.s3PutObjectLegalHold())
&& Objects.equals(s3PutObjectRetention(), other.s3PutObjectRetention());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("JobOperation").add("LambdaInvoke", lambdaInvoke()).add("S3PutObjectCopy", s3PutObjectCopy())
.add("S3PutObjectAcl", s3PutObjectAcl()).add("S3PutObjectTagging", s3PutObjectTagging())
.add("S3DeleteObjectTagging", s3DeleteObjectTagging()).add("S3InitiateRestoreObject", s3InitiateRestoreObject())
.add("S3PutObjectLegalHold", s3PutObjectLegalHold()).add("S3PutObjectRetention", s3PutObjectRetention()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "LambdaInvoke":
return Optional.ofNullable(clazz.cast(lambdaInvoke()));
case "S3PutObjectCopy":
return Optional.ofNullable(clazz.cast(s3PutObjectCopy()));
case "S3PutObjectAcl":
return Optional.ofNullable(clazz.cast(s3PutObjectAcl()));
case "S3PutObjectTagging":
return Optional.ofNullable(clazz.cast(s3PutObjectTagging()));
case "S3DeleteObjectTagging":
return Optional.ofNullable(clazz.cast(s3DeleteObjectTagging()));
case "S3InitiateRestoreObject":
return Optional.ofNullable(clazz.cast(s3InitiateRestoreObject()));
case "S3PutObjectLegalHold":
return Optional.ofNullable(clazz.cast(s3PutObjectLegalHold()));
case "S3PutObjectRetention":
return Optional.ofNullable(clazz.cast(s3PutObjectRetention()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function