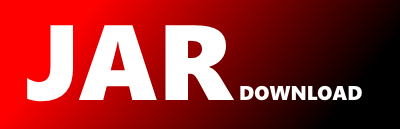
software.amazon.awssdk.services.s3control.model.S3Retention Maven / Gradle / Ivy
Show all versions of s3control Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.s3control.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Contains the S3 Object Lock retention mode to be applied to all objects in the S3 Batch Operations job. If you don't
* provide Mode
and RetainUntilDate
data types in your operation, you will remove the
* retention from your objects. For more information, see Using S3 Object Lock retention
* with S3 Batch Operations in the Amazon Simple Storage Service User Guide.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class S3Retention implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField RETAIN_UNTIL_DATE_FIELD = SdkField
. builder(MarshallingType.INSTANT)
.memberName("RetainUntilDate")
.getter(getter(S3Retention::retainUntilDate))
.setter(setter(Builder::retainUntilDate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RetainUntilDate")
.unmarshallLocationName("RetainUntilDate").build()).build();
private static final SdkField MODE_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("Mode")
.getter(getter(S3Retention::modeAsString))
.setter(setter(Builder::mode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Mode")
.unmarshallLocationName("Mode").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(RETAIN_UNTIL_DATE_FIELD,
MODE_FIELD));
private static final long serialVersionUID = 1L;
private final Instant retainUntilDate;
private final String mode;
private S3Retention(BuilderImpl builder) {
this.retainUntilDate = builder.retainUntilDate;
this.mode = builder.mode;
}
/**
*
* The date when the applied Object Lock retention will expire on all objects set by the Batch Operations job.
*
*
* @return The date when the applied Object Lock retention will expire on all objects set by the Batch Operations
* job.
*/
public final Instant retainUntilDate() {
return retainUntilDate;
}
/**
*
* The Object Lock retention mode to be applied to all objects in the Batch Operations job.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #mode} will return
* {@link S3ObjectLockRetentionMode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #modeAsString}.
*
*
* @return The Object Lock retention mode to be applied to all objects in the Batch Operations job.
* @see S3ObjectLockRetentionMode
*/
public final S3ObjectLockRetentionMode mode() {
return S3ObjectLockRetentionMode.fromValue(mode);
}
/**
*
* The Object Lock retention mode to be applied to all objects in the Batch Operations job.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #mode} will return
* {@link S3ObjectLockRetentionMode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #modeAsString}.
*
*
* @return The Object Lock retention mode to be applied to all objects in the Batch Operations job.
* @see S3ObjectLockRetentionMode
*/
public final String modeAsString() {
return mode;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(retainUntilDate());
hashCode = 31 * hashCode + Objects.hashCode(modeAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof S3Retention)) {
return false;
}
S3Retention other = (S3Retention) obj;
return Objects.equals(retainUntilDate(), other.retainUntilDate()) && Objects.equals(modeAsString(), other.modeAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("S3Retention").add("RetainUntilDate", retainUntilDate()).add("Mode", modeAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "RetainUntilDate":
return Optional.ofNullable(clazz.cast(retainUntilDate()));
case "Mode":
return Optional.ofNullable(clazz.cast(modeAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function