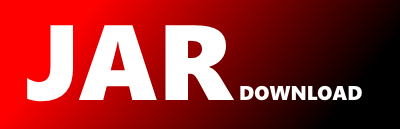
software.amazon.awssdk.services.s3control.S3ControlClient Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.s3control;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkPublicApi;
import software.amazon.awssdk.annotations.ThreadSafe;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.SdkClient;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.regions.ServiceMetadata;
import software.amazon.awssdk.services.s3control.model.BadRequestException;
import software.amazon.awssdk.services.s3control.model.BucketAlreadyExistsException;
import software.amazon.awssdk.services.s3control.model.BucketAlreadyOwnedByYouException;
import software.amazon.awssdk.services.s3control.model.CreateAccessPointForObjectLambdaRequest;
import software.amazon.awssdk.services.s3control.model.CreateAccessPointForObjectLambdaResponse;
import software.amazon.awssdk.services.s3control.model.CreateAccessPointRequest;
import software.amazon.awssdk.services.s3control.model.CreateAccessPointResponse;
import software.amazon.awssdk.services.s3control.model.CreateBucketRequest;
import software.amazon.awssdk.services.s3control.model.CreateBucketResponse;
import software.amazon.awssdk.services.s3control.model.CreateJobRequest;
import software.amazon.awssdk.services.s3control.model.CreateJobResponse;
import software.amazon.awssdk.services.s3control.model.DeleteAccessPointForObjectLambdaRequest;
import software.amazon.awssdk.services.s3control.model.DeleteAccessPointForObjectLambdaResponse;
import software.amazon.awssdk.services.s3control.model.DeleteAccessPointPolicyForObjectLambdaRequest;
import software.amazon.awssdk.services.s3control.model.DeleteAccessPointPolicyForObjectLambdaResponse;
import software.amazon.awssdk.services.s3control.model.DeleteAccessPointPolicyRequest;
import software.amazon.awssdk.services.s3control.model.DeleteAccessPointPolicyResponse;
import software.amazon.awssdk.services.s3control.model.DeleteAccessPointRequest;
import software.amazon.awssdk.services.s3control.model.DeleteAccessPointResponse;
import software.amazon.awssdk.services.s3control.model.DeleteBucketLifecycleConfigurationRequest;
import software.amazon.awssdk.services.s3control.model.DeleteBucketLifecycleConfigurationResponse;
import software.amazon.awssdk.services.s3control.model.DeleteBucketPolicyRequest;
import software.amazon.awssdk.services.s3control.model.DeleteBucketPolicyResponse;
import software.amazon.awssdk.services.s3control.model.DeleteBucketRequest;
import software.amazon.awssdk.services.s3control.model.DeleteBucketResponse;
import software.amazon.awssdk.services.s3control.model.DeleteBucketTaggingRequest;
import software.amazon.awssdk.services.s3control.model.DeleteBucketTaggingResponse;
import software.amazon.awssdk.services.s3control.model.DeleteJobTaggingRequest;
import software.amazon.awssdk.services.s3control.model.DeleteJobTaggingResponse;
import software.amazon.awssdk.services.s3control.model.DeletePublicAccessBlockRequest;
import software.amazon.awssdk.services.s3control.model.DeletePublicAccessBlockResponse;
import software.amazon.awssdk.services.s3control.model.DeleteStorageLensConfigurationRequest;
import software.amazon.awssdk.services.s3control.model.DeleteStorageLensConfigurationResponse;
import software.amazon.awssdk.services.s3control.model.DeleteStorageLensConfigurationTaggingRequest;
import software.amazon.awssdk.services.s3control.model.DeleteStorageLensConfigurationTaggingResponse;
import software.amazon.awssdk.services.s3control.model.DescribeJobRequest;
import software.amazon.awssdk.services.s3control.model.DescribeJobResponse;
import software.amazon.awssdk.services.s3control.model.GetAccessPointConfigurationForObjectLambdaRequest;
import software.amazon.awssdk.services.s3control.model.GetAccessPointConfigurationForObjectLambdaResponse;
import software.amazon.awssdk.services.s3control.model.GetAccessPointForObjectLambdaRequest;
import software.amazon.awssdk.services.s3control.model.GetAccessPointForObjectLambdaResponse;
import software.amazon.awssdk.services.s3control.model.GetAccessPointPolicyForObjectLambdaRequest;
import software.amazon.awssdk.services.s3control.model.GetAccessPointPolicyForObjectLambdaResponse;
import software.amazon.awssdk.services.s3control.model.GetAccessPointPolicyRequest;
import software.amazon.awssdk.services.s3control.model.GetAccessPointPolicyResponse;
import software.amazon.awssdk.services.s3control.model.GetAccessPointPolicyStatusForObjectLambdaRequest;
import software.amazon.awssdk.services.s3control.model.GetAccessPointPolicyStatusForObjectLambdaResponse;
import software.amazon.awssdk.services.s3control.model.GetAccessPointPolicyStatusRequest;
import software.amazon.awssdk.services.s3control.model.GetAccessPointPolicyStatusResponse;
import software.amazon.awssdk.services.s3control.model.GetAccessPointRequest;
import software.amazon.awssdk.services.s3control.model.GetAccessPointResponse;
import software.amazon.awssdk.services.s3control.model.GetBucketLifecycleConfigurationRequest;
import software.amazon.awssdk.services.s3control.model.GetBucketLifecycleConfigurationResponse;
import software.amazon.awssdk.services.s3control.model.GetBucketPolicyRequest;
import software.amazon.awssdk.services.s3control.model.GetBucketPolicyResponse;
import software.amazon.awssdk.services.s3control.model.GetBucketRequest;
import software.amazon.awssdk.services.s3control.model.GetBucketResponse;
import software.amazon.awssdk.services.s3control.model.GetBucketTaggingRequest;
import software.amazon.awssdk.services.s3control.model.GetBucketTaggingResponse;
import software.amazon.awssdk.services.s3control.model.GetJobTaggingRequest;
import software.amazon.awssdk.services.s3control.model.GetJobTaggingResponse;
import software.amazon.awssdk.services.s3control.model.GetPublicAccessBlockRequest;
import software.amazon.awssdk.services.s3control.model.GetPublicAccessBlockResponse;
import software.amazon.awssdk.services.s3control.model.GetStorageLensConfigurationRequest;
import software.amazon.awssdk.services.s3control.model.GetStorageLensConfigurationResponse;
import software.amazon.awssdk.services.s3control.model.GetStorageLensConfigurationTaggingRequest;
import software.amazon.awssdk.services.s3control.model.GetStorageLensConfigurationTaggingResponse;
import software.amazon.awssdk.services.s3control.model.IdempotencyException;
import software.amazon.awssdk.services.s3control.model.InternalServiceException;
import software.amazon.awssdk.services.s3control.model.InvalidNextTokenException;
import software.amazon.awssdk.services.s3control.model.InvalidRequestException;
import software.amazon.awssdk.services.s3control.model.JobStatusException;
import software.amazon.awssdk.services.s3control.model.ListAccessPointsForObjectLambdaRequest;
import software.amazon.awssdk.services.s3control.model.ListAccessPointsForObjectLambdaResponse;
import software.amazon.awssdk.services.s3control.model.ListAccessPointsRequest;
import software.amazon.awssdk.services.s3control.model.ListAccessPointsResponse;
import software.amazon.awssdk.services.s3control.model.ListJobsRequest;
import software.amazon.awssdk.services.s3control.model.ListJobsResponse;
import software.amazon.awssdk.services.s3control.model.ListRegionalBucketsRequest;
import software.amazon.awssdk.services.s3control.model.ListRegionalBucketsResponse;
import software.amazon.awssdk.services.s3control.model.ListStorageLensConfigurationsRequest;
import software.amazon.awssdk.services.s3control.model.ListStorageLensConfigurationsResponse;
import software.amazon.awssdk.services.s3control.model.NoSuchPublicAccessBlockConfigurationException;
import software.amazon.awssdk.services.s3control.model.NotFoundException;
import software.amazon.awssdk.services.s3control.model.PutAccessPointConfigurationForObjectLambdaRequest;
import software.amazon.awssdk.services.s3control.model.PutAccessPointConfigurationForObjectLambdaResponse;
import software.amazon.awssdk.services.s3control.model.PutAccessPointPolicyForObjectLambdaRequest;
import software.amazon.awssdk.services.s3control.model.PutAccessPointPolicyForObjectLambdaResponse;
import software.amazon.awssdk.services.s3control.model.PutAccessPointPolicyRequest;
import software.amazon.awssdk.services.s3control.model.PutAccessPointPolicyResponse;
import software.amazon.awssdk.services.s3control.model.PutBucketLifecycleConfigurationRequest;
import software.amazon.awssdk.services.s3control.model.PutBucketLifecycleConfigurationResponse;
import software.amazon.awssdk.services.s3control.model.PutBucketPolicyRequest;
import software.amazon.awssdk.services.s3control.model.PutBucketPolicyResponse;
import software.amazon.awssdk.services.s3control.model.PutBucketTaggingRequest;
import software.amazon.awssdk.services.s3control.model.PutBucketTaggingResponse;
import software.amazon.awssdk.services.s3control.model.PutJobTaggingRequest;
import software.amazon.awssdk.services.s3control.model.PutJobTaggingResponse;
import software.amazon.awssdk.services.s3control.model.PutPublicAccessBlockRequest;
import software.amazon.awssdk.services.s3control.model.PutPublicAccessBlockResponse;
import software.amazon.awssdk.services.s3control.model.PutStorageLensConfigurationRequest;
import software.amazon.awssdk.services.s3control.model.PutStorageLensConfigurationResponse;
import software.amazon.awssdk.services.s3control.model.PutStorageLensConfigurationTaggingRequest;
import software.amazon.awssdk.services.s3control.model.PutStorageLensConfigurationTaggingResponse;
import software.amazon.awssdk.services.s3control.model.S3ControlException;
import software.amazon.awssdk.services.s3control.model.TooManyRequestsException;
import software.amazon.awssdk.services.s3control.model.TooManyTagsException;
import software.amazon.awssdk.services.s3control.model.UpdateJobPriorityRequest;
import software.amazon.awssdk.services.s3control.model.UpdateJobPriorityResponse;
import software.amazon.awssdk.services.s3control.model.UpdateJobStatusRequest;
import software.amazon.awssdk.services.s3control.model.UpdateJobStatusResponse;
import software.amazon.awssdk.services.s3control.paginators.ListAccessPointsForObjectLambdaIterable;
import software.amazon.awssdk.services.s3control.paginators.ListAccessPointsIterable;
import software.amazon.awssdk.services.s3control.paginators.ListJobsIterable;
import software.amazon.awssdk.services.s3control.paginators.ListRegionalBucketsIterable;
import software.amazon.awssdk.services.s3control.paginators.ListStorageLensConfigurationsIterable;
/**
* Service client for accessing AWS S3 Control. This can be created using the static {@link #builder()} method.
*
*
* AWS S3 Control provides access to Amazon S3 control plane actions.
*
*/
@Generated("software.amazon.awssdk:codegen")
@SdkPublicApi
@ThreadSafe
public interface S3ControlClient extends SdkClient {
String SERVICE_NAME = "s3";
/**
* Value for looking up the service's metadata from the
* {@link software.amazon.awssdk.regions.ServiceMetadataProvider}.
*/
String SERVICE_METADATA_ID = "s3-control";
/**
* Create a {@link S3ControlClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static S3ControlClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link S3ControlClient}.
*/
static S3ControlClientBuilder builder() {
return new DefaultS3ControlClientBuilder();
}
/**
*
* Creates an access point and associates it with the specified bucket. For more information, see Managing Data Access with Amazon
* S3 Access Points in the Amazon Simple Storage Service User Guide.
*
*
*
*
* S3 on Outposts only supports VPC-style Access Points.
*
*
* For more information, see
* Accessing Amazon S3 on Outposts using virtual private cloud (VPC) only Access Points in the Amazon Simple
* Storage Service User Guide.
*
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request and an S3 on Outposts endpoint hostname prefix
* instead of s3-control
. For an example of the request syntax for Amazon S3 on Outposts that uses the
* S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived using the access point ARN,
* see the Examples section.
*
*
*
* The following actions are related to CreateAccessPoint
:
*
*
* -
*
* GetAccessPoint
*
*
* -
*
*
* -
*
* ListAccessPoints
*
*
*
*
* @param createAccessPointRequest
* @return Result of the CreateAccessPoint operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.CreateAccessPoint
* @see AWS
* API Documentation
*/
default CreateAccessPointResponse createAccessPoint(CreateAccessPointRequest createAccessPointRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
throw new UnsupportedOperationException();
}
/**
*
* Creates an access point and associates it with the specified bucket. For more information, see Managing Data Access with Amazon
* S3 Access Points in the Amazon Simple Storage Service User Guide.
*
*
*
*
* S3 on Outposts only supports VPC-style Access Points.
*
*
* For more information, see
* Accessing Amazon S3 on Outposts using virtual private cloud (VPC) only Access Points in the Amazon Simple
* Storage Service User Guide.
*
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request and an S3 on Outposts endpoint hostname prefix
* instead of s3-control
. For an example of the request syntax for Amazon S3 on Outposts that uses the
* S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived using the access point ARN,
* see the Examples section.
*
*
*
* The following actions are related to CreateAccessPoint
:
*
*
* -
*
* GetAccessPoint
*
*
* -
*
*
* -
*
* ListAccessPoints
*
*
*
*
*
* This is a convenience which creates an instance of the {@link CreateAccessPointRequest.Builder} avoiding the need
* to create one manually via {@link CreateAccessPointRequest#builder()}
*
*
* @param createAccessPointRequest
* A {@link Consumer} that will call methods on {@link CreateAccessPointRequest.Builder} to create a request.
* @return Result of the CreateAccessPoint operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.CreateAccessPoint
* @see AWS
* API Documentation
*/
default CreateAccessPointResponse createAccessPoint(Consumer createAccessPointRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
return createAccessPoint(CreateAccessPointRequest.builder().applyMutation(createAccessPointRequest).build());
}
/**
*
* Creates an Object Lambda Access Point. For more information, see Transforming objects with
* Object Lambda Access Points in the Amazon Simple Storage Service User Guide.
*
*
* The following actions are related to CreateAccessPointForObjectLambda
:
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* @param createAccessPointForObjectLambdaRequest
* @return Result of the CreateAccessPointForObjectLambda operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.CreateAccessPointForObjectLambda
* @see AWS API Documentation
*/
default CreateAccessPointForObjectLambdaResponse createAccessPointForObjectLambda(
CreateAccessPointForObjectLambdaRequest createAccessPointForObjectLambdaRequest) throws AwsServiceException,
SdkClientException, S3ControlException {
throw new UnsupportedOperationException();
}
/**
*
* Creates an Object Lambda Access Point. For more information, see Transforming objects with
* Object Lambda Access Points in the Amazon Simple Storage Service User Guide.
*
*
* The following actions are related to CreateAccessPointForObjectLambda
:
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
*
* This is a convenience which creates an instance of the {@link CreateAccessPointForObjectLambdaRequest.Builder}
* avoiding the need to create one manually via {@link CreateAccessPointForObjectLambdaRequest#builder()}
*
*
* @param createAccessPointForObjectLambdaRequest
* A {@link Consumer} that will call methods on {@link CreateAccessPointForObjectLambdaRequest.Builder} to
* create a request.
* @return Result of the CreateAccessPointForObjectLambda operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.CreateAccessPointForObjectLambda
* @see AWS API Documentation
*/
default CreateAccessPointForObjectLambdaResponse createAccessPointForObjectLambda(
Consumer createAccessPointForObjectLambdaRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
return createAccessPointForObjectLambda(CreateAccessPointForObjectLambdaRequest.builder()
.applyMutation(createAccessPointForObjectLambdaRequest).build());
}
/**
*
*
* This action creates an Amazon S3 on Outposts bucket. To create an S3 bucket, see Create Bucket in the Amazon
* Simple Storage Service API.
*
*
*
* Creates a new Outposts bucket. By creating the bucket, you become the bucket owner. To create an Outposts bucket,
* you must have S3 on Outposts. For more information, see Using Amazon S3 on Outposts in
* Amazon Simple Storage Service User Guide.
*
*
* Not every string is an acceptable bucket name. For information on bucket naming restrictions, see Working
* with Amazon S3 Buckets.
*
*
* S3 on Outposts buckets support:
*
*
* -
*
* Tags
*
*
* -
*
* LifecycleConfigurations for deleting expired objects
*
*
*
*
* For a complete list of restrictions and Amazon S3 feature limitations on S3 on Outposts, see Amazon S3
* on Outposts Restrictions and Limitations.
*
*
* For an example of the request syntax for Amazon S3 on Outposts that uses the S3 on Outposts endpoint hostname
* prefix and x-amz-outpost-id
in your API request, see the Examples section.
*
*
* The following actions are related to CreateBucket
for Amazon S3 on Outposts:
*
*
* -
*
* PutObject
*
*
* -
*
* GetBucket
*
*
* -
*
* DeleteBucket
*
*
* -
*
*
* -
*
*
*
*
* @param createBucketRequest
* @return Result of the CreateBucket operation returned by the service.
* @throws BucketAlreadyExistsException
* The requested Outposts bucket name is not available. The bucket namespace is shared by all users of the
* AWS Outposts in this Region. Select a different name and try again.
* @throws BucketAlreadyOwnedByYouException
* The Outposts bucket you tried to create already exists, and you own it.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.CreateBucket
* @see AWS API
* Documentation
*/
default CreateBucketResponse createBucket(CreateBucketRequest createBucketRequest) throws BucketAlreadyExistsException,
BucketAlreadyOwnedByYouException, AwsServiceException, SdkClientException, S3ControlException {
throw new UnsupportedOperationException();
}
/**
*
*
* This action creates an Amazon S3 on Outposts bucket. To create an S3 bucket, see Create Bucket in the Amazon
* Simple Storage Service API.
*
*
*
* Creates a new Outposts bucket. By creating the bucket, you become the bucket owner. To create an Outposts bucket,
* you must have S3 on Outposts. For more information, see Using Amazon S3 on Outposts in
* Amazon Simple Storage Service User Guide.
*
*
* Not every string is an acceptable bucket name. For information on bucket naming restrictions, see Working
* with Amazon S3 Buckets.
*
*
* S3 on Outposts buckets support:
*
*
* -
*
* Tags
*
*
* -
*
* LifecycleConfigurations for deleting expired objects
*
*
*
*
* For a complete list of restrictions and Amazon S3 feature limitations on S3 on Outposts, see Amazon S3
* on Outposts Restrictions and Limitations.
*
*
* For an example of the request syntax for Amazon S3 on Outposts that uses the S3 on Outposts endpoint hostname
* prefix and x-amz-outpost-id
in your API request, see the Examples section.
*
*
* The following actions are related to CreateBucket
for Amazon S3 on Outposts:
*
*
* -
*
* PutObject
*
*
* -
*
* GetBucket
*
*
* -
*
* DeleteBucket
*
*
* -
*
*
* -
*
*
*
*
*
* This is a convenience which creates an instance of the {@link CreateBucketRequest.Builder} avoiding the need to
* create one manually via {@link CreateBucketRequest#builder()}
*
*
* @param createBucketRequest
* A {@link Consumer} that will call methods on {@link CreateBucketRequest.Builder} to create a request.
* @return Result of the CreateBucket operation returned by the service.
* @throws BucketAlreadyExistsException
* The requested Outposts bucket name is not available. The bucket namespace is shared by all users of the
* AWS Outposts in this Region. Select a different name and try again.
* @throws BucketAlreadyOwnedByYouException
* The Outposts bucket you tried to create already exists, and you own it.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.CreateBucket
* @see AWS API
* Documentation
*/
default CreateBucketResponse createBucket(Consumer createBucketRequest)
throws BucketAlreadyExistsException, BucketAlreadyOwnedByYouException, AwsServiceException, SdkClientException,
S3ControlException {
return createBucket(CreateBucketRequest.builder().applyMutation(createBucketRequest).build());
}
/**
*
* You can use S3 Batch Operations to perform large-scale batch actions on Amazon S3 objects. Batch Operations can
* run a single action on lists of Amazon S3 objects that you specify. For more information, see S3 Batch Operations in the
* Amazon Simple Storage Service User Guide.
*
*
* This action creates a S3 Batch Operations job.
*
*
*
* Related actions include:
*
*
* -
*
* DescribeJob
*
*
* -
*
* ListJobs
*
*
* -
*
*
* -
*
* UpdateJobStatus
*
*
* -
*
* JobOperation
*
*
*
*
* @param createJobRequest
* @return Result of the CreateJob operation returned by the service.
* @throws TooManyRequestsException
* @throws BadRequestException
* @throws IdempotencyException
* @throws InternalServiceException
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.CreateJob
* @see AWS API
* Documentation
*/
default CreateJobResponse createJob(CreateJobRequest createJobRequest) throws TooManyRequestsException, BadRequestException,
IdempotencyException, InternalServiceException, AwsServiceException, SdkClientException, S3ControlException {
throw new UnsupportedOperationException();
}
/**
*
* You can use S3 Batch Operations to perform large-scale batch actions on Amazon S3 objects. Batch Operations can
* run a single action on lists of Amazon S3 objects that you specify. For more information, see S3 Batch Operations in the
* Amazon Simple Storage Service User Guide.
*
*
* This action creates a S3 Batch Operations job.
*
*
*
* Related actions include:
*
*
* -
*
* DescribeJob
*
*
* -
*
* ListJobs
*
*
* -
*
*
* -
*
* UpdateJobStatus
*
*
* -
*
* JobOperation
*
*
*
*
*
* This is a convenience which creates an instance of the {@link CreateJobRequest.Builder} avoiding the need to
* create one manually via {@link CreateJobRequest#builder()}
*
*
* @param createJobRequest
* A {@link Consumer} that will call methods on {@link CreateJobRequest.Builder} to create a request.
* @return Result of the CreateJob operation returned by the service.
* @throws TooManyRequestsException
* @throws BadRequestException
* @throws IdempotencyException
* @throws InternalServiceException
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.CreateJob
* @see AWS API
* Documentation
*/
default CreateJobResponse createJob(Consumer createJobRequest) throws TooManyRequestsException,
BadRequestException, IdempotencyException, InternalServiceException, AwsServiceException, SdkClientException,
S3ControlException {
return createJob(CreateJobRequest.builder().applyMutation(createJobRequest).build());
}
/**
*
* Deletes the specified access point.
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request and an S3 on Outposts endpoint hostname prefix
* instead of s3-control
. For an example of the request syntax for Amazon S3 on Outposts that uses the
* S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived using the access point ARN,
* see the Examples section.
*
*
* The following actions are related to DeleteAccessPoint
:
*
*
* -
*
*
* -
*
* GetAccessPoint
*
*
* -
*
* ListAccessPoints
*
*
*
*
* @param deleteAccessPointRequest
* @return Result of the DeleteAccessPoint operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.DeleteAccessPoint
* @see AWS
* API Documentation
*/
default DeleteAccessPointResponse deleteAccessPoint(DeleteAccessPointRequest deleteAccessPointRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the specified access point.
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request and an S3 on Outposts endpoint hostname prefix
* instead of s3-control
. For an example of the request syntax for Amazon S3 on Outposts that uses the
* S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived using the access point ARN,
* see the Examples section.
*
*
* The following actions are related to DeleteAccessPoint
:
*
*
* -
*
*
* -
*
* GetAccessPoint
*
*
* -
*
* ListAccessPoints
*
*
*
*
*
* This is a convenience which creates an instance of the {@link DeleteAccessPointRequest.Builder} avoiding the need
* to create one manually via {@link DeleteAccessPointRequest#builder()}
*
*
* @param deleteAccessPointRequest
* A {@link Consumer} that will call methods on {@link DeleteAccessPointRequest.Builder} to create a request.
* @return Result of the DeleteAccessPoint operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.DeleteAccessPoint
* @see AWS
* API Documentation
*/
default DeleteAccessPointResponse deleteAccessPoint(Consumer deleteAccessPointRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
return deleteAccessPoint(DeleteAccessPointRequest.builder().applyMutation(deleteAccessPointRequest).build());
}
/**
*
* Deletes the specified Object Lambda Access Point.
*
*
* The following actions are related to DeleteAccessPointForObjectLambda
:
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* @param deleteAccessPointForObjectLambdaRequest
* @return Result of the DeleteAccessPointForObjectLambda operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.DeleteAccessPointForObjectLambda
* @see AWS API Documentation
*/
default DeleteAccessPointForObjectLambdaResponse deleteAccessPointForObjectLambda(
DeleteAccessPointForObjectLambdaRequest deleteAccessPointForObjectLambdaRequest) throws AwsServiceException,
SdkClientException, S3ControlException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the specified Object Lambda Access Point.
*
*
* The following actions are related to DeleteAccessPointForObjectLambda
:
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
*
* This is a convenience which creates an instance of the {@link DeleteAccessPointForObjectLambdaRequest.Builder}
* avoiding the need to create one manually via {@link DeleteAccessPointForObjectLambdaRequest#builder()}
*
*
* @param deleteAccessPointForObjectLambdaRequest
* A {@link Consumer} that will call methods on {@link DeleteAccessPointForObjectLambdaRequest.Builder} to
* create a request.
* @return Result of the DeleteAccessPointForObjectLambda operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.DeleteAccessPointForObjectLambda
* @see AWS API Documentation
*/
default DeleteAccessPointForObjectLambdaResponse deleteAccessPointForObjectLambda(
Consumer deleteAccessPointForObjectLambdaRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
return deleteAccessPointForObjectLambda(DeleteAccessPointForObjectLambdaRequest.builder()
.applyMutation(deleteAccessPointForObjectLambdaRequest).build());
}
/**
*
* Deletes the access point policy for the specified access point.
*
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request and an S3 on Outposts endpoint hostname prefix
* instead of s3-control
. For an example of the request syntax for Amazon S3 on Outposts that uses the
* S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived using the access point ARN,
* see the Examples section.
*
*
* The following actions are related to DeleteAccessPointPolicy
:
*
*
* -
*
*
* -
*
*
*
*
* @param deleteAccessPointPolicyRequest
* @return Result of the DeleteAccessPointPolicy operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.DeleteAccessPointPolicy
* @see AWS API Documentation
*/
default DeleteAccessPointPolicyResponse deleteAccessPointPolicy(DeleteAccessPointPolicyRequest deleteAccessPointPolicyRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the access point policy for the specified access point.
*
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request and an S3 on Outposts endpoint hostname prefix
* instead of s3-control
. For an example of the request syntax for Amazon S3 on Outposts that uses the
* S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived using the access point ARN,
* see the Examples section.
*
*
* The following actions are related to DeleteAccessPointPolicy
:
*
*
* -
*
*
* -
*
*
*
*
*
* This is a convenience which creates an instance of the {@link DeleteAccessPointPolicyRequest.Builder} avoiding
* the need to create one manually via {@link DeleteAccessPointPolicyRequest#builder()}
*
*
* @param deleteAccessPointPolicyRequest
* A {@link Consumer} that will call methods on {@link DeleteAccessPointPolicyRequest.Builder} to create a
* request.
* @return Result of the DeleteAccessPointPolicy operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.DeleteAccessPointPolicy
* @see AWS API Documentation
*/
default DeleteAccessPointPolicyResponse deleteAccessPointPolicy(
Consumer deleteAccessPointPolicyRequest) throws AwsServiceException,
SdkClientException, S3ControlException {
return deleteAccessPointPolicy(DeleteAccessPointPolicyRequest.builder().applyMutation(deleteAccessPointPolicyRequest)
.build());
}
/**
*
* Removes the resource policy for an Object Lambda Access Point.
*
*
* The following actions are related to DeleteAccessPointPolicyForObjectLambda
:
*
*
* -
*
*
* -
*
*
*
*
* @param deleteAccessPointPolicyForObjectLambdaRequest
* @return Result of the DeleteAccessPointPolicyForObjectLambda operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.DeleteAccessPointPolicyForObjectLambda
* @see AWS API Documentation
*/
default DeleteAccessPointPolicyForObjectLambdaResponse deleteAccessPointPolicyForObjectLambda(
DeleteAccessPointPolicyForObjectLambdaRequest deleteAccessPointPolicyForObjectLambdaRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
throw new UnsupportedOperationException();
}
/**
*
* Removes the resource policy for an Object Lambda Access Point.
*
*
* The following actions are related to DeleteAccessPointPolicyForObjectLambda
:
*
*
* -
*
*
* -
*
*
*
*
*
* This is a convenience which creates an instance of the
* {@link DeleteAccessPointPolicyForObjectLambdaRequest.Builder} avoiding the need to create one manually via
* {@link DeleteAccessPointPolicyForObjectLambdaRequest#builder()}
*
*
* @param deleteAccessPointPolicyForObjectLambdaRequest
* A {@link Consumer} that will call methods on {@link DeleteAccessPointPolicyForObjectLambdaRequest.Builder}
* to create a request.
* @return Result of the DeleteAccessPointPolicyForObjectLambda operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.DeleteAccessPointPolicyForObjectLambda
* @see AWS API Documentation
*/
default DeleteAccessPointPolicyForObjectLambdaResponse deleteAccessPointPolicyForObjectLambda(
Consumer deleteAccessPointPolicyForObjectLambdaRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
return deleteAccessPointPolicyForObjectLambda(DeleteAccessPointPolicyForObjectLambdaRequest.builder()
.applyMutation(deleteAccessPointPolicyForObjectLambdaRequest).build());
}
/**
*
*
* This action deletes an Amazon S3 on Outposts bucket. To delete an S3 bucket, see DeleteBucket in the Amazon
* Simple Storage Service API.
*
*
*
* Deletes the Amazon S3 on Outposts bucket. All objects (including all object versions and delete markers) in the
* bucket must be deleted before the bucket itself can be deleted. For more information, see Using Amazon S3 on Outposts in
* Amazon Simple Storage Service User Guide.
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request and an S3 on Outposts endpoint hostname prefix
* instead of s3-control
. For an example of the request syntax for Amazon S3 on Outposts that uses the
* S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived using the access point ARN,
* see the Examples section.
*
*
* Related Resources
*
*
* -
*
* CreateBucket
*
*
* -
*
* GetBucket
*
*
* -
*
* DeleteObject
*
*
*
*
* @param deleteBucketRequest
* @return Result of the DeleteBucket operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.DeleteBucket
* @see AWS API
* Documentation
*/
default DeleteBucketResponse deleteBucket(DeleteBucketRequest deleteBucketRequest) throws AwsServiceException,
SdkClientException, S3ControlException {
throw new UnsupportedOperationException();
}
/**
*
*
* This action deletes an Amazon S3 on Outposts bucket. To delete an S3 bucket, see DeleteBucket in the Amazon
* Simple Storage Service API.
*
*
*
* Deletes the Amazon S3 on Outposts bucket. All objects (including all object versions and delete markers) in the
* bucket must be deleted before the bucket itself can be deleted. For more information, see Using Amazon S3 on Outposts in
* Amazon Simple Storage Service User Guide.
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request and an S3 on Outposts endpoint hostname prefix
* instead of s3-control
. For an example of the request syntax for Amazon S3 on Outposts that uses the
* S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived using the access point ARN,
* see the Examples section.
*
*
* Related Resources
*
*
* -
*
* CreateBucket
*
*
* -
*
* GetBucket
*
*
* -
*
* DeleteObject
*
*
*
*
*
* This is a convenience which creates an instance of the {@link DeleteBucketRequest.Builder} avoiding the need to
* create one manually via {@link DeleteBucketRequest#builder()}
*
*
* @param deleteBucketRequest
* A {@link Consumer} that will call methods on {@link DeleteBucketRequest.Builder} to create a request.
* @return Result of the DeleteBucket operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.DeleteBucket
* @see AWS API
* Documentation
*/
default DeleteBucketResponse deleteBucket(Consumer deleteBucketRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
return deleteBucket(DeleteBucketRequest.builder().applyMutation(deleteBucketRequest).build());
}
/**
*
*
* This action deletes an Amazon S3 on Outposts bucket's lifecycle configuration. To delete an S3 bucket's lifecycle
* configuration, see DeleteBucketLifecycle
* in the Amazon Simple Storage Service API.
*
*
*
* Deletes the lifecycle configuration from the specified Outposts bucket. Amazon S3 on Outposts removes all the
* lifecycle configuration rules in the lifecycle subresource associated with the bucket. Your objects never expire,
* and Amazon S3 on Outposts no longer automatically deletes any objects on the basis of rules contained in the
* deleted lifecycle configuration. For more information, see Using Amazon S3 on Outposts in
* Amazon Simple Storage Service User Guide.
*
*
* To use this action, you must have permission to perform the s3-outposts:DeleteLifecycleConfiguration
* action. By default, the bucket owner has this permission and the Outposts bucket owner can grant this permission
* to others.
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request and an S3 on Outposts endpoint hostname prefix
* instead of s3-control
. For an example of the request syntax for Amazon S3 on Outposts that uses the
* S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived using the access point ARN,
* see the Examples section.
*
*
* For more information about object expiration, see Elements to Describe Lifecycle Actions.
*
*
* Related actions include:
*
*
* -
*
*
* -
*
*
*
*
* @param deleteBucketLifecycleConfigurationRequest
* @return Result of the DeleteBucketLifecycleConfiguration operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.DeleteBucketLifecycleConfiguration
* @see AWS API Documentation
*/
default DeleteBucketLifecycleConfigurationResponse deleteBucketLifecycleConfiguration(
DeleteBucketLifecycleConfigurationRequest deleteBucketLifecycleConfigurationRequest) throws AwsServiceException,
SdkClientException, S3ControlException {
throw new UnsupportedOperationException();
}
/**
*
*
* This action deletes an Amazon S3 on Outposts bucket's lifecycle configuration. To delete an S3 bucket's lifecycle
* configuration, see DeleteBucketLifecycle
* in the Amazon Simple Storage Service API.
*
*
*
* Deletes the lifecycle configuration from the specified Outposts bucket. Amazon S3 on Outposts removes all the
* lifecycle configuration rules in the lifecycle subresource associated with the bucket. Your objects never expire,
* and Amazon S3 on Outposts no longer automatically deletes any objects on the basis of rules contained in the
* deleted lifecycle configuration. For more information, see Using Amazon S3 on Outposts in
* Amazon Simple Storage Service User Guide.
*
*
* To use this action, you must have permission to perform the s3-outposts:DeleteLifecycleConfiguration
* action. By default, the bucket owner has this permission and the Outposts bucket owner can grant this permission
* to others.
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request and an S3 on Outposts endpoint hostname prefix
* instead of s3-control
. For an example of the request syntax for Amazon S3 on Outposts that uses the
* S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived using the access point ARN,
* see the Examples section.
*
*
* For more information about object expiration, see Elements to Describe Lifecycle Actions.
*
*
* Related actions include:
*
*
* -
*
*
* -
*
*
*
*
*
* This is a convenience which creates an instance of the {@link DeleteBucketLifecycleConfigurationRequest.Builder}
* avoiding the need to create one manually via {@link DeleteBucketLifecycleConfigurationRequest#builder()}
*
*
* @param deleteBucketLifecycleConfigurationRequest
* A {@link Consumer} that will call methods on {@link DeleteBucketLifecycleConfigurationRequest.Builder} to
* create a request.
* @return Result of the DeleteBucketLifecycleConfiguration operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.DeleteBucketLifecycleConfiguration
* @see AWS API Documentation
*/
default DeleteBucketLifecycleConfigurationResponse deleteBucketLifecycleConfiguration(
Consumer deleteBucketLifecycleConfigurationRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
return deleteBucketLifecycleConfiguration(DeleteBucketLifecycleConfigurationRequest.builder()
.applyMutation(deleteBucketLifecycleConfigurationRequest).build());
}
/**
*
*
* This action deletes an Amazon S3 on Outposts bucket policy. To delete an S3 bucket policy, see DeleteBucketPolicy in the
* Amazon Simple Storage Service API.
*
*
*
* This implementation of the DELETE action uses the policy subresource to delete the policy of a specified Amazon
* S3 on Outposts bucket. If you are using an identity other than the root user of the AWS account that owns the
* bucket, the calling identity must have the s3-outposts:DeleteBucketPolicy
permissions on the
* specified Outposts bucket and belong to the bucket owner's account to use this action. For more information, see
* Using Amazon S3 on Outposts
* in Amazon Simple Storage Service User Guide.
*
*
* If you don't have DeleteBucketPolicy
permissions, Amazon S3 returns a 403 Access Denied
* error. If you have the correct permissions, but you're not using an identity that belongs to the bucket owner's
* account, Amazon S3 returns a 405 Method Not Allowed
error.
*
*
*
* As a security precaution, the root user of the AWS account that owns a bucket can always use this action, even if
* the policy explicitly denies the root user the ability to perform this action.
*
*
*
* For more information about bucket policies, see Using Bucket Policies and User
* Policies.
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request and an S3 on Outposts endpoint hostname prefix
* instead of s3-control
. For an example of the request syntax for Amazon S3 on Outposts that uses the
* S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived using the access point ARN,
* see the Examples section.
*
*
* The following actions are related to DeleteBucketPolicy
:
*
*
* -
*
* GetBucketPolicy
*
*
* -
*
* PutBucketPolicy
*
*
*
*
* @param deleteBucketPolicyRequest
* @return Result of the DeleteBucketPolicy operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.DeleteBucketPolicy
* @see AWS
* API Documentation
*/
default DeleteBucketPolicyResponse deleteBucketPolicy(DeleteBucketPolicyRequest deleteBucketPolicyRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
throw new UnsupportedOperationException();
}
/**
*
*
* This action deletes an Amazon S3 on Outposts bucket policy. To delete an S3 bucket policy, see DeleteBucketPolicy in the
* Amazon Simple Storage Service API.
*
*
*
* This implementation of the DELETE action uses the policy subresource to delete the policy of a specified Amazon
* S3 on Outposts bucket. If you are using an identity other than the root user of the AWS account that owns the
* bucket, the calling identity must have the s3-outposts:DeleteBucketPolicy
permissions on the
* specified Outposts bucket and belong to the bucket owner's account to use this action. For more information, see
* Using Amazon S3 on Outposts
* in Amazon Simple Storage Service User Guide.
*
*
* If you don't have DeleteBucketPolicy
permissions, Amazon S3 returns a 403 Access Denied
* error. If you have the correct permissions, but you're not using an identity that belongs to the bucket owner's
* account, Amazon S3 returns a 405 Method Not Allowed
error.
*
*
*
* As a security precaution, the root user of the AWS account that owns a bucket can always use this action, even if
* the policy explicitly denies the root user the ability to perform this action.
*
*
*
* For more information about bucket policies, see Using Bucket Policies and User
* Policies.
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request and an S3 on Outposts endpoint hostname prefix
* instead of s3-control
. For an example of the request syntax for Amazon S3 on Outposts that uses the
* S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived using the access point ARN,
* see the Examples section.
*
*
* The following actions are related to DeleteBucketPolicy
:
*
*
* -
*
* GetBucketPolicy
*
*
* -
*
* PutBucketPolicy
*
*
*
*
*
* This is a convenience which creates an instance of the {@link DeleteBucketPolicyRequest.Builder} avoiding the
* need to create one manually via {@link DeleteBucketPolicyRequest#builder()}
*
*
* @param deleteBucketPolicyRequest
* A {@link Consumer} that will call methods on {@link DeleteBucketPolicyRequest.Builder} to create a
* request.
* @return Result of the DeleteBucketPolicy operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.DeleteBucketPolicy
* @see AWS
* API Documentation
*/
default DeleteBucketPolicyResponse deleteBucketPolicy(Consumer deleteBucketPolicyRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
return deleteBucketPolicy(DeleteBucketPolicyRequest.builder().applyMutation(deleteBucketPolicyRequest).build());
}
/**
*
*
* This action deletes an Amazon S3 on Outposts bucket's tags. To delete an S3 bucket tags, see DeleteBucketTagging in
* the Amazon Simple Storage Service API.
*
*
*
* Deletes the tags from the Outposts bucket. For more information, see Using Amazon S3 on Outposts in
* Amazon Simple Storage Service User Guide.
*
*
* To use this action, you must have permission to perform the PutBucketTagging
action. By default, the
* bucket owner has this permission and can grant this permission to others.
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request and an S3 on Outposts endpoint hostname prefix
* instead of s3-control
. For an example of the request syntax for Amazon S3 on Outposts that uses the
* S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived using the access point ARN,
* see the Examples section.
*
*
* The following actions are related to DeleteBucketTagging
:
*
*
* -
*
* GetBucketTagging
*
*
* -
*
* PutBucketTagging
*
*
*
*
* @param deleteBucketTaggingRequest
* @return Result of the DeleteBucketTagging operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.DeleteBucketTagging
* @see AWS
* API Documentation
*/
default DeleteBucketTaggingResponse deleteBucketTagging(DeleteBucketTaggingRequest deleteBucketTaggingRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
throw new UnsupportedOperationException();
}
/**
*
*
* This action deletes an Amazon S3 on Outposts bucket's tags. To delete an S3 bucket tags, see DeleteBucketTagging in
* the Amazon Simple Storage Service API.
*
*
*
* Deletes the tags from the Outposts bucket. For more information, see Using Amazon S3 on Outposts in
* Amazon Simple Storage Service User Guide.
*
*
* To use this action, you must have permission to perform the PutBucketTagging
action. By default, the
* bucket owner has this permission and can grant this permission to others.
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request and an S3 on Outposts endpoint hostname prefix
* instead of s3-control
. For an example of the request syntax for Amazon S3 on Outposts that uses the
* S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived using the access point ARN,
* see the Examples section.
*
*
* The following actions are related to DeleteBucketTagging
:
*
*
* -
*
* GetBucketTagging
*
*
* -
*
* PutBucketTagging
*
*
*
*
*
* This is a convenience which creates an instance of the {@link DeleteBucketTaggingRequest.Builder} avoiding the
* need to create one manually via {@link DeleteBucketTaggingRequest#builder()}
*
*
* @param deleteBucketTaggingRequest
* A {@link Consumer} that will call methods on {@link DeleteBucketTaggingRequest.Builder} to create a
* request.
* @return Result of the DeleteBucketTagging operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.DeleteBucketTagging
* @see AWS
* API Documentation
*/
default DeleteBucketTaggingResponse deleteBucketTagging(
Consumer deleteBucketTaggingRequest) throws AwsServiceException,
SdkClientException, S3ControlException {
return deleteBucketTagging(DeleteBucketTaggingRequest.builder().applyMutation(deleteBucketTaggingRequest).build());
}
/**
*
* Removes the entire tag set from the specified S3 Batch Operations job. To use this operation, you must have
* permission to perform the s3:DeleteJobTagging
action. For more information, see Controlling access and labeling jobs using tags in the Amazon Simple Storage Service User Guide.
*
*
*
* Related actions include:
*
*
* -
*
* CreateJob
*
*
* -
*
* GetJobTagging
*
*
* -
*
* PutJobTagging
*
*
*
*
* @param deleteJobTaggingRequest
* @return Result of the DeleteJobTagging operation returned by the service.
* @throws InternalServiceException
* @throws TooManyRequestsException
* @throws NotFoundException
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.DeleteJobTagging
* @see AWS
* API Documentation
*/
default DeleteJobTaggingResponse deleteJobTagging(DeleteJobTaggingRequest deleteJobTaggingRequest)
throws InternalServiceException, TooManyRequestsException, NotFoundException, AwsServiceException,
SdkClientException, S3ControlException {
throw new UnsupportedOperationException();
}
/**
*
* Removes the entire tag set from the specified S3 Batch Operations job. To use this operation, you must have
* permission to perform the s3:DeleteJobTagging
action. For more information, see Controlling access and labeling jobs using tags in the Amazon Simple Storage Service User Guide.
*
*
*
* Related actions include:
*
*
* -
*
* CreateJob
*
*
* -
*
* GetJobTagging
*
*
* -
*
* PutJobTagging
*
*
*
*
*
* This is a convenience which creates an instance of the {@link DeleteJobTaggingRequest.Builder} avoiding the need
* to create one manually via {@link DeleteJobTaggingRequest#builder()}
*
*
* @param deleteJobTaggingRequest
* A {@link Consumer} that will call methods on {@link DeleteJobTaggingRequest.Builder} to create a request.
* @return Result of the DeleteJobTagging operation returned by the service.
* @throws InternalServiceException
* @throws TooManyRequestsException
* @throws NotFoundException
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.DeleteJobTagging
* @see AWS
* API Documentation
*/
default DeleteJobTaggingResponse deleteJobTagging(Consumer deleteJobTaggingRequest)
throws InternalServiceException, TooManyRequestsException, NotFoundException, AwsServiceException,
SdkClientException, S3ControlException {
return deleteJobTagging(DeleteJobTaggingRequest.builder().applyMutation(deleteJobTaggingRequest).build());
}
/**
*
* Removes the PublicAccessBlock
configuration for an AWS account. For more information, see Using Amazon S3
* block public access.
*
*
* Related actions include:
*
*
* -
*
*
* -
*
*
*
*
* @param deletePublicAccessBlockRequest
* @return Result of the DeletePublicAccessBlock operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.DeletePublicAccessBlock
* @see AWS API Documentation
*/
default DeletePublicAccessBlockResponse deletePublicAccessBlock(DeletePublicAccessBlockRequest deletePublicAccessBlockRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
throw new UnsupportedOperationException();
}
/**
*
* Removes the PublicAccessBlock
configuration for an AWS account. For more information, see Using Amazon S3
* block public access.
*
*
* Related actions include:
*
*
* -
*
*
* -
*
*
*
*
*
* This is a convenience which creates an instance of the {@link DeletePublicAccessBlockRequest.Builder} avoiding
* the need to create one manually via {@link DeletePublicAccessBlockRequest#builder()}
*
*
* @param deletePublicAccessBlockRequest
* A {@link Consumer} that will call methods on {@link DeletePublicAccessBlockRequest.Builder} to create a
* request.
* @return Result of the DeletePublicAccessBlock operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.DeletePublicAccessBlock
* @see AWS API Documentation
*/
default DeletePublicAccessBlockResponse deletePublicAccessBlock(
Consumer deletePublicAccessBlockRequest) throws AwsServiceException,
SdkClientException, S3ControlException {
return deletePublicAccessBlock(DeletePublicAccessBlockRequest.builder().applyMutation(deletePublicAccessBlockRequest)
.build());
}
/**
*
* Deletes the Amazon S3 Storage Lens configuration. For more information about S3 Storage Lens, see Assessing your storage activity and
* usage with Amazon S3 Storage Lens in the Amazon Simple Storage Service User Guide.
*
*
*
* To use this action, you must have permission to perform the s3:DeleteStorageLensConfiguration
* action. For more information, see Setting permissions to
* use Amazon S3 Storage Lens in the Amazon Simple Storage Service User Guide.
*
*
*
* @param deleteStorageLensConfigurationRequest
* @return Result of the DeleteStorageLensConfiguration operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.DeleteStorageLensConfiguration
* @see AWS API Documentation
*/
default DeleteStorageLensConfigurationResponse deleteStorageLensConfiguration(
DeleteStorageLensConfigurationRequest deleteStorageLensConfigurationRequest) throws AwsServiceException,
SdkClientException, S3ControlException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the Amazon S3 Storage Lens configuration. For more information about S3 Storage Lens, see Assessing your storage activity and
* usage with Amazon S3 Storage Lens in the Amazon Simple Storage Service User Guide.
*
*
*
* To use this action, you must have permission to perform the s3:DeleteStorageLensConfiguration
* action. For more information, see Setting permissions to
* use Amazon S3 Storage Lens in the Amazon Simple Storage Service User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteStorageLensConfigurationRequest.Builder}
* avoiding the need to create one manually via {@link DeleteStorageLensConfigurationRequest#builder()}
*
*
* @param deleteStorageLensConfigurationRequest
* A {@link Consumer} that will call methods on {@link DeleteStorageLensConfigurationRequest.Builder} to
* create a request.
* @return Result of the DeleteStorageLensConfiguration operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.DeleteStorageLensConfiguration
* @see AWS API Documentation
*/
default DeleteStorageLensConfigurationResponse deleteStorageLensConfiguration(
Consumer deleteStorageLensConfigurationRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
return deleteStorageLensConfiguration(DeleteStorageLensConfigurationRequest.builder()
.applyMutation(deleteStorageLensConfigurationRequest).build());
}
/**
*
* Deletes the Amazon S3 Storage Lens configuration tags. For more information about S3 Storage Lens, see Assessing your storage activity and
* usage with Amazon S3 Storage Lens in the Amazon Simple Storage Service User Guide.
*
*
*
* To use this action, you must have permission to perform the s3:DeleteStorageLensConfigurationTagging
* action. For more information, see Setting permissions to
* use Amazon S3 Storage Lens in the Amazon Simple Storage Service User Guide.
*
*
*
* @param deleteStorageLensConfigurationTaggingRequest
* @return Result of the DeleteStorageLensConfigurationTagging operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.DeleteStorageLensConfigurationTagging
* @see AWS API Documentation
*/
default DeleteStorageLensConfigurationTaggingResponse deleteStorageLensConfigurationTagging(
DeleteStorageLensConfigurationTaggingRequest deleteStorageLensConfigurationTaggingRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the Amazon S3 Storage Lens configuration tags. For more information about S3 Storage Lens, see Assessing your storage activity and
* usage with Amazon S3 Storage Lens in the Amazon Simple Storage Service User Guide.
*
*
*
* To use this action, you must have permission to perform the s3:DeleteStorageLensConfigurationTagging
* action. For more information, see Setting permissions to
* use Amazon S3 Storage Lens in the Amazon Simple Storage Service User Guide.
*
*
*
* This is a convenience which creates an instance of the
* {@link DeleteStorageLensConfigurationTaggingRequest.Builder} avoiding the need to create one manually via
* {@link DeleteStorageLensConfigurationTaggingRequest#builder()}
*
*
* @param deleteStorageLensConfigurationTaggingRequest
* A {@link Consumer} that will call methods on {@link DeleteStorageLensConfigurationTaggingRequest.Builder}
* to create a request.
* @return Result of the DeleteStorageLensConfigurationTagging operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.DeleteStorageLensConfigurationTagging
* @see AWS API Documentation
*/
default DeleteStorageLensConfigurationTaggingResponse deleteStorageLensConfigurationTagging(
Consumer deleteStorageLensConfigurationTaggingRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
return deleteStorageLensConfigurationTagging(DeleteStorageLensConfigurationTaggingRequest.builder()
.applyMutation(deleteStorageLensConfigurationTaggingRequest).build());
}
/**
*
* Retrieves the configuration parameters and status for a Batch Operations job. For more information, see S3 Batch Operations in the
* Amazon Simple Storage Service User Guide.
*
*
*
* Related actions include:
*
*
* -
*
* CreateJob
*
*
* -
*
* ListJobs
*
*
* -
*
*
* -
*
* UpdateJobStatus
*
*
*
*
* @param describeJobRequest
* @return Result of the DescribeJob operation returned by the service.
* @throws BadRequestException
* @throws TooManyRequestsException
* @throws NotFoundException
* @throws InternalServiceException
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.DescribeJob
* @see AWS API
* Documentation
*/
default DescribeJobResponse describeJob(DescribeJobRequest describeJobRequest) throws BadRequestException,
TooManyRequestsException, NotFoundException, InternalServiceException, AwsServiceException, SdkClientException,
S3ControlException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the configuration parameters and status for a Batch Operations job. For more information, see S3 Batch Operations in the
* Amazon Simple Storage Service User Guide.
*
*
*
* Related actions include:
*
*
* -
*
* CreateJob
*
*
* -
*
* ListJobs
*
*
* -
*
*
* -
*
* UpdateJobStatus
*
*
*
*
*
* This is a convenience which creates an instance of the {@link DescribeJobRequest.Builder} avoiding the need to
* create one manually via {@link DescribeJobRequest#builder()}
*
*
* @param describeJobRequest
* A {@link Consumer} that will call methods on {@link DescribeJobRequest.Builder} to create a request.
* @return Result of the DescribeJob operation returned by the service.
* @throws BadRequestException
* @throws TooManyRequestsException
* @throws NotFoundException
* @throws InternalServiceException
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.DescribeJob
* @see AWS API
* Documentation
*/
default DescribeJobResponse describeJob(Consumer describeJobRequest) throws BadRequestException,
TooManyRequestsException, NotFoundException, InternalServiceException, AwsServiceException, SdkClientException,
S3ControlException {
return describeJob(DescribeJobRequest.builder().applyMutation(describeJobRequest).build());
}
/**
*
* Returns configuration information about the specified access point.
*
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request and an S3 on Outposts endpoint hostname prefix
* instead of s3-control
. For an example of the request syntax for Amazon S3 on Outposts that uses the
* S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived using the access point ARN,
* see the Examples section.
*
*
* The following actions are related to GetAccessPoint
:
*
*
* -
*
*
* -
*
*
* -
*
* ListAccessPoints
*
*
*
*
* @param getAccessPointRequest
* @return Result of the GetAccessPoint operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.GetAccessPoint
* @see AWS API
* Documentation
*/
default GetAccessPointResponse getAccessPoint(GetAccessPointRequest getAccessPointRequest) throws AwsServiceException,
SdkClientException, S3ControlException {
throw new UnsupportedOperationException();
}
/**
*
* Returns configuration information about the specified access point.
*
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request and an S3 on Outposts endpoint hostname prefix
* instead of s3-control
. For an example of the request syntax for Amazon S3 on Outposts that uses the
* S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived using the access point ARN,
* see the Examples section.
*
*
* The following actions are related to GetAccessPoint
:
*
*
* -
*
*
* -
*
*
* -
*
* ListAccessPoints
*
*
*
*
*
* This is a convenience which creates an instance of the {@link GetAccessPointRequest.Builder} avoiding the need to
* create one manually via {@link GetAccessPointRequest#builder()}
*
*
* @param getAccessPointRequest
* A {@link Consumer} that will call methods on {@link GetAccessPointRequest.Builder} to create a request.
* @return Result of the GetAccessPoint operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.GetAccessPoint
* @see AWS API
* Documentation
*/
default GetAccessPointResponse getAccessPoint(Consumer getAccessPointRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
return getAccessPoint(GetAccessPointRequest.builder().applyMutation(getAccessPointRequest).build());
}
/**
*
* Returns configuration for an Object Lambda Access Point.
*
*
* The following actions are related to GetAccessPointConfigurationForObjectLambda
:
*
*
* -
*
*
*
*
* @param getAccessPointConfigurationForObjectLambdaRequest
* @return Result of the GetAccessPointConfigurationForObjectLambda operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.GetAccessPointConfigurationForObjectLambda
* @see AWS API Documentation
*/
default GetAccessPointConfigurationForObjectLambdaResponse getAccessPointConfigurationForObjectLambda(
GetAccessPointConfigurationForObjectLambdaRequest getAccessPointConfigurationForObjectLambdaRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
throw new UnsupportedOperationException();
}
/**
*
* Returns configuration for an Object Lambda Access Point.
*
*
* The following actions are related to GetAccessPointConfigurationForObjectLambda
:
*
*
* -
*
*
*
*
*
* This is a convenience which creates an instance of the
* {@link GetAccessPointConfigurationForObjectLambdaRequest.Builder} avoiding the need to create one manually via
* {@link GetAccessPointConfigurationForObjectLambdaRequest#builder()}
*
*
* @param getAccessPointConfigurationForObjectLambdaRequest
* A {@link Consumer} that will call methods on
* {@link GetAccessPointConfigurationForObjectLambdaRequest.Builder} to create a request.
* @return Result of the GetAccessPointConfigurationForObjectLambda operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.GetAccessPointConfigurationForObjectLambda
* @see AWS API Documentation
*/
default GetAccessPointConfigurationForObjectLambdaResponse getAccessPointConfigurationForObjectLambda(
Consumer getAccessPointConfigurationForObjectLambdaRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
return getAccessPointConfigurationForObjectLambda(GetAccessPointConfigurationForObjectLambdaRequest.builder()
.applyMutation(getAccessPointConfigurationForObjectLambdaRequest).build());
}
/**
*
* Returns configuration information about the specified Object Lambda Access Point
*
*
* The following actions are related to GetAccessPointForObjectLambda
:
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* @param getAccessPointForObjectLambdaRequest
* @return Result of the GetAccessPointForObjectLambda operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.GetAccessPointForObjectLambda
* @see AWS API Documentation
*/
default GetAccessPointForObjectLambdaResponse getAccessPointForObjectLambda(
GetAccessPointForObjectLambdaRequest getAccessPointForObjectLambdaRequest) throws AwsServiceException,
SdkClientException, S3ControlException {
throw new UnsupportedOperationException();
}
/**
*
* Returns configuration information about the specified Object Lambda Access Point
*
*
* The following actions are related to GetAccessPointForObjectLambda
:
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
*
* This is a convenience which creates an instance of the {@link GetAccessPointForObjectLambdaRequest.Builder}
* avoiding the need to create one manually via {@link GetAccessPointForObjectLambdaRequest#builder()}
*
*
* @param getAccessPointForObjectLambdaRequest
* A {@link Consumer} that will call methods on {@link GetAccessPointForObjectLambdaRequest.Builder} to
* create a request.
* @return Result of the GetAccessPointForObjectLambda operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.GetAccessPointForObjectLambda
* @see AWS API Documentation
*/
default GetAccessPointForObjectLambdaResponse getAccessPointForObjectLambda(
Consumer getAccessPointForObjectLambdaRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
return getAccessPointForObjectLambda(GetAccessPointForObjectLambdaRequest.builder()
.applyMutation(getAccessPointForObjectLambdaRequest).build());
}
/**
*
* Returns the access point policy associated with the specified access point.
*
*
* The following actions are related to GetAccessPointPolicy
:
*
*
* -
*
*
* -
*
*
*
*
* @param getAccessPointPolicyRequest
* @return Result of the GetAccessPointPolicy operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.GetAccessPointPolicy
* @see AWS API Documentation
*/
default GetAccessPointPolicyResponse getAccessPointPolicy(GetAccessPointPolicyRequest getAccessPointPolicyRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
throw new UnsupportedOperationException();
}
/**
*
* Returns the access point policy associated with the specified access point.
*
*
* The following actions are related to GetAccessPointPolicy
:
*
*
* -
*
*
* -
*
*
*
*
*
* This is a convenience which creates an instance of the {@link GetAccessPointPolicyRequest.Builder} avoiding the
* need to create one manually via {@link GetAccessPointPolicyRequest#builder()}
*
*
* @param getAccessPointPolicyRequest
* A {@link Consumer} that will call methods on {@link GetAccessPointPolicyRequest.Builder} to create a
* request.
* @return Result of the GetAccessPointPolicy operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.GetAccessPointPolicy
* @see AWS API Documentation
*/
default GetAccessPointPolicyResponse getAccessPointPolicy(
Consumer getAccessPointPolicyRequest) throws AwsServiceException,
SdkClientException, S3ControlException {
return getAccessPointPolicy(GetAccessPointPolicyRequest.builder().applyMutation(getAccessPointPolicyRequest).build());
}
/**
*
* Returns the resource policy for an Object Lambda Access Point.
*
*
* The following actions are related to GetAccessPointPolicyForObjectLambda
:
*
*
* -
*
*
* -
*
*
*
*
* @param getAccessPointPolicyForObjectLambdaRequest
* @return Result of the GetAccessPointPolicyForObjectLambda operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.GetAccessPointPolicyForObjectLambda
* @see AWS API Documentation
*/
default GetAccessPointPolicyForObjectLambdaResponse getAccessPointPolicyForObjectLambda(
GetAccessPointPolicyForObjectLambdaRequest getAccessPointPolicyForObjectLambdaRequest) throws AwsServiceException,
SdkClientException, S3ControlException {
throw new UnsupportedOperationException();
}
/**
*
* Returns the resource policy for an Object Lambda Access Point.
*
*
* The following actions are related to GetAccessPointPolicyForObjectLambda
:
*
*
* -
*
*
* -
*
*
*
*
*
* This is a convenience which creates an instance of the {@link GetAccessPointPolicyForObjectLambdaRequest.Builder}
* avoiding the need to create one manually via {@link GetAccessPointPolicyForObjectLambdaRequest#builder()}
*
*
* @param getAccessPointPolicyForObjectLambdaRequest
* A {@link Consumer} that will call methods on {@link GetAccessPointPolicyForObjectLambdaRequest.Builder} to
* create a request.
* @return Result of the GetAccessPointPolicyForObjectLambda operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.GetAccessPointPolicyForObjectLambda
* @see AWS API Documentation
*/
default GetAccessPointPolicyForObjectLambdaResponse getAccessPointPolicyForObjectLambda(
Consumer getAccessPointPolicyForObjectLambdaRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
return getAccessPointPolicyForObjectLambda(GetAccessPointPolicyForObjectLambdaRequest.builder()
.applyMutation(getAccessPointPolicyForObjectLambdaRequest).build());
}
/**
*
* Indicates whether the specified access point currently has a policy that allows public access. For more
* information about public access through access points, see Managing Data Access with Amazon
* S3 Access Points in the Amazon Simple Storage Service Developer Guide.
*
*
* @param getAccessPointPolicyStatusRequest
* @return Result of the GetAccessPointPolicyStatus operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.GetAccessPointPolicyStatus
* @see AWS API Documentation
*/
default GetAccessPointPolicyStatusResponse getAccessPointPolicyStatus(
GetAccessPointPolicyStatusRequest getAccessPointPolicyStatusRequest) throws AwsServiceException, SdkClientException,
S3ControlException {
throw new UnsupportedOperationException();
}
/**
*
* Indicates whether the specified access point currently has a policy that allows public access. For more
* information about public access through access points, see Managing Data Access with Amazon
* S3 Access Points in the Amazon Simple Storage Service Developer Guide.
*
*
*
* This is a convenience which creates an instance of the {@link GetAccessPointPolicyStatusRequest.Builder} avoiding
* the need to create one manually via {@link GetAccessPointPolicyStatusRequest#builder()}
*
*
* @param getAccessPointPolicyStatusRequest
* A {@link Consumer} that will call methods on {@link GetAccessPointPolicyStatusRequest.Builder} to create a
* request.
* @return Result of the GetAccessPointPolicyStatus operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.GetAccessPointPolicyStatus
* @see AWS API Documentation
*/
default GetAccessPointPolicyStatusResponse getAccessPointPolicyStatus(
Consumer getAccessPointPolicyStatusRequest) throws AwsServiceException,
SdkClientException, S3ControlException {
return getAccessPointPolicyStatus(GetAccessPointPolicyStatusRequest.builder()
.applyMutation(getAccessPointPolicyStatusRequest).build());
}
/**
*
* Returns the status of the resource policy associated with an Object Lambda Access Point.
*
*
* @param getAccessPointPolicyStatusForObjectLambdaRequest
* @return Result of the GetAccessPointPolicyStatusForObjectLambda operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.GetAccessPointPolicyStatusForObjectLambda
* @see AWS API Documentation
*/
default GetAccessPointPolicyStatusForObjectLambdaResponse getAccessPointPolicyStatusForObjectLambda(
GetAccessPointPolicyStatusForObjectLambdaRequest getAccessPointPolicyStatusForObjectLambdaRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
throw new UnsupportedOperationException();
}
/**
*
* Returns the status of the resource policy associated with an Object Lambda Access Point.
*
*
*
* This is a convenience which creates an instance of the
* {@link GetAccessPointPolicyStatusForObjectLambdaRequest.Builder} avoiding the need to create one manually via
* {@link GetAccessPointPolicyStatusForObjectLambdaRequest#builder()}
*
*
* @param getAccessPointPolicyStatusForObjectLambdaRequest
* A {@link Consumer} that will call methods on
* {@link GetAccessPointPolicyStatusForObjectLambdaRequest.Builder} to create a request.
* @return Result of the GetAccessPointPolicyStatusForObjectLambda operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.GetAccessPointPolicyStatusForObjectLambda
* @see AWS API Documentation
*/
default GetAccessPointPolicyStatusForObjectLambdaResponse getAccessPointPolicyStatusForObjectLambda(
Consumer getAccessPointPolicyStatusForObjectLambdaRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
return getAccessPointPolicyStatusForObjectLambda(GetAccessPointPolicyStatusForObjectLambdaRequest.builder()
.applyMutation(getAccessPointPolicyStatusForObjectLambdaRequest).build());
}
/**
*
* Gets an Amazon S3 on Outposts bucket. For more information, see Using Amazon S3 on Outposts
* in the Amazon Simple Storage Service User Guide.
*
*
* If you are using an identity other than the root user of the AWS account that owns the Outposts bucket, the
* calling identity must have the s3-outposts:GetBucket
permissions on the specified Outposts bucket
* and belong to the Outposts bucket owner's account in order to use this action. Only users from Outposts bucket
* owner account with the right permissions can perform actions on an Outposts bucket.
*
*
* If you don't have s3-outposts:GetBucket
permissions or you're not using an identity that belongs to
* the bucket owner's account, Amazon S3 returns a 403 Access Denied
error.
*
*
* The following actions are related to GetBucket
for Amazon S3 on Outposts:
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request and an S3 on Outposts endpoint hostname prefix
* instead of s3-control
. For an example of the request syntax for Amazon S3 on Outposts that uses the
* S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived using the access point ARN,
* see the Examples section.
*
*
* -
*
* PutObject
*
*
* -
*
* CreateBucket
*
*
* -
*
* DeleteBucket
*
*
*
*
* @param getBucketRequest
* @return Result of the GetBucket operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.GetBucket
* @see AWS API
* Documentation
*/
default GetBucketResponse getBucket(GetBucketRequest getBucketRequest) throws AwsServiceException, SdkClientException,
S3ControlException {
throw new UnsupportedOperationException();
}
/**
*
* Gets an Amazon S3 on Outposts bucket. For more information, see Using Amazon S3 on Outposts
* in the Amazon Simple Storage Service User Guide.
*
*
* If you are using an identity other than the root user of the AWS account that owns the Outposts bucket, the
* calling identity must have the s3-outposts:GetBucket
permissions on the specified Outposts bucket
* and belong to the Outposts bucket owner's account in order to use this action. Only users from Outposts bucket
* owner account with the right permissions can perform actions on an Outposts bucket.
*
*
* If you don't have s3-outposts:GetBucket
permissions or you're not using an identity that belongs to
* the bucket owner's account, Amazon S3 returns a 403 Access Denied
error.
*
*
* The following actions are related to GetBucket
for Amazon S3 on Outposts:
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request and an S3 on Outposts endpoint hostname prefix
* instead of s3-control
. For an example of the request syntax for Amazon S3 on Outposts that uses the
* S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived using the access point ARN,
* see the Examples section.
*
*
* -
*
* PutObject
*
*
* -
*
* CreateBucket
*
*
* -
*
* DeleteBucket
*
*
*
*
*
* This is a convenience which creates an instance of the {@link GetBucketRequest.Builder} avoiding the need to
* create one manually via {@link GetBucketRequest#builder()}
*
*
* @param getBucketRequest
* A {@link Consumer} that will call methods on {@link GetBucketRequest.Builder} to create a request.
* @return Result of the GetBucket operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.GetBucket
* @see AWS API
* Documentation
*/
default GetBucketResponse getBucket(Consumer getBucketRequest) throws AwsServiceException,
SdkClientException, S3ControlException {
return getBucket(GetBucketRequest.builder().applyMutation(getBucketRequest).build());
}
/**
*
*
* This action gets an Amazon S3 on Outposts bucket's lifecycle configuration. To get an S3 bucket's lifecycle
* configuration, see GetBucketLifecycleConfiguration in the Amazon Simple Storage Service API.
*
*
*
* Returns the lifecycle configuration information set on the Outposts bucket. For more information, see Using Amazon S3 on Outposts
* and for information about lifecycle configuration, see Object Lifecycle
* Management in Amazon Simple Storage Service User Guide.
*
*
* To use this action, you must have permission to perform the s3-outposts:GetLifecycleConfiguration
* action. The Outposts bucket owner has this permission, by default. The bucket owner can grant this permission to
* others. For more information about permissions, see Permissions Related to Bucket Subresource Operations and Managing Access Permissions
* to Your Amazon S3 Resources.
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request and an S3 on Outposts endpoint hostname prefix
* instead of s3-control
. For an example of the request syntax for Amazon S3 on Outposts that uses the
* S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived using the access point ARN,
* see the Examples section.
*
*
* GetBucketLifecycleConfiguration
has the following special error:
*
*
* -
*
* Error code: NoSuchLifecycleConfiguration
*
*
* -
*
* Description: The lifecycle configuration does not exist.
*
*
* -
*
* HTTP Status Code: 404 Not Found
*
*
* -
*
* SOAP Fault Code Prefix: Client
*
*
*
*
*
*
* The following actions are related to GetBucketLifecycleConfiguration
:
*
*
* -
*
*
* -
*
*
*
*
* @param getBucketLifecycleConfigurationRequest
* @return Result of the GetBucketLifecycleConfiguration operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.GetBucketLifecycleConfiguration
* @see AWS API Documentation
*/
default GetBucketLifecycleConfigurationResponse getBucketLifecycleConfiguration(
GetBucketLifecycleConfigurationRequest getBucketLifecycleConfigurationRequest) throws AwsServiceException,
SdkClientException, S3ControlException {
throw new UnsupportedOperationException();
}
/**
*
*
* This action gets an Amazon S3 on Outposts bucket's lifecycle configuration. To get an S3 bucket's lifecycle
* configuration, see GetBucketLifecycleConfiguration in the Amazon Simple Storage Service API.
*
*
*
* Returns the lifecycle configuration information set on the Outposts bucket. For more information, see Using Amazon S3 on Outposts
* and for information about lifecycle configuration, see Object Lifecycle
* Management in Amazon Simple Storage Service User Guide.
*
*
* To use this action, you must have permission to perform the s3-outposts:GetLifecycleConfiguration
* action. The Outposts bucket owner has this permission, by default. The bucket owner can grant this permission to
* others. For more information about permissions, see Permissions Related to Bucket Subresource Operations and Managing Access Permissions
* to Your Amazon S3 Resources.
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request and an S3 on Outposts endpoint hostname prefix
* instead of s3-control
. For an example of the request syntax for Amazon S3 on Outposts that uses the
* S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived using the access point ARN,
* see the Examples section.
*
*
* GetBucketLifecycleConfiguration
has the following special error:
*
*
* -
*
* Error code: NoSuchLifecycleConfiguration
*
*
* -
*
* Description: The lifecycle configuration does not exist.
*
*
* -
*
* HTTP Status Code: 404 Not Found
*
*
* -
*
* SOAP Fault Code Prefix: Client
*
*
*
*
*
*
* The following actions are related to GetBucketLifecycleConfiguration
:
*
*
* -
*
*
* -
*
*
*
*
*
* This is a convenience which creates an instance of the {@link GetBucketLifecycleConfigurationRequest.Builder}
* avoiding the need to create one manually via {@link GetBucketLifecycleConfigurationRequest#builder()}
*
*
* @param getBucketLifecycleConfigurationRequest
* A {@link Consumer} that will call methods on {@link GetBucketLifecycleConfigurationRequest.Builder} to
* create a request.
* @return Result of the GetBucketLifecycleConfiguration operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.GetBucketLifecycleConfiguration
* @see AWS API Documentation
*/
default GetBucketLifecycleConfigurationResponse getBucketLifecycleConfiguration(
Consumer getBucketLifecycleConfigurationRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
return getBucketLifecycleConfiguration(GetBucketLifecycleConfigurationRequest.builder()
.applyMutation(getBucketLifecycleConfigurationRequest).build());
}
/**
*
*
* This action gets a bucket policy for an Amazon S3 on Outposts bucket. To get a policy for an S3 bucket, see GetBucketPolicy in the
* Amazon Simple Storage Service API.
*
*
*
* Returns the policy of a specified Outposts bucket. For more information, see Using Amazon S3 on Outposts in
* the Amazon Simple Storage Service User Guide.
*
*
* If you are using an identity other than the root user of the AWS account that owns the bucket, the calling
* identity must have the GetBucketPolicy
permissions on the specified bucket and belong to the bucket
* owner's account in order to use this action.
*
*
* Only users from Outposts bucket owner account with the right permissions can perform actions on an Outposts
* bucket. If you don't have s3-outposts:GetBucketPolicy
permissions or you're not using an identity
* that belongs to the bucket owner's account, Amazon S3 returns a 403 Access Denied
error.
*
*
*
* As a security precaution, the root user of the AWS account that owns a bucket can always use this action, even if
* the policy explicitly denies the root user the ability to perform this action.
*
*
*
* For more information about bucket policies, see Using Bucket Policies and User
* Policies.
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request and an S3 on Outposts endpoint hostname prefix
* instead of s3-control
. For an example of the request syntax for Amazon S3 on Outposts that uses the
* S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived using the access point ARN,
* see the Examples section.
*
*
* The following actions are related to GetBucketPolicy
:
*
*
* -
*
* GetObject
*
*
* -
*
* PutBucketPolicy
*
*
* -
*
*
*
*
* @param getBucketPolicyRequest
* @return Result of the GetBucketPolicy operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.GetBucketPolicy
* @see AWS API
* Documentation
*/
default GetBucketPolicyResponse getBucketPolicy(GetBucketPolicyRequest getBucketPolicyRequest) throws AwsServiceException,
SdkClientException, S3ControlException {
throw new UnsupportedOperationException();
}
/**
*
*
* This action gets a bucket policy for an Amazon S3 on Outposts bucket. To get a policy for an S3 bucket, see GetBucketPolicy in the
* Amazon Simple Storage Service API.
*
*
*
* Returns the policy of a specified Outposts bucket. For more information, see Using Amazon S3 on Outposts in
* the Amazon Simple Storage Service User Guide.
*
*
* If you are using an identity other than the root user of the AWS account that owns the bucket, the calling
* identity must have the GetBucketPolicy
permissions on the specified bucket and belong to the bucket
* owner's account in order to use this action.
*
*
* Only users from Outposts bucket owner account with the right permissions can perform actions on an Outposts
* bucket. If you don't have s3-outposts:GetBucketPolicy
permissions or you're not using an identity
* that belongs to the bucket owner's account, Amazon S3 returns a 403 Access Denied
error.
*
*
*
* As a security precaution, the root user of the AWS account that owns a bucket can always use this action, even if
* the policy explicitly denies the root user the ability to perform this action.
*
*
*
* For more information about bucket policies, see Using Bucket Policies and User
* Policies.
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request and an S3 on Outposts endpoint hostname prefix
* instead of s3-control
. For an example of the request syntax for Amazon S3 on Outposts that uses the
* S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived using the access point ARN,
* see the Examples section.
*
*
* The following actions are related to GetBucketPolicy
:
*
*
* -
*
* GetObject
*
*
* -
*
* PutBucketPolicy
*
*
* -
*
*
*
*
*
* This is a convenience which creates an instance of the {@link GetBucketPolicyRequest.Builder} avoiding the need
* to create one manually via {@link GetBucketPolicyRequest#builder()}
*
*
* @param getBucketPolicyRequest
* A {@link Consumer} that will call methods on {@link GetBucketPolicyRequest.Builder} to create a request.
* @return Result of the GetBucketPolicy operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.GetBucketPolicy
* @see AWS API
* Documentation
*/
default GetBucketPolicyResponse getBucketPolicy(Consumer getBucketPolicyRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
return getBucketPolicy(GetBucketPolicyRequest.builder().applyMutation(getBucketPolicyRequest).build());
}
/**
*
*
* This action gets an Amazon S3 on Outposts bucket's tags. To get an S3 bucket tags, see GetBucketTagging in the
* Amazon Simple Storage Service API.
*
*
*
* Returns the tag set associated with the Outposts bucket. For more information, see Using Amazon S3 on Outposts in
* the Amazon Simple Storage Service User Guide.
*
*
* To use this action, you must have permission to perform the GetBucketTagging
action. By default, the
* bucket owner has this permission and can grant this permission to others.
*
*
* GetBucketTagging
has the following special error:
*
*
* -
*
* Error code: NoSuchTagSetError
*
*
* -
*
* Description: There is no tag set associated with the bucket.
*
*
*
*
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request and an S3 on Outposts endpoint hostname prefix
* instead of s3-control
. For an example of the request syntax for Amazon S3 on Outposts that uses the
* S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived using the access point ARN,
* see the Examples section.
*
*
* The following actions are related to GetBucketTagging
:
*
*
* -
*
* PutBucketTagging
*
*
* -
*
*
*
*
* @param getBucketTaggingRequest
* @return Result of the GetBucketTagging operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.GetBucketTagging
* @see AWS
* API Documentation
*/
default GetBucketTaggingResponse getBucketTagging(GetBucketTaggingRequest getBucketTaggingRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
throw new UnsupportedOperationException();
}
/**
*
*
* This action gets an Amazon S3 on Outposts bucket's tags. To get an S3 bucket tags, see GetBucketTagging in the
* Amazon Simple Storage Service API.
*
*
*
* Returns the tag set associated with the Outposts bucket. For more information, see Using Amazon S3 on Outposts in
* the Amazon Simple Storage Service User Guide.
*
*
* To use this action, you must have permission to perform the GetBucketTagging
action. By default, the
* bucket owner has this permission and can grant this permission to others.
*
*
* GetBucketTagging
has the following special error:
*
*
* -
*
* Error code: NoSuchTagSetError
*
*
* -
*
* Description: There is no tag set associated with the bucket.
*
*
*
*
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request and an S3 on Outposts endpoint hostname prefix
* instead of s3-control
. For an example of the request syntax for Amazon S3 on Outposts that uses the
* S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived using the access point ARN,
* see the Examples section.
*
*
* The following actions are related to GetBucketTagging
:
*
*
* -
*
* PutBucketTagging
*
*
* -
*
*
*
*
*
* This is a convenience which creates an instance of the {@link GetBucketTaggingRequest.Builder} avoiding the need
* to create one manually via {@link GetBucketTaggingRequest#builder()}
*
*
* @param getBucketTaggingRequest
* A {@link Consumer} that will call methods on {@link GetBucketTaggingRequest.Builder} to create a request.
* @return Result of the GetBucketTagging operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.GetBucketTagging
* @see AWS
* API Documentation
*/
default GetBucketTaggingResponse getBucketTagging(Consumer getBucketTaggingRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
return getBucketTagging(GetBucketTaggingRequest.builder().applyMutation(getBucketTaggingRequest).build());
}
/**
*
* Returns the tags on an S3 Batch Operations job. To use this operation, you must have permission to perform the
* s3:GetJobTagging
action. For more information, see Controlling access and labeling jobs using tags in the Amazon Simple Storage Service User Guide.
*
*
*
* Related actions include:
*
*
* -
*
* CreateJob
*
*
* -
*
* PutJobTagging
*
*
* -
*
* DeleteJobTagging
*
*
*
*
* @param getJobTaggingRequest
* @return Result of the GetJobTagging operation returned by the service.
* @throws InternalServiceException
* @throws TooManyRequestsException
* @throws NotFoundException
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.GetJobTagging
* @see AWS API
* Documentation
*/
default GetJobTaggingResponse getJobTagging(GetJobTaggingRequest getJobTaggingRequest) throws InternalServiceException,
TooManyRequestsException, NotFoundException, AwsServiceException, SdkClientException, S3ControlException {
throw new UnsupportedOperationException();
}
/**
*
* Returns the tags on an S3 Batch Operations job. To use this operation, you must have permission to perform the
* s3:GetJobTagging
action. For more information, see Controlling access and labeling jobs using tags in the Amazon Simple Storage Service User Guide.
*
*
*
* Related actions include:
*
*
* -
*
* CreateJob
*
*
* -
*
* PutJobTagging
*
*
* -
*
* DeleteJobTagging
*
*
*
*
*
* This is a convenience which creates an instance of the {@link GetJobTaggingRequest.Builder} avoiding the need to
* create one manually via {@link GetJobTaggingRequest#builder()}
*
*
* @param getJobTaggingRequest
* A {@link Consumer} that will call methods on {@link GetJobTaggingRequest.Builder} to create a request.
* @return Result of the GetJobTagging operation returned by the service.
* @throws InternalServiceException
* @throws TooManyRequestsException
* @throws NotFoundException
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.GetJobTagging
* @see AWS API
* Documentation
*/
default GetJobTaggingResponse getJobTagging(Consumer getJobTaggingRequest)
throws InternalServiceException, TooManyRequestsException, NotFoundException, AwsServiceException,
SdkClientException, S3ControlException {
return getJobTagging(GetJobTaggingRequest.builder().applyMutation(getJobTaggingRequest).build());
}
/**
*
* Retrieves the PublicAccessBlock
configuration for an AWS account. For more information, see Using Amazon S3
* block public access.
*
*
* Related actions include:
*
*
* -
*
*
* -
*
*
*
*
* @param getPublicAccessBlockRequest
* @return Result of the GetPublicAccessBlock operation returned by the service.
* @throws NoSuchPublicAccessBlockConfigurationException
* Amazon S3 throws this exception if you make a GetPublicAccessBlock
request against an
* account that doesn't have a PublicAccessBlockConfiguration
set.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.GetPublicAccessBlock
* @see AWS API Documentation
*/
default GetPublicAccessBlockResponse getPublicAccessBlock(GetPublicAccessBlockRequest getPublicAccessBlockRequest)
throws NoSuchPublicAccessBlockConfigurationException, AwsServiceException, SdkClientException, S3ControlException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the PublicAccessBlock
configuration for an AWS account. For more information, see Using Amazon S3
* block public access.
*
*
* Related actions include:
*
*
* -
*
*
* -
*
*
*
*
*
* This is a convenience which creates an instance of the {@link GetPublicAccessBlockRequest.Builder} avoiding the
* need to create one manually via {@link GetPublicAccessBlockRequest#builder()}
*
*
* @param getPublicAccessBlockRequest
* A {@link Consumer} that will call methods on {@link GetPublicAccessBlockRequest.Builder} to create a
* request.
* @return Result of the GetPublicAccessBlock operation returned by the service.
* @throws NoSuchPublicAccessBlockConfigurationException
* Amazon S3 throws this exception if you make a GetPublicAccessBlock
request against an
* account that doesn't have a PublicAccessBlockConfiguration
set.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.GetPublicAccessBlock
* @see AWS API Documentation
*/
default GetPublicAccessBlockResponse getPublicAccessBlock(
Consumer getPublicAccessBlockRequest)
throws NoSuchPublicAccessBlockConfigurationException, AwsServiceException, SdkClientException, S3ControlException {
return getPublicAccessBlock(GetPublicAccessBlockRequest.builder().applyMutation(getPublicAccessBlockRequest).build());
}
/**
*
* Gets the Amazon S3 Storage Lens configuration. For more information, see Assessing your storage activity and
* usage with Amazon S3 Storage Lens in the Amazon Simple Storage Service User Guide.
*
*
*
* To use this action, you must have permission to perform the s3:GetStorageLensConfiguration
action.
* For more information, see Setting permissions to
* use Amazon S3 Storage Lens in the Amazon Simple Storage Service User Guide.
*
*
*
* @param getStorageLensConfigurationRequest
* @return Result of the GetStorageLensConfiguration operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.GetStorageLensConfiguration
* @see AWS API Documentation
*/
default GetStorageLensConfigurationResponse getStorageLensConfiguration(
GetStorageLensConfigurationRequest getStorageLensConfigurationRequest) throws AwsServiceException,
SdkClientException, S3ControlException {
throw new UnsupportedOperationException();
}
/**
*
* Gets the Amazon S3 Storage Lens configuration. For more information, see Assessing your storage activity and
* usage with Amazon S3 Storage Lens in the Amazon Simple Storage Service User Guide.
*
*
*
* To use this action, you must have permission to perform the s3:GetStorageLensConfiguration
action.
* For more information, see Setting permissions to
* use Amazon S3 Storage Lens in the Amazon Simple Storage Service User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link GetStorageLensConfigurationRequest.Builder}
* avoiding the need to create one manually via {@link GetStorageLensConfigurationRequest#builder()}
*
*
* @param getStorageLensConfigurationRequest
* A {@link Consumer} that will call methods on {@link GetStorageLensConfigurationRequest.Builder} to create
* a request.
* @return Result of the GetStorageLensConfiguration operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.GetStorageLensConfiguration
* @see AWS API Documentation
*/
default GetStorageLensConfigurationResponse getStorageLensConfiguration(
Consumer getStorageLensConfigurationRequest) throws AwsServiceException,
SdkClientException, S3ControlException {
return getStorageLensConfiguration(GetStorageLensConfigurationRequest.builder()
.applyMutation(getStorageLensConfigurationRequest).build());
}
/**
*
* Gets the tags of Amazon S3 Storage Lens configuration. For more information about S3 Storage Lens, see Assessing your storage activity and
* usage with Amazon S3 Storage Lens in the Amazon Simple Storage Service User Guide.
*
*
*
* To use this action, you must have permission to perform the s3:GetStorageLensConfigurationTagging
* action. For more information, see Setting permissions to
* use Amazon S3 Storage Lens in the Amazon Simple Storage Service User Guide.
*
*
*
* @param getStorageLensConfigurationTaggingRequest
* @return Result of the GetStorageLensConfigurationTagging operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.GetStorageLensConfigurationTagging
* @see AWS API Documentation
*/
default GetStorageLensConfigurationTaggingResponse getStorageLensConfigurationTagging(
GetStorageLensConfigurationTaggingRequest getStorageLensConfigurationTaggingRequest) throws AwsServiceException,
SdkClientException, S3ControlException {
throw new UnsupportedOperationException();
}
/**
*
* Gets the tags of Amazon S3 Storage Lens configuration. For more information about S3 Storage Lens, see Assessing your storage activity and
* usage with Amazon S3 Storage Lens in the Amazon Simple Storage Service User Guide.
*
*
*
* To use this action, you must have permission to perform the s3:GetStorageLensConfigurationTagging
* action. For more information, see Setting permissions to
* use Amazon S3 Storage Lens in the Amazon Simple Storage Service User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link GetStorageLensConfigurationTaggingRequest.Builder}
* avoiding the need to create one manually via {@link GetStorageLensConfigurationTaggingRequest#builder()}
*
*
* @param getStorageLensConfigurationTaggingRequest
* A {@link Consumer} that will call methods on {@link GetStorageLensConfigurationTaggingRequest.Builder} to
* create a request.
* @return Result of the GetStorageLensConfigurationTagging operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.GetStorageLensConfigurationTagging
* @see AWS API Documentation
*/
default GetStorageLensConfigurationTaggingResponse getStorageLensConfigurationTagging(
Consumer getStorageLensConfigurationTaggingRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
return getStorageLensConfigurationTagging(GetStorageLensConfigurationTaggingRequest.builder()
.applyMutation(getStorageLensConfigurationTaggingRequest).build());
}
/**
*
* Returns a list of the access points currently associated with the specified bucket. You can retrieve up to 1000
* access points per call. If the specified bucket has more than 1,000 access points (or the number specified in
* maxResults
, whichever is less), the response will include a continuation token that you can use to
* list the additional access points.
*
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request and an S3 on Outposts endpoint hostname prefix
* instead of s3-control
. For an example of the request syntax for Amazon S3 on Outposts that uses the
* S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived using the access point ARN,
* see the Examples section.
*
*
* The following actions are related to ListAccessPoints
:
*
*
* -
*
*
* -
*
*
* -
*
* GetAccessPoint
*
*
*
*
* @param listAccessPointsRequest
* @return Result of the ListAccessPoints operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.ListAccessPoints
* @see AWS
* API Documentation
*/
default ListAccessPointsResponse listAccessPoints(ListAccessPointsRequest listAccessPointsRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of the access points currently associated with the specified bucket. You can retrieve up to 1000
* access points per call. If the specified bucket has more than 1,000 access points (or the number specified in
* maxResults
, whichever is less), the response will include a continuation token that you can use to
* list the additional access points.
*
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request and an S3 on Outposts endpoint hostname prefix
* instead of s3-control
. For an example of the request syntax for Amazon S3 on Outposts that uses the
* S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived using the access point ARN,
* see the Examples section.
*
*
* The following actions are related to ListAccessPoints
:
*
*
* -
*
*
* -
*
*
* -
*
* GetAccessPoint
*
*
*
*
*
* This is a convenience which creates an instance of the {@link ListAccessPointsRequest.Builder} avoiding the need
* to create one manually via {@link ListAccessPointsRequest#builder()}
*
*
* @param listAccessPointsRequest
* A {@link Consumer} that will call methods on {@link ListAccessPointsRequest.Builder} to create a request.
* @return Result of the ListAccessPoints operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.ListAccessPoints
* @see AWS
* API Documentation
*/
default ListAccessPointsResponse listAccessPoints(Consumer listAccessPointsRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
return listAccessPoints(ListAccessPointsRequest.builder().applyMutation(listAccessPointsRequest).build());
}
/**
*
* Returns a list of the access points currently associated with the specified bucket. You can retrieve up to 1000
* access points per call. If the specified bucket has more than 1,000 access points (or the number specified in
* maxResults
, whichever is less), the response will include a continuation token that you can use to
* list the additional access points.
*
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request and an S3 on Outposts endpoint hostname prefix
* instead of s3-control
. For an example of the request syntax for Amazon S3 on Outposts that uses the
* S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived using the access point ARN,
* see the Examples section.
*
*
* The following actions are related to ListAccessPoints
:
*
*
* -
*
*
* -
*
*
* -
*
* GetAccessPoint
*
*
*
*
*
* This is a variant of
* {@link #listAccessPoints(software.amazon.awssdk.services.s3control.model.ListAccessPointsRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.s3control.paginators.ListAccessPointsIterable responses = client.listAccessPointsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.s3control.paginators.ListAccessPointsIterable responses = client
* .listAccessPointsPaginator(request);
* for (software.amazon.awssdk.services.s3control.model.ListAccessPointsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.s3control.paginators.ListAccessPointsIterable responses = client.listAccessPointsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listAccessPoints(software.amazon.awssdk.services.s3control.model.ListAccessPointsRequest)} operation.
*
*
* @param listAccessPointsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.ListAccessPoints
* @see AWS
* API Documentation
*/
default ListAccessPointsIterable listAccessPointsPaginator(ListAccessPointsRequest listAccessPointsRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of the access points currently associated with the specified bucket. You can retrieve up to 1000
* access points per call. If the specified bucket has more than 1,000 access points (or the number specified in
* maxResults
, whichever is less), the response will include a continuation token that you can use to
* list the additional access points.
*
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request and an S3 on Outposts endpoint hostname prefix
* instead of s3-control
. For an example of the request syntax for Amazon S3 on Outposts that uses the
* S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived using the access point ARN,
* see the Examples section.
*
*
* The following actions are related to ListAccessPoints
:
*
*
* -
*
*
* -
*
*
* -
*
* GetAccessPoint
*
*
*
*
*
* This is a variant of
* {@link #listAccessPoints(software.amazon.awssdk.services.s3control.model.ListAccessPointsRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.s3control.paginators.ListAccessPointsIterable responses = client.listAccessPointsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.s3control.paginators.ListAccessPointsIterable responses = client
* .listAccessPointsPaginator(request);
* for (software.amazon.awssdk.services.s3control.model.ListAccessPointsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.s3control.paginators.ListAccessPointsIterable responses = client.listAccessPointsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listAccessPoints(software.amazon.awssdk.services.s3control.model.ListAccessPointsRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListAccessPointsRequest.Builder} avoiding the need
* to create one manually via {@link ListAccessPointsRequest#builder()}
*
*
* @param listAccessPointsRequest
* A {@link Consumer} that will call methods on {@link ListAccessPointsRequest.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.ListAccessPoints
* @see AWS
* API Documentation
*/
default ListAccessPointsIterable listAccessPointsPaginator(Consumer listAccessPointsRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
return listAccessPointsPaginator(ListAccessPointsRequest.builder().applyMutation(listAccessPointsRequest).build());
}
/**
*
* Returns a list of the access points associated with the Object Lambda Access Point. You can retrieve up to 1000
* access points per call. If there are more than 1,000 access points (or the number specified in
* maxResults
, whichever is less), the response will include a continuation token that you can use to
* list the additional access points.
*
*
* The following actions are related to ListAccessPointsForObjectLambda
:
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* @param listAccessPointsForObjectLambdaRequest
* @return Result of the ListAccessPointsForObjectLambda operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.ListAccessPointsForObjectLambda
* @see AWS API Documentation
*/
default ListAccessPointsForObjectLambdaResponse listAccessPointsForObjectLambda(
ListAccessPointsForObjectLambdaRequest listAccessPointsForObjectLambdaRequest) throws AwsServiceException,
SdkClientException, S3ControlException {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of the access points associated with the Object Lambda Access Point. You can retrieve up to 1000
* access points per call. If there are more than 1,000 access points (or the number specified in
* maxResults
, whichever is less), the response will include a continuation token that you can use to
* list the additional access points.
*
*
* The following actions are related to ListAccessPointsForObjectLambda
:
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
*
* This is a convenience which creates an instance of the {@link ListAccessPointsForObjectLambdaRequest.Builder}
* avoiding the need to create one manually via {@link ListAccessPointsForObjectLambdaRequest#builder()}
*
*
* @param listAccessPointsForObjectLambdaRequest
* A {@link Consumer} that will call methods on {@link ListAccessPointsForObjectLambdaRequest.Builder} to
* create a request.
* @return Result of the ListAccessPointsForObjectLambda operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.ListAccessPointsForObjectLambda
* @see AWS API Documentation
*/
default ListAccessPointsForObjectLambdaResponse listAccessPointsForObjectLambda(
Consumer listAccessPointsForObjectLambdaRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
return listAccessPointsForObjectLambda(ListAccessPointsForObjectLambdaRequest.builder()
.applyMutation(listAccessPointsForObjectLambdaRequest).build());
}
/**
*
* Returns a list of the access points associated with the Object Lambda Access Point. You can retrieve up to 1000
* access points per call. If there are more than 1,000 access points (or the number specified in
* maxResults
, whichever is less), the response will include a continuation token that you can use to
* list the additional access points.
*
*
* The following actions are related to ListAccessPointsForObjectLambda
:
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
*
* This is a variant of
* {@link #listAccessPointsForObjectLambda(software.amazon.awssdk.services.s3control.model.ListAccessPointsForObjectLambdaRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.s3control.paginators.ListAccessPointsForObjectLambdaIterable responses = client.listAccessPointsForObjectLambdaPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.s3control.paginators.ListAccessPointsForObjectLambdaIterable responses = client
* .listAccessPointsForObjectLambdaPaginator(request);
* for (software.amazon.awssdk.services.s3control.model.ListAccessPointsForObjectLambdaResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.s3control.paginators.ListAccessPointsForObjectLambdaIterable responses = client.listAccessPointsForObjectLambdaPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listAccessPointsForObjectLambda(software.amazon.awssdk.services.s3control.model.ListAccessPointsForObjectLambdaRequest)}
* operation.
*
*
* @param listAccessPointsForObjectLambdaRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.ListAccessPointsForObjectLambda
* @see AWS API Documentation
*/
default ListAccessPointsForObjectLambdaIterable listAccessPointsForObjectLambdaPaginator(
ListAccessPointsForObjectLambdaRequest listAccessPointsForObjectLambdaRequest) throws AwsServiceException,
SdkClientException, S3ControlException {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of the access points associated with the Object Lambda Access Point. You can retrieve up to 1000
* access points per call. If there are more than 1,000 access points (or the number specified in
* maxResults
, whichever is less), the response will include a continuation token that you can use to
* list the additional access points.
*
*
* The following actions are related to ListAccessPointsForObjectLambda
:
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
*
* This is a variant of
* {@link #listAccessPointsForObjectLambda(software.amazon.awssdk.services.s3control.model.ListAccessPointsForObjectLambdaRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.s3control.paginators.ListAccessPointsForObjectLambdaIterable responses = client.listAccessPointsForObjectLambdaPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.s3control.paginators.ListAccessPointsForObjectLambdaIterable responses = client
* .listAccessPointsForObjectLambdaPaginator(request);
* for (software.amazon.awssdk.services.s3control.model.ListAccessPointsForObjectLambdaResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.s3control.paginators.ListAccessPointsForObjectLambdaIterable responses = client.listAccessPointsForObjectLambdaPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listAccessPointsForObjectLambda(software.amazon.awssdk.services.s3control.model.ListAccessPointsForObjectLambdaRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListAccessPointsForObjectLambdaRequest.Builder}
* avoiding the need to create one manually via {@link ListAccessPointsForObjectLambdaRequest#builder()}
*
*
* @param listAccessPointsForObjectLambdaRequest
* A {@link Consumer} that will call methods on {@link ListAccessPointsForObjectLambdaRequest.Builder} to
* create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.ListAccessPointsForObjectLambda
* @see AWS API Documentation
*/
default ListAccessPointsForObjectLambdaIterable listAccessPointsForObjectLambdaPaginator(
Consumer listAccessPointsForObjectLambdaRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
return listAccessPointsForObjectLambdaPaginator(ListAccessPointsForObjectLambdaRequest.builder()
.applyMutation(listAccessPointsForObjectLambdaRequest).build());
}
/**
*
* Lists current S3 Batch Operations jobs and jobs that have ended within the last 30 days for the AWS account
* making the request. For more information, see S3 Batch Operations in the
* Amazon Simple Storage Service User Guide.
*
*
* Related actions include:
*
*
*
* -
*
* CreateJob
*
*
* -
*
* DescribeJob
*
*
* -
*
*
* -
*
* UpdateJobStatus
*
*
*
*
* @param listJobsRequest
* @return Result of the ListJobs operation returned by the service.
* @throws InvalidRequestException
* @throws InternalServiceException
* @throws InvalidNextTokenException
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.ListJobs
* @see AWS API
* Documentation
*/
default ListJobsResponse listJobs(ListJobsRequest listJobsRequest) throws InvalidRequestException, InternalServiceException,
InvalidNextTokenException, AwsServiceException, SdkClientException, S3ControlException {
throw new UnsupportedOperationException();
}
/**
*
* Lists current S3 Batch Operations jobs and jobs that have ended within the last 30 days for the AWS account
* making the request. For more information, see S3 Batch Operations in the
* Amazon Simple Storage Service User Guide.
*
*
* Related actions include:
*
*
*
* -
*
* CreateJob
*
*
* -
*
* DescribeJob
*
*
* -
*
*
* -
*
* UpdateJobStatus
*
*
*
*
*
* This is a convenience which creates an instance of the {@link ListJobsRequest.Builder} avoiding the need to
* create one manually via {@link ListJobsRequest#builder()}
*
*
* @param listJobsRequest
* A {@link Consumer} that will call methods on {@link ListJobsRequest.Builder} to create a request.
* @return Result of the ListJobs operation returned by the service.
* @throws InvalidRequestException
* @throws InternalServiceException
* @throws InvalidNextTokenException
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.ListJobs
* @see AWS API
* Documentation
*/
default ListJobsResponse listJobs(Consumer listJobsRequest) throws InvalidRequestException,
InternalServiceException, InvalidNextTokenException, AwsServiceException, SdkClientException, S3ControlException {
return listJobs(ListJobsRequest.builder().applyMutation(listJobsRequest).build());
}
/**
*
* Lists current S3 Batch Operations jobs and jobs that have ended within the last 30 days for the AWS account
* making the request. For more information, see S3 Batch Operations in the
* Amazon Simple Storage Service User Guide.
*
*
* Related actions include:
*
*
*
* -
*
* CreateJob
*
*
* -
*
* DescribeJob
*
*
* -
*
*
* -
*
* UpdateJobStatus
*
*
*
*
*
* This is a variant of {@link #listJobs(software.amazon.awssdk.services.s3control.model.ListJobsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.s3control.paginators.ListJobsIterable responses = client.listJobsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.s3control.paginators.ListJobsIterable responses = client.listJobsPaginator(request);
* for (software.amazon.awssdk.services.s3control.model.ListJobsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.s3control.paginators.ListJobsIterable responses = client.listJobsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listJobs(software.amazon.awssdk.services.s3control.model.ListJobsRequest)} operation.
*
*
* @param listJobsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidRequestException
* @throws InternalServiceException
* @throws InvalidNextTokenException
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.ListJobs
* @see AWS API
* Documentation
*/
default ListJobsIterable listJobsPaginator(ListJobsRequest listJobsRequest) throws InvalidRequestException,
InternalServiceException, InvalidNextTokenException, AwsServiceException, SdkClientException, S3ControlException {
throw new UnsupportedOperationException();
}
/**
*
* Lists current S3 Batch Operations jobs and jobs that have ended within the last 30 days for the AWS account
* making the request. For more information, see S3 Batch Operations in the
* Amazon Simple Storage Service User Guide.
*
*
* Related actions include:
*
*
*
* -
*
* CreateJob
*
*
* -
*
* DescribeJob
*
*
* -
*
*
* -
*
* UpdateJobStatus
*
*
*
*
*
* This is a variant of {@link #listJobs(software.amazon.awssdk.services.s3control.model.ListJobsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.s3control.paginators.ListJobsIterable responses = client.listJobsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.s3control.paginators.ListJobsIterable responses = client.listJobsPaginator(request);
* for (software.amazon.awssdk.services.s3control.model.ListJobsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.s3control.paginators.ListJobsIterable responses = client.listJobsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listJobs(software.amazon.awssdk.services.s3control.model.ListJobsRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListJobsRequest.Builder} avoiding the need to
* create one manually via {@link ListJobsRequest#builder()}
*
*
* @param listJobsRequest
* A {@link Consumer} that will call methods on {@link ListJobsRequest.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidRequestException
* @throws InternalServiceException
* @throws InvalidNextTokenException
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.ListJobs
* @see AWS API
* Documentation
*/
default ListJobsIterable listJobsPaginator(Consumer listJobsRequest) throws InvalidRequestException,
InternalServiceException, InvalidNextTokenException, AwsServiceException, SdkClientException, S3ControlException {
return listJobsPaginator(ListJobsRequest.builder().applyMutation(listJobsRequest).build());
}
/**
*
* Returns a list of all Outposts buckets in an Outpost that are owned by the authenticated sender of the request.
* For more information, see Using
* Amazon S3 on Outposts in the Amazon Simple Storage Service User Guide.
*
*
* For an example of the request syntax for Amazon S3 on Outposts that uses the S3 on Outposts endpoint hostname
* prefix and x-amz-outpost-id
in your request, see the Examples section.
*
*
* @param listRegionalBucketsRequest
* @return Result of the ListRegionalBuckets operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.ListRegionalBuckets
* @see AWS
* API Documentation
*/
default ListRegionalBucketsResponse listRegionalBuckets(ListRegionalBucketsRequest listRegionalBucketsRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of all Outposts buckets in an Outpost that are owned by the authenticated sender of the request.
* For more information, see Using
* Amazon S3 on Outposts in the Amazon Simple Storage Service User Guide.
*
*
* For an example of the request syntax for Amazon S3 on Outposts that uses the S3 on Outposts endpoint hostname
* prefix and x-amz-outpost-id
in your request, see the Examples section.
*
*
*
* This is a convenience which creates an instance of the {@link ListRegionalBucketsRequest.Builder} avoiding the
* need to create one manually via {@link ListRegionalBucketsRequest#builder()}
*
*
* @param listRegionalBucketsRequest
* A {@link Consumer} that will call methods on {@link ListRegionalBucketsRequest.Builder} to create a
* request.
* @return Result of the ListRegionalBuckets operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.ListRegionalBuckets
* @see AWS
* API Documentation
*/
default ListRegionalBucketsResponse listRegionalBuckets(
Consumer listRegionalBucketsRequest) throws AwsServiceException,
SdkClientException, S3ControlException {
return listRegionalBuckets(ListRegionalBucketsRequest.builder().applyMutation(listRegionalBucketsRequest).build());
}
/**
*
* Returns a list of all Outposts buckets in an Outpost that are owned by the authenticated sender of the request.
* For more information, see Using
* Amazon S3 on Outposts in the Amazon Simple Storage Service User Guide.
*
*
* For an example of the request syntax for Amazon S3 on Outposts that uses the S3 on Outposts endpoint hostname
* prefix and x-amz-outpost-id
in your request, see the Examples section.
*
*
*
* This is a variant of
* {@link #listRegionalBuckets(software.amazon.awssdk.services.s3control.model.ListRegionalBucketsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.s3control.paginators.ListRegionalBucketsIterable responses = client.listRegionalBucketsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.s3control.paginators.ListRegionalBucketsIterable responses = client
* .listRegionalBucketsPaginator(request);
* for (software.amazon.awssdk.services.s3control.model.ListRegionalBucketsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.s3control.paginators.ListRegionalBucketsIterable responses = client.listRegionalBucketsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listRegionalBuckets(software.amazon.awssdk.services.s3control.model.ListRegionalBucketsRequest)}
* operation.
*
*
* @param listRegionalBucketsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.ListRegionalBuckets
* @see AWS
* API Documentation
*/
default ListRegionalBucketsIterable listRegionalBucketsPaginator(ListRegionalBucketsRequest listRegionalBucketsRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of all Outposts buckets in an Outpost that are owned by the authenticated sender of the request.
* For more information, see Using
* Amazon S3 on Outposts in the Amazon Simple Storage Service User Guide.
*
*
* For an example of the request syntax for Amazon S3 on Outposts that uses the S3 on Outposts endpoint hostname
* prefix and x-amz-outpost-id
in your request, see the Examples section.
*
*
*
* This is a variant of
* {@link #listRegionalBuckets(software.amazon.awssdk.services.s3control.model.ListRegionalBucketsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.s3control.paginators.ListRegionalBucketsIterable responses = client.listRegionalBucketsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.s3control.paginators.ListRegionalBucketsIterable responses = client
* .listRegionalBucketsPaginator(request);
* for (software.amazon.awssdk.services.s3control.model.ListRegionalBucketsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.s3control.paginators.ListRegionalBucketsIterable responses = client.listRegionalBucketsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listRegionalBuckets(software.amazon.awssdk.services.s3control.model.ListRegionalBucketsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListRegionalBucketsRequest.Builder} avoiding the
* need to create one manually via {@link ListRegionalBucketsRequest#builder()}
*
*
* @param listRegionalBucketsRequest
* A {@link Consumer} that will call methods on {@link ListRegionalBucketsRequest.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.ListRegionalBuckets
* @see AWS
* API Documentation
*/
default ListRegionalBucketsIterable listRegionalBucketsPaginator(
Consumer listRegionalBucketsRequest) throws AwsServiceException,
SdkClientException, S3ControlException {
return listRegionalBucketsPaginator(ListRegionalBucketsRequest.builder().applyMutation(listRegionalBucketsRequest)
.build());
}
/**
*
* Gets a list of Amazon S3 Storage Lens configurations. For more information about S3 Storage Lens, see Assessing your storage activity and
* usage with Amazon S3 Storage Lens in the Amazon Simple Storage Service User Guide.
*
*
*
* To use this action, you must have permission to perform the s3:ListStorageLensConfigurations
action.
* For more information, see Setting permissions to
* use Amazon S3 Storage Lens in the Amazon Simple Storage Service User Guide.
*
*
*
* @param listStorageLensConfigurationsRequest
* @return Result of the ListStorageLensConfigurations operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.ListStorageLensConfigurations
* @see AWS API Documentation
*/
default ListStorageLensConfigurationsResponse listStorageLensConfigurations(
ListStorageLensConfigurationsRequest listStorageLensConfigurationsRequest) throws AwsServiceException,
SdkClientException, S3ControlException {
throw new UnsupportedOperationException();
}
/**
*
* Gets a list of Amazon S3 Storage Lens configurations. For more information about S3 Storage Lens, see Assessing your storage activity and
* usage with Amazon S3 Storage Lens in the Amazon Simple Storage Service User Guide.
*
*
*
* To use this action, you must have permission to perform the s3:ListStorageLensConfigurations
action.
* For more information, see Setting permissions to
* use Amazon S3 Storage Lens in the Amazon Simple Storage Service User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link ListStorageLensConfigurationsRequest.Builder}
* avoiding the need to create one manually via {@link ListStorageLensConfigurationsRequest#builder()}
*
*
* @param listStorageLensConfigurationsRequest
* A {@link Consumer} that will call methods on {@link ListStorageLensConfigurationsRequest.Builder} to
* create a request.
* @return Result of the ListStorageLensConfigurations operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.ListStorageLensConfigurations
* @see AWS API Documentation
*/
default ListStorageLensConfigurationsResponse listStorageLensConfigurations(
Consumer listStorageLensConfigurationsRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
return listStorageLensConfigurations(ListStorageLensConfigurationsRequest.builder()
.applyMutation(listStorageLensConfigurationsRequest).build());
}
/**
*
* Gets a list of Amazon S3 Storage Lens configurations. For more information about S3 Storage Lens, see Assessing your storage activity and
* usage with Amazon S3 Storage Lens in the Amazon Simple Storage Service User Guide.
*
*
*
* To use this action, you must have permission to perform the s3:ListStorageLensConfigurations
action.
* For more information, see Setting permissions to
* use Amazon S3 Storage Lens in the Amazon Simple Storage Service User Guide.
*
*
*
* This is a variant of
* {@link #listStorageLensConfigurations(software.amazon.awssdk.services.s3control.model.ListStorageLensConfigurationsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.s3control.paginators.ListStorageLensConfigurationsIterable responses = client.listStorageLensConfigurationsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.s3control.paginators.ListStorageLensConfigurationsIterable responses = client
* .listStorageLensConfigurationsPaginator(request);
* for (software.amazon.awssdk.services.s3control.model.ListStorageLensConfigurationsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.s3control.paginators.ListStorageLensConfigurationsIterable responses = client.listStorageLensConfigurationsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listStorageLensConfigurations(software.amazon.awssdk.services.s3control.model.ListStorageLensConfigurationsRequest)}
* operation.
*
*
* @param listStorageLensConfigurationsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.ListStorageLensConfigurations
* @see AWS API Documentation
*/
default ListStorageLensConfigurationsIterable listStorageLensConfigurationsPaginator(
ListStorageLensConfigurationsRequest listStorageLensConfigurationsRequest) throws AwsServiceException,
SdkClientException, S3ControlException {
throw new UnsupportedOperationException();
}
/**
*
* Gets a list of Amazon S3 Storage Lens configurations. For more information about S3 Storage Lens, see Assessing your storage activity and
* usage with Amazon S3 Storage Lens in the Amazon Simple Storage Service User Guide.
*
*
*
* To use this action, you must have permission to perform the s3:ListStorageLensConfigurations
action.
* For more information, see Setting permissions to
* use Amazon S3 Storage Lens in the Amazon Simple Storage Service User Guide.
*
*
*
* This is a variant of
* {@link #listStorageLensConfigurations(software.amazon.awssdk.services.s3control.model.ListStorageLensConfigurationsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.s3control.paginators.ListStorageLensConfigurationsIterable responses = client.listStorageLensConfigurationsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.s3control.paginators.ListStorageLensConfigurationsIterable responses = client
* .listStorageLensConfigurationsPaginator(request);
* for (software.amazon.awssdk.services.s3control.model.ListStorageLensConfigurationsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.s3control.paginators.ListStorageLensConfigurationsIterable responses = client.listStorageLensConfigurationsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listStorageLensConfigurations(software.amazon.awssdk.services.s3control.model.ListStorageLensConfigurationsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListStorageLensConfigurationsRequest.Builder}
* avoiding the need to create one manually via {@link ListStorageLensConfigurationsRequest#builder()}
*
*
* @param listStorageLensConfigurationsRequest
* A {@link Consumer} that will call methods on {@link ListStorageLensConfigurationsRequest.Builder} to
* create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.ListStorageLensConfigurations
* @see AWS API Documentation
*/
default ListStorageLensConfigurationsIterable listStorageLensConfigurationsPaginator(
Consumer listStorageLensConfigurationsRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
return listStorageLensConfigurationsPaginator(ListStorageLensConfigurationsRequest.builder()
.applyMutation(listStorageLensConfigurationsRequest).build());
}
/**
*
* Replaces configuration for an Object Lambda Access Point.
*
*
* The following actions are related to PutAccessPointConfigurationForObjectLambda
:
*
*
* -
*
*
*
*
* @param putAccessPointConfigurationForObjectLambdaRequest
* @return Result of the PutAccessPointConfigurationForObjectLambda operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.PutAccessPointConfigurationForObjectLambda
* @see AWS API Documentation
*/
default PutAccessPointConfigurationForObjectLambdaResponse putAccessPointConfigurationForObjectLambda(
PutAccessPointConfigurationForObjectLambdaRequest putAccessPointConfigurationForObjectLambdaRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
throw new UnsupportedOperationException();
}
/**
*
* Replaces configuration for an Object Lambda Access Point.
*
*
* The following actions are related to PutAccessPointConfigurationForObjectLambda
:
*
*
* -
*
*
*
*
*
* This is a convenience which creates an instance of the
* {@link PutAccessPointConfigurationForObjectLambdaRequest.Builder} avoiding the need to create one manually via
* {@link PutAccessPointConfigurationForObjectLambdaRequest#builder()}
*
*
* @param putAccessPointConfigurationForObjectLambdaRequest
* A {@link Consumer} that will call methods on
* {@link PutAccessPointConfigurationForObjectLambdaRequest.Builder} to create a request.
* @return Result of the PutAccessPointConfigurationForObjectLambda operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.PutAccessPointConfigurationForObjectLambda
* @see AWS API Documentation
*/
default PutAccessPointConfigurationForObjectLambdaResponse putAccessPointConfigurationForObjectLambda(
Consumer putAccessPointConfigurationForObjectLambdaRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
return putAccessPointConfigurationForObjectLambda(PutAccessPointConfigurationForObjectLambdaRequest.builder()
.applyMutation(putAccessPointConfigurationForObjectLambdaRequest).build());
}
/**
*
* Associates an access policy with the specified access point. Each access point can have only one policy, so a
* request made to this API replaces any existing policy associated with the specified access point.
*
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request and an S3 on Outposts endpoint hostname prefix
* instead of s3-control
. For an example of the request syntax for Amazon S3 on Outposts that uses the
* S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived using the access point ARN,
* see the Examples section.
*
*
* The following actions are related to PutAccessPointPolicy
:
*
*
* -
*
*
* -
*
*
*
*
* @param putAccessPointPolicyRequest
* @return Result of the PutAccessPointPolicy operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.PutAccessPointPolicy
* @see AWS API Documentation
*/
default PutAccessPointPolicyResponse putAccessPointPolicy(PutAccessPointPolicyRequest putAccessPointPolicyRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
throw new UnsupportedOperationException();
}
/**
*
* Associates an access policy with the specified access point. Each access point can have only one policy, so a
* request made to this API replaces any existing policy associated with the specified access point.
*
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request and an S3 on Outposts endpoint hostname prefix
* instead of s3-control
. For an example of the request syntax for Amazon S3 on Outposts that uses the
* S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived using the access point ARN,
* see the Examples section.
*
*
* The following actions are related to PutAccessPointPolicy
:
*
*
* -
*
*
* -
*
*
*
*
*
* This is a convenience which creates an instance of the {@link PutAccessPointPolicyRequest.Builder} avoiding the
* need to create one manually via {@link PutAccessPointPolicyRequest#builder()}
*
*
* @param putAccessPointPolicyRequest
* A {@link Consumer} that will call methods on {@link PutAccessPointPolicyRequest.Builder} to create a
* request.
* @return Result of the PutAccessPointPolicy operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.PutAccessPointPolicy
* @see AWS API Documentation
*/
default PutAccessPointPolicyResponse putAccessPointPolicy(
Consumer putAccessPointPolicyRequest) throws AwsServiceException,
SdkClientException, S3ControlException {
return putAccessPointPolicy(PutAccessPointPolicyRequest.builder().applyMutation(putAccessPointPolicyRequest).build());
}
/**
*
* Creates or replaces resource policy for an Object Lambda Access Point. For an example policy, see Creating Object
* Lambda Access Points in the Amazon Simple Storage Service User Guide.
*
*
* The following actions are related to PutAccessPointPolicyForObjectLambda
:
*
*
* -
*
*
* -
*
*
*
*
* @param putAccessPointPolicyForObjectLambdaRequest
* @return Result of the PutAccessPointPolicyForObjectLambda operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.PutAccessPointPolicyForObjectLambda
* @see AWS API Documentation
*/
default PutAccessPointPolicyForObjectLambdaResponse putAccessPointPolicyForObjectLambda(
PutAccessPointPolicyForObjectLambdaRequest putAccessPointPolicyForObjectLambdaRequest) throws AwsServiceException,
SdkClientException, S3ControlException {
throw new UnsupportedOperationException();
}
/**
*
* Creates or replaces resource policy for an Object Lambda Access Point. For an example policy, see Creating Object
* Lambda Access Points in the Amazon Simple Storage Service User Guide.
*
*
* The following actions are related to PutAccessPointPolicyForObjectLambda
:
*
*
* -
*
*
* -
*
*
*
*
*
* This is a convenience which creates an instance of the {@link PutAccessPointPolicyForObjectLambdaRequest.Builder}
* avoiding the need to create one manually via {@link PutAccessPointPolicyForObjectLambdaRequest#builder()}
*
*
* @param putAccessPointPolicyForObjectLambdaRequest
* A {@link Consumer} that will call methods on {@link PutAccessPointPolicyForObjectLambdaRequest.Builder} to
* create a request.
* @return Result of the PutAccessPointPolicyForObjectLambda operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.PutAccessPointPolicyForObjectLambda
* @see AWS API Documentation
*/
default PutAccessPointPolicyForObjectLambdaResponse putAccessPointPolicyForObjectLambda(
Consumer putAccessPointPolicyForObjectLambdaRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
return putAccessPointPolicyForObjectLambda(PutAccessPointPolicyForObjectLambdaRequest.builder()
.applyMutation(putAccessPointPolicyForObjectLambdaRequest).build());
}
/**
*
*
* This action puts a lifecycle configuration to an Amazon S3 on Outposts bucket. To put a lifecycle configuration
* to an S3 bucket, see PutBucketLifecycleConfiguration in the Amazon Simple Storage Service API.
*
*
*
* Creates a new lifecycle configuration for the S3 on Outposts bucket or replaces an existing lifecycle
* configuration. Outposts buckets only support lifecycle configurations that delete/expire objects after a certain
* period of time and abort incomplete multipart uploads.
*
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request and an S3 on Outposts endpoint hostname prefix
* instead of s3-control
. For an example of the request syntax for Amazon S3 on Outposts that uses the
* S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived using the access point ARN,
* see the Examples section.
*
*
* The following actions are related to PutBucketLifecycleConfiguration
:
*
*
* -
*
*
* -
*
*
*
*
* @param putBucketLifecycleConfigurationRequest
* @return Result of the PutBucketLifecycleConfiguration operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.PutBucketLifecycleConfiguration
* @see AWS API Documentation
*/
default PutBucketLifecycleConfigurationResponse putBucketLifecycleConfiguration(
PutBucketLifecycleConfigurationRequest putBucketLifecycleConfigurationRequest) throws AwsServiceException,
SdkClientException, S3ControlException {
throw new UnsupportedOperationException();
}
/**
*
*
* This action puts a lifecycle configuration to an Amazon S3 on Outposts bucket. To put a lifecycle configuration
* to an S3 bucket, see PutBucketLifecycleConfiguration in the Amazon Simple Storage Service API.
*
*
*
* Creates a new lifecycle configuration for the S3 on Outposts bucket or replaces an existing lifecycle
* configuration. Outposts buckets only support lifecycle configurations that delete/expire objects after a certain
* period of time and abort incomplete multipart uploads.
*
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request and an S3 on Outposts endpoint hostname prefix
* instead of s3-control
. For an example of the request syntax for Amazon S3 on Outposts that uses the
* S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived using the access point ARN,
* see the Examples section.
*
*
* The following actions are related to PutBucketLifecycleConfiguration
:
*
*
* -
*
*
* -
*
*
*
*
*
* This is a convenience which creates an instance of the {@link PutBucketLifecycleConfigurationRequest.Builder}
* avoiding the need to create one manually via {@link PutBucketLifecycleConfigurationRequest#builder()}
*
*
* @param putBucketLifecycleConfigurationRequest
* A {@link Consumer} that will call methods on {@link PutBucketLifecycleConfigurationRequest.Builder} to
* create a request.
* @return Result of the PutBucketLifecycleConfiguration operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.PutBucketLifecycleConfiguration
* @see AWS API Documentation
*/
default PutBucketLifecycleConfigurationResponse putBucketLifecycleConfiguration(
Consumer putBucketLifecycleConfigurationRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
return putBucketLifecycleConfiguration(PutBucketLifecycleConfigurationRequest.builder()
.applyMutation(putBucketLifecycleConfigurationRequest).build());
}
/**
*
*
* This action puts a bucket policy to an Amazon S3 on Outposts bucket. To put a policy on an S3 bucket, see PutBucketPolicy in the
* Amazon Simple Storage Service API.
*
*
*
* Applies an Amazon S3 bucket policy to an Outposts bucket. For more information, see Using Amazon S3 on Outposts in
* the Amazon Simple Storage Service User Guide.
*
*
* If you are using an identity other than the root user of the AWS account that owns the Outposts bucket, the
* calling identity must have the PutBucketPolicy
permissions on the specified Outposts bucket and
* belong to the bucket owner's account in order to use this action.
*
*
* If you don't have PutBucketPolicy
permissions, Amazon S3 returns a 403 Access Denied
* error. If you have the correct permissions, but you're not using an identity that belongs to the bucket owner's
* account, Amazon S3 returns a 405 Method Not Allowed
error.
*
*
*
* As a security precaution, the root user of the AWS account that owns a bucket can always use this action, even if
* the policy explicitly denies the root user the ability to perform this action.
*
*
*
* For more information about bucket policies, see Using Bucket Policies and User
* Policies.
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request and an S3 on Outposts endpoint hostname prefix
* instead of s3-control
. For an example of the request syntax for Amazon S3 on Outposts that uses the
* S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived using the access point ARN,
* see the Examples section.
*
*
* The following actions are related to PutBucketPolicy
:
*
*
* -
*
* GetBucketPolicy
*
*
* -
*
*
*
*
* @param putBucketPolicyRequest
* @return Result of the PutBucketPolicy operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.PutBucketPolicy
* @see AWS API
* Documentation
*/
default PutBucketPolicyResponse putBucketPolicy(PutBucketPolicyRequest putBucketPolicyRequest) throws AwsServiceException,
SdkClientException, S3ControlException {
throw new UnsupportedOperationException();
}
/**
*
*
* This action puts a bucket policy to an Amazon S3 on Outposts bucket. To put a policy on an S3 bucket, see PutBucketPolicy in the
* Amazon Simple Storage Service API.
*
*
*
* Applies an Amazon S3 bucket policy to an Outposts bucket. For more information, see Using Amazon S3 on Outposts in
* the Amazon Simple Storage Service User Guide.
*
*
* If you are using an identity other than the root user of the AWS account that owns the Outposts bucket, the
* calling identity must have the PutBucketPolicy
permissions on the specified Outposts bucket and
* belong to the bucket owner's account in order to use this action.
*
*
* If you don't have PutBucketPolicy
permissions, Amazon S3 returns a 403 Access Denied
* error. If you have the correct permissions, but you're not using an identity that belongs to the bucket owner's
* account, Amazon S3 returns a 405 Method Not Allowed
error.
*
*
*
* As a security precaution, the root user of the AWS account that owns a bucket can always use this action, even if
* the policy explicitly denies the root user the ability to perform this action.
*
*
*
* For more information about bucket policies, see Using Bucket Policies and User
* Policies.
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request and an S3 on Outposts endpoint hostname prefix
* instead of s3-control
. For an example of the request syntax for Amazon S3 on Outposts that uses the
* S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived using the access point ARN,
* see the Examples section.
*
*
* The following actions are related to PutBucketPolicy
:
*
*
* -
*
* GetBucketPolicy
*
*
* -
*
*
*
*
*
* This is a convenience which creates an instance of the {@link PutBucketPolicyRequest.Builder} avoiding the need
* to create one manually via {@link PutBucketPolicyRequest#builder()}
*
*
* @param putBucketPolicyRequest
* A {@link Consumer} that will call methods on {@link PutBucketPolicyRequest.Builder} to create a request.
* @return Result of the PutBucketPolicy operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.PutBucketPolicy
* @see AWS API
* Documentation
*/
default PutBucketPolicyResponse putBucketPolicy(Consumer putBucketPolicyRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
return putBucketPolicy(PutBucketPolicyRequest.builder().applyMutation(putBucketPolicyRequest).build());
}
/**
*
*
* This action puts tags on an Amazon S3 on Outposts bucket. To put tags on an S3 bucket, see PutBucketTagging in the
* Amazon Simple Storage Service API.
*
*
*
* Sets the tags for an S3 on Outposts bucket. For more information, see Using Amazon S3 on Outposts in
* the Amazon Simple Storage Service User Guide.
*
*
* Use tags to organize your AWS bill to reflect your own cost structure. To do this, sign up to get your AWS
* account bill with tag key values included. Then, to see the cost of combined resources, organize your billing
* information according to resources with the same tag key values. For example, you can tag several resources with
* a specific application name, and then organize your billing information to see the total cost of that application
* across several services. For more information, see Cost allocation and
* tagging.
*
*
*
* Within a bucket, if you add a tag that has the same key as an existing tag, the new value overwrites the old
* value. For more information, see Using cost allocation in
* Amazon S3 bucket tags.
*
*
*
* To use this action, you must have permissions to perform the s3-outposts:PutBucketTagging
action.
* The Outposts bucket owner has this permission by default and can grant this permission to others. For more
* information about permissions, see Permissions Related to Bucket Subresource Operations and Managing access permissions
* to your Amazon S3 resources.
*
*
* PutBucketTagging
has the following special errors:
*
*
* -
*
* Error code: InvalidTagError
*
*
* -
*
* Description: The tag provided was not a valid tag. This error can occur if the tag did not pass input validation.
* For information about tag restrictions, see
* User-Defined Tag Restrictions and AWS-Generated Cost
* Allocation Tag Restrictions.
*
*
*
*
* -
*
* Error code: MalformedXMLError
*
*
* -
*
* Description: The XML provided does not match the schema.
*
*
*
*
* -
*
* Error code: OperationAbortedError
*
*
* -
*
* Description: A conflicting conditional action is currently in progress against this resource. Try again.
*
*
*
*
* -
*
* Error code: InternalError
*
*
* -
*
* Description: The service was unable to apply the provided tag to the bucket.
*
*
*
*
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request and an S3 on Outposts endpoint hostname prefix
* instead of s3-control
. For an example of the request syntax for Amazon S3 on Outposts that uses the
* S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived using the access point ARN,
* see the Examples section.
*
*
* The following actions are related to PutBucketTagging
:
*
*
* -
*
* GetBucketTagging
*
*
* -
*
*
*
*
* @param putBucketTaggingRequest
* @return Result of the PutBucketTagging operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.PutBucketTagging
* @see AWS
* API Documentation
*/
default PutBucketTaggingResponse putBucketTagging(PutBucketTaggingRequest putBucketTaggingRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
throw new UnsupportedOperationException();
}
/**
*
*
* This action puts tags on an Amazon S3 on Outposts bucket. To put tags on an S3 bucket, see PutBucketTagging in the
* Amazon Simple Storage Service API.
*
*
*
* Sets the tags for an S3 on Outposts bucket. For more information, see Using Amazon S3 on Outposts in
* the Amazon Simple Storage Service User Guide.
*
*
* Use tags to organize your AWS bill to reflect your own cost structure. To do this, sign up to get your AWS
* account bill with tag key values included. Then, to see the cost of combined resources, organize your billing
* information according to resources with the same tag key values. For example, you can tag several resources with
* a specific application name, and then organize your billing information to see the total cost of that application
* across several services. For more information, see Cost allocation and
* tagging.
*
*
*
* Within a bucket, if you add a tag that has the same key as an existing tag, the new value overwrites the old
* value. For more information, see Using cost allocation in
* Amazon S3 bucket tags.
*
*
*
* To use this action, you must have permissions to perform the s3-outposts:PutBucketTagging
action.
* The Outposts bucket owner has this permission by default and can grant this permission to others. For more
* information about permissions, see Permissions Related to Bucket Subresource Operations and Managing access permissions
* to your Amazon S3 resources.
*
*
* PutBucketTagging
has the following special errors:
*
*
* -
*
* Error code: InvalidTagError
*
*
* -
*
* Description: The tag provided was not a valid tag. This error can occur if the tag did not pass input validation.
* For information about tag restrictions, see
* User-Defined Tag Restrictions and AWS-Generated Cost
* Allocation Tag Restrictions.
*
*
*
*
* -
*
* Error code: MalformedXMLError
*
*
* -
*
* Description: The XML provided does not match the schema.
*
*
*
*
* -
*
* Error code: OperationAbortedError
*
*
* -
*
* Description: A conflicting conditional action is currently in progress against this resource. Try again.
*
*
*
*
* -
*
* Error code: InternalError
*
*
* -
*
* Description: The service was unable to apply the provided tag to the bucket.
*
*
*
*
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request and an S3 on Outposts endpoint hostname prefix
* instead of s3-control
. For an example of the request syntax for Amazon S3 on Outposts that uses the
* S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived using the access point ARN,
* see the Examples section.
*
*
* The following actions are related to PutBucketTagging
:
*
*
* -
*
* GetBucketTagging
*
*
* -
*
*
*
*
*
* This is a convenience which creates an instance of the {@link PutBucketTaggingRequest.Builder} avoiding the need
* to create one manually via {@link PutBucketTaggingRequest#builder()}
*
*
* @param putBucketTaggingRequest
* A {@link Consumer} that will call methods on {@link PutBucketTaggingRequest.Builder} to create a request.
* @return Result of the PutBucketTagging operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.PutBucketTagging
* @see AWS
* API Documentation
*/
default PutBucketTaggingResponse putBucketTagging(Consumer putBucketTaggingRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
return putBucketTagging(PutBucketTaggingRequest.builder().applyMutation(putBucketTaggingRequest).build());
}
/**
*
* Sets the supplied tag-set on an S3 Batch Operations job.
*
*
* A tag is a key-value pair. You can associate S3 Batch Operations tags with any job by sending a PUT request
* against the tagging subresource that is associated with the job. To modify the existing tag set, you can either
* replace the existing tag set entirely, or make changes within the existing tag set by retrieving the existing tag
* set using GetJobTagging, modify
* that tag set, and use this action to replace the tag set with the one you modified. For more information, see Controlling
* access and labeling jobs using tags in the Amazon Simple Storage Service User Guide.
*
*
*
*
* -
*
* If you send this request with an empty tag set, Amazon S3 deletes the existing tag set on the Batch Operations
* job. If you use this method, you are charged for a Tier 1 Request (PUT). For more information, see Amazon S3 pricing.
*
*
* -
*
* For deleting existing tags for your Batch Operations job, a DeleteJobTagging
* request is preferred because it achieves the same result without incurring charges.
*
*
* -
*
* A few things to consider about using tags:
*
*
* -
*
* Amazon S3 limits the maximum number of tags to 50 tags per job.
*
*
* -
*
* You can associate up to 50 tags with a job as long as they have unique tag keys.
*
*
* -
*
* A tag key can be up to 128 Unicode characters in length, and tag values can be up to 256 Unicode characters in
* length.
*
*
* -
*
* The key and values are case sensitive.
*
*
* -
*
* For tagging-related restrictions related to characters and encodings, see User-Defined
* Tag Restrictions in the AWS Billing and Cost Management User Guide.
*
*
*
*
*
*
*
*
* To use this action, you must have permission to perform the s3:PutJobTagging
action.
*
*
* Related actions include:
*
*
* -
*
* CreatJob
*
*
* -
*
* GetJobTagging
*
*
* -
*
* DeleteJobTagging
*
*
*
*
* @param putJobTaggingRequest
* @return Result of the PutJobTagging operation returned by the service.
* @throws InternalServiceException
* @throws TooManyRequestsException
* @throws NotFoundException
* @throws TooManyTagsException
* Amazon S3 throws this exception if you have too many tags in your tag set.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.PutJobTagging
* @see AWS API
* Documentation
*/
default PutJobTaggingResponse putJobTagging(PutJobTaggingRequest putJobTaggingRequest) throws InternalServiceException,
TooManyRequestsException, NotFoundException, TooManyTagsException, AwsServiceException, SdkClientException,
S3ControlException {
throw new UnsupportedOperationException();
}
/**
*
* Sets the supplied tag-set on an S3 Batch Operations job.
*
*
* A tag is a key-value pair. You can associate S3 Batch Operations tags with any job by sending a PUT request
* against the tagging subresource that is associated with the job. To modify the existing tag set, you can either
* replace the existing tag set entirely, or make changes within the existing tag set by retrieving the existing tag
* set using GetJobTagging, modify
* that tag set, and use this action to replace the tag set with the one you modified. For more information, see Controlling
* access and labeling jobs using tags in the Amazon Simple Storage Service User Guide.
*
*
*
*
* -
*
* If you send this request with an empty tag set, Amazon S3 deletes the existing tag set on the Batch Operations
* job. If you use this method, you are charged for a Tier 1 Request (PUT). For more information, see Amazon S3 pricing.
*
*
* -
*
* For deleting existing tags for your Batch Operations job, a DeleteJobTagging
* request is preferred because it achieves the same result without incurring charges.
*
*
* -
*
* A few things to consider about using tags:
*
*
* -
*
* Amazon S3 limits the maximum number of tags to 50 tags per job.
*
*
* -
*
* You can associate up to 50 tags with a job as long as they have unique tag keys.
*
*
* -
*
* A tag key can be up to 128 Unicode characters in length, and tag values can be up to 256 Unicode characters in
* length.
*
*
* -
*
* The key and values are case sensitive.
*
*
* -
*
* For tagging-related restrictions related to characters and encodings, see User-Defined
* Tag Restrictions in the AWS Billing and Cost Management User Guide.
*
*
*
*
*
*
*
*
* To use this action, you must have permission to perform the s3:PutJobTagging
action.
*
*
* Related actions include:
*
*
* -
*
* CreatJob
*
*
* -
*
* GetJobTagging
*
*
* -
*
* DeleteJobTagging
*
*
*
*
*
* This is a convenience which creates an instance of the {@link PutJobTaggingRequest.Builder} avoiding the need to
* create one manually via {@link PutJobTaggingRequest#builder()}
*
*
* @param putJobTaggingRequest
* A {@link Consumer} that will call methods on {@link PutJobTaggingRequest.Builder} to create a request.
* @return Result of the PutJobTagging operation returned by the service.
* @throws InternalServiceException
* @throws TooManyRequestsException
* @throws NotFoundException
* @throws TooManyTagsException
* Amazon S3 throws this exception if you have too many tags in your tag set.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.PutJobTagging
* @see AWS API
* Documentation
*/
default PutJobTaggingResponse putJobTagging(Consumer putJobTaggingRequest)
throws InternalServiceException, TooManyRequestsException, NotFoundException, TooManyTagsException,
AwsServiceException, SdkClientException, S3ControlException {
return putJobTagging(PutJobTaggingRequest.builder().applyMutation(putJobTaggingRequest).build());
}
/**
*
* Creates or modifies the PublicAccessBlock
configuration for an AWS account. For more information,
* see Using
* Amazon S3 block public access.
*
*
* Related actions include:
*
*
* -
*
*
* -
*
*
*
*
* @param putPublicAccessBlockRequest
* @return Result of the PutPublicAccessBlock operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.PutPublicAccessBlock
* @see AWS API Documentation
*/
default PutPublicAccessBlockResponse putPublicAccessBlock(PutPublicAccessBlockRequest putPublicAccessBlockRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
throw new UnsupportedOperationException();
}
/**
*
* Creates or modifies the PublicAccessBlock
configuration for an AWS account. For more information,
* see Using
* Amazon S3 block public access.
*
*
* Related actions include:
*
*
* -
*
*
* -
*
*
*
*
*
* This is a convenience which creates an instance of the {@link PutPublicAccessBlockRequest.Builder} avoiding the
* need to create one manually via {@link PutPublicAccessBlockRequest#builder()}
*
*
* @param putPublicAccessBlockRequest
* A {@link Consumer} that will call methods on {@link PutPublicAccessBlockRequest.Builder} to create a
* request.
* @return Result of the PutPublicAccessBlock operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.PutPublicAccessBlock
* @see AWS API Documentation
*/
default PutPublicAccessBlockResponse putPublicAccessBlock(
Consumer putPublicAccessBlockRequest) throws AwsServiceException,
SdkClientException, S3ControlException {
return putPublicAccessBlock(PutPublicAccessBlockRequest.builder().applyMutation(putPublicAccessBlockRequest).build());
}
/**
*
* Puts an Amazon S3 Storage Lens configuration. For more information about S3 Storage Lens, see Working with Amazon S3 Storage Lens
* in the Amazon Simple Storage Service User Guide.
*
*
*
* To use this action, you must have permission to perform the s3:PutStorageLensConfiguration
action.
* For more information, see Setting permissions to
* use Amazon S3 Storage Lens in the Amazon Simple Storage Service User Guide.
*
*
*
* @param putStorageLensConfigurationRequest
* @return Result of the PutStorageLensConfiguration operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.PutStorageLensConfiguration
* @see AWS API Documentation
*/
default PutStorageLensConfigurationResponse putStorageLensConfiguration(
PutStorageLensConfigurationRequest putStorageLensConfigurationRequest) throws AwsServiceException,
SdkClientException, S3ControlException {
throw new UnsupportedOperationException();
}
/**
*
* Puts an Amazon S3 Storage Lens configuration. For more information about S3 Storage Lens, see Working with Amazon S3 Storage Lens
* in the Amazon Simple Storage Service User Guide.
*
*
*
* To use this action, you must have permission to perform the s3:PutStorageLensConfiguration
action.
* For more information, see Setting permissions to
* use Amazon S3 Storage Lens in the Amazon Simple Storage Service User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link PutStorageLensConfigurationRequest.Builder}
* avoiding the need to create one manually via {@link PutStorageLensConfigurationRequest#builder()}
*
*
* @param putStorageLensConfigurationRequest
* A {@link Consumer} that will call methods on {@link PutStorageLensConfigurationRequest.Builder} to create
* a request.
* @return Result of the PutStorageLensConfiguration operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.PutStorageLensConfiguration
* @see AWS API Documentation
*/
default PutStorageLensConfigurationResponse putStorageLensConfiguration(
Consumer putStorageLensConfigurationRequest) throws AwsServiceException,
SdkClientException, S3ControlException {
return putStorageLensConfiguration(PutStorageLensConfigurationRequest.builder()
.applyMutation(putStorageLensConfigurationRequest).build());
}
/**
*
* Put or replace tags on an existing Amazon S3 Storage Lens configuration. For more information about S3 Storage
* Lens, see Assessing your storage
* activity and usage with Amazon S3 Storage Lens in the Amazon Simple Storage Service User Guide.
*
*
*
* To use this action, you must have permission to perform the s3:PutStorageLensConfigurationTagging
* action. For more information, see Setting permissions to
* use Amazon S3 Storage Lens in the Amazon Simple Storage Service User Guide.
*
*
*
* @param putStorageLensConfigurationTaggingRequest
* @return Result of the PutStorageLensConfigurationTagging operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.PutStorageLensConfigurationTagging
* @see AWS API Documentation
*/
default PutStorageLensConfigurationTaggingResponse putStorageLensConfigurationTagging(
PutStorageLensConfigurationTaggingRequest putStorageLensConfigurationTaggingRequest) throws AwsServiceException,
SdkClientException, S3ControlException {
throw new UnsupportedOperationException();
}
/**
*
* Put or replace tags on an existing Amazon S3 Storage Lens configuration. For more information about S3 Storage
* Lens, see Assessing your storage
* activity and usage with Amazon S3 Storage Lens in the Amazon Simple Storage Service User Guide.
*
*
*
* To use this action, you must have permission to perform the s3:PutStorageLensConfigurationTagging
* action. For more information, see Setting permissions to
* use Amazon S3 Storage Lens in the Amazon Simple Storage Service User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link PutStorageLensConfigurationTaggingRequest.Builder}
* avoiding the need to create one manually via {@link PutStorageLensConfigurationTaggingRequest#builder()}
*
*
* @param putStorageLensConfigurationTaggingRequest
* A {@link Consumer} that will call methods on {@link PutStorageLensConfigurationTaggingRequest.Builder} to
* create a request.
* @return Result of the PutStorageLensConfigurationTagging operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.PutStorageLensConfigurationTagging
* @see AWS API Documentation
*/
default PutStorageLensConfigurationTaggingResponse putStorageLensConfigurationTagging(
Consumer putStorageLensConfigurationTaggingRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
return putStorageLensConfigurationTagging(PutStorageLensConfigurationTaggingRequest.builder()
.applyMutation(putStorageLensConfigurationTaggingRequest).build());
}
/**
*
* Updates an existing S3 Batch Operations job's priority. For more information, see S3 Batch Operations in the
* Amazon Simple Storage Service User Guide.
*
*
*
* Related actions include:
*
*
* -
*
* CreateJob
*
*
* -
*
* ListJobs
*
*
* -
*
* DescribeJob
*
*
* -
*
* UpdateJobStatus
*
*
*
*
* @param updateJobPriorityRequest
* @return Result of the UpdateJobPriority operation returned by the service.
* @throws BadRequestException
* @throws TooManyRequestsException
* @throws NotFoundException
* @throws InternalServiceException
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.UpdateJobPriority
* @see AWS
* API Documentation
*/
default UpdateJobPriorityResponse updateJobPriority(UpdateJobPriorityRequest updateJobPriorityRequest)
throws BadRequestException, TooManyRequestsException, NotFoundException, InternalServiceException,
AwsServiceException, SdkClientException, S3ControlException {
throw new UnsupportedOperationException();
}
/**
*
* Updates an existing S3 Batch Operations job's priority. For more information, see S3 Batch Operations in the
* Amazon Simple Storage Service User Guide.
*
*
*
* Related actions include:
*
*
* -
*
* CreateJob
*
*
* -
*
* ListJobs
*
*
* -
*
* DescribeJob
*
*
* -
*
* UpdateJobStatus
*
*
*
*
*
* This is a convenience which creates an instance of the {@link UpdateJobPriorityRequest.Builder} avoiding the need
* to create one manually via {@link UpdateJobPriorityRequest#builder()}
*
*
* @param updateJobPriorityRequest
* A {@link Consumer} that will call methods on {@link UpdateJobPriorityRequest.Builder} to create a request.
* @return Result of the UpdateJobPriority operation returned by the service.
* @throws BadRequestException
* @throws TooManyRequestsException
* @throws NotFoundException
* @throws InternalServiceException
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.UpdateJobPriority
* @see AWS
* API Documentation
*/
default UpdateJobPriorityResponse updateJobPriority(Consumer updateJobPriorityRequest)
throws BadRequestException, TooManyRequestsException, NotFoundException, InternalServiceException,
AwsServiceException, SdkClientException, S3ControlException {
return updateJobPriority(UpdateJobPriorityRequest.builder().applyMutation(updateJobPriorityRequest).build());
}
/**
*
* Updates the status for the specified job. Use this action to confirm that you want to run a job or to cancel an
* existing job. For more information, see S3 Batch Operations in the
* Amazon Simple Storage Service User Guide.
*
*
*
* Related actions include:
*
*
* -
*
* CreateJob
*
*
* -
*
* ListJobs
*
*
* -
*
* DescribeJob
*
*
* -
*
* UpdateJobStatus
*
*
*
*
* @param updateJobStatusRequest
* @return Result of the UpdateJobStatus operation returned by the service.
* @throws BadRequestException
* @throws TooManyRequestsException
* @throws NotFoundException
* @throws JobStatusException
* @throws InternalServiceException
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.UpdateJobStatus
* @see AWS API
* Documentation
*/
default UpdateJobStatusResponse updateJobStatus(UpdateJobStatusRequest updateJobStatusRequest) throws BadRequestException,
TooManyRequestsException, NotFoundException, JobStatusException, InternalServiceException, AwsServiceException,
SdkClientException, S3ControlException {
throw new UnsupportedOperationException();
}
/**
*
* Updates the status for the specified job. Use this action to confirm that you want to run a job or to cancel an
* existing job. For more information, see S3 Batch Operations in the
* Amazon Simple Storage Service User Guide.
*
*
*
* Related actions include:
*
*
* -
*
* CreateJob
*
*
* -
*
* ListJobs
*
*
* -
*
* DescribeJob
*
*
* -
*
* UpdateJobStatus
*
*
*
*
*
* This is a convenience which creates an instance of the {@link UpdateJobStatusRequest.Builder} avoiding the need
* to create one manually via {@link UpdateJobStatusRequest#builder()}
*
*
* @param updateJobStatusRequest
* A {@link Consumer} that will call methods on {@link UpdateJobStatusRequest.Builder} to create a request.
* @return Result of the UpdateJobStatus operation returned by the service.
* @throws BadRequestException
* @throws TooManyRequestsException
* @throws NotFoundException
* @throws JobStatusException
* @throws InternalServiceException
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.UpdateJobStatus
* @see AWS API
* Documentation
*/
default UpdateJobStatusResponse updateJobStatus(Consumer updateJobStatusRequest)
throws BadRequestException, TooManyRequestsException, NotFoundException, JobStatusException,
InternalServiceException, AwsServiceException, SdkClientException, S3ControlException {
return updateJobStatus(UpdateJobStatusRequest.builder().applyMutation(updateJobStatusRequest).build());
}
static ServiceMetadata serviceMetadata() {
return ServiceMetadata.of(SERVICE_METADATA_ID);
}
}