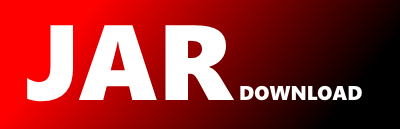
software.amazon.awssdk.services.s3control.model.AccountLevel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of s3control Show documentation
Show all versions of s3control Show documentation
The AWS Java SDK for Amazon S3 Control module holds the client classes that are used for communicating with
Amazon Simple Storage Service Control Plane
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.s3control.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* A container for the account level Amazon S3 Storage Lens configuration.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class AccountLevel implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField ACTIVITY_METRICS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("ActivityMetrics")
.getter(getter(AccountLevel::activityMetrics))
.setter(setter(Builder::activityMetrics))
.constructor(ActivityMetrics::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ActivityMetrics")
.unmarshallLocationName("ActivityMetrics").build()).build();
private static final SdkField BUCKET_LEVEL_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("BucketLevel")
.getter(getter(AccountLevel::bucketLevel))
.setter(setter(Builder::bucketLevel))
.constructor(BucketLevel::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("BucketLevel")
.unmarshallLocationName("BucketLevel").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ACTIVITY_METRICS_FIELD,
BUCKET_LEVEL_FIELD));
private static final long serialVersionUID = 1L;
private final ActivityMetrics activityMetrics;
private final BucketLevel bucketLevel;
private AccountLevel(BuilderImpl builder) {
this.activityMetrics = builder.activityMetrics;
this.bucketLevel = builder.bucketLevel;
}
/**
*
* A container for the S3 Storage Lens activity metrics.
*
*
* @return A container for the S3 Storage Lens activity metrics.
*/
public final ActivityMetrics activityMetrics() {
return activityMetrics;
}
/**
*
* A container for the S3 Storage Lens bucket-level configuration.
*
*
* @return A container for the S3 Storage Lens bucket-level configuration.
*/
public final BucketLevel bucketLevel() {
return bucketLevel;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(activityMetrics());
hashCode = 31 * hashCode + Objects.hashCode(bucketLevel());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof AccountLevel)) {
return false;
}
AccountLevel other = (AccountLevel) obj;
return Objects.equals(activityMetrics(), other.activityMetrics()) && Objects.equals(bucketLevel(), other.bucketLevel());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("AccountLevel").add("ActivityMetrics", activityMetrics()).add("BucketLevel", bucketLevel())
.build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ActivityMetrics":
return Optional.ofNullable(clazz.cast(activityMetrics()));
case "BucketLevel":
return Optional.ofNullable(clazz.cast(bucketLevel()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
© 2015 - 2025 Weber Informatics LLC | Privacy Policy