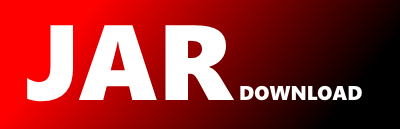
software.amazon.awssdk.services.s3control.DefaultS3ControlClient Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.s3control;
import java.util.Collections;
import java.util.List;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.awscore.client.handler.AwsSyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.ApiName;
import software.amazon.awssdk.core.RequestOverrideConfiguration;
import software.amazon.awssdk.core.Response;
import software.amazon.awssdk.core.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientOption;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.client.handler.SyncClientHandler;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.interceptor.SdkInternalExecutionAttribute;
import software.amazon.awssdk.core.interceptor.trait.HttpChecksumRequired;
import software.amazon.awssdk.core.metrics.CoreMetric;
import software.amazon.awssdk.core.util.VersionInfo;
import software.amazon.awssdk.metrics.MetricCollector;
import software.amazon.awssdk.metrics.MetricPublisher;
import software.amazon.awssdk.metrics.NoOpMetricCollector;
import software.amazon.awssdk.protocols.core.ExceptionMetadata;
import software.amazon.awssdk.protocols.xml.AwsXmlProtocolFactory;
import software.amazon.awssdk.protocols.xml.XmlOperationMetadata;
import software.amazon.awssdk.services.s3control.model.BadRequestException;
import software.amazon.awssdk.services.s3control.model.BucketAlreadyExistsException;
import software.amazon.awssdk.services.s3control.model.BucketAlreadyOwnedByYouException;
import software.amazon.awssdk.services.s3control.model.CreateAccessPointForObjectLambdaRequest;
import software.amazon.awssdk.services.s3control.model.CreateAccessPointForObjectLambdaResponse;
import software.amazon.awssdk.services.s3control.model.CreateAccessPointRequest;
import software.amazon.awssdk.services.s3control.model.CreateAccessPointResponse;
import software.amazon.awssdk.services.s3control.model.CreateBucketRequest;
import software.amazon.awssdk.services.s3control.model.CreateBucketResponse;
import software.amazon.awssdk.services.s3control.model.CreateJobRequest;
import software.amazon.awssdk.services.s3control.model.CreateJobResponse;
import software.amazon.awssdk.services.s3control.model.CreateMultiRegionAccessPointRequest;
import software.amazon.awssdk.services.s3control.model.CreateMultiRegionAccessPointResponse;
import software.amazon.awssdk.services.s3control.model.DeleteAccessPointForObjectLambdaRequest;
import software.amazon.awssdk.services.s3control.model.DeleteAccessPointForObjectLambdaResponse;
import software.amazon.awssdk.services.s3control.model.DeleteAccessPointPolicyForObjectLambdaRequest;
import software.amazon.awssdk.services.s3control.model.DeleteAccessPointPolicyForObjectLambdaResponse;
import software.amazon.awssdk.services.s3control.model.DeleteAccessPointPolicyRequest;
import software.amazon.awssdk.services.s3control.model.DeleteAccessPointPolicyResponse;
import software.amazon.awssdk.services.s3control.model.DeleteAccessPointRequest;
import software.amazon.awssdk.services.s3control.model.DeleteAccessPointResponse;
import software.amazon.awssdk.services.s3control.model.DeleteBucketLifecycleConfigurationRequest;
import software.amazon.awssdk.services.s3control.model.DeleteBucketLifecycleConfigurationResponse;
import software.amazon.awssdk.services.s3control.model.DeleteBucketPolicyRequest;
import software.amazon.awssdk.services.s3control.model.DeleteBucketPolicyResponse;
import software.amazon.awssdk.services.s3control.model.DeleteBucketRequest;
import software.amazon.awssdk.services.s3control.model.DeleteBucketResponse;
import software.amazon.awssdk.services.s3control.model.DeleteBucketTaggingRequest;
import software.amazon.awssdk.services.s3control.model.DeleteBucketTaggingResponse;
import software.amazon.awssdk.services.s3control.model.DeleteJobTaggingRequest;
import software.amazon.awssdk.services.s3control.model.DeleteJobTaggingResponse;
import software.amazon.awssdk.services.s3control.model.DeleteMultiRegionAccessPointRequest;
import software.amazon.awssdk.services.s3control.model.DeleteMultiRegionAccessPointResponse;
import software.amazon.awssdk.services.s3control.model.DeletePublicAccessBlockRequest;
import software.amazon.awssdk.services.s3control.model.DeletePublicAccessBlockResponse;
import software.amazon.awssdk.services.s3control.model.DeleteStorageLensConfigurationRequest;
import software.amazon.awssdk.services.s3control.model.DeleteStorageLensConfigurationResponse;
import software.amazon.awssdk.services.s3control.model.DeleteStorageLensConfigurationTaggingRequest;
import software.amazon.awssdk.services.s3control.model.DeleteStorageLensConfigurationTaggingResponse;
import software.amazon.awssdk.services.s3control.model.DescribeJobRequest;
import software.amazon.awssdk.services.s3control.model.DescribeJobResponse;
import software.amazon.awssdk.services.s3control.model.DescribeMultiRegionAccessPointOperationRequest;
import software.amazon.awssdk.services.s3control.model.DescribeMultiRegionAccessPointOperationResponse;
import software.amazon.awssdk.services.s3control.model.GetAccessPointConfigurationForObjectLambdaRequest;
import software.amazon.awssdk.services.s3control.model.GetAccessPointConfigurationForObjectLambdaResponse;
import software.amazon.awssdk.services.s3control.model.GetAccessPointForObjectLambdaRequest;
import software.amazon.awssdk.services.s3control.model.GetAccessPointForObjectLambdaResponse;
import software.amazon.awssdk.services.s3control.model.GetAccessPointPolicyForObjectLambdaRequest;
import software.amazon.awssdk.services.s3control.model.GetAccessPointPolicyForObjectLambdaResponse;
import software.amazon.awssdk.services.s3control.model.GetAccessPointPolicyRequest;
import software.amazon.awssdk.services.s3control.model.GetAccessPointPolicyResponse;
import software.amazon.awssdk.services.s3control.model.GetAccessPointPolicyStatusForObjectLambdaRequest;
import software.amazon.awssdk.services.s3control.model.GetAccessPointPolicyStatusForObjectLambdaResponse;
import software.amazon.awssdk.services.s3control.model.GetAccessPointPolicyStatusRequest;
import software.amazon.awssdk.services.s3control.model.GetAccessPointPolicyStatusResponse;
import software.amazon.awssdk.services.s3control.model.GetAccessPointRequest;
import software.amazon.awssdk.services.s3control.model.GetAccessPointResponse;
import software.amazon.awssdk.services.s3control.model.GetBucketLifecycleConfigurationRequest;
import software.amazon.awssdk.services.s3control.model.GetBucketLifecycleConfigurationResponse;
import software.amazon.awssdk.services.s3control.model.GetBucketPolicyRequest;
import software.amazon.awssdk.services.s3control.model.GetBucketPolicyResponse;
import software.amazon.awssdk.services.s3control.model.GetBucketRequest;
import software.amazon.awssdk.services.s3control.model.GetBucketResponse;
import software.amazon.awssdk.services.s3control.model.GetBucketTaggingRequest;
import software.amazon.awssdk.services.s3control.model.GetBucketTaggingResponse;
import software.amazon.awssdk.services.s3control.model.GetBucketVersioningRequest;
import software.amazon.awssdk.services.s3control.model.GetBucketVersioningResponse;
import software.amazon.awssdk.services.s3control.model.GetJobTaggingRequest;
import software.amazon.awssdk.services.s3control.model.GetJobTaggingResponse;
import software.amazon.awssdk.services.s3control.model.GetMultiRegionAccessPointPolicyRequest;
import software.amazon.awssdk.services.s3control.model.GetMultiRegionAccessPointPolicyResponse;
import software.amazon.awssdk.services.s3control.model.GetMultiRegionAccessPointPolicyStatusRequest;
import software.amazon.awssdk.services.s3control.model.GetMultiRegionAccessPointPolicyStatusResponse;
import software.amazon.awssdk.services.s3control.model.GetMultiRegionAccessPointRequest;
import software.amazon.awssdk.services.s3control.model.GetMultiRegionAccessPointResponse;
import software.amazon.awssdk.services.s3control.model.GetMultiRegionAccessPointRoutesRequest;
import software.amazon.awssdk.services.s3control.model.GetMultiRegionAccessPointRoutesResponse;
import software.amazon.awssdk.services.s3control.model.GetPublicAccessBlockRequest;
import software.amazon.awssdk.services.s3control.model.GetPublicAccessBlockResponse;
import software.amazon.awssdk.services.s3control.model.GetStorageLensConfigurationRequest;
import software.amazon.awssdk.services.s3control.model.GetStorageLensConfigurationResponse;
import software.amazon.awssdk.services.s3control.model.GetStorageLensConfigurationTaggingRequest;
import software.amazon.awssdk.services.s3control.model.GetStorageLensConfigurationTaggingResponse;
import software.amazon.awssdk.services.s3control.model.IdempotencyException;
import software.amazon.awssdk.services.s3control.model.InternalServiceException;
import software.amazon.awssdk.services.s3control.model.InvalidNextTokenException;
import software.amazon.awssdk.services.s3control.model.InvalidRequestException;
import software.amazon.awssdk.services.s3control.model.JobStatusException;
import software.amazon.awssdk.services.s3control.model.ListAccessPointsForObjectLambdaRequest;
import software.amazon.awssdk.services.s3control.model.ListAccessPointsForObjectLambdaResponse;
import software.amazon.awssdk.services.s3control.model.ListAccessPointsRequest;
import software.amazon.awssdk.services.s3control.model.ListAccessPointsResponse;
import software.amazon.awssdk.services.s3control.model.ListJobsRequest;
import software.amazon.awssdk.services.s3control.model.ListJobsResponse;
import software.amazon.awssdk.services.s3control.model.ListMultiRegionAccessPointsRequest;
import software.amazon.awssdk.services.s3control.model.ListMultiRegionAccessPointsResponse;
import software.amazon.awssdk.services.s3control.model.ListRegionalBucketsRequest;
import software.amazon.awssdk.services.s3control.model.ListRegionalBucketsResponse;
import software.amazon.awssdk.services.s3control.model.ListStorageLensConfigurationsRequest;
import software.amazon.awssdk.services.s3control.model.ListStorageLensConfigurationsResponse;
import software.amazon.awssdk.services.s3control.model.NoSuchPublicAccessBlockConfigurationException;
import software.amazon.awssdk.services.s3control.model.NotFoundException;
import software.amazon.awssdk.services.s3control.model.PutAccessPointConfigurationForObjectLambdaRequest;
import software.amazon.awssdk.services.s3control.model.PutAccessPointConfigurationForObjectLambdaResponse;
import software.amazon.awssdk.services.s3control.model.PutAccessPointPolicyForObjectLambdaRequest;
import software.amazon.awssdk.services.s3control.model.PutAccessPointPolicyForObjectLambdaResponse;
import software.amazon.awssdk.services.s3control.model.PutAccessPointPolicyRequest;
import software.amazon.awssdk.services.s3control.model.PutAccessPointPolicyResponse;
import software.amazon.awssdk.services.s3control.model.PutBucketLifecycleConfigurationRequest;
import software.amazon.awssdk.services.s3control.model.PutBucketLifecycleConfigurationResponse;
import software.amazon.awssdk.services.s3control.model.PutBucketPolicyRequest;
import software.amazon.awssdk.services.s3control.model.PutBucketPolicyResponse;
import software.amazon.awssdk.services.s3control.model.PutBucketTaggingRequest;
import software.amazon.awssdk.services.s3control.model.PutBucketTaggingResponse;
import software.amazon.awssdk.services.s3control.model.PutBucketVersioningRequest;
import software.amazon.awssdk.services.s3control.model.PutBucketVersioningResponse;
import software.amazon.awssdk.services.s3control.model.PutJobTaggingRequest;
import software.amazon.awssdk.services.s3control.model.PutJobTaggingResponse;
import software.amazon.awssdk.services.s3control.model.PutMultiRegionAccessPointPolicyRequest;
import software.amazon.awssdk.services.s3control.model.PutMultiRegionAccessPointPolicyResponse;
import software.amazon.awssdk.services.s3control.model.PutPublicAccessBlockRequest;
import software.amazon.awssdk.services.s3control.model.PutPublicAccessBlockResponse;
import software.amazon.awssdk.services.s3control.model.PutStorageLensConfigurationRequest;
import software.amazon.awssdk.services.s3control.model.PutStorageLensConfigurationResponse;
import software.amazon.awssdk.services.s3control.model.PutStorageLensConfigurationTaggingRequest;
import software.amazon.awssdk.services.s3control.model.PutStorageLensConfigurationTaggingResponse;
import software.amazon.awssdk.services.s3control.model.S3ControlException;
import software.amazon.awssdk.services.s3control.model.S3ControlRequest;
import software.amazon.awssdk.services.s3control.model.SubmitMultiRegionAccessPointRoutesRequest;
import software.amazon.awssdk.services.s3control.model.SubmitMultiRegionAccessPointRoutesResponse;
import software.amazon.awssdk.services.s3control.model.TooManyRequestsException;
import software.amazon.awssdk.services.s3control.model.TooManyTagsException;
import software.amazon.awssdk.services.s3control.model.UpdateJobPriorityRequest;
import software.amazon.awssdk.services.s3control.model.UpdateJobPriorityResponse;
import software.amazon.awssdk.services.s3control.model.UpdateJobStatusRequest;
import software.amazon.awssdk.services.s3control.model.UpdateJobStatusResponse;
import software.amazon.awssdk.services.s3control.paginators.ListAccessPointsForObjectLambdaIterable;
import software.amazon.awssdk.services.s3control.paginators.ListAccessPointsIterable;
import software.amazon.awssdk.services.s3control.paginators.ListJobsIterable;
import software.amazon.awssdk.services.s3control.paginators.ListMultiRegionAccessPointsIterable;
import software.amazon.awssdk.services.s3control.paginators.ListRegionalBucketsIterable;
import software.amazon.awssdk.services.s3control.paginators.ListStorageLensConfigurationsIterable;
import software.amazon.awssdk.services.s3control.transform.CreateAccessPointForObjectLambdaRequestMarshaller;
import software.amazon.awssdk.services.s3control.transform.CreateAccessPointRequestMarshaller;
import software.amazon.awssdk.services.s3control.transform.CreateBucketRequestMarshaller;
import software.amazon.awssdk.services.s3control.transform.CreateJobRequestMarshaller;
import software.amazon.awssdk.services.s3control.transform.CreateMultiRegionAccessPointRequestMarshaller;
import software.amazon.awssdk.services.s3control.transform.DeleteAccessPointForObjectLambdaRequestMarshaller;
import software.amazon.awssdk.services.s3control.transform.DeleteAccessPointPolicyForObjectLambdaRequestMarshaller;
import software.amazon.awssdk.services.s3control.transform.DeleteAccessPointPolicyRequestMarshaller;
import software.amazon.awssdk.services.s3control.transform.DeleteAccessPointRequestMarshaller;
import software.amazon.awssdk.services.s3control.transform.DeleteBucketLifecycleConfigurationRequestMarshaller;
import software.amazon.awssdk.services.s3control.transform.DeleteBucketPolicyRequestMarshaller;
import software.amazon.awssdk.services.s3control.transform.DeleteBucketRequestMarshaller;
import software.amazon.awssdk.services.s3control.transform.DeleteBucketTaggingRequestMarshaller;
import software.amazon.awssdk.services.s3control.transform.DeleteJobTaggingRequestMarshaller;
import software.amazon.awssdk.services.s3control.transform.DeleteMultiRegionAccessPointRequestMarshaller;
import software.amazon.awssdk.services.s3control.transform.DeletePublicAccessBlockRequestMarshaller;
import software.amazon.awssdk.services.s3control.transform.DeleteStorageLensConfigurationRequestMarshaller;
import software.amazon.awssdk.services.s3control.transform.DeleteStorageLensConfigurationTaggingRequestMarshaller;
import software.amazon.awssdk.services.s3control.transform.DescribeJobRequestMarshaller;
import software.amazon.awssdk.services.s3control.transform.DescribeMultiRegionAccessPointOperationRequestMarshaller;
import software.amazon.awssdk.services.s3control.transform.GetAccessPointConfigurationForObjectLambdaRequestMarshaller;
import software.amazon.awssdk.services.s3control.transform.GetAccessPointForObjectLambdaRequestMarshaller;
import software.amazon.awssdk.services.s3control.transform.GetAccessPointPolicyForObjectLambdaRequestMarshaller;
import software.amazon.awssdk.services.s3control.transform.GetAccessPointPolicyRequestMarshaller;
import software.amazon.awssdk.services.s3control.transform.GetAccessPointPolicyStatusForObjectLambdaRequestMarshaller;
import software.amazon.awssdk.services.s3control.transform.GetAccessPointPolicyStatusRequestMarshaller;
import software.amazon.awssdk.services.s3control.transform.GetAccessPointRequestMarshaller;
import software.amazon.awssdk.services.s3control.transform.GetBucketLifecycleConfigurationRequestMarshaller;
import software.amazon.awssdk.services.s3control.transform.GetBucketPolicyRequestMarshaller;
import software.amazon.awssdk.services.s3control.transform.GetBucketRequestMarshaller;
import software.amazon.awssdk.services.s3control.transform.GetBucketTaggingRequestMarshaller;
import software.amazon.awssdk.services.s3control.transform.GetBucketVersioningRequestMarshaller;
import software.amazon.awssdk.services.s3control.transform.GetJobTaggingRequestMarshaller;
import software.amazon.awssdk.services.s3control.transform.GetMultiRegionAccessPointPolicyRequestMarshaller;
import software.amazon.awssdk.services.s3control.transform.GetMultiRegionAccessPointPolicyStatusRequestMarshaller;
import software.amazon.awssdk.services.s3control.transform.GetMultiRegionAccessPointRequestMarshaller;
import software.amazon.awssdk.services.s3control.transform.GetMultiRegionAccessPointRoutesRequestMarshaller;
import software.amazon.awssdk.services.s3control.transform.GetPublicAccessBlockRequestMarshaller;
import software.amazon.awssdk.services.s3control.transform.GetStorageLensConfigurationRequestMarshaller;
import software.amazon.awssdk.services.s3control.transform.GetStorageLensConfigurationTaggingRequestMarshaller;
import software.amazon.awssdk.services.s3control.transform.ListAccessPointsForObjectLambdaRequestMarshaller;
import software.amazon.awssdk.services.s3control.transform.ListAccessPointsRequestMarshaller;
import software.amazon.awssdk.services.s3control.transform.ListJobsRequestMarshaller;
import software.amazon.awssdk.services.s3control.transform.ListMultiRegionAccessPointsRequestMarshaller;
import software.amazon.awssdk.services.s3control.transform.ListRegionalBucketsRequestMarshaller;
import software.amazon.awssdk.services.s3control.transform.ListStorageLensConfigurationsRequestMarshaller;
import software.amazon.awssdk.services.s3control.transform.PutAccessPointConfigurationForObjectLambdaRequestMarshaller;
import software.amazon.awssdk.services.s3control.transform.PutAccessPointPolicyForObjectLambdaRequestMarshaller;
import software.amazon.awssdk.services.s3control.transform.PutAccessPointPolicyRequestMarshaller;
import software.amazon.awssdk.services.s3control.transform.PutBucketLifecycleConfigurationRequestMarshaller;
import software.amazon.awssdk.services.s3control.transform.PutBucketPolicyRequestMarshaller;
import software.amazon.awssdk.services.s3control.transform.PutBucketTaggingRequestMarshaller;
import software.amazon.awssdk.services.s3control.transform.PutBucketVersioningRequestMarshaller;
import software.amazon.awssdk.services.s3control.transform.PutJobTaggingRequestMarshaller;
import software.amazon.awssdk.services.s3control.transform.PutMultiRegionAccessPointPolicyRequestMarshaller;
import software.amazon.awssdk.services.s3control.transform.PutPublicAccessBlockRequestMarshaller;
import software.amazon.awssdk.services.s3control.transform.PutStorageLensConfigurationRequestMarshaller;
import software.amazon.awssdk.services.s3control.transform.PutStorageLensConfigurationTaggingRequestMarshaller;
import software.amazon.awssdk.services.s3control.transform.SubmitMultiRegionAccessPointRoutesRequestMarshaller;
import software.amazon.awssdk.services.s3control.transform.UpdateJobPriorityRequestMarshaller;
import software.amazon.awssdk.services.s3control.transform.UpdateJobStatusRequestMarshaller;
import software.amazon.awssdk.utils.Logger;
/**
* Internal implementation of {@link S3ControlClient}.
*
* @see S3ControlClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultS3ControlClient implements S3ControlClient {
private static final Logger log = Logger.loggerFor(DefaultS3ControlClient.class);
private final SyncClientHandler clientHandler;
private final AwsXmlProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
protected DefaultS3ControlClient(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsSyncClientHandler(clientConfiguration);
this.clientConfiguration = clientConfiguration;
this.protocolFactory = init();
}
/**
*
* Creates an access point and associates it with the specified bucket. For more information, see Managing Data Access with Amazon
* S3 Access Points in the Amazon S3 User Guide.
*
*
*
*
* S3 on Outposts only supports VPC-style access points.
*
*
* For more information, see
* Accessing Amazon S3 on Outposts using virtual private cloud (VPC) only access points in the Amazon S3 User
* Guide.
*
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request. In addition, you must use an S3 on Outposts endpoint
* hostname prefix instead of s3-control
. For an example of the request syntax for Amazon S3 on
* Outposts that uses the S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived by
* using the access point ARN, see the Examples section.
*
*
*
* The following actions are related to CreateAccessPoint
:
*
*
* -
*
* GetAccessPoint
*
*
* -
*
*
* -
*
* ListAccessPoints
*
*
*
*
* @param createAccessPointRequest
* @return Result of the CreateAccessPoint operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.CreateAccessPoint
* @see AWS
* API Documentation
*/
@Override
public CreateAccessPointResponse createAccessPoint(CreateAccessPointRequest createAccessPointRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
HttpResponseHandler> responseHandler = protocolFactory.createCombinedResponseHandler(
CreateAccessPointResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
List metricPublishers = resolveMetricPublishers(clientConfiguration, createAccessPointRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3 Control");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateAccessPoint");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateAccessPoint").withCombinedResponseHandler(responseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(createAccessPointRequest)
.withMarshaller(new CreateAccessPointRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates an Object Lambda Access Point. For more information, see Transforming objects with
* Object Lambda Access Points in the Amazon S3 User Guide.
*
*
* The following actions are related to CreateAccessPointForObjectLambda
:
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* @param createAccessPointForObjectLambdaRequest
* @return Result of the CreateAccessPointForObjectLambda operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.CreateAccessPointForObjectLambda
* @see AWS API Documentation
*/
@Override
public CreateAccessPointForObjectLambdaResponse createAccessPointForObjectLambda(
CreateAccessPointForObjectLambdaRequest createAccessPointForObjectLambdaRequest) throws AwsServiceException,
SdkClientException, S3ControlException {
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(CreateAccessPointForObjectLambdaResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
List metricPublishers = resolveMetricPublishers(clientConfiguration,
createAccessPointForObjectLambdaRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3 Control");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateAccessPointForObjectLambda");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateAccessPointForObjectLambda").withCombinedResponseHandler(responseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(createAccessPointForObjectLambdaRequest)
.withMarshaller(new CreateAccessPointForObjectLambdaRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
*
* This action creates an Amazon S3 on Outposts bucket. To create an S3 bucket, see Create Bucket in the Amazon
* S3 API Reference.
*
*
*
* Creates a new Outposts bucket. By creating the bucket, you become the bucket owner. To create an Outposts bucket,
* you must have S3 on Outposts. For more information, see Using Amazon S3 on Outposts in
* Amazon S3 User Guide.
*
*
* Not every string is an acceptable bucket name. For information on bucket naming restrictions, see Working
* with Amazon S3 Buckets.
*
*
* S3 on Outposts buckets support:
*
*
* -
*
* Tags
*
*
* -
*
* LifecycleConfigurations for deleting expired objects
*
*
*
*
* For a complete list of restrictions and Amazon S3 feature limitations on S3 on Outposts, see Amazon S3
* on Outposts Restrictions and Limitations.
*
*
* For an example of the request syntax for Amazon S3 on Outposts that uses the S3 on Outposts endpoint hostname
* prefix and x-amz-outpost-id
in your API request, see the Examples section.
*
*
* The following actions are related to CreateBucket
for Amazon S3 on Outposts:
*
*
* -
*
* PutObject
*
*
* -
*
* GetBucket
*
*
* -
*
* DeleteBucket
*
*
* -
*
*
* -
*
*
*
*
* @param createBucketRequest
* @return Result of the CreateBucket operation returned by the service.
* @throws BucketAlreadyExistsException
* The requested Outposts bucket name is not available. The bucket namespace is shared by all users of the
* Outposts in this Region. Select a different name and try again.
* @throws BucketAlreadyOwnedByYouException
* The Outposts bucket you tried to create already exists, and you own it.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.CreateBucket
* @see AWS API
* Documentation
*/
@Override
public CreateBucketResponse createBucket(CreateBucketRequest createBucketRequest) throws BucketAlreadyExistsException,
BucketAlreadyOwnedByYouException, AwsServiceException, SdkClientException, S3ControlException {
HttpResponseHandler> responseHandler = protocolFactory.createCombinedResponseHandler(
CreateBucketResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
List metricPublishers = resolveMetricPublishers(clientConfiguration, createBucketRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3 Control");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateBucket");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateBucket").withCombinedResponseHandler(responseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(createBucketRequest)
.putExecutionAttribute(SdkInternalExecutionAttribute.HTTP_CHECKSUM_REQUIRED, HttpChecksumRequired.create())
.withMarshaller(new CreateBucketRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* You can use S3 Batch Operations to perform large-scale batch actions on Amazon S3 objects. Batch Operations can
* run a single action on lists of Amazon S3 objects that you specify. For more information, see S3 Batch Operations in the
* Amazon S3 User Guide.
*
*
* This action creates a S3 Batch Operations job.
*
*
*
* Related actions include:
*
*
* -
*
* DescribeJob
*
*
* -
*
* ListJobs
*
*
* -
*
*
* -
*
* UpdateJobStatus
*
*
* -
*
* JobOperation
*
*
*
*
* @param createJobRequest
* @return Result of the CreateJob operation returned by the service.
* @throws TooManyRequestsException
* @throws BadRequestException
* @throws IdempotencyException
* @throws InternalServiceException
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.CreateJob
* @see AWS API
* Documentation
*/
@Override
public CreateJobResponse createJob(CreateJobRequest createJobRequest) throws TooManyRequestsException, BadRequestException,
IdempotencyException, InternalServiceException, AwsServiceException, SdkClientException, S3ControlException {
HttpResponseHandler> responseHandler = protocolFactory.createCombinedResponseHandler(
CreateJobResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
List metricPublishers = resolveMetricPublishers(clientConfiguration, createJobRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3 Control");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateJob");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateJob").withCombinedResponseHandler(responseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(createJobRequest)
.withMarshaller(new CreateJobRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates a Multi-Region Access Point and associates it with the specified buckets. For more information about
* creating Multi-Region Access Points, see Creating
* Multi-Region Access Points in the Amazon S3 User Guide.
*
*
* This action will always be routed to the US West (Oregon) Region. For more information about the restrictions
* around managing Multi-Region Access Points, see Managing
* Multi-Region Access Points in the Amazon S3 User Guide.
*
*
* This request is asynchronous, meaning that you might receive a response before the command has completed. When
* this request provides a response, it provides a token that you can use to monitor the status of the request with
* DescribeMultiRegionAccessPointOperation
.
*
*
* The following actions are related to CreateMultiRegionAccessPoint
:
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* @param createMultiRegionAccessPointRequest
* @return Result of the CreateMultiRegionAccessPoint operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.CreateMultiRegionAccessPoint
* @see AWS API Documentation
*/
@Override
public CreateMultiRegionAccessPointResponse createMultiRegionAccessPoint(
CreateMultiRegionAccessPointRequest createMultiRegionAccessPointRequest) throws AwsServiceException,
SdkClientException, S3ControlException {
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(CreateMultiRegionAccessPointResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
List metricPublishers = resolveMetricPublishers(clientConfiguration, createMultiRegionAccessPointRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3 Control");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateMultiRegionAccessPoint");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateMultiRegionAccessPoint")
.withCombinedResponseHandler(responseHandler)
.withMetricCollector(apiCallMetricCollector)
.withInput(createMultiRegionAccessPointRequest)
.putExecutionAttribute(SdkInternalExecutionAttribute.HTTP_CHECKSUM_REQUIRED,
HttpChecksumRequired.create())
.withMarshaller(new CreateMultiRegionAccessPointRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes the specified access point.
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request. In addition, you must use an S3 on Outposts endpoint
* hostname prefix instead of s3-control
. For an example of the request syntax for Amazon S3 on
* Outposts that uses the S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived by
* using the access point ARN, see the Examples section.
*
*
* The following actions are related to DeleteAccessPoint
:
*
*
* -
*
*
* -
*
* GetAccessPoint
*
*
* -
*
* ListAccessPoints
*
*
*
*
* @param deleteAccessPointRequest
* @return Result of the DeleteAccessPoint operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.DeleteAccessPoint
* @see AWS
* API Documentation
*/
@Override
public DeleteAccessPointResponse deleteAccessPoint(DeleteAccessPointRequest deleteAccessPointRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
HttpResponseHandler> responseHandler = protocolFactory.createCombinedResponseHandler(
DeleteAccessPointResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteAccessPointRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3 Control");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteAccessPoint");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteAccessPoint").withCombinedResponseHandler(responseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deleteAccessPointRequest)
.withMarshaller(new DeleteAccessPointRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes the specified Object Lambda Access Point.
*
*
* The following actions are related to DeleteAccessPointForObjectLambda
:
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* @param deleteAccessPointForObjectLambdaRequest
* @return Result of the DeleteAccessPointForObjectLambda operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.DeleteAccessPointForObjectLambda
* @see AWS API Documentation
*/
@Override
public DeleteAccessPointForObjectLambdaResponse deleteAccessPointForObjectLambda(
DeleteAccessPointForObjectLambdaRequest deleteAccessPointForObjectLambdaRequest) throws AwsServiceException,
SdkClientException, S3ControlException {
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(DeleteAccessPointForObjectLambdaResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
List metricPublishers = resolveMetricPublishers(clientConfiguration,
deleteAccessPointForObjectLambdaRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3 Control");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteAccessPointForObjectLambda");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteAccessPointForObjectLambda").withCombinedResponseHandler(responseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deleteAccessPointForObjectLambdaRequest)
.withMarshaller(new DeleteAccessPointForObjectLambdaRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes the access point policy for the specified access point.
*
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request. In addition, you must use an S3 on Outposts endpoint
* hostname prefix instead of s3-control
. For an example of the request syntax for Amazon S3 on
* Outposts that uses the S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived by
* using the access point ARN, see the Examples section.
*
*
* The following actions are related to DeleteAccessPointPolicy
:
*
*
* -
*
*
* -
*
*
*
*
* @param deleteAccessPointPolicyRequest
* @return Result of the DeleteAccessPointPolicy operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.DeleteAccessPointPolicy
* @see AWS API Documentation
*/
@Override
public DeleteAccessPointPolicyResponse deleteAccessPointPolicy(DeleteAccessPointPolicyRequest deleteAccessPointPolicyRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(DeleteAccessPointPolicyResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteAccessPointPolicyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3 Control");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteAccessPointPolicy");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteAccessPointPolicy").withCombinedResponseHandler(responseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deleteAccessPointPolicyRequest)
.withMarshaller(new DeleteAccessPointPolicyRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Removes the resource policy for an Object Lambda Access Point.
*
*
* The following actions are related to DeleteAccessPointPolicyForObjectLambda
:
*
*
* -
*
*
* -
*
*
*
*
* @param deleteAccessPointPolicyForObjectLambdaRequest
* @return Result of the DeleteAccessPointPolicyForObjectLambda operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.DeleteAccessPointPolicyForObjectLambda
* @see AWS API Documentation
*/
@Override
public DeleteAccessPointPolicyForObjectLambdaResponse deleteAccessPointPolicyForObjectLambda(
DeleteAccessPointPolicyForObjectLambdaRequest deleteAccessPointPolicyForObjectLambdaRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(DeleteAccessPointPolicyForObjectLambdaResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
List metricPublishers = resolveMetricPublishers(clientConfiguration,
deleteAccessPointPolicyForObjectLambdaRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3 Control");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteAccessPointPolicyForObjectLambda");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteAccessPointPolicyForObjectLambda")
.withCombinedResponseHandler(responseHandler).withMetricCollector(apiCallMetricCollector)
.withInput(deleteAccessPointPolicyForObjectLambdaRequest)
.withMarshaller(new DeleteAccessPointPolicyForObjectLambdaRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
*
* This action deletes an Amazon S3 on Outposts bucket. To delete an S3 bucket, see DeleteBucket in the Amazon S3
* API Reference.
*
*
*
* Deletes the Amazon S3 on Outposts bucket. All objects (including all object versions and delete markers) in the
* bucket must be deleted before the bucket itself can be deleted. For more information, see Using Amazon S3 on Outposts in
* Amazon S3 User Guide.
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request. In addition, you must use an S3 on Outposts endpoint
* hostname prefix instead of s3-control
. For an example of the request syntax for Amazon S3 on
* Outposts that uses the S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived by
* using the access point ARN, see the Examples section.
*
*
* Related Resources
*
*
* -
*
* CreateBucket
*
*
* -
*
* GetBucket
*
*
* -
*
* DeleteObject
*
*
*
*
* @param deleteBucketRequest
* @return Result of the DeleteBucket operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.DeleteBucket
* @see AWS API
* Documentation
*/
@Override
public DeleteBucketResponse deleteBucket(DeleteBucketRequest deleteBucketRequest) throws AwsServiceException,
SdkClientException, S3ControlException {
HttpResponseHandler> responseHandler = protocolFactory.createCombinedResponseHandler(
DeleteBucketResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteBucketRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3 Control");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteBucket");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteBucket").withCombinedResponseHandler(responseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deleteBucketRequest)
.withMarshaller(new DeleteBucketRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
*
* This action deletes an Amazon S3 on Outposts bucket's lifecycle configuration. To delete an S3 bucket's lifecycle
* configuration, see DeleteBucketLifecycle
* in the Amazon S3 API Reference.
*
*
*
* Deletes the lifecycle configuration from the specified Outposts bucket. Amazon S3 on Outposts removes all the
* lifecycle configuration rules in the lifecycle subresource associated with the bucket. Your objects never expire,
* and Amazon S3 on Outposts no longer automatically deletes any objects on the basis of rules contained in the
* deleted lifecycle configuration. For more information, see Using Amazon S3 on Outposts in
* Amazon S3 User Guide.
*
*
* To use this action, you must have permission to perform the s3-outposts:DeleteLifecycleConfiguration
* action. By default, the bucket owner has this permission and the Outposts bucket owner can grant this permission
* to others.
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request. In addition, you must use an S3 on Outposts endpoint
* hostname prefix instead of s3-control
. For an example of the request syntax for Amazon S3 on
* Outposts that uses the S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived by
* using the access point ARN, see the Examples section.
*
*
* For more information about object expiration, see Elements to Describe Lifecycle Actions.
*
*
* Related actions include:
*
*
* -
*
*
* -
*
*
*
*
* @param deleteBucketLifecycleConfigurationRequest
* @return Result of the DeleteBucketLifecycleConfiguration operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.DeleteBucketLifecycleConfiguration
* @see AWS API Documentation
*/
@Override
public DeleteBucketLifecycleConfigurationResponse deleteBucketLifecycleConfiguration(
DeleteBucketLifecycleConfigurationRequest deleteBucketLifecycleConfigurationRequest) throws AwsServiceException,
SdkClientException, S3ControlException {
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(DeleteBucketLifecycleConfigurationResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
List metricPublishers = resolveMetricPublishers(clientConfiguration,
deleteBucketLifecycleConfigurationRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3 Control");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteBucketLifecycleConfiguration");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteBucketLifecycleConfiguration").withCombinedResponseHandler(responseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deleteBucketLifecycleConfigurationRequest)
.withMarshaller(new DeleteBucketLifecycleConfigurationRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
*
* This action deletes an Amazon S3 on Outposts bucket policy. To delete an S3 bucket policy, see DeleteBucketPolicy in the
* Amazon S3 API Reference.
*
*
*
* This implementation of the DELETE action uses the policy subresource to delete the policy of a specified Amazon
* S3 on Outposts bucket. If you are using an identity other than the root user of the Amazon Web Services account
* that owns the bucket, the calling identity must have the s3-outposts:DeleteBucketPolicy
permissions
* on the specified Outposts bucket and belong to the bucket owner's account to use this action. For more
* information, see Using Amazon
* S3 on Outposts in Amazon S3 User Guide.
*
*
* If you don't have DeleteBucketPolicy
permissions, Amazon S3 returns a 403 Access Denied
* error. If you have the correct permissions, but you're not using an identity that belongs to the bucket owner's
* account, Amazon S3 returns a 405 Method Not Allowed
error.
*
*
*
* As a security precaution, the root user of the Amazon Web Services account that owns a bucket can always use this
* action, even if the policy explicitly denies the root user the ability to perform this action.
*
*
*
* For more information about bucket policies, see Using Bucket Policies and User
* Policies.
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request. In addition, you must use an S3 on Outposts endpoint
* hostname prefix instead of s3-control
. For an example of the request syntax for Amazon S3 on
* Outposts that uses the S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived by
* using the access point ARN, see the Examples section.
*
*
* The following actions are related to DeleteBucketPolicy
:
*
*
* -
*
* GetBucketPolicy
*
*
* -
*
* PutBucketPolicy
*
*
*
*
* @param deleteBucketPolicyRequest
* @return Result of the DeleteBucketPolicy operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.DeleteBucketPolicy
* @see AWS
* API Documentation
*/
@Override
public DeleteBucketPolicyResponse deleteBucketPolicy(DeleteBucketPolicyRequest deleteBucketPolicyRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(DeleteBucketPolicyResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteBucketPolicyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3 Control");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteBucketPolicy");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteBucketPolicy").withCombinedResponseHandler(responseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deleteBucketPolicyRequest)
.withMarshaller(new DeleteBucketPolicyRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
*
* This action deletes an Amazon S3 on Outposts bucket's tags. To delete an S3 bucket tags, see DeleteBucketTagging in
* the Amazon S3 API Reference.
*
*
*
* Deletes the tags from the Outposts bucket. For more information, see Using Amazon S3 on Outposts in
* Amazon S3 User Guide.
*
*
* To use this action, you must have permission to perform the PutBucketTagging
action. By default, the
* bucket owner has this permission and can grant this permission to others.
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request. In addition, you must use an S3 on Outposts endpoint
* hostname prefix instead of s3-control
. For an example of the request syntax for Amazon S3 on
* Outposts that uses the S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived by
* using the access point ARN, see the Examples section.
*
*
* The following actions are related to DeleteBucketTagging
:
*
*
* -
*
* GetBucketTagging
*
*
* -
*
* PutBucketTagging
*
*
*
*
* @param deleteBucketTaggingRequest
* @return Result of the DeleteBucketTagging operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.DeleteBucketTagging
* @see AWS
* API Documentation
*/
@Override
public DeleteBucketTaggingResponse deleteBucketTagging(DeleteBucketTaggingRequest deleteBucketTaggingRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(DeleteBucketTaggingResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteBucketTaggingRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3 Control");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteBucketTagging");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteBucketTagging").withCombinedResponseHandler(responseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deleteBucketTaggingRequest)
.withMarshaller(new DeleteBucketTaggingRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Removes the entire tag set from the specified S3 Batch Operations job. To use this operation, you must have
* permission to perform the s3:DeleteJobTagging
action. For more information, see Controlling access and labeling jobs using tags in the Amazon S3 User Guide.
*
*
*
* Related actions include:
*
*
* -
*
* CreateJob
*
*
* -
*
* GetJobTagging
*
*
* -
*
* PutJobTagging
*
*
*
*
* @param deleteJobTaggingRequest
* @return Result of the DeleteJobTagging operation returned by the service.
* @throws InternalServiceException
* @throws TooManyRequestsException
* @throws NotFoundException
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.DeleteJobTagging
* @see AWS
* API Documentation
*/
@Override
public DeleteJobTaggingResponse deleteJobTagging(DeleteJobTaggingRequest deleteJobTaggingRequest)
throws InternalServiceException, TooManyRequestsException, NotFoundException, AwsServiceException,
SdkClientException, S3ControlException {
HttpResponseHandler> responseHandler = protocolFactory.createCombinedResponseHandler(
DeleteJobTaggingResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteJobTaggingRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3 Control");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteJobTagging");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteJobTagging").withCombinedResponseHandler(responseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deleteJobTaggingRequest)
.withMarshaller(new DeleteJobTaggingRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes a Multi-Region Access Point. This action does not delete the buckets associated with the Multi-Region
* Access Point, only the Multi-Region Access Point itself.
*
*
* This action will always be routed to the US West (Oregon) Region. For more information about the restrictions
* around managing Multi-Region Access Points, see Managing
* Multi-Region Access Points in the Amazon S3 User Guide.
*
*
* This request is asynchronous, meaning that you might receive a response before the command has completed. When
* this request provides a response, it provides a token that you can use to monitor the status of the request with
* DescribeMultiRegionAccessPointOperation
.
*
*
* The following actions are related to DeleteMultiRegionAccessPoint
:
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* @param deleteMultiRegionAccessPointRequest
* @return Result of the DeleteMultiRegionAccessPoint operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.DeleteMultiRegionAccessPoint
* @see AWS API Documentation
*/
@Override
public DeleteMultiRegionAccessPointResponse deleteMultiRegionAccessPoint(
DeleteMultiRegionAccessPointRequest deleteMultiRegionAccessPointRequest) throws AwsServiceException,
SdkClientException, S3ControlException {
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(DeleteMultiRegionAccessPointResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteMultiRegionAccessPointRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3 Control");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteMultiRegionAccessPoint");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteMultiRegionAccessPoint")
.withCombinedResponseHandler(responseHandler)
.withMetricCollector(apiCallMetricCollector)
.withInput(deleteMultiRegionAccessPointRequest)
.putExecutionAttribute(SdkInternalExecutionAttribute.HTTP_CHECKSUM_REQUIRED,
HttpChecksumRequired.create())
.withMarshaller(new DeleteMultiRegionAccessPointRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Removes the PublicAccessBlock
configuration for an Amazon Web Services account. For more
* information, see Using Amazon S3
* block public access.
*
*
* Related actions include:
*
*
* -
*
*
* -
*
*
*
*
* @param deletePublicAccessBlockRequest
* @return Result of the DeletePublicAccessBlock operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.DeletePublicAccessBlock
* @see AWS API Documentation
*/
@Override
public DeletePublicAccessBlockResponse deletePublicAccessBlock(DeletePublicAccessBlockRequest deletePublicAccessBlockRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(DeletePublicAccessBlockResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
List metricPublishers = resolveMetricPublishers(clientConfiguration, deletePublicAccessBlockRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3 Control");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeletePublicAccessBlock");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeletePublicAccessBlock").withCombinedResponseHandler(responseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deletePublicAccessBlockRequest)
.withMarshaller(new DeletePublicAccessBlockRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes the Amazon S3 Storage Lens configuration. For more information about S3 Storage Lens, see Assessing your storage activity and
* usage with Amazon S3 Storage Lens in the Amazon S3 User Guide.
*
*
*
* To use this action, you must have permission to perform the s3:DeleteStorageLensConfiguration
* action. For more information, see Setting permissions to
* use Amazon S3 Storage Lens in the Amazon S3 User Guide.
*
*
*
* @param deleteStorageLensConfigurationRequest
* @return Result of the DeleteStorageLensConfiguration operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.DeleteStorageLensConfiguration
* @see AWS API Documentation
*/
@Override
public DeleteStorageLensConfigurationResponse deleteStorageLensConfiguration(
DeleteStorageLensConfigurationRequest deleteStorageLensConfigurationRequest) throws AwsServiceException,
SdkClientException, S3ControlException {
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(DeleteStorageLensConfigurationResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
List metricPublishers = resolveMetricPublishers(clientConfiguration,
deleteStorageLensConfigurationRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3 Control");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteStorageLensConfiguration");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteStorageLensConfiguration").withCombinedResponseHandler(responseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deleteStorageLensConfigurationRequest)
.withMarshaller(new DeleteStorageLensConfigurationRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes the Amazon S3 Storage Lens configuration tags. For more information about S3 Storage Lens, see Assessing your storage activity and
* usage with Amazon S3 Storage Lens in the Amazon S3 User Guide.
*
*
*
* To use this action, you must have permission to perform the s3:DeleteStorageLensConfigurationTagging
* action. For more information, see Setting permissions to
* use Amazon S3 Storage Lens in the Amazon S3 User Guide.
*
*
*
* @param deleteStorageLensConfigurationTaggingRequest
* @return Result of the DeleteStorageLensConfigurationTagging operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.DeleteStorageLensConfigurationTagging
* @see AWS API Documentation
*/
@Override
public DeleteStorageLensConfigurationTaggingResponse deleteStorageLensConfigurationTagging(
DeleteStorageLensConfigurationTaggingRequest deleteStorageLensConfigurationTaggingRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(DeleteStorageLensConfigurationTaggingResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
List metricPublishers = resolveMetricPublishers(clientConfiguration,
deleteStorageLensConfigurationTaggingRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3 Control");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteStorageLensConfigurationTagging");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteStorageLensConfigurationTagging")
.withCombinedResponseHandler(responseHandler).withMetricCollector(apiCallMetricCollector)
.withInput(deleteStorageLensConfigurationTaggingRequest)
.withMarshaller(new DeleteStorageLensConfigurationTaggingRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves the configuration parameters and status for a Batch Operations job. For more information, see S3 Batch Operations in the
* Amazon S3 User Guide.
*
*
*
* Related actions include:
*
*
* -
*
* CreateJob
*
*
* -
*
* ListJobs
*
*
* -
*
*
* -
*
* UpdateJobStatus
*
*
*
*
* @param describeJobRequest
* @return Result of the DescribeJob operation returned by the service.
* @throws BadRequestException
* @throws TooManyRequestsException
* @throws NotFoundException
* @throws InternalServiceException
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.DescribeJob
* @see AWS API
* Documentation
*/
@Override
public DescribeJobResponse describeJob(DescribeJobRequest describeJobRequest) throws BadRequestException,
TooManyRequestsException, NotFoundException, InternalServiceException, AwsServiceException, SdkClientException,
S3ControlException {
HttpResponseHandler> responseHandler = protocolFactory.createCombinedResponseHandler(
DescribeJobResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeJobRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3 Control");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeJob");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeJob").withCombinedResponseHandler(responseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(describeJobRequest)
.withMarshaller(new DescribeJobRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves the status of an asynchronous request to manage a Multi-Region Access Point. For more information about
* managing Multi-Region Access Points and how asynchronous requests work, see Managing
* Multi-Region Access Points in the Amazon S3 User Guide.
*
*
* The following actions are related to GetMultiRegionAccessPoint
:
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* @param describeMultiRegionAccessPointOperationRequest
* @return Result of the DescribeMultiRegionAccessPointOperation operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.DescribeMultiRegionAccessPointOperation
* @see AWS API Documentation
*/
@Override
public DescribeMultiRegionAccessPointOperationResponse describeMultiRegionAccessPointOperation(
DescribeMultiRegionAccessPointOperationRequest describeMultiRegionAccessPointOperationRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(DescribeMultiRegionAccessPointOperationResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
List metricPublishers = resolveMetricPublishers(clientConfiguration,
describeMultiRegionAccessPointOperationRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3 Control");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeMultiRegionAccessPointOperation");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeMultiRegionAccessPointOperation")
.withCombinedResponseHandler(responseHandler)
.withMetricCollector(apiCallMetricCollector)
.withInput(describeMultiRegionAccessPointOperationRequest)
.putExecutionAttribute(SdkInternalExecutionAttribute.HTTP_CHECKSUM_REQUIRED,
HttpChecksumRequired.create())
.withMarshaller(new DescribeMultiRegionAccessPointOperationRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns configuration information about the specified access point.
*
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request. In addition, you must use an S3 on Outposts endpoint
* hostname prefix instead of s3-control
. For an example of the request syntax for Amazon S3 on
* Outposts that uses the S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived by
* using the access point ARN, see the Examples section.
*
*
* The following actions are related to GetAccessPoint
:
*
*
* -
*
*
* -
*
*
* -
*
* ListAccessPoints
*
*
*
*
* @param getAccessPointRequest
* @return Result of the GetAccessPoint operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.GetAccessPoint
* @see AWS API
* Documentation
*/
@Override
public GetAccessPointResponse getAccessPoint(GetAccessPointRequest getAccessPointRequest) throws AwsServiceException,
SdkClientException, S3ControlException {
HttpResponseHandler> responseHandler = protocolFactory.createCombinedResponseHandler(
GetAccessPointResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
List metricPublishers = resolveMetricPublishers(clientConfiguration, getAccessPointRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3 Control");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetAccessPoint");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetAccessPoint").withCombinedResponseHandler(responseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(getAccessPointRequest)
.withMarshaller(new GetAccessPointRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns configuration for an Object Lambda Access Point.
*
*
* The following actions are related to GetAccessPointConfigurationForObjectLambda
:
*
*
* -
*
*
*
*
* @param getAccessPointConfigurationForObjectLambdaRequest
* @return Result of the GetAccessPointConfigurationForObjectLambda operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.GetAccessPointConfigurationForObjectLambda
* @see AWS API Documentation
*/
@Override
public GetAccessPointConfigurationForObjectLambdaResponse getAccessPointConfigurationForObjectLambda(
GetAccessPointConfigurationForObjectLambdaRequest getAccessPointConfigurationForObjectLambdaRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(GetAccessPointConfigurationForObjectLambdaResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
List metricPublishers = resolveMetricPublishers(clientConfiguration,
getAccessPointConfigurationForObjectLambdaRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3 Control");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetAccessPointConfigurationForObjectLambda");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetAccessPointConfigurationForObjectLambda")
.withCombinedResponseHandler(responseHandler).withMetricCollector(apiCallMetricCollector)
.withInput(getAccessPointConfigurationForObjectLambdaRequest)
.withMarshaller(new GetAccessPointConfigurationForObjectLambdaRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns configuration information about the specified Object Lambda Access Point
*
*
* The following actions are related to GetAccessPointForObjectLambda
:
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* @param getAccessPointForObjectLambdaRequest
* @return Result of the GetAccessPointForObjectLambda operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.GetAccessPointForObjectLambda
* @see AWS API Documentation
*/
@Override
public GetAccessPointForObjectLambdaResponse getAccessPointForObjectLambda(
GetAccessPointForObjectLambdaRequest getAccessPointForObjectLambdaRequest) throws AwsServiceException,
SdkClientException, S3ControlException {
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(GetAccessPointForObjectLambdaResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
List metricPublishers = resolveMetricPublishers(clientConfiguration,
getAccessPointForObjectLambdaRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3 Control");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetAccessPointForObjectLambda");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetAccessPointForObjectLambda").withCombinedResponseHandler(responseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(getAccessPointForObjectLambdaRequest)
.withMarshaller(new GetAccessPointForObjectLambdaRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns the access point policy associated with the specified access point.
*
*
* The following actions are related to GetAccessPointPolicy
:
*
*
* -
*
*
* -
*
*
*
*
* @param getAccessPointPolicyRequest
* @return Result of the GetAccessPointPolicy operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.GetAccessPointPolicy
* @see AWS API Documentation
*/
@Override
public GetAccessPointPolicyResponse getAccessPointPolicy(GetAccessPointPolicyRequest getAccessPointPolicyRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(GetAccessPointPolicyResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
List metricPublishers = resolveMetricPublishers(clientConfiguration, getAccessPointPolicyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3 Control");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetAccessPointPolicy");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetAccessPointPolicy").withCombinedResponseHandler(responseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(getAccessPointPolicyRequest)
.withMarshaller(new GetAccessPointPolicyRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns the resource policy for an Object Lambda Access Point.
*
*
* The following actions are related to GetAccessPointPolicyForObjectLambda
:
*
*
* -
*
*
* -
*
*
*
*
* @param getAccessPointPolicyForObjectLambdaRequest
* @return Result of the GetAccessPointPolicyForObjectLambda operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.GetAccessPointPolicyForObjectLambda
* @see AWS API Documentation
*/
@Override
public GetAccessPointPolicyForObjectLambdaResponse getAccessPointPolicyForObjectLambda(
GetAccessPointPolicyForObjectLambdaRequest getAccessPointPolicyForObjectLambdaRequest) throws AwsServiceException,
SdkClientException, S3ControlException {
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(GetAccessPointPolicyForObjectLambdaResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
List metricPublishers = resolveMetricPublishers(clientConfiguration,
getAccessPointPolicyForObjectLambdaRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3 Control");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetAccessPointPolicyForObjectLambda");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetAccessPointPolicyForObjectLambda")
.withCombinedResponseHandler(responseHandler).withMetricCollector(apiCallMetricCollector)
.withInput(getAccessPointPolicyForObjectLambdaRequest)
.withMarshaller(new GetAccessPointPolicyForObjectLambdaRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Indicates whether the specified access point currently has a policy that allows public access. For more
* information about public access through access points, see Managing Data Access with Amazon
* S3 access points in the Amazon S3 User Guide.
*
*
* @param getAccessPointPolicyStatusRequest
* @return Result of the GetAccessPointPolicyStatus operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.GetAccessPointPolicyStatus
* @see AWS API Documentation
*/
@Override
public GetAccessPointPolicyStatusResponse getAccessPointPolicyStatus(
GetAccessPointPolicyStatusRequest getAccessPointPolicyStatusRequest) throws AwsServiceException, SdkClientException,
S3ControlException {
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(GetAccessPointPolicyStatusResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
List metricPublishers = resolveMetricPublishers(clientConfiguration, getAccessPointPolicyStatusRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3 Control");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetAccessPointPolicyStatus");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetAccessPointPolicyStatus").withCombinedResponseHandler(responseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(getAccessPointPolicyStatusRequest)
.withMarshaller(new GetAccessPointPolicyStatusRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns the status of the resource policy associated with an Object Lambda Access Point.
*
*
* @param getAccessPointPolicyStatusForObjectLambdaRequest
* @return Result of the GetAccessPointPolicyStatusForObjectLambda operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.GetAccessPointPolicyStatusForObjectLambda
* @see AWS API Documentation
*/
@Override
public GetAccessPointPolicyStatusForObjectLambdaResponse getAccessPointPolicyStatusForObjectLambda(
GetAccessPointPolicyStatusForObjectLambdaRequest getAccessPointPolicyStatusForObjectLambdaRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(GetAccessPointPolicyStatusForObjectLambdaResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
List metricPublishers = resolveMetricPublishers(clientConfiguration,
getAccessPointPolicyStatusForObjectLambdaRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3 Control");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetAccessPointPolicyStatusForObjectLambda");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetAccessPointPolicyStatusForObjectLambda")
.withCombinedResponseHandler(responseHandler).withMetricCollector(apiCallMetricCollector)
.withInput(getAccessPointPolicyStatusForObjectLambdaRequest)
.withMarshaller(new GetAccessPointPolicyStatusForObjectLambdaRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Gets an Amazon S3 on Outposts bucket. For more information, see Using Amazon S3 on Outposts
* in the Amazon S3 User Guide.
*
*
* If you are using an identity other than the root user of the Amazon Web Services account that owns the Outposts
* bucket, the calling identity must have the s3-outposts:GetBucket
permissions on the specified
* Outposts bucket and belong to the Outposts bucket owner's account in order to use this action. Only users from
* Outposts bucket owner account with the right permissions can perform actions on an Outposts bucket.
*
*
* If you don't have s3-outposts:GetBucket
permissions or you're not using an identity that belongs to
* the bucket owner's account, Amazon S3 returns a 403 Access Denied
error.
*
*
* The following actions are related to GetBucket
for Amazon S3 on Outposts:
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request. In addition, you must use an S3 on Outposts endpoint
* hostname prefix instead of s3-control
. For an example of the request syntax for Amazon S3 on
* Outposts that uses the S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived by
* using the access point ARN, see the Examples section.
*
*
* -
*
* PutObject
*
*
* -
*
* CreateBucket
*
*
* -
*
* DeleteBucket
*
*
*
*
* @param getBucketRequest
* @return Result of the GetBucket operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.GetBucket
* @see AWS API
* Documentation
*/
@Override
public GetBucketResponse getBucket(GetBucketRequest getBucketRequest) throws AwsServiceException, SdkClientException,
S3ControlException {
HttpResponseHandler> responseHandler = protocolFactory.createCombinedResponseHandler(
GetBucketResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
List metricPublishers = resolveMetricPublishers(clientConfiguration, getBucketRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3 Control");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetBucket");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetBucket").withCombinedResponseHandler(responseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(getBucketRequest)
.withMarshaller(new GetBucketRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
*
* This action gets an Amazon S3 on Outposts bucket's lifecycle configuration. To get an S3 bucket's lifecycle
* configuration, see GetBucketLifecycleConfiguration in the Amazon S3 API Reference.
*
*
*
* Returns the lifecycle configuration information set on the Outposts bucket. For more information, see Using Amazon S3 on Outposts
* and for information about lifecycle configuration, see Object Lifecycle
* Management in Amazon S3 User Guide.
*
*
* To use this action, you must have permission to perform the s3-outposts:GetLifecycleConfiguration
* action. The Outposts bucket owner has this permission, by default. The bucket owner can grant this permission to
* others. For more information about permissions, see Permissions Related to Bucket Subresource Operations and Managing Access Permissions
* to Your Amazon S3 Resources.
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request. In addition, you must use an S3 on Outposts endpoint
* hostname prefix instead of s3-control
. For an example of the request syntax for Amazon S3 on
* Outposts that uses the S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived by
* using the access point ARN, see the Examples section.
*
*
* GetBucketLifecycleConfiguration
has the following special error:
*
*
* -
*
* Error code: NoSuchLifecycleConfiguration
*
*
* -
*
* Description: The lifecycle configuration does not exist.
*
*
* -
*
* HTTP Status Code: 404 Not Found
*
*
* -
*
* SOAP Fault Code Prefix: Client
*
*
*
*
*
*
* The following actions are related to GetBucketLifecycleConfiguration
:
*
*
* -
*
*
* -
*
*
*
*
* @param getBucketLifecycleConfigurationRequest
* @return Result of the GetBucketLifecycleConfiguration operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.GetBucketLifecycleConfiguration
* @see AWS API Documentation
*/
@Override
public GetBucketLifecycleConfigurationResponse getBucketLifecycleConfiguration(
GetBucketLifecycleConfigurationRequest getBucketLifecycleConfigurationRequest) throws AwsServiceException,
SdkClientException, S3ControlException {
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(GetBucketLifecycleConfigurationResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
List metricPublishers = resolveMetricPublishers(clientConfiguration,
getBucketLifecycleConfigurationRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3 Control");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetBucketLifecycleConfiguration");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetBucketLifecycleConfiguration").withCombinedResponseHandler(responseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(getBucketLifecycleConfigurationRequest)
.withMarshaller(new GetBucketLifecycleConfigurationRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
*
* This action gets a bucket policy for an Amazon S3 on Outposts bucket. To get a policy for an S3 bucket, see GetBucketPolicy in the
* Amazon S3 API Reference.
*
*
*
* Returns the policy of a specified Outposts bucket. For more information, see Using Amazon S3 on Outposts in
* the Amazon S3 User Guide.
*
*
* If you are using an identity other than the root user of the Amazon Web Services account that owns the bucket,
* the calling identity must have the GetBucketPolicy
permissions on the specified bucket and belong to
* the bucket owner's account in order to use this action.
*
*
* Only users from Outposts bucket owner account with the right permissions can perform actions on an Outposts
* bucket. If you don't have s3-outposts:GetBucketPolicy
permissions or you're not using an identity
* that belongs to the bucket owner's account, Amazon S3 returns a 403 Access Denied
error.
*
*
*
* As a security precaution, the root user of the Amazon Web Services account that owns a bucket can always use this
* action, even if the policy explicitly denies the root user the ability to perform this action.
*
*
*
* For more information about bucket policies, see Using Bucket Policies and User
* Policies.
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request. In addition, you must use an S3 on Outposts endpoint
* hostname prefix instead of s3-control
. For an example of the request syntax for Amazon S3 on
* Outposts that uses the S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived by
* using the access point ARN, see the Examples section.
*
*
* The following actions are related to GetBucketPolicy
:
*
*
* -
*
* GetObject
*
*
* -
*
* PutBucketPolicy
*
*
* -
*
*
*
*
* @param getBucketPolicyRequest
* @return Result of the GetBucketPolicy operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.GetBucketPolicy
* @see AWS API
* Documentation
*/
@Override
public GetBucketPolicyResponse getBucketPolicy(GetBucketPolicyRequest getBucketPolicyRequest) throws AwsServiceException,
SdkClientException, S3ControlException {
HttpResponseHandler> responseHandler = protocolFactory.createCombinedResponseHandler(
GetBucketPolicyResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
List metricPublishers = resolveMetricPublishers(clientConfiguration, getBucketPolicyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3 Control");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetBucketPolicy");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetBucketPolicy").withCombinedResponseHandler(responseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(getBucketPolicyRequest)
.withMarshaller(new GetBucketPolicyRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
*
* This action gets an Amazon S3 on Outposts bucket's tags. To get an S3 bucket tags, see GetBucketTagging in the
* Amazon S3 API Reference.
*
*
*
* Returns the tag set associated with the Outposts bucket. For more information, see Using Amazon S3 on Outposts in
* the Amazon S3 User Guide.
*
*
* To use this action, you must have permission to perform the GetBucketTagging
action. By default, the
* bucket owner has this permission and can grant this permission to others.
*
*
* GetBucketTagging
has the following special error:
*
*
* -
*
* Error code: NoSuchTagSetError
*
*
* -
*
* Description: There is no tag set associated with the bucket.
*
*
*
*
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request. In addition, you must use an S3 on Outposts endpoint
* hostname prefix instead of s3-control
. For an example of the request syntax for Amazon S3 on
* Outposts that uses the S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived by
* using the access point ARN, see the Examples section.
*
*
* The following actions are related to GetBucketTagging
:
*
*
* -
*
* PutBucketTagging
*
*
* -
*
*
*
*
* @param getBucketTaggingRequest
* @return Result of the GetBucketTagging operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.GetBucketTagging
* @see AWS
* API Documentation
*/
@Override
public GetBucketTaggingResponse getBucketTagging(GetBucketTaggingRequest getBucketTaggingRequest) throws AwsServiceException,
SdkClientException, S3ControlException {
HttpResponseHandler> responseHandler = protocolFactory.createCombinedResponseHandler(
GetBucketTaggingResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
List metricPublishers = resolveMetricPublishers(clientConfiguration, getBucketTaggingRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3 Control");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetBucketTagging");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetBucketTagging").withCombinedResponseHandler(responseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(getBucketTaggingRequest)
.withMarshaller(new GetBucketTaggingRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
*
* This operation returns the versioning state only for S3 on Outposts buckets. To return the versioning state for
* an S3 bucket, see GetBucketVersioning in
* the Amazon S3 API Reference.
*
*
*
* Returns the versioning state for an S3 on Outposts bucket. With versioning, you can save multiple distinct copies
* of your data and recover from unintended user actions and application failures.
*
*
* If you've never set versioning on your bucket, it has no versioning state. In that case, the
* GetBucketVersioning
request does not return a versioning state value.
*
*
* For more information about versioning, see Versioning in the Amazon S3
* User Guide.
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request. In addition, you must use an S3 on Outposts endpoint
* hostname prefix instead of s3-control
. For an example of the request syntax for Amazon S3 on
* Outposts that uses the S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived by
* using the access point ARN, see the Examples section.
*
*
* The following operations are related to GetBucketVersioning
for S3 on Outposts.
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* @param getBucketVersioningRequest
* @return Result of the GetBucketVersioning operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.GetBucketVersioning
* @see AWS
* API Documentation
*/
@Override
public GetBucketVersioningResponse getBucketVersioning(GetBucketVersioningRequest getBucketVersioningRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(GetBucketVersioningResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
List metricPublishers = resolveMetricPublishers(clientConfiguration, getBucketVersioningRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3 Control");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetBucketVersioning");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetBucketVersioning").withCombinedResponseHandler(responseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(getBucketVersioningRequest)
.withMarshaller(new GetBucketVersioningRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns the tags on an S3 Batch Operations job. To use this operation, you must have permission to perform the
* s3:GetJobTagging
action. For more information, see Controlling access and labeling jobs using tags in the Amazon S3 User Guide.
*
*
*
* Related actions include:
*
*
* -
*
* CreateJob
*
*
* -
*
* PutJobTagging
*
*
* -
*
* DeleteJobTagging
*
*
*
*
* @param getJobTaggingRequest
* @return Result of the GetJobTagging operation returned by the service.
* @throws InternalServiceException
* @throws TooManyRequestsException
* @throws NotFoundException
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.GetJobTagging
* @see AWS API
* Documentation
*/
@Override
public GetJobTaggingResponse getJobTagging(GetJobTaggingRequest getJobTaggingRequest) throws InternalServiceException,
TooManyRequestsException, NotFoundException, AwsServiceException, SdkClientException, S3ControlException {
HttpResponseHandler> responseHandler = protocolFactory.createCombinedResponseHandler(
GetJobTaggingResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
List metricPublishers = resolveMetricPublishers(clientConfiguration, getJobTaggingRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3 Control");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetJobTagging");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetJobTagging").withCombinedResponseHandler(responseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(getJobTaggingRequest)
.withMarshaller(new GetJobTaggingRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns configuration information about the specified Multi-Region Access Point.
*
*
* This action will always be routed to the US West (Oregon) Region. For more information about the restrictions
* around managing Multi-Region Access Points, see Managing
* Multi-Region Access Points in the Amazon S3 User Guide.
*
*
* The following actions are related to GetMultiRegionAccessPoint
:
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* @param getMultiRegionAccessPointRequest
* @return Result of the GetMultiRegionAccessPoint operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.GetMultiRegionAccessPoint
* @see AWS API Documentation
*/
@Override
public GetMultiRegionAccessPointResponse getMultiRegionAccessPoint(
GetMultiRegionAccessPointRequest getMultiRegionAccessPointRequest) throws AwsServiceException, SdkClientException,
S3ControlException {
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(GetMultiRegionAccessPointResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
List metricPublishers = resolveMetricPublishers(clientConfiguration, getMultiRegionAccessPointRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3 Control");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetMultiRegionAccessPoint");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetMultiRegionAccessPoint")
.withCombinedResponseHandler(responseHandler)
.withMetricCollector(apiCallMetricCollector)
.withInput(getMultiRegionAccessPointRequest)
.putExecutionAttribute(SdkInternalExecutionAttribute.HTTP_CHECKSUM_REQUIRED,
HttpChecksumRequired.create())
.withMarshaller(new GetMultiRegionAccessPointRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns the access control policy of the specified Multi-Region Access Point.
*
*
* This action will always be routed to the US West (Oregon) Region. For more information about the restrictions
* around managing Multi-Region Access Points, see Managing
* Multi-Region Access Points in the Amazon S3 User Guide.
*
*
* The following actions are related to GetMultiRegionAccessPointPolicy
:
*
*
* -
*
*
* -
*
*
*
*
* @param getMultiRegionAccessPointPolicyRequest
* @return Result of the GetMultiRegionAccessPointPolicy operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.GetMultiRegionAccessPointPolicy
* @see AWS API Documentation
*/
@Override
public GetMultiRegionAccessPointPolicyResponse getMultiRegionAccessPointPolicy(
GetMultiRegionAccessPointPolicyRequest getMultiRegionAccessPointPolicyRequest) throws AwsServiceException,
SdkClientException, S3ControlException {
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(GetMultiRegionAccessPointPolicyResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
List metricPublishers = resolveMetricPublishers(clientConfiguration,
getMultiRegionAccessPointPolicyRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3 Control");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetMultiRegionAccessPointPolicy");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetMultiRegionAccessPointPolicy")
.withCombinedResponseHandler(responseHandler)
.withMetricCollector(apiCallMetricCollector)
.withInput(getMultiRegionAccessPointPolicyRequest)
.putExecutionAttribute(SdkInternalExecutionAttribute.HTTP_CHECKSUM_REQUIRED,
HttpChecksumRequired.create())
.withMarshaller(new GetMultiRegionAccessPointPolicyRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Indicates whether the specified Multi-Region Access Point has an access control policy that allows public access.
*
*
* This action will always be routed to the US West (Oregon) Region. For more information about the restrictions
* around managing Multi-Region Access Points, see Managing
* Multi-Region Access Points in the Amazon S3 User Guide.
*
*
* The following actions are related to GetMultiRegionAccessPointPolicyStatus
:
*
*
* -
*
*
* -
*
*
*
*
* @param getMultiRegionAccessPointPolicyStatusRequest
* @return Result of the GetMultiRegionAccessPointPolicyStatus operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.GetMultiRegionAccessPointPolicyStatus
* @see AWS API Documentation
*/
@Override
public GetMultiRegionAccessPointPolicyStatusResponse getMultiRegionAccessPointPolicyStatus(
GetMultiRegionAccessPointPolicyStatusRequest getMultiRegionAccessPointPolicyStatusRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(GetMultiRegionAccessPointPolicyStatusResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
List metricPublishers = resolveMetricPublishers(clientConfiguration,
getMultiRegionAccessPointPolicyStatusRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3 Control");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetMultiRegionAccessPointPolicyStatus");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetMultiRegionAccessPointPolicyStatus")
.withCombinedResponseHandler(responseHandler)
.withMetricCollector(apiCallMetricCollector)
.withInput(getMultiRegionAccessPointPolicyStatusRequest)
.putExecutionAttribute(SdkInternalExecutionAttribute.HTTP_CHECKSUM_REQUIRED,
HttpChecksumRequired.create())
.withMarshaller(new GetMultiRegionAccessPointPolicyStatusRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns the routing configuration for a Multi-Region Access Point, indicating which Regions are active or
* passive.
*
*
* To obtain routing control changes and failover requests, use the Amazon S3 failover control infrastructure
* endpoints in these five Amazon Web Services Regions:
*
*
* -
*
* us-east-1
*
*
* -
*
* us-west-2
*
*
* -
*
* ap-southeast-2
*
*
* -
*
* ap-northeast-1
*
*
* -
*
* eu-west-1
*
*
*
*
*
* Your Amazon S3 bucket does not need to be in these five Regions.
*
*
*
* @param getMultiRegionAccessPointRoutesRequest
* @return Result of the GetMultiRegionAccessPointRoutes operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.GetMultiRegionAccessPointRoutes
* @see AWS API Documentation
*/
@Override
public GetMultiRegionAccessPointRoutesResponse getMultiRegionAccessPointRoutes(
GetMultiRegionAccessPointRoutesRequest getMultiRegionAccessPointRoutesRequest) throws AwsServiceException,
SdkClientException, S3ControlException {
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(GetMultiRegionAccessPointRoutesResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
List metricPublishers = resolveMetricPublishers(clientConfiguration,
getMultiRegionAccessPointRoutesRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3 Control");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetMultiRegionAccessPointRoutes");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetMultiRegionAccessPointRoutes")
.withCombinedResponseHandler(responseHandler)
.withMetricCollector(apiCallMetricCollector)
.withInput(getMultiRegionAccessPointRoutesRequest)
.putExecutionAttribute(SdkInternalExecutionAttribute.HTTP_CHECKSUM_REQUIRED,
HttpChecksumRequired.create())
.withMarshaller(new GetMultiRegionAccessPointRoutesRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves the PublicAccessBlock
configuration for an Amazon Web Services account. For more
* information, see Using Amazon S3
* block public access.
*
*
* Related actions include:
*
*
* -
*
*
* -
*
*
*
*
* @param getPublicAccessBlockRequest
* @return Result of the GetPublicAccessBlock operation returned by the service.
* @throws NoSuchPublicAccessBlockConfigurationException
* Amazon S3 throws this exception if you make a GetPublicAccessBlock
request against an
* account that doesn't have a PublicAccessBlockConfiguration
set.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.GetPublicAccessBlock
* @see AWS API Documentation
*/
@Override
public GetPublicAccessBlockResponse getPublicAccessBlock(GetPublicAccessBlockRequest getPublicAccessBlockRequest)
throws NoSuchPublicAccessBlockConfigurationException, AwsServiceException, SdkClientException, S3ControlException {
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(GetPublicAccessBlockResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
List metricPublishers = resolveMetricPublishers(clientConfiguration, getPublicAccessBlockRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3 Control");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetPublicAccessBlock");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetPublicAccessBlock").withCombinedResponseHandler(responseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(getPublicAccessBlockRequest)
.withMarshaller(new GetPublicAccessBlockRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Gets the Amazon S3 Storage Lens configuration. For more information, see Assessing your storage activity and
* usage with Amazon S3 Storage Lens in the Amazon S3 User Guide. For a complete list of S3 Storage Lens
* metrics, see S3 Storage Lens
* metrics glossary in the Amazon S3 User Guide.
*
*
*
* To use this action, you must have permission to perform the s3:GetStorageLensConfiguration
action.
* For more information, see Setting permissions to
* use Amazon S3 Storage Lens in the Amazon S3 User Guide.
*
*
*
* @param getStorageLensConfigurationRequest
* @return Result of the GetStorageLensConfiguration operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.GetStorageLensConfiguration
* @see AWS API Documentation
*/
@Override
public GetStorageLensConfigurationResponse getStorageLensConfiguration(
GetStorageLensConfigurationRequest getStorageLensConfigurationRequest) throws AwsServiceException,
SdkClientException, S3ControlException {
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(GetStorageLensConfigurationResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
List metricPublishers = resolveMetricPublishers(clientConfiguration, getStorageLensConfigurationRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3 Control");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetStorageLensConfiguration");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetStorageLensConfiguration").withCombinedResponseHandler(responseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(getStorageLensConfigurationRequest)
.withMarshaller(new GetStorageLensConfigurationRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Gets the tags of Amazon S3 Storage Lens configuration. For more information about S3 Storage Lens, see Assessing your storage activity and
* usage with Amazon S3 Storage Lens in the Amazon S3 User Guide.
*
*
*
* To use this action, you must have permission to perform the s3:GetStorageLensConfigurationTagging
* action. For more information, see Setting permissions to
* use Amazon S3 Storage Lens in the Amazon S3 User Guide.
*
*
*
* @param getStorageLensConfigurationTaggingRequest
* @return Result of the GetStorageLensConfigurationTagging operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.GetStorageLensConfigurationTagging
* @see AWS API Documentation
*/
@Override
public GetStorageLensConfigurationTaggingResponse getStorageLensConfigurationTagging(
GetStorageLensConfigurationTaggingRequest getStorageLensConfigurationTaggingRequest) throws AwsServiceException,
SdkClientException, S3ControlException {
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(GetStorageLensConfigurationTaggingResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
List metricPublishers = resolveMetricPublishers(clientConfiguration,
getStorageLensConfigurationTaggingRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3 Control");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetStorageLensConfigurationTagging");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetStorageLensConfigurationTagging").withCombinedResponseHandler(responseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(getStorageLensConfigurationTaggingRequest)
.withMarshaller(new GetStorageLensConfigurationTaggingRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns a list of the access points owned by the current account associated with the specified bucket. You can
* retrieve up to 1000 access points per call. If the specified bucket has more than 1,000 access points (or the
* number specified in maxResults
, whichever is less), the response will include a continuation token
* that you can use to list the additional access points.
*
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request. In addition, you must use an S3 on Outposts endpoint
* hostname prefix instead of s3-control
. For an example of the request syntax for Amazon S3 on
* Outposts that uses the S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived by
* using the access point ARN, see the Examples section.
*
*
* The following actions are related to ListAccessPoints
:
*
*
* -
*
*
* -
*
*
* -
*
* GetAccessPoint
*
*
*
*
* @param listAccessPointsRequest
* @return Result of the ListAccessPoints operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.ListAccessPoints
* @see AWS
* API Documentation
*/
@Override
public ListAccessPointsResponse listAccessPoints(ListAccessPointsRequest listAccessPointsRequest) throws AwsServiceException,
SdkClientException, S3ControlException {
HttpResponseHandler> responseHandler = protocolFactory.createCombinedResponseHandler(
ListAccessPointsResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
List metricPublishers = resolveMetricPublishers(clientConfiguration, listAccessPointsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3 Control");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListAccessPoints");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListAccessPoints").withCombinedResponseHandler(responseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(listAccessPointsRequest)
.withMarshaller(new ListAccessPointsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns a list of the access points owned by the current account associated with the specified bucket. You can
* retrieve up to 1000 access points per call. If the specified bucket has more than 1,000 access points (or the
* number specified in maxResults
, whichever is less), the response will include a continuation token
* that you can use to list the additional access points.
*
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request. In addition, you must use an S3 on Outposts endpoint
* hostname prefix instead of s3-control
. For an example of the request syntax for Amazon S3 on
* Outposts that uses the S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived by
* using the access point ARN, see the Examples section.
*
*
* The following actions are related to ListAccessPoints
:
*
*
* -
*
*
* -
*
*
* -
*
* GetAccessPoint
*
*
*
*
*
* This is a variant of
* {@link #listAccessPoints(software.amazon.awssdk.services.s3control.model.ListAccessPointsRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.s3control.paginators.ListAccessPointsIterable responses = client.listAccessPointsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.s3control.paginators.ListAccessPointsIterable responses = client
* .listAccessPointsPaginator(request);
* for (software.amazon.awssdk.services.s3control.model.ListAccessPointsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.s3control.paginators.ListAccessPointsIterable responses = client.listAccessPointsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listAccessPoints(software.amazon.awssdk.services.s3control.model.ListAccessPointsRequest)} operation.
*
*
* @param listAccessPointsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.ListAccessPoints
* @see AWS
* API Documentation
*/
@Override
public ListAccessPointsIterable listAccessPointsPaginator(ListAccessPointsRequest listAccessPointsRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
return new ListAccessPointsIterable(this, applyPaginatorUserAgent(listAccessPointsRequest));
}
/**
*
* Returns some or all (up to 1,000) access points associated with the Object Lambda Access Point per call. If there
* are more access points than what can be returned in one call, the response will include a continuation token that
* you can use to list the additional access points.
*
*
* The following actions are related to ListAccessPointsForObjectLambda
:
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* @param listAccessPointsForObjectLambdaRequest
* @return Result of the ListAccessPointsForObjectLambda operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.ListAccessPointsForObjectLambda
* @see AWS API Documentation
*/
@Override
public ListAccessPointsForObjectLambdaResponse listAccessPointsForObjectLambda(
ListAccessPointsForObjectLambdaRequest listAccessPointsForObjectLambdaRequest) throws AwsServiceException,
SdkClientException, S3ControlException {
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(ListAccessPointsForObjectLambdaResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
List metricPublishers = resolveMetricPublishers(clientConfiguration,
listAccessPointsForObjectLambdaRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3 Control");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListAccessPointsForObjectLambda");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListAccessPointsForObjectLambda").withCombinedResponseHandler(responseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(listAccessPointsForObjectLambdaRequest)
.withMarshaller(new ListAccessPointsForObjectLambdaRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns some or all (up to 1,000) access points associated with the Object Lambda Access Point per call. If there
* are more access points than what can be returned in one call, the response will include a continuation token that
* you can use to list the additional access points.
*
*
* The following actions are related to ListAccessPointsForObjectLambda
:
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
*
* This is a variant of
* {@link #listAccessPointsForObjectLambda(software.amazon.awssdk.services.s3control.model.ListAccessPointsForObjectLambdaRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.s3control.paginators.ListAccessPointsForObjectLambdaIterable responses = client.listAccessPointsForObjectLambdaPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.s3control.paginators.ListAccessPointsForObjectLambdaIterable responses = client
* .listAccessPointsForObjectLambdaPaginator(request);
* for (software.amazon.awssdk.services.s3control.model.ListAccessPointsForObjectLambdaResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.s3control.paginators.ListAccessPointsForObjectLambdaIterable responses = client.listAccessPointsForObjectLambdaPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listAccessPointsForObjectLambda(software.amazon.awssdk.services.s3control.model.ListAccessPointsForObjectLambdaRequest)}
* operation.
*
*
* @param listAccessPointsForObjectLambdaRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.ListAccessPointsForObjectLambda
* @see AWS API Documentation
*/
@Override
public ListAccessPointsForObjectLambdaIterable listAccessPointsForObjectLambdaPaginator(
ListAccessPointsForObjectLambdaRequest listAccessPointsForObjectLambdaRequest) throws AwsServiceException,
SdkClientException, S3ControlException {
return new ListAccessPointsForObjectLambdaIterable(this, applyPaginatorUserAgent(listAccessPointsForObjectLambdaRequest));
}
/**
*
* Lists current S3 Batch Operations jobs and jobs that have ended within the last 30 days for the Amazon Web
* Services account making the request. For more information, see S3 Batch Operations in the
* Amazon S3 User Guide.
*
*
* Related actions include:
*
*
*
* -
*
* CreateJob
*
*
* -
*
* DescribeJob
*
*
* -
*
*
* -
*
* UpdateJobStatus
*
*
*
*
* @param listJobsRequest
* @return Result of the ListJobs operation returned by the service.
* @throws InvalidRequestException
* @throws InternalServiceException
* @throws InvalidNextTokenException
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.ListJobs
* @see AWS API
* Documentation
*/
@Override
public ListJobsResponse listJobs(ListJobsRequest listJobsRequest) throws InvalidRequestException, InternalServiceException,
InvalidNextTokenException, AwsServiceException, SdkClientException, S3ControlException {
HttpResponseHandler> responseHandler = protocolFactory.createCombinedResponseHandler(
ListJobsResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
List metricPublishers = resolveMetricPublishers(clientConfiguration, listJobsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3 Control");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListJobs");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListJobs").withCombinedResponseHandler(responseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(listJobsRequest)
.withMarshaller(new ListJobsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Lists current S3 Batch Operations jobs and jobs that have ended within the last 30 days for the Amazon Web
* Services account making the request. For more information, see S3 Batch Operations in the
* Amazon S3 User Guide.
*
*
* Related actions include:
*
*
*
* -
*
* CreateJob
*
*
* -
*
* DescribeJob
*
*
* -
*
*
* -
*
* UpdateJobStatus
*
*
*
*
*
* This is a variant of {@link #listJobs(software.amazon.awssdk.services.s3control.model.ListJobsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.s3control.paginators.ListJobsIterable responses = client.listJobsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.s3control.paginators.ListJobsIterable responses = client.listJobsPaginator(request);
* for (software.amazon.awssdk.services.s3control.model.ListJobsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.s3control.paginators.ListJobsIterable responses = client.listJobsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listJobs(software.amazon.awssdk.services.s3control.model.ListJobsRequest)} operation.
*
*
* @param listJobsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidRequestException
* @throws InternalServiceException
* @throws InvalidNextTokenException
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.ListJobs
* @see AWS API
* Documentation
*/
@Override
public ListJobsIterable listJobsPaginator(ListJobsRequest listJobsRequest) throws InvalidRequestException,
InternalServiceException, InvalidNextTokenException, AwsServiceException, SdkClientException, S3ControlException {
return new ListJobsIterable(this, applyPaginatorUserAgent(listJobsRequest));
}
/**
*
* Returns a list of the Multi-Region Access Points currently associated with the specified Amazon Web Services
* account. Each call can return up to 100 Multi-Region Access Points, the maximum number of Multi-Region Access
* Points that can be associated with a single account.
*
*
* This action will always be routed to the US West (Oregon) Region. For more information about the restrictions
* around managing Multi-Region Access Points, see Managing
* Multi-Region Access Points in the Amazon S3 User Guide.
*
*
* The following actions are related to ListMultiRegionAccessPoint
:
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* @param listMultiRegionAccessPointsRequest
* @return Result of the ListMultiRegionAccessPoints operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.ListMultiRegionAccessPoints
* @see AWS API Documentation
*/
@Override
public ListMultiRegionAccessPointsResponse listMultiRegionAccessPoints(
ListMultiRegionAccessPointsRequest listMultiRegionAccessPointsRequest) throws AwsServiceException,
SdkClientException, S3ControlException {
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(ListMultiRegionAccessPointsResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
List metricPublishers = resolveMetricPublishers(clientConfiguration, listMultiRegionAccessPointsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3 Control");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListMultiRegionAccessPoints");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListMultiRegionAccessPoints")
.withCombinedResponseHandler(responseHandler)
.withMetricCollector(apiCallMetricCollector)
.withInput(listMultiRegionAccessPointsRequest)
.putExecutionAttribute(SdkInternalExecutionAttribute.HTTP_CHECKSUM_REQUIRED,
HttpChecksumRequired.create())
.withMarshaller(new ListMultiRegionAccessPointsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns a list of the Multi-Region Access Points currently associated with the specified Amazon Web Services
* account. Each call can return up to 100 Multi-Region Access Points, the maximum number of Multi-Region Access
* Points that can be associated with a single account.
*
*
* This action will always be routed to the US West (Oregon) Region. For more information about the restrictions
* around managing Multi-Region Access Points, see Managing
* Multi-Region Access Points in the Amazon S3 User Guide.
*
*
* The following actions are related to ListMultiRegionAccessPoint
:
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
*
* This is a variant of
* {@link #listMultiRegionAccessPoints(software.amazon.awssdk.services.s3control.model.ListMultiRegionAccessPointsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.s3control.paginators.ListMultiRegionAccessPointsIterable responses = client.listMultiRegionAccessPointsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.s3control.paginators.ListMultiRegionAccessPointsIterable responses = client
* .listMultiRegionAccessPointsPaginator(request);
* for (software.amazon.awssdk.services.s3control.model.ListMultiRegionAccessPointsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.s3control.paginators.ListMultiRegionAccessPointsIterable responses = client.listMultiRegionAccessPointsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listMultiRegionAccessPoints(software.amazon.awssdk.services.s3control.model.ListMultiRegionAccessPointsRequest)}
* operation.
*
*
* @param listMultiRegionAccessPointsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.ListMultiRegionAccessPoints
* @see AWS API Documentation
*/
@Override
public ListMultiRegionAccessPointsIterable listMultiRegionAccessPointsPaginator(
ListMultiRegionAccessPointsRequest listMultiRegionAccessPointsRequest) throws AwsServiceException,
SdkClientException, S3ControlException {
return new ListMultiRegionAccessPointsIterable(this, applyPaginatorUserAgent(listMultiRegionAccessPointsRequest));
}
/**
*
* Returns a list of all Outposts buckets in an Outpost that are owned by the authenticated sender of the request.
* For more information, see Using
* Amazon S3 on Outposts in the Amazon S3 User Guide.
*
*
* For an example of the request syntax for Amazon S3 on Outposts that uses the S3 on Outposts endpoint hostname
* prefix and x-amz-outpost-id
in your request, see the Examples section.
*
*
* @param listRegionalBucketsRequest
* @return Result of the ListRegionalBuckets operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.ListRegionalBuckets
* @see AWS
* API Documentation
*/
@Override
public ListRegionalBucketsResponse listRegionalBuckets(ListRegionalBucketsRequest listRegionalBucketsRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(ListRegionalBucketsResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
List metricPublishers = resolveMetricPublishers(clientConfiguration, listRegionalBucketsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3 Control");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListRegionalBuckets");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListRegionalBuckets").withCombinedResponseHandler(responseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(listRegionalBucketsRequest)
.withMarshaller(new ListRegionalBucketsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns a list of all Outposts buckets in an Outpost that are owned by the authenticated sender of the request.
* For more information, see Using
* Amazon S3 on Outposts in the Amazon S3 User Guide.
*
*
* For an example of the request syntax for Amazon S3 on Outposts that uses the S3 on Outposts endpoint hostname
* prefix and x-amz-outpost-id
in your request, see the Examples section.
*
*
*
* This is a variant of
* {@link #listRegionalBuckets(software.amazon.awssdk.services.s3control.model.ListRegionalBucketsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.s3control.paginators.ListRegionalBucketsIterable responses = client.listRegionalBucketsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.s3control.paginators.ListRegionalBucketsIterable responses = client
* .listRegionalBucketsPaginator(request);
* for (software.amazon.awssdk.services.s3control.model.ListRegionalBucketsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.s3control.paginators.ListRegionalBucketsIterable responses = client.listRegionalBucketsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listRegionalBuckets(software.amazon.awssdk.services.s3control.model.ListRegionalBucketsRequest)}
* operation.
*
*
* @param listRegionalBucketsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.ListRegionalBuckets
* @see AWS
* API Documentation
*/
@Override
public ListRegionalBucketsIterable listRegionalBucketsPaginator(ListRegionalBucketsRequest listRegionalBucketsRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
return new ListRegionalBucketsIterable(this, applyPaginatorUserAgent(listRegionalBucketsRequest));
}
/**
*
* Gets a list of Amazon S3 Storage Lens configurations. For more information about S3 Storage Lens, see Assessing your storage activity and
* usage with Amazon S3 Storage Lens in the Amazon S3 User Guide.
*
*
*
* To use this action, you must have permission to perform the s3:ListStorageLensConfigurations
action.
* For more information, see Setting permissions to
* use Amazon S3 Storage Lens in the Amazon S3 User Guide.
*
*
*
* @param listStorageLensConfigurationsRequest
* @return Result of the ListStorageLensConfigurations operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.ListStorageLensConfigurations
* @see AWS API Documentation
*/
@Override
public ListStorageLensConfigurationsResponse listStorageLensConfigurations(
ListStorageLensConfigurationsRequest listStorageLensConfigurationsRequest) throws AwsServiceException,
SdkClientException, S3ControlException {
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(ListStorageLensConfigurationsResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
List metricPublishers = resolveMetricPublishers(clientConfiguration,
listStorageLensConfigurationsRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3 Control");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListStorageLensConfigurations");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListStorageLensConfigurations").withCombinedResponseHandler(responseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(listStorageLensConfigurationsRequest)
.withMarshaller(new ListStorageLensConfigurationsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Gets a list of Amazon S3 Storage Lens configurations. For more information about S3 Storage Lens, see Assessing your storage activity and
* usage with Amazon S3 Storage Lens in the Amazon S3 User Guide.
*
*
*
* To use this action, you must have permission to perform the s3:ListStorageLensConfigurations
action.
* For more information, see Setting permissions to
* use Amazon S3 Storage Lens in the Amazon S3 User Guide.
*
*
*
* This is a variant of
* {@link #listStorageLensConfigurations(software.amazon.awssdk.services.s3control.model.ListStorageLensConfigurationsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.s3control.paginators.ListStorageLensConfigurationsIterable responses = client.listStorageLensConfigurationsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.s3control.paginators.ListStorageLensConfigurationsIterable responses = client
* .listStorageLensConfigurationsPaginator(request);
* for (software.amazon.awssdk.services.s3control.model.ListStorageLensConfigurationsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.s3control.paginators.ListStorageLensConfigurationsIterable responses = client.listStorageLensConfigurationsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listStorageLensConfigurations(software.amazon.awssdk.services.s3control.model.ListStorageLensConfigurationsRequest)}
* operation.
*
*
* @param listStorageLensConfigurationsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.ListStorageLensConfigurations
* @see AWS API Documentation
*/
@Override
public ListStorageLensConfigurationsIterable listStorageLensConfigurationsPaginator(
ListStorageLensConfigurationsRequest listStorageLensConfigurationsRequest) throws AwsServiceException,
SdkClientException, S3ControlException {
return new ListStorageLensConfigurationsIterable(this, applyPaginatorUserAgent(listStorageLensConfigurationsRequest));
}
/**
*
* Replaces configuration for an Object Lambda Access Point.
*
*
* The following actions are related to PutAccessPointConfigurationForObjectLambda
:
*
*
* -
*
*
*
*
* @param putAccessPointConfigurationForObjectLambdaRequest
* @return Result of the PutAccessPointConfigurationForObjectLambda operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.PutAccessPointConfigurationForObjectLambda
* @see AWS API Documentation
*/
@Override
public PutAccessPointConfigurationForObjectLambdaResponse putAccessPointConfigurationForObjectLambda(
PutAccessPointConfigurationForObjectLambdaRequest putAccessPointConfigurationForObjectLambdaRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(PutAccessPointConfigurationForObjectLambdaResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
List metricPublishers = resolveMetricPublishers(clientConfiguration,
putAccessPointConfigurationForObjectLambdaRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3 Control");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "PutAccessPointConfigurationForObjectLambda");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("PutAccessPointConfigurationForObjectLambda")
.withCombinedResponseHandler(responseHandler).withMetricCollector(apiCallMetricCollector)
.withInput(putAccessPointConfigurationForObjectLambdaRequest)
.withMarshaller(new PutAccessPointConfigurationForObjectLambdaRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Associates an access policy with the specified access point. Each access point can have only one policy, so a
* request made to this API replaces any existing policy associated with the specified access point.
*
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request. In addition, you must use an S3 on Outposts endpoint
* hostname prefix instead of s3-control
. For an example of the request syntax for Amazon S3 on
* Outposts that uses the S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived by
* using the access point ARN, see the Examples section.
*
*
* The following actions are related to PutAccessPointPolicy
:
*
*
* -
*
*
* -
*
*
*
*
* @param putAccessPointPolicyRequest
* @return Result of the PutAccessPointPolicy operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.PutAccessPointPolicy
* @see AWS API Documentation
*/
@Override
public PutAccessPointPolicyResponse putAccessPointPolicy(PutAccessPointPolicyRequest putAccessPointPolicyRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(PutAccessPointPolicyResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
List metricPublishers = resolveMetricPublishers(clientConfiguration, putAccessPointPolicyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3 Control");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "PutAccessPointPolicy");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("PutAccessPointPolicy").withCombinedResponseHandler(responseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(putAccessPointPolicyRequest)
.withMarshaller(new PutAccessPointPolicyRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates or replaces resource policy for an Object Lambda Access Point. For an example policy, see Creating Object
* Lambda Access Points in the Amazon S3 User Guide.
*
*
* The following actions are related to PutAccessPointPolicyForObjectLambda
:
*
*
* -
*
*
* -
*
*
*
*
* @param putAccessPointPolicyForObjectLambdaRequest
* @return Result of the PutAccessPointPolicyForObjectLambda operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.PutAccessPointPolicyForObjectLambda
* @see AWS API Documentation
*/
@Override
public PutAccessPointPolicyForObjectLambdaResponse putAccessPointPolicyForObjectLambda(
PutAccessPointPolicyForObjectLambdaRequest putAccessPointPolicyForObjectLambdaRequest) throws AwsServiceException,
SdkClientException, S3ControlException {
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(PutAccessPointPolicyForObjectLambdaResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
List metricPublishers = resolveMetricPublishers(clientConfiguration,
putAccessPointPolicyForObjectLambdaRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3 Control");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "PutAccessPointPolicyForObjectLambda");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("PutAccessPointPolicyForObjectLambda")
.withCombinedResponseHandler(responseHandler).withMetricCollector(apiCallMetricCollector)
.withInput(putAccessPointPolicyForObjectLambdaRequest)
.withMarshaller(new PutAccessPointPolicyForObjectLambdaRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
*
* This action puts a lifecycle configuration to an Amazon S3 on Outposts bucket. To put a lifecycle configuration
* to an S3 bucket, see PutBucketLifecycleConfiguration in the Amazon S3 API Reference.
*
*
*
* Creates a new lifecycle configuration for the S3 on Outposts bucket or replaces an existing lifecycle
* configuration. Outposts buckets only support lifecycle configurations that delete/expire objects after a certain
* period of time and abort incomplete multipart uploads.
*
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request. In addition, you must use an S3 on Outposts endpoint
* hostname prefix instead of s3-control
. For an example of the request syntax for Amazon S3 on
* Outposts that uses the S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived by
* using the access point ARN, see the Examples section.
*
*
* The following actions are related to PutBucketLifecycleConfiguration
:
*
*
* -
*
*
* -
*
*
*
*
* @param putBucketLifecycleConfigurationRequest
* @return Result of the PutBucketLifecycleConfiguration operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.PutBucketLifecycleConfiguration
* @see AWS API Documentation
*/
@Override
public PutBucketLifecycleConfigurationResponse putBucketLifecycleConfiguration(
PutBucketLifecycleConfigurationRequest putBucketLifecycleConfigurationRequest) throws AwsServiceException,
SdkClientException, S3ControlException {
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(PutBucketLifecycleConfigurationResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
List metricPublishers = resolveMetricPublishers(clientConfiguration,
putBucketLifecycleConfigurationRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3 Control");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "PutBucketLifecycleConfiguration");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("PutBucketLifecycleConfiguration")
.withCombinedResponseHandler(responseHandler)
.withMetricCollector(apiCallMetricCollector)
.withInput(putBucketLifecycleConfigurationRequest)
.putExecutionAttribute(SdkInternalExecutionAttribute.HTTP_CHECKSUM_REQUIRED,
HttpChecksumRequired.create())
.withMarshaller(new PutBucketLifecycleConfigurationRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
*
* This action puts a bucket policy to an Amazon S3 on Outposts bucket. To put a policy on an S3 bucket, see PutBucketPolicy in the
* Amazon S3 API Reference.
*
*
*
* Applies an Amazon S3 bucket policy to an Outposts bucket. For more information, see Using Amazon S3 on Outposts in
* the Amazon S3 User Guide.
*
*
* If you are using an identity other than the root user of the Amazon Web Services account that owns the Outposts
* bucket, the calling identity must have the PutBucketPolicy
permissions on the specified Outposts
* bucket and belong to the bucket owner's account in order to use this action.
*
*
* If you don't have PutBucketPolicy
permissions, Amazon S3 returns a 403 Access Denied
* error. If you have the correct permissions, but you're not using an identity that belongs to the bucket owner's
* account, Amazon S3 returns a 405 Method Not Allowed
error.
*
*
*
* As a security precaution, the root user of the Amazon Web Services account that owns a bucket can always use this
* action, even if the policy explicitly denies the root user the ability to perform this action.
*
*
*
* For more information about bucket policies, see Using Bucket Policies and User
* Policies.
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request. In addition, you must use an S3 on Outposts endpoint
* hostname prefix instead of s3-control
. For an example of the request syntax for Amazon S3 on
* Outposts that uses the S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived by
* using the access point ARN, see the Examples section.
*
*
* The following actions are related to PutBucketPolicy
:
*
*
* -
*
* GetBucketPolicy
*
*
* -
*
*
*
*
* @param putBucketPolicyRequest
* @return Result of the PutBucketPolicy operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.PutBucketPolicy
* @see AWS API
* Documentation
*/
@Override
public PutBucketPolicyResponse putBucketPolicy(PutBucketPolicyRequest putBucketPolicyRequest) throws AwsServiceException,
SdkClientException, S3ControlException {
HttpResponseHandler> responseHandler = protocolFactory.createCombinedResponseHandler(
PutBucketPolicyResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
List metricPublishers = resolveMetricPublishers(clientConfiguration, putBucketPolicyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3 Control");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "PutBucketPolicy");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("PutBucketPolicy").withCombinedResponseHandler(responseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(putBucketPolicyRequest)
.putExecutionAttribute(SdkInternalExecutionAttribute.HTTP_CHECKSUM_REQUIRED, HttpChecksumRequired.create())
.withMarshaller(new PutBucketPolicyRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
*
* This action puts tags on an Amazon S3 on Outposts bucket. To put tags on an S3 bucket, see PutBucketTagging in the
* Amazon S3 API Reference.
*
*
*
* Sets the tags for an S3 on Outposts bucket. For more information, see Using Amazon S3 on Outposts in
* the Amazon S3 User Guide.
*
*
* Use tags to organize your Amazon Web Services bill to reflect your own cost structure. To do this, sign up to get
* your Amazon Web Services account bill with tag key values included. Then, to see the cost of combined resources,
* organize your billing information according to resources with the same tag key values. For example, you can tag
* several resources with a specific application name, and then organize your billing information to see the total
* cost of that application across several services. For more information, see Cost allocation and
* tagging.
*
*
*
* Within a bucket, if you add a tag that has the same key as an existing tag, the new value overwrites the old
* value. For more information, see Using cost allocation in
* Amazon S3 bucket tags.
*
*
*
* To use this action, you must have permissions to perform the s3-outposts:PutBucketTagging
action.
* The Outposts bucket owner has this permission by default and can grant this permission to others. For more
* information about permissions, see Permissions Related to Bucket Subresource Operations and Managing access permissions
* to your Amazon S3 resources.
*
*
* PutBucketTagging
has the following special errors:
*
*
* -
*
* Error code: InvalidTagError
*
*
* -
*
* Description: The tag provided was not a valid tag. This error can occur if the tag did not pass input validation.
* For information about tag restrictions, see
* User-Defined Tag Restrictions and Amazon Web
* Services-Generated Cost Allocation Tag Restrictions.
*
*
*
*
* -
*
* Error code: MalformedXMLError
*
*
* -
*
* Description: The XML provided does not match the schema.
*
*
*
*
* -
*
* Error code: OperationAbortedError
*
*
* -
*
* Description: A conflicting conditional action is currently in progress against this resource. Try again.
*
*
*
*
* -
*
* Error code: InternalError
*
*
* -
*
* Description: The service was unable to apply the provided tag to the bucket.
*
*
*
*
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request. In addition, you must use an S3 on Outposts endpoint
* hostname prefix instead of s3-control
. For an example of the request syntax for Amazon S3 on
* Outposts that uses the S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived by
* using the access point ARN, see the Examples section.
*
*
* The following actions are related to PutBucketTagging
:
*
*
* -
*
* GetBucketTagging
*
*
* -
*
*
*
*
* @param putBucketTaggingRequest
* @return Result of the PutBucketTagging operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.PutBucketTagging
* @see AWS
* API Documentation
*/
@Override
public PutBucketTaggingResponse putBucketTagging(PutBucketTaggingRequest putBucketTaggingRequest) throws AwsServiceException,
SdkClientException, S3ControlException {
HttpResponseHandler> responseHandler = protocolFactory.createCombinedResponseHandler(
PutBucketTaggingResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
List metricPublishers = resolveMetricPublishers(clientConfiguration, putBucketTaggingRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3 Control");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "PutBucketTagging");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("PutBucketTagging").withCombinedResponseHandler(responseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(putBucketTaggingRequest)
.putExecutionAttribute(SdkInternalExecutionAttribute.HTTP_CHECKSUM_REQUIRED, HttpChecksumRequired.create())
.withMarshaller(new PutBucketTaggingRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
*
* This operation sets the versioning state only for S3 on Outposts buckets. To set the versioning state for an S3
* bucket, see PutBucketVersioning in
* the Amazon S3 API Reference.
*
*
*
* Sets the versioning state for an S3 on Outposts bucket. With versioning, you can save multiple distinct copies of
* your data and recover from unintended user actions and application failures.
*
*
* You can set the versioning state to one of the following:
*
*
* -
*
* Enabled - Enables versioning for the objects in the bucket. All objects added to the bucket receive a
* unique version ID.
*
*
* -
*
* Suspended - Suspends versioning for the objects in the bucket. All objects added to the bucket receive the
* version ID null
.
*
*
*
*
* If you've never set versioning on your bucket, it has no versioning state. In that case, a
* GetBucketVersioning request does not return a versioning state value.
*
*
* When you enable S3 Versioning, for each object in your bucket, you have a current version and zero or more
* noncurrent versions. You can configure your bucket S3 Lifecycle rules to expire noncurrent versions after a
* specified time period. For more information, see Creating and
* managing a lifecycle configuration for your S3 on Outposts bucket in the Amazon S3 User Guide.
*
*
* If you have an object expiration lifecycle policy in your non-versioned bucket and you want to maintain the same
* permanent delete behavior when you enable versioning, you must add a noncurrent expiration policy. The noncurrent
* expiration lifecycle policy will manage the deletes of the noncurrent object versions in the version-enabled
* bucket. For more information, see Versioning in the Amazon S3
* User Guide.
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request. In addition, you must use an S3 on Outposts endpoint
* hostname prefix instead of s3-control
. For an example of the request syntax for Amazon S3 on
* Outposts that uses the S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived by
* using the access point ARN, see the Examples section.
*
*
* The following operations are related to PutBucketVersioning
for S3 on Outposts.
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* @param putBucketVersioningRequest
* @return Result of the PutBucketVersioning operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.PutBucketVersioning
* @see AWS
* API Documentation
*/
@Override
public PutBucketVersioningResponse putBucketVersioning(PutBucketVersioningRequest putBucketVersioningRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(PutBucketVersioningResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
List metricPublishers = resolveMetricPublishers(clientConfiguration, putBucketVersioningRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3 Control");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "PutBucketVersioning");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("PutBucketVersioning").withCombinedResponseHandler(responseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(putBucketVersioningRequest)
.putExecutionAttribute(SdkInternalExecutionAttribute.HTTP_CHECKSUM_REQUIRED, HttpChecksumRequired.create())
.withMarshaller(new PutBucketVersioningRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Sets the supplied tag-set on an S3 Batch Operations job.
*
*
* A tag is a key-value pair. You can associate S3 Batch Operations tags with any job by sending a PUT request
* against the tagging subresource that is associated with the job. To modify the existing tag set, you can either
* replace the existing tag set entirely, or make changes within the existing tag set by retrieving the existing tag
* set using GetJobTagging, modify
* that tag set, and use this action to replace the tag set with the one you modified. For more information, see Controlling
* access and labeling jobs using tags in the Amazon S3 User Guide.
*
*
*
*
* -
*
* If you send this request with an empty tag set, Amazon S3 deletes the existing tag set on the Batch Operations
* job. If you use this method, you are charged for a Tier 1 Request (PUT). For more information, see Amazon S3 pricing.
*
*
* -
*
* For deleting existing tags for your Batch Operations job, a DeleteJobTagging
* request is preferred because it achieves the same result without incurring charges.
*
*
* -
*
* A few things to consider about using tags:
*
*
* -
*
* Amazon S3 limits the maximum number of tags to 50 tags per job.
*
*
* -
*
* You can associate up to 50 tags with a job as long as they have unique tag keys.
*
*
* -
*
* A tag key can be up to 128 Unicode characters in length, and tag values can be up to 256 Unicode characters in
* length.
*
*
* -
*
* The key and values are case sensitive.
*
*
* -
*
* For tagging-related restrictions related to characters and encodings, see User-Defined
* Tag Restrictions in the Billing and Cost Management User Guide.
*
*
*
*
*
*
*
*
* To use this action, you must have permission to perform the s3:PutJobTagging
action.
*
*
* Related actions include:
*
*
* -
*
* CreateJob
*
*
* -
*
* GetJobTagging
*
*
* -
*
* DeleteJobTagging
*
*
*
*
* @param putJobTaggingRequest
* @return Result of the PutJobTagging operation returned by the service.
* @throws InternalServiceException
* @throws TooManyRequestsException
* @throws NotFoundException
* @throws TooManyTagsException
* Amazon S3 throws this exception if you have too many tags in your tag set.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.PutJobTagging
* @see AWS API
* Documentation
*/
@Override
public PutJobTaggingResponse putJobTagging(PutJobTaggingRequest putJobTaggingRequest) throws InternalServiceException,
TooManyRequestsException, NotFoundException, TooManyTagsException, AwsServiceException, SdkClientException,
S3ControlException {
HttpResponseHandler> responseHandler = protocolFactory.createCombinedResponseHandler(
PutJobTaggingResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
List metricPublishers = resolveMetricPublishers(clientConfiguration, putJobTaggingRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3 Control");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "PutJobTagging");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("PutJobTagging").withCombinedResponseHandler(responseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(putJobTaggingRequest)
.withMarshaller(new PutJobTaggingRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Associates an access control policy with the specified Multi-Region Access Point. Each Multi-Region Access Point
* can have only one policy, so a request made to this action replaces any existing policy that is associated with
* the specified Multi-Region Access Point.
*
*
* This action will always be routed to the US West (Oregon) Region. For more information about the restrictions
* around managing Multi-Region Access Points, see Managing
* Multi-Region Access Points in the Amazon S3 User Guide.
*
*
* The following actions are related to PutMultiRegionAccessPointPolicy
:
*
*
* -
*
*
* -
*
*
*
*
* @param putMultiRegionAccessPointPolicyRequest
* @return Result of the PutMultiRegionAccessPointPolicy operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.PutMultiRegionAccessPointPolicy
* @see AWS API Documentation
*/
@Override
public PutMultiRegionAccessPointPolicyResponse putMultiRegionAccessPointPolicy(
PutMultiRegionAccessPointPolicyRequest putMultiRegionAccessPointPolicyRequest) throws AwsServiceException,
SdkClientException, S3ControlException {
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(PutMultiRegionAccessPointPolicyResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
List metricPublishers = resolveMetricPublishers(clientConfiguration,
putMultiRegionAccessPointPolicyRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3 Control");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "PutMultiRegionAccessPointPolicy");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("PutMultiRegionAccessPointPolicy")
.withCombinedResponseHandler(responseHandler)
.withMetricCollector(apiCallMetricCollector)
.withInput(putMultiRegionAccessPointPolicyRequest)
.putExecutionAttribute(SdkInternalExecutionAttribute.HTTP_CHECKSUM_REQUIRED,
HttpChecksumRequired.create())
.withMarshaller(new PutMultiRegionAccessPointPolicyRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates or modifies the PublicAccessBlock
configuration for an Amazon Web Services account. For this
* operation, users must have the s3:PutAccountPublicAccessBlock
permission. For more information, see
* Using Amazon
* S3 block public access.
*
*
* Related actions include:
*
*
* -
*
*
* -
*
*
*
*
* @param putPublicAccessBlockRequest
* @return Result of the PutPublicAccessBlock operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.PutPublicAccessBlock
* @see AWS API Documentation
*/
@Override
public PutPublicAccessBlockResponse putPublicAccessBlock(PutPublicAccessBlockRequest putPublicAccessBlockRequest)
throws AwsServiceException, SdkClientException, S3ControlException {
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(PutPublicAccessBlockResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
List metricPublishers = resolveMetricPublishers(clientConfiguration, putPublicAccessBlockRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3 Control");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "PutPublicAccessBlock");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("PutPublicAccessBlock").withCombinedResponseHandler(responseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(putPublicAccessBlockRequest)
.withMarshaller(new PutPublicAccessBlockRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Puts an Amazon S3 Storage Lens configuration. For more information about S3 Storage Lens, see Working with Amazon S3 Storage Lens
* in the Amazon S3 User Guide. For a complete list of S3 Storage Lens metrics, see S3 Storage Lens
* metrics glossary in the Amazon S3 User Guide.
*
*
*
* To use this action, you must have permission to perform the s3:PutStorageLensConfiguration
action.
* For more information, see Setting permissions to
* use Amazon S3 Storage Lens in the Amazon S3 User Guide.
*
*
*
* @param putStorageLensConfigurationRequest
* @return Result of the PutStorageLensConfiguration operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.PutStorageLensConfiguration
* @see AWS API Documentation
*/
@Override
public PutStorageLensConfigurationResponse putStorageLensConfiguration(
PutStorageLensConfigurationRequest putStorageLensConfigurationRequest) throws AwsServiceException,
SdkClientException, S3ControlException {
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(PutStorageLensConfigurationResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
List metricPublishers = resolveMetricPublishers(clientConfiguration, putStorageLensConfigurationRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3 Control");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "PutStorageLensConfiguration");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("PutStorageLensConfiguration").withCombinedResponseHandler(responseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(putStorageLensConfigurationRequest)
.withMarshaller(new PutStorageLensConfigurationRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Put or replace tags on an existing Amazon S3 Storage Lens configuration. For more information about S3 Storage
* Lens, see Assessing your storage
* activity and usage with Amazon S3 Storage Lens in the Amazon S3 User Guide.
*
*
*
* To use this action, you must have permission to perform the s3:PutStorageLensConfigurationTagging
* action. For more information, see Setting permissions to
* use Amazon S3 Storage Lens in the Amazon S3 User Guide.
*
*
*
* @param putStorageLensConfigurationTaggingRequest
* @return Result of the PutStorageLensConfigurationTagging operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.PutStorageLensConfigurationTagging
* @see AWS API Documentation
*/
@Override
public PutStorageLensConfigurationTaggingResponse putStorageLensConfigurationTagging(
PutStorageLensConfigurationTaggingRequest putStorageLensConfigurationTaggingRequest) throws AwsServiceException,
SdkClientException, S3ControlException {
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(PutStorageLensConfigurationTaggingResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
List metricPublishers = resolveMetricPublishers(clientConfiguration,
putStorageLensConfigurationTaggingRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3 Control");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "PutStorageLensConfigurationTagging");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("PutStorageLensConfigurationTagging").withCombinedResponseHandler(responseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(putStorageLensConfigurationTaggingRequest)
.withMarshaller(new PutStorageLensConfigurationTaggingRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Submits an updated route configuration for a Multi-Region Access Point. This API operation updates the routing
* status for the specified Regions from active to passive, or from passive to active. A value of 0
* indicates a passive status, which means that traffic won't be routed to the specified Region. A value of
* 100
indicates an active status, which means that traffic will be routed to the specified Region. At
* least one Region must be active at all times.
*
*
* When the routing configuration is changed, any in-progress operations (uploads, copies, deletes, and so on) to
* formerly active Regions will continue to run to their final completion state (success or failure). The routing
* configurations of any Regions that aren’t specified remain unchanged.
*
*
*
* Updated routing configurations might not be immediately applied. It can take up to 2 minutes for your changes to
* take effect.
*
*
*
* To submit routing control changes and failover requests, use the Amazon S3 failover control infrastructure
* endpoints in these five Amazon Web Services Regions:
*
*
* -
*
* us-east-1
*
*
* -
*
* us-west-2
*
*
* -
*
* ap-southeast-2
*
*
* -
*
* ap-northeast-1
*
*
* -
*
* eu-west-1
*
*
*
*
*
* Your Amazon S3 bucket does not need to be in these five Regions.
*
*
*
* @param submitMultiRegionAccessPointRoutesRequest
* @return Result of the SubmitMultiRegionAccessPointRoutes operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.SubmitMultiRegionAccessPointRoutes
* @see AWS API Documentation
*/
@Override
public SubmitMultiRegionAccessPointRoutesResponse submitMultiRegionAccessPointRoutes(
SubmitMultiRegionAccessPointRoutesRequest submitMultiRegionAccessPointRoutesRequest) throws AwsServiceException,
SdkClientException, S3ControlException {
HttpResponseHandler> responseHandler = protocolFactory
.createCombinedResponseHandler(SubmitMultiRegionAccessPointRoutesResponse::builder,
new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
List metricPublishers = resolveMetricPublishers(clientConfiguration,
submitMultiRegionAccessPointRoutesRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3 Control");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "SubmitMultiRegionAccessPointRoutes");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("SubmitMultiRegionAccessPointRoutes")
.withCombinedResponseHandler(responseHandler)
.withMetricCollector(apiCallMetricCollector)
.withInput(submitMultiRegionAccessPointRoutesRequest)
.putExecutionAttribute(SdkInternalExecutionAttribute.HTTP_CHECKSUM_REQUIRED,
HttpChecksumRequired.create())
.withMarshaller(new SubmitMultiRegionAccessPointRoutesRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Updates an existing S3 Batch Operations job's priority. For more information, see S3 Batch Operations in the
* Amazon S3 User Guide.
*
*
*
* Related actions include:
*
*
* -
*
* CreateJob
*
*
* -
*
* ListJobs
*
*
* -
*
* DescribeJob
*
*
* -
*
* UpdateJobStatus
*
*
*
*
* @param updateJobPriorityRequest
* @return Result of the UpdateJobPriority operation returned by the service.
* @throws BadRequestException
* @throws TooManyRequestsException
* @throws NotFoundException
* @throws InternalServiceException
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.UpdateJobPriority
* @see AWS
* API Documentation
*/
@Override
public UpdateJobPriorityResponse updateJobPriority(UpdateJobPriorityRequest updateJobPriorityRequest)
throws BadRequestException, TooManyRequestsException, NotFoundException, InternalServiceException,
AwsServiceException, SdkClientException, S3ControlException {
HttpResponseHandler> responseHandler = protocolFactory.createCombinedResponseHandler(
UpdateJobPriorityResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
List metricPublishers = resolveMetricPublishers(clientConfiguration, updateJobPriorityRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3 Control");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UpdateJobPriority");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("UpdateJobPriority").withCombinedResponseHandler(responseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(updateJobPriorityRequest)
.withMarshaller(new UpdateJobPriorityRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Updates the status for the specified job. Use this action to confirm that you want to run a job or to cancel an
* existing job. For more information, see S3 Batch Operations in the
* Amazon S3 User Guide.
*
*
*
* Related actions include:
*
*
* -
*
* CreateJob
*
*
* -
*
* ListJobs
*
*
* -
*
* DescribeJob
*
*
* -
*
* UpdateJobStatus
*
*
*
*
* @param updateJobStatusRequest
* @return Result of the UpdateJobStatus operation returned by the service.
* @throws BadRequestException
* @throws TooManyRequestsException
* @throws NotFoundException
* @throws JobStatusException
* @throws InternalServiceException
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws S3ControlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample S3ControlClient.UpdateJobStatus
* @see AWS API
* Documentation
*/
@Override
public UpdateJobStatusResponse updateJobStatus(UpdateJobStatusRequest updateJobStatusRequest) throws BadRequestException,
TooManyRequestsException, NotFoundException, JobStatusException, InternalServiceException, AwsServiceException,
SdkClientException, S3ControlException {
HttpResponseHandler> responseHandler = protocolFactory.createCombinedResponseHandler(
UpdateJobStatusResponse::builder, new XmlOperationMetadata().withHasStreamingSuccessResponse(false));
List metricPublishers = resolveMetricPublishers(clientConfiguration, updateJobStatusRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "S3 Control");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UpdateJobStatus");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("UpdateJobStatus").withCombinedResponseHandler(responseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(updateJobStatusRequest)
.withMarshaller(new UpdateJobStatusRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
private T applyPaginatorUserAgent(T request) {
Consumer userAgentApplier = b -> b.addApiName(ApiName.builder()
.version(VersionInfo.SDK_VERSION).name("PAGINATED").build());
AwsRequestOverrideConfiguration overrideConfiguration = request.overrideConfiguration()
.map(c -> c.toBuilder().applyMutation(userAgentApplier).build())
.orElse((AwsRequestOverrideConfiguration.builder().applyMutation(userAgentApplier).build()));
return (T) request.toBuilder().overrideConfiguration(overrideConfiguration).build();
}
@Override
public final String serviceName() {
return SERVICE_NAME;
}
private static List resolveMetricPublishers(SdkClientConfiguration clientConfiguration,
RequestOverrideConfiguration requestOverrideConfiguration) {
List publishers = null;
if (requestOverrideConfiguration != null) {
publishers = requestOverrideConfiguration.metricPublishers();
}
if (publishers == null || publishers.isEmpty()) {
publishers = clientConfiguration.option(SdkClientOption.METRIC_PUBLISHERS);
}
if (publishers == null) {
publishers = Collections.emptyList();
}
return publishers;
}
private AwsXmlProtocolFactory init() {
return AwsXmlProtocolFactory
.builder()
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidRequestException")
.exceptionBuilderSupplier(InvalidRequestException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidNextTokenException")
.exceptionBuilderSupplier(InvalidNextTokenException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("JobStatusException")
.exceptionBuilderSupplier(JobStatusException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("NoSuchPublicAccessBlockConfiguration")
.exceptionBuilderSupplier(NoSuchPublicAccessBlockConfigurationException::builder)
.httpStatusCode(404).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InternalServiceException")
.exceptionBuilderSupplier(InternalServiceException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("BucketAlreadyExists")
.exceptionBuilderSupplier(BucketAlreadyExistsException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("NotFoundException")
.exceptionBuilderSupplier(NotFoundException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("TooManyTagsException")
.exceptionBuilderSupplier(TooManyTagsException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("IdempotencyException")
.exceptionBuilderSupplier(IdempotencyException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("BucketAlreadyOwnedByYou")
.exceptionBuilderSupplier(BucketAlreadyOwnedByYouException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("TooManyRequestsException")
.exceptionBuilderSupplier(TooManyRequestsException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("BadRequestException")
.exceptionBuilderSupplier(BadRequestException::builder).build())
.clientConfiguration(clientConfiguration).defaultServiceExceptionSupplier(S3ControlException::builder).build();
}
@Override
public void close() {
clientHandler.close();
}
}