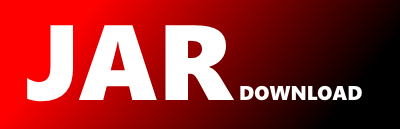
software.amazon.awssdk.services.s3control.model.CreateJobRequest Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.s3control.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.DefaultValueTrait;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class CreateJobRequest extends S3ControlRequest implements
ToCopyableBuilder {
private static final SdkField ACCOUNT_ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("AccountId")
.getter(getter(CreateJobRequest::accountId))
.setter(setter(Builder::accountId))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("x-amz-account-id")
.unmarshallLocationName("x-amz-account-id").build()).build();
private static final SdkField CONFIRMATION_REQUIRED_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("ConfirmationRequired")
.getter(getter(CreateJobRequest::confirmationRequired))
.setter(setter(Builder::confirmationRequired))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ConfirmationRequired")
.unmarshallLocationName("ConfirmationRequired").build()).build();
private static final SdkField OPERATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("Operation")
.getter(getter(CreateJobRequest::operation))
.setter(setter(Builder::operation))
.constructor(JobOperation::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Operation")
.unmarshallLocationName("Operation").build()).build();
private static final SdkField REPORT_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("Report")
.getter(getter(CreateJobRequest::report))
.setter(setter(Builder::report))
.constructor(JobReport::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Report")
.unmarshallLocationName("Report").build()).build();
private static final SdkField CLIENT_REQUEST_TOKEN_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("ClientRequestToken")
.getter(getter(CreateJobRequest::clientRequestToken))
.setter(setter(Builder::clientRequestToken))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ClientRequestToken")
.unmarshallLocationName("ClientRequestToken").build(), DefaultValueTrait.idempotencyToken()).build();
private static final SdkField MANIFEST_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("Manifest")
.getter(getter(CreateJobRequest::manifest))
.setter(setter(Builder::manifest))
.constructor(JobManifest::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Manifest")
.unmarshallLocationName("Manifest").build()).build();
private static final SdkField DESCRIPTION_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("Description")
.getter(getter(CreateJobRequest::description))
.setter(setter(Builder::description))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Description")
.unmarshallLocationName("Description").build()).build();
private static final SdkField PRIORITY_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("Priority")
.getter(getter(CreateJobRequest::priority))
.setter(setter(Builder::priority))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Priority")
.unmarshallLocationName("Priority").build()).build();
private static final SdkField ROLE_ARN_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("RoleArn")
.getter(getter(CreateJobRequest::roleArn))
.setter(setter(Builder::roleArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RoleArn")
.unmarshallLocationName("RoleArn").build()).build();
private static final SdkField> TAGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Tags")
.getter(getter(CreateJobRequest::tags))
.setter(setter(Builder::tags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Tags")
.unmarshallLocationName("Tags").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(S3Tag::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").unmarshallLocationName("member").build()).build())
.build()).build();
private static final SdkField MANIFEST_GENERATOR_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("ManifestGenerator")
.getter(getter(CreateJobRequest::manifestGenerator))
.setter(setter(Builder::manifestGenerator))
.constructor(JobManifestGenerator::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ManifestGenerator")
.unmarshallLocationName("ManifestGenerator").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ACCOUNT_ID_FIELD,
CONFIRMATION_REQUIRED_FIELD, OPERATION_FIELD, REPORT_FIELD, CLIENT_REQUEST_TOKEN_FIELD, MANIFEST_FIELD,
DESCRIPTION_FIELD, PRIORITY_FIELD, ROLE_ARN_FIELD, TAGS_FIELD, MANIFEST_GENERATOR_FIELD));
private final String accountId;
private final Boolean confirmationRequired;
private final JobOperation operation;
private final JobReport report;
private final String clientRequestToken;
private final JobManifest manifest;
private final String description;
private final Integer priority;
private final String roleArn;
private final List tags;
private final JobManifestGenerator manifestGenerator;
private CreateJobRequest(BuilderImpl builder) {
super(builder);
this.accountId = builder.accountId;
this.confirmationRequired = builder.confirmationRequired;
this.operation = builder.operation;
this.report = builder.report;
this.clientRequestToken = builder.clientRequestToken;
this.manifest = builder.manifest;
this.description = builder.description;
this.priority = builder.priority;
this.roleArn = builder.roleArn;
this.tags = builder.tags;
this.manifestGenerator = builder.manifestGenerator;
}
/**
*
* The Amazon Web Services account ID that creates the job.
*
*
* @return The Amazon Web Services account ID that creates the job.
*/
public final String accountId() {
return accountId;
}
/**
*
* Indicates whether confirmation is required before Amazon S3 runs the job. Confirmation is only required for jobs
* created through the Amazon S3 console.
*
*
* @return Indicates whether confirmation is required before Amazon S3 runs the job. Confirmation is only required
* for jobs created through the Amazon S3 console.
*/
public final Boolean confirmationRequired() {
return confirmationRequired;
}
/**
*
* The action that you want this job to perform on every object listed in the manifest. For more information about
* the available actions, see Operations in the Amazon S3
* User Guide.
*
*
* @return The action that you want this job to perform on every object listed in the manifest. For more information
* about the available actions, see Operations in the
* Amazon S3 User Guide.
*/
public final JobOperation operation() {
return operation;
}
/**
*
* Configuration parameters for the optional job-completion report.
*
*
* @return Configuration parameters for the optional job-completion report.
*/
public final JobReport report() {
return report;
}
/**
*
* An idempotency token to ensure that you don't accidentally submit the same request twice. You can use any string
* up to the maximum length.
*
*
* @return An idempotency token to ensure that you don't accidentally submit the same request twice. You can use any
* string up to the maximum length.
*/
public final String clientRequestToken() {
return clientRequestToken;
}
/**
*
* Configuration parameters for the manifest.
*
*
* @return Configuration parameters for the manifest.
*/
public final JobManifest manifest() {
return manifest;
}
/**
*
* A description for this job. You can use any string within the permitted length. Descriptions don't need to be
* unique and can be used for multiple jobs.
*
*
* @return A description for this job. You can use any string within the permitted length. Descriptions don't need
* to be unique and can be used for multiple jobs.
*/
public final String description() {
return description;
}
/**
*
* The numerical priority for this job. Higher numbers indicate higher priority.
*
*
* @return The numerical priority for this job. Higher numbers indicate higher priority.
*/
public final Integer priority() {
return priority;
}
/**
*
* The Amazon Resource Name (ARN) for the Identity and Access Management (IAM) role that Batch Operations will use
* to run this job's action on every object in the manifest.
*
*
* @return The Amazon Resource Name (ARN) for the Identity and Access Management (IAM) role that Batch Operations
* will use to run this job's action on every object in the manifest.
*/
public final String roleArn() {
return roleArn;
}
/**
* For responses, this returns true if the service returned a value for the Tags property. This DOES NOT check that
* the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is useful
* because the SDK will never return a null collection or map, but you may need to differentiate between the service
* returning nothing (or null) and the service returning an empty collection or map. For requests, this returns true
* if a value for the property was specified in the request builder, and false if a value was not specified.
*/
public final boolean hasTags() {
return tags != null && !(tags instanceof SdkAutoConstructList);
}
/**
*
* A set of tags to associate with the S3 Batch Operations job. This is an optional parameter.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasTags} method.
*
*
* @return A set of tags to associate with the S3 Batch Operations job. This is an optional parameter.
*/
public final List tags() {
return tags;
}
/**
*
* The attribute container for the ManifestGenerator details. Jobs must be created with either a manifest file or a
* ManifestGenerator, but not both.
*
*
* @return The attribute container for the ManifestGenerator details. Jobs must be created with either a manifest
* file or a ManifestGenerator, but not both.
*/
public final JobManifestGenerator manifestGenerator() {
return manifestGenerator;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(accountId());
hashCode = 31 * hashCode + Objects.hashCode(confirmationRequired());
hashCode = 31 * hashCode + Objects.hashCode(operation());
hashCode = 31 * hashCode + Objects.hashCode(report());
hashCode = 31 * hashCode + Objects.hashCode(clientRequestToken());
hashCode = 31 * hashCode + Objects.hashCode(manifest());
hashCode = 31 * hashCode + Objects.hashCode(description());
hashCode = 31 * hashCode + Objects.hashCode(priority());
hashCode = 31 * hashCode + Objects.hashCode(roleArn());
hashCode = 31 * hashCode + Objects.hashCode(hasTags() ? tags() : null);
hashCode = 31 * hashCode + Objects.hashCode(manifestGenerator());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CreateJobRequest)) {
return false;
}
CreateJobRequest other = (CreateJobRequest) obj;
return Objects.equals(accountId(), other.accountId())
&& Objects.equals(confirmationRequired(), other.confirmationRequired())
&& Objects.equals(operation(), other.operation()) && Objects.equals(report(), other.report())
&& Objects.equals(clientRequestToken(), other.clientRequestToken())
&& Objects.equals(manifest(), other.manifest()) && Objects.equals(description(), other.description())
&& Objects.equals(priority(), other.priority()) && Objects.equals(roleArn(), other.roleArn())
&& hasTags() == other.hasTags() && Objects.equals(tags(), other.tags())
&& Objects.equals(manifestGenerator(), other.manifestGenerator());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("CreateJobRequest").add("AccountId", accountId())
.add("ConfirmationRequired", confirmationRequired()).add("Operation", operation()).add("Report", report())
.add("ClientRequestToken", clientRequestToken()).add("Manifest", manifest()).add("Description", description())
.add("Priority", priority()).add("RoleArn", roleArn()).add("Tags", hasTags() ? tags() : null)
.add("ManifestGenerator", manifestGenerator()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "AccountId":
return Optional.ofNullable(clazz.cast(accountId()));
case "ConfirmationRequired":
return Optional.ofNullable(clazz.cast(confirmationRequired()));
case "Operation":
return Optional.ofNullable(clazz.cast(operation()));
case "Report":
return Optional.ofNullable(clazz.cast(report()));
case "ClientRequestToken":
return Optional.ofNullable(clazz.cast(clientRequestToken()));
case "Manifest":
return Optional.ofNullable(clazz.cast(manifest()));
case "Description":
return Optional.ofNullable(clazz.cast(description()));
case "Priority":
return Optional.ofNullable(clazz.cast(priority()));
case "RoleArn":
return Optional.ofNullable(clazz.cast(roleArn()));
case "Tags":
return Optional.ofNullable(clazz.cast(tags()));
case "ManifestGenerator":
return Optional.ofNullable(clazz.cast(manifestGenerator()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function