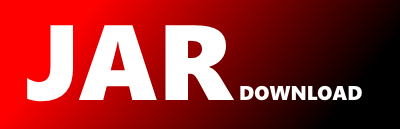
software.amazon.awssdk.services.s3control.model.S3GeneratedManifestDescriptor Maven / Gradle / Ivy
Show all versions of s3control Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.s3control.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Describes the specified job's generated manifest. Batch Operations jobs created with a ManifestGenerator populate
* details of this descriptor after execution of the ManifestGenerator.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class S3GeneratedManifestDescriptor implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField FORMAT_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("Format")
.getter(getter(S3GeneratedManifestDescriptor::formatAsString))
.setter(setter(Builder::format))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Format")
.unmarshallLocationName("Format").build()).build();
private static final SdkField LOCATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("Location")
.getter(getter(S3GeneratedManifestDescriptor::location))
.setter(setter(Builder::location))
.constructor(JobManifestLocation::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Location")
.unmarshallLocationName("Location").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(FORMAT_FIELD, LOCATION_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private static final long serialVersionUID = 1L;
private final String format;
private final JobManifestLocation location;
private S3GeneratedManifestDescriptor(BuilderImpl builder) {
this.format = builder.format;
this.location = builder.location;
}
/**
*
* The format of the generated manifest.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #format} will
* return {@link GeneratedManifestFormat#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #formatAsString}.
*
*
* @return The format of the generated manifest.
* @see GeneratedManifestFormat
*/
public final GeneratedManifestFormat format() {
return GeneratedManifestFormat.fromValue(format);
}
/**
*
* The format of the generated manifest.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #format} will
* return {@link GeneratedManifestFormat#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #formatAsString}.
*
*
* @return The format of the generated manifest.
* @see GeneratedManifestFormat
*/
public final String formatAsString() {
return format;
}
/**
* Returns the value of the Location property for this object.
*
* @return The value of the Location property for this object.
*/
public final JobManifestLocation location() {
return location;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(formatAsString());
hashCode = 31 * hashCode + Objects.hashCode(location());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof S3GeneratedManifestDescriptor)) {
return false;
}
S3GeneratedManifestDescriptor other = (S3GeneratedManifestDescriptor) obj;
return Objects.equals(formatAsString(), other.formatAsString()) && Objects.equals(location(), other.location());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("S3GeneratedManifestDescriptor").add("Format", formatAsString()).add("Location", location())
.build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Format":
return Optional.ofNullable(clazz.cast(formatAsString()));
case "Location":
return Optional.ofNullable(clazz.cast(location()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("Format", FORMAT_FIELD);
map.put("Location", LOCATION_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function